This is a library for the DIGI-DOT-BOOSTER which can control LED stripes using the single wire protocol - ws2812 and compatible as well as RGBW LEDs like SK6812. Detailed information including the datasheet and protocol description are available here: http://www.led-genial.de/DIGI-DOT-Booster-WS2812-und-SK6812-ueber-SPI-Schnittstelle-ansteuern DIGI-DOT-BOOSTER acts as a SPI slave and waits for commands sent by a SPI master. This Library provides an easy to use abstraction layer for commands supported by the DD-Booster and adds some additional effects.
Dependents: DD-Booster-waterdrop BLE_DD-Booster
DDBooster Class Reference
Class acts as a wrapper around SPI calls to control the Digi-Dot-Booster. More...
#include <DDBooster.h>
Public Types | |
enum | LedType |
LED type. More... | |
enum | LedColorOrder |
LED color order. More... | |
Public Member Functions | |
DDBooster (PinName MOSI, PinName SCK, PinName CS, PinName RESET=NC) | |
Default constructor. | |
void | init (uint16_t ledCount, LedType ledType=LED_RGB, LedColorOrder colorOrder=ORDER_GRB) |
Performs initial configuration of the DD-Booster to set the number of used LEDs and their type. | |
void | reset () |
Performs a hardware reset of the DD-Booster by toggling it's RESET pin. | |
void | setRGB (uint8_t r, uint8_t g, uint8_t b) |
Sets a LED color for next operations using RGB format until another color set operation overwrites it. | |
void | setRGBW (uint8_t r, uint8_t g, uint8_t b, uint8_t w) |
Sets a LED color for next operations using RGBW format until another color set operation overwrites it. | |
void | setHSV (uint16_t h, uint8_t s, uint8_t v) |
Sets a LED color for next operations using HSV format until another color set operation overwrites it. | |
void | setLED (uint8_t index) |
Assign the previously set color value to a single LED. | |
void | clearLED (uint8_t index) |
Clears a single LED by setting its color to RGB(0,0,0). | |
void | setAll () |
Assign the previously set color value to a all LEDs. | |
void | clearAll () |
Clears all LEDs by setting their color to RGB(0,0,0). | |
void | setRange (uint8_t start, uint8_t end) |
Assign the previously set color value to a range of LEDs. | |
void | setRainbow (uint16_t h, uint8_t s, uint8_t v, uint8_t start, uint8_t end, uint8_t step) |
Creates a rainbow effect in a range. | |
void | setGradient (int start, int end, uint8_t from[3], uint8_t to[3]) |
Creates a gradient from one color to another. | |
void | shiftUp (uint8_t start, uint8_t end, uint8_t count) |
Shifts up the color values of the LEDs in a range. | |
void | shiftDown (uint8_t start, uint8_t end, uint8_t count) |
Shifts down the color values of the LEDs in a range. | |
void | copyLED (uint8_t from, uint8_t to) |
Copies a color value of a LED to another one. | |
void | repeat (uint8_t start, uint8_t end, uint8_t count) |
Copies the whole range several times in a row. | |
void | show () |
Shows the changes previously made by sending all values to the LEDs. | |
void | sendRawBytes (const uint8_t *buffer, uint8_t length) |
Sends raw byte buffer with commands to DD-Booster. |
Detailed Description
Class acts as a wrapper around SPI calls to control the Digi-Dot-Booster.
After creation of the class instance SPI is configured with default values (12MHz, MSB first, mode 0). Used SPI pins has to be passed to the constructor. RESET pin is optional. If omitted reset() call has no effect.
Before calling any functions you have first to initialize the DD-Booster by calling init() with the number of the LEDs (max. 256 for RGB and max. 192 for RGBW), their type (RGB or RGBW) and the color order (RGB or GRB). Both last parameters are optional - DD-Booster is configured for the ws2812 LEDS (RGB type with GRB color order).
When calling the functions the corresponding values are sent to the DD-Booster, but only after the show() call the LEDs are really addressed with the current state of the values buffer.
Definition at line 25 of file DDBooster.h.
Member Enumeration Documentation
enum LedColorOrder |
LED color order.
ws2812 is using GRB order and it's the default color order for the DD-Booster.
Definition at line 41 of file DDBooster.h.
enum LedType |
LED type.
Stores the number of bits used for the color of one LED. LED_RGB is the default value used by the DD-Booster
Definition at line 32 of file DDBooster.h.
Constructor & Destructor Documentation
DDBooster | ( | PinName | MOSI, |
PinName | SCK, | ||
PinName | CS, | ||
PinName | RESET = NC |
||
) |
Default constructor.
Initializes SPI interface at 12MHz, MSB first, mode 0 Assigns used pins for SPI communication and reset pin to reset DD-Booster. To be able to do a hardware reset of the DD-Booster, connect a digital IO pin to the RESET pin of the DD-Booster.
- Parameters:
-
MOSI - Digital pin of SPI MOSI line SCK - Digital pin of SPI clock CS - Digital pin of SPI chip select resetPin - Digital pin connected to the RESET pin of the DD-Booster, Optional, set to NC if missing
Definition at line 31 of file DDBooster.cpp.
Member Function Documentation
void clearAll | ( | ) |
Clears all LEDs by setting their color to RGB(0,0,0).
Internally it simply sends setRGB(0,0,0) and setAll().
Definition at line 152 of file DDBooster.cpp.
void clearLED | ( | uint8_t | index ) |
Clears a single LED by setting its color to RGB(0,0,0).
Internally it simply sends setRGB(0,0,0) and setLED(index).
- Parameters:
-
index - Index of of the LED to clear. Index starts with 0
Definition at line 129 of file DDBooster.cpp.
void copyLED | ( | uint8_t | from, |
uint8_t | to | ||
) |
Copies a color value of a LED to another one.
- Parameters:
-
from - Index of the LED to copy from to - Index of the LED to copy to
Definition at line 260 of file DDBooster.cpp.
void init | ( | uint16_t | ledCount, |
LedType | ledType = LED_RGB , |
||
LedColorOrder | colorOrder = ORDER_GRB |
||
) |
Performs initial configuration of the DD-Booster to set the number of used LEDs and their type.
DD-Booster supports max. 256 LEDs.
- Parameters:
-
ledCount - Number of used LEDs ledType - Type of LEDs used. RGB is default colorOrder - LED color order. GRB is default
Definition at line 41 of file DDBooster.cpp.
void repeat | ( | uint8_t | start, |
uint8_t | end, | ||
uint8_t | count | ||
) |
Copies the whole range several times in a row.
- Parameters:
-
start - Index of the first LED in the range. Index starts with 0 end - Index of the last LED in the range count - Number of copy operation
Definition at line 273 of file DDBooster.cpp.
void reset | ( | ) |
Performs a hardware reset of the DD-Booster by toggling it's RESET pin.
Does nothing if RESET pin was not configured in the constructor. the call of reset() does nothing.
Definition at line 69 of file DDBooster.cpp.
void sendRawBytes | ( | const uint8_t * | buffer, |
uint8_t | length | ||
) |
Sends raw byte buffer with commands to DD-Booster.
Waits 2ms after transmission.
Definition at line 294 of file DDBooster.cpp.
void setAll | ( | ) |
Assign the previously set color value to a all LEDs.
Definition at line 146 of file DDBooster.cpp.
void setGradient | ( | int | start, |
int | end, | ||
uint8_t | from[3], | ||
uint8_t | to[3] | ||
) |
Creates a gradient from one color to another.
start and end index can have negative values to make a gradient starting outside the visible area showing only it's currently visible part considering the intermediate color values.
- Parameters:
-
start - Index of the first LED in the range. Can be negative. end - Index of the last LED in the range. Can be greater than the number of LEDs from - RGB value of the start color to - RGB value of the end color
Definition at line 199 of file DDBooster.cpp.
void setHSV | ( | uint16_t | h, |
uint8_t | s, | ||
uint8_t | v | ||
) |
Sets a LED color for next operations using HSV format until another color set operation overwrites it.
- Parameters:
-
h - Hue part of the color value (0 - 359) s - Saturation part of the color value (0 - 255) v - Value part of the color value (0 - 255)
Definition at line 102 of file DDBooster.cpp.
void setLED | ( | uint8_t | index ) |
Assign the previously set color value to a single LED.
- Parameters:
-
index - Index of of the LED to set. Index starts with 0
Definition at line 117 of file DDBooster.cpp.
void setRainbow | ( | uint16_t | h, |
uint8_t | s, | ||
uint8_t | v, | ||
uint8_t | start, | ||
uint8_t | end, | ||
uint8_t | step | ||
) |
Creates a rainbow effect in a range.
- Parameters:
-
h - Hue part of the color value (0 - 359) s - Saturation part of the color value (0 - 255) v - Value part of the color value (0 - 255) start - Index of the first LED in the range to set. Index starts with 0 end - Index of the last LED in the range to set step - Step value to increment between 2 LEDs. Recommended values 2 - 20
Definition at line 178 of file DDBooster.cpp.
void setRange | ( | uint8_t | start, |
uint8_t | end | ||
) |
Assign the previously set color value to a range of LEDs.
- Parameters:
-
start - Index of the first LED in the range to set. Index starts with 0 end - Index of the last LED in the range to set
Definition at line 165 of file DDBooster.cpp.
void setRGB | ( | uint8_t | r, |
uint8_t | g, | ||
uint8_t | b | ||
) |
Sets a LED color for next operations using RGB format until another color set operation overwrites it.
- Parameters:
-
r - Red part of the color value (0 - 255) g - Green part of the color value (0 - 255) b - Blue part of the color value (0 - 255)
Definition at line 79 of file DDBooster.cpp.
void setRGBW | ( | uint8_t | r, |
uint8_t | g, | ||
uint8_t | b, | ||
uint8_t | w | ||
) |
Sets a LED color for next operations using RGBW format until another color set operation overwrites it.
- Parameters:
-
r - Red part of the color value (0 - 255) g - Green part of the color value (0 - 255) b - Blue part of the color value (0 - 255) w - White LED level (0 - 255)
Definition at line 90 of file DDBooster.cpp.
void shiftDown | ( | uint8_t | start, |
uint8_t | end, | ||
uint8_t | count | ||
) |
Shifts down the color values of the LEDs in a range.
- Parameters:
-
start - Index of the first LED in the range. Index starts with 0 end - Index of the last LED in the range count - Number of LEDs/steps to shift down
Definition at line 246 of file DDBooster.cpp.
void shiftUp | ( | uint8_t | start, |
uint8_t | end, | ||
uint8_t | count | ||
) |
Shifts up the color values of the LEDs in a range.
- Parameters:
-
start - Index of the first LED in the range. Index starts with 0 end - Index of the last LED in the range count - Number of LEDs/steps to shift up
Definition at line 232 of file DDBooster.cpp.
void show | ( | ) |
Shows the changes previously made by sending all values to the LEDs.
Definition at line 287 of file DDBooster.cpp.
Generated on Tue Jul 12 2022 19:56:05 by
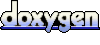