
Program to demonstrate the use of the LowPowerSleep class on the u-blox C030 platform.
Dependencies: gnss low-power-sleep
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "low_power.h" 00019 #include "gnss.h" 00020 00021 #define GNSS_WAIT_TIME_SECONDS 120 00022 #define STOP_TIME_SECONDS 5 00023 #define STANDBY_TIME_SECONDS 5 00024 #define CHECK_TALKER(s) ((buffer[3] == s[0]) && (buffer[4] == s[1]) && (buffer[5] == s[2])) 00025 #define BACKUP_SRAM_STRING "Back from Standby mode!" 00026 00027 // Put the time at the start of back-up SRAM 00028 BACKUP_SRAM 00029 static time_t timeNow; 00030 00031 // The rest of backup SRAM 00032 BACKUP_SRAM 00033 static char backupSram[BACKUP_SRAM_SIZE - sizeof (timeNow)]; 00034 00035 // LEDs 00036 DigitalOut ledRed(LED1, 1); 00037 DigitalOut ledGreen(LED2, 1); 00038 DigitalOut ledBlue(LED3, 1); 00039 00040 /* IMPORTANT: this code puts the STM32F4xx chip into its lowest power state. 00041 * The ability to do this is affected by the state of the debug chip on the C030 00042 * board. To be sure that this code executes correctly, you MUST completely 00043 * power off the board after downloading code, and power it back on again. 00044 */ 00045 00046 /* This example program for the u-blox C030 board demonstrates the use of 00047 * the low power driver. It waits for GNSS to obtain the time, entering Stop 00048 * mode while waiting for the GNSS to return results and, once the time has 00049 * been obtained, it enters Standby mode, putting some stored information 00050 * into backup SRAM where it will remain safe during Standby mode. 00051 * Note: the use of GNSS is in no way related to the LowPowerSleep class, 00052 * it's simply something to do in this demonstration. 00053 * Progress may be monitored with a serial terminal running at 9600 baud. 00054 * The LED on the C030 board will turn green when this program is operating 00055 * correctly, flash white when GNSS time is received, and turn red if GNSS 00056 * time was not received or there is a failure. 00057 */ 00058 00059 // Get the time from GNSS 00060 static bool gnssGetTime(GnssSerial *gnss) 00061 { 00062 char buffer[256]; 00063 bool gotTime = false; 00064 int gnssReturnCode; 00065 int32_t length; 00066 00067 while ((gnssReturnCode = gnss->getMessage(buffer, sizeof(buffer))) > 0) { 00068 length = LENGTH(gnssReturnCode); 00069 00070 if ((PROTOCOL(gnssReturnCode) == GnssParser::NMEA) && (length > 6)) { 00071 // Talker is $GA=Galileo $GB=Beidou $GL=Glonass $GN=Combined $GP=GNSS 00072 if ((buffer[0] == '$') || buffer[1] == 'G') { 00073 if (CHECK_TALKER("GGA") || CHECK_TALKER("GNS")) { 00074 const char *timeString = NULL; 00075 // Retrieve the time 00076 timeString = gnss->findNmeaItemPos(1, buffer, buffer + length); 00077 if (timeString != NULL) { 00078 gotTime = true; 00079 printf("\nGNSS: time is %.6s (UTC).\n", timeString); 00080 ledBlue = 0; 00081 ledGreen = 0; 00082 ledRed = 0; 00083 wait_ms(1000); 00084 } 00085 } 00086 } 00087 } 00088 } 00089 00090 return gotTime; 00091 } 00092 00093 // Main 00094 int main() 00095 { 00096 GnssSerial gnss; 00097 LowPower lowPower; 00098 bool gotTime = false; 00099 00100 // First exit Debug mode on the chip, otherwise it will not be 00101 // able to enter Standby mode 00102 lowPower.exitDebugMode(); 00103 00104 ledGreen = 0; 00105 ledRed = 1; 00106 ledBlue = 1; 00107 00108 // Initialise GNSS 00109 if (gnss.init()) { 00110 if (time(NULL) != 0) { 00111 // If the RTC is running, we must have been awake previously 00112 printf ("Awake from Standby mode after %d second(s).\n", 00113 (int) (time(NULL) - timeNow)); 00114 printf ("Backup RAM contains \"%.*s\".\n\n", sizeof(BACKUP_SRAM_STRING), 00115 backupSram); 00116 } else { 00117 printf("\n\nStarting up from a cold start.\n"); 00118 printf("IMPORTANT: this code puts the STM32F4xx chip into its lowest power state.\n"); 00119 printf("The ability to do this is affected by the state of the debug chip on the C030\n"); 00120 printf("board. To be sure that this code executes correctly, you must completely power\n"); 00121 printf("off the board after downloading code, and power it back on again.\n\n"); 00122 } 00123 00124 printf ("Waiting up to %d second(s) for GNSS to receive the time...\n", GNSS_WAIT_TIME_SECONDS); 00125 for (uint32_t x = 0; (x < GNSS_WAIT_TIME_SECONDS) && !gotTime; x+= STOP_TIME_SECONDS) { 00126 gotTime = gnssGetTime(&gnss); 00127 00128 if (!gotTime) { 00129 printf (" Entering Stop mode for %d second(s) while waiting...\n", STOP_TIME_SECONDS); 00130 // Let the printf leave the building 00131 wait_ms(100); 00132 time_t y = time(NULL); 00133 lowPower.enterStop(STOP_TIME_SECONDS * 1000); 00134 printf (" Awake from Stop mode after %d second(s).\n", (int) (time(NULL) - y)); 00135 } 00136 } 00137 00138 ledGreen = 1; 00139 ledRed = 1; 00140 ledBlue = 1; 00141 if (!gotTime) { 00142 ledRed = 0; 00143 } 00144 00145 printf ("\nPutting \"%s\" into BKPSRAM...\n", BACKUP_SRAM_STRING); 00146 memcpy (backupSram, BACKUP_SRAM_STRING, sizeof(BACKUP_SRAM_STRING)); 00147 00148 printf ("Entering Standby mode, losing all RAM contents, for %d second(s)...\n\n", STANDBY_TIME_SECONDS); 00149 // Let the printf leave the building 00150 wait_ms(100); 00151 // We will return at the start of main() when the Standby time expires 00152 timeNow = time(NULL); 00153 lowPower.enterStandby(STANDBY_TIME_SECONDS * 1000); 00154 } else { 00155 printf("Unable to initialise GNSS.\n"); 00156 } 00157 00158 ledRed = 0; 00159 ledGreen = 1; 00160 ledBlue = 1; 00161 printf("Should never get here.\n"); 00162 MBED_ASSERT(false); 00163 } 00164 00165 // End Of File
Generated on Sat Jul 16 2022 00:41:41 by
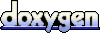