
[SIMPLE PROGRAM] HYT humidity & temp sensor polling / showing received data at TFT with capacitive touchscreen
Dependencies: FT800_2 HYT mbed
display.Draw_MainMenu.cpp
00001 #include "display.h" 00002 00003 /************************************************************************************************************************** 00004 ************************** Display Main Menu ****************************************************************************** 00005 **************************************************************************************************************************/ 00006 void Display::MainMenu(float humidity, float temperature) 00007 { 00008 // start FT800 display list 00009 StartDL(); 00010 00011 // write main title 00012 (*_TFT).DL(COLOR_RGB(0, 0, 0)); 00013 (*_TFT).Text(11, 15, 30, 0, "HYT-271 sensor from IST"); 00014 00015 // create blue rectangle with current humididty including 00016 // rectangle is tagged as CURR_HUM_PRESS 00017 (*_TFT).DL(TAG_MASK(1)); 00018 (*_TFT).DL(TAG(CURR_HUM_PRESS)); 00019 (*_TFT).DL(COLOR_RGB(9, 0, 63)); 00020 // if rectangle is already pressed, draw it with lighter color 00021 if (pressedButton == CURR_HUM_PRESS) { 00022 (*_TFT).DL(COLOR_RGB(75, 70, 108)); 00023 } 00024 (*_TFT).DL(BEGIN(RECTS)); 00025 (*_TFT).DL(VERTEX2II(12, 62, 0, 0)); 00026 (*_TFT).DL(VERTEX2II(12 + 400, 62 + 93, 0, 0)); 00027 (*_TFT).DL(COLOR_RGB(255, 255, 255)); 00028 (*_TFT).Text(12 + 10, 62 + 5, 30, 0, "Current humidity (rH)"); 00029 CreateStringTempHum(humidityStr, humidity, 0); 00030 (*_TFT).Text(12 + 10, 62 + 45, 31, 0, humidityStr); 00031 (*_TFT).DL(TAG_MASK(0)); 00032 00033 // create blue rectangle with current temperature including 00034 // rectangle is tagged as CURR_TEMP_PRESS 00035 (*_TFT).DL(TAG_MASK(1)); 00036 (*_TFT).DL(TAG(CURR_TEMP_PRESS)); 00037 (*_TFT).DL(COLOR_RGB(9, 0, 63)); 00038 // if rectangle is already pressed, draw it with lighter color 00039 if (pressedButton == CURR_TEMP_PRESS) { 00040 (*_TFT).DL(COLOR_RGB(75, 70, 108)); 00041 } 00042 (*_TFT).DL(BEGIN(RECTS)); 00043 (*_TFT).DL(VERTEX2II(12, 62 + 93 + 12, 0, 0)); 00044 (*_TFT).DL(VERTEX2II(12 + 400, 62 + 93 + 12 + 93, 0, 0)); 00045 (*_TFT).DL(COLOR_RGB(255, 255, 255)); 00046 (*_TFT).Text(12 + 10, 62 + 93 + 12 + 5, 30, 0, "Current temperature"); 00047 CreateStringTempHum(temperatureStr, temperature, 1); 00048 (*_TFT).Text(12 + 10, 62 + 93 + 12 + 45, 31, 0, temperatureStr); 00049 (*_TFT).DL(TAG_MASK(0)); 00050 00051 // finish FT800 display list 00052 FinishDL(); 00053 }
Generated on Fri Jul 22 2022 13:11:57 by
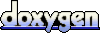