Library to access LPC17xx peripherals. It uses static inline functions, constant propagation and dead code elimination to be as fast as possible.
Dependents: Chua-VGA Wolfram-1D-VGA WolframRnd-1D-VGA Basin-VGA ... more
power.h
00001 /* Copyright (C) 2010, 2011 by Ivo van Poorten <ivop@euronet.nl> 00002 * This file is licensed under the terms of the GNU Lesser 00003 * General Public License, version 3. 00004 */ 00005 00006 /* see chapter 4 of user manual for details on clocks and power */ 00007 00008 #ifndef FASTLIB_POWER_H 00009 #define FASTLIB_POWER_H 00010 00011 #include "fastlib/common.h" 00012 00013 #define FL_PCON ((volatile uint32_t *) 0x400FC0C0) 00014 #define FL_PCONP ((volatile uint32_t *) 0x400FC0C4) 00015 00016 #define FL_POWER_DOWN (1<<0) 00017 #define FL_DEEP_POWER_DOWN (1<<1) 00018 #define FL_BROWN_OUT_REDUCED_POWER_MODE (1<<2) 00019 #define FL_BROWN_OUT_GLOBAL_DISABLE (1<<3) 00020 #define FL_BROWN_OUT_RESET_DISABLE (1<<4) 00021 00022 #define FL_FLAG_SLEEP_MODE (1<<8) 00023 #define FL_FLAG_DEEP_SLEEP_MODE (1<<9) 00024 #define FL_FLAG_POWER_DOWN (1<<10) 00025 #define FL_FLAG_DEEP_POWER_DOWN (1<<11) 00026 00027 static inline void fl_set_power_mode(const unsigned mode) { 00028 *FL_PCON = mode; 00029 } 00030 00031 static inline unsigned fl_get_power_mode(void) { 00032 return *FL_PCON; 00033 } 00034 00035 #define FL_POWER(peripheral, pos) \ 00036 static inline void fl_power_##peripheral(const unsigned on_off) { \ 00037 *FL_PCONP &= ~( 1U<<pos); \ 00038 *FL_PCONP |= (unsigned)on_off<<pos; \ 00039 } 00040 00041 FL_POWER(timer0, 1) 00042 FL_POWER(timer1, 2) 00043 FL_POWER(uart0, 3) 00044 FL_POWER(uart1, 4) 00045 FL_POWER(pwm1, 6) 00046 FL_POWER(i2c0, 7) 00047 FL_POWER(spi, 8) 00048 FL_POWER(rtc, 9) 00049 FL_POWER(ssp1, 10) 00050 FL_POWER(adc, 12) 00051 FL_POWER(can1, 13) 00052 FL_POWER(can2, 14) 00053 FL_POWER(gpio, 15) 00054 FL_POWER(rit, 16) 00055 FL_POWER(mcpwm, 17) 00056 FL_POWER(qei, 18) 00057 FL_POWER(i2c1, 19) 00058 FL_POWER(ssp0, 21) 00059 FL_POWER(timer2, 22) 00060 FL_POWER(timer3, 23) 00061 FL_POWER(uart2, 24) 00062 FL_POWER(uart3, 25) 00063 FL_POWER(i2c2, 26) 00064 FL_POWER(i2s, 27) 00065 FL_POWER(gpdma, 29) 00066 FL_POWER(enet, 30) 00067 FL_POWER(usb, 31) 00068 00069 static inline void fl_power_off_all_peripherals(void) { 00070 *FL_PCONP = 0; 00071 } 00072 00073 static inline void fl_power_on_all_peripherals(void) { 00074 *FL_PCONP = ~((1<<0) | (1<<5) | (1<<11) | (1<<20) | (1<<28)); 00075 } 00076 00077 #endif
Generated on Thu Jul 14 2022 22:04:23 by
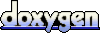