
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
ServiceClient.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef __SERVICE_CLIENT_H__ 00020 #define __SERVICE_CLIENT_H__ 00021 00022 #include "mbed-cloud-client/MbedCloudClientConfig.h" 00023 #ifdef MBED_CLOUD_CLIENT_SUPPORT_UPDATE 00024 #include "UpdateClient.h" 00025 #endif 00026 #include "mbed-client/m2minterface.h" 00027 #include "mbed-client/m2mdevice.h" 00028 #include "ConnectorClient.h" 00029 00030 #include <string> 00031 00032 class M2MSecurity; 00033 class ConnectorClientCallback; 00034 struct MbedClientDeviceInfo; 00035 struct MBedClientInterfaceInfo; 00036 00037 /** 00038 * \brief ServiceClientCallback 00039 * A callback class for passing the client progress and error condition to the 00040 * MbedCloudClient class object. 00041 */ 00042 class ServiceClientCallback { 00043 public: 00044 00045 typedef enum { 00046 Service_Client_Status_Failure = -1, 00047 Service_Client_Status_Registered = 0, 00048 Service_Client_Status_Unregistered = 1, 00049 Service_Client_Status_Register_Updated = 2 00050 } ServiceClientCallbackStatus; 00051 00052 /** 00053 * \brief Indicates that the setup or close operation is complete 00054 * with success or failure. 00055 * \param status, Indicates success or failure in terms of status code. 00056 */ 00057 virtual void complete(ServiceClientCallbackStatus status) = 0; 00058 00059 /** 00060 * \brief Indicates an error condition from one of the underlying clients, including 00061 * identity, connector or update client. 00062 * \param error, Indicates an error code translated to MbedCloudClient::Error. 00063 * \param reason, Indicates human readable text for error description. 00064 */ 00065 virtual void error(int error, const char *reason) = 0; 00066 00067 /** 00068 * \brief A callback indicating that the value of the resource object is updated 00069 * by the LWM2M Cloud server. 00070 * \param base, The object whose value is updated. 00071 * \param type, The type of the object. 00072 */ 00073 virtual void value_updated(M2MBase *base, M2MBase::BaseType type) = 0; 00074 }; 00075 00076 00077 /** 00078 * \brief ServiceClient 00079 * This class handles all internal interactions between various client 00080 * components including connector, identity and update. 00081 * This class maintains the state machine for the use case flow of mbed Cloud 00082 * Client. 00083 */ 00084 00085 class ServiceClient : private ConnectorClientCallback 00086 { 00087 public: 00088 00089 /** 00090 * \brief An enum defining the different states of 00091 * ServiceClient during the client flow. 00092 */ 00093 enum StartupMainState { 00094 State_Init, 00095 State_Bootstrap, 00096 State_Register, 00097 State_Success, 00098 State_Failure, 00099 State_Unregister 00100 }; 00101 00102 public: 00103 00104 /** 00105 * \brief Constructor. 00106 * \param interface, Takes the structure that contains the 00107 * needed information for an endpoint client to register. 00108 */ 00109 ServiceClient(ServiceClientCallback& callback); 00110 00111 /** 00112 * \brief Destructor. 00113 */ 00114 virtual ~ServiceClient(); 00115 00116 /** 00117 * \brief Starts the registration or bootstrap sequence from MbedCloudClient. 00118 * \param callback, Takes the callback for the status from ConnectorClient. 00119 * \param client_objs, A list of objects to be registered to Cloud. 00120 */ 00121 void initialize_and_register(M2MBaseList& reg_objs); 00122 00123 /** 00124 * \brief Returns the ConnectorClient handler. 00125 * \return ConnectorClient, handled for ConnectorClient. 00126 */ 00127 ConnectorClient &connector_client(); 00128 00129 /** 00130 * \brief Returns const ConnectorClient handler. 00131 * \return const ConnectorClient, handled for ConnectorClient. 00132 */ 00133 const ConnectorClient &connector_client() const; 00134 00135 /** 00136 * \brief Set resource value in the Device Object 00137 * 00138 * \param resource Device enum to have value set. 00139 * \param value String object. 00140 * \return True if successful, false otherwise. 00141 */ 00142 bool set_device_resource_value(M2MDevice::DeviceResource resource, 00143 const std::string& value); 00144 00145 /** 00146 * \brief Set resource value in the Device Object 00147 * 00148 * \param resource Device enum to have value set. 00149 * \param value Byte buffer. 00150 * \param length Buffer length. 00151 * \return True if successful, false otherwise. 00152 */ 00153 bool set_device_resource_value(M2MDevice::DeviceResource resource, 00154 const char* value, 00155 uint32_t length); 00156 00157 #ifdef MBED_CLOUD_CLIENT_SUPPORT_UPDATE 00158 /** 00159 * \brief Registers a callback function for authorizing firmware downloads and reboots. 00160 * \param handler Callback function. 00161 */ 00162 void set_update_authorize_handler(void (*handler)(int32_t request)); 00163 00164 /** 00165 * \brief Authorize request passed to authorization handler. 00166 * \param request Request being authorized. 00167 */ 00168 void update_authorize(int32_t request); 00169 00170 /** 00171 * \brief Registers a callback function for monitoring download progress. 00172 * \param handler Callback function. 00173 */ 00174 void set_update_progress_handler(void (*handler)(uint32_t progress, uint32_t total)); 00175 00176 /** 00177 * \brief Callback function for the Update Client. 00178 * \param error Internal Update Client error code. 00179 */ 00180 void update_error_callback(int32_t error); 00181 #endif 00182 00183 protected : 00184 00185 // Implementation of ConnectorClientCallback 00186 /** 00187 * \brief Indicates that the registration or unregistration operation is complete 00188 * with success or failure. 00189 * \param status, Indicates success or failure in terms of status code. 00190 */ 00191 virtual void registration_process_result(ConnectorClient::StartupSubStateRegistration status); 00192 00193 /** 00194 * \brief Indicates a connector error condition from an underlying M2MInterface client. 00195 * \param error, Indicates an error code translated from M2MInterface::Error. 00196 */ 00197 virtual void connector_error(M2MInterface::Error error, const char *reason); 00198 00199 /** 00200 * \brief A callback indicating that the value of the resource object is updated 00201 * by the LWM2M Cloud server. 00202 * \param base, The object whose value is updated. 00203 * \param type, The type of the object. 00204 */ 00205 virtual void value_updated(M2MBase *base, M2MBase::BaseType type); 00206 00207 /** 00208 * \brief Redirects the state machine to the right function. 00209 * \param current_state, The current state to be set. 00210 * \param data, The data to be passed to the state function. 00211 */ 00212 void state_function(StartupMainState current_state); 00213 00214 /** 00215 * \brief The state engine maintaining the state machine logic. 00216 */ 00217 void state_engine(void); 00218 00219 /** 00220 * An external event that can trigger the state machine. 00221 * \param new_state, The new state to which the state machine should go. 00222 * \param data, The data to be passed to the state machine. 00223 */ 00224 void external_event(StartupMainState new_state); 00225 00226 /** 00227 * An internal event generated by the state machine. 00228 * \param new_state, The new state to which the state machine should go. 00229 * \param data, The data to be passed to the state machine. 00230 */ 00231 void internal_event(StartupMainState new_state); 00232 00233 /** 00234 * When the bootstrap is started. 00235 */ 00236 void state_bootstrap(); 00237 00238 /** 00239 * When the registration is started. 00240 */ 00241 void state_register(); 00242 00243 /** 00244 * When the registration is successful. 00245 */ 00246 void state_success(); 00247 00248 /** 00249 * When the registration has failed. 00250 */ 00251 00252 void state_failure(); 00253 00254 /** 00255 * When the client unregisters. 00256 */ 00257 void state_unregister(); 00258 00259 private: 00260 M2MDevice* device_object_from_storage(); 00261 00262 /* lookup table for printing hexadecimal values */ 00263 static const uint8_t hex_table[16]; 00264 00265 ServiceClientCallback &_service_callback; 00266 // data which is pending for the registration 00267 const char *_service_uri; 00268 void *_stack; 00269 M2MBaseList *_client_objs; 00270 StartupMainState _current_state; 00271 bool _event_generated; 00272 bool _state_engine_running; 00273 #ifdef MBED_CLOUD_CLIENT_SUPPORT_UPDATE 00274 bool _setup_update_client; 00275 #endif 00276 ConnectorClient _connector_client; 00277 }; 00278 00279 #endif // !__SERVICE_CLIENT_H__
Generated on Tue Jul 12 2022 19:12:15 by
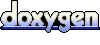