Library to access LPC17xx peripherals. It uses static inline functions, constant propagation and dead code elimination to be as fast as possible.
Dependents: Chua-VGA Wolfram-1D-VGA WolframRnd-1D-VGA Basin-VGA ... more
nvic.h
00001 /* Copyright (C) 2011 by Ivo van Poorten <ivop@euronet.nl> 00002 * This file is licensed under the terms of the GNU Lesser 00003 * General Public License, version 3. 00004 */ 00005 00006 #ifndef FASTLIB_NVIC_H 00007 #define FASTLIB_NVIC_H 00008 00009 #include "fastlib/common.h" 00010 00011 #define FL_NVIC_ISER0 ((volatile uint32_t *) 0xE000E100) 00012 #define FL_NVIC_ISER1 ((volatile uint32_t *) 0xE000E104) 00013 #define FL_NVIC_ICER0 ((volatile uint32_t *) 0xE000E180) 00014 #define FL_NVIC_ICER1 ((volatile uint32_t *) 0xE000E184) 00015 #define FL_NVIC_ISPR0 ((volatile uint32_t *) 0xE000E200) 00016 #define FL_NVIC_ISPR1 ((volatile uint32_t *) 0xE000E204) 00017 #define FL_NVIC_ICPR0 ((volatile uint32_t *) 0xE000E280) 00018 #define FL_NVIC_ICPR1 ((volatile uint32_t *) 0xE000E284) 00019 #define FL_NVIC_IABR0 ((volatile uint32_t *) 0xE000E300) 00020 #define FL_NVIC_IABR1 ((volatile uint32_t *) 0xE000E304) 00021 #define FL_NVIC_IPR0 ((volatile uint32_t *) 0xE000E400) 00022 #define FL_NVIC_IPR1 ((volatile uint32_t *) 0xE000E404) 00023 #define FL_NVIC_IPR2 ((volatile uint32_t *) 0xE000E408) 00024 #define FL_NVIC_IPR3 ((volatile uint32_t *) 0xE000E40C) 00025 #define FL_NVIC_IPR4 ((volatile uint32_t *) 0xE000E410) 00026 #define FL_NVIC_IPR5 ((volatile uint32_t *) 0xE000E414) 00027 #define FL_NVIC_IPR6 ((volatile uint32_t *) 0xE000E418) 00028 #define FL_NVIC_IPR7 ((volatile uint32_t *) 0xE000E41C) 00029 #define FL_NVIC_IPR8 ((volatile uint32_t *) 0xE000E420) 00030 #define FL_NVIC_STIR ((volatile uint32_t *) 0xE000EF00) 00031 00032 #define FL_NVIC_INT_WDT 0 00033 #define FL_NVIC_INT_TIMER0 1 00034 #define FL_NVIC_INT_TIMER1 2 00035 #define FL_NVIC_INT_TIMER2 3 00036 #define FL_NVIC_INT_TIMER3 4 00037 #define FL_NVIC_INT_UART0 5 00038 #define FL_NVIC_INT_UART1 6 00039 #define FL_NVIC_INT_UART2 7 00040 #define FL_NVIC_INT_UART3 8 00041 #define FL_NVIC_INT_PWM 9 00042 #define FL_NVIC_INT_I2C0 10 00043 #define FL_NVIC_INT_I2C1 11 00044 #define FL_NVIC_INT_I2C2 12 00045 #define FL_NVIC_INT_SPI 13 00046 #define FL_NVIC_INT_SSP0 14 00047 #define FL_NVIC_INT_SSP1 15 00048 #define FL_NVIC_INT_PLL0 16 00049 #define FL_NVIC_INT_RTC 17 00050 #define FL_NVIC_INT_EINT0 18 00051 #define FL_NVIC_INT_EINT1 19 00052 #define FL_NVIC_INT_EINT2 20 00053 #define FL_NVIC_INT_EINT3 21 00054 #define FL_NVIC_INT_ADC 22 00055 #define FL_NVIC_INT_BOD 23 00056 #define FL_NVIC_INT_USB 24 00057 #define FL_NVIC_INT_CAN 25 00058 #define FL_NVIC_INT_DMA 26 00059 #define FL_NVIC_INT_I2S 27 00060 #define FL_NVIC_INT_ENET 28 00061 #define FL_NVIC_INT_RIT 29 00062 #define FL_NVIC_INT_MCPWM 30 00063 #define FL_NVIC_INT_QEI 31 00064 00065 #define FL_NVIC_INT_PLL1 32 00066 #define FL_NVIC_INT_USBACT 33 00067 #define FL_NVIC_INT_CANACT 34 00068 00069 #define FL_NVIC_INTERRUPT_FUNC(x, y) \ 00070 static inline void fl_nvic_interrupt_##x(const unsigned interrupt) { \ 00071 if (interrupt < 32) *FL_NVIC_##y##0 |= 1U<<interrupt; \ 00072 else *FL_NVIC_##y##1 |= 1U<<(interrupt-32); \ 00073 } \ 00074 static inline unsigned fl_nvic_interrupt_##x##_status(const unsigned interrupt) { \ 00075 if (interrupt < 32) return (*FL_NVIC_##y##0) & (1U<<interrupt); \ 00076 else return (*FL_NVIC_##y##1) & (1U<<(interrupt-32)); \ 00077 } 00078 00079 FL_NVIC_INTERRUPT_FUNC(set_enable, ISER) 00080 FL_NVIC_INTERRUPT_FUNC(clear_enable, ICER) 00081 FL_NVIC_INTERRUPT_FUNC(set_pending, ISPR) 00082 FL_NVIC_INTERRUPT_FUNC(clear_pending, ICPR) 00083 00084 static inline unsigned fl_nvic_interrupt_active_bit_status(const unsigned interrupt) { 00085 if (interrupt < 32) return (*FL_NVIC_IABR0) & (1U<<interrupt); 00086 else return (*FL_NVIC_IABR1) & (1U<<(interrupt-32)); 00087 } 00088 00089 static inline void fl_nvic_interrupt_set_priority(const unsigned interrupt, const unsigned priority) { 00090 const unsigned i = interrupt >> 3, 00091 j = (31 <<3) << ((interrupt&3)<<3), 00092 k = (priority<<3) << ((interrupt&3)<<3); 00093 *(FL_NVIC_IPR0 + i) &= ~j; 00094 *(FL_NVIC_IPR0 + i) |= k; 00095 } 00096 00097 static inline void fl_nvic_interrupt_software_trigger(const unsigned interrupt) { 00098 *FL_NVIC_STIR = interrupt; 00099 } 00100 00101 #endif
Generated on Thu Jul 14 2022 22:04:23 by
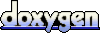