Library to access LPC17xx peripherals. It uses static inline functions, constant propagation and dead code elimination to be as fast as possible.
Dependents: Chua-VGA Wolfram-1D-VGA WolframRnd-1D-VGA Basin-VGA ... more
systick.h
00001 /* Copyright (C) 2011 by Ivo van Poorten <ivop@euronet.nl> 00002 * This file is licensed under the terms of the GNU Lesser 00003 * General Public License, version 3. 00004 */ 00005 00006 #ifndef FASTLIB_SYSTICK_H 00007 #define FASTLIB_SYSTICK_H 00008 00009 #include "fastlib/common.h" 00010 00011 #define FL_STCTRL ((volatile uint32_t *) 0xE000E010) 00012 #define FL_STRELOAD ((volatile uint32_t *) 0xE000E014) 00013 #define FL_STCURR ((volatile uint32_t *) 0xE000E018) 00014 #define FL_STCALIB ((volatile uint32_t *) 0xE000E01C) 00015 00016 static inline void fl_systick_control(const unsigned enable, 00017 const unsigned interrupt_enable, 00018 const unsigned use_cpu_clock) { 00019 *FL_STCTRL = enable | (interrupt_enable<<1) | (use_cpu_clock<<2); 00020 } 00021 00022 static inline unsigned fl_systick_get_counter_flag(void) { 00023 return *FL_STCTRL & (1U<<16); 00024 } 00025 00026 static inline void fl_systick_set_reload_value(const unsigned value) { 00027 *FL_STRELOAD = value; 00028 } 00029 00030 static inline unsigned fl_systick_get_reload_value(void) { 00031 return *FL_STRELOAD; 00032 } 00033 00034 static inline unsigned fl_systick_get_current_value(void) { 00035 return *FL_STCURR; 00036 } 00037 00038 static inline void fl_systick_set_calibration(const unsigned reload_value, 00039 const unsigned has_skew, 00040 const unsigned no_ext_ref_clock) { 00041 *FL_STCALIB = reload_value | (has_skew<<30) | (no_ext_ref_clock<<31); 00042 } 00043 00044 static inline unsigned fl_systick_get_calibration_reload_value(void) { 00045 return *FL_STCALIB & ((1U<<24)-1); 00046 } 00047 00048 static inline unsigned fl_systick_get_calibration_skew(void) { 00049 return *FL_STCALIB & (1U<<30); 00050 } 00051 00052 static inline unsigned fl_systick_get_calibration_noref(void) { 00053 return *FL_STCALIB & (1U<<31); 00054 } 00055 00056 #endif
Generated on Thu Jul 14 2022 22:04:23 by
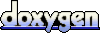