
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_network.h File Reference
PAL network - platform. This file contains the network APIs that need to be implemented in the platform layer. More...
Go to the source code of this file.
Functions | |
palStatus_t | pal_plat_socketsInit (void *context) |
PAL network socket API /n PAL network socket configuration options:
| |
palStatus_t | pal_plat_registerNetworkInterface (void *networkInterfaceContext, uint32_t *interfaceIndex) |
palStatus_t | pal_plat_socketsTerminate (void *context) |
palStatus_t | pal_plat_socket (palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palSocket_t *socket) |
palStatus_t | pal_plat_setSocketOptions (palSocket_t socket, int optionName, const void *optionValue, palSocketLength_t optionLength) |
palStatus_t | pal_plat_isNonBlocking (palSocket_t socket, bool *isNonBlocking) |
palStatus_t | pal_plat_bind (palSocket_t socket, palSocketAddress_t *myAddress, palSocketLength_t addressLength) |
palStatus_t | pal_plat_receiveFrom (palSocket_t socket, void *buffer, size_t length, palSocketAddress_t *from, palSocketLength_t *fromLength, size_t *bytesReceived) |
palStatus_t | pal_plat_sendTo (palSocket_t socket, const void *buffer, size_t length, const palSocketAddress_t *to, palSocketLength_t toLength, size_t *bytesSent) |
palStatus_t | pal_plat_close (palSocket_t *socket) |
palStatus_t | pal_plat_getNumberOfNetInterfaces (uint32_t *numInterfaces) |
palStatus_t | pal_plat_getNetInterfaceInfo (uint32_t interfaceNum, palNetInterfaceInfo_t *interfaceInfo) |
palStatus_t | pal_plat_listen (palSocket_t socket, int backlog) |
palStatus_t | pal_plat_accept (palSocket_t socket, palSocketAddress_t *address, palSocketLength_t *addressLen, palSocket_t *acceptedSocket) |
palStatus_t | pal_plat_connect (palSocket_t socket, const palSocketAddress_t *address, palSocketLength_t addressLen) |
palStatus_t | pal_plat_recv (palSocket_t socket, void *buf, size_t len, size_t *recievedDataSize) |
palStatus_t | pal_plat_send (palSocket_t socket, const void *buf, size_t len, size_t *sentDataSize) |
palStatus_t | pal_plat_asynchronousSocket (palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback, void *callbackArgument, palSocket_t *socket) |
palStatus_t | pal_plat_getAddressInfo (const char *url, palSocketAddress_t *address, palSocketLength_t *addressLength) |
palStatus_t | pal_plat_getAddressInfoAsync (pal_asyncAddressInfo_t *info) |
palStatus_t | pal_plat_cancelAddressInfoAsync (palDNSQuery_t queryHandle) |
Detailed Description
PAL network - platform. This file contains the network APIs that need to be implemented in the platform layer.
Definition in file pal_plat_network.h.
Function Documentation
palStatus_t pal_plat_accept | ( | palSocket_t | socket, |
palSocketAddress_t * | address, | ||
palSocketLength_t * | addressLen, | ||
palSocket_t * | acceptedSocket | ||
) |
Accept a connection on the given socket.
- Parameters:
-
[in] socket The socket on which to accept the connection. The socket needs to be created and bound and listen must have been called on it. [sockets passed to this function should be of type PAL_SOCK_STREAM_SERVER (the implementation may support other types as well)]. [out] address The source address of the incoming connection. [in,out] addressLen The length of the address field on input, the length of the data returned on output. [out] acceptedSocket The socket of the accepted connection is returned if the connection is accepted successfully.
- Returns:
- PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure.
Definition at line 698 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_asynchronousSocket | ( | palSocketDomain_t | domain, |
palSocketType_t | type, | ||
bool | nonBlockingSocket, | ||
uint32_t | interfaceNum, | ||
palAsyncSocketCallback_t | callback, | ||
void * | callbackArgument, | ||
palSocket_t * | socket | ||
) |
Get an asynchronous network socket.
- Parameters:
-
[in] domain The domain of the created socket (see enum `palSocketDomain_t` for supported types). [in] type The type of the created socket (see enum `palSocketType_t` for supported types). [in] callback A callback function that is called when any supported event takes place in the given asynchronous socket. [in] callbackArgument the argument with which the callback will be called when any supported event takes place in the given asynchronous socket. [out] socket This output parameter returns the socket.
- Returns:
- PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure.
Definition at line 923 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_bind | ( | palSocket_t | socket, |
palSocketAddress_t * | myAddress, | ||
palSocketLength_t | addressLength | ||
) |
Bind a given socket to a local address.
- Parameters:
-
[in] socket The socket to bind. [in] myAddress The address to bind to. [in] addressLength The length of the address passed in `myAddress`.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 372 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_cancelAddressInfoAsync | ( | palDNSQuery_t | queryHandle ) |
This function is cancelation for pal_plat_getAddressInfoAsync.
- Parameters:
-
[in] queryHandle Id of ongoing DNS query.
Definition at line 1249 of file pal_plat_network.cpp.
palStatus_t pal_plat_close | ( | palSocket_t * | socket ) |
Close a network socket.
- Note:
- The function recieves `palSocket_t*` and not `palSocket_t` so that it can zero the socket to avoid re-use.
- Parameters:
-
[in,out] socket Release and zero socket pointed to by given pointer.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 554 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_connect | ( | palSocket_t | socket, |
const palSocketAddress_t * | address, | ||
palSocketLength_t | addressLen | ||
) |
Open a connection from the given socket to the given address.
- Parameters:
-
[in] socket The socket to use for the connection to the given address [sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well)]. [in] address The destination address of the connection. [in] addressLen The length of the address field.
- Returns:
- PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure.
Definition at line 767 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_getAddressInfo | ( | const char * | url, |
palSocketAddress_t * | address, | ||
palSocketLength_t * | addressLength | ||
) |
This function translates the URL to a `palSocketAddress_t` that can be used with PAL sockets.
- Parameters:
-
[in] url The URL to be translated to a `palSocketAddress_t`. [out] address The address for the output of the translation.
Definition at line 993 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_getAddressInfoAsync | ( | pal_asyncAddressInfo_t * | info ) |
This function translates the URL to a `palSocketAddress_t` that can be used with PAL sockets.
- Parameters:
-
[in] info address of `pal_asyncAddressInfo_t`.
Definition at line 1218 of file pal_plat_network.cpp.
palStatus_t pal_plat_getNetInterfaceInfo | ( | uint32_t | interfaceNum, |
palNetInterfaceInfo_t * | interfaceInfo | ||
) |
Get information regarding the socket at the index/interface number given (this number is returned when registering the socket).
- Parameters:
-
[in] interfaceNum The number of the interface to get information for. [out] interfaceInfo The information for the given interface number.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 606 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_getNumberOfNetInterfaces | ( | uint32_t * | numInterfaces ) |
Get the number of current network interfaces (interfaces that have been registered through).
- Parameters:
-
[out] numInterfaces The number of interfaces after a successful call.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 600 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_isNonBlocking | ( | palSocket_t | socket, |
bool * | isNonBlocking | ||
) |
Check if the given socket is non-blocking.
- Parameters:
-
[in] socket The socket for which to check non-blocking status. [out] isNonBlocking The non-blocking status for the socket (true if non-blocking, otherwise false).
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 350 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_listen | ( | palSocket_t | socket, |
int | backlog | ||
) |
Use a socket to listen to incoming connections. You may also limit the queue of incoming connections.
- Parameters:
-
[in] socket The socket to listen to [sockets passed to this function should be of type PAL_SOCK_STREAM_SERVER (the implementation may support other types as well)]. [in] backlog The number of pending connections that can be saved for the socket.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 680 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_receiveFrom | ( | palSocket_t | socket, |
void * | buffer, | ||
size_t | length, | ||
palSocketAddress_t * | from, | ||
palSocketLength_t * | fromLength, | ||
size_t * | bytesReceived | ||
) |
Receive a payload from the given socket.
- Parameters:
-
[in] socket The socket to receive from [sockets passed to this function should be of type PAL_SOCK_DGRAM (the implementation may support other types as well)]. [out] buffer The buffer for the payload data. [in] length The length of the buffer for the payload data. [out] from The address that sent the payload [optional - if not required pass NULL]. [in,out] fromLength The length of the `from` address. When completed, this contains the amount of data actually written to the `from` address [optional - if not required pass NULL]. [out] bytesReceived The actual amount of payload data received in the buffer.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 405 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_recv | ( | palSocket_t | socket, |
void * | buf, | ||
size_t | len, | ||
size_t * | recievedDataSize | ||
) |
Receive data from the given connected socket.
- Parameters:
-
[in] socket The connected socket on which to receive data [sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well)]. [out] buf The output buffer for the message data. [in] len The length of the input data buffer. [out] recievedDataSize The length of the data actually received.
- Returns:
- PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure.
Definition at line 818 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_registerNetworkInterface | ( | void * | networkInterfaceContext, |
uint32_t * | interfaceIndex | ||
) |
Register a network interface for use with PAL sockets - must be called before other socket functions - most APIs will not work before a single interface is added.
- Parameters:
-
[in] networkInterfaceContext The context of the network interface to be added (OS specific. In mbed OS, this is the NetworkInterface object pointer for the network adapter [**note:** We assume connect has already been called on this]). - if not available use NULL (may not be required on some OSs). [out] interfaceIndex Contains the index assigned to the interface if it has been assigned successfully. This index can be used when creating a socket to bind the socket to the interface.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 112 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_send | ( | palSocket_t | socket, |
const void * | buf, | ||
size_t | len, | ||
size_t * | sentDataSize | ||
) |
Send a given buffer via the given connected socket.
- Parameters:
-
[in] socket The connected socket on which to send data [sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well)]. [in] buf The output buffer for the message data. [in] len The length of the input data buffer. [out] sentDataSize The length of the data sent.
- Returns:
- PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure.
Definition at line 883 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_sendTo | ( | palSocket_t | socket, |
const void * | buffer, | ||
size_t | length, | ||
const palSocketAddress_t * | to, | ||
palSocketLength_t | toLength, | ||
size_t * | bytesSent | ||
) |
Send a payload to the given address using the given socket.
- Parameters:
-
[in] socket The socket to use for sending the payload [sockets passed to this function should be of type PAL_SOCK_DGRAM (the implementation may support other types as well)]. [in] buffer The buffer for the payload data. [in] length The length of the buffer for the payload data. [in] to The address to which the payload should be sent. [in] toLength The length of the `to` address. [out] bytesSent The actual amount of payload data sent.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 487 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_setSocketOptions | ( | palSocket_t | socket, |
int | optionName, | ||
const void * | optionValue, | ||
palSocketLength_t | optionLength | ||
) |
Set options for a given network socket. Only a few options are supported (see `palSocketOptionName_t`).
- Parameters:
-
[in] socket The socket to get options for. [in] optionName The name to set the option for (see enum palSocketOptionName_t for supported types). [in] optionValue The buffer holding the value to set for the given option. [in] optionLength The size of the buffer provided for `optionValue`.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 292 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_socket | ( | palSocketDomain_t | domain, |
palSocketType_t | type, | ||
bool | nonBlockingSocket, | ||
uint32_t | interfaceNum, | ||
palSocket_t * | socket | ||
) |
Get a network socket.
- Parameters:
-
[in] domain The domain of the created socket (see `palSocketDomain_t` for supported types). [in] type The type of the created socket (see `palSocketType_t` for supported types). [in] nonBlockingSocket If true, the socket is non-blocking (with O_NONBLOCK set). [in] interfaceNum The number of the network interface used for this socket (info in interfaces supported via `pal_getNumberOfNetInterfaces` and `pal_getNetInterfaceInfo`), select PAL_NET_DEFAULT_INTERFACE as the default interface. [out] socket The socket is returned through this output parameter.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 212 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_socketsInit | ( | void * | context ) |
PAL network socket API /n PAL network socket configuration options:
- define PAL_NET_TCP_AND_TLS_SUPPORT if TCP is supported by the platform and is required.
- define PAL_NET_ASYNCHRONOUS_SOCKET_API if asynchronous socket API is supported by the platform. Currently MANDATORY.
- define PAL_NET_DNS_SUPPORT if DNS name resolution is supported.
Initialize sockets - must be called before other socket functions (is called from PAL init).
- Parameters:
-
[in] context Optional context - if not available/applicable use NULL.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 105 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
palStatus_t pal_plat_socketsTerminate | ( | void * | context ) |
Initialize terminate - can be called when sockets are no longer needed to free socket resources allocated by init.
- Parameters:
-
[in] context Optional context - if not available use NULL.
- Returns:
- PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure.
Definition at line 145 of file FreeRTOS/Networking/LWIP/pal_plat_network.c.
Generated on Tue Jul 12 2022 19:12:18 by
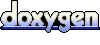