Library to access LPC17xx peripherals. It uses static inline functions, constant propagation and dead code elimination to be as fast as possible.
Dependents: Chua-VGA Wolfram-1D-VGA WolframRnd-1D-VGA Basin-VGA ... more
pwm.h
00001 /* Copyright (C) 2010, 2011 by Ivo van Poorten <ivop@euronet.nl> 00002 * This file is licensed under the terms of the GNU Lesser 00003 * General Public License, version 3. 00004 */ 00005 00006 #ifndef FASTLIB_PWM_H 00007 #define FASTLIB_PWM_H 00008 00009 #include "fastlib/common.h" 00010 00011 #define FL_PWM1IR ((volatile uint32_t *) 0x40018000) 00012 00013 #define FL_PWM1TCR ((volatile uint32_t *) 0x40018004) 00014 #define FL_PWM1TC ((volatile uint32_t *) 0x40018008) 00015 00016 #define FL_PWM1PR ((volatile uint32_t *) 0x4001800C) 00017 #define FL_PWM1PC ((volatile uint32_t *) 0x40018010) 00018 00019 #define FL_PWM1MCR ((volatile uint32_t *) 0x40018014) 00020 #define FL_PWM1MR0 ((volatile uint32_t *) 0x40018018) 00021 #define FL_PWM1MR1 ((volatile uint32_t *) 0x4001801C) 00022 #define FL_PWM1MR2 ((volatile uint32_t *) 0x40018020) 00023 #define FL_PWM1MR3 ((volatile uint32_t *) 0x40018024) 00024 #define FL_PWM1MR4 ((volatile uint32_t *) 0x40018040) 00025 #define FL_PWM1MR5 ((volatile uint32_t *) 0x40018044) 00026 #define FL_PWM1MR6 ((volatile uint32_t *) 0x40018048) 00027 00028 #define FL_PWM1CCR ((volatile uint32_t *) 0x40018028) 00029 #define FL_PWM1CR0 ((volatile uint32_t *) 0x4001802C) 00030 #define FL_PWM1CR1 ((volatile uint32_t *) 0x40018030) 00031 #define FL_PWM1CR2 ((volatile uint32_t *) 0x40018034) 00032 #define FL_PWM1CR3 ((volatile uint32_t *) 0x40018038) 00033 00034 #define FL_PWM1PCR ((volatile uint32_t *) 0x4001804C) 00035 #define FL_PWM1LER ((volatile uint32_t *) 0x40018050) 00036 #define FL_PWM1CTCR ((volatile uint32_t *) 0x40018070) 00037 00038 /* match channel: 0-8 (0-6 pwm, 7-8 cap0-1) 00039 * returns 0 or !0 00040 */ 00041 static inline int fl_pwm_interrupt_status(const unsigned channel) { 00042 int remap; 00043 if (channel >= 7) remap = -3; 00044 else if (channel >= 4) remap = 4; 00045 return *FL_PWM1IR & (1U << (channel+remap)); 00046 } 00047 00048 static inline void fl_pwm_interrupt_clear(const unsigned channel) { 00049 int remap; 00050 if (channel >= 7) remap = -3; 00051 else if (channel >= 4) remap = 4; 00052 *FL_PWM1IR = 1U << (channel+remap); 00053 } 00054 00055 static inline void fl_pwm_timer_counter_enable(const unsigned state) { 00056 if (state) *FL_PWM1TCR |= 1; 00057 else *FL_PWM1TCR &= ~1U; 00058 } 00059 00060 static inline void fl_pwm_timer_counter_reset(const unsigned state) { 00061 if (state) *FL_PWM1TCR |= 2; 00062 else *FL_PWM1TCR &= ~2U; 00063 } 00064 00065 static inline void fl_pwm_enable(const unsigned state) { 00066 if (state) *FL_PWM1TCR |= 8; 00067 else *FL_PWM1TCR &= ~8U; 00068 } 00069 00070 static inline unsigned fl_pwm_get_timer_counter(void) { 00071 return *FL_PWM1TC; 00072 } 00073 00074 static inline void fl_pwm_set_timer_counter(unsigned value) { 00075 *FL_PWM1TC = value; 00076 } 00077 00078 /* value: 1-... */ 00079 static inline void fl_pwm_set_prescale(const unsigned value) { 00080 *FL_PWM1PR = value - 1; 00081 } 00082 00083 static inline unsigned fl_pwm_get_prescale_counter(void) { 00084 return *FL_PWM1PR + 1; 00085 } 00086 00087 static inline void fl_pwm_set_timer_mode(void) { 00088 *FL_PWM1CTCR &= ~3U; 00089 } 00090 00091 /* mode: 1-3 (FL_RISE/FALL/BOTH) pcap1: 0-1 */ 00092 static inline void fl_pwm_set_counter_mode(const unsigned mode, const unsigned pcap1n) { 00093 *FL_PWM1CTCR = mode | (pcap1n << 2); 00094 } 00095 00096 /* channel: 0-6 */ 00097 static inline void fl_pwm_config_match(const unsigned channel, const unsigned interrupt, const unsigned reset, const unsigned stop) { 00098 const unsigned mask = (interrupt | (reset << 1) | (stop << 2)) << ((channel<<1)+channel); 00099 *FL_PWM1MCR &= ~mask; 00100 *FL_PWM1MCR |= mask; 00101 } 00102 00103 /* channel: 0-6 */ 00104 static inline void fl_pwm_set_match(const unsigned channel, const unsigned value) { 00105 if (channel <= 3) *(FL_PWM1MR0+channel) = value; 00106 else *(FL_PWM1MR0+channel+0x18) = value; 00107 } 00108 00109 /* pcap1n: 0-1 */ 00110 static inline void fl_pwm_config_capture(const unsigned pcap1n, const unsigned rise, const unsigned fall, const unsigned interrupt) { 00111 const unsigned mask = (rise | (fall << 1) | (interrupt << 2)) << ((pcap1n<<1)+pcap1n); 00112 *FL_PWM1CCR &= ~mask; 00113 *FL_PWM1CCR |= mask; 00114 } 00115 00116 /* pcap1n: 0-1 (2,3?) */ 00117 static inline unsigned fl_pwm_get_capture(const unsigned pcap1n) { 00118 return *(FL_PWM1CR0+pcap1n); 00119 } 00120 00121 /* channel: 2-6 edges: 0-1 (single/double) */ 00122 static inline void fl_pwm_config_edges(const unsigned channel, const unsigned edges) { 00123 if (edges) *FL_PWM1PCR |= edges << channel ; 00124 else *FL_PWM1PCR &= ~(edges << channel); 00125 } 00126 00127 /* channel: 1-6 state: 0-1 */ 00128 static inline void fl_pwm_output_enable(const unsigned channel, const unsigned state) { 00129 if (state) *FL_PWM1PCR |= 1U << (channel+8) ; 00130 else *FL_PWM1PCR &= ~(1U << (channel+8)); 00131 } 00132 00133 static inline void fl_pwm_latch_match_channel(const unsigned channel) { 00134 *FL_PWM1LER |= 1U << channel; 00135 } 00136 00137 static inline void fl_pwm_latch_match_mask(const unsigned mask) { 00138 *FL_PWM1LER = mask; 00139 } 00140 00141 static inline void fl_pwm_latch_match_all(void) { 00142 fl_pwm_latch_match_mask(0x7f); 00143 } 00144 00145 #endif
Generated on Thu Jul 14 2022 22:04:23 by
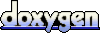