
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
x509.h
Go to the documentation of this file.
00001 /** 00002 * \file x509.h 00003 * 00004 * \brief X.509 generic defines and structures 00005 * 00006 * Copyright (C) 2006-2014, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 #ifndef POLARSSL_X509_H 00028 #define POLARSSL_X509_H 00029 00030 #if !defined(POLARSSL_CONFIG_FILE) 00031 #include "config.h" 00032 #else 00033 #include POLARSSL_CONFIG_FILE 00034 #endif 00035 00036 #include "asn1.h" 00037 #include "pk.h" 00038 00039 #if defined(POLARSSL_RSA_C) 00040 #include "rsa.h" 00041 #endif 00042 00043 /** 00044 * \addtogroup x509_module 00045 * \{ 00046 */ 00047 00048 /** 00049 * \name X509 Error codes 00050 * \{ 00051 */ 00052 #define POLARSSL_ERR_X509_FEATURE_UNAVAILABLE -0x2080 /**< Unavailable feature, e.g. RSA hashing/encryption combination. */ 00053 #define POLARSSL_ERR_X509_UNKNOWN_OID -0x2100 /**< Requested OID is unknown. */ 00054 #define POLARSSL_ERR_X509_INVALID_FORMAT -0x2180 /**< The CRT/CRL/CSR format is invalid, e.g. different type expected. */ 00055 #define POLARSSL_ERR_X509_INVALID_VERSION -0x2200 /**< The CRT/CRL/CSR version element is invalid. */ 00056 #define POLARSSL_ERR_X509_INVALID_SERIAL -0x2280 /**< The serial tag or value is invalid. */ 00057 #define POLARSSL_ERR_X509_INVALID_ALG -0x2300 /**< The algorithm tag or value is invalid. */ 00058 #define POLARSSL_ERR_X509_INVALID_NAME -0x2380 /**< The name tag or value is invalid. */ 00059 #define POLARSSL_ERR_X509_INVALID_DATE -0x2400 /**< The date tag or value is invalid. */ 00060 #define POLARSSL_ERR_X509_INVALID_SIGNATURE -0x2480 /**< The signature tag or value invalid. */ 00061 #define POLARSSL_ERR_X509_INVALID_EXTENSIONS -0x2500 /**< The extension tag or value is invalid. */ 00062 #define POLARSSL_ERR_X509_UNKNOWN_VERSION -0x2580 /**< CRT/CRL/CSR has an unsupported version number. */ 00063 #define POLARSSL_ERR_X509_UNKNOWN_SIG_ALG -0x2600 /**< Signature algorithm (oid) is unsupported. */ 00064 #define POLARSSL_ERR_X509_SIG_MISMATCH -0x2680 /**< Signature algorithms do not match. (see \c ::x509_crt sig_oid) */ 00065 #define POLARSSL_ERR_X509_CERT_VERIFY_FAILED -0x2700 /**< Certificate verification failed, e.g. CRL, CA or signature check failed. */ 00066 #define POLARSSL_ERR_X509_CERT_UNKNOWN_FORMAT -0x2780 /**< Format not recognized as DER or PEM. */ 00067 #define POLARSSL_ERR_X509_BAD_INPUT_DATA -0x2800 /**< Input invalid. */ 00068 #define POLARSSL_ERR_X509_MALLOC_FAILED -0x2880 /**< Allocation of memory failed. */ 00069 #define POLARSSL_ERR_X509_FILE_IO_ERROR -0x2900 /**< Read/write of file failed. */ 00070 /* \} name */ 00071 00072 /** 00073 * \name X509 Verify codes 00074 * \{ 00075 */ 00076 #define BADCERT_EXPIRED 0x01 /**< The certificate validity has expired. */ 00077 #define BADCERT_REVOKED 0x02 /**< The certificate has been revoked (is on a CRL). */ 00078 #define BADCERT_CN_MISMATCH 0x04 /**< The certificate Common Name (CN) does not match with the expected CN. */ 00079 #define BADCERT_NOT_TRUSTED 0x08 /**< The certificate is not correctly signed by the trusted CA. */ 00080 #define BADCRL_NOT_TRUSTED 0x10 /**< CRL is not correctly signed by the trusted CA. */ 00081 #define BADCRL_EXPIRED 0x20 /**< CRL is expired. */ 00082 #define BADCERT_MISSING 0x40 /**< Certificate was missing. */ 00083 #define BADCERT_SKIP_VERIFY 0x80 /**< Certificate verification was skipped. */ 00084 #define BADCERT_OTHER 0x0100 /**< Other reason (can be used by verify callback) */ 00085 #define BADCERT_FUTURE 0x0200 /**< The certificate validity starts in the future. */ 00086 #define BADCRL_FUTURE 0x0400 /**< The CRL is from the future */ 00087 /* \} name */ 00088 /* \} addtogroup x509_module */ 00089 00090 /* 00091 * X.509 v3 Key Usage Extension flags 00092 */ 00093 #define KU_DIGITAL_SIGNATURE (0x80) /* bit 0 */ 00094 #define KU_NON_REPUDIATION (0x40) /* bit 1 */ 00095 #define KU_KEY_ENCIPHERMENT (0x20) /* bit 2 */ 00096 #define KU_DATA_ENCIPHERMENT (0x10) /* bit 3 */ 00097 #define KU_KEY_AGREEMENT (0x08) /* bit 4 */ 00098 #define KU_KEY_CERT_SIGN (0x04) /* bit 5 */ 00099 #define KU_CRL_SIGN (0x02) /* bit 6 */ 00100 00101 /* 00102 * Netscape certificate types 00103 * (http://www.mozilla.org/projects/security/pki/nss/tech-notes/tn3.html) 00104 */ 00105 00106 #define NS_CERT_TYPE_SSL_CLIENT (0x80) /* bit 0 */ 00107 #define NS_CERT_TYPE_SSL_SERVER (0x40) /* bit 1 */ 00108 #define NS_CERT_TYPE_EMAIL (0x20) /* bit 2 */ 00109 #define NS_CERT_TYPE_OBJECT_SIGNING (0x10) /* bit 3 */ 00110 #define NS_CERT_TYPE_RESERVED (0x08) /* bit 4 */ 00111 #define NS_CERT_TYPE_SSL_CA (0x04) /* bit 5 */ 00112 #define NS_CERT_TYPE_EMAIL_CA (0x02) /* bit 6 */ 00113 #define NS_CERT_TYPE_OBJECT_SIGNING_CA (0x01) /* bit 7 */ 00114 00115 /* 00116 * X.509 extension types 00117 * 00118 * Comments refer to the status for using certificates. Status can be 00119 * different for writing certificates or reading CRLs or CSRs. 00120 */ 00121 #define EXT_AUTHORITY_KEY_IDENTIFIER (1 << 0) 00122 #define EXT_SUBJECT_KEY_IDENTIFIER (1 << 1) 00123 #define EXT_KEY_USAGE (1 << 2) /* Parsed but not used */ 00124 #define EXT_CERTIFICATE_POLICIES (1 << 3) 00125 #define EXT_POLICY_MAPPINGS (1 << 4) 00126 #define EXT_SUBJECT_ALT_NAME (1 << 5) /* Supported (DNS) */ 00127 #define EXT_ISSUER_ALT_NAME (1 << 6) 00128 #define EXT_SUBJECT_DIRECTORY_ATTRS (1 << 7) 00129 #define EXT_BASIC_CONSTRAINTS (1 << 8) /* Supported */ 00130 #define EXT_NAME_CONSTRAINTS (1 << 9) 00131 #define EXT_POLICY_CONSTRAINTS (1 << 10) 00132 #define EXT_EXTENDED_KEY_USAGE (1 << 11) /* Parsed but not used */ 00133 #define EXT_CRL_DISTRIBUTION_POINTS (1 << 12) 00134 #define EXT_INIHIBIT_ANYPOLICY (1 << 13) 00135 #define EXT_FRESHEST_CRL (1 << 14) 00136 00137 #define EXT_NS_CERT_TYPE (1 << 16) /* Parsed (and then ?) */ 00138 00139 /* 00140 * Storage format identifiers 00141 * Recognized formats: PEM and DER 00142 */ 00143 #define X509_FORMAT_DER 1 00144 #define X509_FORMAT_PEM 2 00145 00146 #ifdef __cplusplus 00147 extern "C" { 00148 #endif 00149 00150 /** 00151 * \addtogroup x509_module 00152 * \{ */ 00153 00154 /** 00155 * \name Structures for parsing X.509 certificates, CRLs and CSRs 00156 * \{ 00157 */ 00158 00159 /** 00160 * Type-length-value structure that allows for ASN1 using DER. 00161 */ 00162 typedef asn1_buf x509_buf; 00163 00164 /** 00165 * Container for ASN1 bit strings. 00166 */ 00167 typedef asn1_bitstring x509_bitstring; 00168 00169 /** 00170 * Container for ASN1 named information objects. 00171 * It allows for Relative Distinguished Names (e.g. cn=polarssl,ou=code,etc.). 00172 */ 00173 typedef asn1_named_data x509_name; 00174 00175 /** 00176 * Container for a sequence of ASN.1 items 00177 */ 00178 typedef asn1_sequence x509_sequence; 00179 00180 /** Container for date and time (precision in seconds). */ 00181 typedef struct _x509_time 00182 { 00183 int year, mon, day; /**< Date. */ 00184 int hour, min, sec; /**< Time. */ 00185 } 00186 x509_time; 00187 00188 /** \} name Structures for parsing X.509 certificates, CRLs and CSRs */ 00189 /** \} addtogroup x509_module */ 00190 00191 /** 00192 * \brief Store the certificate DN in printable form into buf; 00193 * no more than size characters will be written. 00194 * 00195 * \param buf Buffer to write to 00196 * \param size Maximum size of buffer 00197 * \param dn The X509 name to represent 00198 * 00199 * \return The amount of data written to the buffer, or -1 in 00200 * case of an error. 00201 */ 00202 int x509_dn_gets( char *buf, size_t size, const x509_name *dn ); 00203 00204 /** 00205 * \brief Store the certificate serial in printable form into buf; 00206 * no more than size characters will be written. 00207 * 00208 * \param buf Buffer to write to 00209 * \param size Maximum size of buffer 00210 * \param serial The X509 serial to represent 00211 * 00212 * \return The amount of data written to the buffer, or -1 in 00213 * case of an error. 00214 */ 00215 int x509_serial_gets( char *buf, size_t size, const x509_buf *serial ); 00216 00217 /** 00218 * \brief Give an known OID, return its descriptive string. 00219 * (Deprecated. Use oid_get_extended_key_usage() instead.) 00220 * Warning: only works for extended_key_usage OIDs! 00221 * 00222 * \param oid buffer containing the oid 00223 * 00224 * \return Return a string if the OID is known, 00225 * or NULL otherwise. 00226 */ 00227 const char *x509_oid_get_description( x509_buf *oid ); 00228 00229 /** 00230 * \brief Give an OID, return a string version of its OID number. 00231 * (Deprecated. Use oid_get_numeric_string() instead) 00232 * 00233 * \param buf Buffer to write to 00234 * \param size Maximum size of buffer 00235 * \param oid Buffer containing the OID 00236 * 00237 * \return Length of the string written (excluding final NULL) or 00238 * POLARSSL_ERR_OID_BUF_TO_SMALL in case of error 00239 */ 00240 int x509_oid_get_numeric_string( char *buf, size_t size, x509_buf *oid ); 00241 00242 /** 00243 * \brief Check a given x509_time against the system time and check 00244 * if it is not expired. 00245 * 00246 * \param time x509_time to check 00247 * 00248 * \return 0 if the x509_time is still valid, 00249 * 1 otherwise. 00250 */ 00251 int x509_time_expired( const x509_time *time ); 00252 00253 /** 00254 * \brief Check a given x509_time against the system time and check 00255 * if it is not from the future. 00256 * 00257 * \param time x509_time to check 00258 * 00259 * \return 0 if the x509_time is already valid, 00260 * 1 otherwise. 00261 */ 00262 int x509_time_future( const x509_time *time ); 00263 00264 /** 00265 * \brief Checkup routine 00266 * 00267 * \return 0 if successful, or 1 if the test failed 00268 */ 00269 int x509_self_test( int verbose ); 00270 00271 /* 00272 * Internal module functions. You probably do not want to use these unless you 00273 * know you do. 00274 */ 00275 int x509_get_name( unsigned char **p, const unsigned char *end, 00276 x509_name *cur ); 00277 int x509_get_alg_null( unsigned char **p, const unsigned char *end, 00278 x509_buf *alg ); 00279 int x509_get_sig( unsigned char **p, const unsigned char *end, x509_buf *sig ); 00280 int x509_get_sig_alg( const x509_buf *sig_oid, md_type_t *md_alg, 00281 pk_type_t *pk_alg ); 00282 int x509_get_time( unsigned char **p, const unsigned char *end, 00283 x509_time *time ); 00284 int x509_get_serial( unsigned char **p, const unsigned char *end, 00285 x509_buf *serial ); 00286 int x509_get_ext( unsigned char **p, const unsigned char *end, 00287 x509_buf *ext, int tag ); 00288 int x509_load_file( const char *path, unsigned char **buf, size_t *n ); 00289 int x509_key_size_helper( char *buf, size_t size, const char *name ); 00290 int x509_string_to_names( asn1_named_data **head, const char *name ); 00291 int x509_set_extension( asn1_named_data **head, const char *oid, size_t oid_len, 00292 int critical, const unsigned char *val, 00293 size_t val_len ); 00294 int x509_write_extensions( unsigned char **p, unsigned char *start, 00295 asn1_named_data *first ); 00296 int x509_write_names( unsigned char **p, unsigned char *start, 00297 asn1_named_data *first ); 00298 int x509_write_sig( unsigned char **p, unsigned char *start, 00299 const char *oid, size_t oid_len, 00300 unsigned char *sig, size_t size ); 00301 00302 #ifdef __cplusplus 00303 } 00304 #endif 00305 00306 #endif /* x509.h */ 00307 00308
Generated on Tue Jul 12 2022 19:40:21 by
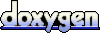