
Русифицированная версия программы для измерения температуры и отн. влажности и вывода информации на сенсорный TFT
Dependencies: FT800_2 HYT mbed
display.Draw_24hrsStatistics.cpp
00001 #include "display.h" 00002 00003 /************************************************************************************************************************** 00004 ************************** Display data about humidity in the 24-hour interval ******************************************** 00005 **************************************************************************************************************************/ 00006 00007 void Display::StatHumidity_24hrs(volatile uint64_t seconds, short int statistics24hrs[3][288], uint64_t gridSecondsOffset) 00008 { 00009 // start FT800 display list 00010 StartDL(); 00011 00012 // mark 8 areas for touch input (for zoom in), 00013 // make each every second zone gray, make the active zone green 00014 char ZonesCnt = 0; 00015 for (int i = 25; i < 433; i += 17 * 3) { 00016 (*_TFT).DL(COLOR_RGB(255, 255, 255)); 00017 if (ZonesCnt % 2 == 0) { 00018 (*_TFT).DL(COLOR_RGB(233, 233, 233)); 00019 } 00020 if (pressedButton - ZONE_1_PRESS == ZonesCnt) { 00021 (*_TFT).DL(COLOR_RGB(200, 255, 200)); 00022 timePoint3hrs = 36 * ZonesCnt; 00023 } 00024 (*_TFT).DL(TAG_MASK(1)); 00025 (*_TFT).DL(TAG(ZONE_1_PRESS + ZonesCnt)); 00026 (*_TFT).DL(BEGIN(RECTS)); 00027 (*_TFT).DL(VERTEX2II(i, 100, 0, 0)); 00028 (*_TFT).DL(VERTEX2II(i + 17 * 3, 200, 0, 0)); 00029 (*_TFT).DL(TAG_MASK(0)); 00030 ZonesCnt++; 00031 } 00032 00033 // create time string, GridLines, link to main menu 00034 TimeSinceTurnOn(seconds); 00035 VerticalGrid24hrs(gridSecondsOffset); 00036 HorisontalGrid_Statistics_Humidity(); 00037 MainMenuReference(); 00038 00039 // draw graph line 00040 (*_TFT).DL(COLOR_RGB(0, 0, 0)); 00041 for (int i = 1; i < 287; i++) { 00042 short int currentValue = statistics24hrs[0][i]; 00043 if (currentValue != INIT_STATISTICS_NUMBER) { 00044 char previousValue = statistics24hrs[0][i - 1]; 00045 char delta = statistics24hrs[2][i] - statistics24hrs[1][i]; 00046 if (delta >= DELTA_HUMIDITY) { 00047 (*_TFT).DL(COLOR_RGB(255, 0, 0)); 00048 (*_TFT).DL(BEGIN(LINES)); 00049 (*_TFT).DL(LINE_WIDTH(8)); 00050 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (200 - currentValue) * 16)); 00051 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (180 - currentValue) * 16)); 00052 (*_TFT).DL(END()); 00053 (*_TFT).DL(BEGIN(POINTS)); 00054 (*_TFT).DL(POINT_SIZE(33)); 00055 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (180 - currentValue) * 16)); 00056 (*_TFT).DL(END()); 00057 } 00058 (*_TFT).DL(COLOR_RGB(0, 0, 0)); 00059 (*_TFT).DL(BEGIN(LINES)); 00060 (*_TFT).DL(LINE_WIDTH(16)); 00061 (*_TFT).DL(VERTEX2F((i - 1) * 23 + 25 * 16, (200 - previousValue) * 16)); 00062 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (200 - currentValue) * 16)); 00063 (*_TFT).DL(END()); 00064 } 00065 } 00066 00067 // write main title 00068 (*_TFT).DL(COLOR_RGB(0, 0, 0)); 00069 CreateStringRussian("Отн. влажность за 24 часа"); 00070 (*_TFT).Text(15, 15, 3, 0, russianStr); 00071 00072 // finish FT800 display list 00073 FinishDL(); 00074 } 00075 00076 /************************************************************************************************************************** 00077 ************************** Display data about temperature in the 24-hour interval ***************************************** 00078 **************************************************************************************************************************/ 00079 00080 void Display::StatTemperature_24hrs(volatile uint64_t seconds, short int statistics24hrs[3][288], uint64_t gridSecondsOffset) 00081 { 00082 // start FT800 display list 00083 StartDL(); 00084 00085 // mark 8 areas for touch input (for zoom in), 00086 // make each every second zone gray, make the active zone green 00087 char ZonesCnt = 0; 00088 for (int i = 25; i < 433; i += 17 * 3) { 00089 (*_TFT).DL(COLOR_RGB(255, 255, 255)); 00090 if (ZonesCnt % 2 == 0) { 00091 (*_TFT).DL(COLOR_RGB(233, 233, 233)); 00092 } 00093 if (pressedButton - ZONE_1_PRESS == ZonesCnt) { 00094 (*_TFT).DL(COLOR_RGB(200, 255, 200)); 00095 timePoint3hrs = 36 * ZonesCnt; 00096 } 00097 (*_TFT).DL(TAG_MASK(1)); 00098 (*_TFT).DL(TAG(ZONE_1_PRESS + ZonesCnt)); 00099 (*_TFT).DL(BEGIN(RECTS)); 00100 (*_TFT).DL(VERTEX2II(i, 100, 0, 0)); 00101 (*_TFT).DL(VERTEX2II(i + 17 * 3, 200, 0, 0)); 00102 (*_TFT).DL(TAG_MASK(0)); 00103 ZonesCnt++; 00104 } 00105 00106 // create time string, GridLines, link to main menu 00107 TimeSinceTurnOn(seconds); 00108 VerticalGrid24hrs(gridSecondsOffset); 00109 HorisontalGrid_Statistics_Temperature(); 00110 MainMenuReference(); 00111 00112 // draw graph line 00113 (*_TFT).DL(COLOR_RGB(0, 0, 0)); 00114 for (int i = 1; i < 287; i++) { 00115 char previousValue = (statistics24hrs[0][i - 1] / TEMPERATURE_MULTIPLIER + 50) * 0.57; 00116 char currentValue = (statistics24hrs[0][i] / TEMPERATURE_MULTIPLIER + 50) * 0.57; 00117 char delta = statistics24hrs[2][i] - statistics24hrs[1][i]; 00118 if (statistics24hrs[0][i] != INIT_STATISTICS_NUMBER) { 00119 if (delta >= DELTA_TEMPERATURE * TEMPERATURE_MULTIPLIER) { 00120 (*_TFT).DL(COLOR_RGB(255, 0, 0)); 00121 (*_TFT).DL(BEGIN(LINES)); 00122 (*_TFT).DL(LINE_WIDTH(8)); 00123 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (200 - currentValue) * 16)); 00124 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (180 - currentValue) * 16)); 00125 (*_TFT).DL(END()); 00126 (*_TFT).DL(BEGIN(POINTS)); 00127 (*_TFT).DL(POINT_SIZE(33)); 00128 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (180 - currentValue) * 16)); 00129 (*_TFT).DL(END()); 00130 } 00131 (*_TFT).DL(COLOR_RGB(0, 0, 0)); 00132 (*_TFT).DL(BEGIN(LINES)); 00133 (*_TFT).DL(LINE_WIDTH(16)); 00134 (*_TFT).DL(VERTEX2F((i - 1) * 23 + 25 * 16, (200 - previousValue) * 16)); 00135 (*_TFT).DL(VERTEX2F(i * 23 + 25 * 16, (200 - currentValue) * 16)); 00136 } 00137 } 00138 00139 // write main title 00140 (*_TFT).DL(COLOR_RGB(0, 0, 0)); 00141 CreateStringRussian("Температура за 24 часа"); 00142 (*_TFT).Text(15, 15, 3, 0, russianStr); 00143 00144 // finish FT800 display list 00145 FinishDL(); 00146 }
Generated on Wed Jul 13 2022 04:41:34 by
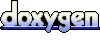