Provides an API software interface to TIMER2 to control upto four stepper motors.
SimpleStepper Class Reference
#include <SimpleSteppers.h>
Inherited by SimpleStepperProfiler.
Public Member Functions | |
SimpleStepper (PinName pulse, SimpleStepperOutput *direction=0) | |
Constructor. | |
SimpleStepper (SimpleStepperController *con, PinName pulse, SimpleStepperOutput *direction) | |
Constructor. | |
void | setPulseSense (int i=1) |
setPulseSense | |
void | setDirectionSense (int i=1) |
setDirectionSense | |
int | setPulseLen (int i=0) |
setPulseLen | |
void | setSpeed (int steps_per_second=0, uint32_t raw=0) |
setSpeed | |
void | setSpeed (double steps_per_second=0, uint32_t raw=0) |
setSpeed | |
int | getSpeed (void) |
getSpeed | |
void | setInterval (int i=0, uint32_t raw=0) |
setInterval | |
int64_t | getPosition (void) |
getPosition | |
void | setWrapPos (int64_t i=0) |
setWrapPos | |
void | setWrapNeg (int64_t i=0) |
setWrapNeg | |
void | setMaintainPositionData (bool b) |
setMaintainPositionData |
Detailed Description
SimpleStepper is a class designed to handle the pulse and direction signals for a stepper motor. A simple API is exposed to allow the setting of the pulse width and the number of pulses per second.
Note, SimpleStepper uses the SimpleStepperController class to manage the TIMER2. SimpleStepperController and SimpleStepper take total control of the LPC17xx's TIMER2. Your application or other library that your application uses, should notuse TIMER2.
This library is a software interface to the TIMER2 MATCH system. It does not provide position and/or acceleration, PID control, etc, of the motors. Your application or other library should provide the control function needed to position/run your stepper motor(s).
#include "mbed.h" #include "SimpleSteppers.h" SimpleStepperOutput led1(LED1); // SimpleStepperOutput is basically the same as // Mbed's DigitalOut class. However, it's more // portable to other platforms without having // to also port the entire Mbed library. SimpleStepperOutput sdir0(p17); SimpleStepperOutput sdir1(p18); SimpleStepperOutput sdir2(p19); SimpleStepperOutput sdir3(p20); // Create four steppers. // Stepper0 has the pulse output on p8 and dir on p17 SimpleStepper stepper0(p8, &sdir0); // Stepper1 has the pulse output on p7 and dir on p18 SimpleStepper stepper1(p7, &sdir1); // Stepper2 has the pulse output on p7 and dir on p19 SimpleStepper stepper2(p6, &sdir2); // Stepper3 has the pulse output on p7 and dir on p20 SimpleStepper stepper3(p5, &sdir3); int main() { // We do not need to maintain the stepper position // for this simple example. This reduces the amount // of work the ISR has to do. stepper0.setMaintainPositionData(false); stepper1.setMaintainPositionData(false); stepper2.setMaintainPositionData(false); stepper3.setMaintainPositionData(false); // Set all steppers to top speed of 5000 pulses/second. stepper0.setSpeed(5000); stepper1.setSpeed(5000); stepper2.setSpeed(5000); stepper3.setSpeed(5000); while(1) { led1 = !led1; wait(0.2); } }
- See also:
- example1.h
Definition at line 109 of file SimpleSteppers.h.
Constructor & Destructor Documentation
SimpleStepper | ( | PinName | pulse, |
SimpleStepperOutput * | direction = 0 |
||
) |
Constructor.
PinName pulse can be p5, p6, p7 or p8 SimpleStepperOutput *direction is a pointer to an output.
- Parameters:
-
PinName The output for the pulse output. SimpleStepperOutput *direction The output pin for direction control
Definition at line 35 of file SimpleSteppers.cpp.
SimpleStepper | ( | SimpleStepperController * | con, |
PinName | pulse, | ||
SimpleStepperOutput * | direction | ||
) |
Constructor.
PinName pulse can be p5, p6, p7 or p8 SimpleStepperOutput *direction is a pointer to an output.
- Parameters:
-
SimpleStepperController *con A pointer to a base controller. PinName The output for the pulse output. SimpleStepperOutput *direction The output pin for direction control
Definition at line 43 of file SimpleSteppers.cpp.
Member Function Documentation
int64_t getPosition | ( | void | ) |
getPosition
Get the current position (the pulse counter).
- Returns:
- int64_t The current position as maintained by the interrupts.
Definition at line 278 of file SimpleSteppers.h.
int getSpeed | ( | void | ) |
getSpeed
Get the demanded motor speed in pulses per second. Zero stops all motor pulses.
- Returns:
- int steps_per_second Number of pulses per second demanded.
Definition at line 263 of file SimpleSteppers.h.
void setDirectionSense | ( | int | i = 1 ) |
setDirectionSense
Set's the logic value that direction forward assumes. Default is 1.
1 means that the direction output is 1 for forward and 0 for reverse. 0 means that the direction output is 0 for forward and 1 for reverse.
Additionally, for position:- 1 means forward pulses are counted upwards, reverse means counted downwards. 0 means forward pulses are counted downwards, reverse means counted upwards.
- Parameters:
-
int What is the logic sense for the direction output.
Definition at line 199 of file SimpleSteppers.h.
void setInterval | ( | int | i = 0 , |
uint32_t | raw = 0 |
||
) |
setInterval
Set the motor speed pulse interval. Zero stops all motor pulses.
- Parameters:
-
int interval
Definition at line 124 of file SimpleSteppers.cpp.
void setMaintainPositionData | ( | bool | b ) |
setMaintainPositionData
Setting this false removes the maintainence of positional data. This allows the interrupt service routine to not perform these steps (if not required) thus making the ISR run slightly fatser. You might want to do this if positional data isn't required.
Definition at line 305 of file SimpleSteppers.h.
int setPulseLen | ( | int | i = 0 ) |
setPulseLen
Used to set the pulse length. Default pulse length is 50us. If no arg supplied returns the current pulse length. Note, the length is specified as 10us increments. The default is 5 which is 50us
- Parameters:
-
int pulse length
- Returns:
- int the value of the pulse length.
Definition at line 210 of file SimpleSteppers.h.
void setPulseSense | ( | int | i = 1 ) |
setPulseSense
Set's the logic value that pulse asserted assumes. Default is 1.
1 means pulse goes from 0 to 1 and remains 1 for pulseLen time then goes to 0. 0 means pulse goes from 1 to 0 and remains 0 for pulseLen time then goes to 1.
- Parameters:
-
int What is the logic sense for the pulse output.
Definition at line 184 of file SimpleSteppers.h.
void setSpeed | ( | double | steps_per_second = 0 , |
uint32_t | raw = 0 |
||
) |
setSpeed
Set the motor speed in pulses per second. Zero stops all motor pulses.
- Parameters:
-
double steps_per_second Number of pulses per second required.
Definition at line 157 of file SimpleSteppers.cpp.
void setSpeed | ( | int | steps_per_second = 0 , |
uint32_t | raw = 0 |
||
) |
setSpeed
Set the motor speed in pulses per second. Zero stops all motor pulses.
With the default pulseLen of 50us (5 timer "ticks") the maximum speed that can be set is 10000 pulses per second (pps) which will produce a square wave with 50% duty cycle. Pushing it beyond will give a square wave a duty cycle shifts so that the off time between pulses becomes shorter than the on pulse length.
The pulseLen can be adjusted using setPulseLen() down even more to eek out some extra bandwidth. However, you really should be checking both the datasheet for your stepper motor amplifier and stepper motor. Running a stepper motor at such high speed may have electrical and mechanical issues.
The arg raw is used when you want to "sync" channels. Suppose for example you have the following code to set the speed to two front wheels:-
stepper0.setSpeed(100); stepper1.setSpeed(100);
The problem here is they will be a few "clock ticks" out from each other as the TIMER2 TC increments between the two calls. If you wanted to make two motors sync together you can pass in the raw value of TIMER2 TC to make both functions calculate the same stepp values and so sync together:-
uint32_t i = LPC_TIM2->TC; // Capture TC.
stepper0.setSpeed(100, i);
stepper1.setSpeed(100, i);
The above code forces setSpeed() to calculate both axis in sync with respect to a common TC value.
- Parameters:
-
int steps_per_second Number of pulses per second required.
Definition at line 194 of file SimpleSteppers.cpp.
void setWrapNeg | ( | int64_t | i = 0 ) |
setWrapNeg
Set the value that we should wrap the pulse position counter. Zero (default) means no wrap occurs.
- Parameters:
-
int64_t The value to wrap to POS at.
Definition at line 296 of file SimpleSteppers.h.
void setWrapPos | ( | int64_t | i = 0 ) |
setWrapPos
Set the value that we should wrap the pulse position counter. Zero (default) means no wrap occurs.
- Parameters:
-
int64_t The value to wrap to NEG at.
Definition at line 287 of file SimpleSteppers.h.
Generated on Thu Jul 14 2022 17:06:02 by
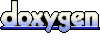