
Origin firmware.
Dependencies: PID QEI SB1602E mbed-rtos mbed
Fork of PreHeater by
main.cpp
00001 /*The MIT License (MIT) 00002 00003 Copyright (c) <2015> <Kazumichi Aoki> 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 00025 00026 #include "mbed.h" 00027 #include "PID.h" 00028 #include "math.h" 00029 #include "SB1602E.h" 00030 #include "QEI.h" 00031 #include "rtos.h" 00032 //GPIO initilaize 00033 AnalogIn THAI(dp4); 00034 PwmOut out(dp1); 00035 DigitalOut ledR(dp28),ledG(dp26),ledB(dp14); 00036 InterruptIn RunPB(dp13); 00037 Serial pc(dp16, dp15); // tx, rx 00038 00039 00040 /* PID constant initialize Kc, Ti, Td, interval */ 00041 #define P 0.1 //propotional band 00042 #define I 1.5 //Integral 00043 #define D 1.0 //Devide 00044 #define RATE 0.05 //update time sec 00045 #define InitialSP 30.0 // Boot Setpoint initial temeprature 00046 PID TIC(P, I, D, RATE); 00047 00048 /*LCD I2C pin initialize */ 00049 char *init_massage = "Hello!"; 00050 SB1602E lcd(dp5, dp27, init_massage); // SDA, SCL 00051 /*QEI initialize */ 00052 #define ROTATE_PER_REVOLUTIONS 24 00053 QEI wheel(dp17, dp18, NC, ROTATE_PER_REVOLUTIONS, QEI::X2_ENCODING); 00054 00055 //Initial 00056 float temp_sv_input = InitialSP, waittime,OV_LL,ticout,qei,Bias; 00057 bool Run(false); 00058 double temp_pv,temp_cal; 00059 int rtostime; 00060 00061 /*PB control*/ 00062 void runmode() 00063 { 00064 Run = !Run; 00065 } 00066 00067 void cal_temp(void const *argument) 00068 { 00069 while(1) { 00070 /*input for change to 0 to 100% range by 30C to 120C */ 00071 /*Temperature setpoint low high range */ 00072 #define RangeSPL 30.0 //Celcius low side temperature 00073 temp_sv_input = wheel.getPulses() / 20.0 + RangeSPL; 00074 if (temp_sv_input <= RangeSPL) { 00075 temp_sv_input = RangeSPL; 00076 #define RangeSPH 100.0 //Celcius high side temperature 00077 } else if (temp_sv_input >= RangeSPH) { 00078 temp_sv_input = RangeSPH; 00079 } 00080 00081 /*six order polynomial calculation value 00082 Thermister pull up resiter 560R 00083 Thermister B value 3380K 00084 Thermister Resistance 10K ohm at 25C 00085 This NTC is NCP18XH103F03RB muRata 00086 */ 00087 temp_cal = THAI.read() * 3.3; 00088 temp_pv =-0.7964*pow(temp_cal,6.0) - 2.5431*pow(temp_cal,5.0) +63.605*pow(temp_cal,4.0) - 274.1*pow(temp_cal,3.0) + 522.57*pow(temp_cal,2.0) - 539.26*temp_cal + 405.76; 00089 00090 /*LCD Display section */ 00091 /*LCD Display section */ 00092 lcd.printf(0, "SP %.1f", temp_sv_input); 00093 lcd.printf(0, "PV %.1f\n", temp_pv); 00094 lcd.printf(1, "OUT %.2f", out.read() * 100); 00095 lcd.printf(1, "PB %s\n", Run); 00096 /*Tenperature indicater */ 00097 /* 1.5C high temperature */ 00098 if (Run == true) { 00099 if ((temp_pv - temp_sv_input) >= 1.5) { 00100 ledR = 0; 00101 ledG = 1; 00102 ledB = 1; 00103 /* 1.5C low temperature */ 00104 } else if ((temp_sv_input - temp_pv ) >= 1.5 ) { 00105 ledR = 1; 00106 ledG = 1; 00107 ledB = 0; 00108 } else { 00109 /* control green */ 00110 ledR = 1; 00111 ledG = 0; 00112 ledB = 1; 00113 } 00114 } else { 00115 ledR = 1; 00116 ledG = 1; 00117 ledB = 1; 00118 } 00119 Thread::wait(rtostime * 2); 00120 } 00121 } 00122 00123 00124 int main() 00125 { 00126 RunPB.mode(PullDown); 00127 #define LCDCont 0x32 //LCD contrast set from 00 to 3f 64resolution defult set is 32step 00128 lcd.contrast(LCDCont); 00129 out.period(0.02); 00130 RunPB.rise(&runmode); 00131 Thread thread(cal_temp); 00132 rtostime = RATE * 1000; 00133 00134 /* Initial startup bias culcuration */ 00135 if ((temp_sv_input - temp_pv) >= 50) { 00136 Bias = 50.0; 00137 }else if ((temp_sv_input - temp_pv) >= 30){ 00138 Bias = 30.0; 00139 }else if ((temp_sv_input - temp_pv) >= 20){ 00140 Bias = 20.0; 00141 } else { 00142 Bias = 0.0; 00143 } 00144 00145 while (1) { 00146 /*PID control*/ 00147 #define SV_LL 30.0 //PID setpoint TempC value for lo limit 00148 #define SV_HL 100.0 //PID setpoint TempC value for high limit 00149 TIC.setInputLimits(SV_LL, SV_HL); 00150 #define OV_LL 0.0 //PID calcurate output value 0.0 = 0% 00151 #define OV_HL 100.0 //PID calcurate output value 1.0 = 100% 00152 if (Run == true) { 00153 TIC.setOutputLimits(OV_LL, OV_HL); 00154 TIC.setSetPoint(temp_sv_input); 00155 TIC.setProcessValue(temp_pv); 00156 TIC.setBias(Bias); //control output bias 00157 TIC.setMode(1); 00158 out = TIC.compute() /100; 00159 TIC.setInterval(RATE); 00160 } else if (Run == false) { 00161 TIC.setMode(0); 00162 TIC.reset(); 00163 out = 0.0; 00164 } 00165 Thread::wait(rtostime); 00166 00167 } 00168 }
Generated on Sun Jul 17 2022 17:31:05 by
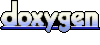