corrected version (with typedef struct IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE_DATA* IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE;) included in the sources
Dependents: STM32F746_iothub_client_sample_mqtt
Fork of iothub_client by
iothub_message.c
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 #include <stdlib.h> 00005 #ifdef _CRTDBG_MAP_ALLOC 00006 #include <crtdbg.h> 00007 #endif 00008 #include "azure_c_shared_utility/gballoc.h" 00009 #include "azure_c_shared_utility/iot_logging.h" 00010 #include "azure_c_shared_utility/buffer_.h" 00011 00012 #include "iothub_message.h" 00013 00014 DEFINE_ENUM_STRINGS(IOTHUB_MESSAGE_RESULT, IOTHUB_MESSAGE_RESULT_VALUES); 00015 DEFINE_ENUM_STRINGS(IOTHUBMESSAGE_CONTENT_TYPE, IOTHUBMESSAGE_CONTENT_TYPE_VALUES); 00016 00017 #define LOG_IOTHUB_MESSAGE_ERROR() \ 00018 LogError("(result = %s)", ENUM_TO_STRING(IOTHUB_MESSAGE_RESULT, result)); 00019 00020 typedef struct IOTHUB_MESSAGE_HANDLE_DATA_TAG 00021 { 00022 IOTHUBMESSAGE_CONTENT_TYPE contentType; 00023 union 00024 { 00025 BUFFER_HANDLE byteArray; 00026 STRING_HANDLE string; 00027 } value; 00028 MAP_HANDLE properties; 00029 char* messageId; 00030 char* correlationId; 00031 }IOTHUB_MESSAGE_HANDLE_DATA; 00032 00033 static bool ContainsOnlyUsAscii(const char* asciiValue) 00034 { 00035 bool result = true; 00036 const char* iterator = asciiValue; 00037 while (iterator != NULL && *iterator != '\0') 00038 { 00039 // Allow only printable ascii char 00040 if (*iterator < ' ' || *iterator > '~') 00041 { 00042 result = false; 00043 break; 00044 } 00045 iterator++; 00046 } 00047 return result; 00048 } 00049 00050 /* Codes_SRS_IOTHUBMESSAGE_07_008: [ValidateAsciiCharactersFilter shall loop through the mapKey and mapValue strings to ensure that they only contain valid US-Ascii characters Ascii value 32 - 126.] */ 00051 static int ValidateAsciiCharactersFilter(const char* mapKey, const char* mapValue) 00052 { 00053 int result; 00054 if (!ContainsOnlyUsAscii(mapKey) || !ContainsOnlyUsAscii(mapValue) ) 00055 { 00056 result = __LINE__; 00057 } 00058 else 00059 { 00060 result = 0; 00061 } 00062 return result; 00063 } 00064 00065 IOTHUB_MESSAGE_HANDLE IoTHubMessage_CreateFromByteArray(const unsigned char* byteArray, size_t size) 00066 { 00067 IOTHUB_MESSAGE_HANDLE_DATA* result; 00068 result = malloc(sizeof(IOTHUB_MESSAGE_HANDLE_DATA)); 00069 if (result == NULL) 00070 { 00071 LogError("unable to malloc"); 00072 /*Codes_SRS_IOTHUBMESSAGE_02_024: [If there are any errors then IoTHubMessage_CreateFromByteArray shall return NULL.] */ 00073 /*let it go through*/ 00074 } 00075 else 00076 { 00077 const unsigned char* source; 00078 unsigned char temp = 0x00; 00079 if (size != 0) 00080 { 00081 /*Codes_SRS_IOTHUBMESSAGE_06_002: [If size is NOT zero then byteArray MUST NOT be NULL*/ 00082 if (byteArray == NULL) 00083 { 00084 LogError("Attempted to create a Hub Message from a NULL pointer!"); 00085 free(result); 00086 result = NULL; 00087 source = NULL; 00088 } 00089 else 00090 { 00091 source = byteArray; 00092 } 00093 } 00094 else 00095 { 00096 /*Codes_SRS_IOTHUBMESSAGE_06_001: [If size is zero then byteArray may be NULL.]*/ 00097 source = &temp; 00098 } 00099 if (result != NULL) 00100 { 00101 /*Codes_SRS_IOTHUBMESSAGE_02_022: [IoTHubMessage_CreateFromByteArray shall call BUFFER_create passing byteArray and size as parameters.] */ 00102 if ((result->value.byteArray = BUFFER_create(source, size)) == NULL) 00103 { 00104 LogError("BUFFER_create failed"); 00105 /*Codes_SRS_IOTHUBMESSAGE_02_024: [If there are any errors then IoTHubMessage_CreateFromByteArray shall return NULL.] */ 00106 free(result); 00107 result = NULL; 00108 } 00109 /*Codes_SRS_IOTHUBMESSAGE_02_023: [IoTHubMessage_CreateFromByteArray shall call Map_Create to create the message properties.] */ 00110 else if ((result->properties = Map_Create(ValidateAsciiCharactersFilter)) == NULL) 00111 { 00112 LogError("Map_Create failed"); 00113 /*Codes_SRS_IOTHUBMESSAGE_02_024: [If there are any errors then IoTHubMessage_CreateFromByteArray shall return NULL.] */ 00114 BUFFER_delete(result->value.byteArray); 00115 free(result); 00116 result = NULL; 00117 } 00118 else 00119 { 00120 /*Codes_SRS_IOTHUBMESSAGE_02_025: [Otherwise, IoTHubMessage_CreateFromByteArray shall return a non-NULL handle.] */ 00121 /*Codes_SRS_IOTHUBMESSAGE_02_026: [The type of the new message shall be IOTHUBMESSAGE_BYTEARRAY.] */ 00122 result->contentType = IOTHUBMESSAGE_BYTEARRAY; 00123 result->messageId = NULL; 00124 result->correlationId = NULL; 00125 /*all is fine, return result*/ 00126 } 00127 } 00128 } 00129 return result; 00130 } 00131 IOTHUB_MESSAGE_HANDLE IoTHubMessage_CreateFromString(const char* source) 00132 { 00133 IOTHUB_MESSAGE_HANDLE_DATA* result; 00134 result = malloc(sizeof(IOTHUB_MESSAGE_HANDLE_DATA)); 00135 if (result == NULL) 00136 { 00137 LogError("malloc failed"); 00138 /*Codes_SRS_IOTHUBMESSAGE_02_029: [If there are any encountered in the execution of IoTHubMessage_CreateFromString then IoTHubMessage_CreateFromString shall return NULL.] */ 00139 /*let it go through*/ 00140 } 00141 else 00142 { 00143 /*Codes_SRS_IOTHUBMESSAGE_02_027: [IoTHubMessage_CreateFromString shall call STRING_construct passing source as parameter.] */ 00144 if ((result->value.string = STRING_construct(source)) == NULL) 00145 { 00146 LogError("STRING_construct failed"); 00147 /*Codes_SRS_IOTHUBMESSAGE_02_029: [If there are any encountered in the execution of IoTHubMessage_CreateFromString then IoTHubMessage_CreateFromString shall return NULL.] */ 00148 free(result); 00149 result = NULL; 00150 } 00151 /*Codes_SRS_IOTHUBMESSAGE_02_028: [IoTHubMessage_CreateFromString shall call Map_Create to create the message properties.] */ 00152 else if ((result->properties = Map_Create(ValidateAsciiCharactersFilter)) == NULL) 00153 { 00154 LogError("Map_Create failed"); 00155 /*Codes_SRS_IOTHUBMESSAGE_02_029: [If there are any encountered in the execution of IoTHubMessage_CreateFromString then IoTHubMessage_CreateFromString shall return NULL.] */ 00156 STRING_delete(result->value.string); 00157 free(result); 00158 result = NULL; 00159 } 00160 else 00161 { 00162 /*Codes_SRS_IOTHUBMESSAGE_02_031: [Otherwise, IoTHubMessage_CreateFromString shall return a non-NULL handle.] */ 00163 /*Codes_SRS_IOTHUBMESSAGE_02_032: [The type of the new message shall be IOTHUBMESSAGE_STRING.] */ 00164 result->contentType = IOTHUBMESSAGE_STRING; 00165 result->messageId = NULL; 00166 result->correlationId = NULL; 00167 } 00168 } 00169 return result; 00170 } 00171 00172 /*Codes_SRS_IOTHUBMESSAGE_03_001: [IoTHubMessage_Clone shall create a new IoT hub message with data content identical to that of the iotHubMessageHandle parameter.]*/ 00173 IOTHUB_MESSAGE_HANDLE IoTHubMessage_Clone(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle) 00174 { 00175 IOTHUB_MESSAGE_HANDLE_DATA* result; 00176 const IOTHUB_MESSAGE_HANDLE_DATA* source = (const IOTHUB_MESSAGE_HANDLE_DATA*)iotHubMessageHandle; 00177 /* Codes_SRS_IOTHUBMESSAGE_03_005: [IoTHubMessage_Clone shall return NULL if iotHubMessageHandle is NULL.] */ 00178 if (source == NULL) 00179 { 00180 result = NULL; 00181 LogError("iotHubMessageHandle parameter cannot be NULL for IoTHubMessage_Clone"); 00182 } 00183 else 00184 { 00185 result = (IOTHUB_MESSAGE_HANDLE_DATA*)malloc(sizeof(IOTHUB_MESSAGE_HANDLE_DATA)); 00186 /*Codes_SRS_IOTHUBMESSAGE_03_004: [IoTHubMessage_Clone shall return NULL if it fails for any reason.]*/ 00187 if (result == NULL) 00188 { 00189 /*Codes_SRS_IOTHUBMESSAGE_03_004: [IoTHubMessage_Clone shall return NULL if it fails for any reason.]*/ 00190 /*do nothing and return as is*/ 00191 LogError("unable to malloc"); 00192 } 00193 else 00194 { 00195 result->messageId = NULL; 00196 result->correlationId = NULL; 00197 if (source->messageId != NULL && mallocAndStrcpy_s(&result->messageId, source->messageId) != 0) 00198 { 00199 LogError("unable to Copy messageId"); 00200 free(result); 00201 result = NULL; 00202 } 00203 else if (source->correlationId != NULL && mallocAndStrcpy_s(&result->correlationId, source->correlationId) != 0) 00204 { 00205 LogError("unable to Copy correlationId"); 00206 if (result->messageId != NULL) 00207 { 00208 free(result->messageId); 00209 result->messageId = NULL; 00210 } 00211 free(result); 00212 result = NULL; 00213 } 00214 else if (source->contentType == IOTHUBMESSAGE_BYTEARRAY) 00215 { 00216 /*Codes_SRS_IOTHUBMESSAGE_02_006: [IoTHubMessage_Clone shall clone to content by a call to BUFFER_clone] */ 00217 if ((result->value.byteArray = BUFFER_clone(source->value.byteArray)) == NULL) 00218 { 00219 /*Codes_SRS_IOTHUBMESSAGE_03_004: [IoTHubMessage_Clone shall return NULL if it fails for any reason.]*/ 00220 LogError("unable to BUFFER_clone"); 00221 if (result->messageId) 00222 { 00223 free(result->messageId); 00224 result->messageId = NULL; 00225 } 00226 if (result->correlationId != NULL) 00227 { 00228 free(result->correlationId); 00229 result->correlationId = NULL; 00230 } 00231 free(result); 00232 result = NULL; 00233 } 00234 /*Codes_SRS_IOTHUBMESSAGE_02_005: [IoTHubMessage_Clone shall clone the properties map by using Map_Clone.] */ 00235 else if ((result->properties = Map_Clone(source->properties)) == NULL) 00236 { 00237 /*Codes_SRS_IOTHUBMESSAGE_03_004: [IoTHubMessage_Clone shall return NULL if it fails for any reason.]*/ 00238 LogError("unable to Map_Clone"); 00239 BUFFER_delete(result->value.byteArray); 00240 if (result->messageId) 00241 { 00242 free(result->messageId); 00243 result->messageId = NULL; 00244 } 00245 if (result->correlationId != NULL) 00246 { 00247 free(result->correlationId); 00248 result->correlationId = NULL; 00249 } 00250 free(result); 00251 result = NULL; 00252 } 00253 else 00254 { 00255 result->contentType = IOTHUBMESSAGE_BYTEARRAY; 00256 /*Codes_SRS_IOTHUBMESSAGE_03_002: [IoTHubMessage_Clone shall return upon success a non-NULL handle to the newly created IoT hub message.]*/ 00257 /*return as is, this is a good result*/ 00258 } 00259 } 00260 else /*can only be STRING*/ 00261 { 00262 /*Codes_SRS_IOTHUBMESSAGE_02_006: [IoTHubMessage_Clone shall clone the content by a call to BUFFER_clone or STRING_clone] */ 00263 if ((result->value.string = STRING_clone(source->value.string)) == NULL) 00264 { 00265 /*Codes_SRS_IOTHUBMESSAGE_03_004: [IoTHubMessage_Clone shall return NULL if it fails for any reason.]*/ 00266 if (result->messageId) 00267 { 00268 free(result->messageId); 00269 result->messageId = NULL; 00270 } 00271 if (result->correlationId != NULL) 00272 { 00273 free(result->correlationId); 00274 result->correlationId = NULL; 00275 } 00276 free(result); 00277 result = NULL; 00278 LogError("failed to STRING_clone"); 00279 } 00280 /*Codes_SRS_IOTHUBMESSAGE_02_005: [IoTHubMessage_Clone shall clone the properties map by using Map_Clone.] */ 00281 else if ((result->properties = Map_Clone(source->properties)) == NULL) 00282 { 00283 /*Codes_SRS_IOTHUBMESSAGE_03_004: [IoTHubMessage_Clone shall return NULL if it fails for any reason.]*/ 00284 LogError("unable to Map_Clone"); 00285 STRING_delete(result->value.string); 00286 if (result->messageId) 00287 { 00288 free(result->messageId); 00289 result->messageId = NULL; 00290 } 00291 if (result->correlationId != NULL) 00292 { 00293 free(result->correlationId); 00294 result->correlationId = NULL; 00295 } 00296 free(result); 00297 result = NULL; 00298 } 00299 else 00300 { 00301 result->contentType = IOTHUBMESSAGE_STRING; 00302 /*all is fine*/ 00303 } 00304 } 00305 } 00306 } 00307 return result; 00308 } 00309 00310 IOTHUB_MESSAGE_RESULT IoTHubMessage_GetByteArray(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle, const unsigned char** buffer, size_t* size) 00311 { 00312 IOTHUB_MESSAGE_RESULT result; 00313 if ( 00314 (iotHubMessageHandle == NULL) || 00315 (buffer == NULL) || 00316 (size == NULL) 00317 ) 00318 { 00319 /*Codes_SRS_IOTHUBMESSAGE_01_014: [If any of the arguments passed to IoTHubMessage_GetByteArray is NULL IoTHubMessage_GetByteArray shall return IOTHUBMESSAGE_INVALID_ARG.] */ 00320 LogError("invalid parameter (NULL) to IoTHubMessage_GetByteArray IOTHUB_MESSAGE_HANDLE iotHubMessageHandle=%p, const unsigned char** buffer=%p, size_t* size=%p", iotHubMessageHandle, buffer, size); 00321 result = IOTHUB_MESSAGE_INVALID_ARG; 00322 } 00323 else 00324 { 00325 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00326 if (handleData->contentType != IOTHUBMESSAGE_BYTEARRAY) 00327 { 00328 /*Codes_SRS_IOTHUBMESSAGE_02_021: [If iotHubMessageHandle is not a iothubmessage containing BYTEARRAY data, then IoTHubMessage_GetData shall write in *buffer NULL and shall set *size to 0.] */ 00329 result = IOTHUB_MESSAGE_INVALID_ARG; 00330 LogError("invalid type of message %s", ENUM_TO_STRING(IOTHUBMESSAGE_CONTENT_TYPE, handleData->contentType)); 00331 } 00332 else 00333 { 00334 /*Codes_SRS_IOTHUBMESSAGE_01_011: [The pointer shall be obtained by using BUFFER_u_char and it shall be copied in the buffer argument.]*/ 00335 *buffer = BUFFER_u_char(handleData->value.byteArray); 00336 /*Codes_SRS_IOTHUBMESSAGE_01_012: [The size of the associated data shall be obtained by using BUFFER_length and it shall be copied to the size argument.]*/ 00337 *size = BUFFER_length(handleData->value.byteArray); 00338 result = IOTHUB_MESSAGE_OK; 00339 } 00340 } 00341 return result; 00342 } 00343 00344 const char* IoTHubMessage_GetString(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle) 00345 { 00346 const char* result; 00347 if (iotHubMessageHandle == NULL) 00348 { 00349 /*Codes_SRS_IOTHUBMESSAGE_02_016: [If any parameter is NULL then IoTHubMessage_GetString shall return NULL.] */ 00350 result = NULL; 00351 } 00352 else 00353 { 00354 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00355 if (handleData->contentType != IOTHUBMESSAGE_STRING) 00356 { 00357 /*Codes_SRS_IOTHUBMESSAGE_02_017: [IoTHubMessage_GetString shall return NULL if the iotHubMessageHandle does not refer to a IOTHUBMESSAGE of type STRING.] */ 00358 result = NULL; 00359 } 00360 else 00361 { 00362 /*Codes_SRS_IOTHUBMESSAGE_02_018: [IoTHubMessage_GetStringData shall return the currently stored null terminated string.] */ 00363 result = STRING_c_str(handleData->value.string); 00364 } 00365 } 00366 return result; 00367 } 00368 00369 IOTHUBMESSAGE_CONTENT_TYPE IoTHubMessage_GetContentType(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle) 00370 { 00371 IOTHUBMESSAGE_CONTENT_TYPE result; 00372 /*Codes_SRS_IOTHUBMESSAGE_02_008: [If any parameter is NULL then IoTHubMessage_GetContentType shall return IOTHUBMESSAGE_UNKNOWN.] */ 00373 if (iotHubMessageHandle == NULL) 00374 { 00375 result = IOTHUBMESSAGE_UNKNOWN; 00376 } 00377 else 00378 { 00379 /*Codes_SRS_IOTHUBMESSAGE_02_009: [Otherwise IoTHubMessage_GetContentType shall return the type of the message.] */ 00380 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00381 result = handleData->contentType; 00382 } 00383 return result; 00384 } 00385 00386 MAP_HANDLE IoTHubMessage_Properties(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle) 00387 { 00388 MAP_HANDLE result; 00389 /*Codes_SRS_IOTHUBMESSAGE_02_001: [If iotHubMessageHandle is NULL then IoTHubMessage_Properties shall return NULL.]*/ 00390 if (iotHubMessageHandle == NULL) 00391 { 00392 LogError("invalid arg (NULL) passed to IoTHubMessage_Properties"); 00393 result = NULL; 00394 } 00395 else 00396 { 00397 /*Codes_SRS_IOTHUBMESSAGE_02_002: [Otherwise, for any non-NULL iotHubMessageHandle it shall return a non-NULL MAP_HANDLE.]*/ 00398 IOTHUB_MESSAGE_HANDLE_DATA* handleData = (IOTHUB_MESSAGE_HANDLE_DATA*)iotHubMessageHandle; 00399 result = handleData->properties; 00400 } 00401 return result; 00402 } 00403 00404 const char* IoTHubMessage_GetCorrelationId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle) 00405 { 00406 const char* result; 00407 /* Codes_SRS_IOTHUBMESSAGE_07_016: [if the iotHubMessageHandle parameter is NULL then IoTHubMessage_GetCorrelationId shall return a NULL value.] */ 00408 if (iotHubMessageHandle == NULL) 00409 { 00410 LogError("invalid arg (NULL) passed to IoTHubMessage_GetCorrelationId"); 00411 result = NULL; 00412 } 00413 else 00414 { 00415 /* Codes_SRS_IOTHUBMESSAGE_07_017: [IoTHubMessage_GetCorrelationId shall return the correlationId as a const char*.] */ 00416 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00417 result = handleData->correlationId; 00418 } 00419 return result; 00420 } 00421 00422 IOTHUB_MESSAGE_RESULT IoTHubMessage_SetCorrelationId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle, const char* correlationId) 00423 { 00424 IOTHUB_MESSAGE_RESULT result; 00425 /* Codes_SRS_IOTHUBMESSAGE_07_018: [if any of the parameters are NULL then IoTHubMessage_SetCorrelationId shall return a IOTHUB_MESSAGE_INVALID_ARG value.]*/ 00426 if (iotHubMessageHandle == NULL || correlationId == NULL) 00427 { 00428 LogError("invalid arg (NULL) passed to IoTHubMessage_SetCorrelationId"); 00429 result = IOTHUB_MESSAGE_INVALID_ARG; 00430 } 00431 else 00432 { 00433 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00434 /* Codes_SRS_IOTHUBMESSAGE_07_019: [If the IOTHUB_MESSAGE_HANDLE correlationId is not NULL, then the IOTHUB_MESSAGE_HANDLE correlationId will be deallocated.] */ 00435 if (handleData->correlationId != NULL) 00436 { 00437 free(handleData->correlationId); 00438 } 00439 00440 if (mallocAndStrcpy_s(&handleData->correlationId, correlationId) != 0) 00441 { 00442 /* Codes_SRS_IOTHUBMESSAGE_07_020: [If the allocation or the copying of the correlationId fails, then IoTHubMessage_SetCorrelationId shall return IOTHUB_MESSAGE_ERROR.] */ 00443 result = IOTHUB_MESSAGE_ERROR; 00444 } 00445 else 00446 { 00447 /* Codes_SRS_IOTHUBMESSAGE_07_021: [IoTHubMessage_SetCorrelationId finishes successfully it shall return IOTHUB_MESSAGE_OK.] */ 00448 result = IOTHUB_MESSAGE_OK; 00449 } 00450 } 00451 return result; 00452 } 00453 00454 IOTHUB_MESSAGE_RESULT IoTHubMessage_SetMessageId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle, const char* messageId) 00455 { 00456 IOTHUB_MESSAGE_RESULT result; 00457 /* Codes_SRS_IOTHUBMESSAGE_07_012: [if any of the parameters are NULL then IoTHubMessage_SetMessageId shall return a IOTHUB_MESSAGE_INVALID_ARG value.] */ 00458 if (iotHubMessageHandle == NULL || messageId == NULL) 00459 { 00460 LogError("invalid arg (NULL) passed to IoTHubMessage_SetMessageId"); 00461 result = IOTHUB_MESSAGE_INVALID_ARG; 00462 } 00463 else 00464 { 00465 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00466 /* Codes_SRS_IOTHUBMESSAGE_07_013: [If the IOTHUB_MESSAGE_HANDLE messageId is not NULL, then the IOTHUB_MESSAGE_HANDLE messageId will be freed] */ 00467 if (handleData->messageId != NULL) 00468 { 00469 free(handleData->messageId); 00470 } 00471 00472 /* Codes_SRS_IOTHUBMESSAGE_07_014: [If the allocation or the copying of the messageId fails, then IoTHubMessage_SetMessageId shall return IOTHUB_MESSAGE_ERROR.] */ 00473 if (mallocAndStrcpy_s(&handleData->messageId, messageId) != 0) 00474 { 00475 result = IOTHUB_MESSAGE_ERROR; 00476 } 00477 else 00478 { 00479 result = IOTHUB_MESSAGE_OK; 00480 } 00481 } 00482 return result; 00483 } 00484 00485 const char* IoTHubMessage_GetMessageId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle) 00486 { 00487 const char* result; 00488 /* Codes_SRS_IOTHUBMESSAGE_07_010: [if the iotHubMessageHandle parameter is NULL then IoTHubMessage_MessageId shall return a NULL value.] */ 00489 if (iotHubMessageHandle == NULL) 00490 { 00491 LogError("invalid arg (NULL) passed to IoTHubMessage_GetMessageId"); 00492 result = NULL; 00493 } 00494 else 00495 { 00496 /* Codes_SRS_IOTHUBMESSAGE_07_011: [IoTHubMessage_MessageId shall return the messageId as a const char*.] */ 00497 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00498 result = handleData->messageId; 00499 } 00500 return result; 00501 } 00502 00503 void IoTHubMessage_Destroy(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle) 00504 { 00505 /*Codes_SRS_IOTHUBMESSAGE_01_004: [If iotHubMessageHandle is NULL, IoTHubMessage_Destroy shall do nothing.] */ 00506 if (iotHubMessageHandle != NULL) 00507 { 00508 /*Codes_SRS_IOTHUBMESSAGE_01_003: [IoTHubMessage_Destroy shall free all resources associated with iotHubMessageHandle.] */ 00509 IOTHUB_MESSAGE_HANDLE_DATA* handleData = iotHubMessageHandle; 00510 if (handleData->contentType == IOTHUBMESSAGE_BYTEARRAY) 00511 { 00512 BUFFER_delete(handleData->value.byteArray); 00513 } 00514 else 00515 { 00516 /*can only be STRING*/ 00517 STRING_delete(handleData->value.string); 00518 } 00519 Map_Destroy(handleData->properties); 00520 free(handleData->messageId); 00521 handleData->messageId = NULL; 00522 free(handleData->correlationId); 00523 handleData->correlationId = NULL; 00524 free(handleData); 00525 } 00526 }
Generated on Tue Jul 12 2022 19:44:54 by
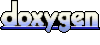