Library to access LPC17xx peripherals. It uses static inline functions, constant propagation and dead code elimination to be as fast as possible.
Dependents: Chua-VGA Wolfram-1D-VGA WolframRnd-1D-VGA Basin-VGA ... more
clock.h
00001 /* Copyright (C) 2010, 2011 by Ivo van Poorten <ivop@euronet.nl> 00002 * This file is licensed under the terms of the GNU Lesser 00003 * General Public License, version 3. 00004 */ 00005 00006 /* see chapter 4 of user manual for details on clocks and power */ 00007 00008 #ifndef FASTLIB_CLKPWR_H 00009 #define FASTLIB_CLKPWR_H 00010 00011 #include "fastlib/common.h" 00012 00013 #define FL_CLKSRCSEL ((volatile uint32_t *) 0x400FC10C) 00014 00015 #define FL_PLL0CON ((volatile uint32_t *) 0x400FC080) 00016 #define FL_PLL0CFG ((volatile uint32_t *) 0x400FC084) 00017 #define FL_PLL0STAT ((volatile uint32_t *) 0x400FC088) 00018 #define FL_PLL0FEED ((volatile uint32_t *) 0x400FC08C) 00019 00020 #define FL_PLL1CON ((volatile uint32_t *) 0x400FC0A0) 00021 #define FL_PLL1CFG ((volatile uint32_t *) 0x400FC0A4) 00022 #define FL_PLL1STAT ((volatile uint32_t *) 0x400FC0A8) 00023 #define FL_PLL1FEED ((volatile uint32_t *) 0x400FC0AC) 00024 00025 #define FL_CCLKCFG ((volatile uint32_t *) 0x400FC104) 00026 #define FL_USBCLKCFG ((volatile uint32_t *) 0x400FC108) 00027 #define FL_PCLKSEL0 ((volatile uint32_t *) 0x400FC1A8) 00028 #define FL_PCLKSEL1 ((volatile uint32_t *) 0x400FC1AC) 00029 00030 #define FL_CLKOUTCFG ((volatile uint32_t *) 0x400FC1C8) 00031 00032 /* Internal RC oscillator, nominal frequency is 4MHz 00033 * Main oscillator --> mbed: 12MHz slave mode 00034 * RTC oscillator --> mbed: FC-135 32.7680kHz 12.5pF 00035 */ 00036 00037 /* for USB, use only Main oscillator 00038 * for CAN over 100 kbit/s, do not use Internal RC oscillator 00039 */ 00040 00041 #define FL_PLL0_CLOCK_SOURCE_INTERNAL 0 00042 #define FL_PLL0_CLOCK_SOURCE_MAIN 1 00043 #define FL_PLL0_CLOCK_SOURCE_RTC 2 00044 00045 static inline void fl_select_pll0_clock_source(const int source) { 00046 *FL_CLKSRCSEL = source; 00047 } 00048 00049 /* PLL0: 00050 * input source --> PLL input divider (N) --> PLL multiplier (M) 00051 * 00052 * input: 32kHz-50MHz --> 00053 * N: 1-256 --> 00054 * M: 6-512 --> 275MHz-550MHz 00055 * 00056 * PLL0 output dividers below 00057 */ 00058 00059 static inline void fl_pll0_control(const int enable, const int connect) { 00060 *FL_PLL0CON = enable | (connect<<1); 00061 } 00062 00063 static inline void fl_pll0_config(const unsigned int N, const unsigned int M) { 00064 *FL_PLL0CFG = (M-1) | ((N-1)<<16); 00065 } 00066 00067 static inline unsigned fl_pll0_get_M(void) { 00068 return (*FL_PLL0STAT & 0x7fff) + 1; 00069 } 00070 00071 static inline unsigned fl_pll0_get_N(void) { 00072 return ((*FL_PLL0STAT >> 16) & 0xff) + 1; 00073 } 00074 00075 static inline int fl_pll0_get_enable(void) { 00076 return *FL_PLL0STAT & (1U<<24); 00077 } 00078 00079 static inline int fl_pll0_get_connect(void) { 00080 return *FL_PLL0STAT & (1U<<25); 00081 } 00082 00083 static inline int fl_pll0_get_lock(void) { 00084 return *FL_PLL0STAT & (1U<<26); 00085 } 00086 00087 static inline void fl_pll0_feed(void) { 00088 *FL_PLL0FEED = 0xaa; 00089 *FL_PLL0FEED = 0x55; 00090 } 00091 00092 /* PLL1: 00093 * input main oscillator --> PLL multiplier (M) --> output divider (2P) 00094 */ 00095 00096 static inline void fl_pll1_control(const int enable, const int connect) { 00097 *FL_PLL1CON = enable | (connect<<1); 00098 } 00099 00100 static inline void fl_pll1_config(const unsigned int M, const unsigned int P) { 00101 *FL_PLL1CFG = M | (P<<5); 00102 } 00103 00104 static inline int fl_pll1_get_M(void) { 00105 return *FL_PLL1STAT & 0x1f; 00106 } 00107 00108 static inline int fl_pll1_get_P(void) { 00109 return (*FL_PLL1STAT >> 5) & 3; 00110 } 00111 00112 static inline int fl_pll1_get_enable(void) { 00113 return *FL_PLL1STAT & (1U<<8); 00114 } 00115 00116 static inline int fl_pll1_get_connect(void) { 00117 return *FL_PLL1STAT & (1U<<9); 00118 } 00119 00120 static inline int fl_pll1_get_lock(void) { 00121 return *FL_PLL1STAT & (1U<<10); 00122 } 00123 00124 static inline void fl_pll1_feed(void) { 00125 *FL_PLL1FEED = 0xaa; 00126 *FL_PLL1FEED = 0x55; 00127 } 00128 00129 /* PLL0 output --> CPU Clock */ 00130 00131 /* CPU clock must be at least 18MHz for USB to function */ 00132 00133 /* div: 3-256 */ 00134 static inline void fl_set_cpu_clock_divider(const int div) { 00135 *FL_CCLKCFG = div - 1; 00136 } 00137 00138 static inline int fl_get_cpu_clock_divider(void) { 00139 return *FL_CCLKCFG + 1; 00140 } 00141 00142 /* use only if USBPLL/PLL1 is not connected */ 00143 /* div: 1-16 (only 6, 8 and 10 produce even number multiples */ 00144 static inline void fl_set_pll0_usb_divider(const unsigned int div) { 00145 *FL_USBCLKCFG = div - 1; 00146 } 00147 00148 static inline int fl_get_pll0_usb_divider(void) { 00149 return *FL_USBCLKCFG + 1; 00150 } 00151 00152 #define FL_CLOCK_DIV4 0 00153 #define FL_CLOCK_DIV1 1 00154 #define FL_CLOCK_DIV2 2 00155 #define FL_CLOCK_DIV8 3 00156 00157 #define FL_SELECT_CLOCK(peripheral, reg, pos) \ 00158 static inline void fl_select_clock_##peripheral(const unsigned int clock) { \ 00159 *reg &= ~(3U << pos); \ 00160 *reg |= clock << pos; \ 00161 } 00162 00163 FL_SELECT_CLOCK(wdt, FL_PCLKSEL0, 0) 00164 FL_SELECT_CLOCK(timer0, FL_PCLKSEL0, 2) 00165 FL_SELECT_CLOCK(timer1, FL_PCLKSEL0, 4) 00166 FL_SELECT_CLOCK(uart0, FL_PCLKSEL0, 6) 00167 FL_SELECT_CLOCK(uart1, FL_PCLKSEL0, 8) 00168 FL_SELECT_CLOCK(pwm1, FL_PCLKSEL0, 12) 00169 FL_SELECT_CLOCK(i2c0, FL_PCLKSEL0, 14) 00170 FL_SELECT_CLOCK(spi, FL_PCLKSEL0, 16) 00171 FL_SELECT_CLOCK(ssp1, FL_PCLKSEL0, 20) 00172 FL_SELECT_CLOCK(dac, FL_PCLKSEL0, 22) 00173 FL_SELECT_CLOCK(adc, FL_PCLKSEL0, 24) 00174 FL_SELECT_CLOCK(can1, FL_PCLKSEL0, 26) 00175 FL_SELECT_CLOCK(can2, FL_PCLKSEL0, 28) 00176 FL_SELECT_CLOCK(acf, FL_PCLKSEL0, 30) 00177 00178 FL_SELECT_CLOCK(qei, FL_PCLKSEL1, 0) 00179 FL_SELECT_CLOCK(gpioint, FL_PCLKSEL1, 2) 00180 FL_SELECT_CLOCK(pcb, FL_PCLKSEL1, 4) 00181 FL_SELECT_CLOCK(i2c1, FL_PCLKSEL1, 6) 00182 FL_SELECT_CLOCK(ssp0, FL_PCLKSEL1, 10) 00183 FL_SELECT_CLOCK(timer2, FL_PCLKSEL1, 12) 00184 FL_SELECT_CLOCK(timer3, FL_PCLKSEL1, 14) 00185 FL_SELECT_CLOCK(uart2, FL_PCLKSEL1, 16) 00186 FL_SELECT_CLOCK(uart3, FL_PCLKSEL1, 18) 00187 FL_SELECT_CLOCK(i2c2, FL_PCLKSEL1, 20) 00188 FL_SELECT_CLOCK(i2s, FL_PCLKSEL1, 22) 00189 FL_SELECT_CLOCK(rit, FL_PCLKSEL1, 26) 00190 FL_SELECT_CLOCK(syscon, FL_PCLKSEL1, 28) 00191 FL_SELECT_CLOCK(mcpwm, FL_PCLKSEL1, 30) 00192 00193 #define FL_CLOCK_OUT_CPU 0 00194 #define FL_CLOCK_OUT_MAIN 1 00195 #define FL_CLOCK_OUT_IRC 2 00196 #define FL_CLOCK_OUT_USB 3 00197 #define FL_CLOCK_OUT_RTC 4 00198 00199 static inline void fl_set_output_clock(const int clock) { 00200 *FL_CLKOUTCFG = clock; 00201 } 00202 00203 #endif
Generated on Thu Jul 14 2022 22:04:23 by
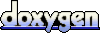