
Measuring air flow, relative humidity & temperature, then showing results at TFT
Dependencies: FT800_2 HYT mbed
StringsTransforming.cpp
00001 #include "Display.h" 00002 00003 /*************************************************************************************************************************/ 00004 void Display::CreateStringRussian(const string rustext) 00005 { 00006 // CHANGED ASCII: 00007 // 0123456789АБВГДЕЖЗИЙКЛМНОПРСТУФХЦЧШЩЪЫЬЭЮЯабвгдежзийклмнопрстуфхцчшщъыьэюя.,:-°±%<>rHYTICS 00008 int len = rustext.length(); 00009 int j = 0; 00010 for (int i = 0; i < len; i ++) { 00011 uint16_t res = uint8_t(rustext[i]); 00012 if (res > 0x7F) { 00013 res = res << 8 | uint8_t(rustext[i + 1]); 00014 // АБВ ... ноп 00015 if ((res >= 0xD090) && (res <= 0xD0BF)) { 00016 char offset = (char)(res - 0xD090); 00017 russianStr[j] = 32 + 11 + offset; 00018 // рст ... эюя 00019 } else if ((res >= 0xD180) && (res <= 0xD18F)) { 00020 char offset = (char)(res - 0xD180); 00021 russianStr[j] = 32 + 59 + offset; 00022 } 00023 // Degree sign 00024 else if (res == 0xC2B0) { 00025 russianStr[j] = 32 + 79; 00026 } 00027 // Plus-minus sign 00028 else if (res == 0xC2B1) { 00029 russianStr[j] = 32 + 80; 00030 } 00031 i++; 00032 } else { 00033 // Space 00034 if (res == 0x20) { 00035 russianStr[j] = 32; 00036 } 00037 // Numbers 00038 else if (res >= 0x30 && res <= 0x39) { 00039 russianStr[j] = 32 + 1 + (res - 0x30); 00040 } 00041 // . 00042 else if (res == 0x2E) { 00043 russianStr[j] = 32 + 75; 00044 } 00045 // , 00046 else if (res == 0x2C) { 00047 russianStr[j] = 32 + 76; 00048 } 00049 // : 00050 else if (res == 0x3A) { 00051 russianStr[j] = 32 + 77; 00052 } 00053 // - 00054 else if (res == 0x2D) { 00055 russianStr[j] = 32 + 78; 00056 } 00057 // % 00058 else if (res == 0x25) { 00059 russianStr[j] = 32 + 81; 00060 } 00061 // < 00062 else if (res == 0x3C) { 00063 russianStr[j] = 32 + 82; 00064 } 00065 // > 00066 else if (res == 0x3C) { 00067 russianStr[j] = 32 + 83; 00068 } 00069 // "r" 00070 else if (res == 0x72) { 00071 russianStr[j] = 32 + 84; 00072 } 00073 // "H" 00074 else if (res == 0x48) { 00075 russianStr[j] = 32 + 85; 00076 } 00077 // "Y" 00078 else if (res == 0x59) { 00079 russianStr[j] = 32 + 86; 00080 } 00081 // "T" 00082 else if (res == 0x54) { 00083 russianStr[j] = 32 + 87; 00084 } 00085 // "I" 00086 else if (res == 0x49) { 00087 russianStr[j] = 32 + 88; 00088 } 00089 // "C" 00090 else if (res == 0x43) { 00091 russianStr[j] = 32 + 89; 00092 } 00093 // "S" 00094 else if (res == 0x53) { 00095 russianStr[j] = 32 + 90; 00096 } 00097 } 00098 j++; 00099 } 00100 russianStr[j] = 0; 00101 } 00102 00103 /*************************************************************************************************************************/ 00104 void Display::CreateStringHumidity(char *str, uint8_t humidity) 00105 { 00106 uint8_t strCnt = 0; 00107 if (humidity == 100) { 00108 str[strCnt] = 32 + 2; 00109 strCnt++; 00110 } 00111 if (humidity >= 10) { 00112 str[strCnt] = 32 + 1 + humidity / 10; 00113 strCnt++; 00114 } 00115 str[strCnt] = 32 + 1 + humidity % 10; 00116 strCnt++; 00117 str[strCnt] = 32 + 81; 00118 strCnt++; 00119 // str[strCnt] = 32 + 84; 00120 // strCnt++; 00121 // str[strCnt] = 32 + 85; 00122 // strCnt++; 00123 str[strCnt] = 0; 00124 } 00125 00126 /*************************************************************************************************************************/ 00127 void Display::CreateStringFlow(char *str, float flow) 00128 { 00129 int8_t multipedFlow = (int8_t)(flow * 10); 00130 uint8_t strCnt = 0; 00131 if (multipedFlow == 100) { 00132 str[strCnt] = 32 + 2; 00133 strCnt++; 00134 } 00135 if (multipedFlow >= 10) { 00136 str[strCnt] = 32 + 1 + multipedFlow / 10; 00137 } else { 00138 str[strCnt] = 32 + 1; 00139 } 00140 strCnt++; 00141 str[strCnt] = 32 + 75; 00142 strCnt++; 00143 str[strCnt] = 32 + 1 + multipedFlow % 10; 00144 strCnt++; 00145 str[strCnt] = 32; 00146 strCnt++; 00147 str[strCnt] = 32 + 55; 00148 strCnt++; 00149 str[strCnt] = 32 + 86; 00150 strCnt++; 00151 str[strCnt] = 32 + 60; 00152 strCnt++; 00153 str[strCnt] = 0; 00154 } 00155 00156 /*************************************************************************************************************************/ 00157 void Display::CreateStringTemperature(char *str, int8_t temperature) 00158 { 00159 int8_t strCnt = 0; 00160 if (temperature < 0) { 00161 temperature = -temperature; 00162 str[strCnt] = 32 + 78; 00163 strCnt++; 00164 } 00165 if (temperature >= 10) { 00166 str[strCnt] = 32 + 1 + temperature / 10; 00167 strCnt++; 00168 } 00169 str[strCnt] = 32 + 1 + temperature % 10; 00170 strCnt++; 00171 str[strCnt] = 32 + 79; 00172 strCnt++; 00173 str[strCnt] = 32 + 87; 00174 strCnt++; 00175 str[strCnt] = 0; 00176 }
Generated on Fri Jul 15 2022 19:43:07 by
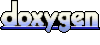