Double Tap Enable MMA8451Q library
Dependents: FRDM_MMA8451Q_DTAP_DEMO FRDM_TSI data_logger quadCommand2 ... more
Fork of MMA8451Q by
MMA8451Q.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef MMA8451Q_H 00020 #define MMA8451Q_H 00021 00022 #include "mbed.h" 00023 00024 /** 00025 * MMA8451Q accelerometer example 00026 * 00027 * @code 00028 * #include "mbed.h" 00029 * #include "MMA8451Q.h" 00030 * 00031 * #define MMA8451_I2C_ADDRESS (0x1d<<1) 00032 * 00033 * int main(void) { 00034 * 00035 * MMA8451Q acc(P_E25, P_E24, MMA8451_I2C_ADDRESS); 00036 * PwmOut rled(LED_RED); 00037 * PwmOut gled(LED_GREEN); 00038 * PwmOut bled(LED_BLUE); 00039 * 00040 * while (true) { 00041 * rled = 1.0 - abs(acc.getAccX()); 00042 * gled = 1.0 - abs(acc.getAccY()); 00043 * bled = 1.0 - abs(acc.getAccZ()); 00044 * wait(0.1); 00045 * } 00046 * } 00047 * @endcode 00048 */ 00049 class MMA8451Q 00050 { 00051 public: 00052 /** 00053 * MMA8451Q constructor 00054 * 00055 * @param sda SDA pin 00056 * @param sdl SCL pin 00057 * @param addr addr of the I2C peripheral 00058 */ 00059 MMA8451Q(PinName sda, PinName scl, int addr); 00060 00061 /** 00062 * MMA8451Q destructor 00063 */ 00064 ~MMA8451Q(); 00065 00066 /** 00067 * Get the value of the WHO_AM_I register 00068 * 00069 * @returns WHO_AM_I value 00070 */ 00071 uint8_t getWhoAmI(); 00072 00073 /** 00074 * Get X axis acceleration 00075 * 00076 * @returns X axis acceleration 00077 */ 00078 float getAccX(); 00079 00080 /** 00081 * Get Y axis acceleration 00082 * 00083 * @returns Y axis acceleration 00084 */ 00085 float getAccY(); 00086 00087 /** 00088 * Get Z axis acceleration 00089 * 00090 * @returns Z axis acceleration 00091 */ 00092 float getAccZ(); 00093 00094 /** 00095 * Get XYZ axis acceleration 00096 * 00097 * @param res array where acceleration data will be stored 00098 */ 00099 void getAccAllAxis(float * res); 00100 00101 /** JK 00102 * Setup Double Tap detection 00103 00104 00105 Example: 00106 00107 #include "mbed.h" 00108 #include "MMA8451Q.h" 00109 00110 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00111 #define ON 0 00112 #define OFF !ON 00113 00114 //Setup the interrupts for the MMA8451Q 00115 InterruptIn accInt1(PTA14); 00116 InterruptIn accInt2(PTA15);//not used in this prog but this is the other int from the accelorometer 00117 00118 uint8_t togstat=0;//Led status 00119 DigitalOut bled(LED_BLUE); 00120 00121 00122 void tapTrue(void){//ISR 00123 if(togstat == 0){ 00124 togstat = 1; 00125 bled=ON; 00126 } else { 00127 togstat = 0; 00128 bled=OFF; 00129 } 00130 00131 } 00132 00133 00134 int main(void) { 00135 00136 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS);//accelorometer instance 00137 00138 acc.setDoubleTap();//Setup the MMA8451Q to look for a double Tap 00139 accInt1.rise(&tapTrue);//call tapTrue when an interrupt is generated on PTA14 00140 00141 while (true) { 00142 //Interrupt driven so nothing in main loop 00143 } 00144 } 00145 00146 00147 */ 00148 void setDoubleTap(void); 00149 00150 private: 00151 I2C m_i2c; 00152 int m_addr; 00153 void readRegs(int addr, uint8_t * data, int len); 00154 void writeRegs(uint8_t * data, int len); 00155 int16_t getAccAxis(uint8_t addr); 00156 00157 }; 00158 00159 #endif
Generated on Thu Jul 21 2022 09:00:08 by
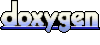