
Roomba robot class
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 #include "Roomba.h" 00003 00004 DigitalOut myled(LED1); 00005 Roomba roomba(p9, p10); 00006 00007 int main() { 00008 00009 // Examples of sending commands. Note that when 00010 // teh function returns, if it was successful 00011 // it returns the command code. Otherwise it 00012 // will return Roomba::NotIdle which means it 00013 // couldn't send the command because the serial 00014 // port was busy reading sensor data. 00015 int result = roomba.command(Roomba::CmdPower); 00016 if (result != Roomba::CmdPower) { 00017 // Failed to send command because the 00018 // serial port was busy getting sensor data 00019 } 00020 00021 // A sure fire way to make the command happen. 00022 while (roomba.command(Roomba::CmdPower) != Roomba::CmdPower); 00023 00024 // Some commands have additional bytes, heres an example 00025 char leds[3]; 00026 leds[0] = 0x01; // First byte sent 00027 leds[1] = 0x05; 00028 leds[2] = 0x76; // Last byte sent 00029 roomba.command(Roomba::CmdLeds, 3, leds); 00030 00031 // Sensors are read automartically. Here's how we 00032 // get the wall sensor and voltage values. 00033 uint8_t wall = roomba.sensor(Roomba::wall); 00034 uint16_t voltage = roomba.sensor(Roomba::voltage); 00035 00036 // Note, when reading sensor data make sure your variable 00037 // is of the right type, either uint8_t for single byte 00038 // and uint16_t for double byte variables. 00039 00040 while(1) { 00041 myled = 1; 00042 wait(0.2); 00043 myled = 0; 00044 wait(0.2); 00045 } 00046 }
Generated on Fri Jul 15 2022 04:40:23 by
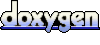