corrected version (with typedef struct IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE_DATA* IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE;) included in the sources
Dependents: STM32F746_iothub_client_sample_mqtt
Fork of iothub_client by
parson.c
00001 /* 00002 Parson ( http://kgabis.github.com/parson/ ) 00003 Copyright (c) 2012 - 2016 Krzysztof Gabis 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 #ifdef _MSC_VER 00024 #ifndef _CRT_SECURE_NO_WARNINGS 00025 #define _CRT_SECURE_NO_WARNINGS 00026 #endif /* _CRT_SECURE_NO_WARNINGS */ 00027 #endif /* _MSC_VER */ 00028 00029 #include "parson.h" 00030 00031 #include <stdio.h> 00032 #include <stdlib.h> 00033 #include <string.h> 00034 #include <ctype.h> 00035 #include <math.h> 00036 00037 #define STARTING_CAPACITY 15 00038 #define ARRAY_MAX_CAPACITY 122880 /* 15*(2^13) */ 00039 #define OBJECT_MAX_CAPACITY 960 /* 15*(2^6) */ 00040 #define MAX_NESTING 19 00041 #define DOUBLE_SERIALIZATION_FORMAT "%f" 00042 00043 #define SIZEOF_TOKEN(a) (sizeof(a) - 1) 00044 #define SKIP_CHAR(str) ((*str)++) 00045 #define SKIP_WHITESPACES(str) while (isspace(**str)) { SKIP_CHAR(str); } 00046 #define MAX(a, b) ((a) > (b) ? (a) : (b)) 00047 00048 #undef malloc 00049 #undef free 00050 00051 static JSON_Malloc_Function parson_malloc = malloc; 00052 static JSON_Free_Function parson_free = free; 00053 00054 #define IS_CONT(b) (((unsigned char)(b) & 0xC0) == 0x80) /* is utf-8 continuation byte */ 00055 00056 /* Type definitions */ 00057 typedef union json_value_value { 00058 char *string; 00059 double number; 00060 JSON_Object *object; 00061 JSON_Array *array; 00062 int boolean; 00063 int null; 00064 } JSON_Value_Value; 00065 00066 struct json_value_t { 00067 JSON_Value_Type type; 00068 JSON_Value_Value value; 00069 }; 00070 00071 struct json_object_t { 00072 char **names; 00073 JSON_Value **values; 00074 size_t count; 00075 size_t capacity; 00076 }; 00077 00078 struct json_array_t { 00079 JSON_Value **items; 00080 size_t count; 00081 size_t capacity; 00082 }; 00083 00084 /* Various */ 00085 static char * read_file(const char *filename); 00086 static void remove_comments(char *string, const char *start_token, const char *end_token); 00087 static char * parson_strndup(const char *string, size_t n); 00088 static char * parson_strdup(const char *string); 00089 static int is_utf16_hex(const unsigned char *string); 00090 static int num_bytes_in_utf8_sequence(unsigned char c); 00091 static int verify_utf8_sequence(const unsigned char *string, int *len); 00092 static int is_valid_utf8(const char *string, size_t string_len); 00093 static int is_decimal(const char *string, size_t length); 00094 00095 /* JSON Object */ 00096 static JSON_Object * json_object_init(void); 00097 static JSON_Status json_object_add(JSON_Object *object, const char *name, JSON_Value *value); 00098 static JSON_Status json_object_resize(JSON_Object *object, size_t new_capacity); 00099 static JSON_Value * json_object_nget_value(const JSON_Object *object, const char *name, size_t n); 00100 static void json_object_free(JSON_Object *object); 00101 00102 /* JSON Array */ 00103 static JSON_Array * json_array_init(void); 00104 static JSON_Status json_array_add(JSON_Array *array, JSON_Value *value); 00105 static JSON_Status json_array_resize(JSON_Array *array, size_t new_capacity); 00106 static void json_array_free(JSON_Array *array); 00107 00108 /* JSON Value */ 00109 static JSON_Value * json_value_init_string_no_copy(char *string); 00110 00111 /* Parser */ 00112 static void skip_quotes(const char **string); 00113 static int parse_utf_16(const char **unprocessed, char **processed); 00114 static char * process_string(const char *input, size_t len); 00115 static char * get_quoted_string(const char **string); 00116 static JSON_Value * parse_object_value(const char **string, size_t nesting); 00117 static JSON_Value * parse_array_value(const char **string, size_t nesting); 00118 static JSON_Value * parse_string_value(const char **string); 00119 static JSON_Value * parse_boolean_value(const char **string); 00120 static JSON_Value * parse_number_value(const char **string); 00121 static JSON_Value * parse_null_value(const char **string); 00122 static JSON_Value * parse_value(const char **string, size_t nesting); 00123 00124 /* Serialization */ 00125 static int json_serialize_to_buffer_r(const JSON_Value *value, char *buf, int level, int is_pretty, char *num_buf); 00126 static int json_serialize_string(const char *string, char *buf); 00127 static int append_indent(char *buf, int level); 00128 static int append_string(char *buf, const char *string); 00129 00130 /* Various */ 00131 static char * parson_strndup(const char *string, size_t n) { 00132 char *output_string = (char*)parson_malloc(n + 1); 00133 if (!output_string) 00134 return NULL; 00135 output_string[n] = '\0'; 00136 strncpy(output_string, string, n); 00137 return output_string; 00138 } 00139 00140 static char * parson_strdup(const char *string) { 00141 return parson_strndup(string, strlen(string)); 00142 } 00143 00144 static int is_utf16_hex(const unsigned char *s) { 00145 return isxdigit(s[0]) && isxdigit(s[1]) && isxdigit(s[2]) && isxdigit(s[3]); 00146 } 00147 00148 static int num_bytes_in_utf8_sequence(unsigned char c) { 00149 if (c == 0xC0 || c == 0xC1 || c > 0xF4 || IS_CONT(c)) { 00150 return 0; 00151 } else if ((c & 0x80) == 0) { /* 0xxxxxxx */ 00152 return 1; 00153 } else if ((c & 0xE0) == 0xC0) { /* 110xxxxx */ 00154 return 2; 00155 } else if ((c & 0xF0) == 0xE0) { /* 1110xxxx */ 00156 return 3; 00157 } else if ((c & 0xF8) == 0xF0) { /* 11110xxx */ 00158 return 4; 00159 } 00160 return 0; /* won't happen */ 00161 } 00162 00163 static int verify_utf8_sequence(const unsigned char *string, int *len) { 00164 unsigned int cp = 0; 00165 *len = num_bytes_in_utf8_sequence(string[0]); 00166 00167 if (*len == 1) { 00168 cp = string[0]; 00169 } else if (*len == 2 && IS_CONT(string[1])) { 00170 cp = string[0] & 0x1F; 00171 cp = (cp << 6) | (string[1] & 0x3F); 00172 } else if (*len == 3 && IS_CONT(string[1]) && IS_CONT(string[2])) { 00173 cp = ((unsigned char)string[0]) & 0xF; 00174 cp = (cp << 6) | (string[1] & 0x3F); 00175 cp = (cp << 6) | (string[2] & 0x3F); 00176 } else if (*len == 4 && IS_CONT(string[1]) && IS_CONT(string[2]) && IS_CONT(string[3])) { 00177 cp = string[0] & 0x7; 00178 cp = (cp << 6) | (string[1] & 0x3F); 00179 cp = (cp << 6) | (string[2] & 0x3F); 00180 cp = (cp << 6) | (string[3] & 0x3F); 00181 } else { 00182 return 0; 00183 } 00184 00185 /* overlong encodings */ 00186 if ((cp < 0x80 && *len > 1) || 00187 (cp < 0x800 && *len > 2) || 00188 (cp < 0x10000 && *len > 3)) { 00189 return 0; 00190 } 00191 00192 /* invalid unicode */ 00193 if (cp > 0x10FFFF) { 00194 return 0; 00195 } 00196 00197 /* surrogate halves */ 00198 if (cp >= 0xD800 && cp <= 0xDFFF) { 00199 return 0; 00200 } 00201 00202 return 1; 00203 } 00204 00205 static int is_valid_utf8(const char *string, size_t string_len) { 00206 int len = 0; 00207 const char *string_end = string + string_len; 00208 while (string < string_end) { 00209 if (!verify_utf8_sequence((const unsigned char*)string, &len)) { 00210 return 0; 00211 } 00212 string += len; 00213 } 00214 return 1; 00215 } 00216 00217 static int is_decimal(const char *string, size_t length) { 00218 if (length > 1 && string[0] == '0' && string[1] != '.') 00219 return 0; 00220 if (length > 2 && !strncmp(string, "-0", 2) && string[2] != '.') 00221 return 0; 00222 while (length--) 00223 if (strchr("xX", string[length])) 00224 return 0; 00225 return 1; 00226 } 00227 00228 static char * read_file(const char * filename) { 00229 FILE *fp = fopen(filename, "r"); 00230 size_t file_size; 00231 long pos; 00232 char *file_contents; 00233 if (!fp) 00234 return NULL; 00235 fseek(fp, 0L, SEEK_END); 00236 pos = ftell(fp); 00237 if (pos < 0) { 00238 fclose(fp); 00239 return NULL; 00240 } 00241 file_size = pos; 00242 rewind(fp); 00243 file_contents = (char*)parson_malloc(sizeof(char) * (file_size + 1)); 00244 if (!file_contents) { 00245 fclose(fp); 00246 return NULL; 00247 } 00248 if (fread(file_contents, file_size, 1, fp) < 1) { 00249 if (ferror(fp)) { 00250 fclose(fp); 00251 parson_free(file_contents); 00252 return NULL; 00253 } 00254 } 00255 fclose(fp); 00256 file_contents[file_size] = '\0'; 00257 return file_contents; 00258 } 00259 00260 static void remove_comments(char *string, const char *start_token, const char *end_token) { 00261 int in_string = 0, escaped = 0; 00262 size_t i; 00263 char *ptr = NULL, current_char; 00264 size_t start_token_len = strlen(start_token); 00265 size_t end_token_len = strlen(end_token); 00266 if (start_token_len == 0 || end_token_len == 0) 00267 return; 00268 while ((current_char = *string) != '\0') { 00269 if (current_char == '\\' && !escaped) { 00270 escaped = 1; 00271 string++; 00272 continue; 00273 } else if (current_char == '\"' && !escaped) { 00274 in_string = !in_string; 00275 } else if (!in_string && strncmp(string, start_token, start_token_len) == 0) { 00276 for(i = 0; i < start_token_len; i++) 00277 string[i] = ' '; 00278 string = string + start_token_len; 00279 ptr = strstr(string, end_token); 00280 if (!ptr) 00281 return; 00282 for (i = 0; i < (ptr - string) + end_token_len; i++) 00283 string[i] = ' '; 00284 string = ptr + end_token_len - 1; 00285 } 00286 escaped = 0; 00287 string++; 00288 } 00289 } 00290 00291 /* JSON Object */ 00292 static JSON_Object * json_object_init(void) { 00293 JSON_Object *new_obj = (JSON_Object*)parson_malloc(sizeof(JSON_Object)); 00294 if (!new_obj) 00295 return NULL; 00296 new_obj->names = (char**)NULL; 00297 new_obj->values = (JSON_Value**)NULL; 00298 new_obj->capacity = 0; 00299 new_obj->count = 0; 00300 return new_obj; 00301 } 00302 00303 static JSON_Status json_object_add(JSON_Object *object, const char *name, JSON_Value *value) { 00304 size_t index = 0; 00305 if (object == NULL || name == NULL || value == NULL) { 00306 return JSONFailure; 00307 } 00308 if (json_object_get_value(object, name) != NULL) { 00309 return JSONFailure; 00310 } 00311 if (object->count >= object->capacity) { 00312 size_t new_capacity = MAX(object->capacity * 2, STARTING_CAPACITY); 00313 if (new_capacity > OBJECT_MAX_CAPACITY) 00314 return JSONFailure; 00315 if (json_object_resize(object, new_capacity) == JSONFailure) 00316 return JSONFailure; 00317 } 00318 index = object->count; 00319 object->names[index] = parson_strdup(name); 00320 if (object->names[index] == NULL) 00321 return JSONFailure; 00322 object->values[index] = value; 00323 object->count++; 00324 return JSONSuccess; 00325 } 00326 00327 static JSON_Status json_object_resize(JSON_Object *object, size_t new_capacity) { 00328 char **temp_names = NULL; 00329 JSON_Value **temp_values = NULL; 00330 00331 if ((object->names == NULL && object->values != NULL) || 00332 (object->names != NULL && object->values == NULL) || 00333 new_capacity == 0) { 00334 return JSONFailure; /* Shouldn't happen */ 00335 } 00336 00337 temp_names = (char**)parson_malloc(new_capacity * sizeof(char*)); 00338 if (temp_names == NULL) 00339 return JSONFailure; 00340 00341 temp_values = (JSON_Value**)parson_malloc(new_capacity * sizeof(JSON_Value*)); 00342 if (temp_values == NULL) { 00343 parson_free(temp_names); 00344 return JSONFailure; 00345 } 00346 00347 if (object->names != NULL && object->values != NULL && object->count > 0) { 00348 memcpy(temp_names, object->names, object->count * sizeof(char*)); 00349 memcpy(temp_values, object->values, object->count * sizeof(JSON_Value*)); 00350 } 00351 parson_free(object->names); 00352 parson_free(object->values); 00353 object->names = temp_names; 00354 object->values = temp_values; 00355 object->capacity = new_capacity; 00356 return JSONSuccess; 00357 } 00358 00359 static JSON_Value * json_object_nget_value(const JSON_Object *object, const char *name, size_t n) { 00360 size_t i, name_length; 00361 for (i = 0; i < json_object_get_count(object); i++) { 00362 name_length = strlen(object->names[i]); 00363 if (name_length != n) 00364 continue; 00365 if (strncmp(object->names[i], name, n) == 0) 00366 return object->values[i]; 00367 } 00368 return NULL; 00369 } 00370 00371 static void json_object_free(JSON_Object *object) { 00372 while(object->count--) { 00373 parson_free(object->names[object->count]); 00374 json_value_free(object->values[object->count]); 00375 } 00376 parson_free(object->names); 00377 parson_free(object->values); 00378 parson_free(object); 00379 } 00380 00381 /* JSON Array */ 00382 static JSON_Array * json_array_init(void) { 00383 JSON_Array *new_array = (JSON_Array*)parson_malloc(sizeof(JSON_Array)); 00384 if (!new_array) 00385 return NULL; 00386 new_array->items = (JSON_Value**)NULL; 00387 new_array->capacity = 0; 00388 new_array->count = 0; 00389 return new_array; 00390 } 00391 00392 static JSON_Status json_array_add(JSON_Array *array, JSON_Value *value) { 00393 if (array->count >= array->capacity) { 00394 size_t new_capacity = MAX(array->capacity * 2, STARTING_CAPACITY); 00395 if (new_capacity > ARRAY_MAX_CAPACITY) 00396 return JSONFailure; 00397 if (json_array_resize(array, new_capacity) == JSONFailure) 00398 return JSONFailure; 00399 } 00400 array->items[array->count] = value; 00401 array->count++; 00402 return JSONSuccess; 00403 } 00404 00405 static JSON_Status json_array_resize(JSON_Array *array, size_t new_capacity) { 00406 JSON_Value **new_items = NULL; 00407 if (new_capacity == 0) { 00408 return JSONFailure; 00409 } 00410 new_items = (JSON_Value**)parson_malloc(new_capacity * sizeof(JSON_Value*)); 00411 if (new_items == NULL) { 00412 return JSONFailure; 00413 } 00414 if (array->items != NULL && array->count > 0) { 00415 memcpy(new_items, array->items, array->count * sizeof(JSON_Value*)); 00416 } 00417 parson_free(array->items); 00418 array->items = new_items; 00419 array->capacity = new_capacity; 00420 return JSONSuccess; 00421 } 00422 00423 static void json_array_free(JSON_Array *array) { 00424 while (array->count--) 00425 json_value_free(array->items[array->count]); 00426 parson_free(array->items); 00427 parson_free(array); 00428 } 00429 00430 /* JSON Value */ 00431 static JSON_Value * json_value_init_string_no_copy(char *string) { 00432 JSON_Value *new_value = (JSON_Value*)parson_malloc(sizeof(JSON_Value)); 00433 if (!new_value) 00434 return NULL; 00435 new_value->type = JSONString; 00436 new_value->value.string = string; 00437 return new_value; 00438 } 00439 00440 /* Parser */ 00441 static void skip_quotes(const char **string) { 00442 SKIP_CHAR(string); 00443 while (**string != '\"') { 00444 if (**string == '\0') 00445 return; 00446 if (**string == '\\') { 00447 SKIP_CHAR(string); 00448 if (**string == '\0') 00449 return; 00450 } 00451 SKIP_CHAR(string); 00452 } 00453 SKIP_CHAR(string); 00454 } 00455 00456 static int parse_utf_16(const char **unprocessed, char **processed) { 00457 unsigned int cp, lead, trail; 00458 char *processed_ptr = *processed; 00459 const char *unprocessed_ptr = *unprocessed; 00460 unprocessed_ptr++; /* skips u */ 00461 if (!is_utf16_hex((const unsigned char*)unprocessed_ptr) || sscanf(unprocessed_ptr, "%4x", &cp) == EOF) 00462 return JSONFailure; 00463 if (cp < 0x80) { 00464 *processed_ptr = cp; /* 0xxxxxxx */ 00465 } else if (cp < 0x800) { 00466 *processed_ptr++ = ((cp >> 6) & 0x1F) | 0xC0; /* 110xxxxx */ 00467 *processed_ptr = ((cp ) & 0x3F) | 0x80; /* 10xxxxxx */ 00468 } else if (cp < 0xD800 || cp > 0xDFFF) { 00469 *processed_ptr++ = ((cp >> 12) & 0x0F) | 0xE0; /* 1110xxxx */ 00470 *processed_ptr++ = ((cp >> 6) & 0x3F) | 0x80; /* 10xxxxxx */ 00471 *processed_ptr = ((cp ) & 0x3F) | 0x80; /* 10xxxxxx */ 00472 } else if (cp >= 0xD800 && cp <= 0xDBFF) { /* lead surrogate (0xD800..0xDBFF) */ 00473 lead = cp; 00474 unprocessed_ptr += 4; /* should always be within the buffer, otherwise previous sscanf would fail */ 00475 if (*unprocessed_ptr++ != '\\' || *unprocessed_ptr++ != 'u' || /* starts with \u? */ 00476 !is_utf16_hex((const unsigned char*)unprocessed_ptr) || 00477 sscanf(unprocessed_ptr, "%4x", &trail) == EOF || 00478 trail < 0xDC00 || trail > 0xDFFF) { /* valid trail surrogate? (0xDC00..0xDFFF) */ 00479 return JSONFailure; 00480 } 00481 cp = ((((lead-0xD800)&0x3FF)<<10)|((trail-0xDC00)&0x3FF))+0x010000; 00482 *processed_ptr++ = (((cp >> 18) & 0x07) | 0xF0); /* 11110xxx */ 00483 *processed_ptr++ = (((cp >> 12) & 0x3F) | 0x80); /* 10xxxxxx */ 00484 *processed_ptr++ = (((cp >> 6) & 0x3F) | 0x80); /* 10xxxxxx */ 00485 *processed_ptr = (((cp ) & 0x3F) | 0x80); /* 10xxxxxx */ 00486 } else { /* trail surrogate before lead surrogate */ 00487 return JSONFailure; 00488 } 00489 unprocessed_ptr += 3; 00490 *processed = processed_ptr; 00491 *unprocessed = unprocessed_ptr; 00492 return JSONSuccess; 00493 } 00494 00495 00496 /* Copies and processes passed string up to supplied length. 00497 Example: "\u006Corem ipsum" -> lorem ipsum */ 00498 static char* process_string(const char *input, size_t len) { 00499 const char *input_ptr = input; 00500 size_t initial_size = (len + 1) * sizeof(char); 00501 size_t final_size = 0; 00502 char *output = (char*)parson_malloc(initial_size); 00503 char *output_ptr = output; 00504 char *resized_output = NULL; 00505 while ((*input_ptr != '\0') && (size_t)(input_ptr - input) < len) { 00506 if (*input_ptr == '\\') { 00507 input_ptr++; 00508 switch (*input_ptr) { 00509 case '\"': *output_ptr = '\"'; break; 00510 case '\\': *output_ptr = '\\'; break; 00511 case '/': *output_ptr = '/'; break; 00512 case 'b': *output_ptr = '\b'; break; 00513 case 'f': *output_ptr = '\f'; break; 00514 case 'n': *output_ptr = '\n'; break; 00515 case 'r': *output_ptr = '\r'; break; 00516 case 't': *output_ptr = '\t'; break; 00517 case 'u': 00518 if (parse_utf_16(&input_ptr, &output_ptr) == JSONFailure) 00519 goto error; 00520 break; 00521 default: 00522 goto error; 00523 } 00524 } else if ((unsigned char)*input_ptr < 0x20) { 00525 goto error; /* 0x00-0x19 are invalid characters for json string (http://www.ietf.org/rfc/rfc4627.txt) */ 00526 } else { 00527 *output_ptr = *input_ptr; 00528 } 00529 output_ptr++; 00530 input_ptr++; 00531 } 00532 *output_ptr = '\0'; 00533 /* resize to new length */ 00534 final_size = (size_t)(output_ptr-output) + 1; 00535 resized_output = (char*)parson_malloc(final_size); 00536 if (resized_output == NULL) 00537 goto error; 00538 memcpy(resized_output, output, final_size); 00539 parson_free(output); 00540 return resized_output; 00541 error: 00542 parson_free(output); 00543 return NULL; 00544 } 00545 00546 /* Return processed contents of a string between quotes and 00547 skips passed argument to a matching quote. */ 00548 static char * get_quoted_string(const char **string) { 00549 const char *string_start = *string; 00550 size_t string_len = 0; 00551 skip_quotes(string); 00552 string_len = *string - string_start - 2; /* length without quotes */ 00553 return process_string(string_start + 1, string_len); 00554 } 00555 00556 static JSON_Value * parse_value(const char **string, size_t nesting) { 00557 if (nesting > MAX_NESTING) 00558 return NULL; 00559 SKIP_WHITESPACES(string); 00560 switch (**string) { 00561 case '{': 00562 return parse_object_value(string, nesting + 1); 00563 case '[': 00564 return parse_array_value(string, nesting + 1); 00565 case '\"': 00566 return parse_string_value(string); 00567 case 'f': case 't': 00568 return parse_boolean_value(string); 00569 case '-': 00570 case '0': case '1': case '2': case '3': case '4': 00571 case '5': case '6': case '7': case '8': case '9': 00572 return parse_number_value(string); 00573 case 'n': 00574 return parse_null_value(string); 00575 default: 00576 return NULL; 00577 } 00578 } 00579 00580 static JSON_Value * parse_object_value(const char **string, size_t nesting) { 00581 JSON_Value *output_value = json_value_init_object(), *new_value = NULL; 00582 JSON_Object *output_object = json_value_get_object(output_value); 00583 char *new_key = NULL; 00584 if (output_value == NULL) 00585 return NULL; 00586 SKIP_CHAR(string); 00587 SKIP_WHITESPACES(string); 00588 if (**string == '}') { /* empty object */ 00589 SKIP_CHAR(string); 00590 return output_value; 00591 } 00592 while (**string != '\0') { 00593 new_key = get_quoted_string(string); 00594 SKIP_WHITESPACES(string); 00595 if (new_key == NULL || **string != ':') { 00596 json_value_free(output_value); 00597 return NULL; 00598 } 00599 SKIP_CHAR(string); 00600 new_value = parse_value(string, nesting); 00601 if (new_value == NULL) { 00602 parson_free(new_key); 00603 json_value_free(output_value); 00604 return NULL; 00605 } 00606 if(json_object_add(output_object, new_key, new_value) == JSONFailure) { 00607 parson_free(new_key); 00608 parson_free(new_value); 00609 json_value_free(output_value); 00610 return NULL; 00611 } 00612 parson_free(new_key); 00613 SKIP_WHITESPACES(string); 00614 if (**string != ',') 00615 break; 00616 SKIP_CHAR(string); 00617 SKIP_WHITESPACES(string); 00618 } 00619 SKIP_WHITESPACES(string); 00620 if (**string != '}' || /* Trim object after parsing is over */ 00621 json_object_resize(output_object, json_object_get_count(output_object)) == JSONFailure) { 00622 json_value_free(output_value); 00623 return NULL; 00624 } 00625 SKIP_CHAR(string); 00626 return output_value; 00627 } 00628 00629 static JSON_Value * parse_array_value(const char **string, size_t nesting) { 00630 JSON_Value *output_value = json_value_init_array(), *new_array_value = NULL; 00631 JSON_Array *output_array = json_value_get_array(output_value); 00632 if (!output_value) 00633 return NULL; 00634 SKIP_CHAR(string); 00635 SKIP_WHITESPACES(string); 00636 if (**string == ']') { /* empty array */ 00637 SKIP_CHAR(string); 00638 return output_value; 00639 } 00640 while (**string != '\0') { 00641 new_array_value = parse_value(string, nesting); 00642 if (!new_array_value) { 00643 json_value_free(output_value); 00644 return NULL; 00645 } 00646 if(json_array_add(output_array, new_array_value) == JSONFailure) { 00647 parson_free(new_array_value); 00648 json_value_free(output_value); 00649 return NULL; 00650 } 00651 SKIP_WHITESPACES(string); 00652 if (**string != ',') 00653 break; 00654 SKIP_CHAR(string); 00655 SKIP_WHITESPACES(string); 00656 } 00657 SKIP_WHITESPACES(string); 00658 if (**string != ']' || /* Trim array after parsing is over */ 00659 json_array_resize(output_array, json_array_get_count(output_array)) == JSONFailure) { 00660 json_value_free(output_value); 00661 return NULL; 00662 } 00663 SKIP_CHAR(string); 00664 return output_value; 00665 } 00666 00667 static JSON_Value * parse_string_value(const char **string) { 00668 JSON_Value *value = NULL; 00669 char *new_string = get_quoted_string(string); 00670 if (new_string == NULL) 00671 return NULL; 00672 value = json_value_init_string_no_copy(new_string); 00673 if (value == NULL) { 00674 parson_free(new_string); 00675 return NULL; 00676 } 00677 return value; 00678 } 00679 00680 static JSON_Value * parse_boolean_value(const char **string) { 00681 size_t true_token_size = SIZEOF_TOKEN("true"); 00682 size_t false_token_size = SIZEOF_TOKEN("false"); 00683 if (strncmp("true", *string, true_token_size) == 0) { 00684 *string += true_token_size; 00685 return json_value_init_boolean(1); 00686 } else if (strncmp("false", *string, false_token_size) == 0) { 00687 *string += false_token_size; 00688 return json_value_init_boolean(0); 00689 } 00690 return NULL; 00691 } 00692 00693 static JSON_Value * parse_number_value(const char **string) { 00694 char *end; 00695 double number = strtod(*string, &end); 00696 JSON_Value *output_value; 00697 if (is_decimal(*string, end - *string)) { 00698 *string = end; 00699 output_value = json_value_init_number(number); 00700 } else { 00701 output_value = NULL; 00702 } 00703 return output_value; 00704 } 00705 00706 static JSON_Value * parse_null_value(const char **string) { 00707 size_t token_size = SIZEOF_TOKEN("null"); 00708 if (strncmp("null", *string, token_size) == 0) { 00709 *string += token_size; 00710 return json_value_init_null(); 00711 } 00712 return NULL; 00713 } 00714 00715 /* Serialization */ 00716 #define APPEND_STRING(str) do { written = append_string(buf, (str));\ 00717 if (written < 0) { return -1; }\ 00718 if (buf != NULL) { buf += written; }\ 00719 written_total += written; } while(0) 00720 00721 #define APPEND_INDENT(level) do { written = append_indent(buf, (level));\ 00722 if (written < 0) { return -1; }\ 00723 if (buf != NULL) { buf += written; }\ 00724 written_total += written; } while(0) 00725 00726 static int json_serialize_to_buffer_r(const JSON_Value *value, char *buf, int level, int is_pretty, char *num_buf) 00727 { 00728 const char *key = NULL, *string = NULL; 00729 JSON_Value *temp_value = NULL; 00730 JSON_Array *array = NULL; 00731 JSON_Object *object = NULL; 00732 size_t i = 0, count = 0; 00733 double num = 0.0; 00734 int written = -1, written_total = 0; 00735 00736 switch (json_value_get_type(value)) { 00737 case JSONArray: 00738 array = json_value_get_array(value); 00739 count = json_array_get_count(array); 00740 APPEND_STRING("["); 00741 if (count > 0 && is_pretty) 00742 APPEND_STRING("\n"); 00743 for (i = 0; i < count; i++) { 00744 if (is_pretty) 00745 APPEND_INDENT(level+1); 00746 temp_value = json_array_get_value(array, i); 00747 written = json_serialize_to_buffer_r(temp_value, buf, level+1, is_pretty, num_buf); 00748 if (written < 0) 00749 return -1; 00750 if (buf != NULL) 00751 buf += written; 00752 written_total += written; 00753 if (i < (count - 1)) 00754 APPEND_STRING(","); 00755 if (is_pretty) 00756 APPEND_STRING("\n"); 00757 } 00758 if (count > 0 && is_pretty) 00759 APPEND_INDENT(level); 00760 APPEND_STRING("]"); 00761 return written_total; 00762 case JSONObject: 00763 object = json_value_get_object(value); 00764 count = json_object_get_count(object); 00765 APPEND_STRING("{"); 00766 if (count > 0 && is_pretty) 00767 APPEND_STRING("\n"); 00768 for (i = 0; i < count; i++) { 00769 key = json_object_get_name(object, i); 00770 if (is_pretty) 00771 APPEND_INDENT(level+1); 00772 written = json_serialize_string(key, buf); 00773 if (written < 0) 00774 return -1; 00775 if (buf != NULL) 00776 buf += written; 00777 written_total += written; 00778 APPEND_STRING(":"); 00779 if (is_pretty) 00780 APPEND_STRING(" "); 00781 temp_value = json_object_get_value(object, key); 00782 written = json_serialize_to_buffer_r(temp_value, buf, level+1, is_pretty, num_buf); 00783 if (written < 0) 00784 return -1; 00785 if (buf != NULL) 00786 buf += written; 00787 written_total += written; 00788 if (i < (count - 1)) 00789 APPEND_STRING(","); 00790 if (is_pretty) 00791 APPEND_STRING("\n"); 00792 } 00793 if (count > 0 && is_pretty) 00794 APPEND_INDENT(level); 00795 APPEND_STRING("}"); 00796 return written_total; 00797 case JSONString: 00798 string = json_value_get_string(value); 00799 written = json_serialize_string(string, buf); 00800 if (written < 0) 00801 return -1; 00802 if (buf != NULL) 00803 buf += written; 00804 written_total += written; 00805 return written_total; 00806 case JSONBoolean: 00807 if (json_value_get_boolean(value)) 00808 APPEND_STRING("true"); 00809 else 00810 APPEND_STRING("false"); 00811 return written_total; 00812 case JSONNumber: 00813 num = json_value_get_number(value); 00814 if (buf != NULL) 00815 num_buf = buf; 00816 if (num == ((double)(int)num)) /* check if num is integer */ 00817 written = sprintf(num_buf, "%d", (int)num); 00818 else 00819 written = sprintf(num_buf, DOUBLE_SERIALIZATION_FORMAT, num); 00820 if (written < 0) 00821 return -1; 00822 if (buf != NULL) 00823 buf += written; 00824 written_total += written; 00825 return written_total; 00826 case JSONNull: 00827 APPEND_STRING("null"); 00828 return written_total; 00829 case JSONError: 00830 return -1; 00831 default: 00832 return -1; 00833 } 00834 } 00835 00836 static int json_serialize_string(const char *string, char *buf) { 00837 size_t i = 0, len = strlen(string); 00838 char c = '\0'; 00839 int written = -1, written_total = 0; 00840 APPEND_STRING("\""); 00841 for (i = 0; i < len; i++) { 00842 c = string[i]; 00843 switch (c) { 00844 case '\"': APPEND_STRING("\\\""); break; 00845 case '\\': APPEND_STRING("\\\\"); break; 00846 case '/': APPEND_STRING("\\/"); break; /* to make json embeddable in xml\/html */ 00847 case '\b': APPEND_STRING("\\b"); break; 00848 case '\f': APPEND_STRING("\\f"); break; 00849 case '\n': APPEND_STRING("\\n"); break; 00850 case '\r': APPEND_STRING("\\r"); break; 00851 case '\t': APPEND_STRING("\\t"); break; 00852 default: 00853 if (buf != NULL) { 00854 buf[0] = c; 00855 buf += 1; 00856 } 00857 written_total += 1; 00858 break; 00859 } 00860 } 00861 APPEND_STRING("\""); 00862 return written_total; 00863 } 00864 00865 static int append_indent(char *buf, int level) { 00866 int i; 00867 int written = -1, written_total = 0; 00868 for (i = 0; i < level; i++) { 00869 APPEND_STRING(" "); 00870 } 00871 return written_total; 00872 } 00873 00874 static int append_string(char *buf, const char *string) { 00875 if (buf == NULL) { 00876 return (int)strlen(string); 00877 } 00878 return sprintf(buf, "%s", string); 00879 } 00880 00881 #undef APPEND_STRING 00882 #undef APPEND_INDENT 00883 00884 /* Parser API */ 00885 JSON_Value * json_parse_file(const char *filename) { 00886 char *file_contents = read_file(filename); 00887 JSON_Value *output_value = NULL; 00888 if (file_contents == NULL) 00889 return NULL; 00890 output_value = json_parse_string(file_contents); 00891 parson_free(file_contents); 00892 return output_value; 00893 } 00894 00895 JSON_Value * json_parse_file_with_comments(const char *filename) { 00896 char *file_contents = read_file(filename); 00897 JSON_Value *output_value = NULL; 00898 if (file_contents == NULL) 00899 return NULL; 00900 output_value = json_parse_string_with_comments(file_contents); 00901 parson_free(file_contents); 00902 return output_value; 00903 } 00904 00905 JSON_Value * json_parse_string(const char *string) { 00906 if (string == NULL) 00907 return NULL; 00908 return parse_value((const char**)&string, 0); 00909 } 00910 00911 JSON_Value * json_parse_string_with_comments(const char *string) { 00912 JSON_Value *result = NULL; 00913 char *string_mutable_copy = NULL, *string_mutable_copy_ptr = NULL; 00914 string_mutable_copy = parson_strdup(string); 00915 if (string_mutable_copy == NULL) 00916 return NULL; 00917 remove_comments(string_mutable_copy, "/*", "*/"); 00918 remove_comments(string_mutable_copy, "//", "\n"); 00919 string_mutable_copy_ptr = string_mutable_copy; 00920 result = parse_value((const char**)&string_mutable_copy_ptr, 0); 00921 parson_free(string_mutable_copy); 00922 return result; 00923 } 00924 00925 /* JSON Object API */ 00926 00927 JSON_Value * json_object_get_value(const JSON_Object *object, const char *name) { 00928 if (object == NULL || name == NULL) 00929 return NULL; 00930 return json_object_nget_value(object, name, strlen(name)); 00931 } 00932 00933 const char * json_object_get_string(const JSON_Object *object, const char *name) { 00934 return json_value_get_string(json_object_get_value(object, name)); 00935 } 00936 00937 double json_object_get_number(const JSON_Object *object, const char *name) { 00938 return json_value_get_number(json_object_get_value(object, name)); 00939 } 00940 00941 JSON_Object * json_object_get_object(const JSON_Object *object, const char *name) { 00942 return json_value_get_object(json_object_get_value(object, name)); 00943 } 00944 00945 JSON_Array * json_object_get_array(const JSON_Object *object, const char *name) { 00946 return json_value_get_array(json_object_get_value(object, name)); 00947 } 00948 00949 int json_object_get_boolean(const JSON_Object *object, const char *name) { 00950 return json_value_get_boolean(json_object_get_value(object, name)); 00951 } 00952 00953 JSON_Value * json_object_dotget_value(const JSON_Object *object, const char *name) { 00954 const char *dot_position = strchr(name, '.'); 00955 if (!dot_position) 00956 return json_object_get_value(object, name); 00957 object = json_value_get_object(json_object_nget_value(object, name, dot_position - name)); 00958 return json_object_dotget_value(object, dot_position + 1); 00959 } 00960 00961 const char * json_object_dotget_string(const JSON_Object *object, const char *name) { 00962 return json_value_get_string(json_object_dotget_value(object, name)); 00963 } 00964 00965 double json_object_dotget_number(const JSON_Object *object, const char *name) { 00966 return json_value_get_number(json_object_dotget_value(object, name)); 00967 } 00968 00969 JSON_Object * json_object_dotget_object(const JSON_Object *object, const char *name) { 00970 return json_value_get_object(json_object_dotget_value(object, name)); 00971 } 00972 00973 JSON_Array * json_object_dotget_array(const JSON_Object *object, const char *name) { 00974 return json_value_get_array(json_object_dotget_value(object, name)); 00975 } 00976 00977 int json_object_dotget_boolean(const JSON_Object *object, const char *name) { 00978 return json_value_get_boolean(json_object_dotget_value(object, name)); 00979 } 00980 00981 size_t json_object_get_count(const JSON_Object *object) { 00982 return object ? object->count : 0; 00983 } 00984 00985 const char * json_object_get_name(const JSON_Object *object, size_t index) { 00986 if (object == NULL || index >= json_object_get_count(object)) 00987 return NULL; 00988 return object->names[index]; 00989 } 00990 00991 JSON_Value * json_object_get_value_at(const JSON_Object *object, size_t index) { 00992 if (object == NULL || index >= json_object_get_count(object)) 00993 return NULL; 00994 return object->values[index]; 00995 } 00996 00997 /* JSON Array API */ 00998 JSON_Value * json_array_get_value(const JSON_Array *array, size_t index) { 00999 if (array == NULL || index >= json_array_get_count(array)) 01000 return NULL; 01001 return array->items[index]; 01002 } 01003 01004 const char * json_array_get_string(const JSON_Array *array, size_t index) { 01005 return json_value_get_string(json_array_get_value(array, index)); 01006 } 01007 01008 double json_array_get_number(const JSON_Array *array, size_t index) { 01009 return json_value_get_number(json_array_get_value(array, index)); 01010 } 01011 01012 JSON_Object * json_array_get_object(const JSON_Array *array, size_t index) { 01013 return json_value_get_object(json_array_get_value(array, index)); 01014 } 01015 01016 JSON_Array * json_array_get_array(const JSON_Array *array, size_t index) { 01017 return json_value_get_array(json_array_get_value(array, index)); 01018 } 01019 01020 int json_array_get_boolean(const JSON_Array *array, size_t index) { 01021 return json_value_get_boolean(json_array_get_value(array, index)); 01022 } 01023 01024 size_t json_array_get_count(const JSON_Array *array) { 01025 return array ? array->count : 0; 01026 } 01027 01028 /* JSON Value API */ 01029 JSON_Value_Type json_value_get_type(const JSON_Value *value) { 01030 return value ? value->type : JSONError; 01031 } 01032 01033 JSON_Object * json_value_get_object(const JSON_Value *value) { 01034 return json_value_get_type(value) == JSONObject ? value->value.object : NULL; 01035 } 01036 01037 JSON_Array * json_value_get_array(const JSON_Value *value) { 01038 return json_value_get_type(value) == JSONArray ? value->value.array : NULL; 01039 } 01040 01041 const char * json_value_get_string(const JSON_Value *value) { 01042 return json_value_get_type(value) == JSONString ? value->value.string : NULL; 01043 } 01044 01045 double json_value_get_number(const JSON_Value *value) { 01046 return json_value_get_type(value) == JSONNumber ? value->value.number : 0; 01047 } 01048 01049 int json_value_get_boolean(const JSON_Value *value) { 01050 return json_value_get_type(value) == JSONBoolean ? value->value.boolean : -1; 01051 } 01052 01053 void json_value_free(JSON_Value *value) { 01054 switch (json_value_get_type(value)) { 01055 case JSONObject: 01056 json_object_free(value->value.object); 01057 break; 01058 case JSONString: 01059 if (value->value.string) { parson_free(value->value.string); } 01060 break; 01061 case JSONArray: 01062 json_array_free(value->value.array); 01063 break; 01064 default: 01065 break; 01066 } 01067 parson_free(value); 01068 } 01069 01070 JSON_Value * json_value_init_object(void) { 01071 JSON_Value *new_value = (JSON_Value*)parson_malloc(sizeof(JSON_Value)); 01072 if (!new_value) 01073 return NULL; 01074 new_value->type = JSONObject; 01075 new_value->value.object = json_object_init(); 01076 if (!new_value->value.object) { 01077 parson_free(new_value); 01078 return NULL; 01079 } 01080 return new_value; 01081 } 01082 01083 JSON_Value * json_value_init_array(void) { 01084 JSON_Value *new_value = (JSON_Value*)parson_malloc(sizeof(JSON_Value)); 01085 if (!new_value) 01086 return NULL; 01087 new_value->type = JSONArray; 01088 new_value->value.array = json_array_init(); 01089 if (!new_value->value.array) { 01090 parson_free(new_value); 01091 return NULL; 01092 } 01093 return new_value; 01094 } 01095 01096 JSON_Value * json_value_init_string(const char *string) { 01097 char *copy = NULL; 01098 JSON_Value *value; 01099 size_t string_len = 0; 01100 if (string == NULL) 01101 return NULL; 01102 string_len = strlen(string); 01103 if (!is_valid_utf8(string, string_len)) 01104 return NULL; 01105 copy = parson_strndup(string, string_len); 01106 if (copy == NULL) 01107 return NULL; 01108 value = json_value_init_string_no_copy(copy); 01109 if (value == NULL) 01110 parson_free(copy); 01111 return value; 01112 } 01113 01114 JSON_Value * json_value_init_number(double number) { 01115 JSON_Value *new_value = (JSON_Value*)parson_malloc(sizeof(JSON_Value)); 01116 if (!new_value) 01117 return NULL; 01118 new_value->type = JSONNumber; 01119 new_value->value.number = number; 01120 return new_value; 01121 } 01122 01123 JSON_Value * json_value_init_boolean(int boolean) { 01124 JSON_Value *new_value = (JSON_Value*)parson_malloc(sizeof(JSON_Value)); 01125 if (!new_value) 01126 return NULL; 01127 new_value->type = JSONBoolean; 01128 new_value->value.boolean = boolean ? 1 : 0; 01129 return new_value; 01130 } 01131 01132 JSON_Value * json_value_init_null(void) { 01133 JSON_Value *new_value = (JSON_Value*)parson_malloc(sizeof(JSON_Value)); 01134 if (!new_value) 01135 return NULL; 01136 new_value->type = JSONNull; 01137 return new_value; 01138 } 01139 01140 JSON_Value * json_value_deep_copy(const JSON_Value *value) { 01141 size_t i = 0; 01142 JSON_Value *return_value = NULL, *temp_value_copy = NULL, *temp_value = NULL; 01143 const char *temp_string = NULL, *temp_key = NULL; 01144 char *temp_string_copy = NULL; 01145 JSON_Array *temp_array = NULL, *temp_array_copy = NULL; 01146 JSON_Object *temp_object = NULL, *temp_object_copy = NULL; 01147 01148 switch (json_value_get_type(value)) { 01149 case JSONArray: 01150 temp_array = json_value_get_array(value); 01151 return_value = json_value_init_array(); 01152 if (return_value == NULL) 01153 return NULL; 01154 temp_array_copy = json_value_get_array(return_value); 01155 for (i = 0; i < json_array_get_count(temp_array); i++) { 01156 temp_value = json_array_get_value(temp_array, i); 01157 temp_value_copy = json_value_deep_copy(temp_value); 01158 if (temp_value_copy == NULL) { 01159 json_value_free(return_value); 01160 return NULL; 01161 } 01162 if (json_array_add(temp_array_copy, temp_value_copy) == JSONFailure) { 01163 json_value_free(return_value); 01164 json_value_free(temp_value_copy); 01165 return NULL; 01166 } 01167 } 01168 return return_value; 01169 case JSONObject: 01170 temp_object = json_value_get_object(value); 01171 return_value = json_value_init_object(); 01172 if (return_value == NULL) 01173 return NULL; 01174 temp_object_copy = json_value_get_object(return_value); 01175 for (i = 0; i < json_object_get_count(temp_object); i++) { 01176 temp_key = json_object_get_name(temp_object, i); 01177 temp_value = json_object_get_value(temp_object, temp_key); 01178 temp_value_copy = json_value_deep_copy(temp_value); 01179 if (temp_value_copy == NULL) { 01180 json_value_free(return_value); 01181 return NULL; 01182 } 01183 if (json_object_add(temp_object_copy, temp_key, temp_value_copy) == JSONFailure) { 01184 json_value_free(return_value); 01185 json_value_free(temp_value_copy); 01186 return NULL; 01187 } 01188 } 01189 return return_value; 01190 case JSONBoolean: 01191 return json_value_init_boolean(json_value_get_boolean(value)); 01192 case JSONNumber: 01193 return json_value_init_number(json_value_get_number(value)); 01194 case JSONString: 01195 temp_string = json_value_get_string(value); 01196 temp_string_copy = parson_strdup(temp_string); 01197 if (temp_string_copy == NULL) 01198 return NULL; 01199 return_value = json_value_init_string_no_copy(temp_string_copy); 01200 if (return_value == NULL) 01201 parson_free(temp_string_copy); 01202 return return_value; 01203 case JSONNull: 01204 return json_value_init_null(); 01205 case JSONError: 01206 return NULL; 01207 default: 01208 return NULL; 01209 } 01210 } 01211 01212 size_t json_serialization_size(const JSON_Value *value) { 01213 char num_buf[1100]; /* recursively allocating buffer on stack is a bad idea, so let's do it only once */ 01214 int res = json_serialize_to_buffer_r(value, NULL, 0, 0, num_buf); 01215 return res < 0 ? 0 : (size_t)(res + 1); 01216 } 01217 01218 JSON_Status json_serialize_to_buffer(const JSON_Value *value, char *buf, size_t buf_size_in_bytes) { 01219 int written = -1; 01220 size_t needed_size_in_bytes = json_serialization_size(value); 01221 if (needed_size_in_bytes == 0 || buf_size_in_bytes < needed_size_in_bytes) { 01222 return JSONFailure; 01223 } 01224 written = json_serialize_to_buffer_r(value, buf, 0, 0, NULL); 01225 if (written < 0) 01226 return JSONFailure; 01227 return JSONSuccess; 01228 } 01229 01230 JSON_Status json_serialize_to_file(const JSON_Value *value, const char *filename) { 01231 JSON_Status return_code = JSONSuccess; 01232 FILE *fp = NULL; 01233 char *serialized_string = json_serialize_to_string(value); 01234 if (serialized_string == NULL) { 01235 return JSONFailure; 01236 } 01237 fp = fopen (filename, "w"); 01238 if (fp == NULL) { 01239 json_free_serialized_string(serialized_string); 01240 return JSONFailure; 01241 } 01242 if (fputs(serialized_string, fp) == EOF) { 01243 return_code = JSONFailure; 01244 } 01245 if (fclose(fp) == EOF) { 01246 return_code = JSONFailure; 01247 } 01248 json_free_serialized_string(serialized_string); 01249 return return_code; 01250 } 01251 01252 char * json_serialize_to_string(const JSON_Value *value) { 01253 JSON_Status serialization_result = JSONFailure; 01254 size_t buf_size_bytes = json_serialization_size(value); 01255 char *buf = NULL; 01256 if (buf_size_bytes == 0) { 01257 return NULL; 01258 } 01259 buf = (char*)parson_malloc(buf_size_bytes); 01260 if (buf == NULL) 01261 return NULL; 01262 serialization_result = json_serialize_to_buffer(value, buf, buf_size_bytes); 01263 if (serialization_result == JSONFailure) { 01264 json_free_serialized_string(buf); 01265 return NULL; 01266 } 01267 return buf; 01268 } 01269 01270 size_t json_serialization_size_pretty(const JSON_Value *value) { 01271 char num_buf[1100]; /* recursively allocating buffer on stack is a bad idea, so let's do it only once */ 01272 int res = json_serialize_to_buffer_r(value, NULL, 0, 1, num_buf); 01273 return res < 0 ? 0 : (size_t)(res + 1); 01274 } 01275 01276 JSON_Status json_serialize_to_buffer_pretty(const JSON_Value *value, char *buf, size_t buf_size_in_bytes) { 01277 int written = -1; 01278 size_t needed_size_in_bytes = json_serialization_size_pretty(value); 01279 if (needed_size_in_bytes == 0 || buf_size_in_bytes < needed_size_in_bytes) 01280 return JSONFailure; 01281 written = json_serialize_to_buffer_r(value, buf, 0, 1, NULL); 01282 if (written < 0) 01283 return JSONFailure; 01284 return JSONSuccess; 01285 } 01286 01287 JSON_Status json_serialize_to_file_pretty(const JSON_Value *value, const char *filename) { 01288 JSON_Status return_code = JSONSuccess; 01289 FILE *fp = NULL; 01290 char *serialized_string = json_serialize_to_string_pretty(value); 01291 if (serialized_string == NULL) { 01292 return JSONFailure; 01293 } 01294 fp = fopen (filename, "w"); 01295 if (fp == NULL) { 01296 json_free_serialized_string(serialized_string); 01297 return JSONFailure; 01298 } 01299 if (fputs(serialized_string, fp) == EOF) { 01300 return_code = JSONFailure; 01301 } 01302 if (fclose(fp) == EOF) { 01303 return_code = JSONFailure; 01304 } 01305 json_free_serialized_string(serialized_string); 01306 return return_code; 01307 } 01308 01309 char * json_serialize_to_string_pretty(const JSON_Value *value) { 01310 JSON_Status serialization_result = JSONFailure; 01311 size_t buf_size_bytes = json_serialization_size_pretty(value); 01312 char *buf = NULL; 01313 if (buf_size_bytes == 0) { 01314 return NULL; 01315 } 01316 buf = (char*)parson_malloc(buf_size_bytes); 01317 if (buf == NULL) 01318 return NULL; 01319 serialization_result = json_serialize_to_buffer_pretty(value, buf, buf_size_bytes); 01320 if (serialization_result == JSONFailure) { 01321 json_free_serialized_string(buf); 01322 return NULL; 01323 } 01324 return buf; 01325 } 01326 01327 void json_free_serialized_string(char *string) { 01328 parson_free(string); 01329 } 01330 01331 JSON_Status json_array_remove(JSON_Array *array, size_t ix) { 01332 JSON_Value *temp_value = NULL; 01333 size_t last_element_ix = 0; 01334 if (array == NULL || ix >= json_array_get_count(array)) { 01335 return JSONFailure; 01336 } 01337 last_element_ix = json_array_get_count(array) - 1; 01338 json_value_free(json_array_get_value(array, ix)); 01339 if (ix != last_element_ix) { /* Replace value with one from the end of array */ 01340 temp_value = json_array_get_value(array, last_element_ix); 01341 if (temp_value == NULL) { 01342 return JSONFailure; 01343 } 01344 array->items[ix] = temp_value; 01345 } 01346 array->count -= 1; 01347 return JSONSuccess; 01348 } 01349 01350 JSON_Status json_array_replace_value(JSON_Array *array, size_t ix, JSON_Value *value) { 01351 if (array == NULL || value == NULL || ix >= json_array_get_count(array)) { 01352 return JSONFailure; 01353 } 01354 json_value_free(json_array_get_value(array, ix)); 01355 array->items[ix] = value; 01356 return JSONSuccess; 01357 } 01358 01359 JSON_Status json_array_replace_string(JSON_Array *array, size_t i, const char* string) { 01360 JSON_Value *value = json_value_init_string(string); 01361 if (value == NULL) 01362 return JSONFailure; 01363 if (json_array_replace_value(array, i, value) == JSONFailure) { 01364 json_value_free(value); 01365 return JSONFailure; 01366 } 01367 return JSONSuccess; 01368 } 01369 01370 JSON_Status json_array_replace_number(JSON_Array *array, size_t i, double number) { 01371 JSON_Value *value = json_value_init_number(number); 01372 if (value == NULL) 01373 return JSONFailure; 01374 if (json_array_replace_value(array, i, value) == JSONFailure) { 01375 json_value_free(value); 01376 return JSONFailure; 01377 } 01378 return JSONSuccess; 01379 } 01380 01381 JSON_Status json_array_replace_boolean(JSON_Array *array, size_t i, int boolean) { 01382 JSON_Value *value = json_value_init_boolean(boolean); 01383 if (value == NULL) 01384 return JSONFailure; 01385 if (json_array_replace_value(array, i, value) == JSONFailure) { 01386 json_value_free(value); 01387 return JSONFailure; 01388 } 01389 return JSONSuccess; 01390 } 01391 01392 JSON_Status json_array_replace_null(JSON_Array *array, size_t i) { 01393 JSON_Value *value = json_value_init_null(); 01394 if (value == NULL) 01395 return JSONFailure; 01396 if (json_array_replace_value(array, i, value) == JSONFailure) { 01397 json_value_free(value); 01398 return JSONFailure; 01399 } 01400 return JSONSuccess; 01401 } 01402 01403 JSON_Status json_array_clear(JSON_Array *array) { 01404 size_t i = 0; 01405 if (array == NULL) 01406 return JSONFailure; 01407 for (i = 0; i < json_array_get_count(array); i++) { 01408 json_value_free(json_array_get_value(array, i)); 01409 } 01410 array->count = 0; 01411 return JSONSuccess; 01412 } 01413 01414 JSON_Status json_array_append_value(JSON_Array *array, JSON_Value *value) { 01415 if (array == NULL || value == NULL) 01416 return JSONFailure; 01417 return json_array_add(array, value); 01418 } 01419 01420 JSON_Status json_array_append_string(JSON_Array *array, const char *string) { 01421 JSON_Value *value = json_value_init_string(string); 01422 if (value == NULL) 01423 return JSONFailure; 01424 if (json_array_append_value(array, value) == JSONFailure) { 01425 json_value_free(value); 01426 return JSONFailure; 01427 } 01428 return JSONSuccess; 01429 } 01430 01431 JSON_Status json_array_append_number(JSON_Array *array, double number) { 01432 JSON_Value *value = json_value_init_number(number); 01433 if (value == NULL) 01434 return JSONFailure; 01435 if (json_array_append_value(array, value) == JSONFailure) { 01436 json_value_free(value); 01437 return JSONFailure; 01438 } 01439 return JSONSuccess; 01440 } 01441 01442 JSON_Status json_array_append_boolean(JSON_Array *array, int boolean) { 01443 JSON_Value *value = json_value_init_boolean(boolean); 01444 if (value == NULL) 01445 return JSONFailure; 01446 if (json_array_append_value(array, value) == JSONFailure) { 01447 json_value_free(value); 01448 return JSONFailure; 01449 } 01450 return JSONSuccess; 01451 } 01452 01453 JSON_Status json_array_append_null(JSON_Array *array) { 01454 JSON_Value *value = json_value_init_null(); 01455 if (value == NULL) 01456 return JSONFailure; 01457 if (json_array_append_value(array, value) == JSONFailure) { 01458 json_value_free(value); 01459 return JSONFailure; 01460 } 01461 return JSONSuccess; 01462 } 01463 01464 JSON_Status json_object_set_value(JSON_Object *object, const char *name, JSON_Value *value) { 01465 size_t i = 0; 01466 JSON_Value *old_value; 01467 if (object == NULL || name == NULL || value == NULL) 01468 return JSONFailure; 01469 old_value = json_object_get_value(object, name); 01470 if (old_value != NULL) { /* free and overwrite old value */ 01471 json_value_free(old_value); 01472 for (i = 0; i < json_object_get_count(object); i++) { 01473 if (strcmp(object->names[i], name) == 0) { 01474 object->values[i] = value; 01475 return JSONSuccess; 01476 } 01477 } 01478 } 01479 /* add new key value pair */ 01480 return json_object_add(object, name, value); 01481 } 01482 01483 JSON_Status json_object_set_string(JSON_Object *object, const char *name, const char *string) { 01484 return json_object_set_value(object, name, json_value_init_string(string)); 01485 } 01486 01487 JSON_Status json_object_set_number(JSON_Object *object, const char *name, double number) { 01488 return json_object_set_value(object, name, json_value_init_number(number)); 01489 } 01490 01491 JSON_Status json_object_set_boolean(JSON_Object *object, const char *name, int boolean) { 01492 return json_object_set_value(object, name, json_value_init_boolean(boolean)); 01493 } 01494 01495 JSON_Status json_object_set_null(JSON_Object *object, const char *name) { 01496 return json_object_set_value(object, name, json_value_init_null()); 01497 } 01498 01499 JSON_Status json_object_dotset_value(JSON_Object *object, const char *name, JSON_Value *value) { 01500 const char *dot_pos = NULL; 01501 char *current_name = NULL; 01502 JSON_Object *temp_obj = NULL; 01503 JSON_Value *new_value = NULL; 01504 if (value == NULL || name == NULL || value == NULL) 01505 return JSONFailure; 01506 dot_pos = strchr(name, '.'); 01507 if (dot_pos == NULL) { 01508 return json_object_set_value(object, name, value); 01509 } else { 01510 current_name = parson_strndup(name, dot_pos - name); 01511 temp_obj = json_object_get_object(object, current_name); 01512 if (temp_obj == NULL) { 01513 new_value = json_value_init_object(); 01514 if (new_value == NULL) { 01515 parson_free(current_name); 01516 return JSONFailure; 01517 } 01518 if (json_object_add(object, current_name, new_value) == JSONFailure) { 01519 json_value_free(new_value); 01520 parson_free(current_name); 01521 return JSONFailure; 01522 } 01523 temp_obj = json_object_get_object(object, current_name); 01524 } 01525 parson_free(current_name); 01526 return json_object_dotset_value(temp_obj, dot_pos + 1, value); 01527 } 01528 } 01529 01530 JSON_Status json_object_dotset_string(JSON_Object *object, const char *name, const char *string) { 01531 JSON_Value *value = json_value_init_string(string); 01532 if (value == NULL) 01533 return JSONFailure; 01534 if (json_object_dotset_value(object, name, value) == JSONFailure) { 01535 json_value_free(value); 01536 return JSONFailure; 01537 } 01538 return JSONSuccess; 01539 } 01540 01541 JSON_Status json_object_dotset_number(JSON_Object *object, const char *name, double number) { 01542 JSON_Value *value = json_value_init_number(number); 01543 if (value == NULL) 01544 return JSONFailure; 01545 if (json_object_dotset_value(object, name, value) == JSONFailure) { 01546 json_value_free(value); 01547 return JSONFailure; 01548 } 01549 return JSONSuccess; 01550 } 01551 01552 JSON_Status json_object_dotset_boolean(JSON_Object *object, const char *name, int boolean) { 01553 JSON_Value *value = json_value_init_boolean(boolean); 01554 if (value == NULL) 01555 return JSONFailure; 01556 if (json_object_dotset_value(object, name, value) == JSONFailure) { 01557 json_value_free(value); 01558 return JSONFailure; 01559 } 01560 return JSONSuccess; 01561 } 01562 01563 JSON_Status json_object_dotset_null(JSON_Object *object, const char *name) { 01564 JSON_Value *value = json_value_init_null(); 01565 if (value == NULL) 01566 return JSONFailure; 01567 if (json_object_dotset_value(object, name, value) == JSONFailure) { 01568 json_value_free(value); 01569 return JSONFailure; 01570 } 01571 return JSONSuccess; 01572 } 01573 01574 JSON_Status json_object_remove(JSON_Object *object, const char *name) { 01575 size_t i = 0, last_item_index = 0; 01576 if (object == NULL || json_object_get_value(object, name) == NULL) 01577 return JSONFailure; 01578 last_item_index = json_object_get_count(object) - 1; 01579 for (i = 0; i < json_object_get_count(object); i++) { 01580 if (strcmp(object->names[i], name) == 0) { 01581 parson_free(object->names[i]); 01582 json_value_free(object->values[i]); 01583 if (i != last_item_index) { /* Replace key value pair with one from the end */ 01584 object->names[i] = object->names[last_item_index]; 01585 object->values[i] = object->values[last_item_index]; 01586 } 01587 object->count -= 1; 01588 return JSONSuccess; 01589 } 01590 } 01591 return JSONFailure; /* No execution path should end here */ 01592 } 01593 01594 JSON_Status json_object_dotremove(JSON_Object *object, const char *name) { 01595 const char *dot_pos = strchr(name, '.'); 01596 char *current_name = NULL; 01597 JSON_Object *temp_obj = NULL; 01598 if (dot_pos == NULL) { 01599 return json_object_remove(object, name); 01600 } else { 01601 current_name = parson_strndup(name, dot_pos - name); 01602 temp_obj = json_object_get_object(object, current_name); 01603 if (temp_obj == NULL) { 01604 parson_free(current_name); 01605 return JSONFailure; 01606 } 01607 parson_free(current_name); 01608 return json_object_dotremove(temp_obj, dot_pos + 1); 01609 } 01610 } 01611 01612 JSON_Status json_object_clear(JSON_Object *object) { 01613 size_t i = 0; 01614 if (object == NULL) { 01615 return JSONFailure; 01616 } 01617 for (i = 0; i < json_object_get_count(object); i++) { 01618 parson_free(object->names[i]); 01619 json_value_free(object->values[i]); 01620 } 01621 object->count = 0; 01622 return JSONSuccess; 01623 } 01624 01625 JSON_Status json_validate(const JSON_Value *schema, const JSON_Value *value) { 01626 JSON_Value *temp_schema_value = NULL, *temp_value = NULL; 01627 JSON_Array *schema_array = NULL, *value_array = NULL; 01628 JSON_Object *schema_object = NULL, *value_object = NULL; 01629 JSON_Value_Type schema_type = JSONError, value_type = JSONError; 01630 const char *key = NULL; 01631 size_t i = 0, count = 0; 01632 if (schema == NULL || value == NULL) 01633 return JSONFailure; 01634 schema_type = json_value_get_type(schema); 01635 value_type = json_value_get_type(value); 01636 if (schema_type != value_type && schema_type != JSONNull) /* null represents all values */ 01637 return JSONFailure; 01638 switch (schema_type) { 01639 case JSONArray: 01640 schema_array = json_value_get_array(schema); 01641 value_array = json_value_get_array(value); 01642 count = json_array_get_count(schema_array); 01643 if (count == 0) 01644 return JSONSuccess; /* Empty array allows all types */ 01645 /* Get first value from array, rest is ignored */ 01646 temp_schema_value = json_array_get_value(schema_array, 0); 01647 for (i = 0; i < json_array_get_count(value_array); i++) { 01648 temp_value = json_array_get_value(value_array, i); 01649 if (json_validate(temp_schema_value, temp_value) == 0) { 01650 return JSONFailure; 01651 } 01652 } 01653 return JSONSuccess; 01654 case JSONObject: 01655 schema_object = json_value_get_object(schema); 01656 value_object = json_value_get_object(value); 01657 count = json_object_get_count(schema_object); 01658 if (count == 0) 01659 return JSONSuccess; /* Empty object allows all objects */ 01660 else if (json_object_get_count(value_object) < count) 01661 return JSONFailure; /* Tested object mustn't have less name-value pairs than schema */ 01662 for (i = 0; i < count; i++) { 01663 key = json_object_get_name(schema_object, i); 01664 temp_schema_value = json_object_get_value(schema_object, key); 01665 temp_value = json_object_get_value(value_object, key); 01666 if (temp_value == NULL) 01667 return JSONFailure; 01668 if (json_validate(temp_schema_value, temp_value) == JSONFailure) 01669 return JSONFailure; 01670 } 01671 return JSONSuccess; 01672 case JSONString: case JSONNumber: case JSONBoolean: case JSONNull: 01673 return JSONSuccess; /* equality already tested before switch */ 01674 case JSONError: default: 01675 return JSONFailure; 01676 } 01677 } 01678 01679 JSON_Status json_value_equals(const JSON_Value *a, const JSON_Value *b) { 01680 JSON_Object *a_object = NULL, *b_object = NULL; 01681 JSON_Array *a_array = NULL, *b_array = NULL; 01682 const char *a_string = NULL, *b_string = NULL; 01683 const char *key = NULL; 01684 size_t a_count = 0, b_count = 0, i = 0; 01685 JSON_Value_Type a_type, b_type; 01686 a_type = json_value_get_type(a); 01687 b_type = json_value_get_type(b); 01688 if (a_type != b_type) { 01689 return 0; 01690 } 01691 switch (a_type) { 01692 case JSONArray: 01693 a_array = json_value_get_array(a); 01694 b_array = json_value_get_array(b); 01695 a_count = json_array_get_count(a_array); 01696 b_count = json_array_get_count(b_array); 01697 if (a_count != b_count) { 01698 return 0; 01699 } 01700 for (i = 0; i < a_count; i++) { 01701 if (!json_value_equals(json_array_get_value(a_array, i), 01702 json_array_get_value(b_array, i))) { 01703 return 0; 01704 } 01705 } 01706 return 1; 01707 case JSONObject: 01708 a_object = json_value_get_object(a); 01709 b_object = json_value_get_object(b); 01710 a_count = json_object_get_count(a_object); 01711 b_count = json_object_get_count(b_object); 01712 if (a_count != b_count) { 01713 return 0; 01714 } 01715 for (i = 0; i < a_count; i++) { 01716 key = json_object_get_name(a_object, i); 01717 if (!json_value_equals(json_object_get_value(a_object, key), 01718 json_object_get_value(b_object, key))) { 01719 return 0; 01720 } 01721 } 01722 return 1; 01723 case JSONString: 01724 a_string = json_value_get_string(a); 01725 b_string = json_value_get_string(b); 01726 return strcmp(a_string, b_string) == 0; 01727 case JSONBoolean: 01728 return json_value_get_boolean(a) == json_value_get_boolean(b); 01729 case JSONNumber: 01730 return fabs(json_value_get_number(a) - json_value_get_number(b)) < 0.000001; /* EPSILON */ 01731 case JSONError: 01732 return 1; 01733 case JSONNull: 01734 return 1; 01735 default: 01736 return 1; 01737 } 01738 } 01739 01740 JSON_Value_Type json_type(const JSON_Value *value) { 01741 return json_value_get_type(value); 01742 } 01743 01744 JSON_Object * json_object (const JSON_Value *value) { 01745 return json_value_get_object(value); 01746 } 01747 01748 JSON_Array * json_array (const JSON_Value *value) { 01749 return json_value_get_array(value); 01750 } 01751 01752 const char * json_string (const JSON_Value *value) { 01753 return json_value_get_string(value); 01754 } 01755 01756 double json_number (const JSON_Value *value) { 01757 return json_value_get_number(value); 01758 } 01759 01760 int json_boolean(const JSON_Value *value) { 01761 return json_value_get_boolean(value); 01762 } 01763 01764 void json_set_allocation_functions(JSON_Malloc_Function malloc_fun, JSON_Free_Function free_fun) { 01765 parson_malloc = malloc_fun; 01766 parson_free = free_fun; 01767 }
Generated on Tue Jul 12 2022 19:44:54 by
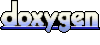