
Example program to test AES-GCM functionality. Used for a workshop
X509_module
Data Structures | |
struct | _x509_time |
Container for date and time (precision in seconds). More... | |
struct | _x509_crl_entry |
Certificate revocation list entry. More... | |
struct | _x509_crl |
Certificate revocation list structure. More... | |
struct | _x509_crt |
Container for an X.509 certificate. More... | |
struct | _x509write_cert |
Container for writing a certificate (CRT) More... | |
struct | _x509_csr |
Certificate Signing Request (CSR) structure. More... | |
struct | _x509write_csr |
Container for writing a CSR. More... | |
Functions | |
int | dhm_parse_dhm (dhm_context *dhm, const unsigned char *dhmin, size_t dhminlen) |
Parse DHM parameters. | |
int | dhm_parse_dhmfile (dhm_context *dhm, const char *path) |
Load and parse DHM parameters. | |
Structures for parsing X.509 certificates, CRLs and CSRs | |
typedef asn1_buf | x509_buf |
Type-length-value structure that allows for ASN1 using DER. | |
typedef asn1_bitstring | x509_bitstring |
Container for ASN1 bit strings. | |
typedef asn1_named_data | x509_name |
Container for ASN1 named information objects. | |
typedef asn1_sequence | x509_sequence |
Container for a sequence of ASN.1 items. | |
typedef struct _x509_time | x509_time |
Container for date and time (precision in seconds). | |
Structures and functions for parsing CRLs | |
typedef struct _x509_crl_entry | x509_crl_entry |
Certificate revocation list entry. | |
typedef struct _x509_crl | x509_crl |
Certificate revocation list structure. | |
int | x509_crl_parse (x509_crl *chain, const unsigned char *buf, size_t buflen) |
Parse one or more CRLs and add them to the chained list. | |
int | x509_crl_parse_file (x509_crl *chain, const char *path) |
Load one or more CRLs and add them to the chained list. | |
int | x509_crl_info (char *buf, size_t size, const char *prefix, const x509_crl *crl) |
Returns an informational string about the CRL. | |
void | x509_crl_init (x509_crl *crl) |
Initialize a CRL (chain) | |
void | x509_crl_free (x509_crl *crl) |
Unallocate all CRL data. | |
Structures and functions for parsing and writing X.509 certificates | |
typedef struct _x509_crt | x509_crt |
Container for an X.509 certificate. | |
typedef struct _x509write_cert | x509write_cert |
Container for writing a certificate (CRT) | |
int | x509_crt_parse_der (x509_crt *chain, const unsigned char *buf, size_t buflen) |
Parse a single DER formatted certificate and add it to the chained list. | |
int | x509_crt_parse (x509_crt *chain, const unsigned char *buf, size_t buflen) |
Parse one or more certificates and add them to the chained list. | |
int | x509_crt_parse_file (x509_crt *chain, const char *path) |
Load one or more certificates and add them to the chained list. | |
int | x509_crt_parse_path (x509_crt *chain, const char *path) |
Load one or more certificate files from a path and add them to the chained list. | |
int | x509_crt_info (char *buf, size_t size, const char *prefix, const x509_crt *crt) |
Returns an informational string about the certificate. | |
int | x509_crt_verify (x509_crt *crt, x509_crt *trust_ca, x509_crl *ca_crl, const char *cn, int *flags, int(*f_vrfy)(void *, x509_crt *, int, int *), void *p_vrfy) |
Verify the certificate signature. | |
int | x509_crt_check_key_usage (const x509_crt *crt, int usage) |
Check usage of certificate against keyUsage extension. | |
int | x509_crt_check_extended_key_usage (const x509_crt *crt, const char *usage_oid, size_t usage_len) |
Check usage of certificate against extentedJeyUsage. | |
int | x509_crt_revoked (const x509_crt *crt, const x509_crl *crl) |
Verify the certificate revocation status. | |
void | x509_crt_init (x509_crt *crt) |
Initialize a certificate (chain) | |
void | x509_crt_free (x509_crt *crt) |
Unallocate all certificate data. | |
Structures and functions for X.509 Certificate Signing Requests (CSR) | |
typedef struct _x509_csr | x509_csr |
Certificate Signing Request (CSR) structure. | |
typedef struct _x509write_csr | x509write_csr |
Container for writing a CSR. | |
int | x509_csr_parse (x509_csr *csr, const unsigned char *buf, size_t buflen) |
Load a Certificate Signing Request (CSR) | |
int | x509_csr_parse_file (x509_csr *csr, const char *path) |
Load a Certificate Signing Request (CSR) | |
int | x509_csr_info (char *buf, size_t size, const char *prefix, const x509_csr *csr) |
Returns an informational string about the CSR. | |
void | x509_csr_init (x509_csr *csr) |
Initialize a CSR. | |
void | x509_csr_free (x509_csr *csr) |
Unallocate all CSR data. |
Typedef Documentation
typedef asn1_bitstring x509_bitstring |
Certificate revocation list structure.
Every CRL may have multiple entries.
typedef struct _x509_crl_entry x509_crl_entry |
Certificate revocation list entry.
Contains the CA-specific serial numbers and revocation dates.
Container for an X.509 certificate.
The certificate may be chained.
typedef asn1_named_data x509_name |
typedef asn1_sequence x509_sequence |
typedef struct _x509_time x509_time |
Container for date and time (precision in seconds).
typedef struct _x509write_cert x509write_cert |
Container for writing a certificate (CRT)
typedef struct _x509write_csr x509write_csr |
Container for writing a CSR.
Function Documentation
int dhm_parse_dhm | ( | dhm_context * | dhm, |
const unsigned char * | dhmin, | ||
size_t | dhminlen | ||
) |
int dhm_parse_dhmfile | ( | dhm_context * | dhm, |
const char * | path | ||
) |
void x509_crl_free | ( | x509_crl * | crl ) |
Unallocate all CRL data.
- Parameters:
-
crl CRL chain to free
Definition at line 701 of file x509_crl.c.
int x509_crl_info | ( | char * | buf, |
size_t | size, | ||
const char * | prefix, | ||
const x509_crl * | crl | ||
) |
Returns an informational string about the CRL.
- Parameters:
-
buf Buffer to write to size Maximum size of buffer prefix A line prefix crl The X509 CRL to represent
- Returns:
- The amount of data written to the buffer, or -1 in case of an error.
Definition at line 614 of file x509_crl.c.
void x509_crl_init | ( | x509_crl * | crl ) |
Initialize a CRL (chain)
- Parameters:
-
crl CRL chain to initialize
Definition at line 693 of file x509_crl.c.
int x509_crl_parse | ( | x509_crl * | chain, |
const unsigned char * | buf, | ||
size_t | buflen | ||
) |
Parse one or more CRLs and add them to the chained list.
- Parameters:
-
chain points to the start of the chain buf buffer holding the CRL data buflen size of the buffer
- Returns:
- 0 if successful, or a specific X509 or PEM error code
Definition at line 253 of file x509_crl.c.
int x509_crl_parse_file | ( | x509_crl * | chain, |
const char * | path | ||
) |
Load one or more CRLs and add them to the chained list.
- Parameters:
-
chain points to the start of the chain path filename to read the CRLs from
- Returns:
- 0 if successful, or a specific X509 or PEM error code
Definition at line 536 of file x509_crl.c.
int x509_crt_check_extended_key_usage | ( | const x509_crt * | crt, |
const char * | usage_oid, | ||
size_t | usage_len | ||
) |
Check usage of certificate against extentedJeyUsage.
- Parameters:
-
crt Leaf certificate used. usage_oid Intended usage (eg OID_SERVER_AUTH or OID_CLIENT_AUTH). usage_len Length of usage_oid (eg given by OID_SIZE()).
- Returns:
- 0 is this use of the certificate is allowed, POLARSSL_ERR_X509_BAD_INPUT_DATA if not.
- Note:
- Usually only makes sense on leaf certificates.
Definition at line 1381 of file x509_crt.c.
int x509_crt_check_key_usage | ( | const x509_crt * | crt, |
int | usage | ||
) |
Check usage of certificate against keyUsage extension.
- Parameters:
-
crt Leaf certificate used. usage Intended usage(s) (eg KU_KEY_ENCIPHERMENT before using the certificate to perform an RSA key exchange).
- Returns:
- 0 is these uses of the certificate are allowed, POLARSSL_ERR_X509_BAD_INPUT_DATA if the keyUsage extension is present but does not contain all the bits set in the usage argument.
- Note:
- You should only call this function on leaf certificates, on (intermediate) CAs the keyUsage extension is automatically checked by
x509_crt_verify()
.
Definition at line 1370 of file x509_crt.c.
void x509_crt_free | ( | x509_crt * | crt ) |
Unallocate all certificate data.
- Parameters:
-
crt Certificate chain to free
Definition at line 1904 of file x509_crt.c.
int x509_crt_info | ( | char * | buf, |
size_t | size, | ||
const char * | prefix, | ||
const x509_crt * | crt | ||
) |
Returns an informational string about the certificate.
- Parameters:
-
buf Buffer to write to size Maximum size of buffer prefix A line prefix crt The X509 certificate to represent
- Returns:
- The amount of data written to the buffer, or -1 in case of an error.
Definition at line 1241 of file x509_crt.c.
void x509_crt_init | ( | x509_crt * | crt ) |
Initialize a certificate (chain)
- Parameters:
-
crt Certificate chain to initialize
Definition at line 1896 of file x509_crt.c.
int x509_crt_parse | ( | x509_crt * | chain, |
const unsigned char * | buf, | ||
size_t | buflen | ||
) |
Parse one or more certificates and add them to the chained list.
Parses permissively. If some certificates can be parsed, the result is the number of failed certificates it encountered. If none complete correctly, the first error is returned.
- Parameters:
-
chain points to the start of the chain buf buffer holding the certificate data buflen size of the buffer
- Returns:
- 0 if all certificates parsed successfully, a positive number if partly successful or a specific X509 or PEM error code
Definition at line 825 of file x509_crt.c.
int x509_crt_parse_der | ( | x509_crt * | chain, |
const unsigned char * | buf, | ||
size_t | buflen | ||
) |
Parse a single DER formatted certificate and add it to the chained list.
- Parameters:
-
chain points to the start of the chain buf buffer holding the certificate DER data buflen size of the buffer
- Returns:
- 0 if successful, or a specific X509 or PEM error code
Definition at line 774 of file x509_crt.c.
int x509_crt_parse_file | ( | x509_crt * | chain, |
const char * | path | ||
) |
Load one or more certificates and add them to the chained list.
Parses permissively. If some certificates can be parsed, the result is the number of failed certificates it encountered. If none complete correctly, the first error is returned.
- Parameters:
-
chain points to the start of the chain path filename to read the certificates from
- Returns:
- 0 if all certificates parsed successfully, a positive number if partly successful or a specific X509 or PEM error code
Definition at line 930 of file x509_crt.c.
int x509_crt_parse_path | ( | x509_crt * | chain, |
const char * | path | ||
) |
Load one or more certificate files from a path and add them to the chained list.
Parses permissively. If some certificates can be parsed, the result is the number of failed certificates it encountered. If none complete correctly, the first error is returned.
- Warning:
- This function is NOT thread-safe unless POLARSSL_THREADING_PTHREADS is defined. If you're using an alternative threading implementation, you should either use this function only in the main thread, or mutex it.
- Parameters:
-
chain points to the start of the chain path directory / folder to read the certificate files from
- Returns:
- 0 if all certificates parsed successfully, a positive number if partly successful or a specific X509 or PEM error code
Definition at line 951 of file x509_crt.c.
Verify the certificate revocation status.
- Parameters:
-
crt a certificate to be verified crl the CRL to verify against
- Returns:
- 1 if the certificate is revoked, 0 otherwise
Definition at line 1416 of file x509_crt.c.
int x509_crt_verify | ( | x509_crt * | crt, |
x509_crt * | trust_ca, | ||
x509_crl * | ca_crl, | ||
const char * | cn, | ||
int * | flags, | ||
int(*)(void *, x509_crt *, int, int *) | f_vrfy, | ||
void * | p_vrfy | ||
) |
Verify the certificate signature.
The verify callback is a user-supplied callback that can clear / modify / add flags for a certificate. If set, the verification callback is called for each certificate in the chain (from the trust-ca down to the presented crt). The parameters for the callback are: (void *parameter, x509_crt *crt, int certificate_depth, int *flags). With the flags representing current flags for that specific certificate and the certificate depth from the bottom (Peer cert depth = 0).
All flags left after returning from the callback are also returned to the application. The function should return 0 for anything but a fatal error.
- Parameters:
-
crt a certificate to be verified trust_ca the trusted CA chain ca_crl the CRL chain for trusted CA's cn expected Common Name (can be set to NULL if the CN must not be verified) flags result of the verification f_vrfy verification function p_vrfy verification parameter
- Returns:
- 0 if successful or POLARSSL_ERR_X509_SIG_VERIFY_FAILED, in which case *flags will have one or more of the following values set: BADCERT_EXPIRED -- BADCERT_REVOKED -- BADCERT_CN_MISMATCH -- BADCERT_NOT_TRUSTED or another error in case of a fatal error encountered during the verification process.
Definition at line 1798 of file x509_crt.c.
void x509_csr_free | ( | x509_csr * | csr ) |
int x509_csr_info | ( | char * | buf, |
size_t | size, | ||
const char * | prefix, | ||
const x509_csr * | csr | ||
) |
Returns an informational string about the CSR.
- Parameters:
-
buf Buffer to write to size Maximum size of buffer prefix A line prefix csr The X509 CSR to represent
- Returns:
- The amount of data written to the buffer, or -1 in case of an error.
Definition at line 358 of file x509_csr.c.
void x509_csr_init | ( | x509_csr * | csr ) |
int x509_csr_parse | ( | x509_csr * | csr, |
const unsigned char * | buf, | ||
size_t | buflen | ||
) |
Load a Certificate Signing Request (CSR)
- Parameters:
-
csr CSR context to fill buf buffer holding the CRL data buflen size of the buffer
- Returns:
- 0 if successful, or a specific X509 or PEM error code
Definition at line 91 of file x509_csr.c.
int x509_csr_parse_file | ( | x509_csr * | csr, |
const char * | path | ||
) |
Load a Certificate Signing Request (CSR)
- Parameters:
-
csr CSR context to fill path filename to read the CSR from
- Returns:
- 0 if successful, or a specific X509 or PEM error code
Definition at line 283 of file x509_csr.c.
Generated on Tue Jul 12 2022 19:40:22 by
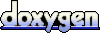