
A basic LCD output test which uses the NXP LPC1768\'s SPI interface to display pixels, characters, and numbers on the Nokia 5110 or Nokia 3310 LCD.
main.cpp
00001 // Project: Nokia5110 - Controlling a NK5110 display from an NXP LPC1768 00002 // File: main.cpp 00003 // Author: Krissi Yan 00004 // Created: January, 2016 00005 // Revised: 00006 // Desc: A basic LCD output test which uses the NXP LPC1768's SPI interface to 00007 // display pixels, characters, and numbers on the Nokia 5110 LCD. 00008 // Created using a sparkfun breakout board with integrated Phillips 8544 driver 00009 // for 48x84 LCDs. 00010 00011 #include "mbed.h" 00012 #include "NOKIA_5110.h" 00013 00014 int main() 00015 { 00016 // Init the data structures and NokiaLcd class 00017 LcdPins myPins; 00018 myPins.sce = p8; 00019 myPins.rst = p9; 00020 myPins.dc = p10; 00021 myPins.mosi = p11; 00022 myPins.miso = NC; 00023 myPins.sclk = p13; 00024 00025 NokiaLcd myLcd( myPins ); 00026 00027 // Start the LCD 00028 myLcd.InitLcd(); 00029 00030 // Draw a test pattern on the LCD and stall for 15 seconds 00031 myLcd.TestLcd( 0xF0 ); 00032 wait( 15 ); 00033 00034 // Turn off the LCD and enter an endless loop 00035 myLcd.ShutdownLcd(); 00036 while( 1 ) 00037 { 00038 //dance 00039 } 00040 }
Generated on Wed Jul 13 2022 23:03:30 by
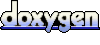