corrected version (with typedef struct IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE_DATA* IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE;) included in the sources
Dependents: STM32F746_iothub_client_sample_mqtt
Fork of iothub_client by
iothub_client_private.h
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 #ifndef IOTHUB_CLIENT_PRIVATE_H 00005 #define IOTHUB_CLIENT_PRIVATE_H 00006 00007 #include <signal.h> 00008 00009 #include "azure_c_shared_utility/macro_utils.h" 00010 #include "azure_c_shared_utility/crt_abstractions.h" 00011 #include "azure_c_shared_utility/doublylinkedlist.h" 00012 00013 #include "iothub_message.h" 00014 #include "iothub_client_ll.h" 00015 00016 #ifdef __cplusplus 00017 extern "C" 00018 { 00019 #endif 00020 00021 #define IOTHUB_BATCHSTATE_RESULT_VALUES IOTHUB_BATCHSTATE_SUCCESS, \ 00022 IOTHUB_BATCHSTATE_FAILED 00023 00024 DEFINE_ENUM(IOTHUB_BATCHSTATE_RESULT, IOTHUB_BATCHSTATE_RESULT_VALUES); 00025 00026 #define EVENT_ENDPOINT "/messages/events" 00027 #define MESSAGE_ENDPOINT "/messages/devicebound" 00028 #define MESSAGE_ENDPOINT_HTTP "/messages/devicebound" 00029 #define MESSAGE_ENDPOINT_HTTP_ETAG "/messages/devicebound/" 00030 #define CLIENT_DEVICE_TYPE_PREFIX "iothubclient" 00031 #define CLIENT_DEVICE_BACKSLASH "/" 00032 #define CBS_REPLY_TO "cbs" 00033 #define CBS_ENDPOINT "/$" CBS_REPLY_TO 00034 #define API_VERSION "?api-version=2016-02-03" 00035 #define REJECT_QUERY_PARAMETER "&reject" 00036 00037 extern void IoTHubClient_LL_SendComplete(IOTHUB_CLIENT_LL_HANDLE handle, PDLIST_ENTRY completed, IOTHUB_BATCHSTATE_RESULT result); 00038 extern IOTHUBMESSAGE_DISPOSITION_RESULT IoTHubClient_LL_MessageCallback(IOTHUB_CLIENT_LL_HANDLE handle, IOTHUB_MESSAGE_HANDLE message); 00039 00040 typedef struct IOTHUB_MESSAGE_LIST_TAG 00041 { 00042 IOTHUB_MESSAGE_HANDLE messageHandle; 00043 IOTHUB_CLIENT_EVENT_CONFIRMATION_CALLBACK callback; 00044 void* context; 00045 DLIST_ENTRY entry; 00046 uint64_t ms_timesOutAfter; /* a value of "0" means "no timeout", if the IOTHUBCLIENT_LL's handle tickcounter > msTimesOutAfer then the message shall timeout*/ 00047 }IOTHUB_MESSAGE_LIST; 00048 00049 00050 #ifdef __cplusplus 00051 } 00052 #endif 00053 00054 #endif /* IOTHUB_CLIENT_PRIVATE_H */
Generated on Tue Jul 12 2022 19:44:54 by
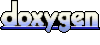