Library that implements the CellularInterface using PPP and LWIP on the mbed MCU. May be used on the C027 and C030 (non-N2xx flavour) boards from mbed 5.5 onwards.
Dependents: example-ublox-cellular-interface HelloMQTT example-ublox-cellular-interface_r410M example-ublox-mbed-client
main.cpp
00001 #include "UbloxPPPCellularInterface.h" 00002 #include "mbed.h" 00003 #include "Callback.h" 00004 #include "greentea-client/test_env.h" 00005 #include "unity.h" 00006 #include "utest.h" 00007 #include "UDPSocket.h" 00008 #ifdef FEATURE_COMMON_PAL 00009 #include "mbed_trace.h" 00010 #define TRACE_GROUP "TEST" 00011 #else 00012 #define tr_debug(format, ...) debug(format "\n", ## __VA_ARGS__) 00013 #define tr_info(format, ...) debug(format "\n", ## __VA_ARGS__) 00014 #define tr_warn(format, ...) debug(format "\n", ## __VA_ARGS__) 00015 #define tr_error(format, ...) debug(format "\n", ## __VA_ARGS__) 00016 #endif 00017 00018 using namespace utest::v1; 00019 00020 #if NSAPI_PPP_AVAILABLE 00021 00022 // IMPORTANT!!! if you make a change to the tests here you should 00023 // check whether the same change should be made to the tests under 00024 // the AT interface. 00025 00026 // NOTE: these test are only as reliable as UDP across the internet 00027 // over a radio link. The tests expect an NTP server to respond 00028 // to UDP packets and, if configured, an echo server to respond 00029 // to UDP packets. This simply may not happen. Please be patient. 00030 00031 // ---------------------------------------------------------------- 00032 // COMPILE-TIME MACROS 00033 // ---------------------------------------------------------------- 00034 00035 // These macros can be overridden with an mbed_app.json file and 00036 // contents of the following form: 00037 // 00038 //{ 00039 // "config": { 00040 // "default-pin": { 00041 // "value": "\"1234\"" 00042 // } 00043 //} 00044 // 00045 // See the template_mbed_app.txt in this directory for a fuller example. 00046 00047 // Whether debug trace is on 00048 #ifndef MBED_CONF_APP_DEBUG_ON 00049 # define MBED_CONF_APP_DEBUG_ON false 00050 #endif 00051 00052 // Run the SIM change tests, which require the DEFAULT_PIN 00053 // above to be correct for the board on which the test 00054 // is being run (and the SIM PIN to be disabled before tests run). 00055 #ifndef MBED_CONF_APP_RUN_SIM_PIN_CHANGE_TESTS 00056 # define MBED_CONF_APP_RUN_SIM_PIN_CHANGE_TESTS 0 00057 #endif 00058 00059 #if MBED_CONF_APP_RUN_SIM_PIN_CHANGE_TESTS 00060 # ifndef MBED_CONF_APP_DEFAULT_PIN 00061 # error "MBED_CONF_APP_DEFAULT_PIN must be defined to run the SIM tests" 00062 # endif 00063 # ifndef MBED_CONF_APP_ALT_PIN 00064 # error "MBED_CONF_APP_ALT_PIN must be defined to run the SIM tests" 00065 # endif 00066 # ifndef MBED_CONF_APP_INCORRECT_PIN 00067 # error "MBED_CONF_APP_INCORRECT_PIN must be defined to run the SIM tests" 00068 # endif 00069 #endif 00070 00071 // The credentials of the SIM in the board. 00072 #ifndef MBED_CONF_APP_DEFAULT_PIN 00073 // Note: if PIN is enabled on your SIM, or you wish to run the SIM PIN change 00074 // tests, you must define the PIN for your SIM (see note above on using 00075 // mbed_app.json to do so). 00076 # define MBED_CONF_APP_DEFAULT_PIN "0000" 00077 #endif 00078 #ifndef MBED_CONF_APP_APN 00079 # define MBED_CONF_APP_APN NULL 00080 #endif 00081 #ifndef MBED_CONF_APP_USERNAME 00082 # define MBED_CONF_APP_USERNAME NULL 00083 #endif 00084 #ifndef MBED_CONF_APP_PASSWORD 00085 # define MBED_CONF_APP_PASSWORD NULL 00086 #endif 00087 00088 // Alternate PIN to use during pin change testing 00089 #ifndef MBED_CONF_APP_ALT_PIN 00090 # define MBED_CONF_APP_ALT_PIN "9876" 00091 #endif 00092 00093 // A PIN that is definitely incorrect 00094 #ifndef MBED_CONF_APP_INCORRECT_PIN 00095 # define MBED_CONF_APP_INCORRECT_PIN "1530" 00096 #endif 00097 00098 // Servers and ports 00099 #ifdef MBED_CONF_APP_ECHO_SERVER 00100 # ifndef MBED_CONF_APP_ECHO_UDP_PORT 00101 # error "MBED_CONF_APP_ECHO_UDP_PORT (the port on which your echo server echoes UDP packets) must be defined" 00102 # endif 00103 # ifndef MBED_CONF_APP_ECHO_TCP_PORT 00104 # error "MBED_CONF_APP_ECHO_TCP_PORT (the port on which your echo server echoes TCP packets) must be defined" 00105 # endif 00106 #endif 00107 00108 #ifndef MBED_CONF_APP_NTP_SERVER 00109 # define MBED_CONF_APP_NTP_SERVER "2.pool.ntp.org" 00110 #else 00111 # ifndef MBED_CONF_APP_NTP_PORT 00112 # error "MBED_CONF_APP_NTP_PORT must be defined if MBED_CONF_APP_NTP_SERVER is defined" 00113 # endif 00114 #endif 00115 #ifndef MBED_CONF_APP_NTP_PORT 00116 # define MBED_CONF_APP_NTP_PORT 123 00117 #endif 00118 00119 #ifndef MBED_CONF_APP_LOCAL_PORT 00120 # define MBED_CONF_APP_LOCAL_PORT 15 00121 #endif 00122 00123 // UDP packet size limit for testing 00124 #ifndef MBED_CONF_APP_UDP_MAX_PACKET_SIZE 00125 # define MBED_CONF_APP_UDP_MAX_PACKET_SIZE 508 00126 #endif 00127 00128 // TCP packet size limit for testing 00129 #ifndef MBED_CONF_APP_MBED_CONF_APP_TCP_MAX_PACKET_SIZE 00130 # define MBED_CONF_APP_TCP_MAX_PACKET_SIZE 1500 00131 #endif 00132 00133 // The number of retries for UDP exchanges 00134 #define NUM_UDP_RETRIES 5 00135 00136 // How long to wait for stuff to travel in the async tests 00137 #define ASYNC_TEST_WAIT_TIME 10000 00138 00139 // ---------------------------------------------------------------- 00140 // PRIVATE VARIABLES 00141 // ---------------------------------------------------------------- 00142 00143 #ifdef FEATURE_COMMON_PAL 00144 // Lock for debug prints 00145 static Mutex mtx; 00146 #endif 00147 00148 // An instance of the cellular interface 00149 static UbloxPPPCellularInterface *interface = new UbloxPPPCellularInterface(MDMTXD, 00150 MDMRXD, 00151 MBED_CONF_UBLOX_CELL_BAUD_RATE, 00152 MBED_CONF_APP_DEBUG_ON); 00153 00154 // Connection flag 00155 static bool connection_has_gone_down = false; 00156 00157 static const char send_data[] = "_____0000:0123456789012345678901234567890123456789" 00158 "01234567890123456789012345678901234567890123456789" 00159 "_____0100:0123456789012345678901234567890123456789" 00160 "01234567890123456789012345678901234567890123456789" 00161 "_____0200:0123456789012345678901234567890123456789" 00162 "01234567890123456789012345678901234567890123456789" 00163 "_____0300:0123456789012345678901234567890123456789" 00164 "01234567890123456789012345678901234567890123456789" 00165 "_____0400:0123456789012345678901234567890123456789" 00166 "01234567890123456789012345678901234567890123456789" 00167 "_____0500:0123456789012345678901234567890123456789" 00168 "01234567890123456789012345678901234567890123456789" 00169 "_____0600:0123456789012345678901234567890123456789" 00170 "01234567890123456789012345678901234567890123456789" 00171 "_____0700:0123456789012345678901234567890123456789" 00172 "01234567890123456789012345678901234567890123456789" 00173 "_____0800:0123456789012345678901234567890123456789" 00174 "01234567890123456789012345678901234567890123456789" 00175 "_____0900:0123456789012345678901234567890123456789" 00176 "01234567890123456789012345678901234567890123456789" 00177 "_____1000:0123456789012345678901234567890123456789" 00178 "01234567890123456789012345678901234567890123456789" 00179 "_____1100:0123456789012345678901234567890123456789" 00180 "01234567890123456789012345678901234567890123456789" 00181 "_____1200:0123456789012345678901234567890123456789" 00182 "01234567890123456789012345678901234567890123456789" 00183 "_____1300:0123456789012345678901234567890123456789" 00184 "01234567890123456789012345678901234567890123456789" 00185 "_____1400:0123456789012345678901234567890123456789" 00186 "01234567890123456789012345678901234567890123456789" 00187 "_____1500:0123456789012345678901234567890123456789" 00188 "01234567890123456789012345678901234567890123456789" 00189 "_____1600:0123456789012345678901234567890123456789" 00190 "01234567890123456789012345678901234567890123456789" 00191 "_____1700:0123456789012345678901234567890123456789" 00192 "01234567890123456789012345678901234567890123456789" 00193 "_____1800:0123456789012345678901234567890123456789" 00194 "01234567890123456789012345678901234567890123456789" 00195 "_____1900:0123456789012345678901234567890123456789" 00196 "01234567890123456789012345678901234567890123456789" 00197 "_____2000:0123456789012345678901234567890123456789" 00198 "01234567890123456789012345678901234567890123456789"; 00199 00200 // ---------------------------------------------------------------- 00201 // PRIVATE FUNCTIONS 00202 // ---------------------------------------------------------------- 00203 00204 #ifdef FEATURE_COMMON_PAL 00205 // Locks for debug prints 00206 static void lock() 00207 { 00208 mtx.lock(); 00209 } 00210 00211 static void unlock() 00212 { 00213 mtx.unlock(); 00214 } 00215 #endif 00216 00217 // Callback in case the connection goes down 00218 void connection_down_cb(nsapi_event_t event, intptr_t x) 00219 { 00220 if ((nsapi_connection_status_t) x == NSAPI_STATUS_DISCONNECTED) { 00221 connection_has_gone_down = true; 00222 } 00223 } 00224 00225 #ifdef MBED_CONF_APP_ECHO_SERVER 00226 // Make sure that size is greater than 0 and no more than limit, 00227 // useful since, when moduloing a very large number number, 00228 // compilers sometimes screw up and produce a small *negative* 00229 // number. Who knew? For example, GCC decided that 00230 // 492318453 (0x1d582ef5) modulo 508 was -47 (0xffffffd1). 00231 static int fix (int size, int limit) 00232 { 00233 if (size <= 0) { 00234 size = limit / 2; // better than 1 00235 } else if (size > limit) { 00236 size = limit; 00237 } 00238 return size; 00239 } 00240 00241 // Do a UDP socket echo test to a given host of a given packet size 00242 static void do_udp_echo(UDPSocket *sock, SocketAddress *host_address, int size) 00243 { 00244 bool success = false; 00245 void * recv_data = malloc (size); 00246 TEST_ASSERT(recv_data != NULL); 00247 00248 // Retry this a few times, don't want to fail due to a flaky link 00249 for (int x = 0; !success && (x < NUM_UDP_RETRIES); x++) { 00250 tr_debug("Echo testing UDP packet size %d byte(s), try %d.", size, x + 1); 00251 if ((sock->sendto(*host_address, (void*) send_data, size) == size) && 00252 (sock->recvfrom(host_address, recv_data, size) == size)) { 00253 TEST_ASSERT (memcmp(send_data, recv_data, size) == 0); 00254 success = true; 00255 } 00256 } 00257 TEST_ASSERT (success); 00258 TEST_ASSERT(!connection_has_gone_down); 00259 00260 free (recv_data); 00261 } 00262 00263 // Send an entire TCP data buffer until done 00264 static int sendAll(TCPSocket *sock, const char *data, int size) 00265 { 00266 int x; 00267 int count = 0; 00268 Timer timer; 00269 00270 timer.start(); 00271 while ((count < size) && (timer.read_ms() < ASYNC_TEST_WAIT_TIME)) { 00272 x = sock->send(data + count, size - count); 00273 if (x > 0) { 00274 count += x; 00275 tr_debug("%d byte(s) sent, %d left to send.", count, size - count); 00276 } 00277 wait_ms(10); 00278 } 00279 timer.stop(); 00280 00281 return count; 00282 } 00283 00284 // The asynchronous callback 00285 static void async_cb(bool *callback_triggered) 00286 { 00287 00288 TEST_ASSERT (callback_triggered != NULL); 00289 *callback_triggered = true; 00290 } 00291 00292 // Do a TCP echo using the asynchronous interface 00293 static void do_tcp_echo_async(TCPSocket *sock, int size, bool *callback_triggered) 00294 { 00295 void * recv_data = malloc (size); 00296 int recv_size = 0; 00297 int x, y; 00298 Timer timer; 00299 TEST_ASSERT(recv_data != NULL); 00300 00301 *callback_triggered = false; 00302 tr_debug("Echo testing TCP packet size %d byte(s) async.", size); 00303 TEST_ASSERT (sendAll(sock, send_data, size) == size); 00304 // Wait for all the echoed data to arrive 00305 timer.start(); 00306 while ((recv_size < size) && (timer.read_ms() < ASYNC_TEST_WAIT_TIME)) { 00307 if (*callback_triggered) { 00308 *callback_triggered = false; 00309 x = sock->recv((char *) recv_data + recv_size, size); 00310 // IMPORTANT: this is different to the version in the AT DATA tests 00311 // In the AT DATA case we know that the only reason the callback 00312 // will be triggered is if there is received data. In the case 00313 // of calling the LWIP implementation other things can also trigger 00314 // it, so don't rely on there being any bytes to receive. 00315 if (x > 0) { 00316 recv_size += x; 00317 tr_debug("%d byte(s) echoed back so far.", recv_size); 00318 } 00319 } 00320 wait_ms(10); 00321 } 00322 TEST_ASSERT(recv_size == size); 00323 y = memcmp(send_data, recv_data, size); 00324 if (y != 0) { 00325 tr_debug("Sent %d, |%*.*s|", size, size, size, send_data); 00326 tr_debug("Rcvd %d, |%*.*s|", size, size, size, (char *) recv_data); 00327 TEST_ASSERT(false); 00328 } 00329 timer.stop(); 00330 00331 TEST_ASSERT(!connection_has_gone_down); 00332 00333 free (recv_data); 00334 } 00335 #endif 00336 00337 // Get NTP time 00338 static void do_ntp(UbloxPPPCellularInterface *interface) 00339 { 00340 char ntp_values[48] = { 0 }; 00341 time_t timestamp = 0; 00342 struct tm *localTime; 00343 char timeString[25]; 00344 time_t TIME1970 = 2208988800U; 00345 int len; 00346 UDPSocket sock; 00347 SocketAddress ntp_address; 00348 bool comms_done = false; 00349 00350 ntp_values[0] = '\x1b'; 00351 00352 TEST_ASSERT(sock.open(interface) == 0) 00353 00354 TEST_ASSERT(interface->gethostbyname(MBED_CONF_APP_NTP_SERVER, &ntp_address) == 0); 00355 ntp_address.set_port(MBED_CONF_APP_NTP_PORT); 00356 00357 tr_debug("UDP: NIST server %s address: %s on port %d.", MBED_CONF_APP_NTP_SERVER, 00358 ntp_address.get_ip_address(), ntp_address.get_port()); 00359 00360 sock.set_timeout(10000); 00361 00362 // Retry this a few times, don't want to fail due to a flaky link 00363 for (unsigned int x = 0; !comms_done && (x < NUM_UDP_RETRIES); x++) { 00364 sock.sendto(ntp_address, (void*) ntp_values, sizeof(ntp_values)); 00365 len = sock.recvfrom(&ntp_address, (void*) ntp_values, sizeof(ntp_values)); 00366 if (len > 0) { 00367 comms_done = true; 00368 } 00369 } 00370 TEST_ASSERT (comms_done); 00371 00372 sock.close(); 00373 00374 tr_debug("UDP: %d byte(s) returned by NTP server.", len); 00375 if (len >= 43) { 00376 timestamp |= ((int) *(ntp_values + 40)) << 24; 00377 timestamp |= ((int) *(ntp_values + 41)) << 16; 00378 timestamp |= ((int) *(ntp_values + 42)) << 8; 00379 timestamp |= ((int) *(ntp_values + 43)); 00380 timestamp -= TIME1970; 00381 srand (timestamp); 00382 tr_debug("srand() called"); 00383 localTime = localtime(×tamp); 00384 if (localTime) { 00385 if (strftime(timeString, sizeof(timeString), "%a %b %d %H:%M:%S %Y", localTime) > 0) { 00386 printf("NTP timestamp is %s.\n", timeString); 00387 } 00388 } 00389 } 00390 } 00391 00392 // Use a connection, checking that it is good 00393 static void use_connection(UbloxPPPCellularInterface *interface) 00394 { 00395 const char * ip_address = interface->get_ip_address(); 00396 const char * net_mask = interface->get_netmask(); 00397 const char * gateway = interface->get_gateway(); 00398 00399 TEST_ASSERT(interface->is_connected()); 00400 00401 TEST_ASSERT(ip_address != NULL); 00402 tr_debug ("IP address %s.", ip_address); 00403 TEST_ASSERT(net_mask != NULL); 00404 tr_debug ("Net mask %s.", net_mask); 00405 TEST_ASSERT(gateway != NULL); 00406 tr_debug ("Gateway %s.", gateway); 00407 00408 do_ntp(interface); 00409 TEST_ASSERT(!connection_has_gone_down); 00410 } 00411 00412 // Drop a connection and check that it has dropped 00413 static void drop_connection(UbloxPPPCellularInterface *interface) 00414 { 00415 TEST_ASSERT(interface->disconnect() == 0); 00416 TEST_ASSERT(connection_has_gone_down); 00417 connection_has_gone_down = false; 00418 TEST_ASSERT(!interface->is_connected()); 00419 } 00420 00421 // ---------------------------------------------------------------- 00422 // TESTS 00423 // ---------------------------------------------------------------- 00424 00425 // Call srand() using the NTP server 00426 void test_set_randomise() { 00427 UDPSocket sock; 00428 SocketAddress host_address; 00429 00430 TEST_ASSERT(interface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00431 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00432 do_ntp(interface); 00433 TEST_ASSERT(!connection_has_gone_down); 00434 drop_connection(interface); 00435 } 00436 00437 #ifdef MBED_CONF_APP_ECHO_SERVER 00438 // Test UDP data exchange 00439 void test_udp_echo() { 00440 UDPSocket sock; 00441 SocketAddress host_address; 00442 int x; 00443 int size; 00444 00445 interface->deinit(); 00446 TEST_ASSERT(interface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00447 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00448 00449 TEST_ASSERT(interface->gethostbyname(MBED_CONF_APP_ECHO_SERVER, &host_address) == 0); 00450 host_address.set_port(MBED_CONF_APP_ECHO_UDP_PORT); 00451 00452 tr_debug("UDP: Server %s address: %s on port %d.", MBED_CONF_APP_ECHO_SERVER, 00453 host_address.get_ip_address(), host_address.get_port()); 00454 00455 TEST_ASSERT(sock.open(interface) == 0) 00456 00457 sock.set_timeout(10000); 00458 00459 // Test min, max, and some random sizes in-between 00460 do_udp_echo(&sock, &host_address, 1); 00461 do_udp_echo(&sock, &host_address, MBED_CONF_APP_UDP_MAX_PACKET_SIZE); 00462 for (x = 0; x < 10; x++) { 00463 size = (rand() % MBED_CONF_APP_UDP_MAX_PACKET_SIZE) + 1; 00464 size = fix(size, MBED_CONF_APP_UDP_MAX_PACKET_SIZE); 00465 do_udp_echo(&sock, &host_address, size); 00466 } 00467 00468 sock.close(); 00469 00470 drop_connection(interface); 00471 00472 tr_debug("%d UDP packets of size up to %d byte(s) echoed successfully.", x, 00473 MBED_CONF_APP_UDP_MAX_PACKET_SIZE); 00474 } 00475 00476 // Test TCP data exchange via the asynchronous sigio() mechanism 00477 void test_tcp_echo_async() { 00478 TCPSocket sock; 00479 SocketAddress host_address; 00480 bool callback_triggered = false; 00481 int x; 00482 int size; 00483 00484 interface->deinit(); 00485 TEST_ASSERT(interface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00486 00487 TEST_ASSERT(interface->gethostbyname(MBED_CONF_APP_ECHO_SERVER, &host_address) == 0); 00488 host_address.set_port(MBED_CONF_APP_ECHO_TCP_PORT); 00489 00490 tr_debug("TCP: Server %s address: %s on port %d.", MBED_CONF_APP_ECHO_SERVER, 00491 host_address.get_ip_address(), host_address.get_port()); 00492 00493 TEST_ASSERT(sock.open(interface) == 0) 00494 00495 // Set up the async callback and set the timeout to zero 00496 sock.sigio(callback(async_cb, &callback_triggered)); 00497 sock.set_timeout(0); 00498 00499 TEST_ASSERT(sock.connect(host_address) == 0); 00500 // Test min, max, and some random sizes in-between 00501 do_tcp_echo_async(&sock, 1, &callback_triggered); 00502 do_tcp_echo_async(&sock, MBED_CONF_APP_TCP_MAX_PACKET_SIZE, &callback_triggered); 00503 for (x = 0; x < 10; x++) { 00504 size = (rand() % MBED_CONF_APP_TCP_MAX_PACKET_SIZE) + 1; 00505 size = fix(size, MBED_CONF_APP_TCP_MAX_PACKET_SIZE); 00506 do_tcp_echo_async(&sock, size, &callback_triggered); 00507 } 00508 00509 sock.close(); 00510 00511 drop_connection(interface); 00512 00513 tr_debug("%d TCP packets of size up to %d byte(s) echoed asynchronously and successfully.", 00514 x, MBED_CONF_APP_TCP_MAX_PACKET_SIZE); 00515 } 00516 #endif 00517 00518 // Connect with credentials included in the connect request 00519 void test_connect_credentials() { 00520 00521 interface->deinit(); 00522 TEST_ASSERT(interface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00523 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00524 use_connection(interface); 00525 drop_connection(interface); 00526 } 00527 00528 // Test with credentials preset 00529 void test_connect_preset_credentials() { 00530 00531 interface->deinit(); 00532 TEST_ASSERT(interface->init(MBED_CONF_APP_DEFAULT_PIN)); 00533 interface->set_credentials(MBED_CONF_APP_APN, MBED_CONF_APP_USERNAME, 00534 MBED_CONF_APP_PASSWORD); 00535 TEST_ASSERT(interface->connect(MBED_CONF_APP_DEFAULT_PIN) == 0); 00536 use_connection(interface); 00537 drop_connection(interface); 00538 } 00539 00540 // Test adding and using a SIM pin, then removing it, using the pending 00541 // mechanism where the change doesn't occur until connect() is called 00542 void test_check_sim_pin_pending() { 00543 00544 interface->deinit(); 00545 00546 // Enable PIN checking (which will use the current PIN) 00547 // and also flag that the PIN should be changed to MBED_CONF_APP_ALT_PIN, 00548 // then try connecting 00549 interface->sim_pin_check_enable(true); 00550 interface->change_sim_pin(MBED_CONF_APP_ALT_PIN); 00551 TEST_ASSERT(interface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00552 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00553 use_connection(interface); 00554 drop_connection(interface); 00555 interface->deinit(); 00556 00557 // Now change the PIN back to what it was before 00558 interface->change_sim_pin(MBED_CONF_APP_DEFAULT_PIN); 00559 TEST_ASSERT(interface->connect(MBED_CONF_APP_ALT_PIN, MBED_CONF_APP_APN, 00560 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00561 use_connection(interface); 00562 drop_connection(interface); 00563 interface->deinit(); 00564 00565 // Check that it was changed back, and this time 00566 // use the other way of entering the PIN 00567 interface->set_sim_pin(MBED_CONF_APP_DEFAULT_PIN); 00568 TEST_ASSERT(interface->connect(NULL, MBED_CONF_APP_APN, MBED_CONF_APP_USERNAME, 00569 MBED_CONF_APP_PASSWORD) == 0); 00570 use_connection(interface); 00571 drop_connection(interface); 00572 interface->deinit(); 00573 00574 // Remove PIN checking again and check that it no 00575 // longer matters what the PIN is 00576 interface->sim_pin_check_enable(false); 00577 TEST_ASSERT(interface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00578 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00579 use_connection(interface); 00580 drop_connection(interface); 00581 interface->deinit(); 00582 TEST_ASSERT(interface->init(NULL)); 00583 TEST_ASSERT(interface->connect(MBED_CONF_APP_INCORRECT_PIN, MBED_CONF_APP_APN, 00584 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00585 use_connection(interface); 00586 drop_connection(interface); 00587 00588 // Put the SIM pin back to the correct value for any subsequent tests 00589 interface->set_sim_pin(MBED_CONF_APP_DEFAULT_PIN); 00590 } 00591 00592 // Test adding and using a SIM pin, then removing it, using the immediate 00593 // mechanism 00594 void test_check_sim_pin_immediate() { 00595 00596 interface->deinit(); 00597 interface->connection_status_cb(callback(connection_down_cb)); 00598 00599 // Enable PIN checking (which will use the current PIN), change 00600 // the PIN to MBED_CONF_APP_ALT_PIN, then try connecting after powering on and 00601 // off the modem 00602 interface->set_sim_pin_check(true, true, MBED_CONF_APP_DEFAULT_PIN); 00603 interface->set_new_sim_pin(MBED_CONF_APP_ALT_PIN, true); 00604 interface->deinit(); 00605 TEST_ASSERT(interface->init(NULL)); 00606 TEST_ASSERT(interface->connect(MBED_CONF_APP_ALT_PIN, MBED_CONF_APP_APN, 00607 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00608 use_connection(interface); 00609 drop_connection(interface); 00610 00611 interface->connection_status_cb(callback(connection_down_cb)); 00612 00613 // Now change the PIN back to what it was before 00614 interface->set_new_sim_pin(MBED_CONF_APP_DEFAULT_PIN, true); 00615 interface->deinit(); 00616 interface->set_sim_pin(MBED_CONF_APP_DEFAULT_PIN); 00617 TEST_ASSERT(interface->init(NULL)); 00618 TEST_ASSERT(interface->connect(NULL, MBED_CONF_APP_APN, MBED_CONF_APP_USERNAME, 00619 MBED_CONF_APP_PASSWORD) == 0); 00620 use_connection(interface); 00621 drop_connection(interface); 00622 00623 interface->connection_status_cb(callback(connection_down_cb)); 00624 00625 // Remove PIN checking again and check that it no 00626 // longer matters what the PIN is 00627 interface->set_sim_pin_check(false, true); 00628 interface->deinit(); 00629 TEST_ASSERT(interface->init(MBED_CONF_APP_INCORRECT_PIN)); 00630 TEST_ASSERT(interface->connect(NULL, MBED_CONF_APP_APN, MBED_CONF_APP_USERNAME, 00631 MBED_CONF_APP_PASSWORD) == 0); 00632 use_connection(interface); 00633 drop_connection(interface); 00634 00635 // Put the SIM pin back to the correct value for any subsequent tests 00636 interface->set_sim_pin(MBED_CONF_APP_DEFAULT_PIN); 00637 } 00638 00639 // Test being able to connect with a local instance of the driver 00640 // NOTE: since this local instance will fiddle with bits of HW that the 00641 // static instance thought it owned, the static instance will no longer 00642 // work afterwards, hence this must be run as the last test in the list 00643 void test_connect_local_instance_last_test() { 00644 00645 UbloxPPPCellularInterface *pLocalInterface = NULL; 00646 00647 pLocalInterface = new UbloxPPPCellularInterface(MDMTXD, MDMRXD, 00648 MBED_CONF_UBLOX_CELL_BAUD_RATE, 00649 MBED_CONF_APP_DEBUG_ON); 00650 pLocalInterface->connection_status_cb(callback(connection_down_cb)); 00651 00652 TEST_ASSERT(pLocalInterface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00653 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00654 use_connection(pLocalInterface); 00655 drop_connection(pLocalInterface); 00656 delete pLocalInterface; 00657 00658 pLocalInterface = new UbloxPPPCellularInterface(MDMTXD, MDMRXD, 00659 MBED_CONF_UBLOX_CELL_BAUD_RATE, 00660 MBED_CONF_APP_DEBUG_ON); 00661 pLocalInterface->connection_status_cb(callback(connection_down_cb)); 00662 00663 TEST_ASSERT(pLocalInterface->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00664 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00665 use_connection(pLocalInterface); 00666 drop_connection(pLocalInterface); 00667 delete pLocalInterface; 00668 } 00669 00670 // ---------------------------------------------------------------- 00671 // TEST ENVIRONMENT 00672 // ---------------------------------------------------------------- 00673 00674 // Setup the test environment 00675 utest::v1::status_t test_setup(const size_t number_of_cases) { 00676 // Setup Greentea with a timeout 00677 GREENTEA_SETUP(600, "default_auto"); 00678 return verbose_test_setup_handler(number_of_cases); 00679 } 00680 00681 // IMPORTANT!!! if you make a change to the tests here you should 00682 // check whether the same change should be made to the tests under 00683 // the AT interface. 00684 00685 // Test cases 00686 Case cases[] = { 00687 Case("Set randomise", test_set_randomise), 00688 #ifdef MBED_CONF_APP_ECHO_SERVER 00689 Case("UDP echo test", test_udp_echo), 00690 # if MBED_CONF_LWIP_TCP_ENABLED 00691 Case("TCP async echo test", test_tcp_echo_async), 00692 # endif 00693 #endif 00694 Case("Connect with credentials", test_connect_credentials), 00695 Case("Connect with preset credentials", test_connect_preset_credentials), 00696 #if MBED_CONF_APP_RUN_SIM_PIN_CHANGE_TESTS 00697 Case("Check SIM pin, pending", test_check_sim_pin_pending), 00698 Case("Check SIM pin, immediate", test_check_sim_pin_immediate), 00699 #endif 00700 #ifndef TARGET_UBLOX_C027 // Not enough RAM on little 'ole C027 for this 00701 Case("Connect using local instance, must be last test", test_connect_local_instance_last_test) 00702 #endif 00703 }; 00704 00705 Specification specification(test_setup, cases); 00706 00707 // ---------------------------------------------------------------- 00708 // MAIN 00709 // ---------------------------------------------------------------- 00710 00711 int main() { 00712 00713 #ifdef FEATURE_COMMON_PAL 00714 mbed_trace_init(); 00715 00716 mbed_trace_mutex_wait_function_set(lock); 00717 mbed_trace_mutex_release_function_set(unlock); 00718 #endif 00719 00720 interface->connection_status_cb(callback(connection_down_cb)); 00721 00722 // Run tests 00723 return !Harness::run(specification); 00724 } 00725 00726 #else 00727 00728 // This looks a bit peculiar. These tests should only be compiled if LWIP is included and PPP support is enabled. 00729 // The way the "mbed test" command, which parses these files, does its filtering is by looking for the pattern: 00730 // 00731 // #ifndef THING 00732 // #error [NOT_SUPPORTED] this test can only be used if THING is defined 00733 // endif 00734 // 00735 // So if THING is not defined, this test will not even be compiled. However, the only compilation switch which 00736 // is visible here for PPP being enabled or not is NSAPI_PPP_AVAILABLE, which is 0 if PPP is not enabled (the default) 00737 // or 1 if PPP is enabled. There is NO visible compilation switch which is or isn't _defined_ when PPP is or 00738 // isn't enabled. 00739 // 00740 // Instead, here is a dummy test body which clearly states that these tests are irrelevant 'cos there's no PPP. 00741 00742 void dummy() { 00743 } 00744 utest::v1::status_t test_setup(const size_t number_of_cases) { 00745 GREENTEA_SETUP(10, "default_auto"); 00746 return verbose_test_setup_handler(number_of_cases); 00747 } 00748 Case cases[] = { 00749 Case("No PPP, no tests to run", dummy) 00750 }; 00751 Specification specification(test_setup, cases); 00752 00753 int main() { 00754 return !Harness::run(specification);; 00755 } 00756 00757 #endif 00758 00759 // End Of File 00760
Generated on Wed Jul 13 2022 20:24:27 by
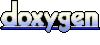