
Demo application for Adafruit NeoPixels.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "neopixel.h" 00003 00004 // This must be an SPI MOSI pin. 00005 #define DATA_PIN p5 00006 00007 void generate(neopixel::Pixel * out, uint32_t index, uintptr_t extra) 00008 { 00009 uint32_t brightness = (index + extra) >> 3; 00010 out->red = ((index + extra) & 0x1) ? brightness : 0; 00011 out->green = ((index + extra) & 0x2) ? brightness : 0; 00012 out->blue = ((index + extra) & 0x4) ? brightness : 0; 00013 } 00014 00015 int main() 00016 { 00017 // Create a temporary DigitalIn so we can configure the pull-down resistor. 00018 // (The mbed API doesn't provide any other way to do this.) 00019 // An alternative is to connect an external pull-down resistor. 00020 DigitalIn(DATA_PIN, PullDown); 00021 00022 // The pixel array control class. 00023 neopixel::PixelArray array(DATA_PIN); 00024 00025 uint32_t offset = 0; 00026 while (1) { 00027 array.update(generate, 100, offset++); 00028 wait_ms(250); 00029 } 00030 }
Generated on Tue Jul 12 2022 16:16:28 by
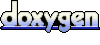