corrected version (with typedef struct IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE_DATA* IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE;) included in the sources
Dependents: STM32F746_iothub_client_sample_mqtt
Fork of iothub_client by
blob.h
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 /** @file blob.h 00005 * @brief Contains blob APIs needed for File Upload feature of IoTHub client. 00006 * 00007 * @details IoTHub client needs to upload a byte array by using blob storage API 00008 * IoTHub service provides the complete SAS URI to execute a PUT request 00009 * that will upload the data. 00010 * 00011 */ 00012 00013 #ifndef BLOB_H 00014 #define BLOB_H 00015 00016 #include "azure_c_shared_utility/macro_utils.h" 00017 #include "azure_c_shared_utility/buffer_.h" 00018 00019 #ifdef __cplusplus 00020 #include <cstddef> 00021 extern "C" 00022 { 00023 #else 00024 #include <stddef.h> 00025 #endif 00026 00027 #include "azure_c_shared_utility/umock_c_prod.h" 00028 00029 #define BLOB_RESULT_VALUES \ 00030 BLOB_OK, \ 00031 BLOB_ERROR, \ 00032 BLOB_NOT_IMPLEMENTED, \ 00033 BLOB_HTTP_ERROR, \ 00034 BLOB_INVALID_ARG 00035 00036 DEFINE_ENUM(BLOB_RESULT, BLOB_RESULT_VALUES) 00037 00038 /** 00039 * @brief Synchronously uploads a byte array to blob storage 00040 * 00041 * @param SASURI The URI to use to upload data 00042 * @param size The size of the data to be uploaded (can be 0) 00043 * @param source A pointer to the byte array to be uploaded (can be NULL, but then size needs to be zero) 00044 * @param httpStatus A pointer to an out argument receiving the HTTP status (available only when the return value is BLOB_OK) 00045 * @param httpResponse A BUFFER_HANDLE that receives the HTTP response from the server (available only when the return value is BLOB_OK) 00046 * 00047 * @return A @c BLOB_RESULT. BLOB_OK means the blob has been uploaded successfully. Any other value indicates an error 00048 */ 00049 MOCKABLE_FUNCTION(, BLOB_RESULT, Blob_UploadFromSasUri,const char*, SASURI, const unsigned char*, source, size_t, size, unsigned int*, httpStatus, BUFFER_HANDLE, httpResponse) 00050 00051 #ifdef __cplusplus 00052 } 00053 #endif 00054 00055 #endif /* BLOB_H */
Generated on Tue Jul 12 2022 19:44:54 by
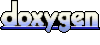