
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
ServiceClient Class Reference
ServiceClient This class handles all internal interactions between various client components including connector, identity and update. More...
#include <ServiceClient.h>
Inherits ConnectorClientCallback.
Public Types | |
enum | StartupMainState |
An enum defining the different states of ServiceClient during the client flow. More... | |
Public Member Functions | |
ServiceClient (ServiceClientCallback &callback) | |
Constructor. | |
virtual | ~ServiceClient () |
Destructor. | |
void | initialize_and_register (M2MBaseList ®_objs) |
Starts the registration or bootstrap sequence from MbedCloudClient. | |
ConnectorClient & | connector_client () |
Returns the ConnectorClient handler. | |
const ConnectorClient & | connector_client () const |
Returns const ConnectorClient handler. | |
bool | set_device_resource_value (M2MDevice::DeviceResource resource, const std::string &value) |
Set resource value in the Device Object. | |
bool | set_device_resource_value (M2MDevice::DeviceResource resource, const char *value, uint32_t length) |
Set resource value in the Device Object. | |
void | set_update_authorize_handler (void(*handler)(int32_t request)) |
Registers a callback function for authorizing firmware downloads and reboots. | |
void | update_authorize (int32_t request) |
Authorize request passed to authorization handler. | |
void | set_update_progress_handler (void(*handler)(uint32_t progress, uint32_t total)) |
Registers a callback function for monitoring download progress. | |
void | update_error_callback (int32_t error) |
Callback function for the Update Client. | |
Protected Member Functions | |
virtual void | registration_process_result (ConnectorClient::StartupSubStateRegistration status) |
Indicates that the registration or unregistration operation is complete with success or failure. | |
virtual void | connector_error (M2MInterface::Error error, const char *reason) |
Indicates a connector error condition from an underlying M2MInterface client. | |
virtual void | value_updated (M2MBase *base, M2MBase::BaseType type) |
A callback indicating that the value of the resource object is updated by the LWM2M Cloud server. | |
void | state_function (StartupMainState current_state) |
Redirects the state machine to the right function. | |
void | state_engine (void) |
The state engine maintaining the state machine logic. | |
void | external_event (StartupMainState new_state) |
An external event that can trigger the state machine. | |
void | internal_event (StartupMainState new_state) |
An internal event generated by the state machine. | |
void | state_bootstrap () |
When the bootstrap is started. | |
void | state_register () |
When the registration is started. | |
void | state_success () |
When the registration is successful. | |
void | state_failure () |
When the registration has failed. | |
void | state_unregister () |
When the client unregisters. |
Detailed Description
ServiceClient This class handles all internal interactions between various client components including connector, identity and update.
This class maintains the state machine for the use case flow of mbed Cloud Client.
Definition at line 85 of file ServiceClient.h.
Member Enumeration Documentation
enum StartupMainState |
An enum defining the different states of ServiceClient during the client flow.
Definition at line 93 of file ServiceClient.h.
Constructor & Destructor Documentation
ServiceClient | ( | ServiceClientCallback & | callback ) |
Constructor.
- Parameters:
-
interface,Takes the structure that contains the needed information for an endpoint client to register.
Definition at line 47 of file ServiceClient.cpp.
~ServiceClient | ( | ) | [virtual] |
Destructor.
Definition at line 62 of file ServiceClient.cpp.
Member Function Documentation
ConnectorClient & connector_client | ( | ) |
Returns the ConnectorClient handler.
- Returns:
- ConnectorClient, handled for ConnectorClient.
Definition at line 179 of file ServiceClient.cpp.
const ConnectorClient & connector_client | ( | ) | const |
Returns const ConnectorClient handler.
- Returns:
- const ConnectorClient, handled for ConnectorClient.
Definition at line 184 of file ServiceClient.cpp.
void connector_error | ( | M2MInterface::Error | error, |
const char * | reason | ||
) | [protected, virtual] |
Indicates a connector error condition from an underlying M2MInterface client.
- Parameters:
-
error,Indicates an error code translated from M2MInterface::Error.
Implements ConnectorClientCallback.
Definition at line 283 of file ServiceClient.cpp.
void external_event | ( | StartupMainState | new_state ) | [protected] |
An external event that can trigger the state machine.
- Parameters:
-
new_state,The new state to which the state machine should go. data,The data to be passed to the state machine.
void initialize_and_register | ( | M2MBaseList & | reg_objs ) |
Starts the registration or bootstrap sequence from MbedCloudClient.
- Parameters:
-
callback,Takes the callback for the status from ConnectorClient. client_objs,A list of objects to be registered to Cloud.
Definition at line 69 of file ServiceClient.cpp.
void internal_event | ( | StartupMainState | new_state ) | [protected] |
An internal event generated by the state machine.
- Parameters:
-
new_state,The new state to which the state machine should go. data,The data to be passed to the state machine.
Definition at line 191 of file ServiceClient.cpp.
void registration_process_result | ( | ConnectorClient::StartupSubStateRegistration | status ) | [protected, virtual] |
Indicates that the registration or unregistration operation is complete with success or failure.
- Parameters:
-
status,Indicates success or failure in terms of status code.
Implements ConnectorClientCallback.
Definition at line 263 of file ServiceClient.cpp.
bool set_device_resource_value | ( | M2MDevice::DeviceResource | resource, |
const std::string & | value | ||
) |
Set resource value in the Device Object.
- Parameters:
-
resource Device enum to have value set. value String object.
- Returns:
- True if successful, false otherwise.
Definition at line 467 of file ServiceClient.cpp.
bool set_device_resource_value | ( | M2MDevice::DeviceResource | resource, |
const char * | value, | ||
uint32_t | length | ||
) |
Set resource value in the Device Object.
- Parameters:
-
resource Device enum to have value set. value Byte buffer. length Buffer length.
- Returns:
- True if successful, false otherwise.
Definition at line 483 of file ServiceClient.cpp.
void set_update_authorize_handler | ( | void(*)(int32_t request) | handler ) |
Registers a callback function for authorizing firmware downloads and reboots.
- Parameters:
-
handler Callback function.
Definition at line 574 of file ServiceClient.cpp.
void set_update_progress_handler | ( | void(*)(uint32_t progress, uint32_t total) | handler ) |
Registers a callback function for monitoring download progress.
- Parameters:
-
handler Callback function.
Definition at line 584 of file ServiceClient.cpp.
void state_bootstrap | ( | ) | [protected] |
When the bootstrap is started.
Definition at line 243 of file ServiceClient.cpp.
void state_engine | ( | void | ) | [protected] |
The state engine maintaining the state machine logic.
Definition at line 204 of file ServiceClient.cpp.
void state_failure | ( | ) | [protected] |
When the registration has failed.
Definition at line 309 of file ServiceClient.cpp.
void state_function | ( | StartupMainState | current_state ) | [protected] |
Redirects the state machine to the right function.
- Parameters:
-
current_state,The current state to be set. data,The data to be passed to the state function.
Definition at line 221 of file ServiceClient.cpp.
void state_register | ( | ) | [protected] |
When the registration is started.
Definition at line 257 of file ServiceClient.cpp.
void state_success | ( | ) | [protected] |
When the registration is successful.
Definition at line 302 of file ServiceClient.cpp.
void state_unregister | ( | ) | [protected] |
When the client unregisters.
Definition at line 315 of file ServiceClient.cpp.
void update_authorize | ( | int32_t | request ) |
Authorize request passed to authorization handler.
- Parameters:
-
request Request being authorized.
Definition at line 579 of file ServiceClient.cpp.
void update_error_callback | ( | int32_t | error ) |
Callback function for the Update Client.
- Parameters:
-
error Internal Update Client error code.
Definition at line 589 of file ServiceClient.cpp.
void value_updated | ( | M2MBase * | base, |
M2MBase::BaseType | type | ||
) | [protected, virtual] |
A callback indicating that the value of the resource object is updated by the LWM2M Cloud server.
- Parameters:
-
base,The object whose value is updated. type,The type of the object.
Implements ConnectorClientCallback.
Definition at line 296 of file ServiceClient.cpp.
Generated on Tue Jul 12 2022 19:12:20 by
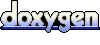