mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
ssl_tls.c
00001 /* 00002 * SSLv3/TLSv1 shared functions 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * The SSL 3.0 specification was drafted by Netscape in 1996, 00023 * and became an IETF standard in 1999. 00024 * 00025 * http://wp.netscape.com/eng/ssl3/ 00026 * http://www.ietf.org/rfc/rfc2246.txt 00027 * http://www.ietf.org/rfc/rfc4346.txt 00028 */ 00029 00030 #if !defined(MBEDTLS_CONFIG_FILE) 00031 #include "mbedtls/config.h" 00032 #else 00033 #include MBEDTLS_CONFIG_FILE 00034 #endif 00035 00036 #if defined(MBEDTLS_SSL_TLS_C) 00037 00038 #include "mbedtls/debug.h" 00039 #include "mbedtls/ssl.h" 00040 #include "mbedtls/ssl_internal.h" 00041 00042 #include <string.h> 00043 00044 #if defined(MBEDTLS_X509_CRT_PARSE_C) && \ 00045 defined(MBEDTLS_X509_CHECK_EXTENDED_KEY_USAGE) 00046 #include "mbedtls/oid.h" 00047 #endif 00048 00049 #if defined(MBEDTLS_PLATFORM_C) 00050 #include "mbedtls/platform.h" 00051 #else 00052 #include <stdlib.h> 00053 #define mbedtls_calloc calloc 00054 #define mbedtls_free free 00055 #endif 00056 00057 /* Implementation that should never be optimized out by the compiler */ 00058 static void mbedtls_zeroize( void *v, size_t n ) { 00059 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00060 } 00061 00062 /* Length of the "epoch" field in the record header */ 00063 static inline size_t ssl_ep_len( const mbedtls_ssl_context *ssl ) 00064 { 00065 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00066 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 00067 return( 2 ); 00068 #else 00069 ((void) ssl); 00070 #endif 00071 return( 0 ); 00072 } 00073 00074 /* 00075 * Start a timer. 00076 * Passing millisecs = 0 cancels a running timer. 00077 */ 00078 static void ssl_set_timer( mbedtls_ssl_context *ssl, uint32_t millisecs ) 00079 { 00080 if( ssl->f_set_timer == NULL ) 00081 return; 00082 00083 MBEDTLS_SSL_DEBUG_MSG( 3, ( "set_timer to %d ms", (int) millisecs ) ); 00084 ssl->f_set_timer( ssl->p_timer, millisecs / 4, millisecs ); 00085 } 00086 00087 /* 00088 * Return -1 is timer is expired, 0 if it isn't. 00089 */ 00090 static int ssl_check_timer( mbedtls_ssl_context *ssl ) 00091 { 00092 if( ssl->f_get_timer == NULL ) 00093 return( 0 ); 00094 00095 if( ssl->f_get_timer( ssl->p_timer ) == 2 ) 00096 { 00097 MBEDTLS_SSL_DEBUG_MSG( 3, ( "timer expired" ) ); 00098 return( -1 ); 00099 } 00100 00101 return( 0 ); 00102 } 00103 00104 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00105 /* 00106 * Double the retransmit timeout value, within the allowed range, 00107 * returning -1 if the maximum value has already been reached. 00108 */ 00109 static int ssl_double_retransmit_timeout( mbedtls_ssl_context *ssl ) 00110 { 00111 uint32_t new_timeout; 00112 00113 if( ssl->handshake->retransmit_timeout >= ssl->conf->hs_timeout_max ) 00114 return( -1 ); 00115 00116 new_timeout = 2 * ssl->handshake->retransmit_timeout; 00117 00118 /* Avoid arithmetic overflow and range overflow */ 00119 if( new_timeout < ssl->handshake->retransmit_timeout || 00120 new_timeout > ssl->conf->hs_timeout_max ) 00121 { 00122 new_timeout = ssl->conf->hs_timeout_max; 00123 } 00124 00125 ssl->handshake->retransmit_timeout = new_timeout; 00126 MBEDTLS_SSL_DEBUG_MSG( 3, ( "update timeout value to %d millisecs", 00127 ssl->handshake->retransmit_timeout ) ); 00128 00129 return( 0 ); 00130 } 00131 00132 static void ssl_reset_retransmit_timeout( mbedtls_ssl_context *ssl ) 00133 { 00134 ssl->handshake->retransmit_timeout = ssl->conf->hs_timeout_min; 00135 MBEDTLS_SSL_DEBUG_MSG( 3, ( "update timeout value to %d millisecs", 00136 ssl->handshake->retransmit_timeout ) ); 00137 } 00138 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 00139 00140 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 00141 /* 00142 * Convert max_fragment_length codes to length. 00143 * RFC 6066 says: 00144 * enum{ 00145 * 2^9(1), 2^10(2), 2^11(3), 2^12(4), (255) 00146 * } MaxFragmentLength; 00147 * and we add 0 -> extension unused 00148 */ 00149 static unsigned int mfl_code_to_length[MBEDTLS_SSL_MAX_FRAG_LEN_INVALID] = 00150 { 00151 MBEDTLS_SSL_MAX_CONTENT_LEN, /* MBEDTLS_SSL_MAX_FRAG_LEN_NONE */ 00152 512, /* MBEDTLS_SSL_MAX_FRAG_LEN_512 */ 00153 1024, /* MBEDTLS_SSL_MAX_FRAG_LEN_1024 */ 00154 2048, /* MBEDTLS_SSL_MAX_FRAG_LEN_2048 */ 00155 4096, /* MBEDTLS_SSL_MAX_FRAG_LEN_4096 */ 00156 }; 00157 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 00158 00159 #if defined(MBEDTLS_SSL_CLI_C) 00160 static int ssl_session_copy( mbedtls_ssl_session *dst, const mbedtls_ssl_session *src ) 00161 { 00162 mbedtls_ssl_session_free( dst ); 00163 memcpy( dst, src, sizeof( mbedtls_ssl_session ) ); 00164 00165 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00166 if( src->peer_cert != NULL ) 00167 { 00168 int ret; 00169 00170 dst->peer_cert = mbedtls_calloc( 1, sizeof(mbedtls_x509_crt) ); 00171 if( dst->peer_cert == NULL ) 00172 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 00173 00174 mbedtls_x509_crt_init( dst->peer_cert ); 00175 00176 if( ( ret = mbedtls_x509_crt_parse_der( dst->peer_cert, src->peer_cert->raw.p, 00177 src->peer_cert->raw.len ) ) != 0 ) 00178 { 00179 mbedtls_free( dst->peer_cert ); 00180 dst->peer_cert = NULL; 00181 return( ret ); 00182 } 00183 } 00184 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 00185 00186 #if defined(MBEDTLS_SSL_SESSION_TICKETS) && defined(MBEDTLS_SSL_CLI_C) 00187 if( src->ticket != NULL ) 00188 { 00189 dst->ticket = mbedtls_calloc( 1, src->ticket_len ); 00190 if( dst->ticket == NULL ) 00191 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 00192 00193 memcpy( dst->ticket, src->ticket, src->ticket_len ); 00194 } 00195 #endif /* MBEDTLS_SSL_SESSION_TICKETS && MBEDTLS_SSL_CLI_C */ 00196 00197 return( 0 ); 00198 } 00199 #endif /* MBEDTLS_SSL_CLI_C */ 00200 00201 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 00202 int (*mbedtls_ssl_hw_record_init)( mbedtls_ssl_context *ssl, 00203 const unsigned char *key_enc, const unsigned char *key_dec, 00204 size_t keylen, 00205 const unsigned char *iv_enc, const unsigned char *iv_dec, 00206 size_t ivlen, 00207 const unsigned char *mac_enc, const unsigned char *mac_dec, 00208 size_t maclen ) = NULL; 00209 int (*mbedtls_ssl_hw_record_activate)( mbedtls_ssl_context *ssl, int direction) = NULL; 00210 int (*mbedtls_ssl_hw_record_reset)( mbedtls_ssl_context *ssl ) = NULL; 00211 int (*mbedtls_ssl_hw_record_write)( mbedtls_ssl_context *ssl ) = NULL; 00212 int (*mbedtls_ssl_hw_record_read)( mbedtls_ssl_context *ssl ) = NULL; 00213 int (*mbedtls_ssl_hw_record_finish)( mbedtls_ssl_context *ssl ) = NULL; 00214 #endif /* MBEDTLS_SSL_HW_RECORD_ACCEL */ 00215 00216 /* 00217 * Key material generation 00218 */ 00219 #if defined(MBEDTLS_SSL_PROTO_SSL3) 00220 static int ssl3_prf( const unsigned char *secret, size_t slen, 00221 const char *label, 00222 const unsigned char *random, size_t rlen, 00223 unsigned char *dstbuf, size_t dlen ) 00224 { 00225 size_t i; 00226 mbedtls_md5_context md5; 00227 mbedtls_sha1_context sha1; 00228 unsigned char padding[16]; 00229 unsigned char sha1sum[20]; 00230 ((void)label); 00231 00232 mbedtls_md5_init( &md5 ); 00233 mbedtls_sha1_init( &sha1 ); 00234 00235 /* 00236 * SSLv3: 00237 * block = 00238 * MD5( secret + SHA1( 'A' + secret + random ) ) + 00239 * MD5( secret + SHA1( 'BB' + secret + random ) ) + 00240 * MD5( secret + SHA1( 'CCC' + secret + random ) ) + 00241 * ... 00242 */ 00243 for( i = 0; i < dlen / 16; i++ ) 00244 { 00245 memset( padding, (unsigned char) ('A' + i), 1 + i ); 00246 00247 mbedtls_sha1_starts( &sha1 ); 00248 mbedtls_sha1_update( &sha1, padding, 1 + i ); 00249 mbedtls_sha1_update( &sha1, secret, slen ); 00250 mbedtls_sha1_update( &sha1, random, rlen ); 00251 mbedtls_sha1_finish( &sha1, sha1sum ); 00252 00253 mbedtls_md5_starts( &md5 ); 00254 mbedtls_md5_update( &md5, secret, slen ); 00255 mbedtls_md5_update( &md5, sha1sum, 20 ); 00256 mbedtls_md5_finish( &md5, dstbuf + i * 16 ); 00257 } 00258 00259 mbedtls_md5_free( &md5 ); 00260 mbedtls_sha1_free( &sha1 ); 00261 00262 mbedtls_zeroize( padding, sizeof( padding ) ); 00263 mbedtls_zeroize( sha1sum, sizeof( sha1sum ) ); 00264 00265 return( 0 ); 00266 } 00267 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 00268 00269 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) 00270 static int tls1_prf( const unsigned char *secret, size_t slen, 00271 const char *label, 00272 const unsigned char *random, size_t rlen, 00273 unsigned char *dstbuf, size_t dlen ) 00274 { 00275 size_t nb, hs; 00276 size_t i, j, k; 00277 const unsigned char *S1, *S2; 00278 unsigned char tmp[128]; 00279 unsigned char h_i[20]; 00280 const mbedtls_md_info_t *md_info; 00281 mbedtls_md_context_t md_ctx; 00282 int ret; 00283 00284 mbedtls_md_init( &md_ctx ); 00285 00286 if( sizeof( tmp ) < 20 + strlen( label ) + rlen ) 00287 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 00288 00289 hs = ( slen + 1 ) / 2; 00290 S1 = secret; 00291 S2 = secret + slen - hs; 00292 00293 nb = strlen( label ); 00294 memcpy( tmp + 20, label, nb ); 00295 memcpy( tmp + 20 + nb, random, rlen ); 00296 nb += rlen; 00297 00298 /* 00299 * First compute P_md5(secret,label+random)[0..dlen] 00300 */ 00301 if( ( md_info = mbedtls_md_info_from_type( MBEDTLS_MD_MD5 ) ) == NULL ) 00302 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00303 00304 if( ( ret = mbedtls_md_setup( &md_ctx, md_info, 1 ) ) != 0 ) 00305 return( ret ); 00306 00307 mbedtls_md_hmac_starts( &md_ctx, S1, hs ); 00308 mbedtls_md_hmac_update( &md_ctx, tmp + 20, nb ); 00309 mbedtls_md_hmac_finish( &md_ctx, 4 + tmp ); 00310 00311 for( i = 0; i < dlen; i += 16 ) 00312 { 00313 mbedtls_md_hmac_reset ( &md_ctx ); 00314 mbedtls_md_hmac_update( &md_ctx, 4 + tmp, 16 + nb ); 00315 mbedtls_md_hmac_finish( &md_ctx, h_i ); 00316 00317 mbedtls_md_hmac_reset ( &md_ctx ); 00318 mbedtls_md_hmac_update( &md_ctx, 4 + tmp, 16 ); 00319 mbedtls_md_hmac_finish( &md_ctx, 4 + tmp ); 00320 00321 k = ( i + 16 > dlen ) ? dlen % 16 : 16; 00322 00323 for( j = 0; j < k; j++ ) 00324 dstbuf[i + j] = h_i[j]; 00325 } 00326 00327 mbedtls_md_free( &md_ctx ); 00328 00329 /* 00330 * XOR out with P_sha1(secret,label+random)[0..dlen] 00331 */ 00332 if( ( md_info = mbedtls_md_info_from_type( MBEDTLS_MD_SHA1 ) ) == NULL ) 00333 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00334 00335 if( ( ret = mbedtls_md_setup( &md_ctx, md_info, 1 ) ) != 0 ) 00336 return( ret ); 00337 00338 mbedtls_md_hmac_starts( &md_ctx, S2, hs ); 00339 mbedtls_md_hmac_update( &md_ctx, tmp + 20, nb ); 00340 mbedtls_md_hmac_finish( &md_ctx, tmp ); 00341 00342 for( i = 0; i < dlen; i += 20 ) 00343 { 00344 mbedtls_md_hmac_reset ( &md_ctx ); 00345 mbedtls_md_hmac_update( &md_ctx, tmp, 20 + nb ); 00346 mbedtls_md_hmac_finish( &md_ctx, h_i ); 00347 00348 mbedtls_md_hmac_reset ( &md_ctx ); 00349 mbedtls_md_hmac_update( &md_ctx, tmp, 20 ); 00350 mbedtls_md_hmac_finish( &md_ctx, tmp ); 00351 00352 k = ( i + 20 > dlen ) ? dlen % 20 : 20; 00353 00354 for( j = 0; j < k; j++ ) 00355 dstbuf[i + j] = (unsigned char)( dstbuf[i + j] ^ h_i[j] ); 00356 } 00357 00358 mbedtls_md_free( &md_ctx ); 00359 00360 mbedtls_zeroize( tmp, sizeof( tmp ) ); 00361 mbedtls_zeroize( h_i, sizeof( h_i ) ); 00362 00363 return( 0 ); 00364 } 00365 #endif /* MBEDTLS_SSL_PROTO_TLS1) || MBEDTLS_SSL_PROTO_TLS1_1 */ 00366 00367 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 00368 static int tls_prf_generic( mbedtls_md_type_t md_type, 00369 const unsigned char *secret, size_t slen, 00370 const char *label, 00371 const unsigned char *random, size_t rlen, 00372 unsigned char *dstbuf, size_t dlen ) 00373 { 00374 size_t nb; 00375 size_t i, j, k, md_len; 00376 unsigned char tmp[128]; 00377 unsigned char h_i[MBEDTLS_MD_MAX_SIZE]; 00378 const mbedtls_md_info_t *md_info; 00379 mbedtls_md_context_t md_ctx; 00380 int ret; 00381 00382 mbedtls_md_init( &md_ctx ); 00383 00384 if( ( md_info = mbedtls_md_info_from_type( md_type ) ) == NULL ) 00385 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00386 00387 md_len = mbedtls_md_get_size( md_info ); 00388 00389 if( sizeof( tmp ) < md_len + strlen( label ) + rlen ) 00390 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 00391 00392 nb = strlen( label ); 00393 memcpy( tmp + md_len, label, nb ); 00394 memcpy( tmp + md_len + nb, random, rlen ); 00395 nb += rlen; 00396 00397 /* 00398 * Compute P_<hash>(secret, label + random)[0..dlen] 00399 */ 00400 if ( ( ret = mbedtls_md_setup( &md_ctx, md_info, 1 ) ) != 0 ) 00401 return( ret ); 00402 00403 mbedtls_md_hmac_starts( &md_ctx, secret, slen ); 00404 mbedtls_md_hmac_update( &md_ctx, tmp + md_len, nb ); 00405 mbedtls_md_hmac_finish( &md_ctx, tmp ); 00406 00407 for( i = 0; i < dlen; i += md_len ) 00408 { 00409 mbedtls_md_hmac_reset ( &md_ctx ); 00410 mbedtls_md_hmac_update( &md_ctx, tmp, md_len + nb ); 00411 mbedtls_md_hmac_finish( &md_ctx, h_i ); 00412 00413 mbedtls_md_hmac_reset ( &md_ctx ); 00414 mbedtls_md_hmac_update( &md_ctx, tmp, md_len ); 00415 mbedtls_md_hmac_finish( &md_ctx, tmp ); 00416 00417 k = ( i + md_len > dlen ) ? dlen % md_len : md_len; 00418 00419 for( j = 0; j < k; j++ ) 00420 dstbuf[i + j] = h_i[j]; 00421 } 00422 00423 mbedtls_md_free( &md_ctx ); 00424 00425 mbedtls_zeroize( tmp, sizeof( tmp ) ); 00426 mbedtls_zeroize( h_i, sizeof( h_i ) ); 00427 00428 return( 0 ); 00429 } 00430 00431 #if defined(MBEDTLS_SHA256_C) 00432 static int tls_prf_sha256( const unsigned char *secret, size_t slen, 00433 const char *label, 00434 const unsigned char *random, size_t rlen, 00435 unsigned char *dstbuf, size_t dlen ) 00436 { 00437 return( tls_prf_generic( MBEDTLS_MD_SHA256, secret, slen, 00438 label, random, rlen, dstbuf, dlen ) ); 00439 } 00440 #endif /* MBEDTLS_SHA256_C */ 00441 00442 #if defined(MBEDTLS_SHA512_C) 00443 static int tls_prf_sha384( const unsigned char *secret, size_t slen, 00444 const char *label, 00445 const unsigned char *random, size_t rlen, 00446 unsigned char *dstbuf, size_t dlen ) 00447 { 00448 return( tls_prf_generic( MBEDTLS_MD_SHA384, secret, slen, 00449 label, random, rlen, dstbuf, dlen ) ); 00450 } 00451 #endif /* MBEDTLS_SHA512_C */ 00452 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 00453 00454 static void ssl_update_checksum_start( mbedtls_ssl_context *, const unsigned char *, size_t ); 00455 00456 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 00457 defined(MBEDTLS_SSL_PROTO_TLS1_1) 00458 static void ssl_update_checksum_md5sha1( mbedtls_ssl_context *, const unsigned char *, size_t ); 00459 #endif 00460 00461 #if defined(MBEDTLS_SSL_PROTO_SSL3) 00462 static void ssl_calc_verify_ssl( mbedtls_ssl_context *, unsigned char * ); 00463 static void ssl_calc_finished_ssl( mbedtls_ssl_context *, unsigned char *, int ); 00464 #endif 00465 00466 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) 00467 static void ssl_calc_verify_tls( mbedtls_ssl_context *, unsigned char * ); 00468 static void ssl_calc_finished_tls( mbedtls_ssl_context *, unsigned char *, int ); 00469 #endif 00470 00471 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 00472 #if defined(MBEDTLS_SHA256_C) 00473 static void ssl_update_checksum_sha256( mbedtls_ssl_context *, const unsigned char *, size_t ); 00474 static void ssl_calc_verify_tls_sha256( mbedtls_ssl_context *,unsigned char * ); 00475 static void ssl_calc_finished_tls_sha256( mbedtls_ssl_context *,unsigned char *, int ); 00476 #endif 00477 00478 #if defined(MBEDTLS_SHA512_C) 00479 static void ssl_update_checksum_sha384( mbedtls_ssl_context *, const unsigned char *, size_t ); 00480 static void ssl_calc_verify_tls_sha384( mbedtls_ssl_context *, unsigned char * ); 00481 static void ssl_calc_finished_tls_sha384( mbedtls_ssl_context *, unsigned char *, int ); 00482 #endif 00483 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 00484 00485 int mbedtls_ssl_derive_keys( mbedtls_ssl_context *ssl ) 00486 { 00487 int ret = 0; 00488 unsigned char tmp[64]; 00489 unsigned char keyblk[256]; 00490 unsigned char *key1; 00491 unsigned char *key2; 00492 unsigned char *mac_enc; 00493 unsigned char *mac_dec; 00494 size_t iv_copy_len; 00495 const mbedtls_cipher_info_t *cipher_info; 00496 const mbedtls_md_info_t *md_info; 00497 00498 mbedtls_ssl_session *session = ssl->session_negotiate; 00499 mbedtls_ssl_transform *transform = ssl->transform_negotiate; 00500 mbedtls_ssl_handshake_params *handshake = ssl->handshake; 00501 00502 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> derive keys" ) ); 00503 00504 cipher_info = mbedtls_cipher_info_from_type( transform->ciphersuite_info->cipher ); 00505 if( cipher_info == NULL ) 00506 { 00507 MBEDTLS_SSL_DEBUG_MSG( 1, ( "cipher info for %d not found", 00508 transform->ciphersuite_info->cipher ) ); 00509 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 00510 } 00511 00512 md_info = mbedtls_md_info_from_type( transform->ciphersuite_info->mac ); 00513 if( md_info == NULL ) 00514 { 00515 MBEDTLS_SSL_DEBUG_MSG( 1, ( "mbedtls_md info for %d not found", 00516 transform->ciphersuite_info->mac ) ); 00517 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 00518 } 00519 00520 /* 00521 * Set appropriate PRF function and other SSL / TLS / TLS1.2 functions 00522 */ 00523 #if defined(MBEDTLS_SSL_PROTO_SSL3) 00524 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 00525 { 00526 handshake->tls_prf = ssl3_prf; 00527 handshake->calc_verify = ssl_calc_verify_ssl; 00528 handshake->calc_finished = ssl_calc_finished_ssl; 00529 } 00530 else 00531 #endif 00532 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) 00533 if( ssl->minor_ver < MBEDTLS_SSL_MINOR_VERSION_3 ) 00534 { 00535 handshake->tls_prf = tls1_prf; 00536 handshake->calc_verify = ssl_calc_verify_tls; 00537 handshake->calc_finished = ssl_calc_finished_tls; 00538 } 00539 else 00540 #endif 00541 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 00542 #if defined(MBEDTLS_SHA512_C) 00543 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 && 00544 transform->ciphersuite_info->mac == MBEDTLS_MD_SHA384 ) 00545 { 00546 handshake->tls_prf = tls_prf_sha384; 00547 handshake->calc_verify = ssl_calc_verify_tls_sha384; 00548 handshake->calc_finished = ssl_calc_finished_tls_sha384; 00549 } 00550 else 00551 #endif 00552 #if defined(MBEDTLS_SHA256_C) 00553 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 00554 { 00555 handshake->tls_prf = tls_prf_sha256; 00556 handshake->calc_verify = ssl_calc_verify_tls_sha256; 00557 handshake->calc_finished = ssl_calc_finished_tls_sha256; 00558 } 00559 else 00560 #endif 00561 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 00562 { 00563 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 00564 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00565 } 00566 00567 /* 00568 * SSLv3: 00569 * master = 00570 * MD5( premaster + SHA1( 'A' + premaster + randbytes ) ) + 00571 * MD5( premaster + SHA1( 'BB' + premaster + randbytes ) ) + 00572 * MD5( premaster + SHA1( 'CCC' + premaster + randbytes ) ) 00573 * 00574 * TLSv1+: 00575 * master = PRF( premaster, "master secret", randbytes )[0..47] 00576 */ 00577 if( handshake->resume == 0 ) 00578 { 00579 MBEDTLS_SSL_DEBUG_BUF( 3, "premaster secret", handshake->premaster, 00580 handshake->pmslen ); 00581 00582 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 00583 if( ssl->handshake->extended_ms == MBEDTLS_SSL_EXTENDED_MS_ENABLED ) 00584 { 00585 unsigned char session_hash[48]; 00586 size_t hash_len; 00587 00588 MBEDTLS_SSL_DEBUG_MSG( 3, ( "using extended master secret" ) ); 00589 00590 ssl->handshake->calc_verify( ssl, session_hash ); 00591 00592 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 00593 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 00594 { 00595 #if defined(MBEDTLS_SHA512_C) 00596 if( ssl->transform_negotiate->ciphersuite_info->mac == 00597 MBEDTLS_MD_SHA384 ) 00598 { 00599 hash_len = 48; 00600 } 00601 else 00602 #endif 00603 hash_len = 32; 00604 } 00605 else 00606 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 00607 hash_len = 36; 00608 00609 MBEDTLS_SSL_DEBUG_BUF( 3, "session hash", session_hash, hash_len ); 00610 00611 ret = handshake->tls_prf( handshake->premaster, handshake->pmslen, 00612 "extended master secret", 00613 session_hash, hash_len, 00614 session->master, 48 ); 00615 if( ret != 0 ) 00616 { 00617 MBEDTLS_SSL_DEBUG_RET( 1, "prf", ret ); 00618 return( ret ); 00619 } 00620 00621 } 00622 else 00623 #endif 00624 ret = handshake->tls_prf( handshake->premaster, handshake->pmslen, 00625 "master secret", 00626 handshake->randbytes, 64, 00627 session->master, 48 ); 00628 if( ret != 0 ) 00629 { 00630 MBEDTLS_SSL_DEBUG_RET( 1, "prf", ret ); 00631 return( ret ); 00632 } 00633 00634 mbedtls_zeroize( handshake->premaster, sizeof(handshake->premaster) ); 00635 } 00636 else 00637 MBEDTLS_SSL_DEBUG_MSG( 3, ( "no premaster (session resumed)" ) ); 00638 00639 /* 00640 * Swap the client and server random values. 00641 */ 00642 memcpy( tmp, handshake->randbytes, 64 ); 00643 memcpy( handshake->randbytes, tmp + 32, 32 ); 00644 memcpy( handshake->randbytes + 32, tmp, 32 ); 00645 mbedtls_zeroize( tmp, sizeof( tmp ) ); 00646 00647 /* 00648 * SSLv3: 00649 * key block = 00650 * MD5( master + SHA1( 'A' + master + randbytes ) ) + 00651 * MD5( master + SHA1( 'BB' + master + randbytes ) ) + 00652 * MD5( master + SHA1( 'CCC' + master + randbytes ) ) + 00653 * MD5( master + SHA1( 'DDDD' + master + randbytes ) ) + 00654 * ... 00655 * 00656 * TLSv1: 00657 * key block = PRF( master, "key expansion", randbytes ) 00658 */ 00659 ret = handshake->tls_prf( session->master, 48, "key expansion", 00660 handshake->randbytes, 64, keyblk, 256 ); 00661 if( ret != 0 ) 00662 { 00663 MBEDTLS_SSL_DEBUG_RET( 1, "prf", ret ); 00664 return( ret ); 00665 } 00666 00667 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite = %s", 00668 mbedtls_ssl_get_ciphersuite_name( session->ciphersuite ) ) ); 00669 MBEDTLS_SSL_DEBUG_BUF( 3, "master secret", session->master, 48 ); 00670 MBEDTLS_SSL_DEBUG_BUF( 4, "random bytes", handshake->randbytes, 64 ); 00671 MBEDTLS_SSL_DEBUG_BUF( 4, "key block", keyblk, 256 ); 00672 00673 mbedtls_zeroize( handshake->randbytes, sizeof( handshake->randbytes ) ); 00674 00675 /* 00676 * Determine the appropriate key, IV and MAC length. 00677 */ 00678 00679 transform->keylen = cipher_info->key_bitlen / 8; 00680 00681 if( cipher_info->mode == MBEDTLS_MODE_GCM || 00682 cipher_info->mode == MBEDTLS_MODE_CCM ) 00683 { 00684 transform->maclen = 0; 00685 00686 transform->ivlen = 12; 00687 transform->fixed_ivlen = 4; 00688 00689 /* Minimum length is expicit IV + tag */ 00690 transform->minlen = transform->ivlen - transform->fixed_ivlen 00691 + ( transform->ciphersuite_info->flags & 00692 MBEDTLS_CIPHERSUITE_SHORT_TAG ? 8 : 16 ); 00693 } 00694 else 00695 { 00696 /* Initialize HMAC contexts */ 00697 if( ( ret = mbedtls_md_setup( &transform->md_ctx_enc, md_info, 1 ) ) != 0 || 00698 ( ret = mbedtls_md_setup( &transform->md_ctx_dec, md_info, 1 ) ) != 0 ) 00699 { 00700 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_md_setup", ret ); 00701 return( ret ); 00702 } 00703 00704 /* Get MAC length */ 00705 transform->maclen = mbedtls_md_get_size( md_info ); 00706 00707 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 00708 /* 00709 * If HMAC is to be truncated, we shall keep the leftmost bytes, 00710 * (rfc 6066 page 13 or rfc 2104 section 4), 00711 * so we only need to adjust the length here. 00712 */ 00713 if( session->trunc_hmac == MBEDTLS_SSL_TRUNC_HMAC_ENABLED ) 00714 transform->maclen = MBEDTLS_SSL_TRUNCATED_HMAC_LEN; 00715 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 00716 00717 /* IV length */ 00718 transform->ivlen = cipher_info->iv_size; 00719 00720 /* Minimum length */ 00721 if( cipher_info->mode == MBEDTLS_MODE_STREAM ) 00722 transform->minlen = transform->maclen; 00723 else 00724 { 00725 /* 00726 * GenericBlockCipher: 00727 * 1. if EtM is in use: one block plus MAC 00728 * otherwise: * first multiple of blocklen greater than maclen 00729 * 2. IV except for SSL3 and TLS 1.0 00730 */ 00731 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 00732 if( session->encrypt_then_mac == MBEDTLS_SSL_ETM_ENABLED ) 00733 { 00734 transform->minlen = transform->maclen 00735 + cipher_info->block_size; 00736 } 00737 else 00738 #endif 00739 { 00740 transform->minlen = transform->maclen 00741 + cipher_info->block_size 00742 - transform->maclen % cipher_info->block_size; 00743 } 00744 00745 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) 00746 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 || 00747 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_1 ) 00748 ; /* No need to adjust minlen */ 00749 else 00750 #endif 00751 #if defined(MBEDTLS_SSL_PROTO_TLS1_1) || defined(MBEDTLS_SSL_PROTO_TLS1_2) 00752 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_2 || 00753 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 00754 { 00755 transform->minlen += transform->ivlen; 00756 } 00757 else 00758 #endif 00759 { 00760 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 00761 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00762 } 00763 } 00764 } 00765 00766 MBEDTLS_SSL_DEBUG_MSG( 3, ( "keylen: %d, minlen: %d, ivlen: %d, maclen: %d", 00767 transform->keylen, transform->minlen, transform->ivlen, 00768 transform->maclen ) ); 00769 00770 /* 00771 * Finally setup the cipher contexts, IVs and MAC secrets. 00772 */ 00773 #if defined(MBEDTLS_SSL_CLI_C) 00774 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT ) 00775 { 00776 key1 = keyblk + transform->maclen * 2; 00777 key2 = keyblk + transform->maclen * 2 + transform->keylen; 00778 00779 mac_enc = keyblk; 00780 mac_dec = keyblk + transform->maclen; 00781 00782 /* 00783 * This is not used in TLS v1.1. 00784 */ 00785 iv_copy_len = ( transform->fixed_ivlen ) ? 00786 transform->fixed_ivlen : transform->ivlen; 00787 memcpy( transform->iv_enc, key2 + transform->keylen, iv_copy_len ); 00788 memcpy( transform->iv_dec, key2 + transform->keylen + iv_copy_len, 00789 iv_copy_len ); 00790 } 00791 else 00792 #endif /* MBEDTLS_SSL_CLI_C */ 00793 #if defined(MBEDTLS_SSL_SRV_C) 00794 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 00795 { 00796 key1 = keyblk + transform->maclen * 2 + transform->keylen; 00797 key2 = keyblk + transform->maclen * 2; 00798 00799 mac_enc = keyblk + transform->maclen; 00800 mac_dec = keyblk; 00801 00802 /* 00803 * This is not used in TLS v1.1. 00804 */ 00805 iv_copy_len = ( transform->fixed_ivlen ) ? 00806 transform->fixed_ivlen : transform->ivlen; 00807 memcpy( transform->iv_dec, key1 + transform->keylen, iv_copy_len ); 00808 memcpy( transform->iv_enc, key1 + transform->keylen + iv_copy_len, 00809 iv_copy_len ); 00810 } 00811 else 00812 #endif /* MBEDTLS_SSL_SRV_C */ 00813 { 00814 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 00815 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00816 } 00817 00818 #if defined(MBEDTLS_SSL_PROTO_SSL3) 00819 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 00820 { 00821 if( transform->maclen > sizeof transform->mac_enc ) 00822 { 00823 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 00824 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00825 } 00826 00827 memcpy( transform->mac_enc, mac_enc, transform->maclen ); 00828 memcpy( transform->mac_dec, mac_dec, transform->maclen ); 00829 } 00830 else 00831 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 00832 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 00833 defined(MBEDTLS_SSL_PROTO_TLS1_2) 00834 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_1 ) 00835 { 00836 mbedtls_md_hmac_starts( &transform->md_ctx_enc, mac_enc, transform->maclen ); 00837 mbedtls_md_hmac_starts( &transform->md_ctx_dec, mac_dec, transform->maclen ); 00838 } 00839 else 00840 #endif 00841 { 00842 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 00843 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00844 } 00845 00846 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 00847 if( mbedtls_ssl_hw_record_init != NULL ) 00848 { 00849 int ret = 0; 00850 00851 MBEDTLS_SSL_DEBUG_MSG( 2, ( "going for mbedtls_ssl_hw_record_init()" ) ); 00852 00853 if( ( ret = mbedtls_ssl_hw_record_init( ssl, key1, key2, transform->keylen, 00854 transform->iv_enc, transform->iv_dec, 00855 iv_copy_len, 00856 mac_enc, mac_dec, 00857 transform->maclen ) ) != 0 ) 00858 { 00859 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_hw_record_init", ret ); 00860 return( MBEDTLS_ERR_SSL_HW_ACCEL_FAILED ); 00861 } 00862 } 00863 #endif /* MBEDTLS_SSL_HW_RECORD_ACCEL */ 00864 00865 #if defined(MBEDTLS_SSL_EXPORT_KEYS) 00866 if( ssl->conf->f_export_keys != NULL ) 00867 { 00868 ssl->conf->f_export_keys( ssl->conf->p_export_keys, 00869 session->master, keyblk, 00870 transform->maclen, transform->keylen, 00871 iv_copy_len ); 00872 } 00873 #endif 00874 00875 if( ( ret = mbedtls_cipher_setup( &transform->cipher_ctx_enc, 00876 cipher_info ) ) != 0 ) 00877 { 00878 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_setup", ret ); 00879 return( ret ); 00880 } 00881 00882 if( ( ret = mbedtls_cipher_setup( &transform->cipher_ctx_dec, 00883 cipher_info ) ) != 0 ) 00884 { 00885 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_setup", ret ); 00886 return( ret ); 00887 } 00888 00889 if( ( ret = mbedtls_cipher_setkey( &transform->cipher_ctx_enc, key1, 00890 cipher_info->key_bitlen, 00891 MBEDTLS_ENCRYPT ) ) != 0 ) 00892 { 00893 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_setkey", ret ); 00894 return( ret ); 00895 } 00896 00897 if( ( ret = mbedtls_cipher_setkey( &transform->cipher_ctx_dec, key2, 00898 cipher_info->key_bitlen, 00899 MBEDTLS_DECRYPT ) ) != 0 ) 00900 { 00901 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_setkey", ret ); 00902 return( ret ); 00903 } 00904 00905 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00906 if( cipher_info->mode == MBEDTLS_MODE_CBC ) 00907 { 00908 if( ( ret = mbedtls_cipher_set_padding_mode( &transform->cipher_ctx_enc, 00909 MBEDTLS_PADDING_NONE ) ) != 0 ) 00910 { 00911 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_set_padding_mode", ret ); 00912 return( ret ); 00913 } 00914 00915 if( ( ret = mbedtls_cipher_set_padding_mode( &transform->cipher_ctx_dec, 00916 MBEDTLS_PADDING_NONE ) ) != 0 ) 00917 { 00918 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_set_padding_mode", ret ); 00919 return( ret ); 00920 } 00921 } 00922 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00923 00924 mbedtls_zeroize( keyblk, sizeof( keyblk ) ); 00925 00926 #if defined(MBEDTLS_ZLIB_SUPPORT) 00927 // Initialize compression 00928 // 00929 if( session->compression == MBEDTLS_SSL_COMPRESS_DEFLATE ) 00930 { 00931 if( ssl->compress_buf == NULL ) 00932 { 00933 MBEDTLS_SSL_DEBUG_MSG( 3, ( "Allocating compression buffer" ) ); 00934 ssl->compress_buf = mbedtls_calloc( 1, MBEDTLS_SSL_BUFFER_LEN ); 00935 if( ssl->compress_buf == NULL ) 00936 { 00937 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc(%d bytes) failed", 00938 MBEDTLS_SSL_BUFFER_LEN ) ); 00939 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 00940 } 00941 } 00942 00943 MBEDTLS_SSL_DEBUG_MSG( 3, ( "Initializing zlib states" ) ); 00944 00945 memset( &transform->ctx_deflate, 0, sizeof( transform->ctx_deflate ) ); 00946 memset( &transform->ctx_inflate, 0, sizeof( transform->ctx_inflate ) ); 00947 00948 if( deflateInit( &transform->ctx_deflate, 00949 Z_DEFAULT_COMPRESSION ) != Z_OK || 00950 inflateInit( &transform->ctx_inflate ) != Z_OK ) 00951 { 00952 MBEDTLS_SSL_DEBUG_MSG( 1, ( "Failed to initialize compression" ) ); 00953 return( MBEDTLS_ERR_SSL_COMPRESSION_FAILED ); 00954 } 00955 } 00956 #endif /* MBEDTLS_ZLIB_SUPPORT */ 00957 00958 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= derive keys" ) ); 00959 00960 return( 0 ); 00961 } 00962 00963 #if defined(MBEDTLS_SSL_PROTO_SSL3) 00964 void ssl_calc_verify_ssl( mbedtls_ssl_context *ssl, unsigned char hash[36] ) 00965 { 00966 mbedtls_md5_context md5; 00967 mbedtls_sha1_context sha1; 00968 unsigned char pad_1[48]; 00969 unsigned char pad_2[48]; 00970 00971 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc verify ssl" ) ); 00972 00973 mbedtls_md5_init( &md5 ); 00974 mbedtls_sha1_init( &sha1 ); 00975 00976 mbedtls_md5_clone( &md5, &ssl->handshake->fin_md5 ); 00977 mbedtls_sha1_clone( &sha1, &ssl->handshake->fin_sha1 ); 00978 00979 memset( pad_1, 0x36, 48 ); 00980 memset( pad_2, 0x5C, 48 ); 00981 00982 mbedtls_md5_update( &md5, ssl->session_negotiate->master, 48 ); 00983 mbedtls_md5_update( &md5, pad_1, 48 ); 00984 mbedtls_md5_finish( &md5, hash ); 00985 00986 mbedtls_md5_starts( &md5 ); 00987 mbedtls_md5_update( &md5, ssl->session_negotiate->master, 48 ); 00988 mbedtls_md5_update( &md5, pad_2, 48 ); 00989 mbedtls_md5_update( &md5, hash, 16 ); 00990 mbedtls_md5_finish( &md5, hash ); 00991 00992 mbedtls_sha1_update( &sha1, ssl->session_negotiate->master, 48 ); 00993 mbedtls_sha1_update( &sha1, pad_1, 40 ); 00994 mbedtls_sha1_finish( &sha1, hash + 16 ); 00995 00996 mbedtls_sha1_starts( &sha1 ); 00997 mbedtls_sha1_update( &sha1, ssl->session_negotiate->master, 48 ); 00998 mbedtls_sha1_update( &sha1, pad_2, 40 ); 00999 mbedtls_sha1_update( &sha1, hash + 16, 20 ); 01000 mbedtls_sha1_finish( &sha1, hash + 16 ); 01001 01002 MBEDTLS_SSL_DEBUG_BUF( 3, "calculated verify result", hash, 36 ); 01003 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc verify" ) ); 01004 01005 mbedtls_md5_free( &md5 ); 01006 mbedtls_sha1_free( &sha1 ); 01007 01008 return; 01009 } 01010 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 01011 01012 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) 01013 void ssl_calc_verify_tls( mbedtls_ssl_context *ssl, unsigned char hash[36] ) 01014 { 01015 mbedtls_md5_context md5; 01016 mbedtls_sha1_context sha1; 01017 01018 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc verify tls" ) ); 01019 01020 mbedtls_md5_init( &md5 ); 01021 mbedtls_sha1_init( &sha1 ); 01022 01023 mbedtls_md5_clone( &md5, &ssl->handshake->fin_md5 ); 01024 mbedtls_sha1_clone( &sha1, &ssl->handshake->fin_sha1 ); 01025 01026 mbedtls_md5_finish( &md5, hash ); 01027 mbedtls_sha1_finish( &sha1, hash + 16 ); 01028 01029 MBEDTLS_SSL_DEBUG_BUF( 3, "calculated verify result", hash, 36 ); 01030 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc verify" ) ); 01031 01032 mbedtls_md5_free( &md5 ); 01033 mbedtls_sha1_free( &sha1 ); 01034 01035 return; 01036 } 01037 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 */ 01038 01039 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 01040 #if defined(MBEDTLS_SHA256_C) 01041 void ssl_calc_verify_tls_sha256( mbedtls_ssl_context *ssl, unsigned char hash[32] ) 01042 { 01043 mbedtls_sha256_context sha256; 01044 01045 mbedtls_sha256_init( &sha256 ); 01046 01047 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc verify sha256" ) ); 01048 01049 mbedtls_sha256_clone( &sha256, &ssl->handshake->fin_sha256 ); 01050 mbedtls_sha256_finish( &sha256, hash ); 01051 01052 MBEDTLS_SSL_DEBUG_BUF( 3, "calculated verify result", hash, 32 ); 01053 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc verify" ) ); 01054 01055 mbedtls_sha256_free( &sha256 ); 01056 01057 return; 01058 } 01059 #endif /* MBEDTLS_SHA256_C */ 01060 01061 #if defined(MBEDTLS_SHA512_C) 01062 void ssl_calc_verify_tls_sha384( mbedtls_ssl_context *ssl, unsigned char hash[48] ) 01063 { 01064 mbedtls_sha512_context sha512; 01065 01066 mbedtls_sha512_init( &sha512 ); 01067 01068 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc verify sha384" ) ); 01069 01070 mbedtls_sha512_clone( &sha512, &ssl->handshake->fin_sha512 ); 01071 mbedtls_sha512_finish( &sha512, hash ); 01072 01073 MBEDTLS_SSL_DEBUG_BUF( 3, "calculated verify result", hash, 48 ); 01074 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc verify" ) ); 01075 01076 mbedtls_sha512_free( &sha512 ); 01077 01078 return; 01079 } 01080 #endif /* MBEDTLS_SHA512_C */ 01081 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 01082 01083 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 01084 int mbedtls_ssl_psk_derive_premaster( mbedtls_ssl_context *ssl, mbedtls_key_exchange_type_t key_ex ) 01085 { 01086 unsigned char *p = ssl->handshake->premaster; 01087 unsigned char *end = p + sizeof( ssl->handshake->premaster ); 01088 const unsigned char *psk = ssl->conf->psk; 01089 size_t psk_len = ssl->conf->psk_len; 01090 01091 /* If the psk callback was called, use its result */ 01092 if( ssl->handshake->psk != NULL ) 01093 { 01094 psk = ssl->handshake->psk; 01095 psk_len = ssl->handshake->psk_len; 01096 } 01097 01098 /* 01099 * PMS = struct { 01100 * opaque other_secret<0..2^16-1>; 01101 * opaque psk<0..2^16-1>; 01102 * }; 01103 * with "other_secret" depending on the particular key exchange 01104 */ 01105 #if defined(MBEDTLS_KEY_EXCHANGE_PSK_ENABLED) 01106 if( key_ex == MBEDTLS_KEY_EXCHANGE_PSK ) 01107 { 01108 if( end - p < 2 ) 01109 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 01110 01111 *(p++) = (unsigned char)( psk_len >> 8 ); 01112 *(p++) = (unsigned char)( psk_len ); 01113 01114 if( end < p || (size_t)( end - p ) < psk_len ) 01115 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 01116 01117 memset( p, 0, psk_len ); 01118 p += psk_len; 01119 } 01120 else 01121 #endif /* MBEDTLS_KEY_EXCHANGE_PSK_ENABLED */ 01122 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 01123 if( key_ex == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 01124 { 01125 /* 01126 * other_secret already set by the ClientKeyExchange message, 01127 * and is 48 bytes long 01128 */ 01129 *p++ = 0; 01130 *p++ = 48; 01131 p += 48; 01132 } 01133 else 01134 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED */ 01135 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 01136 if( key_ex == MBEDTLS_KEY_EXCHANGE_DHE_PSK ) 01137 { 01138 int ret; 01139 size_t len; 01140 01141 /* Write length only when we know the actual value */ 01142 if( ( ret = mbedtls_dhm_calc_secret( &ssl->handshake->dhm_ctx, 01143 p + 2, end - ( p + 2 ), &len, 01144 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 01145 { 01146 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_calc_secret", ret ); 01147 return( ret ); 01148 } 01149 *(p++) = (unsigned char)( len >> 8 ); 01150 *(p++) = (unsigned char)( len ); 01151 p += len; 01152 01153 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: K ", &ssl->handshake->dhm_ctx.K ); 01154 } 01155 else 01156 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 01157 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 01158 if( key_ex == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 01159 { 01160 int ret; 01161 size_t zlen; 01162 01163 if( ( ret = mbedtls_ecdh_calc_secret( &ssl->handshake->ecdh_ctx, &zlen, 01164 p + 2, end - ( p + 2 ), 01165 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 01166 { 01167 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_calc_secret", ret ); 01168 return( ret ); 01169 } 01170 01171 *(p++) = (unsigned char)( zlen >> 8 ); 01172 *(p++) = (unsigned char)( zlen ); 01173 p += zlen; 01174 01175 MBEDTLS_SSL_DEBUG_MPI( 3, "ECDH: z", &ssl->handshake->ecdh_ctx.z ); 01176 } 01177 else 01178 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED */ 01179 { 01180 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01181 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01182 } 01183 01184 /* opaque psk<0..2^16-1>; */ 01185 if( end - p < 2 ) 01186 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 01187 01188 *(p++) = (unsigned char)( psk_len >> 8 ); 01189 *(p++) = (unsigned char)( psk_len ); 01190 01191 if( end < p || (size_t)( end - p ) < psk_len ) 01192 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 01193 01194 memcpy( p, psk, psk_len ); 01195 p += psk_len; 01196 01197 ssl->handshake->pmslen = p - ssl->handshake->premaster; 01198 01199 return( 0 ); 01200 } 01201 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED */ 01202 01203 #if defined(MBEDTLS_SSL_PROTO_SSL3) 01204 /* 01205 * SSLv3.0 MAC functions 01206 */ 01207 static void ssl_mac( mbedtls_md_context_t *md_ctx, unsigned char *secret, 01208 unsigned char *buf, size_t len, 01209 unsigned char *ctr, int type ) 01210 { 01211 unsigned char header[11]; 01212 unsigned char padding[48]; 01213 int padlen; 01214 int md_size = mbedtls_md_get_size( md_ctx->md_info ); 01215 int md_type = mbedtls_md_get_type( md_ctx->md_info ); 01216 01217 /* Only MD5 and SHA-1 supported */ 01218 if( md_type == MBEDTLS_MD_MD5 ) 01219 padlen = 48; 01220 else 01221 padlen = 40; 01222 01223 memcpy( header, ctr, 8 ); 01224 header[ 8] = (unsigned char) type; 01225 header[ 9] = (unsigned char)( len >> 8 ); 01226 header[10] = (unsigned char)( len ); 01227 01228 memset( padding, 0x36, padlen ); 01229 mbedtls_md_starts( md_ctx ); 01230 mbedtls_md_update( md_ctx, secret, md_size ); 01231 mbedtls_md_update( md_ctx, padding, padlen ); 01232 mbedtls_md_update( md_ctx, header, 11 ); 01233 mbedtls_md_update( md_ctx, buf, len ); 01234 mbedtls_md_finish( md_ctx, buf + len ); 01235 01236 memset( padding, 0x5C, padlen ); 01237 mbedtls_md_starts( md_ctx ); 01238 mbedtls_md_update( md_ctx, secret, md_size ); 01239 mbedtls_md_update( md_ctx, padding, padlen ); 01240 mbedtls_md_update( md_ctx, buf + len, md_size ); 01241 mbedtls_md_finish( md_ctx, buf + len ); 01242 } 01243 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 01244 01245 #if defined(MBEDTLS_ARC4_C) || defined(MBEDTLS_CIPHER_NULL_CIPHER) || \ 01246 ( defined(MBEDTLS_CIPHER_MODE_CBC) && \ 01247 ( defined(MBEDTLS_AES_C) || defined(MBEDTLS_CAMELLIA_C) ) ) 01248 #define SSL_SOME_MODES_USE_MAC 01249 #endif 01250 01251 /* 01252 * Encryption/decryption functions 01253 */ 01254 static int ssl_encrypt_buf( mbedtls_ssl_context *ssl ) 01255 { 01256 mbedtls_cipher_mode_t mode; 01257 int auth_done = 0; 01258 01259 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> encrypt buf" ) ); 01260 01261 if( ssl->session_out == NULL || ssl->transform_out == NULL ) 01262 { 01263 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01264 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01265 } 01266 01267 mode = mbedtls_cipher_get_cipher_mode( &ssl->transform_out->cipher_ctx_enc ); 01268 01269 MBEDTLS_SSL_DEBUG_BUF( 4, "before encrypt: output payload", 01270 ssl->out_msg, ssl->out_msglen ); 01271 01272 /* 01273 * Add MAC before if needed 01274 */ 01275 #if defined(SSL_SOME_MODES_USE_MAC) 01276 if( mode == MBEDTLS_MODE_STREAM || 01277 ( mode == MBEDTLS_MODE_CBC 01278 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01279 && ssl->session_out->encrypt_then_mac == MBEDTLS_SSL_ETM_DISABLED 01280 #endif 01281 ) ) 01282 { 01283 #if defined(MBEDTLS_SSL_PROTO_SSL3) 01284 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 01285 { 01286 ssl_mac( &ssl->transform_out->md_ctx_enc, 01287 ssl->transform_out->mac_enc, 01288 ssl->out_msg, ssl->out_msglen, 01289 ssl->out_ctr, ssl->out_msgtype ); 01290 } 01291 else 01292 #endif 01293 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 01294 defined(MBEDTLS_SSL_PROTO_TLS1_2) 01295 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_1 ) 01296 { 01297 mbedtls_md_hmac_update( &ssl->transform_out->md_ctx_enc, ssl->out_ctr, 8 ); 01298 mbedtls_md_hmac_update( &ssl->transform_out->md_ctx_enc, ssl->out_hdr, 3 ); 01299 mbedtls_md_hmac_update( &ssl->transform_out->md_ctx_enc, ssl->out_len, 2 ); 01300 mbedtls_md_hmac_update( &ssl->transform_out->md_ctx_enc, 01301 ssl->out_msg, ssl->out_msglen ); 01302 mbedtls_md_hmac_finish( &ssl->transform_out->md_ctx_enc, 01303 ssl->out_msg + ssl->out_msglen ); 01304 mbedtls_md_hmac_reset( &ssl->transform_out->md_ctx_enc ); 01305 } 01306 else 01307 #endif 01308 { 01309 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01310 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01311 } 01312 01313 MBEDTLS_SSL_DEBUG_BUF( 4, "computed mac", 01314 ssl->out_msg + ssl->out_msglen, 01315 ssl->transform_out->maclen ); 01316 01317 ssl->out_msglen += ssl->transform_out->maclen; 01318 auth_done++; 01319 } 01320 #endif /* AEAD not the only option */ 01321 01322 /* 01323 * Encrypt 01324 */ 01325 #if defined(MBEDTLS_ARC4_C) || defined(MBEDTLS_CIPHER_NULL_CIPHER) 01326 if( mode == MBEDTLS_MODE_STREAM ) 01327 { 01328 int ret; 01329 size_t olen = 0; 01330 01331 MBEDTLS_SSL_DEBUG_MSG( 3, ( "before encrypt: msglen = %d, " 01332 "including %d bytes of padding", 01333 ssl->out_msglen, 0 ) ); 01334 01335 if( ( ret = mbedtls_cipher_crypt( &ssl->transform_out->cipher_ctx_enc, 01336 ssl->transform_out->iv_enc, 01337 ssl->transform_out->ivlen, 01338 ssl->out_msg, ssl->out_msglen, 01339 ssl->out_msg, &olen ) ) != 0 ) 01340 { 01341 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_crypt", ret ); 01342 return( ret ); 01343 } 01344 01345 if( ssl->out_msglen != olen ) 01346 { 01347 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01348 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01349 } 01350 } 01351 else 01352 #endif /* MBEDTLS_ARC4_C || MBEDTLS_CIPHER_NULL_CIPHER */ 01353 #if defined(MBEDTLS_GCM_C) || defined(MBEDTLS_CCM_C) 01354 if( mode == MBEDTLS_MODE_GCM || 01355 mode == MBEDTLS_MODE_CCM ) 01356 { 01357 int ret; 01358 size_t enc_msglen, olen; 01359 unsigned char *enc_msg; 01360 unsigned char add_data[13]; 01361 unsigned char taglen = ssl->transform_out->ciphersuite_info->flags & 01362 MBEDTLS_CIPHERSUITE_SHORT_TAG ? 8 : 16; 01363 01364 memcpy( add_data, ssl->out_ctr, 8 ); 01365 add_data[8] = ssl->out_msgtype; 01366 mbedtls_ssl_write_version( ssl->major_ver, ssl->minor_ver, 01367 ssl->conf->transport, add_data + 9 ); 01368 add_data[11] = ( ssl->out_msglen >> 8 ) & 0xFF; 01369 add_data[12] = ssl->out_msglen & 0xFF; 01370 01371 MBEDTLS_SSL_DEBUG_BUF( 4, "additional data used for AEAD", 01372 add_data, 13 ); 01373 01374 /* 01375 * Generate IV 01376 */ 01377 #if defined(MBEDTLS_SSL_AEAD_RANDOM_IV) 01378 ret = ssl->conf->f_rng( ssl->conf->p_rng, 01379 ssl->transform_out->iv_enc + ssl->transform_out->fixed_ivlen, 01380 ssl->transform_out->ivlen - ssl->transform_out->fixed_ivlen ); 01381 if( ret != 0 ) 01382 return( ret ); 01383 01384 memcpy( ssl->out_iv, 01385 ssl->transform_out->iv_enc + ssl->transform_out->fixed_ivlen, 01386 ssl->transform_out->ivlen - ssl->transform_out->fixed_ivlen ); 01387 #else 01388 if( ssl->transform_out->ivlen - ssl->transform_out->fixed_ivlen != 8 ) 01389 { 01390 /* Reminder if we ever add an AEAD mode with a different size */ 01391 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01392 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01393 } 01394 01395 memcpy( ssl->transform_out->iv_enc + ssl->transform_out->fixed_ivlen, 01396 ssl->out_ctr, 8 ); 01397 memcpy( ssl->out_iv, ssl->out_ctr, 8 ); 01398 #endif 01399 01400 MBEDTLS_SSL_DEBUG_BUF( 4, "IV used", ssl->out_iv, 01401 ssl->transform_out->ivlen - ssl->transform_out->fixed_ivlen ); 01402 01403 /* 01404 * Fix pointer positions and message length with added IV 01405 */ 01406 enc_msg = ssl->out_msg; 01407 enc_msglen = ssl->out_msglen; 01408 ssl->out_msglen += ssl->transform_out->ivlen - 01409 ssl->transform_out->fixed_ivlen; 01410 01411 MBEDTLS_SSL_DEBUG_MSG( 3, ( "before encrypt: msglen = %d, " 01412 "including %d bytes of padding", 01413 ssl->out_msglen, 0 ) ); 01414 01415 /* 01416 * Encrypt and authenticate 01417 */ 01418 if( ( ret = mbedtls_cipher_auth_encrypt( &ssl->transform_out->cipher_ctx_enc, 01419 ssl->transform_out->iv_enc, 01420 ssl->transform_out->ivlen, 01421 add_data, 13, 01422 enc_msg, enc_msglen, 01423 enc_msg, &olen, 01424 enc_msg + enc_msglen, taglen ) ) != 0 ) 01425 { 01426 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_auth_encrypt", ret ); 01427 return( ret ); 01428 } 01429 01430 if( olen != enc_msglen ) 01431 { 01432 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01433 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01434 } 01435 01436 ssl->out_msglen += taglen; 01437 auth_done++; 01438 01439 MBEDTLS_SSL_DEBUG_BUF( 4, "after encrypt: tag", enc_msg + enc_msglen, taglen ); 01440 } 01441 else 01442 #endif /* MBEDTLS_GCM_C || MBEDTLS_CCM_C */ 01443 #if defined(MBEDTLS_CIPHER_MODE_CBC) && \ 01444 ( defined(MBEDTLS_AES_C) || defined(MBEDTLS_CAMELLIA_C) ) 01445 if( mode == MBEDTLS_MODE_CBC ) 01446 { 01447 int ret; 01448 unsigned char *enc_msg; 01449 size_t enc_msglen, padlen, olen = 0, i; 01450 01451 padlen = ssl->transform_out->ivlen - ( ssl->out_msglen + 1 ) % 01452 ssl->transform_out->ivlen; 01453 if( padlen == ssl->transform_out->ivlen ) 01454 padlen = 0; 01455 01456 for( i = 0; i <= padlen; i++ ) 01457 ssl->out_msg[ssl->out_msglen + i] = (unsigned char) padlen; 01458 01459 ssl->out_msglen += padlen + 1; 01460 01461 enc_msglen = ssl->out_msglen; 01462 enc_msg = ssl->out_msg; 01463 01464 #if defined(MBEDTLS_SSL_PROTO_TLS1_1) || defined(MBEDTLS_SSL_PROTO_TLS1_2) 01465 /* 01466 * Prepend per-record IV for block cipher in TLS v1.1 and up as per 01467 * Method 1 (6.2.3.2. in RFC4346 and RFC5246) 01468 */ 01469 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_2 ) 01470 { 01471 /* 01472 * Generate IV 01473 */ 01474 ret = ssl->conf->f_rng( ssl->conf->p_rng, ssl->transform_out->iv_enc, 01475 ssl->transform_out->ivlen ); 01476 if( ret != 0 ) 01477 return( ret ); 01478 01479 memcpy( ssl->out_iv, ssl->transform_out->iv_enc, 01480 ssl->transform_out->ivlen ); 01481 01482 /* 01483 * Fix pointer positions and message length with added IV 01484 */ 01485 enc_msg = ssl->out_msg; 01486 enc_msglen = ssl->out_msglen; 01487 ssl->out_msglen += ssl->transform_out->ivlen; 01488 } 01489 #endif /* MBEDTLS_SSL_PROTO_TLS1_1 || MBEDTLS_SSL_PROTO_TLS1_2 */ 01490 01491 MBEDTLS_SSL_DEBUG_MSG( 3, ( "before encrypt: msglen = %d, " 01492 "including %d bytes of IV and %d bytes of padding", 01493 ssl->out_msglen, ssl->transform_out->ivlen, 01494 padlen + 1 ) ); 01495 01496 if( ( ret = mbedtls_cipher_crypt( &ssl->transform_out->cipher_ctx_enc, 01497 ssl->transform_out->iv_enc, 01498 ssl->transform_out->ivlen, 01499 enc_msg, enc_msglen, 01500 enc_msg, &olen ) ) != 0 ) 01501 { 01502 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_crypt", ret ); 01503 return( ret ); 01504 } 01505 01506 if( enc_msglen != olen ) 01507 { 01508 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01509 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01510 } 01511 01512 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) 01513 if( ssl->minor_ver < MBEDTLS_SSL_MINOR_VERSION_2 ) 01514 { 01515 /* 01516 * Save IV in SSL3 and TLS1 01517 */ 01518 memcpy( ssl->transform_out->iv_enc, 01519 ssl->transform_out->cipher_ctx_enc.iv, 01520 ssl->transform_out->ivlen ); 01521 } 01522 #endif 01523 01524 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01525 if( auth_done == 0 ) 01526 { 01527 /* 01528 * MAC(MAC_write_key, seq_num + 01529 * TLSCipherText.type + 01530 * TLSCipherText.version + 01531 * length_of( (IV +) ENC(...) ) + 01532 * IV + // except for TLS 1.0 01533 * ENC(content + padding + padding_length)); 01534 */ 01535 unsigned char pseudo_hdr[13]; 01536 01537 MBEDTLS_SSL_DEBUG_MSG( 3, ( "using encrypt then mac" ) ); 01538 01539 memcpy( pseudo_hdr + 0, ssl->out_ctr, 8 ); 01540 memcpy( pseudo_hdr + 8, ssl->out_hdr, 3 ); 01541 pseudo_hdr[11] = (unsigned char)( ( ssl->out_msglen >> 8 ) & 0xFF ); 01542 pseudo_hdr[12] = (unsigned char)( ( ssl->out_msglen ) & 0xFF ); 01543 01544 MBEDTLS_SSL_DEBUG_BUF( 4, "MAC'd meta-data", pseudo_hdr, 13 ); 01545 01546 mbedtls_md_hmac_update( &ssl->transform_out->md_ctx_enc, pseudo_hdr, 13 ); 01547 mbedtls_md_hmac_update( &ssl->transform_out->md_ctx_enc, 01548 ssl->out_iv, ssl->out_msglen ); 01549 mbedtls_md_hmac_finish( &ssl->transform_out->md_ctx_enc, 01550 ssl->out_iv + ssl->out_msglen ); 01551 mbedtls_md_hmac_reset( &ssl->transform_out->md_ctx_enc ); 01552 01553 ssl->out_msglen += ssl->transform_out->maclen; 01554 auth_done++; 01555 } 01556 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 01557 } 01558 else 01559 #endif /* MBEDTLS_CIPHER_MODE_CBC && 01560 ( MBEDTLS_AES_C || MBEDTLS_CAMELLIA_C ) */ 01561 { 01562 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01563 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01564 } 01565 01566 /* Make extra sure authentication was performed, exactly once */ 01567 if( auth_done != 1 ) 01568 { 01569 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01570 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01571 } 01572 01573 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= encrypt buf" ) ); 01574 01575 return( 0 ); 01576 } 01577 01578 #define SSL_MAX_MAC_SIZE 48 01579 01580 static int ssl_decrypt_buf( mbedtls_ssl_context *ssl ) 01581 { 01582 size_t i; 01583 mbedtls_cipher_mode_t mode; 01584 int auth_done = 0; 01585 #if defined(SSL_SOME_MODES_USE_MAC) 01586 size_t padlen = 0, correct = 1; 01587 #endif 01588 01589 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> decrypt buf" ) ); 01590 01591 if( ssl->session_in == NULL || ssl->transform_in == NULL ) 01592 { 01593 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01594 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01595 } 01596 01597 mode = mbedtls_cipher_get_cipher_mode( &ssl->transform_in->cipher_ctx_dec ); 01598 01599 if( ssl->in_msglen < ssl->transform_in->minlen ) 01600 { 01601 MBEDTLS_SSL_DEBUG_MSG( 1, ( "in_msglen (%d) < minlen (%d)", 01602 ssl->in_msglen, ssl->transform_in->minlen ) ); 01603 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 01604 } 01605 01606 #if defined(MBEDTLS_ARC4_C) || defined(MBEDTLS_CIPHER_NULL_CIPHER) 01607 if( mode == MBEDTLS_MODE_STREAM ) 01608 { 01609 int ret; 01610 size_t olen = 0; 01611 01612 padlen = 0; 01613 01614 if( ( ret = mbedtls_cipher_crypt( &ssl->transform_in->cipher_ctx_dec, 01615 ssl->transform_in->iv_dec, 01616 ssl->transform_in->ivlen, 01617 ssl->in_msg, ssl->in_msglen, 01618 ssl->in_msg, &olen ) ) != 0 ) 01619 { 01620 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_crypt", ret ); 01621 return( ret ); 01622 } 01623 01624 if( ssl->in_msglen != olen ) 01625 { 01626 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01627 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01628 } 01629 } 01630 else 01631 #endif /* MBEDTLS_ARC4_C || MBEDTLS_CIPHER_NULL_CIPHER */ 01632 #if defined(MBEDTLS_GCM_C) || defined(MBEDTLS_CCM_C) 01633 if( mode == MBEDTLS_MODE_GCM || 01634 mode == MBEDTLS_MODE_CCM ) 01635 { 01636 int ret; 01637 size_t dec_msglen, olen; 01638 unsigned char *dec_msg; 01639 unsigned char *dec_msg_result; 01640 unsigned char add_data[13]; 01641 unsigned char taglen = ssl->transform_in->ciphersuite_info->flags & 01642 MBEDTLS_CIPHERSUITE_SHORT_TAG ? 8 : 16; 01643 size_t explicit_iv_len = ssl->transform_in->ivlen - 01644 ssl->transform_in->fixed_ivlen; 01645 01646 if( ssl->in_msglen < explicit_iv_len + taglen ) 01647 { 01648 MBEDTLS_SSL_DEBUG_MSG( 1, ( "msglen (%d) < explicit_iv_len (%d) " 01649 "+ taglen (%d)", ssl->in_msglen, 01650 explicit_iv_len, taglen ) ); 01651 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 01652 } 01653 dec_msglen = ssl->in_msglen - explicit_iv_len - taglen; 01654 01655 dec_msg = ssl->in_msg; 01656 dec_msg_result = ssl->in_msg; 01657 ssl->in_msglen = dec_msglen; 01658 01659 memcpy( add_data, ssl->in_ctr, 8 ); 01660 add_data[8] = ssl->in_msgtype; 01661 mbedtls_ssl_write_version( ssl->major_ver, ssl->minor_ver, 01662 ssl->conf->transport, add_data + 9 ); 01663 add_data[11] = ( ssl->in_msglen >> 8 ) & 0xFF; 01664 add_data[12] = ssl->in_msglen & 0xFF; 01665 01666 MBEDTLS_SSL_DEBUG_BUF( 4, "additional data used for AEAD", 01667 add_data, 13 ); 01668 01669 memcpy( ssl->transform_in->iv_dec + ssl->transform_in->fixed_ivlen, 01670 ssl->in_iv, 01671 ssl->transform_in->ivlen - ssl->transform_in->fixed_ivlen ); 01672 01673 MBEDTLS_SSL_DEBUG_BUF( 4, "IV used", ssl->transform_in->iv_dec, 01674 ssl->transform_in->ivlen ); 01675 MBEDTLS_SSL_DEBUG_BUF( 4, "TAG used", dec_msg + dec_msglen, taglen ); 01676 01677 /* 01678 * Decrypt and authenticate 01679 */ 01680 if( ( ret = mbedtls_cipher_auth_decrypt( &ssl->transform_in->cipher_ctx_dec, 01681 ssl->transform_in->iv_dec, 01682 ssl->transform_in->ivlen, 01683 add_data, 13, 01684 dec_msg, dec_msglen, 01685 dec_msg_result, &olen, 01686 dec_msg + dec_msglen, taglen ) ) != 0 ) 01687 { 01688 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_auth_decrypt", ret ); 01689 01690 if( ret == MBEDTLS_ERR_CIPHER_AUTH_FAILED ) 01691 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 01692 01693 return( ret ); 01694 } 01695 auth_done++; 01696 01697 if( olen != dec_msglen ) 01698 { 01699 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01700 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01701 } 01702 } 01703 else 01704 #endif /* MBEDTLS_GCM_C || MBEDTLS_CCM_C */ 01705 #if defined(MBEDTLS_CIPHER_MODE_CBC) && \ 01706 ( defined(MBEDTLS_AES_C) || defined(MBEDTLS_CAMELLIA_C) ) 01707 if( mode == MBEDTLS_MODE_CBC ) 01708 { 01709 /* 01710 * Decrypt and check the padding 01711 */ 01712 int ret; 01713 unsigned char *dec_msg; 01714 unsigned char *dec_msg_result; 01715 size_t dec_msglen; 01716 size_t minlen = 0; 01717 size_t olen = 0; 01718 01719 /* 01720 * Check immediate ciphertext sanity 01721 */ 01722 #if defined(MBEDTLS_SSL_PROTO_TLS1_1) || defined(MBEDTLS_SSL_PROTO_TLS1_2) 01723 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_2 ) 01724 minlen += ssl->transform_in->ivlen; 01725 #endif 01726 01727 if( ssl->in_msglen < minlen + ssl->transform_in->ivlen || 01728 ssl->in_msglen < minlen + ssl->transform_in->maclen + 1 ) 01729 { 01730 MBEDTLS_SSL_DEBUG_MSG( 1, ( "msglen (%d) < max( ivlen(%d), maclen (%d) " 01731 "+ 1 ) ( + expl IV )", ssl->in_msglen, 01732 ssl->transform_in->ivlen, 01733 ssl->transform_in->maclen ) ); 01734 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 01735 } 01736 01737 dec_msglen = ssl->in_msglen; 01738 dec_msg = ssl->in_msg; 01739 dec_msg_result = ssl->in_msg; 01740 01741 /* 01742 * Authenticate before decrypt if enabled 01743 */ 01744 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01745 if( ssl->session_in->encrypt_then_mac == MBEDTLS_SSL_ETM_ENABLED ) 01746 { 01747 unsigned char computed_mac[SSL_MAX_MAC_SIZE]; 01748 unsigned char pseudo_hdr[13]; 01749 01750 MBEDTLS_SSL_DEBUG_MSG( 3, ( "using encrypt then mac" ) ); 01751 01752 dec_msglen -= ssl->transform_in->maclen; 01753 ssl->in_msglen -= ssl->transform_in->maclen; 01754 01755 memcpy( pseudo_hdr + 0, ssl->in_ctr, 8 ); 01756 memcpy( pseudo_hdr + 8, ssl->in_hdr, 3 ); 01757 pseudo_hdr[11] = (unsigned char)( ( ssl->in_msglen >> 8 ) & 0xFF ); 01758 pseudo_hdr[12] = (unsigned char)( ( ssl->in_msglen ) & 0xFF ); 01759 01760 MBEDTLS_SSL_DEBUG_BUF( 4, "MAC'd meta-data", pseudo_hdr, 13 ); 01761 01762 mbedtls_md_hmac_update( &ssl->transform_in->md_ctx_dec, pseudo_hdr, 13 ); 01763 mbedtls_md_hmac_update( &ssl->transform_in->md_ctx_dec, 01764 ssl->in_iv, ssl->in_msglen ); 01765 mbedtls_md_hmac_finish( &ssl->transform_in->md_ctx_dec, computed_mac ); 01766 mbedtls_md_hmac_reset( &ssl->transform_in->md_ctx_dec ); 01767 01768 MBEDTLS_SSL_DEBUG_BUF( 4, "message mac", ssl->in_iv + ssl->in_msglen, 01769 ssl->transform_in->maclen ); 01770 MBEDTLS_SSL_DEBUG_BUF( 4, "computed mac", computed_mac, 01771 ssl->transform_in->maclen ); 01772 01773 if( mbedtls_ssl_safer_memcmp( ssl->in_iv + ssl->in_msglen, computed_mac, 01774 ssl->transform_in->maclen ) != 0 ) 01775 { 01776 MBEDTLS_SSL_DEBUG_MSG( 1, ( "message mac does not match" ) ); 01777 01778 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 01779 } 01780 auth_done++; 01781 } 01782 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 01783 01784 /* 01785 * Check length sanity 01786 */ 01787 if( ssl->in_msglen % ssl->transform_in->ivlen != 0 ) 01788 { 01789 MBEDTLS_SSL_DEBUG_MSG( 1, ( "msglen (%d) %% ivlen (%d) != 0", 01790 ssl->in_msglen, ssl->transform_in->ivlen ) ); 01791 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 01792 } 01793 01794 #if defined(MBEDTLS_SSL_PROTO_TLS1_1) || defined(MBEDTLS_SSL_PROTO_TLS1_2) 01795 /* 01796 * Initialize for prepended IV for block cipher in TLS v1.1 and up 01797 */ 01798 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_2 ) 01799 { 01800 dec_msglen -= ssl->transform_in->ivlen; 01801 ssl->in_msglen -= ssl->transform_in->ivlen; 01802 01803 for( i = 0; i < ssl->transform_in->ivlen; i++ ) 01804 ssl->transform_in->iv_dec[i] = ssl->in_iv[i]; 01805 } 01806 #endif /* MBEDTLS_SSL_PROTO_TLS1_1 || MBEDTLS_SSL_PROTO_TLS1_2 */ 01807 01808 if( ( ret = mbedtls_cipher_crypt( &ssl->transform_in->cipher_ctx_dec, 01809 ssl->transform_in->iv_dec, 01810 ssl->transform_in->ivlen, 01811 dec_msg, dec_msglen, 01812 dec_msg_result, &olen ) ) != 0 ) 01813 { 01814 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_cipher_crypt", ret ); 01815 return( ret ); 01816 } 01817 01818 if( dec_msglen != olen ) 01819 { 01820 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01821 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01822 } 01823 01824 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) 01825 if( ssl->minor_ver < MBEDTLS_SSL_MINOR_VERSION_2 ) 01826 { 01827 /* 01828 * Save IV in SSL3 and TLS1 01829 */ 01830 memcpy( ssl->transform_in->iv_dec, 01831 ssl->transform_in->cipher_ctx_dec.iv, 01832 ssl->transform_in->ivlen ); 01833 } 01834 #endif 01835 01836 padlen = 1 + ssl->in_msg[ssl->in_msglen - 1]; 01837 01838 if( ssl->in_msglen < ssl->transform_in->maclen + padlen && 01839 auth_done == 0 ) 01840 { 01841 #if defined(MBEDTLS_SSL_DEBUG_ALL) 01842 MBEDTLS_SSL_DEBUG_MSG( 1, ( "msglen (%d) < maclen (%d) + padlen (%d)", 01843 ssl->in_msglen, ssl->transform_in->maclen, padlen ) ); 01844 #endif 01845 padlen = 0; 01846 correct = 0; 01847 } 01848 01849 #if defined(MBEDTLS_SSL_PROTO_SSL3) 01850 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 01851 { 01852 if( padlen > ssl->transform_in->ivlen ) 01853 { 01854 #if defined(MBEDTLS_SSL_DEBUG_ALL) 01855 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad padding length: is %d, " 01856 "should be no more than %d", 01857 padlen, ssl->transform_in->ivlen ) ); 01858 #endif 01859 correct = 0; 01860 } 01861 } 01862 else 01863 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 01864 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 01865 defined(MBEDTLS_SSL_PROTO_TLS1_2) 01866 if( ssl->minor_ver > MBEDTLS_SSL_MINOR_VERSION_0 ) 01867 { 01868 /* 01869 * TLSv1+: always check the padding up to the first failure 01870 * and fake check up to 256 bytes of padding 01871 */ 01872 size_t pad_count = 0, real_count = 1; 01873 size_t padding_idx = ssl->in_msglen - padlen - 1; 01874 01875 /* 01876 * Padding is guaranteed to be incorrect if: 01877 * 1. padlen >= ssl->in_msglen 01878 * 01879 * 2. padding_idx >= MBEDTLS_SSL_MAX_CONTENT_LEN + 01880 * ssl->transform_in->maclen 01881 * 01882 * In both cases we reset padding_idx to a safe value (0) to 01883 * prevent out-of-buffer reads. 01884 */ 01885 correct &= ( ssl->in_msglen >= padlen + 1 ); 01886 correct &= ( padding_idx < MBEDTLS_SSL_MAX_CONTENT_LEN + 01887 ssl->transform_in->maclen ); 01888 01889 padding_idx *= correct; 01890 01891 for( i = 1; i <= 256; i++ ) 01892 { 01893 real_count &= ( i <= padlen ); 01894 pad_count += real_count * 01895 ( ssl->in_msg[padding_idx + i] == padlen - 1 ); 01896 } 01897 01898 correct &= ( pad_count == padlen ); /* Only 1 on correct padding */ 01899 01900 #if defined(MBEDTLS_SSL_DEBUG_ALL) 01901 if( padlen > 0 && correct == 0 ) 01902 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad padding byte detected" ) ); 01903 #endif 01904 padlen &= correct * 0x1FF; 01905 } 01906 else 01907 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 || \ 01908 MBEDTLS_SSL_PROTO_TLS1_2 */ 01909 { 01910 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01911 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01912 } 01913 01914 ssl->in_msglen -= padlen; 01915 } 01916 else 01917 #endif /* MBEDTLS_CIPHER_MODE_CBC && 01918 ( MBEDTLS_AES_C || MBEDTLS_CAMELLIA_C ) */ 01919 { 01920 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01921 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01922 } 01923 01924 MBEDTLS_SSL_DEBUG_BUF( 4, "raw buffer after decryption", 01925 ssl->in_msg, ssl->in_msglen ); 01926 01927 /* 01928 * Authenticate if not done yet. 01929 * Compute the MAC regardless of the padding result (RFC4346, CBCTIME). 01930 */ 01931 #if defined(SSL_SOME_MODES_USE_MAC) 01932 if( auth_done == 0 ) 01933 { 01934 unsigned char tmp[SSL_MAX_MAC_SIZE]; 01935 01936 ssl->in_msglen -= ssl->transform_in->maclen; 01937 01938 ssl->in_len[0] = (unsigned char)( ssl->in_msglen >> 8 ); 01939 ssl->in_len[1] = (unsigned char)( ssl->in_msglen ); 01940 01941 memcpy( tmp, ssl->in_msg + ssl->in_msglen, ssl->transform_in->maclen ); 01942 01943 #if defined(MBEDTLS_SSL_PROTO_SSL3) 01944 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 01945 { 01946 ssl_mac( &ssl->transform_in->md_ctx_dec, 01947 ssl->transform_in->mac_dec, 01948 ssl->in_msg, ssl->in_msglen, 01949 ssl->in_ctr, ssl->in_msgtype ); 01950 } 01951 else 01952 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 01953 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 01954 defined(MBEDTLS_SSL_PROTO_TLS1_2) 01955 if( ssl->minor_ver > MBEDTLS_SSL_MINOR_VERSION_0 ) 01956 { 01957 /* 01958 * Process MAC and always update for padlen afterwards to make 01959 * total time independent of padlen 01960 * 01961 * extra_run compensates MAC check for padlen 01962 * 01963 * Known timing attacks: 01964 * - Lucky Thirteen (http://www.isg.rhul.ac.uk/tls/TLStiming.pdf) 01965 * 01966 * We use ( ( Lx + 8 ) / 64 ) to handle 'negative Lx' values 01967 * correctly. (We round down instead of up, so -56 is the correct 01968 * value for our calculations instead of -55) 01969 */ 01970 size_t j, extra_run = 0; 01971 extra_run = ( 13 + ssl->in_msglen + padlen + 8 ) / 64 - 01972 ( 13 + ssl->in_msglen + 8 ) / 64; 01973 01974 extra_run &= correct * 0xFF; 01975 01976 mbedtls_md_hmac_update( &ssl->transform_in->md_ctx_dec, ssl->in_ctr, 8 ); 01977 mbedtls_md_hmac_update( &ssl->transform_in->md_ctx_dec, ssl->in_hdr, 3 ); 01978 mbedtls_md_hmac_update( &ssl->transform_in->md_ctx_dec, ssl->in_len, 2 ); 01979 mbedtls_md_hmac_update( &ssl->transform_in->md_ctx_dec, ssl->in_msg, 01980 ssl->in_msglen ); 01981 mbedtls_md_hmac_finish( &ssl->transform_in->md_ctx_dec, 01982 ssl->in_msg + ssl->in_msglen ); 01983 /* Call mbedtls_md_process at least once due to cache attacks */ 01984 for( j = 0; j < extra_run + 1; j++ ) 01985 mbedtls_md_process( &ssl->transform_in->md_ctx_dec, ssl->in_msg ); 01986 01987 mbedtls_md_hmac_reset( &ssl->transform_in->md_ctx_dec ); 01988 } 01989 else 01990 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 || \ 01991 MBEDTLS_SSL_PROTO_TLS1_2 */ 01992 { 01993 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 01994 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 01995 } 01996 01997 MBEDTLS_SSL_DEBUG_BUF( 4, "message mac", tmp, ssl->transform_in->maclen ); 01998 MBEDTLS_SSL_DEBUG_BUF( 4, "computed mac", ssl->in_msg + ssl->in_msglen, 01999 ssl->transform_in->maclen ); 02000 02001 if( mbedtls_ssl_safer_memcmp( tmp, ssl->in_msg + ssl->in_msglen, 02002 ssl->transform_in->maclen ) != 0 ) 02003 { 02004 #if defined(MBEDTLS_SSL_DEBUG_ALL) 02005 MBEDTLS_SSL_DEBUG_MSG( 1, ( "message mac does not match" ) ); 02006 #endif 02007 correct = 0; 02008 } 02009 auth_done++; 02010 02011 /* 02012 * Finally check the correct flag 02013 */ 02014 if( correct == 0 ) 02015 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 02016 } 02017 #endif /* SSL_SOME_MODES_USE_MAC */ 02018 02019 /* Make extra sure authentication was performed, exactly once */ 02020 if( auth_done != 1 ) 02021 { 02022 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02023 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02024 } 02025 02026 if( ssl->in_msglen == 0 ) 02027 { 02028 ssl->nb_zero++; 02029 02030 /* 02031 * Three or more empty messages may be a DoS attack 02032 * (excessive CPU consumption). 02033 */ 02034 if( ssl->nb_zero > 3 ) 02035 { 02036 MBEDTLS_SSL_DEBUG_MSG( 1, ( "received four consecutive empty " 02037 "messages, possible DoS attack" ) ); 02038 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 02039 } 02040 } 02041 else 02042 ssl->nb_zero = 0; 02043 02044 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02045 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 02046 { 02047 ; /* in_ctr read from peer, not maintained internally */ 02048 } 02049 else 02050 #endif 02051 { 02052 for( i = 8; i > ssl_ep_len( ssl ); i-- ) 02053 if( ++ssl->in_ctr[i - 1] != 0 ) 02054 break; 02055 02056 /* The loop goes to its end iff the counter is wrapping */ 02057 if( i == ssl_ep_len( ssl ) ) 02058 { 02059 MBEDTLS_SSL_DEBUG_MSG( 1, ( "incoming message counter would wrap" ) ); 02060 return( MBEDTLS_ERR_SSL_COUNTER_WRAPPING ); 02061 } 02062 } 02063 02064 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= decrypt buf" ) ); 02065 02066 return( 0 ); 02067 } 02068 02069 #undef MAC_NONE 02070 #undef MAC_PLAINTEXT 02071 #undef MAC_CIPHERTEXT 02072 02073 #if defined(MBEDTLS_ZLIB_SUPPORT) 02074 /* 02075 * Compression/decompression functions 02076 */ 02077 static int ssl_compress_buf( mbedtls_ssl_context *ssl ) 02078 { 02079 int ret; 02080 unsigned char *msg_post = ssl->out_msg; 02081 size_t len_pre = ssl->out_msglen; 02082 unsigned char *msg_pre = ssl->compress_buf; 02083 02084 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> compress buf" ) ); 02085 02086 if( len_pre == 0 ) 02087 return( 0 ); 02088 02089 memcpy( msg_pre, ssl->out_msg, len_pre ); 02090 02091 MBEDTLS_SSL_DEBUG_MSG( 3, ( "before compression: msglen = %d, ", 02092 ssl->out_msglen ) ); 02093 02094 MBEDTLS_SSL_DEBUG_BUF( 4, "before compression: output payload", 02095 ssl->out_msg, ssl->out_msglen ); 02096 02097 ssl->transform_out->ctx_deflate.next_in = msg_pre; 02098 ssl->transform_out->ctx_deflate.avail_in = len_pre; 02099 ssl->transform_out->ctx_deflate.next_out = msg_post; 02100 ssl->transform_out->ctx_deflate.avail_out = MBEDTLS_SSL_BUFFER_LEN; 02101 02102 ret = deflate( &ssl->transform_out->ctx_deflate, Z_SYNC_FLUSH ); 02103 if( ret != Z_OK ) 02104 { 02105 MBEDTLS_SSL_DEBUG_MSG( 1, ( "failed to perform compression (%d)", ret ) ); 02106 return( MBEDTLS_ERR_SSL_COMPRESSION_FAILED ); 02107 } 02108 02109 ssl->out_msglen = MBEDTLS_SSL_BUFFER_LEN - 02110 ssl->transform_out->ctx_deflate.avail_out; 02111 02112 MBEDTLS_SSL_DEBUG_MSG( 3, ( "after compression: msglen = %d, ", 02113 ssl->out_msglen ) ); 02114 02115 MBEDTLS_SSL_DEBUG_BUF( 4, "after compression: output payload", 02116 ssl->out_msg, ssl->out_msglen ); 02117 02118 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= compress buf" ) ); 02119 02120 return( 0 ); 02121 } 02122 02123 static int ssl_decompress_buf( mbedtls_ssl_context *ssl ) 02124 { 02125 int ret; 02126 unsigned char *msg_post = ssl->in_msg; 02127 size_t len_pre = ssl->in_msglen; 02128 unsigned char *msg_pre = ssl->compress_buf; 02129 02130 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> decompress buf" ) ); 02131 02132 if( len_pre == 0 ) 02133 return( 0 ); 02134 02135 memcpy( msg_pre, ssl->in_msg, len_pre ); 02136 02137 MBEDTLS_SSL_DEBUG_MSG( 3, ( "before decompression: msglen = %d, ", 02138 ssl->in_msglen ) ); 02139 02140 MBEDTLS_SSL_DEBUG_BUF( 4, "before decompression: input payload", 02141 ssl->in_msg, ssl->in_msglen ); 02142 02143 ssl->transform_in->ctx_inflate.next_in = msg_pre; 02144 ssl->transform_in->ctx_inflate.avail_in = len_pre; 02145 ssl->transform_in->ctx_inflate.next_out = msg_post; 02146 ssl->transform_in->ctx_inflate.avail_out = MBEDTLS_SSL_MAX_CONTENT_LEN; 02147 02148 ret = inflate( &ssl->transform_in->ctx_inflate, Z_SYNC_FLUSH ); 02149 if( ret != Z_OK ) 02150 { 02151 MBEDTLS_SSL_DEBUG_MSG( 1, ( "failed to perform decompression (%d)", ret ) ); 02152 return( MBEDTLS_ERR_SSL_COMPRESSION_FAILED ); 02153 } 02154 02155 ssl->in_msglen = MBEDTLS_SSL_MAX_CONTENT_LEN - 02156 ssl->transform_in->ctx_inflate.avail_out; 02157 02158 MBEDTLS_SSL_DEBUG_MSG( 3, ( "after decompression: msglen = %d, ", 02159 ssl->in_msglen ) ); 02160 02161 MBEDTLS_SSL_DEBUG_BUF( 4, "after decompression: input payload", 02162 ssl->in_msg, ssl->in_msglen ); 02163 02164 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= decompress buf" ) ); 02165 02166 return( 0 ); 02167 } 02168 #endif /* MBEDTLS_ZLIB_SUPPORT */ 02169 02170 #if defined(MBEDTLS_SSL_SRV_C) && defined(MBEDTLS_SSL_RENEGOTIATION) 02171 static int ssl_write_hello_request( mbedtls_ssl_context *ssl ); 02172 02173 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02174 static int ssl_resend_hello_request( mbedtls_ssl_context *ssl ) 02175 { 02176 /* If renegotiation is not enforced, retransmit until we would reach max 02177 * timeout if we were using the usual handshake doubling scheme */ 02178 if( ssl->conf->renego_max_records < 0 ) 02179 { 02180 uint32_t ratio = ssl->conf->hs_timeout_max / ssl->conf->hs_timeout_min + 1; 02181 unsigned char doublings = 1; 02182 02183 while( ratio != 0 ) 02184 { 02185 ++doublings; 02186 ratio >>= 1; 02187 } 02188 02189 if( ++ssl->renego_records_seen > doublings ) 02190 { 02191 MBEDTLS_SSL_DEBUG_MSG( 2, ( "no longer retransmitting hello request" ) ); 02192 return( 0 ); 02193 } 02194 } 02195 02196 return( ssl_write_hello_request( ssl ) ); 02197 } 02198 #endif 02199 #endif /* MBEDTLS_SSL_SRV_C && MBEDTLS_SSL_RENEGOTIATION */ 02200 02201 /* 02202 * Fill the input message buffer by appending data to it. 02203 * The amount of data already fetched is in ssl->in_left. 02204 * 02205 * If we return 0, is it guaranteed that (at least) nb_want bytes are 02206 * available (from this read and/or a previous one). Otherwise, an error code 02207 * is returned (possibly EOF or WANT_READ). 02208 * 02209 * With stream transport (TLS) on success ssl->in_left == nb_want, but 02210 * with datagram transport (DTLS) on success ssl->in_left >= nb_want, 02211 * since we always read a whole datagram at once. 02212 * 02213 * For DTLS, it is up to the caller to set ssl->next_record_offset when 02214 * they're done reading a record. 02215 */ 02216 int mbedtls_ssl_fetch_input( mbedtls_ssl_context *ssl, size_t nb_want ) 02217 { 02218 int ret; 02219 size_t len; 02220 02221 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> fetch input" ) ); 02222 02223 if( ssl->f_recv == NULL && ssl->f_recv_timeout == NULL ) 02224 { 02225 MBEDTLS_SSL_DEBUG_MSG( 1, ( "Bad usage of mbedtls_ssl_set_bio() " 02226 "or mbedtls_ssl_set_bio()" ) ); 02227 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 02228 } 02229 02230 if( nb_want > MBEDTLS_SSL_BUFFER_LEN - (size_t)( ssl->in_hdr - ssl->in_buf ) ) 02231 { 02232 MBEDTLS_SSL_DEBUG_MSG( 1, ( "requesting more data than fits" ) ); 02233 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 02234 } 02235 02236 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02237 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 02238 { 02239 uint32_t timeout; 02240 02241 /* Just to be sure */ 02242 if( ssl->f_set_timer == NULL || ssl->f_get_timer == NULL ) 02243 { 02244 MBEDTLS_SSL_DEBUG_MSG( 1, ( "You must use " 02245 "mbedtls_ssl_set_timer_cb() for DTLS" ) ); 02246 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 02247 } 02248 02249 /* 02250 * The point is, we need to always read a full datagram at once, so we 02251 * sometimes read more then requested, and handle the additional data. 02252 * It could be the rest of the current record (while fetching the 02253 * header) and/or some other records in the same datagram. 02254 */ 02255 02256 /* 02257 * Move to the next record in the already read datagram if applicable 02258 */ 02259 if( ssl->next_record_offset != 0 ) 02260 { 02261 if( ssl->in_left < ssl->next_record_offset ) 02262 { 02263 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02264 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02265 } 02266 02267 ssl->in_left -= ssl->next_record_offset; 02268 02269 if( ssl->in_left != 0 ) 02270 { 02271 MBEDTLS_SSL_DEBUG_MSG( 2, ( "next record in same datagram, offset: %d", 02272 ssl->next_record_offset ) ); 02273 memmove( ssl->in_hdr, 02274 ssl->in_hdr + ssl->next_record_offset, 02275 ssl->in_left ); 02276 } 02277 02278 ssl->next_record_offset = 0; 02279 } 02280 02281 MBEDTLS_SSL_DEBUG_MSG( 2, ( "in_left: %d, nb_want: %d", 02282 ssl->in_left, nb_want ) ); 02283 02284 /* 02285 * Done if we already have enough data. 02286 */ 02287 if( nb_want <= ssl->in_left) 02288 { 02289 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= fetch input" ) ); 02290 return( 0 ); 02291 } 02292 02293 /* 02294 * A record can't be split accross datagrams. If we need to read but 02295 * are not at the beginning of a new record, the caller did something 02296 * wrong. 02297 */ 02298 if( ssl->in_left != 0 ) 02299 { 02300 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02301 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02302 } 02303 02304 /* 02305 * Don't even try to read if time's out already. 02306 * This avoids by-passing the timer when repeatedly receiving messages 02307 * that will end up being dropped. 02308 */ 02309 if( ssl_check_timer( ssl ) != 0 ) 02310 ret = MBEDTLS_ERR_SSL_TIMEOUT; 02311 else 02312 { 02313 len = MBEDTLS_SSL_BUFFER_LEN - ( ssl->in_hdr - ssl->in_buf ); 02314 02315 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER ) 02316 timeout = ssl->handshake->retransmit_timeout; 02317 else 02318 timeout = ssl->conf->read_timeout; 02319 02320 MBEDTLS_SSL_DEBUG_MSG( 3, ( "f_recv_timeout: %u ms", timeout ) ); 02321 02322 if( ssl->f_recv_timeout != NULL ) 02323 ret = ssl->f_recv_timeout( ssl->p_bio, ssl->in_hdr, len, 02324 timeout ); 02325 else 02326 ret = ssl->f_recv( ssl->p_bio, ssl->in_hdr, len ); 02327 02328 MBEDTLS_SSL_DEBUG_RET( 2, "ssl->f_recv(_timeout)", ret ); 02329 02330 if( ret == 0 ) 02331 return( MBEDTLS_ERR_SSL_CONN_EOF ); 02332 } 02333 02334 if( ret == MBEDTLS_ERR_SSL_TIMEOUT ) 02335 { 02336 MBEDTLS_SSL_DEBUG_MSG( 2, ( "timeout" ) ); 02337 ssl_set_timer( ssl, 0 ); 02338 02339 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER ) 02340 { 02341 if( ssl_double_retransmit_timeout( ssl ) != 0 ) 02342 { 02343 MBEDTLS_SSL_DEBUG_MSG( 1, ( "handshake timeout" ) ); 02344 return( MBEDTLS_ERR_SSL_TIMEOUT ); 02345 } 02346 02347 if( ( ret = mbedtls_ssl_resend( ssl ) ) != 0 ) 02348 { 02349 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_resend", ret ); 02350 return( ret ); 02351 } 02352 02353 return( MBEDTLS_ERR_SSL_WANT_READ ); 02354 } 02355 #if defined(MBEDTLS_SSL_SRV_C) && defined(MBEDTLS_SSL_RENEGOTIATION) 02356 else if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 02357 ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_PENDING ) 02358 { 02359 if( ( ret = ssl_resend_hello_request( ssl ) ) != 0 ) 02360 { 02361 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_resend_hello_request", ret ); 02362 return( ret ); 02363 } 02364 02365 return( MBEDTLS_ERR_SSL_WANT_READ ); 02366 } 02367 #endif /* MBEDTLS_SSL_SRV_C && MBEDTLS_SSL_RENEGOTIATION */ 02368 } 02369 02370 if( ret < 0 ) 02371 return( ret ); 02372 02373 ssl->in_left = ret; 02374 } 02375 else 02376 #endif 02377 { 02378 MBEDTLS_SSL_DEBUG_MSG( 2, ( "in_left: %d, nb_want: %d", 02379 ssl->in_left, nb_want ) ); 02380 02381 while( ssl->in_left < nb_want ) 02382 { 02383 len = nb_want - ssl->in_left; 02384 02385 if( ssl_check_timer( ssl ) != 0 ) 02386 ret = MBEDTLS_ERR_SSL_TIMEOUT; 02387 else 02388 { 02389 if( ssl->f_recv_timeout != NULL ) 02390 { 02391 ret = ssl->f_recv_timeout( ssl->p_bio, 02392 ssl->in_hdr + ssl->in_left, len, 02393 ssl->conf->read_timeout ); 02394 } 02395 else 02396 { 02397 ret = ssl->f_recv( ssl->p_bio, 02398 ssl->in_hdr + ssl->in_left, len ); 02399 } 02400 } 02401 02402 MBEDTLS_SSL_DEBUG_MSG( 2, ( "in_left: %d, nb_want: %d", 02403 ssl->in_left, nb_want ) ); 02404 MBEDTLS_SSL_DEBUG_RET( 2, "ssl->f_recv(_timeout)", ret ); 02405 02406 if( ret == 0 ) 02407 return( MBEDTLS_ERR_SSL_CONN_EOF ); 02408 02409 if( ret < 0 ) 02410 return( ret ); 02411 02412 ssl->in_left += ret; 02413 } 02414 } 02415 02416 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= fetch input" ) ); 02417 02418 return( 0 ); 02419 } 02420 02421 /* 02422 * Flush any data not yet written 02423 */ 02424 int mbedtls_ssl_flush_output( mbedtls_ssl_context *ssl ) 02425 { 02426 int ret; 02427 unsigned char *buf, i; 02428 02429 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> flush output" ) ); 02430 02431 if( ssl->f_send == NULL ) 02432 { 02433 MBEDTLS_SSL_DEBUG_MSG( 1, ( "Bad usage of mbedtls_ssl_set_bio() " 02434 "or mbedtls_ssl_set_bio()" ) ); 02435 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 02436 } 02437 02438 /* Avoid incrementing counter if data is flushed */ 02439 if( ssl->out_left == 0 ) 02440 { 02441 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= flush output" ) ); 02442 return( 0 ); 02443 } 02444 02445 while( ssl->out_left > 0 ) 02446 { 02447 MBEDTLS_SSL_DEBUG_MSG( 2, ( "message length: %d, out_left: %d", 02448 mbedtls_ssl_hdr_len( ssl ) + ssl->out_msglen, ssl->out_left ) ); 02449 02450 buf = ssl->out_hdr + mbedtls_ssl_hdr_len( ssl ) + 02451 ssl->out_msglen - ssl->out_left; 02452 ret = ssl->f_send( ssl->p_bio, buf, ssl->out_left ); 02453 02454 MBEDTLS_SSL_DEBUG_RET( 2, "ssl->f_send", ret ); 02455 02456 if( ret <= 0 ) 02457 return( ret ); 02458 02459 ssl->out_left -= ret; 02460 } 02461 02462 for( i = 8; i > ssl_ep_len( ssl ); i-- ) 02463 if( ++ssl->out_ctr[i - 1] != 0 ) 02464 break; 02465 02466 /* The loop goes to its end iff the counter is wrapping */ 02467 if( i == ssl_ep_len( ssl ) ) 02468 { 02469 MBEDTLS_SSL_DEBUG_MSG( 1, ( "outgoing message counter would wrap" ) ); 02470 return( MBEDTLS_ERR_SSL_COUNTER_WRAPPING ); 02471 } 02472 02473 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= flush output" ) ); 02474 02475 return( 0 ); 02476 } 02477 02478 /* 02479 * Functions to handle the DTLS retransmission state machine 02480 */ 02481 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02482 /* 02483 * Append current handshake message to current outgoing flight 02484 */ 02485 static int ssl_flight_append( mbedtls_ssl_context *ssl ) 02486 { 02487 mbedtls_ssl_flight_item *msg; 02488 02489 /* Allocate space for current message */ 02490 if( ( msg = mbedtls_calloc( 1, sizeof( mbedtls_ssl_flight_item ) ) ) == NULL ) 02491 { 02492 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc %d bytes failed", 02493 sizeof( mbedtls_ssl_flight_item ) ) ); 02494 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 02495 } 02496 02497 if( ( msg->p = mbedtls_calloc( 1, ssl->out_msglen ) ) == NULL ) 02498 { 02499 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc %d bytes failed", ssl->out_msglen ) ); 02500 mbedtls_free( msg ); 02501 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 02502 } 02503 02504 /* Copy current handshake message with headers */ 02505 memcpy( msg->p, ssl->out_msg, ssl->out_msglen ); 02506 msg->len = ssl->out_msglen; 02507 msg->type = ssl->out_msgtype; 02508 msg->next = NULL; 02509 02510 /* Append to the current flight */ 02511 if( ssl->handshake->flight == NULL ) 02512 ssl->handshake->flight = msg; 02513 else 02514 { 02515 mbedtls_ssl_flight_item *cur = ssl->handshake->flight; 02516 while( cur->next != NULL ) 02517 cur = cur->next; 02518 cur->next = msg; 02519 } 02520 02521 return( 0 ); 02522 } 02523 02524 /* 02525 * Free the current flight of handshake messages 02526 */ 02527 static void ssl_flight_free( mbedtls_ssl_flight_item *flight ) 02528 { 02529 mbedtls_ssl_flight_item *cur = flight; 02530 mbedtls_ssl_flight_item *next; 02531 02532 while( cur != NULL ) 02533 { 02534 next = cur->next; 02535 02536 mbedtls_free( cur->p ); 02537 mbedtls_free( cur ); 02538 02539 cur = next; 02540 } 02541 } 02542 02543 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 02544 static void ssl_dtls_replay_reset( mbedtls_ssl_context *ssl ); 02545 #endif 02546 02547 /* 02548 * Swap transform_out and out_ctr with the alternative ones 02549 */ 02550 static void ssl_swap_epochs( mbedtls_ssl_context *ssl ) 02551 { 02552 mbedtls_ssl_transform *tmp_transform; 02553 unsigned char tmp_out_ctr[8]; 02554 02555 if( ssl->transform_out == ssl->handshake->alt_transform_out ) 02556 { 02557 MBEDTLS_SSL_DEBUG_MSG( 3, ( "skip swap epochs" ) ); 02558 return; 02559 } 02560 02561 MBEDTLS_SSL_DEBUG_MSG( 3, ( "swap epochs" ) ); 02562 02563 /* Swap transforms */ 02564 tmp_transform = ssl->transform_out; 02565 ssl->transform_out = ssl->handshake->alt_transform_out; 02566 ssl->handshake->alt_transform_out = tmp_transform; 02567 02568 /* Swap epoch + sequence_number */ 02569 memcpy( tmp_out_ctr, ssl->out_ctr, 8 ); 02570 memcpy( ssl->out_ctr, ssl->handshake->alt_out_ctr, 8 ); 02571 memcpy( ssl->handshake->alt_out_ctr, tmp_out_ctr, 8 ); 02572 02573 /* Adjust to the newly activated transform */ 02574 if( ssl->transform_out != NULL && 02575 ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_2 ) 02576 { 02577 ssl->out_msg = ssl->out_iv + ssl->transform_out->ivlen - 02578 ssl->transform_out->fixed_ivlen; 02579 } 02580 else 02581 ssl->out_msg = ssl->out_iv; 02582 02583 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 02584 if( mbedtls_ssl_hw_record_activate != NULL ) 02585 { 02586 if( ( ret = mbedtls_ssl_hw_record_activate( ssl, MBEDTLS_SSL_CHANNEL_OUTBOUND ) ) != 0 ) 02587 { 02588 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_hw_record_activate", ret ); 02589 return( MBEDTLS_ERR_SSL_HW_ACCEL_FAILED ); 02590 } 02591 } 02592 #endif 02593 } 02594 02595 /* 02596 * Retransmit the current flight of messages. 02597 * 02598 * Need to remember the current message in case flush_output returns 02599 * WANT_WRITE, causing us to exit this function and come back later. 02600 * This function must be called until state is no longer SENDING. 02601 */ 02602 int mbedtls_ssl_resend( mbedtls_ssl_context *ssl ) 02603 { 02604 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> mbedtls_ssl_resend" ) ); 02605 02606 if( ssl->handshake->retransmit_state != MBEDTLS_SSL_RETRANS_SENDING ) 02607 { 02608 MBEDTLS_SSL_DEBUG_MSG( 2, ( "initialise resending" ) ); 02609 02610 ssl->handshake->cur_msg = ssl->handshake->flight; 02611 ssl_swap_epochs( ssl ); 02612 02613 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_SENDING; 02614 } 02615 02616 while( ssl->handshake->cur_msg != NULL ) 02617 { 02618 int ret; 02619 mbedtls_ssl_flight_item *cur = ssl->handshake->cur_msg; 02620 02621 /* Swap epochs before sending Finished: we can't do it after 02622 * sending ChangeCipherSpec, in case write returns WANT_READ. 02623 * Must be done before copying, may change out_msg pointer */ 02624 if( cur->type == MBEDTLS_SSL_MSG_HANDSHAKE && 02625 cur->p[0] == MBEDTLS_SSL_HS_FINISHED ) 02626 { 02627 ssl_swap_epochs( ssl ); 02628 } 02629 02630 memcpy( ssl->out_msg, cur->p, cur->len ); 02631 ssl->out_msglen = cur->len; 02632 ssl->out_msgtype = cur->type; 02633 02634 ssl->handshake->cur_msg = cur->next; 02635 02636 MBEDTLS_SSL_DEBUG_BUF( 3, "resent handshake message header", ssl->out_msg, 12 ); 02637 02638 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 02639 { 02640 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 02641 return( ret ); 02642 } 02643 } 02644 02645 if( ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER ) 02646 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_FINISHED; 02647 else 02648 { 02649 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_WAITING; 02650 ssl_set_timer( ssl, ssl->handshake->retransmit_timeout ); 02651 } 02652 02653 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= mbedtls_ssl_resend" ) ); 02654 02655 return( 0 ); 02656 } 02657 02658 /* 02659 * To be called when the last message of an incoming flight is received. 02660 */ 02661 void mbedtls_ssl_recv_flight_completed( mbedtls_ssl_context *ssl ) 02662 { 02663 /* We won't need to resend that one any more */ 02664 ssl_flight_free( ssl->handshake->flight ); 02665 ssl->handshake->flight = NULL; 02666 ssl->handshake->cur_msg = NULL; 02667 02668 /* The next incoming flight will start with this msg_seq */ 02669 ssl->handshake->in_flight_start_seq = ssl->handshake->in_msg_seq; 02670 02671 /* Cancel timer */ 02672 ssl_set_timer( ssl, 0 ); 02673 02674 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE && 02675 ssl->in_msg[0] == MBEDTLS_SSL_HS_FINISHED ) 02676 { 02677 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_FINISHED; 02678 } 02679 else 02680 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_PREPARING; 02681 } 02682 02683 /* 02684 * To be called when the last message of an outgoing flight is send. 02685 */ 02686 void mbedtls_ssl_send_flight_completed( mbedtls_ssl_context *ssl ) 02687 { 02688 ssl_reset_retransmit_timeout( ssl ); 02689 ssl_set_timer( ssl, ssl->handshake->retransmit_timeout ); 02690 02691 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE && 02692 ssl->in_msg[0] == MBEDTLS_SSL_HS_FINISHED ) 02693 { 02694 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_FINISHED; 02695 } 02696 else 02697 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_WAITING; 02698 } 02699 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 02700 02701 /* 02702 * Record layer functions 02703 */ 02704 02705 /* 02706 * Write current record. 02707 * Uses ssl->out_msgtype, ssl->out_msglen and bytes at ssl->out_msg. 02708 */ 02709 int mbedtls_ssl_write_record( mbedtls_ssl_context *ssl ) 02710 { 02711 int ret, done = 0; 02712 size_t len = ssl->out_msglen; 02713 02714 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write record" ) ); 02715 02716 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02717 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 02718 ssl->handshake != NULL && 02719 ssl->handshake->retransmit_state == MBEDTLS_SSL_RETRANS_SENDING ) 02720 { 02721 ; /* Skip special handshake treatment when resending */ 02722 } 02723 else 02724 #endif 02725 if( ssl->out_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE ) 02726 { 02727 if( ssl->out_msg[0] != MBEDTLS_SSL_HS_HELLO_REQUEST && 02728 ssl->handshake == NULL ) 02729 { 02730 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02731 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02732 } 02733 02734 ssl->out_msg[1] = (unsigned char)( ( len - 4 ) >> 16 ); 02735 ssl->out_msg[2] = (unsigned char)( ( len - 4 ) >> 8 ); 02736 ssl->out_msg[3] = (unsigned char)( ( len - 4 ) ); 02737 02738 /* 02739 * DTLS has additional fields in the Handshake layer, 02740 * between the length field and the actual payload: 02741 * uint16 message_seq; 02742 * uint24 fragment_offset; 02743 * uint24 fragment_length; 02744 */ 02745 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02746 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 02747 { 02748 /* Make room for the additional DTLS fields */ 02749 memmove( ssl->out_msg + 12, ssl->out_msg + 4, len - 4 ); 02750 ssl->out_msglen += 8; 02751 len += 8; 02752 02753 /* Write message_seq and update it, except for HelloRequest */ 02754 if( ssl->out_msg[0] != MBEDTLS_SSL_HS_HELLO_REQUEST ) 02755 { 02756 ssl->out_msg[4] = ( ssl->handshake->out_msg_seq >> 8 ) & 0xFF; 02757 ssl->out_msg[5] = ( ssl->handshake->out_msg_seq ) & 0xFF; 02758 ++( ssl->handshake->out_msg_seq ); 02759 } 02760 else 02761 { 02762 ssl->out_msg[4] = 0; 02763 ssl->out_msg[5] = 0; 02764 } 02765 02766 /* We don't fragment, so frag_offset = 0 and frag_len = len */ 02767 memset( ssl->out_msg + 6, 0x00, 3 ); 02768 memcpy( ssl->out_msg + 9, ssl->out_msg + 1, 3 ); 02769 } 02770 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 02771 02772 if( ssl->out_msg[0] != MBEDTLS_SSL_HS_HELLO_REQUEST ) 02773 ssl->handshake->update_checksum( ssl, ssl->out_msg, len ); 02774 } 02775 02776 /* Save handshake and CCS messages for resending */ 02777 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02778 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 02779 ssl->handshake != NULL && 02780 ssl->handshake->retransmit_state != MBEDTLS_SSL_RETRANS_SENDING && 02781 ( ssl->out_msgtype == MBEDTLS_SSL_MSG_CHANGE_CIPHER_SPEC || 02782 ssl->out_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE ) ) 02783 { 02784 if( ( ret = ssl_flight_append( ssl ) ) != 0 ) 02785 { 02786 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_flight_append", ret ); 02787 return( ret ); 02788 } 02789 } 02790 #endif 02791 02792 #if defined(MBEDTLS_ZLIB_SUPPORT) 02793 if( ssl->transform_out != NULL && 02794 ssl->session_out->compression == MBEDTLS_SSL_COMPRESS_DEFLATE ) 02795 { 02796 if( ( ret = ssl_compress_buf( ssl ) ) != 0 ) 02797 { 02798 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_compress_buf", ret ); 02799 return( ret ); 02800 } 02801 02802 len = ssl->out_msglen; 02803 } 02804 #endif /*MBEDTLS_ZLIB_SUPPORT */ 02805 02806 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 02807 if( mbedtls_ssl_hw_record_write != NULL ) 02808 { 02809 MBEDTLS_SSL_DEBUG_MSG( 2, ( "going for mbedtls_ssl_hw_record_write()" ) ); 02810 02811 ret = mbedtls_ssl_hw_record_write( ssl ); 02812 if( ret != 0 && ret != MBEDTLS_ERR_SSL_HW_ACCEL_FALLTHROUGH ) 02813 { 02814 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_hw_record_write", ret ); 02815 return( MBEDTLS_ERR_SSL_HW_ACCEL_FAILED ); 02816 } 02817 02818 if( ret == 0 ) 02819 done = 1; 02820 } 02821 #endif /* MBEDTLS_SSL_HW_RECORD_ACCEL */ 02822 if( !done ) 02823 { 02824 ssl->out_hdr[0] = (unsigned char) ssl->out_msgtype; 02825 mbedtls_ssl_write_version( ssl->major_ver, ssl->minor_ver, 02826 ssl->conf->transport, ssl->out_hdr + 1 ); 02827 02828 ssl->out_len[0] = (unsigned char)( len >> 8 ); 02829 ssl->out_len[1] = (unsigned char)( len ); 02830 02831 if( ssl->transform_out != NULL ) 02832 { 02833 if( ( ret = ssl_encrypt_buf( ssl ) ) != 0 ) 02834 { 02835 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_encrypt_buf", ret ); 02836 return( ret ); 02837 } 02838 02839 len = ssl->out_msglen; 02840 ssl->out_len[0] = (unsigned char)( len >> 8 ); 02841 ssl->out_len[1] = (unsigned char)( len ); 02842 } 02843 02844 ssl->out_left = mbedtls_ssl_hdr_len( ssl ) + ssl->out_msglen; 02845 02846 MBEDTLS_SSL_DEBUG_MSG( 3, ( "output record: msgtype = %d, " 02847 "version = [%d:%d], msglen = %d", 02848 ssl->out_hdr[0], ssl->out_hdr[1], ssl->out_hdr[2], 02849 ( ssl->out_len[0] << 8 ) | ssl->out_len[1] ) ); 02850 02851 MBEDTLS_SSL_DEBUG_BUF( 4, "output record sent to network", 02852 ssl->out_hdr, mbedtls_ssl_hdr_len( ssl ) + ssl->out_msglen ); 02853 } 02854 02855 if( ( ret = mbedtls_ssl_flush_output( ssl ) ) != 0 ) 02856 { 02857 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_flush_output", ret ); 02858 return( ret ); 02859 } 02860 02861 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write record" ) ); 02862 02863 return( 0 ); 02864 } 02865 02866 #if defined(MBEDTLS_SSL_PROTO_DTLS) 02867 /* 02868 * Mark bits in bitmask (used for DTLS HS reassembly) 02869 */ 02870 static void ssl_bitmask_set( unsigned char *mask, size_t offset, size_t len ) 02871 { 02872 unsigned int start_bits, end_bits; 02873 02874 start_bits = 8 - ( offset % 8 ); 02875 if( start_bits != 8 ) 02876 { 02877 size_t first_byte_idx = offset / 8; 02878 02879 /* Special case */ 02880 if( len <= start_bits ) 02881 { 02882 for( ; len != 0; len-- ) 02883 mask[first_byte_idx] |= 1 << ( start_bits - len ); 02884 02885 /* Avoid potential issues with offset or len becoming invalid */ 02886 return; 02887 } 02888 02889 offset += start_bits; /* Now offset % 8 == 0 */ 02890 len -= start_bits; 02891 02892 for( ; start_bits != 0; start_bits-- ) 02893 mask[first_byte_idx] |= 1 << ( start_bits - 1 ); 02894 } 02895 02896 end_bits = len % 8; 02897 if( end_bits != 0 ) 02898 { 02899 size_t last_byte_idx = ( offset + len ) / 8; 02900 02901 len -= end_bits; /* Now len % 8 == 0 */ 02902 02903 for( ; end_bits != 0; end_bits-- ) 02904 mask[last_byte_idx] |= 1 << ( 8 - end_bits ); 02905 } 02906 02907 memset( mask + offset / 8, 0xFF, len / 8 ); 02908 } 02909 02910 /* 02911 * Check that bitmask is full 02912 */ 02913 static int ssl_bitmask_check( unsigned char *mask, size_t len ) 02914 { 02915 size_t i; 02916 02917 for( i = 0; i < len / 8; i++ ) 02918 if( mask[i] != 0xFF ) 02919 return( -1 ); 02920 02921 for( i = 0; i < len % 8; i++ ) 02922 if( ( mask[len / 8] & ( 1 << ( 7 - i ) ) ) == 0 ) 02923 return( -1 ); 02924 02925 return( 0 ); 02926 } 02927 02928 /* 02929 * Reassemble fragmented DTLS handshake messages. 02930 * 02931 * Use a temporary buffer for reassembly, divided in two parts: 02932 * - the first holds the reassembled message (including handshake header), 02933 * - the second holds a bitmask indicating which parts of the message 02934 * (excluding headers) have been received so far. 02935 */ 02936 static int ssl_reassemble_dtls_handshake( mbedtls_ssl_context *ssl ) 02937 { 02938 unsigned char *msg, *bitmask; 02939 size_t frag_len, frag_off; 02940 size_t msg_len = ssl->in_hslen - 12; /* Without headers */ 02941 02942 if( ssl->handshake == NULL ) 02943 { 02944 MBEDTLS_SSL_DEBUG_MSG( 1, ( "not supported outside handshake (for now)" ) ); 02945 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 02946 } 02947 02948 /* 02949 * For first fragment, check size and allocate buffer 02950 */ 02951 if( ssl->handshake->hs_msg == NULL ) 02952 { 02953 size_t alloc_len; 02954 02955 MBEDTLS_SSL_DEBUG_MSG( 2, ( "initialize reassembly, total length = %d", 02956 msg_len ) ); 02957 02958 if( ssl->in_hslen > MBEDTLS_SSL_MAX_CONTENT_LEN ) 02959 { 02960 MBEDTLS_SSL_DEBUG_MSG( 1, ( "handshake message too large" ) ); 02961 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 02962 } 02963 02964 /* The bitmask needs one bit per byte of message excluding header */ 02965 alloc_len = 12 + msg_len + msg_len / 8 + ( msg_len % 8 != 0 ); 02966 02967 ssl->handshake->hs_msg = mbedtls_calloc( 1, alloc_len ); 02968 if( ssl->handshake->hs_msg == NULL ) 02969 { 02970 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc failed (%d bytes)", alloc_len ) ); 02971 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 02972 } 02973 02974 /* Prepare final header: copy msg_type, length and message_seq, 02975 * then add standardised fragment_offset and fragment_length */ 02976 memcpy( ssl->handshake->hs_msg, ssl->in_msg, 6 ); 02977 memset( ssl->handshake->hs_msg + 6, 0, 3 ); 02978 memcpy( ssl->handshake->hs_msg + 9, 02979 ssl->handshake->hs_msg + 1, 3 ); 02980 } 02981 else 02982 { 02983 /* Make sure msg_type and length are consistent */ 02984 if( memcmp( ssl->handshake->hs_msg, ssl->in_msg, 4 ) != 0 ) 02985 { 02986 MBEDTLS_SSL_DEBUG_MSG( 1, ( "fragment header mismatch" ) ); 02987 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 02988 } 02989 } 02990 02991 msg = ssl->handshake->hs_msg + 12; 02992 bitmask = msg + msg_len; 02993 02994 /* 02995 * Check and copy current fragment 02996 */ 02997 frag_off = ( ssl->in_msg[6] << 16 ) | 02998 ( ssl->in_msg[7] << 8 ) | 02999 ssl->in_msg[8]; 03000 frag_len = ( ssl->in_msg[9] << 16 ) | 03001 ( ssl->in_msg[10] << 8 ) | 03002 ssl->in_msg[11]; 03003 03004 if( frag_off + frag_len > msg_len ) 03005 { 03006 MBEDTLS_SSL_DEBUG_MSG( 1, ( "invalid fragment offset/len: %d + %d > %d", 03007 frag_off, frag_len, msg_len ) ); 03008 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03009 } 03010 03011 if( frag_len + 12 > ssl->in_msglen ) 03012 { 03013 MBEDTLS_SSL_DEBUG_MSG( 1, ( "invalid fragment length: %d + 12 > %d", 03014 frag_len, ssl->in_msglen ) ); 03015 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03016 } 03017 03018 MBEDTLS_SSL_DEBUG_MSG( 2, ( "adding fragment, offset = %d, length = %d", 03019 frag_off, frag_len ) ); 03020 03021 memcpy( msg + frag_off, ssl->in_msg + 12, frag_len ); 03022 ssl_bitmask_set( bitmask, frag_off, frag_len ); 03023 03024 /* 03025 * Do we have the complete message by now? 03026 * If yes, finalize it, else ask to read the next record. 03027 */ 03028 if( ssl_bitmask_check( bitmask, msg_len ) != 0 ) 03029 { 03030 MBEDTLS_SSL_DEBUG_MSG( 2, ( "message is not complete yet" ) ); 03031 return( MBEDTLS_ERR_SSL_WANT_READ ); 03032 } 03033 03034 MBEDTLS_SSL_DEBUG_MSG( 2, ( "handshake message completed" ) ); 03035 03036 if( frag_len + 12 < ssl->in_msglen ) 03037 { 03038 /* 03039 * We'got more handshake messages in the same record. 03040 * This case is not handled now because no know implementation does 03041 * that and it's hard to test, so we prefer to fail cleanly for now. 03042 */ 03043 MBEDTLS_SSL_DEBUG_MSG( 1, ( "last fragment not alone in its record" ) ); 03044 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 03045 } 03046 03047 if( ssl->in_left > ssl->next_record_offset ) 03048 { 03049 /* 03050 * We've got more data in the buffer after the current record, 03051 * that we don't want to overwrite. Move it before writing the 03052 * reassembled message, and adjust in_left and next_record_offset. 03053 */ 03054 unsigned char *cur_remain = ssl->in_hdr + ssl->next_record_offset; 03055 unsigned char *new_remain = ssl->in_msg + ssl->in_hslen; 03056 size_t remain_len = ssl->in_left - ssl->next_record_offset; 03057 03058 /* First compute and check new lengths */ 03059 ssl->next_record_offset = new_remain - ssl->in_hdr; 03060 ssl->in_left = ssl->next_record_offset + remain_len; 03061 03062 if( ssl->in_left > MBEDTLS_SSL_BUFFER_LEN - 03063 (size_t)( ssl->in_hdr - ssl->in_buf ) ) 03064 { 03065 MBEDTLS_SSL_DEBUG_MSG( 1, ( "reassembled message too large for buffer" ) ); 03066 return( MBEDTLS_ERR_SSL_BUFFER_TOO_SMALL ); 03067 } 03068 03069 memmove( new_remain, cur_remain, remain_len ); 03070 } 03071 03072 memcpy( ssl->in_msg, ssl->handshake->hs_msg, ssl->in_hslen ); 03073 03074 mbedtls_free( ssl->handshake->hs_msg ); 03075 ssl->handshake->hs_msg = NULL; 03076 03077 MBEDTLS_SSL_DEBUG_BUF( 3, "reassembled handshake message", 03078 ssl->in_msg, ssl->in_hslen ); 03079 03080 return( 0 ); 03081 } 03082 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 03083 03084 static int ssl_prepare_handshake_record( mbedtls_ssl_context *ssl ) 03085 { 03086 if( ssl->in_msglen < mbedtls_ssl_hs_hdr_len( ssl ) ) 03087 { 03088 MBEDTLS_SSL_DEBUG_MSG( 1, ( "handshake message too short: %d", 03089 ssl->in_msglen ) ); 03090 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03091 } 03092 03093 ssl->in_hslen = mbedtls_ssl_hs_hdr_len( ssl ) + ( 03094 ( ssl->in_msg[1] << 16 ) | 03095 ( ssl->in_msg[2] << 8 ) | 03096 ssl->in_msg[3] ); 03097 03098 MBEDTLS_SSL_DEBUG_MSG( 3, ( "handshake message: msglen =" 03099 " %d, type = %d, hslen = %d", 03100 ssl->in_msglen, ssl->in_msg[0], ssl->in_hslen ) ); 03101 03102 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03103 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03104 { 03105 int ret; 03106 unsigned int recv_msg_seq = ( ssl->in_msg[4] << 8 ) | ssl->in_msg[5]; 03107 03108 /* ssl->handshake is NULL when receiving ClientHello for renego */ 03109 if( ssl->handshake != NULL && 03110 recv_msg_seq != ssl->handshake->in_msg_seq ) 03111 { 03112 /* Retransmit only on last message from previous flight, to avoid 03113 * too many retransmissions. 03114 * Besides, No sane server ever retransmits HelloVerifyRequest */ 03115 if( recv_msg_seq == ssl->handshake->in_flight_start_seq - 1 && 03116 ssl->in_msg[0] != MBEDTLS_SSL_HS_HELLO_VERIFY_REQUEST ) 03117 { 03118 MBEDTLS_SSL_DEBUG_MSG( 2, ( "received message from last flight, " 03119 "message_seq = %d, start_of_flight = %d", 03120 recv_msg_seq, 03121 ssl->handshake->in_flight_start_seq ) ); 03122 03123 if( ( ret = mbedtls_ssl_resend( ssl ) ) != 0 ) 03124 { 03125 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_resend", ret ); 03126 return( ret ); 03127 } 03128 } 03129 else 03130 { 03131 MBEDTLS_SSL_DEBUG_MSG( 2, ( "dropping out-of-sequence message: " 03132 "message_seq = %d, expected = %d", 03133 recv_msg_seq, 03134 ssl->handshake->in_msg_seq ) ); 03135 } 03136 03137 return( MBEDTLS_ERR_SSL_WANT_READ ); 03138 } 03139 /* Wait until message completion to increment in_msg_seq */ 03140 03141 /* Reassemble if current message is fragmented or reassembly is 03142 * already in progress */ 03143 if( ssl->in_msglen < ssl->in_hslen || 03144 memcmp( ssl->in_msg + 6, "\0\0\0", 3 ) != 0 || 03145 memcmp( ssl->in_msg + 9, ssl->in_msg + 1, 3 ) != 0 || 03146 ( ssl->handshake != NULL && ssl->handshake->hs_msg != NULL ) ) 03147 { 03148 MBEDTLS_SSL_DEBUG_MSG( 2, ( "found fragmented DTLS handshake message" ) ); 03149 03150 if( ( ret = ssl_reassemble_dtls_handshake( ssl ) ) != 0 ) 03151 { 03152 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_reassemble_dtls_handshake", ret ); 03153 return( ret ); 03154 } 03155 } 03156 } 03157 else 03158 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 03159 /* With TLS we don't handle fragmentation (for now) */ 03160 if( ssl->in_msglen < ssl->in_hslen ) 03161 { 03162 MBEDTLS_SSL_DEBUG_MSG( 1, ( "TLS handshake fragmentation not supported" ) ); 03163 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 03164 } 03165 03166 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER && 03167 ssl->handshake != NULL ) 03168 { 03169 ssl->handshake->update_checksum( ssl, ssl->in_msg, ssl->in_hslen ); 03170 } 03171 03172 /* Handshake message is complete, increment counter */ 03173 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03174 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 03175 ssl->handshake != NULL ) 03176 { 03177 ssl->handshake->in_msg_seq++; 03178 } 03179 #endif 03180 03181 return( 0 ); 03182 } 03183 03184 /* 03185 * DTLS anti-replay: RFC 6347 4.1.2.6 03186 * 03187 * in_window is a field of bits numbered from 0 (lsb) to 63 (msb). 03188 * Bit n is set iff record number in_window_top - n has been seen. 03189 * 03190 * Usually, in_window_top is the last record number seen and the lsb of 03191 * in_window is set. The only exception is the initial state (record number 0 03192 * not seen yet). 03193 */ 03194 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 03195 static void ssl_dtls_replay_reset( mbedtls_ssl_context *ssl ) 03196 { 03197 ssl->in_window_top = 0; 03198 ssl->in_window = 0; 03199 } 03200 03201 static inline uint64_t ssl_load_six_bytes( unsigned char *buf ) 03202 { 03203 return( ( (uint64_t) buf[0] << 40 ) | 03204 ( (uint64_t) buf[1] << 32 ) | 03205 ( (uint64_t) buf[2] << 24 ) | 03206 ( (uint64_t) buf[3] << 16 ) | 03207 ( (uint64_t) buf[4] << 8 ) | 03208 ( (uint64_t) buf[5] ) ); 03209 } 03210 03211 /* 03212 * Return 0 if sequence number is acceptable, -1 otherwise 03213 */ 03214 int mbedtls_ssl_dtls_replay_check( mbedtls_ssl_context *ssl ) 03215 { 03216 uint64_t rec_seqnum = ssl_load_six_bytes( ssl->in_ctr + 2 ); 03217 uint64_t bit; 03218 03219 if( ssl->conf->anti_replay == MBEDTLS_SSL_ANTI_REPLAY_DISABLED ) 03220 return( 0 ); 03221 03222 if( rec_seqnum > ssl->in_window_top ) 03223 return( 0 ); 03224 03225 bit = ssl->in_window_top - rec_seqnum; 03226 03227 if( bit >= 64 ) 03228 return( -1 ); 03229 03230 if( ( ssl->in_window & ( (uint64_t) 1 << bit ) ) != 0 ) 03231 return( -1 ); 03232 03233 return( 0 ); 03234 } 03235 03236 /* 03237 * Update replay window on new validated record 03238 */ 03239 void mbedtls_ssl_dtls_replay_update( mbedtls_ssl_context *ssl ) 03240 { 03241 uint64_t rec_seqnum = ssl_load_six_bytes( ssl->in_ctr + 2 ); 03242 03243 if( ssl->conf->anti_replay == MBEDTLS_SSL_ANTI_REPLAY_DISABLED ) 03244 return; 03245 03246 if( rec_seqnum > ssl->in_window_top ) 03247 { 03248 /* Update window_top and the contents of the window */ 03249 uint64_t shift = rec_seqnum - ssl->in_window_top; 03250 03251 if( shift >= 64 ) 03252 ssl->in_window = 1; 03253 else 03254 { 03255 ssl->in_window <<= shift; 03256 ssl->in_window |= 1; 03257 } 03258 03259 ssl->in_window_top = rec_seqnum; 03260 } 03261 else 03262 { 03263 /* Mark that number as seen in the current window */ 03264 uint64_t bit = ssl->in_window_top - rec_seqnum; 03265 03266 if( bit < 64 ) /* Always true, but be extra sure */ 03267 ssl->in_window |= (uint64_t) 1 << bit; 03268 } 03269 } 03270 #endif /* MBEDTLS_SSL_DTLS_ANTI_REPLAY */ 03271 03272 #if defined(MBEDTLS_SSL_DTLS_CLIENT_PORT_REUSE) && defined(MBEDTLS_SSL_SRV_C) 03273 /* Forward declaration */ 03274 static int ssl_session_reset_int( mbedtls_ssl_context *ssl, int partial ); 03275 03276 /* 03277 * Without any SSL context, check if a datagram looks like a ClientHello with 03278 * a valid cookie, and if it doesn't, generate a HelloVerifyRequest message. 03279 * Both input and output include full DTLS headers. 03280 * 03281 * - if cookie is valid, return 0 03282 * - if ClientHello looks superficially valid but cookie is not, 03283 * fill obuf and set olen, then 03284 * return MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED 03285 * - otherwise return a specific error code 03286 */ 03287 static int ssl_check_dtls_clihlo_cookie( 03288 mbedtls_ssl_cookie_write_t *f_cookie_write, 03289 mbedtls_ssl_cookie_check_t *f_cookie_check, 03290 void *p_cookie, 03291 const unsigned char *cli_id, size_t cli_id_len, 03292 const unsigned char *in, size_t in_len, 03293 unsigned char *obuf, size_t buf_len, size_t *olen ) 03294 { 03295 size_t sid_len, cookie_len; 03296 unsigned char *p; 03297 03298 if( f_cookie_write == NULL || f_cookie_check == NULL ) 03299 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 03300 03301 /* 03302 * Structure of ClientHello with record and handshake headers, 03303 * and expected values. We don't need to check a lot, more checks will be 03304 * done when actually parsing the ClientHello - skipping those checks 03305 * avoids code duplication and does not make cookie forging any easier. 03306 * 03307 * 0-0 ContentType type; copied, must be handshake 03308 * 1-2 ProtocolVersion version; copied 03309 * 3-4 uint16 epoch; copied, must be 0 03310 * 5-10 uint48 sequence_number; copied 03311 * 11-12 uint16 length; (ignored) 03312 * 03313 * 13-13 HandshakeType msg_type; (ignored) 03314 * 14-16 uint24 length; (ignored) 03315 * 17-18 uint16 message_seq; copied 03316 * 19-21 uint24 fragment_offset; copied, must be 0 03317 * 22-24 uint24 fragment_length; (ignored) 03318 * 03319 * 25-26 ProtocolVersion client_version; (ignored) 03320 * 27-58 Random random; (ignored) 03321 * 59-xx SessionID session_id; 1 byte len + sid_len content 03322 * 60+ opaque cookie<0..2^8-1>; 1 byte len + content 03323 * ... 03324 * 03325 * Minimum length is 61 bytes. 03326 */ 03327 if( in_len < 61 || 03328 in[0] != MBEDTLS_SSL_MSG_HANDSHAKE || 03329 in[3] != 0 || in[4] != 0 || 03330 in[19] != 0 || in[20] != 0 || in[21] != 0 ) 03331 { 03332 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 03333 } 03334 03335 sid_len = in[59]; 03336 if( sid_len > in_len - 61 ) 03337 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 03338 03339 cookie_len = in[60 + sid_len]; 03340 if( cookie_len > in_len - 60 ) 03341 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 03342 03343 if( f_cookie_check( p_cookie, in + sid_len + 61, cookie_len, 03344 cli_id, cli_id_len ) == 0 ) 03345 { 03346 /* Valid cookie */ 03347 return( 0 ); 03348 } 03349 03350 /* 03351 * If we get here, we've got an invalid cookie, let's prepare HVR. 03352 * 03353 * 0-0 ContentType type; copied 03354 * 1-2 ProtocolVersion version; copied 03355 * 3-4 uint16 epoch; copied 03356 * 5-10 uint48 sequence_number; copied 03357 * 11-12 uint16 length; olen - 13 03358 * 03359 * 13-13 HandshakeType msg_type; hello_verify_request 03360 * 14-16 uint24 length; olen - 25 03361 * 17-18 uint16 message_seq; copied 03362 * 19-21 uint24 fragment_offset; copied 03363 * 22-24 uint24 fragment_length; olen - 25 03364 * 03365 * 25-26 ProtocolVersion server_version; 0xfe 0xff 03366 * 27-27 opaque cookie<0..2^8-1>; cookie_len = olen - 27, cookie 03367 * 03368 * Minimum length is 28. 03369 */ 03370 if( buf_len < 28 ) 03371 return( MBEDTLS_ERR_SSL_BUFFER_TOO_SMALL ); 03372 03373 /* Copy most fields and adapt others */ 03374 memcpy( obuf, in, 25 ); 03375 obuf[13] = MBEDTLS_SSL_HS_HELLO_VERIFY_REQUEST; 03376 obuf[25] = 0xfe; 03377 obuf[26] = 0xff; 03378 03379 /* Generate and write actual cookie */ 03380 p = obuf + 28; 03381 if( f_cookie_write( p_cookie, 03382 &p, obuf + buf_len, cli_id, cli_id_len ) != 0 ) 03383 { 03384 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03385 } 03386 03387 *olen = p - obuf; 03388 03389 /* Go back and fill length fields */ 03390 obuf[27] = (unsigned char)( *olen - 28 ); 03391 03392 obuf[14] = obuf[22] = (unsigned char)( ( *olen - 25 ) >> 16 ); 03393 obuf[15] = obuf[23] = (unsigned char)( ( *olen - 25 ) >> 8 ); 03394 obuf[16] = obuf[24] = (unsigned char)( ( *olen - 25 ) ); 03395 03396 obuf[11] = (unsigned char)( ( *olen - 13 ) >> 8 ); 03397 obuf[12] = (unsigned char)( ( *olen - 13 ) ); 03398 03399 return( MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED ); 03400 } 03401 03402 /* 03403 * Handle possible client reconnect with the same UDP quadruplet 03404 * (RFC 6347 Section 4.2.8). 03405 * 03406 * Called by ssl_parse_record_header() in case we receive an epoch 0 record 03407 * that looks like a ClientHello. 03408 * 03409 * - if the input looks like a ClientHello without cookies, 03410 * send back HelloVerifyRequest, then 03411 * return MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED 03412 * - if the input looks like a ClientHello with a valid cookie, 03413 * reset the session of the current context, and 03414 * return MBEDTLS_ERR_SSL_CLIENT_RECONNECT 03415 * - if anything goes wrong, return a specific error code 03416 * 03417 * mbedtls_ssl_read_record() will ignore the record if anything else than 03418 * MBEDTLS_ERR_SSL_CLIENT_RECONNECT or 0 is returned, although this function 03419 * cannot not return 0. 03420 */ 03421 static int ssl_handle_possible_reconnect( mbedtls_ssl_context *ssl ) 03422 { 03423 int ret; 03424 size_t len; 03425 03426 ret = ssl_check_dtls_clihlo_cookie( 03427 ssl->conf->f_cookie_write, 03428 ssl->conf->f_cookie_check, 03429 ssl->conf->p_cookie, 03430 ssl->cli_id, ssl->cli_id_len, 03431 ssl->in_buf, ssl->in_left, 03432 ssl->out_buf, MBEDTLS_SSL_MAX_CONTENT_LEN, &len ); 03433 03434 MBEDTLS_SSL_DEBUG_RET( 2, "ssl_check_dtls_clihlo_cookie", ret ); 03435 03436 if( ret == MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED ) 03437 { 03438 /* Dont check write errors as we can't do anything here. 03439 * If the error is permanent we'll catch it later, 03440 * if it's not, then hopefully it'll work next time. */ 03441 (void) ssl->f_send( ssl->p_bio, ssl->out_buf, len ); 03442 03443 return( MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED ); 03444 } 03445 03446 if( ret == 0 ) 03447 { 03448 /* Got a valid cookie, partially reset context */ 03449 if( ( ret = ssl_session_reset_int( ssl, 1 ) ) != 0 ) 03450 { 03451 MBEDTLS_SSL_DEBUG_RET( 1, "reset", ret ); 03452 return( ret ); 03453 } 03454 03455 return( MBEDTLS_ERR_SSL_CLIENT_RECONNECT ); 03456 } 03457 03458 return( ret ); 03459 } 03460 #endif /* MBEDTLS_SSL_DTLS_CLIENT_PORT_REUSE && MBEDTLS_SSL_SRV_C */ 03461 03462 /* 03463 * ContentType type; 03464 * ProtocolVersion version; 03465 * uint16 epoch; // DTLS only 03466 * uint48 sequence_number; // DTLS only 03467 * uint16 length; 03468 */ 03469 static int ssl_parse_record_header( mbedtls_ssl_context *ssl ) 03470 { 03471 int ret; 03472 int major_ver, minor_ver; 03473 03474 MBEDTLS_SSL_DEBUG_BUF( 4, "input record header", ssl->in_hdr, mbedtls_ssl_hdr_len( ssl ) ); 03475 03476 ssl->in_msgtype = ssl->in_hdr[0]; 03477 ssl->in_msglen = ( ssl->in_len[0] << 8 ) | ssl->in_len[1]; 03478 mbedtls_ssl_read_version( &major_ver, &minor_ver, ssl->conf->transport, ssl->in_hdr + 1 ); 03479 03480 MBEDTLS_SSL_DEBUG_MSG( 3, ( "input record: msgtype = %d, " 03481 "version = [%d:%d], msglen = %d", 03482 ssl->in_msgtype, 03483 major_ver, minor_ver, ssl->in_msglen ) ); 03484 03485 /* Check record type */ 03486 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE && 03487 ssl->in_msgtype != MBEDTLS_SSL_MSG_ALERT && 03488 ssl->in_msgtype != MBEDTLS_SSL_MSG_CHANGE_CIPHER_SPEC && 03489 ssl->in_msgtype != MBEDTLS_SSL_MSG_APPLICATION_DATA ) 03490 { 03491 MBEDTLS_SSL_DEBUG_MSG( 1, ( "unknown record type" ) ); 03492 03493 if( ( ret = mbedtls_ssl_send_alert_message( ssl, 03494 MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03495 MBEDTLS_SSL_ALERT_MSG_UNEXPECTED_MESSAGE ) ) != 0 ) 03496 { 03497 return( ret ); 03498 } 03499 03500 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03501 } 03502 03503 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03504 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03505 { 03506 /* Drop unexpected ChangeCipherSpec messages */ 03507 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_CHANGE_CIPHER_SPEC && 03508 ssl->state != MBEDTLS_SSL_CLIENT_CHANGE_CIPHER_SPEC && 03509 ssl->state != MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC ) 03510 { 03511 MBEDTLS_SSL_DEBUG_MSG( 1, ( "dropping unexpected ChangeCipherSpec" ) ); 03512 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03513 } 03514 03515 /* Drop unexpected ApplicationData records, 03516 * except at the beginning of renegotiations */ 03517 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_APPLICATION_DATA && 03518 ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER 03519 #if defined(MBEDTLS_SSL_RENEGOTIATION) 03520 && ! ( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 03521 ssl->state == MBEDTLS_SSL_SERVER_HELLO ) 03522 #endif 03523 ) 03524 { 03525 MBEDTLS_SSL_DEBUG_MSG( 1, ( "dropping unexpected ApplicationData" ) ); 03526 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03527 } 03528 } 03529 #endif 03530 03531 /* Check version */ 03532 if( major_ver != ssl->major_ver ) 03533 { 03534 MBEDTLS_SSL_DEBUG_MSG( 1, ( "major version mismatch" ) ); 03535 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03536 } 03537 03538 if( minor_ver > ssl->conf->max_minor_ver ) 03539 { 03540 MBEDTLS_SSL_DEBUG_MSG( 1, ( "minor version mismatch" ) ); 03541 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03542 } 03543 03544 /* Check epoch (and sequence number) with DTLS */ 03545 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03546 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03547 { 03548 unsigned int rec_epoch = ( ssl->in_ctr[0] << 8 ) | ssl->in_ctr[1]; 03549 03550 if( rec_epoch != ssl->in_epoch ) 03551 { 03552 MBEDTLS_SSL_DEBUG_MSG( 1, ( "record from another epoch: " 03553 "expected %d, received %d", 03554 ssl->in_epoch, rec_epoch ) ); 03555 03556 #if defined(MBEDTLS_SSL_DTLS_CLIENT_PORT_REUSE) && defined(MBEDTLS_SSL_SRV_C) 03557 /* 03558 * Check for an epoch 0 ClientHello. We can't use in_msg here to 03559 * access the first byte of record content (handshake type), as we 03560 * have an active transform (possibly iv_len != 0), so use the 03561 * fact that the record header len is 13 instead. 03562 */ 03563 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 03564 ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER && 03565 rec_epoch == 0 && 03566 ssl->in_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE && 03567 ssl->in_left > 13 && 03568 ssl->in_buf[13] == MBEDTLS_SSL_HS_CLIENT_HELLO ) 03569 { 03570 MBEDTLS_SSL_DEBUG_MSG( 1, ( "possible client reconnect " 03571 "from the same port" ) ); 03572 return( ssl_handle_possible_reconnect( ssl ) ); 03573 } 03574 else 03575 #endif /* MBEDTLS_SSL_DTLS_CLIENT_PORT_REUSE && MBEDTLS_SSL_SRV_C */ 03576 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03577 } 03578 03579 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 03580 /* Replay detection only works for the current epoch */ 03581 if( rec_epoch == ssl->in_epoch && 03582 mbedtls_ssl_dtls_replay_check( ssl ) != 0 ) 03583 { 03584 MBEDTLS_SSL_DEBUG_MSG( 1, ( "replayed record" ) ); 03585 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03586 } 03587 #endif 03588 } 03589 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 03590 03591 /* Check length against the size of our buffer */ 03592 if( ssl->in_msglen > MBEDTLS_SSL_BUFFER_LEN 03593 - (size_t)( ssl->in_msg - ssl->in_buf ) ) 03594 { 03595 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad message length" ) ); 03596 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03597 } 03598 03599 /* Check length against bounds of the current transform and version */ 03600 if( ssl->transform_in == NULL ) 03601 { 03602 if( ssl->in_msglen < 1 || 03603 ssl->in_msglen > MBEDTLS_SSL_MAX_CONTENT_LEN ) 03604 { 03605 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad message length" ) ); 03606 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03607 } 03608 } 03609 else 03610 { 03611 if( ssl->in_msglen < ssl->transform_in->minlen ) 03612 { 03613 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad message length" ) ); 03614 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03615 } 03616 03617 #if defined(MBEDTLS_SSL_PROTO_SSL3) 03618 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 && 03619 ssl->in_msglen > ssl->transform_in->minlen + MBEDTLS_SSL_MAX_CONTENT_LEN ) 03620 { 03621 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad message length" ) ); 03622 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03623 } 03624 #endif 03625 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 03626 defined(MBEDTLS_SSL_PROTO_TLS1_2) 03627 /* 03628 * TLS encrypted messages can have up to 256 bytes of padding 03629 */ 03630 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_1 && 03631 ssl->in_msglen > ssl->transform_in->minlen + 03632 MBEDTLS_SSL_MAX_CONTENT_LEN + 256 ) 03633 { 03634 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad message length" ) ); 03635 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03636 } 03637 #endif 03638 } 03639 03640 return( 0 ); 03641 } 03642 03643 /* 03644 * If applicable, decrypt (and decompress) record content 03645 */ 03646 static int ssl_prepare_record_content( mbedtls_ssl_context *ssl ) 03647 { 03648 int ret, done = 0; 03649 03650 MBEDTLS_SSL_DEBUG_BUF( 4, "input record from network", 03651 ssl->in_hdr, mbedtls_ssl_hdr_len( ssl ) + ssl->in_msglen ); 03652 03653 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 03654 if( mbedtls_ssl_hw_record_read != NULL ) 03655 { 03656 MBEDTLS_SSL_DEBUG_MSG( 2, ( "going for mbedtls_ssl_hw_record_read()" ) ); 03657 03658 ret = mbedtls_ssl_hw_record_read( ssl ); 03659 if( ret != 0 && ret != MBEDTLS_ERR_SSL_HW_ACCEL_FALLTHROUGH ) 03660 { 03661 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_hw_record_read", ret ); 03662 return( MBEDTLS_ERR_SSL_HW_ACCEL_FAILED ); 03663 } 03664 03665 if( ret == 0 ) 03666 done = 1; 03667 } 03668 #endif /* MBEDTLS_SSL_HW_RECORD_ACCEL */ 03669 if( !done && ssl->transform_in != NULL ) 03670 { 03671 if( ( ret = ssl_decrypt_buf( ssl ) ) != 0 ) 03672 { 03673 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_decrypt_buf", ret ); 03674 return( ret ); 03675 } 03676 03677 MBEDTLS_SSL_DEBUG_BUF( 4, "input payload after decrypt", 03678 ssl->in_msg, ssl->in_msglen ); 03679 03680 if( ssl->in_msglen > MBEDTLS_SSL_MAX_CONTENT_LEN ) 03681 { 03682 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad message length" ) ); 03683 return( MBEDTLS_ERR_SSL_INVALID_RECORD ); 03684 } 03685 } 03686 03687 #if defined(MBEDTLS_ZLIB_SUPPORT) 03688 if( ssl->transform_in != NULL && 03689 ssl->session_in->compression == MBEDTLS_SSL_COMPRESS_DEFLATE ) 03690 { 03691 if( ( ret = ssl_decompress_buf( ssl ) ) != 0 ) 03692 { 03693 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_decompress_buf", ret ); 03694 return( ret ); 03695 } 03696 03697 // TODO: what's the purpose of these lines? is in_len used? 03698 ssl->in_len[0] = (unsigned char)( ssl->in_msglen >> 8 ); 03699 ssl->in_len[1] = (unsigned char)( ssl->in_msglen ); 03700 } 03701 #endif /* MBEDTLS_ZLIB_SUPPORT */ 03702 03703 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 03704 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03705 { 03706 mbedtls_ssl_dtls_replay_update( ssl ); 03707 } 03708 #endif 03709 03710 return( 0 ); 03711 } 03712 03713 static void ssl_handshake_wrapup_free_hs_transform( mbedtls_ssl_context *ssl ); 03714 03715 /* 03716 * Read a record. 03717 * 03718 * Silently ignore non-fatal alert (and for DTLS, invalid records as well, 03719 * RFC 6347 4.1.2.7) and continue reading until a valid record is found. 03720 * 03721 */ 03722 int mbedtls_ssl_read_record( mbedtls_ssl_context *ssl ) 03723 { 03724 int ret; 03725 03726 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> read record" ) ); 03727 03728 if( ssl->in_hslen != 0 && ssl->in_hslen < ssl->in_msglen ) 03729 { 03730 /* 03731 * Get next Handshake message in the current record 03732 */ 03733 ssl->in_msglen -= ssl->in_hslen; 03734 03735 memmove( ssl->in_msg, ssl->in_msg + ssl->in_hslen, 03736 ssl->in_msglen ); 03737 03738 MBEDTLS_SSL_DEBUG_BUF( 4, "remaining content in record", 03739 ssl->in_msg, ssl->in_msglen ); 03740 03741 if( ( ret = ssl_prepare_handshake_record( ssl ) ) != 0 ) 03742 return( ret ); 03743 03744 return( 0 ); 03745 } 03746 03747 ssl->in_hslen = 0; 03748 03749 /* 03750 * Read the record header and parse it 03751 */ 03752 read_record_header: 03753 if( ( ret = mbedtls_ssl_fetch_input( ssl, mbedtls_ssl_hdr_len( ssl ) ) ) != 0 ) 03754 { 03755 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 03756 return( ret ); 03757 } 03758 03759 if( ( ret = ssl_parse_record_header( ssl ) ) != 0 ) 03760 { 03761 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03762 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 03763 ret != MBEDTLS_ERR_SSL_CLIENT_RECONNECT ) 03764 { 03765 /* Ignore bad record and get next one; drop the whole datagram 03766 * since current header cannot be trusted to find the next record 03767 * in current datagram */ 03768 ssl->next_record_offset = 0; 03769 ssl->in_left = 0; 03770 03771 MBEDTLS_SSL_DEBUG_MSG( 1, ( "discarding invalid record (header)" ) ); 03772 goto read_record_header; 03773 } 03774 #endif 03775 return( ret ); 03776 } 03777 03778 /* 03779 * Read and optionally decrypt the message contents 03780 */ 03781 if( ( ret = mbedtls_ssl_fetch_input( ssl, 03782 mbedtls_ssl_hdr_len( ssl ) + ssl->in_msglen ) ) != 0 ) 03783 { 03784 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 03785 return( ret ); 03786 } 03787 03788 /* Done reading this record, get ready for the next one */ 03789 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03790 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03791 ssl->next_record_offset = ssl->in_msglen + mbedtls_ssl_hdr_len( ssl ); 03792 else 03793 #endif 03794 ssl->in_left = 0; 03795 03796 if( ( ret = ssl_prepare_record_content( ssl ) ) != 0 ) 03797 { 03798 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03799 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03800 { 03801 /* Silently discard invalid records */ 03802 if( ret == MBEDTLS_ERR_SSL_INVALID_RECORD || 03803 ret == MBEDTLS_ERR_SSL_INVALID_MAC ) 03804 { 03805 /* Except when waiting for Finished as a bad mac here 03806 * probably means something went wrong in the handshake 03807 * (eg wrong psk used, mitm downgrade attempt, etc.) */ 03808 if( ssl->state == MBEDTLS_SSL_CLIENT_FINISHED || 03809 ssl->state == MBEDTLS_SSL_SERVER_FINISHED ) 03810 { 03811 #if defined(MBEDTLS_SSL_ALL_ALERT_MESSAGES) 03812 if( ret == MBEDTLS_ERR_SSL_INVALID_MAC ) 03813 { 03814 mbedtls_ssl_send_alert_message( ssl, 03815 MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03816 MBEDTLS_SSL_ALERT_MSG_BAD_RECORD_MAC ); 03817 } 03818 #endif 03819 return( ret ); 03820 } 03821 03822 #if defined(MBEDTLS_SSL_DTLS_BADMAC_LIMIT) 03823 if( ssl->conf->badmac_limit != 0 && 03824 ++ssl->badmac_seen >= ssl->conf->badmac_limit ) 03825 { 03826 MBEDTLS_SSL_DEBUG_MSG( 1, ( "too many records with bad MAC" ) ); 03827 return( MBEDTLS_ERR_SSL_INVALID_MAC ); 03828 } 03829 #endif 03830 03831 MBEDTLS_SSL_DEBUG_MSG( 1, ( "discarding invalid record (mac)" ) ); 03832 goto read_record_header; 03833 } 03834 03835 return( ret ); 03836 } 03837 else 03838 #endif 03839 { 03840 /* Error out (and send alert) on invalid records */ 03841 #if defined(MBEDTLS_SSL_ALL_ALERT_MESSAGES) 03842 if( ret == MBEDTLS_ERR_SSL_INVALID_MAC ) 03843 { 03844 mbedtls_ssl_send_alert_message( ssl, 03845 MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03846 MBEDTLS_SSL_ALERT_MSG_BAD_RECORD_MAC ); 03847 } 03848 #endif 03849 return( ret ); 03850 } 03851 } 03852 03853 /* 03854 * When we sent the last flight of the handshake, we MUST respond to a 03855 * retransmit of the peer's previous flight with a retransmit. (In 03856 * practice, only the Finished message will make it, other messages 03857 * including CCS use the old transform so they're dropped as invalid.) 03858 * 03859 * If the record we received is not a handshake message, however, it 03860 * means the peer received our last flight so we can clean up 03861 * handshake info. 03862 * 03863 * This check needs to be done before prepare_handshake() due to an edge 03864 * case: if the client immediately requests renegotiation, this 03865 * finishes the current handshake first, avoiding the new ClientHello 03866 * being mistaken for an ancient message in the current handshake. 03867 */ 03868 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03869 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 03870 ssl->handshake != NULL && 03871 ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER ) 03872 { 03873 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE && 03874 ssl->in_msg[0] == MBEDTLS_SSL_HS_FINISHED ) 03875 { 03876 MBEDTLS_SSL_DEBUG_MSG( 2, ( "received retransmit of last flight" ) ); 03877 03878 if( ( ret = mbedtls_ssl_resend( ssl ) ) != 0 ) 03879 { 03880 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_resend", ret ); 03881 return( ret ); 03882 } 03883 03884 return( MBEDTLS_ERR_SSL_WANT_READ ); 03885 } 03886 else 03887 { 03888 ssl_handshake_wrapup_free_hs_transform( ssl ); 03889 } 03890 } 03891 #endif 03892 03893 /* 03894 * Handle particular types of records 03895 */ 03896 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE ) 03897 { 03898 if( ( ret = ssl_prepare_handshake_record( ssl ) ) != 0 ) 03899 return( ret ); 03900 } 03901 03902 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_ALERT ) 03903 { 03904 MBEDTLS_SSL_DEBUG_MSG( 2, ( "got an alert message, type: [%d:%d]", 03905 ssl->in_msg[0], ssl->in_msg[1] ) ); 03906 03907 /* 03908 * Ignore non-fatal alerts, except close_notify and no_renegotiation 03909 */ 03910 if( ssl->in_msg[0] == MBEDTLS_SSL_ALERT_LEVEL_FATAL ) 03911 { 03912 MBEDTLS_SSL_DEBUG_MSG( 1, ( "is a fatal alert message (msg %d)", 03913 ssl->in_msg[1] ) ); 03914 return( MBEDTLS_ERR_SSL_FATAL_ALERT_MESSAGE ); 03915 } 03916 03917 if( ssl->in_msg[0] == MBEDTLS_SSL_ALERT_LEVEL_WARNING && 03918 ssl->in_msg[1] == MBEDTLS_SSL_ALERT_MSG_CLOSE_NOTIFY ) 03919 { 03920 MBEDTLS_SSL_DEBUG_MSG( 2, ( "is a close notify message" ) ); 03921 return( MBEDTLS_ERR_SSL_PEER_CLOSE_NOTIFY ); 03922 } 03923 03924 #if defined(MBEDTLS_SSL_RENEGOTIATION_ENABLED) 03925 if( ssl->in_msg[0] == MBEDTLS_SSL_ALERT_LEVEL_WARNING && 03926 ssl->in_msg[1] == MBEDTLS_SSL_ALERT_MSG_NO_RENEGOTIATION ) 03927 { 03928 MBEDTLS_SSL_DEBUG_MSG( 2, ( "is a SSLv3 no_cert" ) ); 03929 /* Will be handled when trying to parse ServerHello */ 03930 return( 0 ); 03931 } 03932 #endif 03933 03934 #if defined(MBEDTLS_SSL_PROTO_SSL3) && defined(MBEDTLS_SSL_SRV_C) 03935 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 && 03936 ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 03937 ssl->in_msg[0] == MBEDTLS_SSL_ALERT_LEVEL_WARNING && 03938 ssl->in_msg[1] == MBEDTLS_SSL_ALERT_MSG_NO_CERT ) 03939 { 03940 MBEDTLS_SSL_DEBUG_MSG( 2, ( "is a SSLv3 no_cert" ) ); 03941 /* Will be handled in mbedtls_ssl_parse_certificate() */ 03942 return( 0 ); 03943 } 03944 #endif /* MBEDTLS_SSL_PROTO_SSL3 && MBEDTLS_SSL_SRV_C */ 03945 03946 /* Silently ignore: fetch new message */ 03947 goto read_record_header; 03948 } 03949 03950 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= read record" ) ); 03951 03952 return( 0 ); 03953 } 03954 03955 int mbedtls_ssl_send_fatal_handshake_failure( mbedtls_ssl_context *ssl ) 03956 { 03957 int ret; 03958 03959 if( ( ret = mbedtls_ssl_send_alert_message( ssl, 03960 MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03961 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ) ) != 0 ) 03962 { 03963 return( ret ); 03964 } 03965 03966 return( 0 ); 03967 } 03968 03969 int mbedtls_ssl_send_alert_message( mbedtls_ssl_context *ssl, 03970 unsigned char level, 03971 unsigned char message ) 03972 { 03973 int ret; 03974 03975 if( ssl == NULL || ssl->conf == NULL ) 03976 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 03977 03978 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> send alert message" ) ); 03979 03980 ssl->out_msgtype = MBEDTLS_SSL_MSG_ALERT; 03981 ssl->out_msglen = 2; 03982 ssl->out_msg[0] = level; 03983 ssl->out_msg[1] = message; 03984 03985 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03986 { 03987 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03988 return( ret ); 03989 } 03990 03991 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= send alert message" ) ); 03992 03993 return( 0 ); 03994 } 03995 03996 /* 03997 * Handshake functions 03998 */ 03999 #if !defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) && \ 04000 !defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) && \ 04001 !defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) && \ 04002 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) && \ 04003 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) && \ 04004 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) && \ 04005 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 04006 int mbedtls_ssl_write_certificate( mbedtls_ssl_context *ssl ) 04007 { 04008 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 04009 04010 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate" ) ); 04011 04012 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 04013 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 04014 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 04015 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 04016 { 04017 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate" ) ); 04018 ssl->state++; 04019 return( 0 ); 04020 } 04021 04022 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 04023 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 04024 } 04025 04026 int mbedtls_ssl_parse_certificate( mbedtls_ssl_context *ssl ) 04027 { 04028 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 04029 04030 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate" ) ); 04031 04032 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 04033 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 04034 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 04035 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 04036 { 04037 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate" ) ); 04038 ssl->state++; 04039 return( 0 ); 04040 } 04041 04042 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 04043 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 04044 } 04045 #else 04046 int mbedtls_ssl_write_certificate( mbedtls_ssl_context *ssl ) 04047 { 04048 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 04049 size_t i, n; 04050 const mbedtls_x509_crt *crt; 04051 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 04052 04053 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate" ) ); 04054 04055 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 04056 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 04057 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 04058 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 04059 { 04060 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate" ) ); 04061 ssl->state++; 04062 return( 0 ); 04063 } 04064 04065 #if defined(MBEDTLS_SSL_CLI_C) 04066 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT ) 04067 { 04068 if( ssl->client_auth == 0 ) 04069 { 04070 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate" ) ); 04071 ssl->state++; 04072 return( 0 ); 04073 } 04074 04075 #if defined(MBEDTLS_SSL_PROTO_SSL3) 04076 /* 04077 * If using SSLv3 and got no cert, send an Alert message 04078 * (otherwise an empty Certificate message will be sent). 04079 */ 04080 if( mbedtls_ssl_own_cert( ssl ) == NULL && 04081 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 04082 { 04083 ssl->out_msglen = 2; 04084 ssl->out_msgtype = MBEDTLS_SSL_MSG_ALERT; 04085 ssl->out_msg[0] = MBEDTLS_SSL_ALERT_LEVEL_WARNING; 04086 ssl->out_msg[1] = MBEDTLS_SSL_ALERT_MSG_NO_CERT; 04087 04088 MBEDTLS_SSL_DEBUG_MSG( 2, ( "got no certificate to send" ) ); 04089 goto write_msg; 04090 } 04091 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 04092 } 04093 #endif /* MBEDTLS_SSL_CLI_C */ 04094 #if defined(MBEDTLS_SSL_SRV_C) 04095 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 04096 { 04097 if( mbedtls_ssl_own_cert( ssl ) == NULL ) 04098 { 04099 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no certificate to send" ) ); 04100 return( MBEDTLS_ERR_SSL_CERTIFICATE_REQUIRED ); 04101 } 04102 } 04103 #endif 04104 04105 MBEDTLS_SSL_DEBUG_CRT( 3, "own certificate", mbedtls_ssl_own_cert( ssl ) ); 04106 04107 /* 04108 * 0 . 0 handshake type 04109 * 1 . 3 handshake length 04110 * 4 . 6 length of all certs 04111 * 7 . 9 length of cert. 1 04112 * 10 . n-1 peer certificate 04113 * n . n+2 length of cert. 2 04114 * n+3 . ... upper level cert, etc. 04115 */ 04116 i = 7; 04117 crt = mbedtls_ssl_own_cert( ssl ); 04118 04119 while( crt != NULL ) 04120 { 04121 n = crt->raw.len; 04122 if( n > MBEDTLS_SSL_MAX_CONTENT_LEN - 3 - i ) 04123 { 04124 MBEDTLS_SSL_DEBUG_MSG( 1, ( "certificate too large, %d > %d", 04125 i + 3 + n, MBEDTLS_SSL_MAX_CONTENT_LEN ) ); 04126 return( MBEDTLS_ERR_SSL_CERTIFICATE_TOO_LARGE ); 04127 } 04128 04129 ssl->out_msg[i ] = (unsigned char)( n >> 16 ); 04130 ssl->out_msg[i + 1] = (unsigned char)( n >> 8 ); 04131 ssl->out_msg[i + 2] = (unsigned char)( n ); 04132 04133 i += 3; memcpy( ssl->out_msg + i, crt->raw.p, n ); 04134 i += n; crt = crt->next; 04135 } 04136 04137 ssl->out_msg[4] = (unsigned char)( ( i - 7 ) >> 16 ); 04138 ssl->out_msg[5] = (unsigned char)( ( i - 7 ) >> 8 ); 04139 ssl->out_msg[6] = (unsigned char)( ( i - 7 ) ); 04140 04141 ssl->out_msglen = i; 04142 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 04143 ssl->out_msg[0] = MBEDTLS_SSL_HS_CERTIFICATE; 04144 04145 #if defined(MBEDTLS_SSL_PROTO_SSL3) && defined(MBEDTLS_SSL_CLI_C) 04146 write_msg: 04147 #endif 04148 04149 ssl->state++; 04150 04151 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 04152 { 04153 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 04154 return( ret ); 04155 } 04156 04157 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write certificate" ) ); 04158 04159 return( ret ); 04160 } 04161 04162 int mbedtls_ssl_parse_certificate( mbedtls_ssl_context *ssl ) 04163 { 04164 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 04165 size_t i, n; 04166 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 04167 int authmode = ssl->conf->authmode; 04168 04169 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate" ) ); 04170 04171 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 04172 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 04173 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 04174 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 04175 { 04176 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate" ) ); 04177 ssl->state++; 04178 return( 0 ); 04179 } 04180 04181 #if defined(MBEDTLS_SSL_SRV_C) 04182 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 04183 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 04184 { 04185 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate" ) ); 04186 ssl->state++; 04187 return( 0 ); 04188 } 04189 04190 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 04191 if( ssl->handshake->sni_authmode != MBEDTLS_SSL_VERIFY_UNSET ) 04192 authmode = ssl->handshake->sni_authmode; 04193 #endif 04194 04195 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 04196 authmode == MBEDTLS_SSL_VERIFY_NONE ) 04197 { 04198 ssl->session_negotiate->verify_result = MBEDTLS_X509_BADCERT_SKIP_VERIFY; 04199 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate" ) ); 04200 ssl->state++; 04201 return( 0 ); 04202 } 04203 #endif 04204 04205 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 04206 { 04207 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 04208 return( ret ); 04209 } 04210 04211 ssl->state++; 04212 04213 #if defined(MBEDTLS_SSL_SRV_C) 04214 #if defined(MBEDTLS_SSL_PROTO_SSL3) 04215 /* 04216 * Check if the client sent an empty certificate 04217 */ 04218 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 04219 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 04220 { 04221 if( ssl->in_msglen == 2 && 04222 ssl->in_msgtype == MBEDTLS_SSL_MSG_ALERT && 04223 ssl->in_msg[0] == MBEDTLS_SSL_ALERT_LEVEL_WARNING && 04224 ssl->in_msg[1] == MBEDTLS_SSL_ALERT_MSG_NO_CERT ) 04225 { 04226 MBEDTLS_SSL_DEBUG_MSG( 1, ( "SSLv3 client has no certificate" ) ); 04227 04228 ssl->session_negotiate->verify_result = MBEDTLS_X509_BADCERT_MISSING; 04229 if( authmode == MBEDTLS_SSL_VERIFY_OPTIONAL ) 04230 return( 0 ); 04231 else 04232 return( MBEDTLS_ERR_SSL_NO_CLIENT_CERTIFICATE ); 04233 } 04234 } 04235 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 04236 04237 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 04238 defined(MBEDTLS_SSL_PROTO_TLS1_2) 04239 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 04240 ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_0 ) 04241 { 04242 if( ssl->in_hslen == 3 + mbedtls_ssl_hs_hdr_len( ssl ) && 04243 ssl->in_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE && 04244 ssl->in_msg[0] == MBEDTLS_SSL_HS_CERTIFICATE && 04245 memcmp( ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ), "\0\0\0", 3 ) == 0 ) 04246 { 04247 MBEDTLS_SSL_DEBUG_MSG( 1, ( "TLSv1 client has no certificate" ) ); 04248 04249 ssl->session_negotiate->verify_result = MBEDTLS_X509_BADCERT_MISSING; 04250 if( authmode == MBEDTLS_SSL_VERIFY_OPTIONAL ) 04251 return( 0 ); 04252 else 04253 return( MBEDTLS_ERR_SSL_NO_CLIENT_CERTIFICATE ); 04254 } 04255 } 04256 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 || \ 04257 MBEDTLS_SSL_PROTO_TLS1_2 */ 04258 #endif /* MBEDTLS_SSL_SRV_C */ 04259 04260 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 04261 { 04262 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate message" ) ); 04263 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 04264 } 04265 04266 if( ssl->in_msg[0] != MBEDTLS_SSL_HS_CERTIFICATE || 04267 ssl->in_hslen < mbedtls_ssl_hs_hdr_len( ssl ) + 3 + 3 ) 04268 { 04269 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate message" ) ); 04270 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE ); 04271 } 04272 04273 i = mbedtls_ssl_hs_hdr_len( ssl ); 04274 04275 /* 04276 * Same message structure as in mbedtls_ssl_write_certificate() 04277 */ 04278 n = ( ssl->in_msg[i+1] << 8 ) | ssl->in_msg[i+2]; 04279 04280 if( ssl->in_msg[i] != 0 || 04281 ssl->in_hslen != n + 3 + mbedtls_ssl_hs_hdr_len( ssl ) ) 04282 { 04283 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate message" ) ); 04284 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE ); 04285 } 04286 04287 /* In case we tried to reuse a session but it failed */ 04288 if( ssl->session_negotiate->peer_cert != NULL ) 04289 { 04290 mbedtls_x509_crt_free( ssl->session_negotiate->peer_cert ); 04291 mbedtls_free( ssl->session_negotiate->peer_cert ); 04292 } 04293 04294 if( ( ssl->session_negotiate->peer_cert = mbedtls_calloc( 1, 04295 sizeof( mbedtls_x509_crt ) ) ) == NULL ) 04296 { 04297 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc(%d bytes) failed", 04298 sizeof( mbedtls_x509_crt ) ) ); 04299 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 04300 } 04301 04302 mbedtls_x509_crt_init( ssl->session_negotiate->peer_cert ); 04303 04304 i += 3; 04305 04306 while( i < ssl->in_hslen ) 04307 { 04308 if( ssl->in_msg[i] != 0 ) 04309 { 04310 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate message" ) ); 04311 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE ); 04312 } 04313 04314 n = ( (unsigned int) ssl->in_msg[i + 1] << 8 ) 04315 | (unsigned int) ssl->in_msg[i + 2]; 04316 i += 3; 04317 04318 if( n < 128 || i + n > ssl->in_hslen ) 04319 { 04320 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate message" ) ); 04321 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE ); 04322 } 04323 04324 ret = mbedtls_x509_crt_parse_der( ssl->session_negotiate->peer_cert, 04325 ssl->in_msg + i, n ); 04326 if( ret != 0 ) 04327 { 04328 MBEDTLS_SSL_DEBUG_RET( 1, " mbedtls_x509_crt_parse_der", ret ); 04329 return( ret ); 04330 } 04331 04332 i += n; 04333 } 04334 04335 MBEDTLS_SSL_DEBUG_CRT( 3, "peer certificate", ssl->session_negotiate->peer_cert ); 04336 04337 /* 04338 * On client, make sure the server cert doesn't change during renego to 04339 * avoid "triple handshake" attack: https://secure-resumption.com/ 04340 */ 04341 #if defined(MBEDTLS_SSL_RENEGOTIATION) && defined(MBEDTLS_SSL_CLI_C) 04342 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT && 04343 ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 04344 { 04345 if( ssl->session->peer_cert == NULL ) 04346 { 04347 MBEDTLS_SSL_DEBUG_MSG( 1, ( "new server cert during renegotiation" ) ); 04348 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE ); 04349 } 04350 04351 if( ssl->session->peer_cert->raw.len != 04352 ssl->session_negotiate->peer_cert->raw.len || 04353 memcmp( ssl->session->peer_cert->raw.p, 04354 ssl->session_negotiate->peer_cert->raw.p, 04355 ssl->session->peer_cert->raw.len ) != 0 ) 04356 { 04357 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server cert changed during renegotiation" ) ); 04358 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE ); 04359 } 04360 } 04361 #endif /* MBEDTLS_SSL_RENEGOTIATION && MBEDTLS_SSL_CLI_C */ 04362 04363 if( authmode != MBEDTLS_SSL_VERIFY_NONE ) 04364 { 04365 mbedtls_x509_crt *ca_chain; 04366 mbedtls_x509_crl *ca_crl; 04367 04368 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 04369 if( ssl->handshake->sni_ca_chain != NULL ) 04370 { 04371 ca_chain = ssl->handshake->sni_ca_chain; 04372 ca_crl = ssl->handshake->sni_ca_crl; 04373 } 04374 else 04375 #endif 04376 { 04377 ca_chain = ssl->conf->ca_chain; 04378 ca_crl = ssl->conf->ca_crl; 04379 } 04380 04381 if( ca_chain == NULL ) 04382 { 04383 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no CA chain" ) ); 04384 return( MBEDTLS_ERR_SSL_CA_CHAIN_REQUIRED ); 04385 } 04386 04387 /* 04388 * Main check: verify certificate 04389 */ 04390 ret = mbedtls_x509_crt_verify_with_profile( 04391 ssl->session_negotiate->peer_cert, 04392 ca_chain, ca_crl, 04393 ssl->conf->cert_profile, 04394 ssl->hostname, 04395 &ssl->session_negotiate->verify_result, 04396 ssl->conf->f_vrfy, ssl->conf->p_vrfy ); 04397 04398 if( ret != 0 ) 04399 { 04400 MBEDTLS_SSL_DEBUG_RET( 1, "x509_verify_cert", ret ); 04401 } 04402 04403 /* 04404 * Secondary checks: always done, but change 'ret' only if it was 0 04405 */ 04406 04407 #if defined(MBEDTLS_ECP_C) 04408 { 04409 const mbedtls_pk_context *pk = &ssl->session_negotiate->peer_cert->pk; 04410 04411 /* If certificate uses an EC key, make sure the curve is OK */ 04412 if( mbedtls_pk_can_do( pk, MBEDTLS_PK_ECKEY ) && 04413 mbedtls_ssl_check_curve( ssl, mbedtls_pk_ec( *pk )->grp .id ) != 0 ) 04414 { 04415 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate (EC key curve)" ) ); 04416 if( ret == 0 ) 04417 ret = MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE; 04418 } 04419 } 04420 #endif /* MBEDTLS_ECP_C */ 04421 04422 if( mbedtls_ssl_check_cert_usage( ssl->session_negotiate->peer_cert, 04423 ciphersuite_info, 04424 ! ssl->conf->endpoint, 04425 &ssl->session_negotiate->verify_result ) != 0 ) 04426 { 04427 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate (usage extensions)" ) ); 04428 if( ret == 0 ) 04429 ret = MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE; 04430 } 04431 04432 if( authmode == MBEDTLS_SSL_VERIFY_OPTIONAL ) 04433 ret = 0; 04434 } 04435 04436 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse certificate" ) ); 04437 04438 return( ret ); 04439 } 04440 #endif /* !MBEDTLS_KEY_EXCHANGE_RSA_ENABLED 04441 !MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED 04442 !MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED 04443 !MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED 04444 !MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED 04445 !MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED 04446 !MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 04447 04448 int mbedtls_ssl_write_change_cipher_spec( mbedtls_ssl_context *ssl ) 04449 { 04450 int ret; 04451 04452 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write change cipher spec" ) ); 04453 04454 ssl->out_msgtype = MBEDTLS_SSL_MSG_CHANGE_CIPHER_SPEC; 04455 ssl->out_msglen = 1; 04456 ssl->out_msg[0] = 1; 04457 04458 ssl->state++; 04459 04460 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 04461 { 04462 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 04463 return( ret ); 04464 } 04465 04466 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write change cipher spec" ) ); 04467 04468 return( 0 ); 04469 } 04470 04471 int mbedtls_ssl_parse_change_cipher_spec( mbedtls_ssl_context *ssl ) 04472 { 04473 int ret; 04474 04475 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse change cipher spec" ) ); 04476 04477 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 04478 { 04479 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 04480 return( ret ); 04481 } 04482 04483 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_CHANGE_CIPHER_SPEC ) 04484 { 04485 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad change cipher spec message" ) ); 04486 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 04487 } 04488 04489 if( ssl->in_msglen != 1 || ssl->in_msg[0] != 1 ) 04490 { 04491 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad change cipher spec message" ) ); 04492 return( MBEDTLS_ERR_SSL_BAD_HS_CHANGE_CIPHER_SPEC ); 04493 } 04494 04495 /* 04496 * Switch to our negotiated transform and session parameters for inbound 04497 * data. 04498 */ 04499 MBEDTLS_SSL_DEBUG_MSG( 3, ( "switching to new transform spec for inbound data" ) ); 04500 ssl->transform_in = ssl->transform_negotiate; 04501 ssl->session_in = ssl->session_negotiate; 04502 04503 #if defined(MBEDTLS_SSL_PROTO_DTLS) 04504 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 04505 { 04506 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 04507 ssl_dtls_replay_reset( ssl ); 04508 #endif 04509 04510 /* Increment epoch */ 04511 if( ++ssl->in_epoch == 0 ) 04512 { 04513 MBEDTLS_SSL_DEBUG_MSG( 1, ( "DTLS epoch would wrap" ) ); 04514 return( MBEDTLS_ERR_SSL_COUNTER_WRAPPING ); 04515 } 04516 } 04517 else 04518 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 04519 memset( ssl->in_ctr, 0, 8 ); 04520 04521 /* 04522 * Set the in_msg pointer to the correct location based on IV length 04523 */ 04524 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_2 ) 04525 { 04526 ssl->in_msg = ssl->in_iv + ssl->transform_negotiate->ivlen - 04527 ssl->transform_negotiate->fixed_ivlen; 04528 } 04529 else 04530 ssl->in_msg = ssl->in_iv; 04531 04532 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 04533 if( mbedtls_ssl_hw_record_activate != NULL ) 04534 { 04535 if( ( ret = mbedtls_ssl_hw_record_activate( ssl, MBEDTLS_SSL_CHANNEL_INBOUND ) ) != 0 ) 04536 { 04537 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_hw_record_activate", ret ); 04538 return( MBEDTLS_ERR_SSL_HW_ACCEL_FAILED ); 04539 } 04540 } 04541 #endif 04542 04543 ssl->state++; 04544 04545 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse change cipher spec" ) ); 04546 04547 return( 0 ); 04548 } 04549 04550 void mbedtls_ssl_optimize_checksum( mbedtls_ssl_context *ssl, 04551 const mbedtls_ssl_ciphersuite_t *ciphersuite_info ) 04552 { 04553 ((void) ciphersuite_info); 04554 04555 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 04556 defined(MBEDTLS_SSL_PROTO_TLS1_1) 04557 if( ssl->minor_ver < MBEDTLS_SSL_MINOR_VERSION_3 ) 04558 ssl->handshake->update_checksum = ssl_update_checksum_md5sha1; 04559 else 04560 #endif 04561 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 04562 #if defined(MBEDTLS_SHA512_C) 04563 if( ciphersuite_info->mac == MBEDTLS_MD_SHA384 ) 04564 ssl->handshake->update_checksum = ssl_update_checksum_sha384; 04565 else 04566 #endif 04567 #if defined(MBEDTLS_SHA256_C) 04568 if( ciphersuite_info->mac != MBEDTLS_MD_SHA384 ) 04569 ssl->handshake->update_checksum = ssl_update_checksum_sha256; 04570 else 04571 #endif 04572 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 04573 { 04574 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 04575 return; 04576 } 04577 } 04578 04579 void mbedtls_ssl_reset_checksum( mbedtls_ssl_context *ssl ) 04580 { 04581 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 04582 defined(MBEDTLS_SSL_PROTO_TLS1_1) 04583 mbedtls_md5_starts( &ssl->handshake->fin_md5 ); 04584 mbedtls_sha1_starts( &ssl->handshake->fin_sha1 ); 04585 #endif 04586 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 04587 #if defined(MBEDTLS_SHA256_C) 04588 mbedtls_sha256_starts( &ssl->handshake->fin_sha256, 0 ); 04589 #endif 04590 #if defined(MBEDTLS_SHA512_C) 04591 mbedtls_sha512_starts( &ssl->handshake->fin_sha512, 1 ); 04592 #endif 04593 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 04594 } 04595 04596 static void ssl_update_checksum_start( mbedtls_ssl_context *ssl, 04597 const unsigned char *buf, size_t len ) 04598 { 04599 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 04600 defined(MBEDTLS_SSL_PROTO_TLS1_1) 04601 mbedtls_md5_update( &ssl->handshake->fin_md5 , buf, len ); 04602 mbedtls_sha1_update( &ssl->handshake->fin_sha1, buf, len ); 04603 #endif 04604 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 04605 #if defined(MBEDTLS_SHA256_C) 04606 mbedtls_sha256_update( &ssl->handshake->fin_sha256, buf, len ); 04607 #endif 04608 #if defined(MBEDTLS_SHA512_C) 04609 mbedtls_sha512_update( &ssl->handshake->fin_sha512, buf, len ); 04610 #endif 04611 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 04612 } 04613 04614 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 04615 defined(MBEDTLS_SSL_PROTO_TLS1_1) 04616 static void ssl_update_checksum_md5sha1( mbedtls_ssl_context *ssl, 04617 const unsigned char *buf, size_t len ) 04618 { 04619 mbedtls_md5_update( &ssl->handshake->fin_md5 , buf, len ); 04620 mbedtls_sha1_update( &ssl->handshake->fin_sha1, buf, len ); 04621 } 04622 #endif 04623 04624 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 04625 #if defined(MBEDTLS_SHA256_C) 04626 static void ssl_update_checksum_sha256( mbedtls_ssl_context *ssl, 04627 const unsigned char *buf, size_t len ) 04628 { 04629 mbedtls_sha256_update( &ssl->handshake->fin_sha256, buf, len ); 04630 } 04631 #endif 04632 04633 #if defined(MBEDTLS_SHA512_C) 04634 static void ssl_update_checksum_sha384( mbedtls_ssl_context *ssl, 04635 const unsigned char *buf, size_t len ) 04636 { 04637 mbedtls_sha512_update( &ssl->handshake->fin_sha512, buf, len ); 04638 } 04639 #endif 04640 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 04641 04642 #if defined(MBEDTLS_SSL_PROTO_SSL3) 04643 static void ssl_calc_finished_ssl( 04644 mbedtls_ssl_context *ssl, unsigned char *buf, int from ) 04645 { 04646 const char *sender; 04647 mbedtls_md5_context md5; 04648 mbedtls_sha1_context sha1; 04649 04650 unsigned char padbuf[48]; 04651 unsigned char md5sum[16]; 04652 unsigned char sha1sum[20]; 04653 04654 mbedtls_ssl_session *session = ssl->session_negotiate; 04655 if( !session ) 04656 session = ssl->session; 04657 04658 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc finished ssl" ) ); 04659 04660 mbedtls_md5_init( &md5 ); 04661 mbedtls_sha1_init( &sha1 ); 04662 04663 mbedtls_md5_clone( &md5, &ssl->handshake->fin_md5 ); 04664 mbedtls_sha1_clone( &sha1, &ssl->handshake->fin_sha1 ); 04665 04666 /* 04667 * SSLv3: 04668 * hash = 04669 * MD5( master + pad2 + 04670 * MD5( handshake + sender + master + pad1 ) ) 04671 * + SHA1( master + pad2 + 04672 * SHA1( handshake + sender + master + pad1 ) ) 04673 */ 04674 04675 #if !defined(MBEDTLS_MD5_ALT) 04676 MBEDTLS_SSL_DEBUG_BUF( 4, "finished md5 state", (unsigned char *) 04677 md5.state , sizeof( md5.state ) ); 04678 #endif 04679 04680 #if !defined(MBEDTLS_SHA1_ALT) 04681 MBEDTLS_SSL_DEBUG_BUF( 4, "finished sha1 state", (unsigned char *) 04682 sha1.state , sizeof( sha1.state ) ); 04683 #endif 04684 04685 sender = ( from == MBEDTLS_SSL_IS_CLIENT ) ? "CLNT" 04686 : "SRVR"; 04687 04688 memset( padbuf, 0x36, 48 ); 04689 04690 mbedtls_md5_update( &md5, (const unsigned char *) sender, 4 ); 04691 mbedtls_md5_update( &md5, session->master, 48 ); 04692 mbedtls_md5_update( &md5, padbuf, 48 ); 04693 mbedtls_md5_finish( &md5, md5sum ); 04694 04695 mbedtls_sha1_update( &sha1, (const unsigned char *) sender, 4 ); 04696 mbedtls_sha1_update( &sha1, session->master, 48 ); 04697 mbedtls_sha1_update( &sha1, padbuf, 40 ); 04698 mbedtls_sha1_finish( &sha1, sha1sum ); 04699 04700 memset( padbuf, 0x5C, 48 ); 04701 04702 mbedtls_md5_starts( &md5 ); 04703 mbedtls_md5_update( &md5, session->master, 48 ); 04704 mbedtls_md5_update( &md5, padbuf, 48 ); 04705 mbedtls_md5_update( &md5, md5sum, 16 ); 04706 mbedtls_md5_finish( &md5, buf ); 04707 04708 mbedtls_sha1_starts( &sha1 ); 04709 mbedtls_sha1_update( &sha1, session->master, 48 ); 04710 mbedtls_sha1_update( &sha1, padbuf , 40 ); 04711 mbedtls_sha1_update( &sha1, sha1sum, 20 ); 04712 mbedtls_sha1_finish( &sha1, buf + 16 ); 04713 04714 MBEDTLS_SSL_DEBUG_BUF( 3, "calc finished result", buf, 36 ); 04715 04716 mbedtls_md5_free( &md5 ); 04717 mbedtls_sha1_free( &sha1 ); 04718 04719 mbedtls_zeroize( padbuf, sizeof( padbuf ) ); 04720 mbedtls_zeroize( md5sum, sizeof( md5sum ) ); 04721 mbedtls_zeroize( sha1sum, sizeof( sha1sum ) ); 04722 04723 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc finished" ) ); 04724 } 04725 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 04726 04727 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) 04728 static void ssl_calc_finished_tls( 04729 mbedtls_ssl_context *ssl, unsigned char *buf, int from ) 04730 { 04731 int len = 12; 04732 const char *sender; 04733 mbedtls_md5_context md5; 04734 mbedtls_sha1_context sha1; 04735 unsigned char padbuf[36]; 04736 04737 mbedtls_ssl_session *session = ssl->session_negotiate; 04738 if( !session ) 04739 session = ssl->session; 04740 04741 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc finished tls" ) ); 04742 04743 mbedtls_md5_init( &md5 ); 04744 mbedtls_sha1_init( &sha1 ); 04745 04746 mbedtls_md5_clone( &md5, &ssl->handshake->fin_md5 ); 04747 mbedtls_sha1_clone( &sha1, &ssl->handshake->fin_sha1 ); 04748 04749 /* 04750 * TLSv1: 04751 * hash = PRF( master, finished_label, 04752 * MD5( handshake ) + SHA1( handshake ) )[0..11] 04753 */ 04754 04755 #if !defined(MBEDTLS_MD5_ALT) 04756 MBEDTLS_SSL_DEBUG_BUF( 4, "finished md5 state", (unsigned char *) 04757 md5.state , sizeof( md5.state ) ); 04758 #endif 04759 04760 #if !defined(MBEDTLS_SHA1_ALT) 04761 MBEDTLS_SSL_DEBUG_BUF( 4, "finished sha1 state", (unsigned char *) 04762 sha1.state , sizeof( sha1.state ) ); 04763 #endif 04764 04765 sender = ( from == MBEDTLS_SSL_IS_CLIENT ) 04766 ? "client finished" 04767 : "server finished"; 04768 04769 mbedtls_md5_finish( &md5, padbuf ); 04770 mbedtls_sha1_finish( &sha1, padbuf + 16 ); 04771 04772 ssl->handshake->tls_prf( session->master, 48, sender, 04773 padbuf, 36, buf, len ); 04774 04775 MBEDTLS_SSL_DEBUG_BUF( 3, "calc finished result", buf, len ); 04776 04777 mbedtls_md5_free( &md5 ); 04778 mbedtls_sha1_free( &sha1 ); 04779 04780 mbedtls_zeroize( padbuf, sizeof( padbuf ) ); 04781 04782 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc finished" ) ); 04783 } 04784 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 */ 04785 04786 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 04787 #if defined(MBEDTLS_SHA256_C) 04788 static void ssl_calc_finished_tls_sha256( 04789 mbedtls_ssl_context *ssl, unsigned char *buf, int from ) 04790 { 04791 int len = 12; 04792 const char *sender; 04793 mbedtls_sha256_context sha256; 04794 unsigned char padbuf[32]; 04795 04796 mbedtls_ssl_session *session = ssl->session_negotiate; 04797 if( !session ) 04798 session = ssl->session; 04799 04800 mbedtls_sha256_init( &sha256 ); 04801 04802 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc finished tls sha256" ) ); 04803 04804 mbedtls_sha256_clone( &sha256, &ssl->handshake->fin_sha256 ); 04805 04806 /* 04807 * TLSv1.2: 04808 * hash = PRF( master, finished_label, 04809 * Hash( handshake ) )[0.11] 04810 */ 04811 04812 #if !defined(MBEDTLS_SHA256_ALT) 04813 MBEDTLS_SSL_DEBUG_BUF( 4, "finished sha2 state", (unsigned char *) 04814 sha256.state , sizeof( sha256.state ) ); 04815 #endif 04816 04817 sender = ( from == MBEDTLS_SSL_IS_CLIENT ) 04818 ? "client finished" 04819 : "server finished"; 04820 04821 mbedtls_sha256_finish( &sha256, padbuf ); 04822 04823 ssl->handshake->tls_prf( session->master, 48, sender, 04824 padbuf, 32, buf, len ); 04825 04826 MBEDTLS_SSL_DEBUG_BUF( 3, "calc finished result", buf, len ); 04827 04828 mbedtls_sha256_free( &sha256 ); 04829 04830 mbedtls_zeroize( padbuf, sizeof( padbuf ) ); 04831 04832 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc finished" ) ); 04833 } 04834 #endif /* MBEDTLS_SHA256_C */ 04835 04836 #if defined(MBEDTLS_SHA512_C) 04837 static void ssl_calc_finished_tls_sha384( 04838 mbedtls_ssl_context *ssl, unsigned char *buf, int from ) 04839 { 04840 int len = 12; 04841 const char *sender; 04842 mbedtls_sha512_context sha512; 04843 unsigned char padbuf[48]; 04844 04845 mbedtls_ssl_session *session = ssl->session_negotiate; 04846 if( !session ) 04847 session = ssl->session; 04848 04849 mbedtls_sha512_init( &sha512 ); 04850 04851 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> calc finished tls sha384" ) ); 04852 04853 mbedtls_sha512_clone( &sha512, &ssl->handshake->fin_sha512 ); 04854 04855 /* 04856 * TLSv1.2: 04857 * hash = PRF( master, finished_label, 04858 * Hash( handshake ) )[0.11] 04859 */ 04860 04861 #if !defined(MBEDTLS_SHA512_ALT) 04862 MBEDTLS_SSL_DEBUG_BUF( 4, "finished sha512 state", (unsigned char *) 04863 sha512.state , sizeof( sha512.state ) ); 04864 #endif 04865 04866 sender = ( from == MBEDTLS_SSL_IS_CLIENT ) 04867 ? "client finished" 04868 : "server finished"; 04869 04870 mbedtls_sha512_finish( &sha512, padbuf ); 04871 04872 ssl->handshake->tls_prf( session->master, 48, sender, 04873 padbuf, 48, buf, len ); 04874 04875 MBEDTLS_SSL_DEBUG_BUF( 3, "calc finished result", buf, len ); 04876 04877 mbedtls_sha512_free( &sha512 ); 04878 04879 mbedtls_zeroize( padbuf, sizeof( padbuf ) ); 04880 04881 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= calc finished" ) ); 04882 } 04883 #endif /* MBEDTLS_SHA512_C */ 04884 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 04885 04886 static void ssl_handshake_wrapup_free_hs_transform( mbedtls_ssl_context *ssl ) 04887 { 04888 MBEDTLS_SSL_DEBUG_MSG( 3, ( "=> handshake wrapup: final free" ) ); 04889 04890 /* 04891 * Free our handshake params 04892 */ 04893 mbedtls_ssl_handshake_free( ssl->handshake ); 04894 mbedtls_free( ssl->handshake ); 04895 ssl->handshake = NULL; 04896 04897 /* 04898 * Free the previous transform and swith in the current one 04899 */ 04900 if( ssl->transform ) 04901 { 04902 mbedtls_ssl_transform_free( ssl->transform ); 04903 mbedtls_free( ssl->transform ); 04904 } 04905 ssl->transform = ssl->transform_negotiate; 04906 ssl->transform_negotiate = NULL; 04907 04908 MBEDTLS_SSL_DEBUG_MSG( 3, ( "<= handshake wrapup: final free" ) ); 04909 } 04910 04911 void mbedtls_ssl_handshake_wrapup( mbedtls_ssl_context *ssl ) 04912 { 04913 int resume = ssl->handshake->resume; 04914 04915 MBEDTLS_SSL_DEBUG_MSG( 3, ( "=> handshake wrapup" ) ); 04916 04917 #if defined(MBEDTLS_SSL_RENEGOTIATION) 04918 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 04919 { 04920 ssl->renego_status = MBEDTLS_SSL_RENEGOTIATION_DONE; 04921 ssl->renego_records_seen = 0; 04922 } 04923 #endif 04924 04925 /* 04926 * Free the previous session and switch in the current one 04927 */ 04928 if( ssl->session ) 04929 { 04930 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 04931 /* RFC 7366 3.1: keep the EtM state */ 04932 ssl->session_negotiate->encrypt_then_mac = 04933 ssl->session->encrypt_then_mac; 04934 #endif 04935 04936 mbedtls_ssl_session_free( ssl->session ); 04937 mbedtls_free( ssl->session ); 04938 } 04939 ssl->session = ssl->session_negotiate; 04940 ssl->session_negotiate = NULL; 04941 04942 /* 04943 * Add cache entry 04944 */ 04945 if( ssl->conf->f_set_cache != NULL && 04946 ssl->session->id_len != 0 && 04947 resume == 0 ) 04948 { 04949 if( ssl->conf->f_set_cache( ssl->conf->p_cache, ssl->session ) != 0 ) 04950 MBEDTLS_SSL_DEBUG_MSG( 1, ( "cache did not store session" ) ); 04951 } 04952 04953 #if defined(MBEDTLS_SSL_PROTO_DTLS) 04954 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 04955 ssl->handshake->flight != NULL ) 04956 { 04957 /* Cancel handshake timer */ 04958 ssl_set_timer( ssl, 0 ); 04959 04960 /* Keep last flight around in case we need to resend it: 04961 * we need the handshake and transform structures for that */ 04962 MBEDTLS_SSL_DEBUG_MSG( 3, ( "skip freeing handshake and transform" ) ); 04963 } 04964 else 04965 #endif 04966 ssl_handshake_wrapup_free_hs_transform( ssl ); 04967 04968 ssl->state++; 04969 04970 MBEDTLS_SSL_DEBUG_MSG( 3, ( "<= handshake wrapup" ) ); 04971 } 04972 04973 int mbedtls_ssl_write_finished( mbedtls_ssl_context *ssl ) 04974 { 04975 int ret, hash_len; 04976 04977 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write finished" ) ); 04978 04979 /* 04980 * Set the out_msg pointer to the correct location based on IV length 04981 */ 04982 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_2 ) 04983 { 04984 ssl->out_msg = ssl->out_iv + ssl->transform_negotiate->ivlen - 04985 ssl->transform_negotiate->fixed_ivlen; 04986 } 04987 else 04988 ssl->out_msg = ssl->out_iv; 04989 04990 ssl->handshake->calc_finished( ssl, ssl->out_msg + 4, ssl->conf->endpoint ); 04991 04992 // TODO TLS/1.2 Hash length is determined by cipher suite (Page 63) 04993 hash_len = ( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) ? 36 : 12; 04994 04995 #if defined(MBEDTLS_SSL_RENEGOTIATION) 04996 ssl->verify_data_len = hash_len; 04997 memcpy( ssl->own_verify_data, ssl->out_msg + 4, hash_len ); 04998 #endif 04999 05000 ssl->out_msglen = 4 + hash_len; 05001 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 05002 ssl->out_msg[0] = MBEDTLS_SSL_HS_FINISHED; 05003 05004 /* 05005 * In case of session resuming, invert the client and server 05006 * ChangeCipherSpec messages order. 05007 */ 05008 if( ssl->handshake->resume != 0 ) 05009 { 05010 #if defined(MBEDTLS_SSL_CLI_C) 05011 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT ) 05012 ssl->state = MBEDTLS_SSL_HANDSHAKE_WRAPUP; 05013 #endif 05014 #if defined(MBEDTLS_SSL_SRV_C) 05015 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 05016 ssl->state = MBEDTLS_SSL_CLIENT_CHANGE_CIPHER_SPEC; 05017 #endif 05018 } 05019 else 05020 ssl->state++; 05021 05022 /* 05023 * Switch to our negotiated transform and session parameters for outbound 05024 * data. 05025 */ 05026 MBEDTLS_SSL_DEBUG_MSG( 3, ( "switching to new transform spec for outbound data" ) ); 05027 05028 #if defined(MBEDTLS_SSL_PROTO_DTLS) 05029 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 05030 { 05031 unsigned char i; 05032 05033 /* Remember current epoch settings for resending */ 05034 ssl->handshake->alt_transform_out = ssl->transform_out; 05035 memcpy( ssl->handshake->alt_out_ctr, ssl->out_ctr, 8 ); 05036 05037 /* Set sequence_number to zero */ 05038 memset( ssl->out_ctr + 2, 0, 6 ); 05039 05040 /* Increment epoch */ 05041 for( i = 2; i > 0; i-- ) 05042 if( ++ssl->out_ctr[i - 1] != 0 ) 05043 break; 05044 05045 /* The loop goes to its end iff the counter is wrapping */ 05046 if( i == 0 ) 05047 { 05048 MBEDTLS_SSL_DEBUG_MSG( 1, ( "DTLS epoch would wrap" ) ); 05049 return( MBEDTLS_ERR_SSL_COUNTER_WRAPPING ); 05050 } 05051 } 05052 else 05053 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 05054 memset( ssl->out_ctr, 0, 8 ); 05055 05056 ssl->transform_out = ssl->transform_negotiate; 05057 ssl->session_out = ssl->session_negotiate; 05058 05059 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 05060 if( mbedtls_ssl_hw_record_activate != NULL ) 05061 { 05062 if( ( ret = mbedtls_ssl_hw_record_activate( ssl, MBEDTLS_SSL_CHANNEL_OUTBOUND ) ) != 0 ) 05063 { 05064 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_hw_record_activate", ret ); 05065 return( MBEDTLS_ERR_SSL_HW_ACCEL_FAILED ); 05066 } 05067 } 05068 #endif 05069 05070 #if defined(MBEDTLS_SSL_PROTO_DTLS) 05071 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 05072 mbedtls_ssl_send_flight_completed( ssl ); 05073 #endif 05074 05075 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 05076 { 05077 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 05078 return( ret ); 05079 } 05080 05081 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write finished" ) ); 05082 05083 return( 0 ); 05084 } 05085 05086 #if defined(MBEDTLS_SSL_PROTO_SSL3) 05087 #define SSL_MAX_HASH_LEN 36 05088 #else 05089 #define SSL_MAX_HASH_LEN 12 05090 #endif 05091 05092 int mbedtls_ssl_parse_finished( mbedtls_ssl_context *ssl ) 05093 { 05094 int ret; 05095 unsigned int hash_len; 05096 unsigned char buf[SSL_MAX_HASH_LEN]; 05097 05098 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse finished" ) ); 05099 05100 ssl->handshake->calc_finished( ssl, buf, ssl->conf->endpoint ^ 1 ); 05101 05102 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 05103 { 05104 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 05105 return( ret ); 05106 } 05107 05108 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 05109 { 05110 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad finished message" ) ); 05111 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 05112 } 05113 05114 /* There is currently no ciphersuite using another length with TLS 1.2 */ 05115 #if defined(MBEDTLS_SSL_PROTO_SSL3) 05116 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 05117 hash_len = 36; 05118 else 05119 #endif 05120 hash_len = 12; 05121 05122 if( ssl->in_msg[0] != MBEDTLS_SSL_HS_FINISHED || 05123 ssl->in_hslen != mbedtls_ssl_hs_hdr_len( ssl ) + hash_len ) 05124 { 05125 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad finished message" ) ); 05126 return( MBEDTLS_ERR_SSL_BAD_HS_FINISHED ); 05127 } 05128 05129 if( mbedtls_ssl_safer_memcmp( ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ), 05130 buf, hash_len ) != 0 ) 05131 { 05132 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad finished message" ) ); 05133 return( MBEDTLS_ERR_SSL_BAD_HS_FINISHED ); 05134 } 05135 05136 #if defined(MBEDTLS_SSL_RENEGOTIATION) 05137 ssl->verify_data_len = hash_len; 05138 memcpy( ssl->peer_verify_data, buf, hash_len ); 05139 #endif 05140 05141 if( ssl->handshake->resume != 0 ) 05142 { 05143 #if defined(MBEDTLS_SSL_CLI_C) 05144 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT ) 05145 ssl->state = MBEDTLS_SSL_CLIENT_CHANGE_CIPHER_SPEC; 05146 #endif 05147 #if defined(MBEDTLS_SSL_SRV_C) 05148 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 05149 ssl->state = MBEDTLS_SSL_HANDSHAKE_WRAPUP; 05150 #endif 05151 } 05152 else 05153 ssl->state++; 05154 05155 #if defined(MBEDTLS_SSL_PROTO_DTLS) 05156 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 05157 mbedtls_ssl_recv_flight_completed( ssl ); 05158 #endif 05159 05160 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse finished" ) ); 05161 05162 return( 0 ); 05163 } 05164 05165 static void ssl_handshake_params_init( mbedtls_ssl_handshake_params *handshake ) 05166 { 05167 memset( handshake, 0, sizeof( mbedtls_ssl_handshake_params ) ); 05168 05169 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 05170 defined(MBEDTLS_SSL_PROTO_TLS1_1) 05171 mbedtls_md5_init( &handshake->fin_md5 ); 05172 mbedtls_sha1_init( &handshake->fin_sha1 ); 05173 mbedtls_md5_starts( &handshake->fin_md5 ); 05174 mbedtls_sha1_starts( &handshake->fin_sha1 ); 05175 #endif 05176 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 05177 #if defined(MBEDTLS_SHA256_C) 05178 mbedtls_sha256_init( &handshake->fin_sha256 ); 05179 mbedtls_sha256_starts( &handshake->fin_sha256, 0 ); 05180 #endif 05181 #if defined(MBEDTLS_SHA512_C) 05182 mbedtls_sha512_init( &handshake->fin_sha512 ); 05183 mbedtls_sha512_starts( &handshake->fin_sha512, 1 ); 05184 #endif 05185 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 05186 05187 handshake->update_checksum = ssl_update_checksum_start; 05188 handshake->sig_alg = MBEDTLS_SSL_HASH_SHA1; 05189 05190 #if defined(MBEDTLS_DHM_C) 05191 mbedtls_dhm_init( &handshake->dhm_ctx ); 05192 #endif 05193 #if defined(MBEDTLS_ECDH_C) 05194 mbedtls_ecdh_init( &handshake->ecdh_ctx ); 05195 #endif 05196 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 05197 mbedtls_ecjpake_init( &handshake->ecjpake_ctx ); 05198 #if defined(MBEDTLS_SSL_CLI_C) 05199 handshake->ecjpake_cache = NULL; 05200 handshake->ecjpake_cache_len = 0; 05201 #endif 05202 #endif 05203 05204 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 05205 handshake->sni_authmode = MBEDTLS_SSL_VERIFY_UNSET; 05206 #endif 05207 } 05208 05209 static void ssl_transform_init( mbedtls_ssl_transform *transform ) 05210 { 05211 memset( transform, 0, sizeof(mbedtls_ssl_transform) ); 05212 05213 mbedtls_cipher_init( &transform->cipher_ctx_enc ); 05214 mbedtls_cipher_init( &transform->cipher_ctx_dec ); 05215 05216 mbedtls_md_init( &transform->md_ctx_enc ); 05217 mbedtls_md_init( &transform->md_ctx_dec ); 05218 } 05219 05220 void mbedtls_ssl_session_init( mbedtls_ssl_session *session ) 05221 { 05222 memset( session, 0, sizeof(mbedtls_ssl_session) ); 05223 } 05224 05225 static int ssl_handshake_init( mbedtls_ssl_context *ssl ) 05226 { 05227 /* Clear old handshake information if present */ 05228 if( ssl->transform_negotiate ) 05229 mbedtls_ssl_transform_free( ssl->transform_negotiate ); 05230 if( ssl->session_negotiate ) 05231 mbedtls_ssl_session_free( ssl->session_negotiate ); 05232 if( ssl->handshake ) 05233 mbedtls_ssl_handshake_free( ssl->handshake ); 05234 05235 /* 05236 * Either the pointers are now NULL or cleared properly and can be freed. 05237 * Now allocate missing structures. 05238 */ 05239 if( ssl->transform_negotiate == NULL ) 05240 { 05241 ssl->transform_negotiate = mbedtls_calloc( 1, sizeof(mbedtls_ssl_transform) ); 05242 } 05243 05244 if( ssl->session_negotiate == NULL ) 05245 { 05246 ssl->session_negotiate = mbedtls_calloc( 1, sizeof(mbedtls_ssl_session) ); 05247 } 05248 05249 if( ssl->handshake == NULL ) 05250 { 05251 ssl->handshake = mbedtls_calloc( 1, sizeof(mbedtls_ssl_handshake_params) ); 05252 } 05253 05254 /* All pointers should exist and can be directly freed without issue */ 05255 if( ssl->handshake == NULL || 05256 ssl->transform_negotiate == NULL || 05257 ssl->session_negotiate == NULL ) 05258 { 05259 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc() of ssl sub-contexts failed" ) ); 05260 05261 mbedtls_free( ssl->handshake ); 05262 mbedtls_free( ssl->transform_negotiate ); 05263 mbedtls_free( ssl->session_negotiate ); 05264 05265 ssl->handshake = NULL; 05266 ssl->transform_negotiate = NULL; 05267 ssl->session_negotiate = NULL; 05268 05269 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 05270 } 05271 05272 /* Initialize structures */ 05273 mbedtls_ssl_session_init( ssl->session_negotiate ); 05274 ssl_transform_init( ssl->transform_negotiate ); 05275 ssl_handshake_params_init( ssl->handshake ); 05276 05277 #if defined(MBEDTLS_SSL_PROTO_DTLS) 05278 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 05279 { 05280 ssl->handshake->alt_transform_out = ssl->transform_out; 05281 05282 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT ) 05283 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_PREPARING; 05284 else 05285 ssl->handshake->retransmit_state = MBEDTLS_SSL_RETRANS_WAITING; 05286 05287 ssl_set_timer( ssl, 0 ); 05288 } 05289 #endif 05290 05291 return( 0 ); 05292 } 05293 05294 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) && defined(MBEDTLS_SSL_SRV_C) 05295 /* Dummy cookie callbacks for defaults */ 05296 static int ssl_cookie_write_dummy( void *ctx, 05297 unsigned char **p, unsigned char *end, 05298 const unsigned char *cli_id, size_t cli_id_len ) 05299 { 05300 ((void) ctx); 05301 ((void) p); 05302 ((void) end); 05303 ((void) cli_id); 05304 ((void) cli_id_len); 05305 05306 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 05307 } 05308 05309 static int ssl_cookie_check_dummy( void *ctx, 05310 const unsigned char *cookie, size_t cookie_len, 05311 const unsigned char *cli_id, size_t cli_id_len ) 05312 { 05313 ((void) ctx); 05314 ((void) cookie); 05315 ((void) cookie_len); 05316 ((void) cli_id); 05317 ((void) cli_id_len); 05318 05319 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 05320 } 05321 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY && MBEDTLS_SSL_SRV_C */ 05322 05323 /* 05324 * Initialize an SSL context 05325 */ 05326 void mbedtls_ssl_init( mbedtls_ssl_context *ssl ) 05327 { 05328 memset( ssl, 0, sizeof( mbedtls_ssl_context ) ); 05329 } 05330 05331 /* 05332 * Setup an SSL context 05333 */ 05334 int mbedtls_ssl_setup( mbedtls_ssl_context *ssl, 05335 const mbedtls_ssl_config *conf ) 05336 { 05337 int ret; 05338 const size_t len = MBEDTLS_SSL_BUFFER_LEN; 05339 05340 ssl->conf = conf; 05341 05342 /* 05343 * Prepare base structures 05344 */ 05345 if( ( ssl-> in_buf = mbedtls_calloc( 1, len ) ) == NULL || 05346 ( ssl->out_buf = mbedtls_calloc( 1, len ) ) == NULL ) 05347 { 05348 MBEDTLS_SSL_DEBUG_MSG( 1, ( "alloc(%d bytes) failed", len ) ); 05349 mbedtls_free( ssl->in_buf ); 05350 ssl->in_buf = NULL; 05351 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 05352 } 05353 05354 #if defined(MBEDTLS_SSL_PROTO_DTLS) 05355 if( conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 05356 { 05357 ssl->out_hdr = ssl->out_buf; 05358 ssl->out_ctr = ssl->out_buf + 3; 05359 ssl->out_len = ssl->out_buf + 11; 05360 ssl->out_iv = ssl->out_buf + 13; 05361 ssl->out_msg = ssl->out_buf + 13; 05362 05363 ssl->in_hdr = ssl->in_buf; 05364 ssl->in_ctr = ssl->in_buf + 3; 05365 ssl->in_len = ssl->in_buf + 11; 05366 ssl->in_iv = ssl->in_buf + 13; 05367 ssl->in_msg = ssl->in_buf + 13; 05368 } 05369 else 05370 #endif 05371 { 05372 ssl->out_ctr = ssl->out_buf; 05373 ssl->out_hdr = ssl->out_buf + 8; 05374 ssl->out_len = ssl->out_buf + 11; 05375 ssl->out_iv = ssl->out_buf + 13; 05376 ssl->out_msg = ssl->out_buf + 13; 05377 05378 ssl->in_ctr = ssl->in_buf; 05379 ssl->in_hdr = ssl->in_buf + 8; 05380 ssl->in_len = ssl->in_buf + 11; 05381 ssl->in_iv = ssl->in_buf + 13; 05382 ssl->in_msg = ssl->in_buf + 13; 05383 } 05384 05385 if( ( ret = ssl_handshake_init( ssl ) ) != 0 ) 05386 return( ret ); 05387 05388 return( 0 ); 05389 } 05390 05391 /* 05392 * Reset an initialized and used SSL context for re-use while retaining 05393 * all application-set variables, function pointers and data. 05394 * 05395 * If partial is non-zero, keep data in the input buffer and client ID. 05396 * (Use when a DTLS client reconnects from the same port.) 05397 */ 05398 static int ssl_session_reset_int( mbedtls_ssl_context *ssl, int partial ) 05399 { 05400 int ret; 05401 05402 ssl->state = MBEDTLS_SSL_HELLO_REQUEST; 05403 05404 /* Cancel any possibly running timer */ 05405 ssl_set_timer( ssl, 0 ); 05406 05407 #if defined(MBEDTLS_SSL_RENEGOTIATION) 05408 ssl->renego_status = MBEDTLS_SSL_INITIAL_HANDSHAKE; 05409 ssl->renego_records_seen = 0; 05410 05411 ssl->verify_data_len = 0; 05412 memset( ssl->own_verify_data, 0, MBEDTLS_SSL_VERIFY_DATA_MAX_LEN ); 05413 memset( ssl->peer_verify_data, 0, MBEDTLS_SSL_VERIFY_DATA_MAX_LEN ); 05414 #endif 05415 ssl->secure_renegotiation = MBEDTLS_SSL_LEGACY_RENEGOTIATION; 05416 05417 ssl->in_offt = NULL; 05418 05419 ssl->in_msg = ssl->in_buf + 13; 05420 ssl->in_msgtype = 0; 05421 ssl->in_msglen = 0; 05422 if( partial == 0 ) 05423 ssl->in_left = 0; 05424 #if defined(MBEDTLS_SSL_PROTO_DTLS) 05425 ssl->next_record_offset = 0; 05426 ssl->in_epoch = 0; 05427 #endif 05428 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 05429 ssl_dtls_replay_reset( ssl ); 05430 #endif 05431 05432 ssl->in_hslen = 0; 05433 ssl->nb_zero = 0; 05434 ssl->record_read = 0; 05435 05436 ssl->out_msg = ssl->out_buf + 13; 05437 ssl->out_msgtype = 0; 05438 ssl->out_msglen = 0; 05439 ssl->out_left = 0; 05440 #if defined(MBEDTLS_SSL_CBC_RECORD_SPLITTING) 05441 if( ssl->split_done != MBEDTLS_SSL_CBC_RECORD_SPLITTING_DISABLED ) 05442 ssl->split_done = 0; 05443 #endif 05444 05445 ssl->transform_in = NULL; 05446 ssl->transform_out = NULL; 05447 05448 memset( ssl->out_buf, 0, MBEDTLS_SSL_BUFFER_LEN ); 05449 if( partial == 0 ) 05450 memset( ssl->in_buf, 0, MBEDTLS_SSL_BUFFER_LEN ); 05451 05452 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 05453 if( mbedtls_ssl_hw_record_reset != NULL ) 05454 { 05455 MBEDTLS_SSL_DEBUG_MSG( 2, ( "going for mbedtls_ssl_hw_record_reset()" ) ); 05456 if( ( ret = mbedtls_ssl_hw_record_reset( ssl ) ) != 0 ) 05457 { 05458 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_hw_record_reset", ret ); 05459 return( MBEDTLS_ERR_SSL_HW_ACCEL_FAILED ); 05460 } 05461 } 05462 #endif 05463 05464 if( ssl->transform ) 05465 { 05466 mbedtls_ssl_transform_free( ssl->transform ); 05467 mbedtls_free( ssl->transform ); 05468 ssl->transform = NULL; 05469 } 05470 05471 if( ssl->session ) 05472 { 05473 mbedtls_ssl_session_free( ssl->session ); 05474 mbedtls_free( ssl->session ); 05475 ssl->session = NULL; 05476 } 05477 05478 #if defined(MBEDTLS_SSL_ALPN) 05479 ssl->alpn_chosen = NULL; 05480 #endif 05481 05482 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) && defined(MBEDTLS_SSL_SRV_C) 05483 if( partial == 0 ) 05484 { 05485 mbedtls_free( ssl->cli_id ); 05486 ssl->cli_id = NULL; 05487 ssl->cli_id_len = 0; 05488 } 05489 #endif 05490 05491 if( ( ret = ssl_handshake_init( ssl ) ) != 0 ) 05492 return( ret ); 05493 05494 return( 0 ); 05495 } 05496 05497 /* 05498 * Reset an initialized and used SSL context for re-use while retaining 05499 * all application-set variables, function pointers and data. 05500 */ 05501 int mbedtls_ssl_session_reset( mbedtls_ssl_context *ssl ) 05502 { 05503 return( ssl_session_reset_int( ssl, 0 ) ); 05504 } 05505 05506 /* 05507 * SSL set accessors 05508 */ 05509 void mbedtls_ssl_conf_endpoint( mbedtls_ssl_config *conf, int endpoint ) 05510 { 05511 conf->endpoint = endpoint; 05512 } 05513 05514 void mbedtls_ssl_conf_transport( mbedtls_ssl_config *conf, int transport ) 05515 { 05516 conf->transport = transport; 05517 } 05518 05519 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 05520 void mbedtls_ssl_conf_dtls_anti_replay( mbedtls_ssl_config *conf, char mode ) 05521 { 05522 conf->anti_replay = mode; 05523 } 05524 #endif 05525 05526 #if defined(MBEDTLS_SSL_DTLS_BADMAC_LIMIT) 05527 void mbedtls_ssl_conf_dtls_badmac_limit( mbedtls_ssl_config *conf, unsigned limit ) 05528 { 05529 conf->badmac_limit = limit; 05530 } 05531 #endif 05532 05533 #if defined(MBEDTLS_SSL_PROTO_DTLS) 05534 void mbedtls_ssl_conf_handshake_timeout( mbedtls_ssl_config *conf, uint32_t min, uint32_t max ) 05535 { 05536 conf->hs_timeout_min = min; 05537 conf->hs_timeout_max = max; 05538 } 05539 #endif 05540 05541 void mbedtls_ssl_conf_authmode( mbedtls_ssl_config *conf, int authmode ) 05542 { 05543 conf->authmode = authmode; 05544 } 05545 05546 #if defined(MBEDTLS_X509_CRT_PARSE_C) 05547 void mbedtls_ssl_conf_verify( mbedtls_ssl_config *conf, 05548 int (*f_vrfy)(void *, mbedtls_x509_crt *, int, uint32_t *), 05549 void *p_vrfy ) 05550 { 05551 conf->f_vrfy = f_vrfy; 05552 conf->p_vrfy = p_vrfy; 05553 } 05554 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 05555 05556 void mbedtls_ssl_conf_rng( mbedtls_ssl_config *conf, 05557 int (*f_rng)(void *, unsigned char *, size_t), 05558 void *p_rng ) 05559 { 05560 conf->f_rng = f_rng; 05561 conf->p_rng = p_rng; 05562 } 05563 05564 void mbedtls_ssl_conf_dbg( mbedtls_ssl_config *conf, 05565 void (*f_dbg)(void *, int, const char *, int, const char *), 05566 void *p_dbg ) 05567 { 05568 conf->f_dbg = f_dbg; 05569 conf->p_dbg = p_dbg; 05570 } 05571 05572 void mbedtls_ssl_set_bio( mbedtls_ssl_context *ssl, 05573 void *p_bio, 05574 int (*f_send)(void *, const unsigned char *, size_t), 05575 int (*f_recv)(void *, unsigned char *, size_t), 05576 int (*f_recv_timeout)(void *, unsigned char *, size_t, uint32_t) ) 05577 { 05578 ssl->p_bio = p_bio; 05579 ssl->f_send = f_send; 05580 ssl->f_recv = f_recv; 05581 ssl->f_recv_timeout = f_recv_timeout; 05582 } 05583 05584 void mbedtls_ssl_conf_read_timeout( mbedtls_ssl_config *conf, uint32_t timeout ) 05585 { 05586 conf->read_timeout = timeout; 05587 } 05588 05589 void mbedtls_ssl_set_timer_cb( mbedtls_ssl_context *ssl, 05590 void *p_timer, 05591 void (*f_set_timer)(void *, uint32_t int_ms, uint32_t fin_ms), 05592 int (*f_get_timer)(void *) ) 05593 { 05594 ssl->p_timer = p_timer; 05595 ssl->f_set_timer = f_set_timer; 05596 ssl->f_get_timer = f_get_timer; 05597 05598 /* Make sure we start with no timer running */ 05599 ssl_set_timer( ssl, 0 ); 05600 } 05601 05602 #if defined(MBEDTLS_SSL_SRV_C) 05603 void mbedtls_ssl_conf_session_cache( mbedtls_ssl_config *conf, 05604 void *p_cache, 05605 int (*f_get_cache)(void *, mbedtls_ssl_session *), 05606 int (*f_set_cache)(void *, const mbedtls_ssl_session *) ) 05607 { 05608 conf->p_cache = p_cache; 05609 conf->f_get_cache = f_get_cache; 05610 conf->f_set_cache = f_set_cache; 05611 } 05612 #endif /* MBEDTLS_SSL_SRV_C */ 05613 05614 #if defined(MBEDTLS_SSL_CLI_C) 05615 int mbedtls_ssl_set_session( mbedtls_ssl_context *ssl, const mbedtls_ssl_session *session ) 05616 { 05617 int ret; 05618 05619 if( ssl == NULL || 05620 session == NULL || 05621 ssl->session_negotiate == NULL || 05622 ssl->conf->endpoint != MBEDTLS_SSL_IS_CLIENT ) 05623 { 05624 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05625 } 05626 05627 if( ( ret = ssl_session_copy( ssl->session_negotiate, session ) ) != 0 ) 05628 return( ret ); 05629 05630 ssl->handshake->resume = 1; 05631 05632 return( 0 ); 05633 } 05634 #endif /* MBEDTLS_SSL_CLI_C */ 05635 05636 void mbedtls_ssl_conf_ciphersuites( mbedtls_ssl_config *conf, 05637 const int *ciphersuites ) 05638 { 05639 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_0] = ciphersuites; 05640 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_1] = ciphersuites; 05641 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_2] = ciphersuites; 05642 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_3] = ciphersuites; 05643 } 05644 05645 void mbedtls_ssl_conf_ciphersuites_for_version( mbedtls_ssl_config *conf, 05646 const int *ciphersuites, 05647 int major, int minor ) 05648 { 05649 if( major != MBEDTLS_SSL_MAJOR_VERSION_3 ) 05650 return; 05651 05652 if( minor < MBEDTLS_SSL_MINOR_VERSION_0 || minor > MBEDTLS_SSL_MINOR_VERSION_3 ) 05653 return; 05654 05655 conf->ciphersuite_list [minor] = ciphersuites; 05656 } 05657 05658 #if defined(MBEDTLS_X509_CRT_PARSE_C) 05659 void mbedtls_ssl_conf_cert_profile( mbedtls_ssl_config *conf, 05660 const mbedtls_x509_crt_profile *profile ) 05661 { 05662 conf->cert_profile = profile; 05663 } 05664 05665 /* Append a new keycert entry to a (possibly empty) list */ 05666 static int ssl_append_key_cert( mbedtls_ssl_key_cert **head, 05667 mbedtls_x509_crt *cert, 05668 mbedtls_pk_context *key ) 05669 { 05670 mbedtls_ssl_key_cert *new; 05671 05672 new = mbedtls_calloc( 1, sizeof( mbedtls_ssl_key_cert ) ); 05673 if( new == NULL ) 05674 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 05675 05676 new->cert = cert; 05677 new->key = key; 05678 new->next = NULL; 05679 05680 /* Update head is the list was null, else add to the end */ 05681 if( *head == NULL ) 05682 { 05683 *head = new; 05684 } 05685 else 05686 { 05687 mbedtls_ssl_key_cert *cur = *head; 05688 while( cur->next != NULL ) 05689 cur = cur->next; 05690 cur->next = new; 05691 } 05692 05693 return( 0 ); 05694 } 05695 05696 int mbedtls_ssl_conf_own_cert( mbedtls_ssl_config *conf, 05697 mbedtls_x509_crt *own_cert, 05698 mbedtls_pk_context *pk_key ) 05699 { 05700 return( ssl_append_key_cert( &conf->key_cert , own_cert, pk_key ) ); 05701 } 05702 05703 void mbedtls_ssl_conf_ca_chain( mbedtls_ssl_config *conf, 05704 mbedtls_x509_crt *ca_chain, 05705 mbedtls_x509_crl *ca_crl ) 05706 { 05707 conf->ca_chain = ca_chain; 05708 conf->ca_crl = ca_crl; 05709 } 05710 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 05711 05712 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 05713 int mbedtls_ssl_set_hs_own_cert( mbedtls_ssl_context *ssl, 05714 mbedtls_x509_crt *own_cert, 05715 mbedtls_pk_context *pk_key ) 05716 { 05717 return( ssl_append_key_cert( &ssl->handshake->sni_key_cert, 05718 own_cert, pk_key ) ); 05719 } 05720 05721 void mbedtls_ssl_set_hs_ca_chain( mbedtls_ssl_context *ssl, 05722 mbedtls_x509_crt *ca_chain, 05723 mbedtls_x509_crl *ca_crl ) 05724 { 05725 ssl->handshake->sni_ca_chain = ca_chain; 05726 ssl->handshake->sni_ca_crl = ca_crl; 05727 } 05728 05729 void mbedtls_ssl_set_hs_authmode( mbedtls_ssl_context *ssl, 05730 int authmode ) 05731 { 05732 ssl->handshake->sni_authmode = authmode; 05733 } 05734 #endif /* MBEDTLS_SSL_SERVER_NAME_INDICATION */ 05735 05736 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 05737 /* 05738 * Set EC J-PAKE password for current handshake 05739 */ 05740 int mbedtls_ssl_set_hs_ecjpake_password( mbedtls_ssl_context *ssl, 05741 const unsigned char *pw, 05742 size_t pw_len ) 05743 { 05744 mbedtls_ecjpake_role role; 05745 05746 if( ssl->handshake == NULL && ssl->conf == NULL ) 05747 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05748 05749 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 05750 role = MBEDTLS_ECJPAKE_SERVER; 05751 else 05752 role = MBEDTLS_ECJPAKE_CLIENT; 05753 05754 return( mbedtls_ecjpake_setup( &ssl->handshake->ecjpake_ctx, 05755 role, 05756 MBEDTLS_MD_SHA256, 05757 MBEDTLS_ECP_DP_SECP256R1 , 05758 pw, pw_len ) ); 05759 } 05760 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 05761 05762 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 05763 int mbedtls_ssl_conf_psk( mbedtls_ssl_config *conf, 05764 const unsigned char *psk, size_t psk_len, 05765 const unsigned char *psk_identity, size_t psk_identity_len ) 05766 { 05767 if( psk == NULL || psk_identity == NULL ) 05768 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05769 05770 if( psk_len > MBEDTLS_PSK_MAX_LEN ) 05771 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05772 05773 /* Identity len will be encoded on two bytes */ 05774 if( ( psk_identity_len >> 16 ) != 0 || 05775 psk_identity_len > MBEDTLS_SSL_MAX_CONTENT_LEN ) 05776 { 05777 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05778 } 05779 05780 if( conf->psk != NULL || conf->psk_identity != NULL ) 05781 { 05782 mbedtls_free( conf->psk ); 05783 mbedtls_free( conf->psk_identity ); 05784 conf->psk = NULL; 05785 conf->psk_identity = NULL; 05786 } 05787 05788 if( ( conf->psk = mbedtls_calloc( 1, psk_len ) ) == NULL || 05789 ( conf->psk_identity = mbedtls_calloc( 1, psk_identity_len ) ) == NULL ) 05790 { 05791 mbedtls_free( conf->psk ); 05792 mbedtls_free( conf->psk_identity ); 05793 conf->psk = NULL; 05794 conf->psk_identity = NULL; 05795 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 05796 } 05797 05798 conf->psk_len = psk_len; 05799 conf->psk_identity_len = psk_identity_len; 05800 05801 memcpy( conf->psk , psk, conf->psk_len ); 05802 memcpy( conf->psk_identity , psk_identity, conf->psk_identity_len ); 05803 05804 return( 0 ); 05805 } 05806 05807 int mbedtls_ssl_set_hs_psk( mbedtls_ssl_context *ssl, 05808 const unsigned char *psk, size_t psk_len ) 05809 { 05810 if( psk == NULL || ssl->handshake == NULL ) 05811 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05812 05813 if( psk_len > MBEDTLS_PSK_MAX_LEN ) 05814 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05815 05816 if( ssl->handshake->psk != NULL ) 05817 mbedtls_free( ssl->handshake->psk ); 05818 05819 if( ( ssl->handshake->psk = mbedtls_calloc( 1, psk_len ) ) == NULL ) 05820 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 05821 05822 ssl->handshake->psk_len = psk_len; 05823 memcpy( ssl->handshake->psk, psk, ssl->handshake->psk_len ); 05824 05825 return( 0 ); 05826 } 05827 05828 void mbedtls_ssl_conf_psk_cb( mbedtls_ssl_config *conf, 05829 int (*f_psk)(void *, mbedtls_ssl_context *, const unsigned char *, 05830 size_t), 05831 void *p_psk ) 05832 { 05833 conf->f_psk = f_psk; 05834 conf->p_psk = p_psk; 05835 } 05836 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED */ 05837 05838 #if defined(MBEDTLS_DHM_C) && defined(MBEDTLS_SSL_SRV_C) 05839 int mbedtls_ssl_conf_dh_param( mbedtls_ssl_config *conf, const char *dhm_P, const char *dhm_G ) 05840 { 05841 int ret; 05842 05843 if( ( ret = mbedtls_mpi_read_string( &conf->dhm_P , 16, dhm_P ) ) != 0 || 05844 ( ret = mbedtls_mpi_read_string( &conf->dhm_G , 16, dhm_G ) ) != 0 ) 05845 { 05846 mbedtls_mpi_free( &conf->dhm_P ); 05847 mbedtls_mpi_free( &conf->dhm_G ); 05848 return( ret ); 05849 } 05850 05851 return( 0 ); 05852 } 05853 05854 int mbedtls_ssl_conf_dh_param_ctx( mbedtls_ssl_config *conf, mbedtls_dhm_context *dhm_ctx ) 05855 { 05856 int ret; 05857 05858 if( ( ret = mbedtls_mpi_copy( &conf->dhm_P , &dhm_ctx->P ) ) != 0 || 05859 ( ret = mbedtls_mpi_copy( &conf->dhm_G , &dhm_ctx->G ) ) != 0 ) 05860 { 05861 mbedtls_mpi_free( &conf->dhm_P ); 05862 mbedtls_mpi_free( &conf->dhm_G ); 05863 return( ret ); 05864 } 05865 05866 return( 0 ); 05867 } 05868 #endif /* MBEDTLS_DHM_C && MBEDTLS_SSL_SRV_C */ 05869 05870 #if defined(MBEDTLS_DHM_C) && defined(MBEDTLS_SSL_CLI_C) 05871 /* 05872 * Set the minimum length for Diffie-Hellman parameters 05873 */ 05874 void mbedtls_ssl_conf_dhm_min_bitlen( mbedtls_ssl_config *conf, 05875 unsigned int bitlen ) 05876 { 05877 conf->dhm_min_bitlen = bitlen; 05878 } 05879 #endif /* MBEDTLS_DHM_C && MBEDTLS_SSL_CLI_C */ 05880 05881 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 05882 /* 05883 * Set allowed/preferred hashes for handshake signatures 05884 */ 05885 void mbedtls_ssl_conf_sig_hashes( mbedtls_ssl_config *conf, 05886 const int *hashes ) 05887 { 05888 conf->sig_hashes = hashes; 05889 } 05890 #endif 05891 05892 #if defined(MBEDTLS_ECP_C) 05893 /* 05894 * Set the allowed elliptic curves 05895 */ 05896 void mbedtls_ssl_conf_curves( mbedtls_ssl_config *conf, 05897 const mbedtls_ecp_group_id *curve_list ) 05898 { 05899 conf->curve_list = curve_list; 05900 } 05901 #endif 05902 05903 #if defined(MBEDTLS_X509_CRT_PARSE_C) 05904 int mbedtls_ssl_set_hostname( mbedtls_ssl_context *ssl, const char *hostname ) 05905 { 05906 size_t hostname_len; 05907 05908 if( hostname == NULL ) 05909 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05910 05911 hostname_len = strlen( hostname ); 05912 05913 if( hostname_len + 1 == 0 ) 05914 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05915 05916 if( hostname_len > MBEDTLS_SSL_MAX_HOST_NAME_LEN ) 05917 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05918 05919 ssl->hostname = mbedtls_calloc( 1, hostname_len + 1 ); 05920 05921 if( ssl->hostname == NULL ) 05922 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 05923 05924 memcpy( ssl->hostname, hostname, hostname_len ); 05925 05926 ssl->hostname[hostname_len] = '\0'; 05927 05928 return( 0 ); 05929 } 05930 #endif 05931 05932 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 05933 void mbedtls_ssl_conf_sni( mbedtls_ssl_config *conf, 05934 int (*f_sni)(void *, mbedtls_ssl_context *, 05935 const unsigned char *, size_t), 05936 void *p_sni ) 05937 { 05938 conf->f_sni = f_sni; 05939 conf->p_sni = p_sni; 05940 } 05941 #endif /* MBEDTLS_SSL_SERVER_NAME_INDICATION */ 05942 05943 #if defined(MBEDTLS_SSL_ALPN) 05944 int mbedtls_ssl_conf_alpn_protocols( mbedtls_ssl_config *conf, const char **protos ) 05945 { 05946 size_t cur_len, tot_len; 05947 const char **p; 05948 05949 /* 05950 * "Empty strings MUST NOT be included and byte strings MUST NOT be 05951 * truncated". Check lengths now rather than later. 05952 */ 05953 tot_len = 0; 05954 for( p = protos; *p != NULL; p++ ) 05955 { 05956 cur_len = strlen( *p ); 05957 tot_len += cur_len; 05958 05959 if( cur_len == 0 || cur_len > 255 || tot_len > 65535 ) 05960 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 05961 } 05962 05963 conf->alpn_list = protos; 05964 05965 return( 0 ); 05966 } 05967 05968 const char *mbedtls_ssl_get_alpn_protocol( const mbedtls_ssl_context *ssl ) 05969 { 05970 return( ssl->alpn_chosen ); 05971 } 05972 #endif /* MBEDTLS_SSL_ALPN */ 05973 05974 void mbedtls_ssl_conf_max_version( mbedtls_ssl_config *conf, int major, int minor ) 05975 { 05976 conf->max_major_ver = major; 05977 conf->max_minor_ver = minor; 05978 } 05979 05980 void mbedtls_ssl_conf_min_version( mbedtls_ssl_config *conf, int major, int minor ) 05981 { 05982 conf->min_major_ver = major; 05983 conf->min_minor_ver = minor; 05984 } 05985 05986 #if defined(MBEDTLS_SSL_FALLBACK_SCSV) && defined(MBEDTLS_SSL_CLI_C) 05987 void mbedtls_ssl_conf_fallback( mbedtls_ssl_config *conf, char fallback ) 05988 { 05989 conf->fallback = fallback; 05990 } 05991 #endif 05992 05993 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 05994 void mbedtls_ssl_conf_encrypt_then_mac( mbedtls_ssl_config *conf, char etm ) 05995 { 05996 conf->encrypt_then_mac = etm; 05997 } 05998 #endif 05999 06000 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 06001 void mbedtls_ssl_conf_extended_master_secret( mbedtls_ssl_config *conf, char ems ) 06002 { 06003 conf->extended_ms = ems; 06004 } 06005 #endif 06006 06007 #if defined(MBEDTLS_ARC4_C) 06008 void mbedtls_ssl_conf_arc4_support( mbedtls_ssl_config *conf, char arc4 ) 06009 { 06010 conf->arc4_disabled = arc4; 06011 } 06012 #endif 06013 06014 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 06015 int mbedtls_ssl_conf_max_frag_len( mbedtls_ssl_config *conf, unsigned char mfl_code ) 06016 { 06017 if( mfl_code >= MBEDTLS_SSL_MAX_FRAG_LEN_INVALID || 06018 mfl_code_to_length[mfl_code] > MBEDTLS_SSL_MAX_CONTENT_LEN ) 06019 { 06020 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06021 } 06022 06023 conf->mfl_code = mfl_code; 06024 06025 return( 0 ); 06026 } 06027 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 06028 06029 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 06030 void mbedtls_ssl_conf_truncated_hmac( mbedtls_ssl_config *conf, int truncate ) 06031 { 06032 conf->trunc_hmac = truncate; 06033 } 06034 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 06035 06036 #if defined(MBEDTLS_SSL_CBC_RECORD_SPLITTING) 06037 void mbedtls_ssl_conf_cbc_record_splitting( mbedtls_ssl_config *conf, char split ) 06038 { 06039 conf->cbc_record_splitting = split; 06040 } 06041 #endif 06042 06043 void mbedtls_ssl_conf_legacy_renegotiation( mbedtls_ssl_config *conf, int allow_legacy ) 06044 { 06045 conf->allow_legacy_renegotiation = allow_legacy; 06046 } 06047 06048 #if defined(MBEDTLS_SSL_RENEGOTIATION) 06049 void mbedtls_ssl_conf_renegotiation( mbedtls_ssl_config *conf, int renegotiation ) 06050 { 06051 conf->disable_renegotiation = renegotiation; 06052 } 06053 06054 void mbedtls_ssl_conf_renegotiation_enforced( mbedtls_ssl_config *conf, int max_records ) 06055 { 06056 conf->renego_max_records = max_records; 06057 } 06058 06059 void mbedtls_ssl_conf_renegotiation_period( mbedtls_ssl_config *conf, 06060 const unsigned char period[8] ) 06061 { 06062 memcpy( conf->renego_period , period, 8 ); 06063 } 06064 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 06065 06066 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 06067 #if defined(MBEDTLS_SSL_CLI_C) 06068 void mbedtls_ssl_conf_session_tickets( mbedtls_ssl_config *conf, int use_tickets ) 06069 { 06070 conf->session_tickets = use_tickets; 06071 } 06072 #endif 06073 06074 #if defined(MBEDTLS_SSL_SRV_C) 06075 void mbedtls_ssl_conf_session_tickets_cb( mbedtls_ssl_config *conf, 06076 mbedtls_ssl_ticket_write_t *f_ticket_write, 06077 mbedtls_ssl_ticket_parse_t *f_ticket_parse, 06078 void *p_ticket ) 06079 { 06080 conf->f_ticket_write = f_ticket_write; 06081 conf->f_ticket_parse = f_ticket_parse; 06082 conf->p_ticket = p_ticket; 06083 } 06084 #endif 06085 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 06086 06087 #if defined(MBEDTLS_SSL_EXPORT_KEYS) 06088 void mbedtls_ssl_conf_export_keys_cb( mbedtls_ssl_config *conf, 06089 mbedtls_ssl_export_keys_t *f_export_keys, 06090 void *p_export_keys ) 06091 { 06092 conf->f_export_keys = f_export_keys; 06093 conf->p_export_keys = p_export_keys; 06094 } 06095 #endif 06096 06097 /* 06098 * SSL get accessors 06099 */ 06100 size_t mbedtls_ssl_get_bytes_avail( const mbedtls_ssl_context *ssl ) 06101 { 06102 return( ssl->in_offt == NULL ? 0 : ssl->in_msglen ); 06103 } 06104 06105 uint32_t mbedtls_ssl_get_verify_result( const mbedtls_ssl_context *ssl ) 06106 { 06107 if( ssl->session != NULL ) 06108 return( ssl->session->verify_result ); 06109 06110 if( ssl->session_negotiate != NULL ) 06111 return( ssl->session_negotiate->verify_result ); 06112 06113 return( 0xFFFFFFFF ); 06114 } 06115 06116 const char *mbedtls_ssl_get_ciphersuite( const mbedtls_ssl_context *ssl ) 06117 { 06118 if( ssl == NULL || ssl->session == NULL ) 06119 return( NULL ); 06120 06121 return mbedtls_ssl_get_ciphersuite_name( ssl->session->ciphersuite ); 06122 } 06123 06124 const char *mbedtls_ssl_get_version( const mbedtls_ssl_context *ssl ) 06125 { 06126 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06127 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 06128 { 06129 switch( ssl->minor_ver ) 06130 { 06131 case MBEDTLS_SSL_MINOR_VERSION_2: 06132 return( "DTLSv1.0" ); 06133 06134 case MBEDTLS_SSL_MINOR_VERSION_3: 06135 return( "DTLSv1.2" ); 06136 06137 default: 06138 return( "unknown (DTLS)" ); 06139 } 06140 } 06141 #endif 06142 06143 switch( ssl->minor_ver ) 06144 { 06145 case MBEDTLS_SSL_MINOR_VERSION_0: 06146 return( "SSLv3.0" ); 06147 06148 case MBEDTLS_SSL_MINOR_VERSION_1: 06149 return( "TLSv1.0" ); 06150 06151 case MBEDTLS_SSL_MINOR_VERSION_2: 06152 return( "TLSv1.1" ); 06153 06154 case MBEDTLS_SSL_MINOR_VERSION_3: 06155 return( "TLSv1.2" ); 06156 06157 default: 06158 return( "unknown" ); 06159 } 06160 } 06161 06162 int mbedtls_ssl_get_record_expansion( const mbedtls_ssl_context *ssl ) 06163 { 06164 size_t transform_expansion; 06165 const mbedtls_ssl_transform *transform = ssl->transform_out; 06166 06167 #if defined(MBEDTLS_ZLIB_SUPPORT) 06168 if( ssl->session_out->compression != MBEDTLS_SSL_COMPRESS_NULL ) 06169 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 06170 #endif 06171 06172 if( transform == NULL ) 06173 return( (int) mbedtls_ssl_hdr_len( ssl ) ); 06174 06175 switch( mbedtls_cipher_get_cipher_mode( &transform->cipher_ctx_enc ) ) 06176 { 06177 case MBEDTLS_MODE_GCM: 06178 case MBEDTLS_MODE_CCM: 06179 case MBEDTLS_MODE_STREAM: 06180 transform_expansion = transform->minlen; 06181 break; 06182 06183 case MBEDTLS_MODE_CBC: 06184 transform_expansion = transform->maclen 06185 + mbedtls_cipher_get_block_size( &transform->cipher_ctx_enc ); 06186 break; 06187 06188 default: 06189 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 06190 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 06191 } 06192 06193 return( (int)( mbedtls_ssl_hdr_len( ssl ) + transform_expansion ) ); 06194 } 06195 06196 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 06197 size_t mbedtls_ssl_get_max_frag_len( const mbedtls_ssl_context *ssl ) 06198 { 06199 size_t max_len; 06200 06201 /* 06202 * Assume mfl_code is correct since it was checked when set 06203 */ 06204 max_len = mfl_code_to_length[ssl->conf->mfl_code]; 06205 06206 /* 06207 * Check if a smaller max length was negotiated 06208 */ 06209 if( ssl->session_out != NULL && 06210 mfl_code_to_length[ssl->session_out->mfl_code] < max_len ) 06211 { 06212 max_len = mfl_code_to_length[ssl->session_out->mfl_code]; 06213 } 06214 06215 return max_len; 06216 } 06217 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 06218 06219 #if defined(MBEDTLS_X509_CRT_PARSE_C) 06220 const mbedtls_x509_crt *mbedtls_ssl_get_peer_cert( const mbedtls_ssl_context *ssl ) 06221 { 06222 if( ssl == NULL || ssl->session == NULL ) 06223 return( NULL ); 06224 06225 return( ssl->session->peer_cert ); 06226 } 06227 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 06228 06229 #if defined(MBEDTLS_SSL_CLI_C) 06230 int mbedtls_ssl_get_session( const mbedtls_ssl_context *ssl, mbedtls_ssl_session *dst ) 06231 { 06232 if( ssl == NULL || 06233 dst == NULL || 06234 ssl->session == NULL || 06235 ssl->conf->endpoint != MBEDTLS_SSL_IS_CLIENT ) 06236 { 06237 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06238 } 06239 06240 return( ssl_session_copy( dst, ssl->session ) ); 06241 } 06242 #endif /* MBEDTLS_SSL_CLI_C */ 06243 06244 /* 06245 * Perform a single step of the SSL handshake 06246 */ 06247 int mbedtls_ssl_handshake_step( mbedtls_ssl_context *ssl ) 06248 { 06249 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 06250 06251 if( ssl == NULL || ssl->conf == NULL ) 06252 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06253 06254 #if defined(MBEDTLS_SSL_CLI_C) 06255 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT ) 06256 ret = mbedtls_ssl_handshake_client_step( ssl ); 06257 #endif 06258 #if defined(MBEDTLS_SSL_SRV_C) 06259 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 06260 ret = mbedtls_ssl_handshake_server_step( ssl ); 06261 #endif 06262 06263 return( ret ); 06264 } 06265 06266 /* 06267 * Perform the SSL handshake 06268 */ 06269 int mbedtls_ssl_handshake( mbedtls_ssl_context *ssl ) 06270 { 06271 int ret = 0; 06272 06273 if( ssl == NULL || ssl->conf == NULL ) 06274 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06275 06276 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> handshake" ) ); 06277 06278 while( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER ) 06279 { 06280 ret = mbedtls_ssl_handshake_step( ssl ); 06281 06282 if( ret != 0 ) 06283 break; 06284 } 06285 06286 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= handshake" ) ); 06287 06288 return( ret ); 06289 } 06290 06291 #if defined(MBEDTLS_SSL_RENEGOTIATION) 06292 #if defined(MBEDTLS_SSL_SRV_C) 06293 /* 06294 * Write HelloRequest to request renegotiation on server 06295 */ 06296 static int ssl_write_hello_request( mbedtls_ssl_context *ssl ) 06297 { 06298 int ret; 06299 06300 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write hello request" ) ); 06301 06302 ssl->out_msglen = 4; 06303 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 06304 ssl->out_msg[0] = MBEDTLS_SSL_HS_HELLO_REQUEST; 06305 06306 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 06307 { 06308 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 06309 return( ret ); 06310 } 06311 06312 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write hello request" ) ); 06313 06314 return( 0 ); 06315 } 06316 #endif /* MBEDTLS_SSL_SRV_C */ 06317 06318 /* 06319 * Actually renegotiate current connection, triggered by either: 06320 * - any side: calling mbedtls_ssl_renegotiate(), 06321 * - client: receiving a HelloRequest during mbedtls_ssl_read(), 06322 * - server: receiving any handshake message on server during mbedtls_ssl_read() after 06323 * the initial handshake is completed. 06324 * If the handshake doesn't complete due to waiting for I/O, it will continue 06325 * during the next calls to mbedtls_ssl_renegotiate() or mbedtls_ssl_read() respectively. 06326 */ 06327 static int ssl_start_renegotiation( mbedtls_ssl_context *ssl ) 06328 { 06329 int ret; 06330 06331 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> renegotiate" ) ); 06332 06333 if( ( ret = ssl_handshake_init( ssl ) ) != 0 ) 06334 return( ret ); 06335 06336 /* RFC 6347 4.2.2: "[...] the HelloRequest will have message_seq = 0 and 06337 * the ServerHello will have message_seq = 1" */ 06338 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06339 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 06340 ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_PENDING ) 06341 { 06342 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 06343 ssl->handshake->out_msg_seq = 1; 06344 else 06345 ssl->handshake->in_msg_seq = 1; 06346 } 06347 #endif 06348 06349 ssl->state = MBEDTLS_SSL_HELLO_REQUEST; 06350 ssl->renego_status = MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS; 06351 06352 if( ( ret = mbedtls_ssl_handshake( ssl ) ) != 0 ) 06353 { 06354 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_handshake", ret ); 06355 return( ret ); 06356 } 06357 06358 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= renegotiate" ) ); 06359 06360 return( 0 ); 06361 } 06362 06363 /* 06364 * Renegotiate current connection on client, 06365 * or request renegotiation on server 06366 */ 06367 int mbedtls_ssl_renegotiate( mbedtls_ssl_context *ssl ) 06368 { 06369 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 06370 06371 if( ssl == NULL || ssl->conf == NULL ) 06372 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06373 06374 #if defined(MBEDTLS_SSL_SRV_C) 06375 /* On server, just send the request */ 06376 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER ) 06377 { 06378 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER ) 06379 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06380 06381 ssl->renego_status = MBEDTLS_SSL_RENEGOTIATION_PENDING; 06382 06383 /* Did we already try/start sending HelloRequest? */ 06384 if( ssl->out_left != 0 ) 06385 return( mbedtls_ssl_flush_output( ssl ) ); 06386 06387 return( ssl_write_hello_request( ssl ) ); 06388 } 06389 #endif /* MBEDTLS_SSL_SRV_C */ 06390 06391 #if defined(MBEDTLS_SSL_CLI_C) 06392 /* 06393 * On client, either start the renegotiation process or, 06394 * if already in progress, continue the handshake 06395 */ 06396 if( ssl->renego_status != MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 06397 { 06398 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER ) 06399 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06400 06401 if( ( ret = ssl_start_renegotiation( ssl ) ) != 0 ) 06402 { 06403 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_start_renegotiation", ret ); 06404 return( ret ); 06405 } 06406 } 06407 else 06408 { 06409 if( ( ret = mbedtls_ssl_handshake( ssl ) ) != 0 ) 06410 { 06411 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_handshake", ret ); 06412 return( ret ); 06413 } 06414 } 06415 #endif /* MBEDTLS_SSL_CLI_C */ 06416 06417 return( ret ); 06418 } 06419 06420 /* 06421 * Check record counters and renegotiate if they're above the limit. 06422 */ 06423 static int ssl_check_ctr_renegotiate( mbedtls_ssl_context *ssl ) 06424 { 06425 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER || 06426 ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_PENDING || 06427 ssl->conf->disable_renegotiation == MBEDTLS_SSL_RENEGOTIATION_DISABLED ) 06428 { 06429 return( 0 ); 06430 } 06431 06432 if( memcmp( ssl->in_ctr, ssl->conf->renego_period, 8 ) <= 0 && 06433 memcmp( ssl->out_ctr, ssl->conf->renego_period, 8 ) <= 0 ) 06434 { 06435 return( 0 ); 06436 } 06437 06438 MBEDTLS_SSL_DEBUG_MSG( 1, ( "record counter limit reached: renegotiate" ) ); 06439 return( mbedtls_ssl_renegotiate( ssl ) ); 06440 } 06441 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 06442 06443 /* 06444 * Receive application data decrypted from the SSL layer 06445 */ 06446 int mbedtls_ssl_read( mbedtls_ssl_context *ssl, unsigned char *buf, size_t len ) 06447 { 06448 int ret, record_read = 0; 06449 size_t n; 06450 06451 if( ssl == NULL || ssl->conf == NULL ) 06452 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06453 06454 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> read" ) ); 06455 06456 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06457 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 06458 { 06459 if( ( ret = mbedtls_ssl_flush_output( ssl ) ) != 0 ) 06460 return( ret ); 06461 06462 if( ssl->handshake != NULL && 06463 ssl->handshake->retransmit_state == MBEDTLS_SSL_RETRANS_SENDING ) 06464 { 06465 if( ( ret = mbedtls_ssl_resend( ssl ) ) != 0 ) 06466 return( ret ); 06467 } 06468 } 06469 #endif 06470 06471 #if defined(MBEDTLS_SSL_RENEGOTIATION) 06472 if( ( ret = ssl_check_ctr_renegotiate( ssl ) ) != 0 ) 06473 { 06474 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_check_ctr_renegotiate", ret ); 06475 return( ret ); 06476 } 06477 #endif 06478 06479 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER ) 06480 { 06481 ret = mbedtls_ssl_handshake( ssl ); 06482 if( ret == MBEDTLS_ERR_SSL_WAITING_SERVER_HELLO_RENEGO ) 06483 { 06484 record_read = 1; 06485 } 06486 else if( ret != 0 ) 06487 { 06488 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_handshake", ret ); 06489 return( ret ); 06490 } 06491 } 06492 06493 if( ssl->in_offt == NULL ) 06494 { 06495 /* Start timer if not already running */ 06496 if( ssl->f_get_timer != NULL && 06497 ssl->f_get_timer( ssl->p_timer ) == -1 ) 06498 { 06499 ssl_set_timer( ssl, ssl->conf->read_timeout ); 06500 } 06501 06502 if( ! record_read ) 06503 { 06504 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 06505 { 06506 if( ret == MBEDTLS_ERR_SSL_CONN_EOF ) 06507 return( 0 ); 06508 06509 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 06510 return( ret ); 06511 } 06512 } 06513 06514 if( ssl->in_msglen == 0 && 06515 ssl->in_msgtype == MBEDTLS_SSL_MSG_APPLICATION_DATA ) 06516 { 06517 /* 06518 * OpenSSL sends empty messages to randomize the IV 06519 */ 06520 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 06521 { 06522 if( ret == MBEDTLS_ERR_SSL_CONN_EOF ) 06523 return( 0 ); 06524 06525 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 06526 return( ret ); 06527 } 06528 } 06529 06530 #if defined(MBEDTLS_SSL_RENEGOTIATION) 06531 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_HANDSHAKE ) 06532 { 06533 MBEDTLS_SSL_DEBUG_MSG( 1, ( "received handshake message" ) ); 06534 06535 #if defined(MBEDTLS_SSL_CLI_C) 06536 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT && 06537 ( ssl->in_msg[0] != MBEDTLS_SSL_HS_HELLO_REQUEST || 06538 ssl->in_hslen != mbedtls_ssl_hs_hdr_len( ssl ) ) ) 06539 { 06540 MBEDTLS_SSL_DEBUG_MSG( 1, ( "handshake received (not HelloRequest)" ) ); 06541 06542 /* With DTLS, drop the packet (probably from last handshake) */ 06543 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06544 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 06545 return( MBEDTLS_ERR_SSL_WANT_READ ); 06546 #endif 06547 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 06548 } 06549 06550 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 06551 ssl->in_msg[0] != MBEDTLS_SSL_HS_CLIENT_HELLO ) 06552 { 06553 MBEDTLS_SSL_DEBUG_MSG( 1, ( "handshake received (not ClientHello)" ) ); 06554 06555 /* With DTLS, drop the packet (probably from last handshake) */ 06556 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06557 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 06558 return( MBEDTLS_ERR_SSL_WANT_READ ); 06559 #endif 06560 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 06561 } 06562 #endif 06563 06564 if( ssl->conf->disable_renegotiation == MBEDTLS_SSL_RENEGOTIATION_DISABLED || 06565 ( ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 06566 ssl->conf->allow_legacy_renegotiation == 06567 MBEDTLS_SSL_LEGACY_NO_RENEGOTIATION ) ) 06568 { 06569 MBEDTLS_SSL_DEBUG_MSG( 3, ( "refusing renegotiation, sending alert" ) ); 06570 06571 #if defined(MBEDTLS_SSL_PROTO_SSL3) 06572 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 06573 { 06574 /* 06575 * SSLv3 does not have a "no_renegotiation" alert 06576 */ 06577 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 06578 return( ret ); 06579 } 06580 else 06581 #endif /* MBEDTLS_SSL_PROTO_SSL3 */ 06582 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 06583 defined(MBEDTLS_SSL_PROTO_TLS1_2) 06584 if( ssl->minor_ver >= MBEDTLS_SSL_MINOR_VERSION_1 ) 06585 { 06586 if( ( ret = mbedtls_ssl_send_alert_message( ssl, 06587 MBEDTLS_SSL_ALERT_LEVEL_WARNING, 06588 MBEDTLS_SSL_ALERT_MSG_NO_RENEGOTIATION ) ) != 0 ) 06589 { 06590 return( ret ); 06591 } 06592 } 06593 else 06594 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 || 06595 MBEDTLS_SSL_PROTO_TLS1_2 */ 06596 { 06597 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 06598 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 06599 } 06600 } 06601 else 06602 { 06603 /* DTLS clients need to know renego is server-initiated */ 06604 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06605 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 06606 ssl->conf->endpoint == MBEDTLS_SSL_IS_CLIENT ) 06607 { 06608 ssl->renego_status = MBEDTLS_SSL_RENEGOTIATION_PENDING; 06609 } 06610 #endif 06611 ret = ssl_start_renegotiation( ssl ); 06612 if( ret == MBEDTLS_ERR_SSL_WAITING_SERVER_HELLO_RENEGO ) 06613 { 06614 record_read = 1; 06615 } 06616 else if( ret != 0 ) 06617 { 06618 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_start_renegotiation", ret ); 06619 return( ret ); 06620 } 06621 } 06622 06623 /* If a non-handshake record was read during renego, fallthrough, 06624 * else tell the user they should call mbedtls_ssl_read() again */ 06625 if( ! record_read ) 06626 return( MBEDTLS_ERR_SSL_WANT_READ ); 06627 } 06628 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_PENDING ) 06629 { 06630 06631 if( ssl->conf->renego_max_records >= 0 ) 06632 { 06633 if( ++ssl->renego_records_seen > ssl->conf->renego_max_records ) 06634 { 06635 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation requested, " 06636 "but not honored by client" ) ); 06637 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 06638 } 06639 } 06640 } 06641 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 06642 06643 /* Fatal and closure alerts handled by mbedtls_ssl_read_record() */ 06644 if( ssl->in_msgtype == MBEDTLS_SSL_MSG_ALERT ) 06645 { 06646 MBEDTLS_SSL_DEBUG_MSG( 2, ( "ignoring non-fatal non-closure alert" ) ); 06647 return( MBEDTLS_ERR_SSL_WANT_READ ); 06648 } 06649 06650 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_APPLICATION_DATA ) 06651 { 06652 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad application data message" ) ); 06653 return( MBEDTLS_ERR_SSL_UNEXPECTED_MESSAGE ); 06654 } 06655 06656 ssl->in_offt = ssl->in_msg; 06657 06658 /* We're going to return something now, cancel timer, 06659 * except if handshake (renegotiation) is in progress */ 06660 if( ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER ) 06661 ssl_set_timer( ssl, 0 ); 06662 06663 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06664 /* If we requested renego but received AppData, resend HelloRequest. 06665 * Do it now, after setting in_offt, to avoid taking this branch 06666 * again if ssl_write_hello_request() returns WANT_WRITE */ 06667 #if defined(MBEDTLS_SSL_SRV_C) && defined(MBEDTLS_SSL_RENEGOTIATION) 06668 if( ssl->conf->endpoint == MBEDTLS_SSL_IS_SERVER && 06669 ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_PENDING ) 06670 { 06671 if( ( ret = ssl_resend_hello_request( ssl ) ) != 0 ) 06672 { 06673 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_resend_hello_request", ret ); 06674 return( ret ); 06675 } 06676 } 06677 #endif /* MBEDTLS_SSL_SRV_C && MBEDTLS_SSL_RENEGOTIATION */ 06678 #endif 06679 } 06680 06681 n = ( len < ssl->in_msglen ) 06682 ? len : ssl->in_msglen; 06683 06684 memcpy( buf, ssl->in_offt, n ); 06685 ssl->in_msglen -= n; 06686 06687 if( ssl->in_msglen == 0 ) 06688 /* all bytes consumed */ 06689 ssl->in_offt = NULL; 06690 else 06691 /* more data available */ 06692 ssl->in_offt += n; 06693 06694 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= read" ) ); 06695 06696 return( (int) n ); 06697 } 06698 06699 /* 06700 * Send application data to be encrypted by the SSL layer, 06701 * taking care of max fragment length and buffer size 06702 */ 06703 static int ssl_write_real( mbedtls_ssl_context *ssl, 06704 const unsigned char *buf, size_t len ) 06705 { 06706 int ret; 06707 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 06708 size_t max_len = mbedtls_ssl_get_max_frag_len( ssl ); 06709 06710 if( len > max_len ) 06711 { 06712 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06713 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 06714 { 06715 MBEDTLS_SSL_DEBUG_MSG( 1, ( "fragment larger than the (negotiated) " 06716 "maximum fragment length: %d > %d", 06717 len, max_len ) ); 06718 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06719 } 06720 else 06721 #endif 06722 len = max_len; 06723 } 06724 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 06725 06726 if( ssl->out_left != 0 ) 06727 { 06728 if( ( ret = mbedtls_ssl_flush_output( ssl ) ) != 0 ) 06729 { 06730 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_flush_output", ret ); 06731 return( ret ); 06732 } 06733 } 06734 else 06735 { 06736 ssl->out_msglen = len; 06737 ssl->out_msgtype = MBEDTLS_SSL_MSG_APPLICATION_DATA; 06738 memcpy( ssl->out_msg, buf, len ); 06739 06740 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 06741 { 06742 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 06743 return( ret ); 06744 } 06745 } 06746 06747 return( (int) len ); 06748 } 06749 06750 /* 06751 * Write application data, doing 1/n-1 splitting if necessary. 06752 * 06753 * With non-blocking I/O, ssl_write_real() may return WANT_WRITE, 06754 * then the caller will call us again with the same arguments, so 06755 * remember wether we already did the split or not. 06756 */ 06757 #if defined(MBEDTLS_SSL_CBC_RECORD_SPLITTING) 06758 static int ssl_write_split( mbedtls_ssl_context *ssl, 06759 const unsigned char *buf, size_t len ) 06760 { 06761 int ret; 06762 06763 if( ssl->conf->cbc_record_splitting == 06764 MBEDTLS_SSL_CBC_RECORD_SPLITTING_DISABLED || 06765 len <= 1 || 06766 ssl->minor_ver > MBEDTLS_SSL_MINOR_VERSION_1 || 06767 mbedtls_cipher_get_cipher_mode( &ssl->transform_out->cipher_ctx_enc ) 06768 != MBEDTLS_MODE_CBC ) 06769 { 06770 return( ssl_write_real( ssl, buf, len ) ); 06771 } 06772 06773 if( ssl->split_done == 0 ) 06774 { 06775 if( ( ret = ssl_write_real( ssl, buf, 1 ) ) <= 0 ) 06776 return( ret ); 06777 ssl->split_done = 1; 06778 } 06779 06780 if( ( ret = ssl_write_real( ssl, buf + 1, len - 1 ) ) <= 0 ) 06781 return( ret ); 06782 ssl->split_done = 0; 06783 06784 return( ret + 1 ); 06785 } 06786 #endif /* MBEDTLS_SSL_CBC_RECORD_SPLITTING */ 06787 06788 /* 06789 * Write application data (public-facing wrapper) 06790 */ 06791 int mbedtls_ssl_write( mbedtls_ssl_context *ssl, const unsigned char *buf, size_t len ) 06792 { 06793 int ret; 06794 06795 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write" ) ); 06796 06797 if( ssl == NULL || ssl->conf == NULL ) 06798 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06799 06800 #if defined(MBEDTLS_SSL_RENEGOTIATION) 06801 if( ( ret = ssl_check_ctr_renegotiate( ssl ) ) != 0 ) 06802 { 06803 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_check_ctr_renegotiate", ret ); 06804 return( ret ); 06805 } 06806 #endif 06807 06808 if( ssl->state != MBEDTLS_SSL_HANDSHAKE_OVER ) 06809 { 06810 if( ( ret = mbedtls_ssl_handshake( ssl ) ) != 0 ) 06811 { 06812 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_handshake", ret ); 06813 return( ret ); 06814 } 06815 } 06816 06817 #if defined(MBEDTLS_SSL_CBC_RECORD_SPLITTING) 06818 ret = ssl_write_split( ssl, buf, len ); 06819 #else 06820 ret = ssl_write_real( ssl, buf, len ); 06821 #endif 06822 06823 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write" ) ); 06824 06825 return( ret ); 06826 } 06827 06828 /* 06829 * Notify the peer that the connection is being closed 06830 */ 06831 int mbedtls_ssl_close_notify( mbedtls_ssl_context *ssl ) 06832 { 06833 int ret; 06834 06835 if( ssl == NULL || ssl->conf == NULL ) 06836 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 06837 06838 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write close notify" ) ); 06839 06840 if( ssl->out_left != 0 ) 06841 return( mbedtls_ssl_flush_output( ssl ) ); 06842 06843 if( ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER ) 06844 { 06845 if( ( ret = mbedtls_ssl_send_alert_message( ssl, 06846 MBEDTLS_SSL_ALERT_LEVEL_WARNING, 06847 MBEDTLS_SSL_ALERT_MSG_CLOSE_NOTIFY ) ) != 0 ) 06848 { 06849 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_send_alert_message", ret ); 06850 return( ret ); 06851 } 06852 } 06853 06854 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write close notify" ) ); 06855 06856 return( 0 ); 06857 } 06858 06859 void mbedtls_ssl_transform_free( mbedtls_ssl_transform *transform ) 06860 { 06861 if( transform == NULL ) 06862 return; 06863 06864 #if defined(MBEDTLS_ZLIB_SUPPORT) 06865 deflateEnd( &transform->ctx_deflate ); 06866 inflateEnd( &transform->ctx_inflate ); 06867 #endif 06868 06869 mbedtls_cipher_free( &transform->cipher_ctx_enc ); 06870 mbedtls_cipher_free( &transform->cipher_ctx_dec ); 06871 06872 mbedtls_md_free( &transform->md_ctx_enc ); 06873 mbedtls_md_free( &transform->md_ctx_dec ); 06874 06875 mbedtls_zeroize( transform, sizeof( mbedtls_ssl_transform ) ); 06876 } 06877 06878 #if defined(MBEDTLS_X509_CRT_PARSE_C) 06879 static void ssl_key_cert_free( mbedtls_ssl_key_cert *key_cert ) 06880 { 06881 mbedtls_ssl_key_cert *cur = key_cert, *next; 06882 06883 while( cur != NULL ) 06884 { 06885 next = cur->next; 06886 mbedtls_free( cur ); 06887 cur = next; 06888 } 06889 } 06890 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 06891 06892 void mbedtls_ssl_handshake_free( mbedtls_ssl_handshake_params *handshake ) 06893 { 06894 if( handshake == NULL ) 06895 return; 06896 06897 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 06898 defined(MBEDTLS_SSL_PROTO_TLS1_1) 06899 mbedtls_md5_free( &handshake->fin_md5 ); 06900 mbedtls_sha1_free( &handshake->fin_sha1 ); 06901 #endif 06902 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 06903 #if defined(MBEDTLS_SHA256_C) 06904 mbedtls_sha256_free( &handshake->fin_sha256 ); 06905 #endif 06906 #if defined(MBEDTLS_SHA512_C) 06907 mbedtls_sha512_free( &handshake->fin_sha512 ); 06908 #endif 06909 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 06910 06911 #if defined(MBEDTLS_DHM_C) 06912 mbedtls_dhm_free( &handshake->dhm_ctx ); 06913 #endif 06914 #if defined(MBEDTLS_ECDH_C) 06915 mbedtls_ecdh_free( &handshake->ecdh_ctx ); 06916 #endif 06917 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 06918 mbedtls_ecjpake_free( &handshake->ecjpake_ctx ); 06919 #if defined(MBEDTLS_SSL_CLI_C) 06920 mbedtls_free( handshake->ecjpake_cache ); 06921 handshake->ecjpake_cache = NULL; 06922 handshake->ecjpake_cache_len = 0; 06923 #endif 06924 #endif 06925 06926 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) 06927 /* explicit void pointer cast for buggy MS compiler */ 06928 mbedtls_free( (void *) handshake->curves ); 06929 #endif 06930 06931 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 06932 if( handshake->psk != NULL ) 06933 { 06934 mbedtls_zeroize( handshake->psk, handshake->psk_len ); 06935 mbedtls_free( handshake->psk ); 06936 } 06937 #endif 06938 06939 #if defined(MBEDTLS_X509_CRT_PARSE_C) && \ 06940 defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 06941 /* 06942 * Free only the linked list wrapper, not the keys themselves 06943 * since the belong to the SNI callback 06944 */ 06945 if( handshake->sni_key_cert != NULL ) 06946 { 06947 mbedtls_ssl_key_cert *cur = handshake->sni_key_cert, *next; 06948 06949 while( cur != NULL ) 06950 { 06951 next = cur->next; 06952 mbedtls_free( cur ); 06953 cur = next; 06954 } 06955 } 06956 #endif /* MBEDTLS_X509_CRT_PARSE_C && MBEDTLS_SSL_SERVER_NAME_INDICATION */ 06957 06958 #if defined(MBEDTLS_SSL_PROTO_DTLS) 06959 mbedtls_free( handshake->verify_cookie ); 06960 mbedtls_free( handshake->hs_msg ); 06961 ssl_flight_free( handshake->flight ); 06962 #endif 06963 06964 mbedtls_zeroize( handshake, sizeof( mbedtls_ssl_handshake_params ) ); 06965 } 06966 06967 void mbedtls_ssl_session_free( mbedtls_ssl_session *session ) 06968 { 06969 if( session == NULL ) 06970 return; 06971 06972 #if defined(MBEDTLS_X509_CRT_PARSE_C) 06973 if( session->peer_cert != NULL ) 06974 { 06975 mbedtls_x509_crt_free( session->peer_cert ); 06976 mbedtls_free( session->peer_cert ); 06977 } 06978 #endif 06979 06980 #if defined(MBEDTLS_SSL_SESSION_TICKETS) && defined(MBEDTLS_SSL_CLI_C) 06981 mbedtls_free( session->ticket ); 06982 #endif 06983 06984 mbedtls_zeroize( session, sizeof( mbedtls_ssl_session ) ); 06985 } 06986 06987 /* 06988 * Free an SSL context 06989 */ 06990 void mbedtls_ssl_free( mbedtls_ssl_context *ssl ) 06991 { 06992 if( ssl == NULL ) 06993 return; 06994 06995 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> free" ) ); 06996 06997 if( ssl->out_buf != NULL ) 06998 { 06999 mbedtls_zeroize( ssl->out_buf, MBEDTLS_SSL_BUFFER_LEN ); 07000 mbedtls_free( ssl->out_buf ); 07001 } 07002 07003 if( ssl->in_buf != NULL ) 07004 { 07005 mbedtls_zeroize( ssl->in_buf, MBEDTLS_SSL_BUFFER_LEN ); 07006 mbedtls_free( ssl->in_buf ); 07007 } 07008 07009 #if defined(MBEDTLS_ZLIB_SUPPORT) 07010 if( ssl->compress_buf != NULL ) 07011 { 07012 mbedtls_zeroize( ssl->compress_buf, MBEDTLS_SSL_BUFFER_LEN ); 07013 mbedtls_free( ssl->compress_buf ); 07014 } 07015 #endif 07016 07017 if( ssl->transform ) 07018 { 07019 mbedtls_ssl_transform_free( ssl->transform ); 07020 mbedtls_free( ssl->transform ); 07021 } 07022 07023 if( ssl->handshake ) 07024 { 07025 mbedtls_ssl_handshake_free( ssl->handshake ); 07026 mbedtls_ssl_transform_free( ssl->transform_negotiate ); 07027 mbedtls_ssl_session_free( ssl->session_negotiate ); 07028 07029 mbedtls_free( ssl->handshake ); 07030 mbedtls_free( ssl->transform_negotiate ); 07031 mbedtls_free( ssl->session_negotiate ); 07032 } 07033 07034 if( ssl->session ) 07035 { 07036 mbedtls_ssl_session_free( ssl->session ); 07037 mbedtls_free( ssl->session ); 07038 } 07039 07040 #if defined(MBEDTLS_X509_CRT_PARSE_C) 07041 if( ssl->hostname != NULL ) 07042 { 07043 mbedtls_zeroize( ssl->hostname, strlen( ssl->hostname ) ); 07044 mbedtls_free( ssl->hostname ); 07045 } 07046 #endif 07047 07048 #if defined(MBEDTLS_SSL_HW_RECORD_ACCEL) 07049 if( mbedtls_ssl_hw_record_finish != NULL ) 07050 { 07051 MBEDTLS_SSL_DEBUG_MSG( 2, ( "going for mbedtls_ssl_hw_record_finish()" ) ); 07052 mbedtls_ssl_hw_record_finish( ssl ); 07053 } 07054 #endif 07055 07056 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) && defined(MBEDTLS_SSL_SRV_C) 07057 mbedtls_free( ssl->cli_id ); 07058 #endif 07059 07060 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= free" ) ); 07061 07062 /* Actually clear after last debug message */ 07063 mbedtls_zeroize( ssl, sizeof( mbedtls_ssl_context ) ); 07064 } 07065 07066 /* 07067 * Initialze mbedtls_ssl_config 07068 */ 07069 void mbedtls_ssl_config_init( mbedtls_ssl_config *conf ) 07070 { 07071 memset( conf, 0, sizeof( mbedtls_ssl_config ) ); 07072 } 07073 07074 static int ssl_preset_suiteb_ciphersuites[] = { 07075 MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256, 07076 MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_GCM_SHA384, 07077 0 07078 }; 07079 07080 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 07081 static int ssl_preset_suiteb_hashes[] = { 07082 MBEDTLS_MD_SHA256, 07083 MBEDTLS_MD_SHA384, 07084 MBEDTLS_MD_NONE 07085 }; 07086 #endif 07087 07088 #if defined(MBEDTLS_ECP_C) 07089 static mbedtls_ecp_group_id ssl_preset_suiteb_curves[] = { 07090 MBEDTLS_ECP_DP_SECP256R1 , 07091 MBEDTLS_ECP_DP_SECP384R1 , 07092 MBEDTLS_ECP_DP_NONE 07093 }; 07094 #endif 07095 07096 /* 07097 * Load default in mbedtls_ssl_config 07098 */ 07099 int mbedtls_ssl_config_defaults( mbedtls_ssl_config *conf, 07100 int endpoint, int transport, int preset ) 07101 { 07102 #if defined(MBEDTLS_DHM_C) && defined(MBEDTLS_SSL_SRV_C) 07103 int ret; 07104 #endif 07105 07106 /* Use the functions here so that they are covered in tests, 07107 * but otherwise access member directly for efficiency */ 07108 mbedtls_ssl_conf_endpoint( conf, endpoint ); 07109 mbedtls_ssl_conf_transport( conf, transport ); 07110 07111 /* 07112 * Things that are common to all presets 07113 */ 07114 #if defined(MBEDTLS_SSL_CLI_C) 07115 if( endpoint == MBEDTLS_SSL_IS_CLIENT ) 07116 { 07117 conf->authmode = MBEDTLS_SSL_VERIFY_REQUIRED; 07118 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 07119 conf->session_tickets = MBEDTLS_SSL_SESSION_TICKETS_ENABLED; 07120 #endif 07121 } 07122 #endif 07123 07124 #if defined(MBEDTLS_ARC4_C) 07125 conf->arc4_disabled = MBEDTLS_SSL_ARC4_DISABLED; 07126 #endif 07127 07128 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 07129 conf->encrypt_then_mac = MBEDTLS_SSL_ETM_ENABLED; 07130 #endif 07131 07132 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 07133 conf->extended_ms = MBEDTLS_SSL_EXTENDED_MS_ENABLED; 07134 #endif 07135 07136 #if defined(MBEDTLS_SSL_CBC_RECORD_SPLITTING) 07137 conf->cbc_record_splitting = MBEDTLS_SSL_CBC_RECORD_SPLITTING_ENABLED; 07138 #endif 07139 07140 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) && defined(MBEDTLS_SSL_SRV_C) 07141 conf->f_cookie_write = ssl_cookie_write_dummy; 07142 conf->f_cookie_check = ssl_cookie_check_dummy; 07143 #endif 07144 07145 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 07146 conf->anti_replay = MBEDTLS_SSL_ANTI_REPLAY_ENABLED; 07147 #endif 07148 07149 #if defined(MBEDTLS_SSL_PROTO_DTLS) 07150 conf->hs_timeout_min = MBEDTLS_SSL_DTLS_TIMEOUT_DFL_MIN; 07151 conf->hs_timeout_max = MBEDTLS_SSL_DTLS_TIMEOUT_DFL_MAX; 07152 #endif 07153 07154 #if defined(MBEDTLS_SSL_RENEGOTIATION) 07155 conf->renego_max_records = MBEDTLS_SSL_RENEGO_MAX_RECORDS_DEFAULT; 07156 memset( conf->renego_period , 0xFF, 7 ); 07157 conf->renego_period [7] = 0x00; 07158 #endif 07159 07160 #if defined(MBEDTLS_DHM_C) && defined(MBEDTLS_SSL_SRV_C) 07161 if( endpoint == MBEDTLS_SSL_IS_SERVER ) 07162 { 07163 if( ( ret = mbedtls_ssl_conf_dh_param( conf, 07164 MBEDTLS_DHM_RFC5114_MODP_2048_P, 07165 MBEDTLS_DHM_RFC5114_MODP_2048_G ) ) != 0 ) 07166 { 07167 return( ret ); 07168 } 07169 } 07170 #endif 07171 07172 /* 07173 * Preset-specific defaults 07174 */ 07175 switch( preset ) 07176 { 07177 /* 07178 * NSA Suite B 07179 */ 07180 case MBEDTLS_SSL_PRESET_SUITEB: 07181 conf->min_major_ver = MBEDTLS_SSL_MAJOR_VERSION_3; 07182 conf->min_minor_ver = MBEDTLS_SSL_MINOR_VERSION_3; /* TLS 1.2 */ 07183 conf->max_major_ver = MBEDTLS_SSL_MAX_MAJOR_VERSION; 07184 conf->max_minor_ver = MBEDTLS_SSL_MAX_MINOR_VERSION; 07185 07186 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_0] = 07187 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_1] = 07188 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_2] = 07189 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_3] = 07190 ssl_preset_suiteb_ciphersuites; 07191 07192 #if defined(MBEDTLS_X509_CRT_PARSE_C) 07193 conf->cert_profile = &mbedtls_x509_crt_profile_suiteb; 07194 #endif 07195 07196 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 07197 conf->sig_hashes = ssl_preset_suiteb_hashes; 07198 #endif 07199 07200 #if defined(MBEDTLS_ECP_C) 07201 conf->curve_list = ssl_preset_suiteb_curves; 07202 #endif 07203 break; 07204 07205 /* 07206 * Default 07207 */ 07208 default: 07209 conf->min_major_ver = MBEDTLS_SSL_MAJOR_VERSION_3; 07210 conf->min_minor_ver = MBEDTLS_SSL_MINOR_VERSION_1; /* TLS 1.0 */ 07211 conf->max_major_ver = MBEDTLS_SSL_MAX_MAJOR_VERSION; 07212 conf->max_minor_ver = MBEDTLS_SSL_MAX_MINOR_VERSION; 07213 07214 #if defined(MBEDTLS_SSL_PROTO_DTLS) 07215 if( transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 07216 conf->min_minor_ver = MBEDTLS_SSL_MINOR_VERSION_2; 07217 #endif 07218 07219 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_0] = 07220 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_1] = 07221 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_2] = 07222 conf->ciphersuite_list [MBEDTLS_SSL_MINOR_VERSION_3] = 07223 mbedtls_ssl_list_ciphersuites(); 07224 07225 #if defined(MBEDTLS_X509_CRT_PARSE_C) 07226 conf->cert_profile = &mbedtls_x509_crt_profile_default; 07227 #endif 07228 07229 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 07230 conf->sig_hashes = mbedtls_md_list(); 07231 #endif 07232 07233 #if defined(MBEDTLS_ECP_C) 07234 conf->curve_list = mbedtls_ecp_grp_id_list(); 07235 #endif 07236 07237 #if defined(MBEDTLS_DHM_C) && defined(MBEDTLS_SSL_CLI_C) 07238 conf->dhm_min_bitlen = 1024; 07239 #endif 07240 } 07241 07242 return( 0 ); 07243 } 07244 07245 /* 07246 * Free mbedtls_ssl_config 07247 */ 07248 void mbedtls_ssl_config_free( mbedtls_ssl_config *conf ) 07249 { 07250 #if defined(MBEDTLS_DHM_C) 07251 mbedtls_mpi_free( &conf->dhm_P ); 07252 mbedtls_mpi_free( &conf->dhm_G ); 07253 #endif 07254 07255 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 07256 if( conf->psk != NULL ) 07257 { 07258 mbedtls_zeroize( conf->psk , conf->psk_len ); 07259 mbedtls_zeroize( conf->psk_identity , conf->psk_identity_len ); 07260 mbedtls_free( conf->psk ); 07261 mbedtls_free( conf->psk_identity ); 07262 conf->psk_len = 0; 07263 conf->psk_identity_len = 0; 07264 } 07265 #endif 07266 07267 #if defined(MBEDTLS_X509_CRT_PARSE_C) 07268 ssl_key_cert_free( conf->key_cert ); 07269 #endif 07270 07271 mbedtls_zeroize( conf, sizeof( mbedtls_ssl_config ) ); 07272 } 07273 07274 #if defined(MBEDTLS_PK_C) && \ 07275 ( defined(MBEDTLS_RSA_C) || defined(MBEDTLS_ECDSA_C) ) 07276 /* 07277 * Convert between MBEDTLS_PK_XXX and SSL_SIG_XXX 07278 */ 07279 unsigned char mbedtls_ssl_sig_from_pk( mbedtls_pk_context *pk ) 07280 { 07281 #if defined(MBEDTLS_RSA_C) 07282 if( mbedtls_pk_can_do( pk, MBEDTLS_PK_RSA ) ) 07283 return( MBEDTLS_SSL_SIG_RSA ); 07284 #endif 07285 #if defined(MBEDTLS_ECDSA_C) 07286 if( mbedtls_pk_can_do( pk, MBEDTLS_PK_ECDSA ) ) 07287 return( MBEDTLS_SSL_SIG_ECDSA ); 07288 #endif 07289 return( MBEDTLS_SSL_SIG_ANON ); 07290 } 07291 07292 mbedtls_pk_type_t mbedtls_ssl_pk_alg_from_sig( unsigned char sig ) 07293 { 07294 switch( sig ) 07295 { 07296 #if defined(MBEDTLS_RSA_C) 07297 case MBEDTLS_SSL_SIG_RSA: 07298 return( MBEDTLS_PK_RSA ); 07299 #endif 07300 #if defined(MBEDTLS_ECDSA_C) 07301 case MBEDTLS_SSL_SIG_ECDSA: 07302 return( MBEDTLS_PK_ECDSA ); 07303 #endif 07304 default: 07305 return( MBEDTLS_PK_NONE ); 07306 } 07307 } 07308 #endif /* MBEDTLS_PK_C && ( MBEDTLS_RSA_C || MBEDTLS_ECDSA_C ) */ 07309 07310 /* 07311 * Convert from MBEDTLS_SSL_HASH_XXX to MBEDTLS_MD_XXX 07312 */ 07313 mbedtls_md_type_t mbedtls_ssl_md_alg_from_hash( unsigned char hash ) 07314 { 07315 switch( hash ) 07316 { 07317 #if defined(MBEDTLS_MD5_C) 07318 case MBEDTLS_SSL_HASH_MD5: 07319 return( MBEDTLS_MD_MD5 ); 07320 #endif 07321 #if defined(MBEDTLS_SHA1_C) 07322 case MBEDTLS_SSL_HASH_SHA1: 07323 return( MBEDTLS_MD_SHA1 ); 07324 #endif 07325 #if defined(MBEDTLS_SHA256_C) 07326 case MBEDTLS_SSL_HASH_SHA224: 07327 return( MBEDTLS_MD_SHA224 ); 07328 case MBEDTLS_SSL_HASH_SHA256: 07329 return( MBEDTLS_MD_SHA256 ); 07330 #endif 07331 #if defined(MBEDTLS_SHA512_C) 07332 case MBEDTLS_SSL_HASH_SHA384: 07333 return( MBEDTLS_MD_SHA384 ); 07334 case MBEDTLS_SSL_HASH_SHA512: 07335 return( MBEDTLS_MD_SHA512 ); 07336 #endif 07337 default: 07338 return( MBEDTLS_MD_NONE ); 07339 } 07340 } 07341 07342 /* 07343 * Convert from MBEDTLS_MD_XXX to MBEDTLS_SSL_HASH_XXX 07344 */ 07345 unsigned char mbedtls_ssl_hash_from_md_alg( int md ) 07346 { 07347 switch( md ) 07348 { 07349 #if defined(MBEDTLS_MD5_C) 07350 case MBEDTLS_MD_MD5: 07351 return( MBEDTLS_SSL_HASH_MD5 ); 07352 #endif 07353 #if defined(MBEDTLS_SHA1_C) 07354 case MBEDTLS_MD_SHA1: 07355 return( MBEDTLS_SSL_HASH_SHA1 ); 07356 #endif 07357 #if defined(MBEDTLS_SHA256_C) 07358 case MBEDTLS_MD_SHA224: 07359 return( MBEDTLS_SSL_HASH_SHA224 ); 07360 case MBEDTLS_MD_SHA256: 07361 return( MBEDTLS_SSL_HASH_SHA256 ); 07362 #endif 07363 #if defined(MBEDTLS_SHA512_C) 07364 case MBEDTLS_MD_SHA384: 07365 return( MBEDTLS_SSL_HASH_SHA384 ); 07366 case MBEDTLS_MD_SHA512: 07367 return( MBEDTLS_SSL_HASH_SHA512 ); 07368 #endif 07369 default: 07370 return( MBEDTLS_SSL_HASH_NONE ); 07371 } 07372 } 07373 07374 #if defined(MBEDTLS_ECP_C) 07375 /* 07376 * Check if a curve proposed by the peer is in our list. 07377 * Return 0 if we're willing to use it, -1 otherwise. 07378 */ 07379 int mbedtls_ssl_check_curve( const mbedtls_ssl_context *ssl, mbedtls_ecp_group_id grp_id ) 07380 { 07381 const mbedtls_ecp_group_id *gid; 07382 07383 if( ssl->conf->curve_list == NULL ) 07384 return( -1 ); 07385 07386 for( gid = ssl->conf->curve_list; *gid != MBEDTLS_ECP_DP_NONE; gid++ ) 07387 if( *gid == grp_id ) 07388 return( 0 ); 07389 07390 return( -1 ); 07391 } 07392 #endif /* MBEDTLS_ECP_C */ 07393 07394 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 07395 /* 07396 * Check if a hash proposed by the peer is in our list. 07397 * Return 0 if we're willing to use it, -1 otherwise. 07398 */ 07399 int mbedtls_ssl_check_sig_hash( const mbedtls_ssl_context *ssl, 07400 mbedtls_md_type_t md ) 07401 { 07402 const int *cur; 07403 07404 if( ssl->conf->sig_hashes == NULL ) 07405 return( -1 ); 07406 07407 for( cur = ssl->conf->sig_hashes; *cur != MBEDTLS_MD_NONE; cur++ ) 07408 if( *cur == (int) md ) 07409 return( 0 ); 07410 07411 return( -1 ); 07412 } 07413 #endif /* MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 07414 07415 #if defined(MBEDTLS_X509_CRT_PARSE_C) 07416 int mbedtls_ssl_check_cert_usage( const mbedtls_x509_crt *cert, 07417 const mbedtls_ssl_ciphersuite_t *ciphersuite, 07418 int cert_endpoint, 07419 uint32_t *flags ) 07420 { 07421 int ret = 0; 07422 #if defined(MBEDTLS_X509_CHECK_KEY_USAGE) 07423 int usage = 0; 07424 #endif 07425 #if defined(MBEDTLS_X509_CHECK_EXTENDED_KEY_USAGE) 07426 const char *ext_oid; 07427 size_t ext_len; 07428 #endif 07429 07430 #if !defined(MBEDTLS_X509_CHECK_KEY_USAGE) && \ 07431 !defined(MBEDTLS_X509_CHECK_EXTENDED_KEY_USAGE) 07432 ((void) cert); 07433 ((void) cert_endpoint); 07434 ((void) flags); 07435 #endif 07436 07437 #if defined(MBEDTLS_X509_CHECK_KEY_USAGE) 07438 if( cert_endpoint == MBEDTLS_SSL_IS_SERVER ) 07439 { 07440 /* Server part of the key exchange */ 07441 switch( ciphersuite->key_exchange ) 07442 { 07443 case MBEDTLS_KEY_EXCHANGE_RSA: 07444 case MBEDTLS_KEY_EXCHANGE_RSA_PSK: 07445 usage = MBEDTLS_X509_KU_KEY_ENCIPHERMENT; 07446 break; 07447 07448 case MBEDTLS_KEY_EXCHANGE_DHE_RSA: 07449 case MBEDTLS_KEY_EXCHANGE_ECDHE_RSA: 07450 case MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA: 07451 usage = MBEDTLS_X509_KU_DIGITAL_SIGNATURE; 07452 break; 07453 07454 case MBEDTLS_KEY_EXCHANGE_ECDH_RSA: 07455 case MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA: 07456 usage = MBEDTLS_X509_KU_KEY_AGREEMENT; 07457 break; 07458 07459 /* Don't use default: we want warnings when adding new values */ 07460 case MBEDTLS_KEY_EXCHANGE_NONE: 07461 case MBEDTLS_KEY_EXCHANGE_PSK: 07462 case MBEDTLS_KEY_EXCHANGE_DHE_PSK: 07463 case MBEDTLS_KEY_EXCHANGE_ECDHE_PSK: 07464 case MBEDTLS_KEY_EXCHANGE_ECJPAKE: 07465 usage = 0; 07466 } 07467 } 07468 else 07469 { 07470 /* Client auth: we only implement rsa_sign and mbedtls_ecdsa_sign for now */ 07471 usage = MBEDTLS_X509_KU_DIGITAL_SIGNATURE; 07472 } 07473 07474 if( mbedtls_x509_crt_check_key_usage( cert, usage ) != 0 ) 07475 { 07476 *flags |= MBEDTLS_X509_BADCERT_KEY_USAGE; 07477 ret = -1; 07478 } 07479 #else 07480 ((void) ciphersuite); 07481 #endif /* MBEDTLS_X509_CHECK_KEY_USAGE */ 07482 07483 #if defined(MBEDTLS_X509_CHECK_EXTENDED_KEY_USAGE) 07484 if( cert_endpoint == MBEDTLS_SSL_IS_SERVER ) 07485 { 07486 ext_oid = MBEDTLS_OID_SERVER_AUTH; 07487 ext_len = MBEDTLS_OID_SIZE( MBEDTLS_OID_SERVER_AUTH ); 07488 } 07489 else 07490 { 07491 ext_oid = MBEDTLS_OID_CLIENT_AUTH; 07492 ext_len = MBEDTLS_OID_SIZE( MBEDTLS_OID_CLIENT_AUTH ); 07493 } 07494 07495 if( mbedtls_x509_crt_check_extended_key_usage( cert, ext_oid, ext_len ) != 0 ) 07496 { 07497 *flags |= MBEDTLS_X509_BADCERT_EXT_KEY_USAGE; 07498 ret = -1; 07499 } 07500 #endif /* MBEDTLS_X509_CHECK_EXTENDED_KEY_USAGE */ 07501 07502 return( ret ); 07503 } 07504 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 07505 07506 /* 07507 * Convert version numbers to/from wire format 07508 * and, for DTLS, to/from TLS equivalent. 07509 * 07510 * For TLS this is the identity. 07511 * For DTLS, use one complement (v -> 255 - v, and then map as follows: 07512 * 1.0 <-> 3.2 (DTLS 1.0 is based on TLS 1.1) 07513 * 1.x <-> 3.x+1 for x != 0 (DTLS 1.2 based on TLS 1.2) 07514 */ 07515 void mbedtls_ssl_write_version( int major, int minor, int transport, 07516 unsigned char ver[2] ) 07517 { 07518 #if defined(MBEDTLS_SSL_PROTO_DTLS) 07519 if( transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 07520 { 07521 if( minor == MBEDTLS_SSL_MINOR_VERSION_2 ) 07522 --minor; /* DTLS 1.0 stored as TLS 1.1 internally */ 07523 07524 ver[0] = (unsigned char)( 255 - ( major - 2 ) ); 07525 ver[1] = (unsigned char)( 255 - ( minor - 1 ) ); 07526 } 07527 else 07528 #else 07529 ((void) transport); 07530 #endif 07531 { 07532 ver[0] = (unsigned char) major; 07533 ver[1] = (unsigned char) minor; 07534 } 07535 } 07536 07537 void mbedtls_ssl_read_version( int *major, int *minor, int transport, 07538 const unsigned char ver[2] ) 07539 { 07540 #if defined(MBEDTLS_SSL_PROTO_DTLS) 07541 if( transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 07542 { 07543 *major = 255 - ver[0] + 2; 07544 *minor = 255 - ver[1] + 1; 07545 07546 if( *minor == MBEDTLS_SSL_MINOR_VERSION_1 ) 07547 ++*minor; /* DTLS 1.0 stored as TLS 1.1 internally */ 07548 } 07549 else 07550 #else 07551 ((void) transport); 07552 #endif 07553 { 07554 *major = ver[0]; 07555 *minor = ver[1]; 07556 } 07557 } 07558 07559 #endif /* MBEDTLS_SSL_TLS_C */
Generated on Tue Jul 12 2022 12:52:49 by
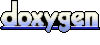