
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
OptionsBuilder Class Reference
OptionsBuilder class. More...
#include <OptionsBuilder.h>
Inherits Connector::Options.
Public Member Functions | |
OptionsBuilder () | |
Default Constructor. | |
OptionsBuilder (const OptionsBuilder &ob) | |
Copy Constructor. | |
virtual | ~OptionsBuilder () |
Destructor. | |
OptionsBuilder & | setLifetime (int lifetime) |
Set the NSDL Endpoint Lifetime. | |
OptionsBuilder & | setDomain (const char *domain) |
Set the NSDL Domain. | |
OptionsBuilder & | setEndpointNodename (const char *node_name) |
Set the Endpoint Node Name. | |
OptionsBuilder & | setEndpointType (const char *endpoint_type) |
Set the NSDL Endpoint Type. | |
OptionsBuilder & | setConnectorURL (const char *connector_url) |
Set the Connector URL. | |
OptionsBuilder & | setDeviceResourcesObject (const void *device_resources_object) |
Set the LWM2M Device Resource Object Instance. | |
OptionsBuilder & | setFirmwareResourcesObject (const void *firmware_resources_object) |
Set the LWM2M Firmware Resource Object Instance. | |
OptionsBuilder & | addResource (const StaticResource *static_resource) |
Add a NSDL endpoint resource (static) | |
OptionsBuilder & | addResource (const DynamicResource *dynamic_resource) |
Add a NSDL endpoint resource (dynamic) | |
OptionsBuilder & | addResource (const DynamicResource *dynamic_resource, const bool use_observer) |
Add a NSDL endpoint resource (dynamic) | |
OptionsBuilder & | addResource (const DynamicResource *dynamic_resource, const int sleep_time) |
Add a NSDL endpoint resource (dynamic) | |
OptionsBuilder & | addResource (const DynamicResource *dynamic_resource, const int sleep_time, const bool use_observer) |
Add a NSDL endpoint resource (dynamic) | |
OptionsBuilder & | setWiFiSSID (char *ssid) |
Set the WiFi SSID. | |
OptionsBuilder & | setWiFiAuthType (WiFiAuthTypes auth_type) |
Set the WiFi AuthType. | |
OptionsBuilder & | setWiFiAuthKey (char *auth_key) |
Set the WiFi AuthKey. | |
OptionsBuilder & | setCoAPConnectionType (CoAPConnectionTypes coap_connection_type) |
Set the CoAP Connection Type. | |
OptionsBuilder & | setIPAddressType (IPAddressTypes ip_address_type) |
Set the IP Address Type. | |
OptionsBuilder & | setImmedateObservationEnabled (bool enable) |
Enable/Disable immediate observationing. | |
OptionsBuilder & | setEnableGETObservationControl (bool enable) |
Enable/Disable GET-based control of observations. | |
OptionsBuilder & | setServerCertificate (uint8_t *cert, int cert_size) |
Set the Server Certificate. | |
OptionsBuilder & | setClientCertificate (uint8_t *cert, int cert_size) |
Set the Client Certificate. | |
OptionsBuilder & | setClientKey (uint8_t *key, int key_size) |
Set the Client Key. | |
Options * | build () |
Build our our immutable self. | |
void | setEndpoint (void *endpoint) |
Set our Endpoint instance. | |
Private Member Functions | |
int | getLifetime () |
Get the node lifetime. | |
string | getDomain () |
Get the NSP domain. | |
string | getEndpointNodename () |
Get the node name. | |
string | getEndpointType () |
Get the node type. | |
char * | getConnectorURL () |
Get the endpoint Connector URL. | |
uint16_t | getConnectorPort () |
Get the connector connection port from the URL. | |
void * | getDeviceResourcesObject () |
Get the Device Resources Object Instance. | |
void * | getFirmwareResourcesObject () |
Get the Firmware Resources Object Instance. | |
StaticResourcesList * | getStaticResourceList () |
Get the list of static resources. | |
DynamicResourcesList * | getDynamicResourceList () |
Get the list of dynamic resources. | |
string | getWiFiSSID () |
Get the WiFi SSID. | |
WiFiAuthTypes | getWiFiAuthType () |
Get the WiFi Auth Type. | |
string | getWiFiAuthKey () |
Get the WiFi Auth Key. | |
CoAPConnectionTypes | getCoAPConnectionType () |
Get the CoAP Connection Type. | |
IPAddressTypes | getIPAddressType () |
Get the IP Address Type. | |
bool | immedateObservationEnabled () |
Enable/Disable Immediate Observationing. | |
bool | enableGETObservationControl () |
Enable/Disable Observation control via GET. | |
uint8_t * | getServerCertificate () |
Get the Server Certificate. | |
int | getServerCertificateSize () |
Get the Server Certificate Size (bytes) | |
uint8_t * | getClientCertificate () |
Get the Client Certificate. | |
int | getClientCertificateSize () |
Get the Client Certificate Size (bytes) | |
uint8_t * | getClientKey () |
Get the Client Key. | |
int | getClientKeySize () |
Get the Client Key Size (bytes) | |
void * | getEndpoint () |
Get Our Endpoint. |
Detailed Description
OptionsBuilder class.
Definition at line 34 of file OptionsBuilder.h.
Constructor & Destructor Documentation
OptionsBuilder | ( | ) |
Default Constructor.
Definition at line 35 of file OptionsBuilder.cpp.
OptionsBuilder | ( | const OptionsBuilder & | ob ) |
Copy Constructor.
- Parameters:
-
ob input options builder instance to deep copy
Definition at line 57 of file OptionsBuilder.cpp.
~OptionsBuilder | ( | ) | [virtual] |
Destructor.
Definition at line 87 of file OptionsBuilder.cpp.
Member Function Documentation
OptionsBuilder & addResource | ( | const StaticResource * | static_resource ) |
Add a NSDL endpoint resource (static)
- Parameters:
-
static_resource input the NSDL static resource
- Returns:
- instance to ourself
Definition at line 152 of file OptionsBuilder.cpp.
OptionsBuilder & addResource | ( | const DynamicResource * | dynamic_resource ) |
Add a NSDL endpoint resource (dynamic)
- Parameters:
-
dynamic_resource input the NSDL dynamic resource
- Returns:
- instance to ourself
Definition at line 162 of file OptionsBuilder.cpp.
OptionsBuilder & addResource | ( | const DynamicResource * | dynamic_resource, |
const bool | use_observer | ||
) |
Add a NSDL endpoint resource (dynamic)
- Parameters:
-
dynamic_resource input the NSDL dynamic resource use_observer input if true, use an appropriate ResourceObserver to observer. if false, the underlying resource will handle it
- Returns:
- instance to ourself
Definition at line 176 of file OptionsBuilder.cpp.
OptionsBuilder & addResource | ( | const DynamicResource * | dynamic_resource, |
const int | sleep_time | ||
) |
Add a NSDL endpoint resource (dynamic)
- Parameters:
-
dynamic_resource input the NSDL dynamic resource sleep_time input the observation sleep time in milliseconds (for observable resource only)
- Returns:
- instance to ourself
Definition at line 169 of file OptionsBuilder.cpp.
OptionsBuilder & addResource | ( | const DynamicResource * | dynamic_resource, |
const int | sleep_time, | ||
const bool | use_observer | ||
) |
Add a NSDL endpoint resource (dynamic)
- Parameters:
-
dynamic_resource input the NSDL dynamic resource sleep_time input the observation sleep time in milliseconds (for observable resource only) use_observer input if true, use an appropriate ResourceObserver to observer. if false, the underlying resource will handle it
- Returns:
- instance to ourself
Definition at line 183 of file OptionsBuilder.cpp.
Options * build | ( | ) |
Build our our immutable self.
Definition at line 252 of file OptionsBuilder.cpp.
OptionsBuilder & setClientCertificate | ( | uint8_t * | cert, |
int | cert_size | ||
) |
Set the Client Certificate.
- Parameters:
-
cert input the client certificate cert_size input the length of the client certificate
Definition at line 277 of file OptionsBuilder.cpp.
OptionsBuilder & setClientKey | ( | uint8_t * | key, |
int | key_size | ||
) |
Set the Client Key.
- Parameters:
-
key input the client key key_size input the length of the client key
Definition at line 284 of file OptionsBuilder.cpp.
OptionsBuilder & setCoAPConnectionType | ( | CoAPConnectionTypes | coap_connection_type ) |
Set the CoAP Connection Type.
- Parameters:
-
coap_connection_type input the CoAP Connection type
Definition at line 238 of file OptionsBuilder.cpp.
OptionsBuilder & setConnectorURL | ( | const char * | connector_url ) |
Set the Connector URL.
- Parameters:
-
url input the Connector URL
- Returns:
- instance to ourself
Definition at line 125 of file OptionsBuilder.cpp.
OptionsBuilder & setDeviceResourcesObject | ( | const void * | device_resources_object ) |
Set the LWM2M Device Resource Object Instance.
- Parameters:
-
device_resources_object input the LWM2M device resource object instance
- Returns:
- instance to ourself
Definition at line 134 of file OptionsBuilder.cpp.
OptionsBuilder & setDomain | ( | const char * | domain ) |
Set the NSDL Domain.
- Parameters:
-
domain input the NSDL domain to set
- Returns:
- instance to ourself
Definition at line 104 of file OptionsBuilder.cpp.
OptionsBuilder & setEnableGETObservationControl | ( | bool | enable ) |
Enable/Disable GET-based control of observations.
- Parameters:
-
enable input enable GET-based observation enable/disable control
Definition at line 264 of file OptionsBuilder.cpp.
void setEndpoint | ( | void * | endpoint ) |
Set our Endpoint instance.
Definition at line 291 of file OptionsBuilder.cpp.
OptionsBuilder & setEndpointNodename | ( | const char * | node_name ) |
Set the Endpoint Node Name.
- Parameters:
-
node_name input the node endpoint name
- Returns:
- instance to ourself
Definition at line 111 of file OptionsBuilder.cpp.
OptionsBuilder & setEndpointType | ( | const char * | endpoint_type ) |
Set the NSDL Endpoint Type.
- Parameters:
-
endpoint_type input the NSDL endpoint type
- Returns:
- instance to ourself
Definition at line 118 of file OptionsBuilder.cpp.
OptionsBuilder & setFirmwareResourcesObject | ( | const void * | firmware_resources_object ) |
Set the LWM2M Firmware Resource Object Instance.
- Parameters:
-
firmware_resources_object input the LWM2M firmware resource object instance
- Returns:
- instance to ourself
Definition at line 143 of file OptionsBuilder.cpp.
OptionsBuilder & setImmedateObservationEnabled | ( | bool | enable ) |
Enable/Disable immediate observationing.
- Parameters:
-
enable input enable immediate observationing without GET
Definition at line 258 of file OptionsBuilder.cpp.
OptionsBuilder & setIPAddressType | ( | IPAddressTypes | ip_address_type ) |
Set the IP Address Type.
- Parameters:
-
ip_address_type input the IP Address type
Definition at line 245 of file OptionsBuilder.cpp.
OptionsBuilder & setLifetime | ( | int | lifetime ) |
Set the NSDL Endpoint Lifetime.
- Parameters:
-
lifetime input the NSDL endpoint lifetime (seconds)
- Returns:
- instance to ourself
Definition at line 97 of file OptionsBuilder.cpp.
OptionsBuilder & setServerCertificate | ( | uint8_t * | cert, |
int | cert_size | ||
) |
Set the Server Certificate.
- Parameters:
-
cert input the server certificate cert_size input the length of the server certificate
Definition at line 270 of file OptionsBuilder.cpp.
OptionsBuilder & setWiFiAuthKey | ( | char * | auth_key ) |
Set the WiFi AuthKey.
- Parameters:
-
auth_key input the WiFi AuthKey
Definition at line 231 of file OptionsBuilder.cpp.
OptionsBuilder & setWiFiAuthType | ( | WiFiAuthTypes | auth_type ) |
Set the WiFi AuthType.
- Parameters:
-
auth_type input the WiFi AuthType
Definition at line 224 of file OptionsBuilder.cpp.
OptionsBuilder & setWiFiSSID | ( | char * | ssid ) |
Set the WiFi SSID.
- Parameters:
-
ssid input the WiFi SSID
Definition at line 217 of file OptionsBuilder.cpp.
Generated on Tue Jul 12 2022 18:38:06 by
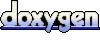