
CUED IIA Project
Dependencies: BLE_API mbed nRF51822
Fork of BLE_GATT_Example by
sensors.h
00001 #include "mbed.h" 00002 00003 #ifndef SENSORS_H 00004 #define SENSORS_H 00005 00006 00007 // Register names, can look up in the datasheet 00008 00009 #define SMPRT_DIV 0x19 // 00010 #define CONFIG 0x1A // 00011 #define GYRO_CONFIG 0x1B // 00012 #define ACCEL_CONFIG 0x1C // 00013 #define FIFO_EN 0x23 // 00014 #define I2C_MST_CTRL 0x24 // 00015 #define INT_PIN_CFG 0x37 // 00016 #define INT_ENABLE 0x38 // 00017 00018 #define ACCX158 0x3B // 00019 #define ACCX70 0x3C // 00020 #define ACCY158 0x3D // 00021 #define ACCY70 0x3E // 00022 #define ACCZ158 0x3F // 00023 #define ACCZ70 0x40 // 00024 #define TEMP158 0x41 // 00025 #define TEMP70 0x42 // Temperature in C = ((signed int16_t) value)/340 + 36.53 00026 #define GYROX158 0x43 // 00027 #define GYROX70 0x44 // 00028 #define GYROY158 0x45 // 00029 #define GYROY70 0x46 // 00030 #define GYROZ158 0x47 // 00031 #define GYROZ70 0x48 // 00032 00033 #define USER_CTRL 0x6A // 00034 #define PWR_MGMT_1 0x6B // 00035 #define PWR_MGMT_2 0x6C // 00036 #define WHO_AM_I 0x75 // Check device number to see if it works 00037 00038 const uint8_t ACC_LEFT = 0x68; // 0b01101000 00039 const uint8_t ACC_RIGHT = 0x69; // 0b01101001 00040 00041 00042 00043 00044 typedef struct { 00045 00046 uint16_t flex[12]; 00047 // Order of the flex sensors: 00048 // 0 - right thumb, 4 - right pinky, 5 - R elbow; 00049 // 6 - left thumb, 10 left pinky, 11 - L elbow; 00050 uint16_t acc[2][7]; 00051 // 7th value is the temperature value 00052 uint16_t temp; 00053 00054 00055 } Datapacket; 00056 00057 typedef struct { 00058 00059 uint8_t pixels[8]; 00060 00061 } Screen; 00062 00063 00064 void readacc(Datapacket data); 00065 00066 void setupacc(uint8_t ADDRESS); 00067 00068 void WriteBytes(uint8_t addr, uint8_t *pbuf, uint16_t length, uint8_t DEV_ADDR); 00069 00070 void WriteByte(uint8_t addr, uint8_t buf, uint8_t DEV_ADDR); 00071 00072 void ReadBytes(uint8_t addr, uint8_t *pbuf, uint16_t length, uint8_t DEV_ADDR); 00073 00074 void readflexs(Datapacket *data); 00075 00076 void readacc(Datapacket *data, uint8_t accadr); 00077 00078 void setupI2C(void); 00079 00080 00081 00082 00083 00084 00085 00086 #endif
Generated on Wed Jul 13 2022 18:04:13 by
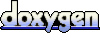