
Example program to demonstrate the use of the BatteryChargerBQ24295 class.
Dependencies: battery-charger-bq24295
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "battery_charger_bq24295.h" 00019 00020 // LEDs 00021 DigitalOut ledRed(LED1, 1); 00022 DigitalOut ledGreen(LED2, 1); 00023 00024 /* This example program for the u-blox C030 board instantiates 00025 * the BQ24295 battery charger interface and performs a few 00026 * example calls to the battery charger API, displaying information 00027 * about the state of the charger chip. Progress may be 00028 * monitored with a serial terminal running at 9600 baud. The 00029 * LED on the C030 board will turn green when this program is 00030 * operating correctly and will turn red if there is a failure. 00031 * 00032 * To understand the meaning of the API calls it is necessary to 00033 * refer to the data sheet for the TI BQ24295 chip. 00034 * IMPORTANT it is NOT necessary to use the BQ24295 class in order 00035 * to charge a battery connected to the C030 board, that will happen 00036 * automatically. The BQ24295 class is only required if the battery 00037 * charger is to be configured or monitored. 00038 */ 00039 00040 int main() 00041 { 00042 I2C i2C(I2C_SDA_B, I2C_SCL_B); 00043 BatteryChargerBq24295 charger; 00044 BatteryChargerBq24295::ChargerState state = BatteryChargerBq24295::CHARGER_STATE_UNKNOWN; 00045 char fault = 0; 00046 00047 printf ("Starting up...\n"); 00048 if (charger.init(&i2C)) { 00049 00050 printf ("BQ24295 battery charger chip is initialised.\n"); 00051 if (charger.isExternalPowerPresent()) { 00052 printf ("External power is present.\n"); 00053 } else { 00054 printf ("External power is NOT present.\n"); 00055 } 00056 if (charger.areInputLimitsEnabled()) { 00057 printf ("Input limits are enabled.\n"); 00058 } else { 00059 printf ("Input limits are disabled.\n"); 00060 } 00061 if (charger.isChargingEnabled()) { 00062 printf ("Charging is already enabled.\n"); 00063 } else { 00064 printf ("Charging is disabled.\n"); 00065 } 00066 if (charger.isOtgEnabled()) { 00067 printf ("OTG is enabled.\n"); 00068 } else { 00069 printf ("OTG is disabled.\n"); 00070 } 00071 if (charger.setWatchdog(160)) { 00072 printf ("Watchdog set to 160 seconds.\n"); 00073 } 00074 00075 while (state < BatteryChargerBq24295::MAX_NUM_CHARGER_STATES) { 00076 // Feed the watchdog timer to keep the charger chip in 00077 // Host mode while we are monitoring it. If we do not 00078 // feed the watchdog it will expire, which does no 00079 // harm at all (the chip is now simply in default mode again 00080 // and will return to host mode if we write to it), but 00081 // it will appear as a fault state. 00082 charger.feedWatchdog(); 00083 fault = charger.getChargerFaults(); 00084 if (fault == BatteryChargerBq24295::CHARGER_FAULT_NONE) { 00085 ledGreen = 0; 00086 ledRed = 1; 00087 state = charger.getChargerState(); 00088 switch (state) { 00089 case BatteryChargerBq24295::CHARGER_STATE_UNKNOWN: 00090 printf("Charger state is not (yet) known.\n"); 00091 break; 00092 case BatteryChargerBq24295::CHARGER_STATE_DISABLED: 00093 printf("Charger state: disabled.\n"); 00094 break; 00095 case BatteryChargerBq24295::CHARGER_STATE_NO_EXTERNAL_POWER: 00096 printf("Charger state: no external power.\n"); 00097 break; 00098 case BatteryChargerBq24295::CHARGER_STATE_NOT_CHARGING: 00099 printf("Charger state: not charging.\n"); 00100 break; 00101 case BatteryChargerBq24295::CHARGER_STATE_PRECHARGE: 00102 printf("Charger state: pre-charge.\n"); 00103 break; 00104 case BatteryChargerBq24295::CHARGER_STATE_FAST_CHARGE: 00105 printf("Charger state: fast charge.\n"); 00106 break; 00107 case BatteryChargerBq24295::CHARGER_STATE_COMPLETE: 00108 printf("Charger state: charging complete.\n"); 00109 break; 00110 default: 00111 printf("Unknown charger state (%d).\n", state); 00112 break; 00113 } 00114 } else { 00115 ledGreen = 1; 00116 ledRed = 0; 00117 if (fault & BatteryChargerBq24295::CHARGER_FAULT_THERMISTOR_TOO_HOT) { 00118 printf("Charger fault: thermistor is too hot.\n"); 00119 } 00120 if (fault & BatteryChargerBq24295::CHARGER_FAULT_THERMISTOR_TOO_COLD) { 00121 printf("Charger fault: thermistor is too cold.\n"); 00122 } 00123 if (fault & BatteryChargerBq24295::CHARGER_FAULT_BATTERY_OVER_VOLTAGE) { 00124 printf("Charger fault: battery over-voltage.\n"); 00125 } 00126 // The testing of the fault bit map looks slightly strange here. 00127 // This is because the definition of the fault bits in the 00128 // BQ24295 chip means that Charger Timer Expired overlaps 00129 // input fault and thermal shutdown. See the definition of the 00130 // meaning of REG09 in the BQ24295 data sheet for more information. 00131 if ((fault & BatteryChargerBq24295::CHARGER_FAULT_INPUT_FAULT) && 00132 !(fault & BatteryChargerBq24295::CHARGER_FAULT_THERMAL_SHUTDOWN)) { 00133 printf("Charger fault: input fault.\n"); 00134 } 00135 if ((fault & BatteryChargerBq24295::CHARGER_FAULT_THERMAL_SHUTDOWN) && 00136 !(fault & BatteryChargerBq24295::CHARGER_FAULT_INPUT_FAULT)) { 00137 printf("Charger fault: thermal shutdown.\n"); 00138 } 00139 if ((fault & BatteryChargerBq24295::CHARGER_FAULT_CHARGE_TIMER_EXPIRED) == 00140 BatteryChargerBq24295::CHARGER_FAULT_CHARGE_TIMER_EXPIRED) { 00141 printf("Charger fault: charge timer expired.\n"); 00142 } 00143 if (fault & BatteryChargerBq24295::CHARGER_FAULT_OTG) { 00144 printf("Charger fault: OTG charging fault.\n"); 00145 } 00146 if (fault & BatteryChargerBq24295::CHARGER_FAULT_WATCHDOG_EXPIRED) { 00147 printf("Charger fault: charger watchdog expired.\n"); 00148 } 00149 } 00150 wait_ms(5000); 00151 } 00152 } 00153 00154 ledGreen = 1; 00155 ledRed = 0; 00156 printf("Should never get here.\n"); 00157 MBED_ASSERT(false); 00158 } 00159 00160 // End Of File
Generated on Sun Jul 17 2022 05:01:16 by
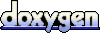