
Morse Code Machine
Fork of TextLCD_HelloWorld by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Morse Code Machine February 27, 2013 00002 00003 #include "mbed.h" 00004 #include "MorseCode.h" 00005 00006 //Need to Setup LCD Screen as TextLCD lcd(p15, p16, p17, p18, p19, p20) - Is included in MorseCode.cpp 00007 DigitalIn Button(p8); // Button for User to Input Morse Code 00008 DigitalOut LED(LED1); // LED to Indicate Time (every 1 seconds) 00009 PwmOut Speaker(p21); // Speaker to play noise when button pushed 00010 Timer Time; // Keeps track of time 00011 00012 int main() { 00013 // Setup 00014 int up = 0; 00015 int read = 0; 00016 Button.mode(PullUp); 00017 Speaker.period(1.0/800.0); 00018 Time.start(); 00019 00020 //Start 00021 while(1) { 00022 00023 //Timer For User Comfort 00024 if(Time.read_ms()%1000<15) { 00025 up = !up; 00026 read = 0; // Reset for Next Second 00027 if((Time.read_ms() - 1740000) > 0) { 00028 Time.reset(); // Reset if more than 29 minutes. 00029 } 00030 wait_ms(15); 00031 } 00032 00033 // LED Ticker 00034 LED = (up)? 1 : 0; 00035 00036 // Audio 00037 if(!Button) { 00038 Speaker = 0.25; // Volume 00039 wait_ms(15); 00040 } 00041 else { 00042 Speaker = 0.0; //Mute 00043 wait_ms(15); 00044 } 00045 00046 // Do Morse Code 00047 if(Time.read_ms()%1000 > 500 && read == 0) { // Half a Second Has Passed 00048 read = 1; 00049 MorseCode(!Button); // Read Button Value 00050 } 00051 00052 }// End While 00053 }
Generated on Wed Jul 13 2022 01:41:04 by
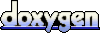