corrected version (with typedef struct IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE_DATA* IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE;) included in the sources
Dependents: STM32F746_iothub_client_sample_mqtt
Fork of iothub_client by
iothub_client_ll.h
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 /** @file iothub_client_ll.h 00005 * @brief APIs that allow a user (usually a device) to communicate 00006 * with an Azure IoTHub. 00007 * 00008 * @details IoTHubClient_LL is a module that allows a user (usually a 00009 * device) to communicate with an Azure IoTHub. It can send events 00010 * and receive messages. At any given moment in time there can only 00011 * be at most 1 message callback function. 00012 * 00013 * This API surface contains a set of APIs that allows the user to 00014 * interact with the lower layer portion of the IoTHubClient. These APIs 00015 * contain @c _LL_ in their name, but retain the same functionality like the 00016 * @c IoTHubClient_... APIs, with one difference. If the @c _LL_ APIs are 00017 * used then the user is responsible for scheduling when the actual work done 00018 * by the IoTHubClient happens (when the data is sent/received on/from the wire). 00019 * This is useful for constrained devices where spinning a separate thread is 00020 * often not desired. 00021 */ 00022 00023 #ifndef IOTHUB_CLIENT_LL_H 00024 #define IOTHUB_CLIENT_LL_H 00025 00026 #include "azure_c_shared_utility/macro_utils.h" 00027 00028 #define IOTHUB_CLIENT_RESULT_VALUES \ 00029 IOTHUB_CLIENT_OK, \ 00030 IOTHUB_CLIENT_INVALID_ARG, \ 00031 IOTHUB_CLIENT_ERROR, \ 00032 IOTHUB_CLIENT_INVALID_SIZE, \ 00033 IOTHUB_CLIENT_INDEFINITE_TIME \ 00034 00035 /** @brief Enumeration specifying the status of calls to various APIs in this module. 00036 */ 00037 00038 DEFINE_ENUM(IOTHUB_CLIENT_RESULT, IOTHUB_CLIENT_RESULT_VALUES); 00039 00040 typedef struct IOTHUBTRANSPORT_CONFIG_TAG IOTHUBTRANSPORT_CONFIG; 00041 00042 typedef struct IOTHUB_CLIENT_LL_HANDLE_DATA_TAG* IOTHUB_CLIENT_LL_HANDLE; 00043 00044 #define IOTHUB_CLIENT_STATUS_VALUES \ 00045 IOTHUB_CLIENT_SEND_STATUS_IDLE, \ 00046 IOTHUB_CLIENT_SEND_STATUS_BUSY \ 00047 00048 /** @brief Enumeration returned by the ::IoTHubClient_LL_GetSendStatus 00049 * API to indicate the current sending status of the IoT Hub client. 00050 */ 00051 DEFINE_ENUM(IOTHUB_CLIENT_STATUS, IOTHUB_CLIENT_STATUS_VALUES); 00052 00053 #include "azure_c_shared_utility/agenttime.h" 00054 #include "azure_c_shared_utility/xio.h" 00055 #include "azure_c_shared_utility/doublylinkedlist.h" 00056 #include "iothub_message.h" 00057 #include "iothub_transport_ll.h" 00058 00059 #ifdef __cplusplus 00060 extern "C" 00061 { 00062 #endif 00063 00064 #define IOTHUB_CLIENT_CONFIRMATION_RESULT_VALUES \ 00065 IOTHUB_CLIENT_CONFIRMATION_OK, \ 00066 IOTHUB_CLIENT_CONFIRMATION_BECAUSE_DESTROY, \ 00067 IOTHUB_CLIENT_CONFIRMATION_MESSAGE_TIMEOUT, \ 00068 IOTHUB_CLIENT_CONFIRMATION_ERROR \ 00069 00070 /** @brief Enumeration passed in by the IoT Hub when the event confirmation 00071 * callback is invoked to indicate status of the event processing in 00072 * the hub. 00073 */ 00074 DEFINE_ENUM(IOTHUB_CLIENT_CONFIRMATION_RESULT, IOTHUB_CLIENT_CONFIRMATION_RESULT_VALUES); 00075 00076 #define TRANSPORT_TYPE_VALUES \ 00077 TRANSPORT_LL, /*LL comes from "LowLevel" */ \ 00078 TRANSPORT_THREADED 00079 00080 DEFINE_ENUM(TRANSPORT_TYPE, TRANSPORT_TYPE_VALUES); 00081 00082 #define IOTHUBMESSAGE_DISPOSITION_RESULT_VALUES \ 00083 IOTHUBMESSAGE_ACCEPTED, \ 00084 IOTHUBMESSAGE_REJECTED, \ 00085 IOTHUBMESSAGE_ABANDONED 00086 00087 /** @brief Enumeration returned by the callback which is invoked whenever the 00088 * IoT Hub sends a message to the device. 00089 */ 00090 DEFINE_ENUM(IOTHUBMESSAGE_DISPOSITION_RESULT, IOTHUBMESSAGE_DISPOSITION_RESULT_VALUES); 00091 00092 00093 typedef void(*IOTHUB_CLIENT_EVENT_CONFIRMATION_CALLBACK)(IOTHUB_CLIENT_CONFIRMATION_RESULT result, void* userContextCallback); 00094 typedef IOTHUBMESSAGE_DISPOSITION_RESULT (*IOTHUB_CLIENT_MESSAGE_CALLBACK_ASYNC)(IOTHUB_MESSAGE_HANDLE message, void* userContextCallback); 00095 typedef const TRANSPORT_PROVIDER*(*IOTHUB_CLIENT_TRANSPORT_PROVIDER)(void); 00096 00097 /** @brief This struct captures IoTHub client configuration. */ 00098 typedef struct IOTHUB_CLIENT_CONFIG_TAG 00099 { 00100 /** @brief A function pointer that is passed into the @c IoTHubClientCreate. 00101 * A function definition for AMQP is defined in the include @c iothubtransportamqp.h. 00102 * A function definition for HTTP is defined in the include @c iothubtransporthttp.h 00103 * A function definition for MQTT is defined in the include @c iothubtransportmqtt.h */ 00104 IOTHUB_CLIENT_TRANSPORT_PROVIDER protocol; 00105 00106 /** @brief A string that identifies the device. */ 00107 const char* deviceId; 00108 00109 /** @brief The device key used to authenticate the device. */ 00110 const char* deviceKey; 00111 00112 /** @brief The device SAS Token used to authenticate the device in place of device key. */ 00113 const char* deviceSasToken; 00114 00115 /** @brief The IoT Hub name to which the device is connecting. */ 00116 const char* iotHubName; 00117 00118 /** @brief IoT Hub suffix goes here, e.g., private.azure-devices-int.net. */ 00119 const char* iotHubSuffix; 00120 00121 const char* protocolGatewayHostName; 00122 } IOTHUB_CLIENT_CONFIG; 00123 00124 /** @brief This struct captures IoTHub client device configuration. */ 00125 typedef struct IOTHUB_CLIENT_DEVICE_CONFIG_TAG 00126 { 00127 /** @brief A function pointer that is passed into the @c IoTHubClientCreate. 00128 * A function definition for AMQP is defined in the include @c iothubtransportamqp.h. 00129 * A function definition for HTTP is defined in the include @c iothubtransporthttp.h 00130 * A function definition for MQTT is defined in the include @c iothubtransportmqtt.h */ 00131 IOTHUB_CLIENT_TRANSPORT_PROVIDER protocol; 00132 00133 /** @brief a transport handle implementing the protocol */ 00134 void * transportHandle; 00135 00136 /** @brief A string that identifies the device. */ 00137 const char* deviceId; 00138 00139 /** @brief The device key used to authenticate the device. */ 00140 const char* deviceKey; 00141 00142 /** @brief The device SAS Token used to authenticate the device in place of device key. */ 00143 const char* deviceSasToken; 00144 } IOTHUB_CLIENT_DEVICE_CONFIG; 00145 00146 /** @brief This struct captures IoTHub transport configuration. */ 00147 typedef struct IOTHUBTRANSPORT_CONFIG_TAG 00148 { 00149 const IOTHUB_CLIENT_CONFIG* upperConfig; 00150 PDLIST_ENTRY waitingToSend; 00151 }IOTHUBTRANSPORT_CONFIG; 00152 00153 00154 /** 00155 * @brief Creates a IoT Hub client for communication with an existing 00156 * IoT Hub using the specified connection string parameter. 00157 * 00158 * @param connectionString Pointer to a character string 00159 * @param protocol Function pointer for protocol implementation 00160 * 00161 * Sample connection string: 00162 * <blockquote> 00163 * <pre>HostName=[IoT Hub name goes here].[IoT Hub suffix goes here, e.g., private.azure-devices-int.net];DeviceId=[Device ID goes here];SharedAccessKey=[Device key goes here];</pre> 00164 * </blockquote> 00165 * 00166 * @return A non-NULL @c IOTHUB_CLIENT_LL_HANDLE value that is used when 00167 * invoking other functions for IoT Hub client and @c NULL on failure. 00168 */ 00169 extern IOTHUB_CLIENT_LL_HANDLE IoTHubClient_LL_CreateFromConnectionString(const char* connectionString, IOTHUB_CLIENT_TRANSPORT_PROVIDER protocol); 00170 00171 /** 00172 * @brief Creates a IoT Hub client for communication with an existing IoT 00173 * Hub using the specified parameters. 00174 * 00175 * @param config Pointer to an @c IOTHUB_CLIENT_CONFIG structure 00176 * 00177 * The API does not allow sharing of a connection across multiple 00178 * devices. This is a blocking call. 00179 * 00180 * @return A non-NULL @c IOTHUB_CLIENT_LL_HANDLE value that is used when 00181 * invoking other functions for IoT Hub client and @c NULL on failure. 00182 */ 00183 extern IOTHUB_CLIENT_LL_HANDLE IoTHubClient_LL_Create(const IOTHUB_CLIENT_CONFIG* config); 00184 00185 /** 00186 * @brief Creates a IoT Hub client for communication with an existing IoT 00187 * Hub using an existing transport. 00188 * 00189 * @param config Pointer to an @c IOTHUB_CLIENT_DEVICE_CONFIG structure 00190 * 00191 * The API *allows* sharing of a connection across multiple 00192 * devices. This is a blocking call. 00193 * 00194 * @return A non-NULL @c IOTHUB_CLIENT_LL_HANDLE value that is used when 00195 * invoking other functions for IoT Hub client and @c NULL on failure. 00196 */ 00197 extern IOTHUB_CLIENT_LL_HANDLE IoTHubClient_LL_CreateWithTransport(const IOTHUB_CLIENT_DEVICE_CONFIG * config); 00198 00199 /** 00200 * @brief Disposes of resources allocated by the IoT Hub client. This is a 00201 * blocking call. 00202 * 00203 * @param iotHubClientHandle The handle created by a call to the create function. 00204 */ 00205 extern void IoTHubClient_LL_Destroy(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle); 00206 00207 /** 00208 * @brief Asynchronous call to send the message specified by @p eventMessageHandle. 00209 * 00210 * @param iotHubClientHandle The handle created by a call to the create function. 00211 * @param eventMessageHandle The handle to an IoT Hub message. 00212 * @param eventConfirmationCallback The callback specified by the device for receiving 00213 * confirmation of the delivery of the IoT Hub message. 00214 * This callback can be expected to invoke the 00215 * ::IoTHubClient_LL_SendEventAsync function for the 00216 * same message in an attempt to retry sending a failing 00217 * message. The user can specify a @c NULL value here to 00218 * indicate that no callback is required. 00219 * @param userContextCallback User specified context that will be provided to the 00220 * callback. This can be @c NULL. 00221 * 00222 * @b NOTE: The application behavior is undefined if the user calls 00223 * the ::IoTHubClient_LL_Destroy function from within any callback. 00224 * 00225 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00226 */ 00227 extern IOTHUB_CLIENT_RESULT IoTHubClient_LL_SendEventAsync(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle, IOTHUB_MESSAGE_HANDLE eventMessageHandle, IOTHUB_CLIENT_EVENT_CONFIRMATION_CALLBACK eventConfirmationCallback, void* userContextCallback); 00228 00229 /** 00230 * @brief This function returns the current sending status for IoTHubClient. 00231 * 00232 * @param iotHubClientHandle The handle created by a call to the create function. 00233 * @param iotHubClientStatus The sending state is populated at the address pointed 00234 * at by this parameter. The value will be set to 00235 * @c IOTHUBCLIENT_SENDSTATUS_IDLE if there is currently 00236 * no item to be sent and @c IOTHUBCLIENT_SENDSTATUS_BUSY 00237 * if there are. 00238 * 00239 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00240 */ 00241 extern IOTHUB_CLIENT_RESULT IoTHubClient_LL_GetSendStatus(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle, IOTHUB_CLIENT_STATUS *iotHubClientStatus); 00242 00243 /** 00244 * @brief Sets up the message callback to be invoked when IoT Hub issues a 00245 * message to the device. This is a blocking call. 00246 * 00247 * @param iotHubClientHandle The handle created by a call to the create function. 00248 * @param messageCallback The callback specified by the device for receiving 00249 * messages from IoT Hub. 00250 * @param userContextCallback User specified context that will be provided to the 00251 * callback. This can be @c NULL. 00252 * 00253 * @b NOTE: The application behavior is undefined if the user calls 00254 * the ::IoTHubClient_LL_Destroy function from within any callback. 00255 * 00256 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00257 */ 00258 extern IOTHUB_CLIENT_RESULT IoTHubClient_LL_SetMessageCallback(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle, IOTHUB_CLIENT_MESSAGE_CALLBACK_ASYNC messageCallback, void* userContextCallback); 00259 00260 /** 00261 * @brief This function returns in the out parameter @p lastMessageReceiveTime 00262 * what was the value of the @c time function when the last message was 00263 * received at the client. 00264 * 00265 * @param iotHubClientHandle The handle created by a call to the create function. 00266 * @param lastMessageReceiveTime Out parameter containing the value of @c time function 00267 * when the last message was received. 00268 * 00269 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00270 */ 00271 extern IOTHUB_CLIENT_RESULT IoTHubClient_LL_GetLastMessageReceiveTime(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle, time_t* lastMessageReceiveTime); 00272 00273 /** 00274 * @brief This function is meant to be called by the user when work 00275 * (sending/receiving) can be done by the IoTHubClient. 00276 * 00277 * @param iotHubClientHandle The handle created by a call to the create function. 00278 * 00279 * All IoTHubClient interactions (in regards to network traffic 00280 * and/or user level callbacks) are the effect of calling this 00281 * function and they take place synchronously inside _DoWork. 00282 */ 00283 extern void IoTHubClient_LL_DoWork(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle); 00284 00285 /** 00286 * @brief This API sets a runtime option identified by parameter @p optionName 00287 * to a value pointed to by @p value. @p optionName and the data type 00288 * @p value is pointing to are specific for every option. 00289 * 00290 * @param iotHubClientHandle The handle created by a call to the create function. 00291 * @param optionName Name of the option. 00292 * @param value The value. 00293 * 00294 * The options that can be set via this API are: 00295 * - @b timeout - the maximum time in milliseconds a communication is 00296 * allowed to use. @p value is a pointer to an @c unsigned @c int with 00297 * the timeout value in milliseconds. This is only supported for the HTTP 00298 * protocol as of now. When the HTTP protocol uses CURL, the meaning of 00299 * the parameter is <em>total request time</em>. When the HTTP protocol uses 00300 * winhttp, the meaning is the same as the @c dwSendTimeout and 00301 * @c dwReceiveTimeout parameters of the 00302 * <a href="https://msdn.microsoft.com/en-us/library/windows/desktop/aa384116(v=vs.85).aspx"> 00303 * WinHttpSetTimeouts</a> API. 00304 * - @b CURLOPT_LOW_SPEED_LIMIT - only available for HTTP protocol and only 00305 * when CURL is used. It has the same meaning as CURL's option with the same 00306 * name. @p value is pointer to a long. 00307 * - @b CURLOPT_LOW_SPEED_TIME - only available for HTTP protocol and only 00308 * when CURL is used. It has the same meaning as CURL's option with the same 00309 * name. @p value is pointer to a long. 00310 * - @b CURLOPT_FORBID_REUSE - only available for HTTP protocol and only 00311 * when CURL is used. It has the same meaning as CURL's option with the same 00312 * name. @p value is pointer to a long. 00313 * - @b CURLOPT_FRESH_CONNECT - only available for HTTP protocol and only 00314 * when CURL is used. It has the same meaning as CURL's option with the same 00315 * name. @p value is pointer to a long. 00316 * - @b CURLOPT_VERBOSE - only available for HTTP protocol and only 00317 * when CURL is used. It has the same meaning as CURL's option with the same 00318 * name. @p value is pointer to a long. 00319 * - @b keepalive - available for MQTT protocol. Integer value that sets the 00320 * interval in seconds when pings are sent to the server. 00321 * - @b logtrace - available for MQTT protocol. Boolean value that turns on and 00322 * off the diagnostic logging. 00323 * 00324 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00325 */ 00326 extern IOTHUB_CLIENT_RESULT IoTHubClient_LL_SetOption(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle, const char* optionName, const void* value); 00327 00328 #ifdef USE_UPLOADTOBLOB 00329 /** 00330 * @brief This API uploads to Azure Storage the content pointed to by @p source having the size @p size 00331 * under the blob name devicename/@pdestinationFileName 00332 * 00333 * @param iotHubClientHandle The handle created by a call to the create function. 00334 * @param destinationFileName name of the file. 00335 * @param source pointer to the source for file content (can be NULL) 00336 * @param size the size of the source in memory (if @p source is NULL then size needs to be 0). 00337 * 00338 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00339 */ 00340 extern IOTHUB_CLIENT_RESULT IoTHubClient_LL_UploadToBlob(IOTHUB_CLIENT_LL_HANDLE iotHubClientHandle, const char* destinationFileName, const unsigned char* source, size_t size); 00341 00342 #endif /*USE_UPLOADTOBLOB*/ 00343 00344 #ifdef __cplusplus 00345 } 00346 #endif 00347 00348 #endif /* IOTHUB_CLIENT_LL_H */
Generated on Tue Jul 12 2022 19:44:54 by
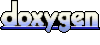