
Roomba robot class
Embed:
(wiki syntax)
Show/hide line numbers
Roomba.h
00001 /* 00002 Copyright (c) 2010 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 00022 @file Roomba.h 00023 @purpose http://mbed.org/forum/mbed/topic/1476 00024 @version 0.1 (untested) 00025 @date Dec 2010 00026 @author Andy Kirkham 00027 @see http://mbed.org/forum/mbed/topic/1476 00028 @require http://mbed.org/cookbook/MODSERIAL 00029 */ 00030 00031 #ifndef ROOMBA_H 00032 #define ROOMBA_H 00033 00034 #include "mbed.h" 00035 #include "MODSERIAL.h" 00036 00037 /** 00038 * @brief Class efinition of a Roomba robot. 00039 * @author Andy Kirkham 00040 * @see http://mbed.org/forum/mbed/topic/1476 00041 * @see http://mbed.org/cookbook/MODSERIAL 00042 * 00043 * <b>Roomba</b> manages communications with teh robot. 00044 * It exports a simple API to send commands or read 00045 * sensor data. 00046 * 00047 * This module requires MODSERIAL 00048 */ 00049 class Roomba { 00050 public: 00051 00052 // States (and commands) 00053 enum RoombaState { 00054 Idle = 0 00055 , GetSensors 00056 , CmdStart = 128 00057 , CmdBaud 00058 , CmdControl 00059 , CmdSafe 00060 , CmdFull 00061 , CmdPower 00062 , CmdSpot 00063 , CmdClean 00064 , CmdMax 00065 , CmdDrive 00066 , CmdMotors 00067 , CmdLeds 00068 , CmdSong 00069 , CmdPlay 00070 , CmdSensors 00071 , CmdForceSeekingDock 00072 }; 00073 00074 // Calltypes and return types 00075 enum CallType { 00076 NotIdle = -1 00077 , Ok = 0 00078 , Invoke = 1 00079 , SerialCallback 00080 }; 00081 00082 00083 // Indexes into array to select what var 00084 enum Sensor8bit { 00085 bumpsWheeldrops = 0 00086 , wall 00087 , cliffLeft 00088 , cliffFrontLeft 00089 , cliffFrontRight 00090 , cliffRight 00091 , virtualWall 00092 , motorOvercurrents 00093 , dirtDetectorLeft 00094 , dirtDetectorRight 00095 , remoteControlCommand 00096 , buttons 00097 , chargingState 00098 , temperature 00099 , NumOf8bitSensors 00100 }; 00101 00102 // Indexes into array to select what var 00103 enum Sensor16bit { 00104 distance 00105 , angle 00106 , voltage 00107 , charge 00108 , current 00109 , capacity 00110 , NumOf16bitSensors 00111 }; 00112 00113 Roomba(PinName tx, PinName rx) { init(tx, rx); } 00114 ~Roomba() { delete uart; delete tick; } 00115 00116 int command(RoombaState cmd, int opts = 0, char *opt = (char *)NULL, bool block = true); 00117 00118 uint8_t sensor(Sensor8bit idx) { return sensors8bit[idx]; } 00119 uint16_t sensor(Sensor16bit idx) { return sensors16bit[idx]; } 00120 00121 // Interrupt callback functions. 00122 void cbSerial(void) { cmdSensors(SerialCallback); } 00123 void cbTicker(void) { cmdSensors(Invoke); } ; 00124 00125 protected: 00126 int state; 00127 MODSERIAL *uart; 00128 Ticker *tick; 00129 00130 // Internal methods 00131 void init(PinName tx, PinName rx); 00132 int cmdSensors(CallType type); 00133 00134 // Sensor values. Populated with data 00135 // via ticker/serial interrupts. Available 00136 // to the user program via the .sensor() API 00137 // function. 00138 uint8_t sensors8bit[NumOf8bitSensors]; 00139 uint16_t sensors16bit[NumOf16bitSensors]; 00140 }; 00141 00142 #endif
Generated on Fri Jul 15 2022 04:40:23 by
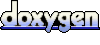