
David's dead reckoning code for the LVBots competition on March 6th. Uses the mbed LPC1768, DRV8835, QTR-3RC, and two DC motors with encoders.
Dependencies: PololuEncoder Pacer mbed GeneralDebouncer
buttons.cpp
00001 #include <mbed.h> 00002 #include "buttons.h" 00003 #include "GeneralDebouncer.h" 00004 00005 #define BUTTON_DEBOUNCE_TIME 20000 00006 00007 DigitalIn button1(p13); 00008 00009 GeneralDebouncer button1Debouncer(5000); 00010 00011 void buttonsInit() 00012 { 00013 button1.mode(PullUp); 00014 } 00015 00016 bool button1IsPressed() 00017 { 00018 return button1.read() == 0; 00019 } 00020 00021 void button1Montior() 00022 { 00023 button1Debouncer.update(button1IsPressed()); 00024 } 00025 00026 bool button1DefinitelyInState(bool state) 00027 { 00028 button1Montior(); 00029 return button1Debouncer.getState() == state && 00030 button1Debouncer.getTimeInCurrentStateMicroseconds() > BUTTON_DEBOUNCE_TIME; 00031 } 00032 00033 bool button1DefinitelyPressed() 00034 { 00035 return button1DefinitelyInState(true); 00036 } 00037 00038 bool button1DefinitelyReleased() 00039 { 00040 return button1DefinitelyInState(false); 00041 } 00042 00043
Generated on Sun Jul 17 2022 15:45:15 by
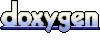