
Example program to demonstrate the use of the BatteryGaugeBQ27441 class.
Dependencies: battery-gauge-bq27441
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "battery_gauge_bq27441.h" 00019 00020 // LEDs 00021 DigitalOut ledRed(LED1, 1); 00022 DigitalOut ledGreen(LED2, 1); 00023 00024 /* This example program for the u-blox C030 board instantiates 00025 * the BQ27441 battery gauge and performs a few example calls to 00026 * the battery gauge API. Progress may be monitored with a 00027 * serial terminal running at 9600 baud. The LED on the C030 00028 * board will turn green when this program is operating correctly 00029 * and will turn red if there is a failure. 00030 */ 00031 00032 int main() 00033 { 00034 I2C i2C(I2C_SDA_B, I2C_SCL_B); 00035 BatteryGaugeBq27441 gauge; 00036 int32_t reading; 00037 bool stop = false; 00038 00039 printf ("Starting up...\n"); 00040 if (gauge.init(&i2C)) { 00041 printf ("BQ27441 battery gauge chip is initialised.\n"); 00042 #ifdef TARGET_UBLOX_C030 00043 if (gauge.disableBatteryDetect()) { 00044 printf ("Battery detection input disabled (it is not connected on C030).\n"); 00045 } 00046 #endif 00047 while (!stop) { 00048 if (gauge.isBatteryDetected()) { 00049 if (!gauge.isGaugeEnabled()) { 00050 gauge.enableGauge(); 00051 } 00052 if (gauge.isGaugeEnabled()) { 00053 // All is good, gauging is enabled, take a few readings 00054 ledGreen = 0; 00055 ledRed = 1; 00056 00057 if (gauge.getRemainingPercentage(&reading)) { 00058 printf("Remaining battery percentage: %d%%.\n", (int) reading); 00059 } else { 00060 ledGreen = 1; 00061 ledRed = 0; 00062 wait_ms(1000); 00063 } 00064 00065 if (gauge.getRemainingCapacity(&reading)) { 00066 printf("Remaining battery capacity: %.3f Ah.\n", ((float) reading) / 1000); 00067 } else { 00068 ledGreen = 1; 00069 ledRed = 0; 00070 wait_ms(1000); 00071 } 00072 00073 if (gauge.getVoltage(&reading)) { 00074 printf("Battery voltage: %.3f V.\n", ((float) reading) / 1000); 00075 } else { 00076 ledGreen = 1; 00077 ledRed = 0; 00078 wait_ms(1000); 00079 } 00080 00081 if (gauge.getCurrent(&reading)) { 00082 printf("Current drawn from battery: %.3f A.\n", ((float) reading) / 1000); 00083 } else { 00084 ledGreen = 1; 00085 ledRed = 0; 00086 wait_ms(1000); 00087 } 00088 00089 if (gauge.getTemperature(&reading)) { 00090 printf("BQ27441 chip temperature: %d C.\n", (int) reading); 00091 } else { 00092 ledGreen = 1; 00093 ledRed = 0; 00094 wait_ms(1000); 00095 } 00096 00097 if (gauge.getPower(&reading)) { 00098 printf("Power consumption : %.3f W.\n", ((float) reading) / 1000); 00099 } else { 00100 ledGreen = 1; 00101 ledRed = 0; 00102 wait_ms(1000); 00103 } 00104 00105 } else { 00106 printf("Battery gauge could not be enabled.\n"); 00107 stop = true; 00108 } 00109 } else { 00110 ledGreen = 1; 00111 ledRed = 0; 00112 printf("Battery not detected.\n"); 00113 } 00114 00115 wait_ms(5000); 00116 } 00117 } 00118 00119 ledGreen = 1; 00120 ledRed = 0; 00121 printf("Should never get here: do you have a battery connected?\n"); 00122 MBED_ASSERT(false); 00123 } 00124 00125 // End Of File
Generated on Sat Jul 16 2022 13:05:11 by
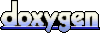