
CUED IIA Project
Dependencies: BLE_API mbed nRF51822
Fork of BLE_GATT_Example by
gloves.cpp
00001 #include "mbed.h" 00002 #include "ble/BLE.h" 00003 #include "sensors.h" 00004 00005 00006 00007 DigitalOut led(LED1); 00008 uint16_t customServiceUUID = 0xABCD; 00009 uint16_t readCharUUID = 0xABCE; 00010 uint16_t writeCharUUID = 0xABCF; 00011 00012 static volatile bool triggerSensorPolling = false; 00013 00014 //Serial pc(USBTX, USBRX); 00015 // pc.baud(9600); 00016 // pc.printf("IIC Demo Start \r\n"); 00017 // For debugging into PC terminal 00018 00019 00020 00021 const static char DEVICE_NAME[] = "WorkGloves"; // change this 00022 static const uint16_t uuid16_list[] = {0xFFFF}; //Custom UUID, FFFF is reserved for development 00023 00024 /* Set Up custom Characteristics */ 00025 static uint16_t readValue[26] = {0x55, 0x33}; 00026 ReadOnlyArrayGattCharacteristic<uint16_t, sizeof(readValue)> readChar(readCharUUID, readValue); 00027 00028 static uint8_t writeValue[8] = {0x00}; 00029 WriteOnlyArrayGattCharacteristic<uint8_t, sizeof(writeValue)> writeChar(writeCharUUID, writeValue); 00030 00031 00032 /* Set up custom service */ 00033 GattCharacteristic *characteristics[] = {&readChar, &writeChar}; 00034 GattService customService(customServiceUUID, characteristics, sizeof(characteristics) / sizeof(GattCharacteristic *)); 00035 00036 void datapackettoarray(Datapacket todump, uint16_t *dest) 00037 { //Need a uint16_t array[26] input for correct functioning 00038 uint8_t j,k; 00039 00040 for (j=0; j<12; j++) 00041 { 00042 *dest=todump.flex[j]; 00043 dest++; 00044 } 00045 for(k=0; k<7; k++) 00046 { 00047 *dest=todump.acc[0][k]; 00048 dest++; 00049 } 00050 00051 for(k=0; k<7; j++, k++) 00052 { 00053 *dest=todump.acc[1][k]; 00054 dest++; 00055 } 00056 00057 00058 00059 } 00060 00061 00062 /* 00063 * Restart advertising when phone app disconnects 00064 */ 00065 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *) 00066 { 00067 BLE::Instance(BLE::DEFAULT_INSTANCE).gap().startAdvertising(); 00068 } 00069 00070 void periodicCallback(void) 00071 { 00072 led = !led; /* Do blinky on LED1 while we're waiting for BLE events */ 00073 00074 /* Note that the periodicCallback() executes in interrupt context, so it is safer to do 00075 * heavy-weight sensor polling from the main thread. */ 00076 triggerSensorPolling = true; 00077 } 00078 00079 /* 00080 * Handle writes to writeCharacteristic for screen data from phone 00081 */ 00082 void writeCharCallback(const GattWriteCallbackParams *params) 00083 { 00084 /* Check to see what characteristic was written, by handle */ 00085 if(params->handle == writeChar.getValueHandle()) { 00086 /* Update the readChar with the value of writeChar */ 00087 // BLE::Instance(BLE::DEFAULT_INSTANCE).gattServer().write(readChar.getValueHandle(), params->data, params->len); 00088 } 00089 } 00090 /* 00091 * Initialization callback 00092 */ 00093 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00094 { 00095 BLE &ble = params->ble; 00096 ble_error_t error = params->error; 00097 00098 if (error != BLE_ERROR_NONE) { 00099 return; 00100 } 00101 00102 ble.gap().onDisconnection(disconnectionCallback); 00103 ble.gattServer().onDataWritten(writeCharCallback); // TODO: update to flush screen !!! 00104 00105 /* Setup advertising */ 00106 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); // BLE only, no classic BT 00107 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); // advertising type 00108 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); // add name 00109 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); // UUID's broadcast in advertising packet 00110 ble.gap().setAdvertisingInterval(250); // 250ms. 00111 00112 /* Add our custom service */ 00113 ble.addService(customService); 00114 00115 /* Start advertising */ 00116 ble.gap().startAdvertising(); 00117 } 00118 00119 /* 00120 * Main loop 00121 */ 00122 int main(void) 00123 { 00124 /* initialize stuff */ 00125 // printf("\n\r********* Starting Main Loop *********\n\r"); 00126 Datapacket readings; 00127 setupI2C(); 00128 setupacc(ACC_LEFT); 00129 setupacc(ACC_RIGHT); 00130 led = 1; 00131 Ticker ticker; 00132 ticker.attach(periodicCallback, 0.2); // blink LED every 0.2 second 00133 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00134 ble.init(bleInitComplete); 00135 uint16_t array[26]; 00136 // uint8_t data[52]={0,0,0,0,0,0,0,0,0,0,0,0,0}; 00137 /* SpinWait for initialization to complete. This is necessary because the 00138 * BLE object is used in the main loop below. */ 00139 while (ble.hasInitialized() == false) { /* spin loop */ } 00140 // uint16_t data[24] = {0x11, 0x22}; 00141 /* Infinite loop waiting for BLE interrupt events */ 00142 while (1) { 00143 // check for trigger// from periodicCallback() 00144 if (triggerSensorPolling && ble.getGapState().connected) { 00145 triggerSensorPolling = false; 00146 // data[0] = data[0]+1;// 00147 readflexs(&readings); 00148 readacc(&readings, ACC_LEFT ); 00149 readacc(&readings, ACC_RIGHT ); 00150 datapackettoarray(readings, array); 00151 BLE::Instance(BLE::DEFAULT_INSTANCE).gattServer().write(readChar.getValueHandle(), (uint8_t*)array, 52 ); 00152 // BLE::Instance(BLE::DEFAULT_INSTANCE).gattServer().write(readChar.getValueHandle(), (uint8_t*)data, 52 ); 00153 00154 00155 } 00156 else { 00157 ble.waitForEvent(); // low power wait for event 00158 } 00159 } 00160 }
Generated on Wed Jul 13 2022 18:04:13 by
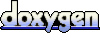