
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
OptionsBuilder.h
00001 /** 00002 * @file OptionsBuilder.h 00003 * @brief mbed CoAP OptionsBuilder class header 00004 * @author Doug Anson/Chris Paola 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __OPTIONS_BUILDER_H__ 00024 #define __OPTIONS_BUILDER_H__ 00025 00026 // base class support 00027 #include "mbed-connector-interface/Options.h" 00028 00029 // Connector namespace 00030 namespace Connector { 00031 00032 /** OptionsBuilder class 00033 */ 00034 class OptionsBuilder : Options 00035 { 00036 public: 00037 /** 00038 Default Constructor 00039 */ 00040 OptionsBuilder(); 00041 00042 /** 00043 Copy Constructor 00044 @param ob input options builder instance to deep copy 00045 */ 00046 OptionsBuilder(const OptionsBuilder &ob); 00047 00048 /** 00049 Destructor 00050 */ 00051 virtual ~OptionsBuilder(); 00052 00053 /** 00054 Set the NSDL Endpoint Lifetime 00055 @param lifetime input the NSDL endpoint lifetime (seconds) 00056 @return instance to ourself 00057 */ 00058 OptionsBuilder &setLifetime(int lifetime); 00059 00060 /** 00061 Set the NSDL Domain 00062 @param domain input the NSDL domain to set 00063 @return instance to ourself 00064 */ 00065 OptionsBuilder &setDomain(const char *domain); 00066 00067 /** 00068 Set the Endpoint Node Name 00069 @param node_name input the node endpoint name 00070 @return instance to ourself 00071 */ 00072 OptionsBuilder &setEndpointNodename(const char *node_name); 00073 00074 /** 00075 Set the NSDL Endpoint Type 00076 @param endpoint_type input the NSDL endpoint type 00077 @return instance to ourself 00078 */ 00079 OptionsBuilder &setEndpointType(const char *endpoint_type); 00080 00081 /** 00082 Set the Connector URL 00083 @param url input the Connector URL 00084 @return instance to ourself 00085 */ 00086 OptionsBuilder &setConnectorURL(const char *connector_url); 00087 00088 /** 00089 Set the LWM2M Device Resource Object Instance 00090 @param device_resources_object input the LWM2M device resource object instance 00091 @return instance to ourself 00092 */ 00093 OptionsBuilder &setDeviceResourcesObject(const void *device_resources_object); 00094 00095 /** 00096 Set the LWM2M Firmware Resource Object Instance 00097 @param firmware_resources_object input the LWM2M firmware resource object instance 00098 @return instance to ourself 00099 */ 00100 OptionsBuilder &setFirmwareResourcesObject(const void *firmware_resources_object); 00101 00102 /** 00103 Add a NSDL endpoint resource (static) 00104 @param static_resource input the NSDL static resource 00105 @return instance to ourself 00106 */ 00107 OptionsBuilder &addResource(const StaticResource *static_resource); 00108 00109 /** 00110 Add a NSDL endpoint resource (dynamic) 00111 @param dynamic_resource input the NSDL dynamic resource 00112 @return instance to ourself 00113 */ 00114 OptionsBuilder &addResource(const DynamicResource *dynamic_resource); 00115 00116 /** 00117 Add a NSDL endpoint resource (dynamic) 00118 @param dynamic_resource input the NSDL dynamic resource 00119 @param use_observer input if true, use an appropriate ResourceObserver to observer. if false, the underlying resource will handle it 00120 @return instance to ourself 00121 */ 00122 OptionsBuilder &addResource(const DynamicResource *dynamic_resource,const bool use_observer); 00123 00124 /** 00125 Add a NSDL endpoint resource (dynamic) 00126 @param dynamic_resource input the NSDL dynamic resource 00127 @param sleep_time input the observation sleep time in milliseconds (for observable resource only) 00128 @return instance to ourself 00129 */ 00130 OptionsBuilder &addResource(const DynamicResource *dynamic_resource,const int sleep_time); 00131 00132 /** 00133 Add a NSDL endpoint resource (dynamic) 00134 @param dynamic_resource input the NSDL dynamic resource 00135 @param sleep_time input the observation sleep time in milliseconds (for observable resource only) 00136 @param use_observer input if true, use an appropriate ResourceObserver to observer. if false, the underlying resource will handle it 00137 @return instance to ourself 00138 */ 00139 OptionsBuilder &addResource(const DynamicResource *dynamic_resource,const int sleep_time,const bool use_observer); 00140 00141 /** 00142 Set the WiFi SSID 00143 @param ssid input the WiFi SSID 00144 */ 00145 OptionsBuilder &setWiFiSSID(char *ssid); 00146 00147 /** 00148 Set the WiFi AuthType 00149 @param auth_type input the WiFi AuthType 00150 */ 00151 OptionsBuilder &setWiFiAuthType(WiFiAuthTypes auth_type); 00152 00153 /** 00154 Set the WiFi AuthKey 00155 @param auth_key input the WiFi AuthKey 00156 */ 00157 OptionsBuilder &setWiFiAuthKey(char *auth_key); 00158 00159 /** 00160 Set the CoAP Connection Type 00161 @param coap_connection_type input the CoAP Connection type 00162 */ 00163 OptionsBuilder &setCoAPConnectionType(CoAPConnectionTypes coap_connection_type); 00164 00165 /** 00166 Set the IP Address Type 00167 @param ip_address_type input the IP Address type 00168 */ 00169 OptionsBuilder &setIPAddressType(IPAddressTypes ip_address_type); 00170 00171 /** 00172 Enable/Disable immediate observationing 00173 @param enable input enable immediate observationing without GET 00174 */ 00175 OptionsBuilder &setImmedateObservationEnabled(bool enable); 00176 00177 /** 00178 Enable/Disable GET-based control of observations 00179 @param enable input enable GET-based observation enable/disable control 00180 */ 00181 OptionsBuilder &setEnableGETObservationControl(bool enable); 00182 00183 /** 00184 Set the Server Certificate 00185 @param cert input the server certificate 00186 @param cert_size input the length of the server certificate 00187 */ 00188 OptionsBuilder &setServerCertificate(uint8_t *cert,int cert_size); 00189 00190 /** 00191 Set the Client Certificate 00192 @param cert input the client certificate 00193 @param cert_size input the length of the client certificate 00194 */ 00195 OptionsBuilder &setClientCertificate(uint8_t *cert,int cert_size); 00196 00197 /** 00198 Set the Client Key 00199 @param key input the client key 00200 @param key_size input the length of the client key 00201 */ 00202 OptionsBuilder &setClientKey(uint8_t *key,int key_size); 00203 00204 /** 00205 Build our our immutable self 00206 */ 00207 Options *build(); 00208 00209 /** 00210 Set our Endpoint instance 00211 */ 00212 void setEndpoint(void *endpoint); 00213 }; 00214 00215 } // namespace Connector 00216 00217 #endif // __OPTIONS_BUILDER_H__
Generated on Tue Jul 12 2022 18:38:05 by
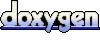