Allows Asynchronous reading from the analog pins to memory. Supports: K64F
Embed:
(wiki syntax)
Show/hide line numbers
asyncADC.h
00001 #ifndef ASYNC_ADC_H__ 00002 #define ASYNC_ADC_H__ 00003 00004 #include "mbed.h" 00005 #include "fsl_edma.h" 00006 #include "fsl_adc16.h" 00007 #include "fsl_pdb.h" 00008 00009 #define DMA_AUTO (-1) 00010 00011 typedef void (*asyncISR)(uint32_t start, uint32_t end); 00012 00013 typedef enum { 00014 SUCCESS = 0, // Return value on successful initialisation of async analog initiation 00015 E_ASYNC_ADC_ACTIVE, // If an async analog read is initiated whilst another is on going 00016 E_DMA_IN_USE, // If the channel is in use (Specified) or there are no free DMA channels (Auto). 00017 E_INVALID_BUFFER_SIZE // If the provided buffer size is non-positive or for a non power of 2 circular buffer 00018 } async_error_t; 00019 00020 /*! 00021 * @brief Computes the nearest achievable frequency to the target frequency. 00022 * 00023 * The floating-point sampling frequency is required for fractional values. 00024 * 00025 * @param targetFrequency The target frequency to achieve 00026 */ 00027 uint32_t approximateFrequency(uint32_t targetFrequency); 00028 00029 /*! 00030 * @brief Computes the nearest achievable frequency to the target frequency. 00031 * 00032 * The integer sampling frequency is recommended for values over 10kHz. 00033 * 00034 * @param targetFrequency The target frequency to achieve 00035 */ 00036 float approximateFrequency(float targetFrequency); 00037 00038 /*! 00039 * @brief Initiates an asynchronus analog read from 'source' to 'destination' 00040 * using the PDB to drive the Analog conversion and DMA to transfer the result 00041 * to the destination buffer. 00042 * 00043 * The read will fill the destination buffer and then terminate. A call to 00044 * terminateAsyncRead() can terminate the process early. 00045 * 00046 * The integer sampling frequency is recommended for values over 10kHz. 00047 * 00048 * @param source The source pin to read from. Must be Analog compatiable 00049 * @param destination A pointer to the destination array. Must be positive. 00050 * @param destSize The size of the destination array. 00051 * @param sampleFrequency The desired sampling frequency from the source pin 00052 * @param callback An optional callback function to be executed once the 00053 * destination buffer is filled. 00054 * @param dmaChannel The optional desired DMA channel to use, or DMA_AUTO if the 00055 * first free dma channel is to be used. Defaults to DMA_AUTO. 00056 */ 00057 async_error_t asyncAnalogToBuffer(PinName source, uint16_t* destination, uint32_t destSize, uint32_t sampleFrequency, asyncISR callback = NULL, int16_t dmaChannel = DMA_AUTO); 00058 00059 /*! 00060 * @brief Initiates an asynchronus analog read from 'source' to 'destination' 00061 * using the PDB to drive the Analog conversion and DMA to transfer the result 00062 * to the destination buffer. 00063 * 00064 * The read will fill the destination buffer, then cycle back to the start of 00065 * the destination buffer and repeat continuously until explicitly terminated by 00066 * a call to terminateAsyncRead(). 00067 * 00068 * The integer sampling frequency is recommended for values over 10kHz. 00069 * 00070 * @brief Initiates an asynchronus analog read from 'source' to 'destination' 00071 * using the PDB to drive the Analog conversion and DMA to transfer the result 00072 * to the destination buffer. The integer sampling frequency is recommended for 00073 * values over 10kHz. 00074 * @param source The source pin to read from. Must be Analog compatiable 00075 * @param destination A pointer to the destination array. 00076 * @param destination A pointer to the destination array. Must be a positive 00077 * power of two. 00078 * @param sampleFrequency The desired sampling frequency from the source pin 00079 * @param callback An optional callback function to be executed after each half 00080 * of the destination buffer is filled. 00081 * @param dmaChannel The optional desired DMA channel to use, or DMA_AUTO if the 00082 * first free dma channel is to be used. Defaults to DMA_AUTO. 00083 */ 00084 async_error_t asyncAnalogToCircularBuffer(PinName source, uint16_t* destination, uint32_t destSize, uint32_t sampleFrequency, asyncISR callback = NULL, int16_t dmaChannel = DMA_AUTO); 00085 00086 /*! 00087 * @brief Initiates an asynchronus analog read from 'source' to 'destination' 00088 * using the PDB to drive the Analog conversion and DMA to transfer the result 00089 * to the destination buffer. 00090 * 00091 * The read will fill the destination buffer and then terminate. A call to 00092 * terminateAsyncRead() can terminate the process early. 00093 * 00094 * The floating-point sampling frequency is required for fractional values. 00095 * 00096 * @param source The source pin to read from. Must be Analog compatiable 00097 * @param destination A pointer to the destination array. 00098 * @param destination A pointer to the destination array. Must be positive. 00099 * @param sampleFrequency The desired sampling frequency from the source pin 00100 * @param callback An optional callback function to be executed once the 00101 * destination buffer is filled. 00102 * @param dmaChannel The optional desired DMA channel to use, or DMA_AUTO if the 00103 * first free dma channel is to be used. Defaults to DMA_AUTO. 00104 */ 00105 async_error_t asyncAnalogToBuffer_f(PinName source, uint16_t* destination, uint32_t destSize, float sampleFrequency, asyncISR callback = NULL, int16_t dmaChannel = DMA_AUTO); 00106 00107 /*! 00108 * @brief Initiates an asynchronus analog read from 'source' to 'destination' 00109 * using the PDB to drive the Analog conversion and DMA to transfer the result 00110 * to the destination buffer. 00111 * 00112 * The read will fill the destination buffer, then cycle back to the start of 00113 * the destination buffer and repeat continuously until explicitly terminated by 00114 * a call to terminateAsyncRead(). 00115 * 00116 * The floating-point sampling frequency is required for fractional values. 00117 * 00118 * @param source The source pin to read from. Must be Analog compatiable 00119 * @param destination A pointer to the destination array. 00120 * @param destination A pointer to the destination array. Must be a positive 00121 * power of two. 00122 * @param sampleFrequency The desired sampling frequency from the source pin 00123 * @param callback An optional callback function to be executed after each half 00124 * of the destination buffer is filled. 00125 * @param dmaChannel The optional desired DMA channel to use, or DMA_AUTO if the 00126 * first free dma channel is to be used. Defaults to DMA_AUTO. 00127 */ 00128 async_error_t asyncAnalogToCircularBuffer_f(PinName source, uint16_t* destination, uint32_t destSize, float sampleFrequency, asyncISR callback = NULL, int16_t dmaChannel = DMA_AUTO); 00129 00130 /*! 00131 * @brief Terminates any on going async analog reads. Mainly used to terminate 00132 * the 00133 */ 00134 void terminateAsyncRead(); 00135 00136 #endif /* ASYNC_ADC_H__ */
Generated on Wed Jul 13 2022 19:36:48 by
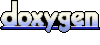