
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_network.h
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 00018 #ifndef _PAL_PLAT_SOCKET_H 00019 #define _PAL_PLAT_SOCKET_H 00020 00021 #include "pal.h" 00022 #include "pal_network.h" 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /*! \file pal_plat_network.h 00029 * \brief PAL network - platform. 00030 * This file contains the network APIs that need to be implemented in the platform layer. 00031 */ 00032 00033 //! PAL network socket API /n 00034 //! PAL network socket configuration options: 00035 //! - define PAL_NET_TCP_AND_TLS_SUPPORT if TCP is supported by the platform and is required. 00036 //! - define PAL_NET_ASYNCHRONOUS_SOCKET_API if asynchronous socket API is supported by the platform. Currently MANDATORY. 00037 //! - define PAL_NET_DNS_SUPPORT if DNS name resolution is supported. 00038 00039 /*! Initialize sockets - must be called before other socket functions (is called from PAL init). 00040 * @param[in] context Optional context - if not available/applicable use NULL. 00041 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00042 */ 00043 palStatus_t pal_plat_socketsInit(void* context); 00044 00045 /*! Register a network interface for use with PAL sockets - must be called before other socket functions - most APIs will not work before a single interface is added. 00046 * @param[in] networkInterfaceContext The context of the network interface to be added (OS specific. In mbed OS, this is the NetworkInterface object pointer for the network adapter [**note:** We assume connect has already been called on this]). - if not available use NULL (may not be required on some OSs). 00047 * @param[out] interfaceIndex Contains the index assigned to the interface if it has been assigned successfully. This index can be used when creating a socket to bind the socket to the interface. 00048 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00049 */ 00050 palStatus_t pal_plat_registerNetworkInterface (void* networkInterfaceContext, uint32_t* interfaceIndex); 00051 00052 /*! Initialize terminate - can be called when sockets are no longer needed to free socket resources allocated by init. 00053 * @param[in] context Optional context - if not available use NULL. 00054 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00055 */ 00056 palStatus_t pal_plat_socketsTerminate (void* context); 00057 00058 /*! Get a network socket. 00059 * @param[in] domain The domain of the created socket (see `palSocketDomain_t` for supported types). 00060 * @param[in] type The type of the created socket (see `palSocketType_t` for supported types). 00061 * @param[in] nonBlockingSocket If true, the socket is non-blocking (with O_NONBLOCK set). 00062 * @param[in] interfaceNum The number of the network interface used for this socket (info in interfaces supported via `pal_getNumberOfNetInterfaces` and `pal_getNetInterfaceInfo`), select PAL_NET_DEFAULT_INTERFACE as the default interface. 00063 * @param[out] socket The socket is returned through this output parameter. 00064 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00065 */ 00066 palStatus_t pal_plat_socket (palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palSocket_t* socket); 00067 00068 /*! Set options for a given network socket. Only a few options are supported (see `palSocketOptionName_t`). 00069 * @param[in] socket The socket to get options for. 00070 * @param[in] optionName The name to set the option for (see enum palSocketOptionName_t for supported types). 00071 * @param[in] optionValue The buffer holding the value to set for the given option. 00072 * @param[in] optionLength The size of the buffer provided for `optionValue`. 00073 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00074 */ 00075 palStatus_t pal_plat_setSocketOptions (palSocket_t socket, int optionName, const void* optionValue, palSocketLength_t optionLength); 00076 00077 /*! Check if the given socket is non-blocking. 00078 * @param[in] socket The socket for which to check non-blocking status. 00079 * @param[out] isNonBlocking The non-blocking status for the socket (true if non-blocking, otherwise false). 00080 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00081 */ 00082 palStatus_t pal_plat_isNonBlocking (palSocket_t socket, bool* isNonBlocking); 00083 00084 /*! Bind a given socket to a local address. 00085 * @param[in] socket The socket to bind. 00086 * @param[in] myAddress The address to bind to. 00087 * @param[in] addressLength The length of the address passed in `myAddress`. 00088 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00089 */ 00090 palStatus_t pal_plat_bind (palSocket_t socket, palSocketAddress_t* myAddress, palSocketLength_t addressLength); 00091 00092 /*! Receive a payload from the given socket. 00093 * @param[in] socket The socket to receive from [sockets passed to this function should be of type PAL_SOCK_DGRAM (the implementation may support other types as well)]. 00094 * @param[out] buffer The buffer for the payload data. 00095 * @param[in] length The length of the buffer for the payload data. 00096 * @param[out] from The address that sent the payload [optional - if not required pass NULL]. 00097 * @param[in, out] fromLength The length of the `from` address. When completed, this contains the amount of data actually written to the `from` address [optional - if not required pass NULL]. 00098 * @param[out] bytesReceived The actual amount of payload data received in the buffer. 00099 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00100 */ 00101 palStatus_t pal_plat_receiveFrom (palSocket_t socket, void* buffer, size_t length, palSocketAddress_t* from, palSocketLength_t* fromLength, size_t* bytesReceived); 00102 00103 /*! Send a payload to the given address using the given socket. 00104 * @param[in] socket The socket to use for sending the payload [sockets passed to this function should be of type PAL_SOCK_DGRAM (the implementation may support other types as well)]. 00105 * @param[in] buffer The buffer for the payload data. 00106 * @param[in] length The length of the buffer for the payload data. 00107 * @param[in] to The address to which the payload should be sent. 00108 * @param[in] toLength The length of the `to` address. 00109 * @param[out] bytesSent The actual amount of payload data sent. 00110 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00111 */ 00112 palStatus_t pal_plat_sendTo (palSocket_t socket, const void* buffer, size_t length, const palSocketAddress_t* to, palSocketLength_t toLength, size_t* bytesSent); 00113 00114 /*! Close a network socket. \n 00115 * \note The function recieves `palSocket_t*` and not `palSocket_t` so that it can zero the socket to avoid re-use. 00116 * @param[in,out] socket Release and zero socket pointed to by given pointer. 00117 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00118 */ 00119 palStatus_t pal_plat_close (palSocket_t* socket); 00120 00121 /*! Get the number of current network interfaces (interfaces that have been registered through). 00122 * @param[out] numInterfaces The number of interfaces after a successful call. 00123 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00124 */ 00125 palStatus_t pal_plat_getNumberOfNetInterfaces (uint32_t* numInterfaces); 00126 00127 /*! Get information regarding the socket at the index/interface number given (this number is returned when registering the socket). 00128 * @param[in] interfaceNum The number of the interface to get information for. 00129 * @param[out] interfaceInfo The information for the given interface number. 00130 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00131 */ 00132 palStatus_t pal_plat_getNetInterfaceInfo (uint32_t interfaceNum, palNetInterfaceInfo_t * interfaceInfo); 00133 00134 00135 #if PAL_NET_TCP_AND_TLS_SUPPORT // The functionality below is supported only if TCP is supported. 00136 00137 00138 /*! Use a socket to listen to incoming connections. You may also limit the queue of incoming connections. 00139 * @param[in] socket The socket to listen to [sockets passed to this function should be of type PAL_SOCK_STREAM_SERVER (the implementation may support other types as well)]. 00140 * @param[in] backlog The number of pending connections that can be saved for the socket. 00141 \return PAL_SUCCESS (0) in case of success. A specific negative error code in case of failure. 00142 */ 00143 palStatus_t pal_plat_listen (palSocket_t socket, int backlog); 00144 00145 /*! Accept a connection on the given socket. 00146 * @param[in] socket The socket on which to accept the connection. The socket needs to be created and bound and listen must have been called on it. [sockets passed to this function should be of type PAL_SOCK_STREAM_SERVER (the implementation may support other types as well)]. 00147 * @param[out] address The source address of the incoming connection. 00148 * @param[in, out] addressLen The length of the address field on input, the length of the data returned on output. 00149 * @param[out] acceptedSocket The socket of the accepted connection is returned if the connection is accepted successfully. 00150 \return PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure. 00151 */ 00152 palStatus_t pal_plat_accept (palSocket_t socket, palSocketAddress_t* address, palSocketLength_t* addressLen, palSocket_t* acceptedSocket); 00153 00154 /*! Open a connection from the given socket to the given address. 00155 * @param[in] socket The socket to use for the connection to the given address [sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well)]. 00156 * @param[in] address The destination address of the connection. 00157 * @param[in] addressLen The length of the address field. 00158 \return PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure. 00159 */ 00160 palStatus_t pal_plat_connect (palSocket_t socket, const palSocketAddress_t* address, palSocketLength_t addressLen); 00161 00162 /*! Receive data from the given connected socket. 00163 * @param[in] socket The connected socket on which to receive data [sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well)]. 00164 * @param[out] buf The output buffer for the message data. 00165 * @param[in] len The length of the input data buffer. 00166 * @param[out] recievedDataSize The length of the data actually received. 00167 \return PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure. 00168 */ 00169 palStatus_t pal_plat_recv (palSocket_t socket, void* buf, size_t len, size_t* recievedDataSize); 00170 00171 /*! Send a given buffer via the given connected socket. 00172 * @param[in] socket The connected socket on which to send data [sockets passed to this function should be of type PAL_SOCK_STREAM (the implementation may support other types as well)]. 00173 * @param[in] buf The output buffer for the message data. 00174 * @param[in] len The length of the input data buffer. 00175 * @param[out] sentDataSize The length of the data sent. 00176 \return PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure. 00177 */ 00178 palStatus_t pal_plat_send (palSocket_t socket, const void* buf, size_t len, size_t* sentDataSize); 00179 00180 00181 #endif //PAL_NET_TCP_AND_TLS_SUPPORT 00182 00183 00184 #if PAL_NET_ASYNCHRONOUS_SOCKET_API 00185 00186 /*! Get an asynchronous network socket. 00187 * @param[in] domain The domain of the created socket (see enum `palSocketDomain_t` for supported types). 00188 * @param[in] type The type of the created socket (see enum `palSocketType_t` for supported types). 00189 * @param[in] callback A callback function that is called when any supported event takes place in the given asynchronous socket. 00190 * @param[in] callbackArgument the argument with which the callback will be called when any supported event takes place in the given asynchronous socket. 00191 * @param[out] socket This output parameter returns the socket. 00192 \return PAL_SUCCESS (0) in case of success, a specific negative error code in case of failure. 00193 */ 00194 palStatus_t pal_plat_asynchronousSocket (palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback, void* callbackArgument , palSocket_t* socket); 00195 00196 #endif 00197 00198 #if PAL_NET_DNS_SUPPORT 00199 00200 /*! This function translates the URL to a `palSocketAddress_t` that can be used with PAL sockets. 00201 * @param[in] url The URL to be translated to a `palSocketAddress_t`. 00202 * @param[out] address The address for the output of the translation. 00203 */ 00204 palStatus_t pal_plat_getAddressInfo (const char* url, palSocketAddress_t* address, palSocketLength_t* addressLength); 00205 00206 #ifdef PAL_DNS_API_V2 00207 /*! This function translates the URL to a `palSocketAddress_t` that can be used with PAL sockets. 00208 * @param[in] info address of `pal_asyncAddressInfo_t`. 00209 */ 00210 palStatus_t pal_plat_getAddressInfoAsync (pal_asyncAddressInfo_t* info); 00211 00212 /*! This function is cancelation for pal_plat_getAddressInfoAsync. 00213 * @param[in] queryHandle Id of ongoing DNS query. 00214 */ 00215 palStatus_t pal_plat_cancelAddressInfoAsync (palDNSQuery_t queryHandle); 00216 #endif // PAL_DNS_API_V2 00217 00218 #endif // PAL_NET_DNS_SUPPORT 00219 00220 00221 #ifdef __cplusplus 00222 } 00223 #endif 00224 #endif //_PAL_PLAT_SOCKET_H
Generated on Tue Jul 12 2022 19:12:14 by
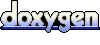