This is a library for the DIGI-DOT-BOOSTER which can control LED stripes using the single wire protocol - ws2812 and compatible as well as RGBW LEDs like SK6812. Detailed information including the datasheet and protocol description are available here: http://www.led-genial.de/DIGI-DOT-Booster-WS2812-und-SK6812-ueber-SPI-Schnittstelle-ansteuern DIGI-DOT-BOOSTER acts as a SPI slave and waits for commands sent by a SPI master. This Library provides an easy to use abstraction layer for commands supported by the DD-Booster and adds some additional effects.
Dependents: DD-Booster-waterdrop BLE_DD-Booster
DDBooster.h
00001 /* 00002 * DDBoster.h - Library to control the Digi-Dot-Booster using a high-level API 00003 * 00004 * https://github.com/Gamadril/DD-Booster-mbed 00005 * MIT License 00006 */ 00007 #ifndef DD_BOOSTER_DDBOOSTER_H 00008 #define DD_BOOSTER_DDBOOSTER_H 00009 00010 #include <mbed.h> 00011 00012 /** 00013 * @brief Class acts as a wrapper around SPI calls to control the Digi-Dot-Booster. 00014 * 00015 * After creation of the class instance SPI is configured with default values (12MHz, MSB first, mode 0). 00016 * Used SPI pins has to be passed to the constructor. RESET pin is optional. If omitted reset() call has no effect. 00017 * 00018 * Before calling any functions you have first to initialize the DD-Booster by calling init() with the number of the LEDs (max. 256 for RGB and max. 192 for RGBW), 00019 * their type (RGB or RGBW) and the color order (RGB or GRB). Both last parameters are optional - DD-Booster is 00020 * configured for the ws2812 LEDS (RGB type with GRB color order). 00021 * 00022 * When calling the functions the corresponding values are sent to the DD-Booster, but only 00023 * after the show() call the LEDs are really addressed with the current state of the values buffer. 00024 */ 00025 class DDBooster { 00026 public: 00027 00028 /** 00029 * LED type. Stores the number of bits used for the color of one LED. 00030 * LED_RGB is the default value used by the DD-Booster 00031 */ 00032 enum LedType { 00033 LED_RGB = 24, 00034 LED_RGBW = 32 00035 }; 00036 00037 /** 00038 * LED color order. ws2812 is using GRB order and it's the default color order 00039 * for the DD-Booster. 00040 */ 00041 enum LedColorOrder { 00042 ORDER_RGB, 00043 ORDER_GRB 00044 }; 00045 00046 /** 00047 * Default constructor. Initializes SPI interface at 12MHz, MSB first, mode 0 00048 * Assigns used pins for SPI communication and reset pin to reset DD-Booster. 00049 * To be able to do a hardware reset of the DD-Booster, connect a digital IO 00050 * pin to the RESET pin of the DD-Booster. 00051 * @param MOSI - Digital pin of SPI MOSI line 00052 * @param SCK - Digital pin of SPI clock 00053 * @param CS - Digital pin of SPI chip select 00054 * @param resetPin - Digital pin connected to the RESET pin of the DD-Booster, Optional, set to NC if missing 00055 */ 00056 DDBooster(PinName MOSI, PinName SCK, PinName CS, PinName RESET = NC); 00057 00058 /** 00059 * Performs initial configuration of the DD-Booster to set the number of used LEDs and their type. 00060 * DD-Booster supports max. 256 LEDs. 00061 * @param ledCount - Number of used LEDs 00062 * @param ledType - Type of LEDs used. RGB is default 00063 * @param colorOrder - LED color order. GRB is default 00064 */ 00065 void init(uint16_t ledCount, LedType ledType = LED_RGB, LedColorOrder colorOrder = ORDER_GRB); 00066 00067 /** 00068 * Performs a hardware reset of the DD-Booster by toggling it's RESET pin. 00069 * Does nothing if RESET pin was not configured in the constructor. 00070 * the call of reset() does nothing. 00071 */ 00072 void reset(); 00073 00074 /** 00075 * Sets a LED color for next operations using RGB format until another color 00076 * set operation overwrites it. 00077 * @param r - Red part of the color value (0 - 255) 00078 * @param g - Green part of the color value (0 - 255) 00079 * @param b - Blue part of the color value (0 - 255) 00080 */ 00081 void setRGB(uint8_t r, uint8_t g, uint8_t b); 00082 00083 /** 00084 * Sets a LED color for next operations using RGBW format until another color 00085 * set operation overwrites it. 00086 * @param r - Red part of the color value (0 - 255) 00087 * @param g - Green part of the color value (0 - 255) 00088 * @param b - Blue part of the color value (0 - 255) 00089 * @param w - White LED level (0 - 255) 00090 */ 00091 void setRGBW(uint8_t r, uint8_t g, uint8_t b, uint8_t w); 00092 00093 /** 00094 * Sets a LED color for next operations using HSV format until another color 00095 * set operation overwrites it. 00096 * @param h - Hue part of the color value (0 - 359) 00097 * @param s - Saturation part of the color value (0 - 255) 00098 * @param v - Value part of the color value (0 - 255) 00099 */ 00100 void setHSV(uint16_t h, uint8_t s, uint8_t v); 00101 00102 /** 00103 * Assign the previously set color value to a single LED. 00104 * @param index - Index of of the LED to set. Index starts with 0 00105 */ 00106 void setLED(uint8_t index); 00107 00108 /** 00109 * Clears a single LED by setting its color to RGB(0,0,0). 00110 * Internally it simply sends setRGB(0,0,0) and setLED(index). 00111 * @param index - Index of of the LED to clear. Index starts with 0 00112 */ 00113 void clearLED(uint8_t index); 00114 00115 /** 00116 * Assign the previously set color value to a all LEDs. 00117 */ 00118 void setAll(); 00119 00120 /** 00121 * Clears all LEDs by setting their color to RGB(0,0,0). 00122 * Internally it simply sends setRGB(0,0,0) and setAll(). 00123 */ 00124 void clearAll(); 00125 00126 /** 00127 * Assign the previously set color value to a range of LEDs. 00128 * @param start - Index of the first LED in the range to set. Index starts with 0 00129 * @param end - Index of the last LED in the range to set 00130 */ 00131 void setRange(uint8_t start, uint8_t end); 00132 00133 /** 00134 * Creates a rainbow effect in a range. 00135 * @param h - Hue part of the color value (0 - 359) 00136 * @param s - Saturation part of the color value (0 - 255) 00137 * @param v - Value part of the color value (0 - 255) 00138 * @param start - Index of the first LED in the range to set. Index starts with 0 00139 * @param end - Index of the last LED in the range to set 00140 * @param step - Step value to increment between 2 LEDs. Recommended values 2 - 20 00141 */ 00142 void setRainbow(uint16_t h, uint8_t s, uint8_t v, uint8_t start, uint8_t end, uint8_t step); 00143 00144 /** 00145 * Creates a gradient from one color to another. start and end index can have negative 00146 * values to make a gradient starting outside the visible area showing only it's 00147 * currently visible part considering the intermediate color values. 00148 * @param start - Index of the first LED in the range. Can be negative. 00149 * @param end - Index of the last LED in the range. Can be greater than the number of LEDs 00150 * @param from - RGB value of the start color 00151 * @param to - RGB value of the end color 00152 */ 00153 void setGradient(int start, int end, uint8_t from[3], uint8_t to[3]); 00154 00155 /** 00156 * Shifts up the color values of the LEDs in a range. 00157 * @param start - Index of the first LED in the range. Index starts with 0 00158 * @param end - Index of the last LED in the range 00159 * @param count - Number of LEDs/steps to shift up 00160 */ 00161 void shiftUp(uint8_t start, uint8_t end, uint8_t count); 00162 00163 /** 00164 * Shifts down the color values of the LEDs in a range. 00165 * @param start - Index of the first LED in the range. Index starts with 0 00166 * @param end - Index of the last LED in the range 00167 * @param count - Number of LEDs/steps to shift down 00168 */ 00169 void shiftDown(uint8_t start, uint8_t end, uint8_t count); 00170 00171 /** 00172 * Copies a color value of a LED to another one. 00173 * @param from - Index of the LED to copy from 00174 * @param to - Index of the LED to copy to 00175 */ 00176 void copyLED(uint8_t from, uint8_t to); 00177 00178 /** 00179 * Copies the whole range several times in a row. 00180 * @param start - Index of the first LED in the range. Index starts with 0 00181 * @param end - Index of the last LED in the range 00182 * @param count - Number of copy operation 00183 */ 00184 void repeat(uint8_t start, uint8_t end, uint8_t count); 00185 00186 /** 00187 * Shows the changes previously made by sending all values to the LEDs. 00188 */ 00189 void show(); 00190 00191 /** 00192 * Sends raw byte buffer with commands to DD-Booster. Waits 2ms after transmission. 00193 */ 00194 void sendRawBytes(const uint8_t* buffer, uint8_t length); 00195 00196 public: 00197 uint8_t _lastIndex; 00198 SPI _device; 00199 DigitalOut _cs; 00200 DigitalOut _reset; 00201 }; 00202 00203 #endif //DD_BOOSTER_DDBOOSTER_H
Generated on Tue Jul 12 2022 19:56:05 by
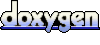