corrected version (with typedef struct IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE_DATA* IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE;) included in the sources
Dependents: STM32F746_iothub_client_sample_mqtt
Fork of iothub_client by
iothub_client.h
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 /** @file iothub_client.h 00005 * @brief Extends the IoTHubCLient_LL module with additional features. 00006 * 00007 * @details IoTHubClient is a module that extends the IoTHubCLient_LL 00008 * module with 2 features: 00009 * - scheduling the work for the IoTHubCLient from a 00010 * thread, so that the user does not need to create their 00011 * own thread 00012 * - thread-safe APIs 00013 */ 00014 00015 #ifndef IOTHUB_CLIENT_H 00016 #define IOTHUB_CLIENT_H 00017 00018 typedef struct IOTHUB_CLIENT_INSTANCE_TAG* IOTHUB_CLIENT_HANDLE; 00019 00020 #include "iothub_client_ll.h" 00021 #include "iothubtransport.h" 00022 00023 #ifdef __cplusplus 00024 extern "C" 00025 { 00026 #endif 00027 00028 #define IOTHUB_CLIENT_FILE_UPLOAD_RESULT_VALUES \ 00029 FILE_UPLOAD_OK ,\ 00030 FILE_UPLOAD_ERROR 00031 00032 DEFINE_ENUM(IOTHUB_CLIENT_FILE_UPLOAD_RESULT, IOTHUB_CLIENT_FILE_UPLOAD_RESULT_VALUES) 00033 typedef void(*IOTHUB_CLIENT_FILE_UPLOAD_CALLBACK)(IOTHUB_CLIENT_FILE_UPLOAD_RESULT result, void* userContextCallback); 00034 00035 /** 00036 * @brief Creates a IoT Hub client for communication with an existing 00037 * IoT Hub using the specified connection string parameter. 00038 * 00039 * @param connectionString Pointer to a character string 00040 * @param protocol Function pointer for protocol implementation 00041 * 00042 * Sample connection string: 00043 * <blockquote> 00044 * <pre>HostName=[IoT Hub name goes here].[IoT Hub suffix goes here, e.g., private.azure-devices-int.net];DeviceId=[Device ID goes here];SharedAccessKey=[Device key goes here];</pre> 00045 * <pre>HostName=[IoT Hub name goes here].[IoT Hub suffix goes here, e.g., private.azure-devices-int.net];DeviceId=[Device ID goes here];SharedAccessSignature=SharedAccessSignature sr=[IoT Hub name goes here].[IoT Hub suffix goes here, e.g., private.azure-devices-int.net]/devices/[Device ID goes here]&sig=[SAS Token goes here]&se=[Expiry Time goes here];</pre> 00046 * </blockquote> 00047 * 00048 * @return A non-NULL @c IOTHUB_CLIENT_HANDLE value that is used when 00049 * invoking other functions for IoT Hub client and @c NULL on failure. 00050 */ 00051 extern IOTHUB_CLIENT_HANDLE IoTHubClient_CreateFromConnectionString(const char* connectionString, IOTHUB_CLIENT_TRANSPORT_PROVIDER protocol); 00052 00053 /** 00054 * @brief Creates a IoT Hub client for communication with an existing IoT 00055 * Hub using the specified parameters. 00056 * 00057 * @param config Pointer to an @c IOTHUB_CLIENT_CONFIG structure 00058 * 00059 * The API does not allow sharing of a connection across multiple 00060 * devices. This is a blocking call. 00061 * 00062 * @return A non-NULL @c IOTHUB_CLIENT_HANDLE value that is used when 00063 * invoking other functions for IoT Hub client and @c NULL on failure. 00064 */ 00065 extern IOTHUB_CLIENT_HANDLE IoTHubClient_Create(const IOTHUB_CLIENT_CONFIG* config); 00066 00067 /** 00068 * @brief Creates a IoT Hub client for communication with an existing IoT 00069 * Hub using the specified parameters. 00070 * 00071 * @param transportHandle TRANSPORT_HANDLE which represents a connection. 00072 * @param config Pointer to an @c IOTHUB_CLIENT_CONFIG structure 00073 * 00074 * The API allows sharing of a connection across multiple 00075 * devices. This is a blocking call. 00076 * 00077 * @return A non-NULL @c IOTHUB_CLIENT_HANDLE value that is used when 00078 * invoking other functions for IoT Hub client and @c NULL on failure. 00079 */ 00080 extern IOTHUB_CLIENT_HANDLE IoTHubClient_CreateWithTransport(TRANSPORT_HANDLE transportHandle, const IOTHUB_CLIENT_CONFIG* config); 00081 00082 /** 00083 * @brief Disposes of resources allocated by the IoT Hub client. This is a 00084 * blocking call. 00085 * 00086 * @param iotHubClientHandle The handle created by a call to the create function. 00087 */ 00088 extern void IoTHubClient_Destroy(IOTHUB_CLIENT_HANDLE iotHubClientHandle); 00089 00090 /** 00091 * @brief Asynchronous call to send the message specified by @p eventMessageHandle. 00092 * 00093 * @param iotHubClientHandle The handle created by a call to the create function. 00094 * @param eventMessageHandle The handle to an IoT Hub message. 00095 * @param eventConfirmationCallback The callback specified by the device for receiving 00096 * confirmation of the delivery of the IoT Hub message. 00097 * This callback can be expected to invoke the 00098 * ::IoTHubClient_SendEventAsync function for the 00099 * same message in an attempt to retry sending a failing 00100 * message. The user can specify a @c NULL value here to 00101 * indicate that no callback is required. 00102 * @param userContextCallback User specified context that will be provided to the 00103 * callback. This can be @c NULL. 00104 * 00105 * @b NOTE: The application behavior is undefined if the user calls 00106 * the ::IoTHubClient_Destroy function from within any callback. 00107 * 00108 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00109 */ 00110 extern IOTHUB_CLIENT_RESULT IoTHubClient_SendEventAsync(IOTHUB_CLIENT_HANDLE iotHubClientHandle, IOTHUB_MESSAGE_HANDLE eventMessageHandle, IOTHUB_CLIENT_EVENT_CONFIRMATION_CALLBACK eventConfirmationCallback, void* userContextCallback); 00111 00112 /** 00113 * @brief This function returns the current sending status for IoTHubClient. 00114 * 00115 * @param iotHubClientHandle The handle created by a call to the create function. 00116 * @param iotHubClientStatus The sending state is populated at the address pointed 00117 * at by this parameter. The value will be set to 00118 * @c IOTHUBCLIENT_SENDSTATUS_IDLE if there is currently 00119 * no item to be sent and @c IOTHUBCLIENT_SENDSTATUS_BUSY 00120 * if there are. 00121 * 00122 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00123 */ 00124 extern IOTHUB_CLIENT_RESULT IoTHubClient_GetSendStatus(IOTHUB_CLIENT_HANDLE iotHubClientHandle, IOTHUB_CLIENT_STATUS *iotHubClientStatus); 00125 00126 /** 00127 * @brief Sets up the message callback to be invoked when IoT Hub issues a 00128 * message to the device. This is a blocking call. 00129 * 00130 * @param iotHubClientHandle The handle created by a call to the create function. 00131 * @param messageCallback The callback specified by the device for receiving 00132 * messages from IoT Hub. 00133 * @param userContextCallback User specified context that will be provided to the 00134 * callback. This can be @c NULL. 00135 * 00136 * @b NOTE: The application behavior is undefined if the user calls 00137 * the ::IoTHubClient_Destroy function from within any callback. 00138 * 00139 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00140 */ 00141 extern IOTHUB_CLIENT_RESULT IoTHubClient_SetMessageCallback(IOTHUB_CLIENT_HANDLE iotHubClientHandle, IOTHUB_CLIENT_MESSAGE_CALLBACK_ASYNC messageCallback, void* userContextCallback); 00142 00143 /** 00144 * @brief This function returns in the out parameter @p lastMessageReceiveTime 00145 * what was the value of the @c time function when the last message was 00146 * received at the client. 00147 * 00148 * @param iotHubClientHandle The handle created by a call to the create function. 00149 * @param lastMessageReceiveTime Out parameter containing the value of @c time function 00150 * when the last message was received. 00151 * 00152 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00153 */ 00154 extern IOTHUB_CLIENT_RESULT IoTHubClient_GetLastMessageReceiveTime(IOTHUB_CLIENT_HANDLE iotHubClientHandle, time_t* lastMessageReceiveTime); 00155 00156 /** 00157 * @brief This API sets a runtime option identified by parameter @p optionName 00158 * to a value pointed to by @p value. @p optionName and the data type 00159 * @p value is pointing to are specific for every option. 00160 * 00161 * @param iotHubClientHandle The handle created by a call to the create function. 00162 * @param optionName Name of the option. 00163 * @param value The value. 00164 * 00165 * The options that can be set via this API are: 00166 * - @b timeout - the maximum time in milliseconds a communication is 00167 * allowed to use. @p value is a pointer to an @c unsigned @c int with 00168 * the timeout value in milliseconds. This is only supported for the HTTP 00169 * protocol as of now. When the HTTP protocol uses CURL, the meaning of 00170 * the parameter is <em>total request time</em>. When the HTTP protocol uses 00171 * winhttp, the meaning is the same as the @c dwSendTimeout and 00172 * @c dwReceiveTimeout parameters of the 00173 * <a href="https://msdn.microsoft.com/en-us/library/windows/desktop/aa384116(v=vs.85).aspx"> 00174 * WinHttpSetTimeouts</a> API. 00175 * - @b CURLOPT_LOW_SPEED_LIMIT - only available for HTTP protocol and only 00176 * when CURL is used. It has the same meaning as CURL's option with the same 00177 * name. @p value is pointer to a long. 00178 * - @b CURLOPT_LOW_SPEED_TIME - only available for HTTP protocol and only 00179 * when CURL is used. It has the same meaning as CURL's option with the same 00180 * name. @p value is pointer to a long. 00181 * - @b CURLOPT_FORBID_REUSE - only available for HTTP protocol and only 00182 * when CURL is used. It has the same meaning as CURL's option with the same 00183 * name. @p value is pointer to a long. 00184 * - @b CURLOPT_FRESH_CONNECT - only available for HTTP protocol and only 00185 * when CURL is used. It has the same meaning as CURL's option with the same 00186 * name. @p value is pointer to a long. 00187 * - @b CURLOPT_VERBOSE - only available for HTTP protocol and only 00188 * when CURL is used. It has the same meaning as CURL's option with the same 00189 * name. @p value is pointer to a long. 00190 * - @b messageTimeout - the maximum time in milliseconds until a message 00191 * is timeouted. The time starts at IoTHubClient_SendEventAsync. By default, 00192 * messages do not expire. @p is a pointer to a uint64_t 00193 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00194 */ 00195 extern IOTHUB_CLIENT_RESULT IoTHubClient_SetOption(IOTHUB_CLIENT_HANDLE iotHubClientHandle, const char* optionName, const void* value); 00196 00197 #ifdef USE_UPLOADTOBLOB 00198 /** 00199 * @brief IoTHubClient_UploadToBlobAsync uploads data from memory to a file in Azure Blob Storage. 00200 * 00201 * @param iotHubClientHandle The handle created by a call to the IoTHubClient_Create function. 00202 * @param destinationFileName The name of the file to be created in Azure Blob Storage. 00203 * @param source The source of data. 00204 * @param size The size of data. 00205 * @param iotHubClientFileUploadCallback A callback to be invoked when the file upload operation has finished. 00206 * @param context A user-provided context to be passed to the file upload callback. 00207 * 00208 * @return IOTHUB_CLIENT_OK upon success or an error code upon failure. 00209 */ 00210 extern IOTHUB_CLIENT_RESULT IoTHubClient_UploadToBlobAsync(IOTHUB_CLIENT_HANDLE iotHubClientHandle, const char* destinationFileName, const unsigned char* source, size_t size, IOTHUB_CLIENT_FILE_UPLOAD_CALLBACK iotHubClientFileUploadCallback, void* context); 00211 #endif 00212 #ifdef __cplusplus 00213 } 00214 #endif 00215 00216 #endif /* IOTHUB_CLIENT_H */
Generated on Tue Jul 12 2022 19:44:54 by
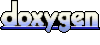