IMU-pressure-tempreture sensors
Dependencies: CMSIS_DSP_401 DHT22 MPU9150_DMP QuaternionMath MODSERIAL mbed-src FATFileSystem111 SDFileSystem11 Camera_LS_Y201_CANSAT
BMP085.cpp
00001 /* 00002 * mbed library to use a Bosch Sensortec BMP085 sensor 00003 * Copyright (c) 2010 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 #include "mbed.h" 00008 #include "BMP085.h" 00009 00010 #define WEATHER_BMP085 0xee 00011 #define xpow(x, y) ((long)1 << y) 00012 const float ALTITUDE_EXPONENT = 1/5.255; 00013 const float ALTITUDE_PRESSURE_REFERENCE = 100000; 00014 const float ALTITUDE_COEFF = 44330.0; 00015 float BMP085::calcAltitude(int pressure) { 00016 //Compute an estimate of altitude 00017 // see page 15 of manual 00018 float float_comp_pressure = (float) pressure; 00019 float altitude = float_comp_pressure/ALTITUDE_PRESSURE_REFERENCE; 00020 altitude = pow(altitude, ALTITUDE_EXPONENT); 00021 altitude = 1.0 - altitude; 00022 altitude *= ALTITUDE_COEFF; 00023 // formula is: altitude = 44330 * (1-pow((p/p0), 1/5.255)) 00024 return altitude; 00025 } 00026 00027 BMP085::BMP085 (PinName p_sda, PinName p_scl, BMP085_oss p_oss) : i2c(p_sda, p_scl) { 00028 init(p_oss); 00029 } 00030 00031 BMP085::BMP085 (I2C& p_i2c, BMP085_oss p_oss) : i2c(p_i2c) { 00032 init(p_oss); 00033 } 00034 00035 float BMP085::get_temperature() { 00036 return temperature; 00037 } 00038 00039 float BMP085::get_pressure() { 00040 return pressure; 00041 00042 } 00043 00044 void BMP085::update () { 00045 long t, p, ut, up, x1, x2, x3, b3, b5, b6; 00046 unsigned long b4, b7; 00047 00048 twi_writechar(WEATHER_BMP085, 0xf4, 0x2e); 00049 wait(0.01); 00050 ut = twi_readshort(WEATHER_BMP085, 0xf6); 00051 00052 twi_writechar(WEATHER_BMP085, 0xf4, 0x34 | (oss << 6)); 00053 wait(0.05); 00054 up = twi_readlong(WEATHER_BMP085, 0xf6) >> (8 - oss); 00055 00056 x1 = (ut - ac6) * ac5 / xpow(2, 15); 00057 x2 = (long)mc * xpow(2, 11) / (x1 + md); 00058 b5 = x1 + x2; 00059 t = (b5 + 8) / xpow(2, 4); 00060 temperature = (float)t / 10.0; 00061 00062 b6 = b5 - 4000; 00063 x1 = (b2 * (b6 * b6 / xpow(2, 12))) / xpow(2, 11); 00064 x2 = ac2 * b6 / xpow(2, 11); 00065 x3 = x1 + x2; 00066 b3 = ((((unsigned long)ac1 * 4 + x3) << oss) + 2) / 4; 00067 x1 = ac3 * b6 / xpow(2, 13); 00068 x2 = (b1 * (b6 * b6 / xpow(2, 12))) / xpow(2, 16); 00069 x3 = ((x1 + x2) + 2) / xpow(2, 2); 00070 b4 = ac4 * (unsigned long)(x3 + 32768) / xpow(2, 15); 00071 b7 = ((unsigned long)up - b3) * (50000 >> oss); 00072 if (b7 < (unsigned long)0x80000000) { 00073 p = (b7 * 2) / b4; 00074 } else { 00075 p = (b7 / b4) * 2; 00076 } 00077 x1 = (p / xpow(2, 8)) * (p / xpow(2, 8)); 00078 x1 = (x1 * 3038) / xpow(2, 16); 00079 x2 = (-7357 * p) / xpow(2, 16); 00080 p = p + (x1 + x2 + 3791) / xpow(2, 4); 00081 pressure = (float)p / 100.0; 00082 } 00083 00084 void BMP085::init (BMP085_oss p_oss) { 00085 ac1 = twi_readshort(WEATHER_BMP085, 0xaa); 00086 ac2 = twi_readshort(WEATHER_BMP085, 0xac); 00087 ac3 = twi_readshort(WEATHER_BMP085, 0xae); 00088 ac4 = twi_readshort(WEATHER_BMP085, 0xb0); 00089 ac5 = twi_readshort(WEATHER_BMP085, 0xb2); 00090 ac6 = twi_readshort(WEATHER_BMP085, 0xb4); 00091 b1 = twi_readshort(WEATHER_BMP085, 0xb6); 00092 b2 = twi_readshort(WEATHER_BMP085, 0xb8); 00093 mb = twi_readshort(WEATHER_BMP085, 0xba); 00094 mc = twi_readshort(WEATHER_BMP085, 0xbc); 00095 md = twi_readshort(WEATHER_BMP085, 0xbe); 00096 oss = p_oss; 00097 } 00098 00099 unsigned short BMP085::twi_readshort (int id, int addr) { 00100 unsigned short i; 00101 00102 i2c.start(); 00103 i2c.write(id); 00104 i2c.write(addr); 00105 00106 i2c.start(); 00107 i2c.write(id | 1); 00108 i = i2c.read(1) << 8; 00109 i |= i2c.read(0); 00110 i2c.stop(); 00111 00112 return i; 00113 } 00114 00115 unsigned long BMP085::twi_readlong (int id, int addr) { 00116 unsigned long i; 00117 00118 i2c.start(); 00119 i2c.write(id); 00120 i2c.write(addr); 00121 00122 i2c.start(); 00123 i2c.write(id | 1); 00124 i = i2c.read(1) << 16; 00125 i |= i2c.read(1) << 8; 00126 i |= i2c.read(0); 00127 i2c.stop(); 00128 00129 return i; 00130 } 00131 00132 void BMP085::twi_writechar (int id, int addr, int dat) { 00133 00134 i2c.start(); 00135 i2c.write(id); 00136 i2c.write(addr); 00137 i2c.write(dat); 00138 i2c.stop(); 00139 }
Generated on Sun Jul 17 2022 18:03:01 by
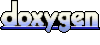