Library to access LPC17xx peripherals. It uses static inline functions, constant propagation and dead code elimination to be as fast as possible.
Dependents: Chua-VGA Wolfram-1D-VGA WolframRnd-1D-VGA Basin-VGA ... more
pinsel.h
00001 /* Copyright (C) 2010, 2011 by Ivo van Poorten <ivop@euronet.nl> 00002 * This file is licensed under the terms of the GNU Lesser 00003 * General Public License, version 3. 00004 */ 00005 00006 #ifndef FASTLIB_PINSEL_H 00007 #define FASTLIB_PINSEL_H 00008 00009 #include "fastlib/common.h" 00010 00011 #define FL_PINSEL0 ((volatile uint32_t *) 0x4002c000) 00012 #define FL_PINMODE0 ((volatile uint32_t *) 0x4002c040) 00013 #define FL_PINMODE_OD0 ((volatile uint32_t *) 0x4002c068) 00014 00015 #define FL_PULLUP 0 00016 #define FL_REPEATER 1 00017 #define FL_TRISTATE 2 00018 #define FL_FLOATING 2 00019 #define FL_PULLDOWN 3 00020 00021 #define FL_FUNC_DEFAULT 0 00022 #define FL_FUNC_ALT1 1 00023 #define FL_FUNC_ALT2 2 00024 #define FL_FUNC_ALT3 3 00025 00026 /* fl_pinsel(port,pin,func,resistor,opendrain) : 00027 * 00028 * port 0-4 00029 * pin 0-31 00030 * func 0-3 (-1 is ignore) default/alt1/alt2/alt3 00031 * resistor 0-3 (-1 is ignore) pull-up, repeater, tri-state, pull-down 00032 * opendrain 0-1 (-1 is ignore) off, on 00033 */ 00034 00035 static inline void fl_pinsel(unsigned port, unsigned pin, const int func, const int resistor, const int opendrain) { 00036 if (opendrain >= 0) { 00037 *(FL_PINMODE_OD0 + port) &= ~( 1U << pin); 00038 *(FL_PINMODE_OD0 + port) |= opendrain << pin ; 00039 } 00040 00041 if (func == -1 && resistor == -1) return; 00042 00043 port <<= 1; 00044 if (pin >= 16) { 00045 pin -= 16; 00046 port++; 00047 } 00048 pin <<= 1; 00049 00050 if (func >= 0) { 00051 *(FL_PINSEL0 + port) &= ~( 3U << pin); 00052 *(FL_PINSEL0 + port) |= func << pin ; 00053 } 00054 if (resistor >= 0) { 00055 *(FL_PINMODE0 + port) &= ~((uint32_t) 3U << pin); 00056 *(FL_PINMODE0 + port) |= (uint32_t)resistor << pin ; 00057 } 00058 } 00059 00060 /* Perhaps move this to i2c.h later??? */ 00061 00062 #define FL_I2CPADCFG ((volatile uint32_t *) 0x4002C07C) 00063 00064 #define FL_SDA0_STANDARD_DRIVE (0<<0) 00065 #define FL_SDA0_FAST_DRIVE (1<<0) 00066 #define FL_SDA0_GLITCH_ENABLE (0<<1) 00067 #define FL_SDA0_GLITCH_DISABLE (1<<1) 00068 #define FL_SCL0_STANDARD_DRIVE (0<<2) 00069 #define FL_SCL0_FAST_DRIVE (1<<2) 00070 #define FL_SCL0_GLITCH_ENABLE (0<<3) 00071 #define FL_SCL0_GLITCH_DISABLE (1<<3) 00072 00073 /* pins P0.27 and P0.28 */ 00074 static inline void fl_i2c_pad_config(const unsigned config) { 00075 *FL_I2CPADCFG = config; 00076 } 00077 00078 #endif
Generated on Thu Jul 14 2022 22:04:23 by
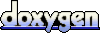