mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
ssl_srv.c
00001 /* 00002 * SSLv3/TLSv1 server-side functions 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 00022 #if !defined(MBEDTLS_CONFIG_FILE) 00023 #include "mbedtls/config.h" 00024 #else 00025 #include MBEDTLS_CONFIG_FILE 00026 #endif 00027 00028 #if defined(MBEDTLS_SSL_SRV_C) 00029 00030 #include "mbedtls/debug.h" 00031 #include "mbedtls/ssl.h" 00032 #include "mbedtls/ssl_internal.h" 00033 00034 #include <string.h> 00035 00036 #if defined(MBEDTLS_ECP_C) 00037 #include "mbedtls/ecp.h" 00038 #endif 00039 00040 #if defined(MBEDTLS_PLATFORM_C) 00041 #include "mbedtls/platform.h" 00042 #else 00043 #include <stdlib.h> 00044 #define mbedtls_calloc calloc 00045 #define mbedtls_free free 00046 #endif 00047 00048 #if defined(MBEDTLS_HAVE_TIME) 00049 #include <time.h> 00050 #endif 00051 00052 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 00053 /* Implementation that should never be optimized out by the compiler */ 00054 static void mbedtls_zeroize( void *v, size_t n ) { 00055 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00056 } 00057 #endif 00058 00059 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 00060 int mbedtls_ssl_set_client_transport_id( mbedtls_ssl_context *ssl, 00061 const unsigned char *info, 00062 size_t ilen ) 00063 { 00064 if( ssl->conf->endpoint != MBEDTLS_SSL_IS_SERVER ) 00065 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 00066 00067 mbedtls_free( ssl->cli_id ); 00068 00069 if( ( ssl->cli_id = mbedtls_calloc( 1, ilen ) ) == NULL ) 00070 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 00071 00072 memcpy( ssl->cli_id, info, ilen ); 00073 ssl->cli_id_len = ilen; 00074 00075 return( 0 ); 00076 } 00077 00078 void mbedtls_ssl_conf_dtls_cookies( mbedtls_ssl_config *conf, 00079 mbedtls_ssl_cookie_write_t *f_cookie_write, 00080 mbedtls_ssl_cookie_check_t *f_cookie_check, 00081 void *p_cookie ) 00082 { 00083 conf->f_cookie_write = f_cookie_write; 00084 conf->f_cookie_check = f_cookie_check; 00085 conf->p_cookie = p_cookie; 00086 } 00087 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 00088 00089 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 00090 static int ssl_parse_servername_ext( mbedtls_ssl_context *ssl, 00091 const unsigned char *buf, 00092 size_t len ) 00093 { 00094 int ret; 00095 size_t servername_list_size, hostname_len; 00096 const unsigned char *p; 00097 00098 MBEDTLS_SSL_DEBUG_MSG( 3, ( "parse ServerName extension" ) ); 00099 00100 servername_list_size = ( ( buf[0] << 8 ) | ( buf[1] ) ); 00101 if( servername_list_size + 2 != len ) 00102 { 00103 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00104 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00105 } 00106 00107 p = buf + 2; 00108 while( servername_list_size > 0 ) 00109 { 00110 hostname_len = ( ( p[1] << 8 ) | p[2] ); 00111 if( hostname_len + 3 > servername_list_size ) 00112 { 00113 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00114 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00115 } 00116 00117 if( p[0] == MBEDTLS_TLS_EXT_SERVERNAME_HOSTNAME ) 00118 { 00119 ret = ssl->conf->f_sni( ssl->conf->p_sni, 00120 ssl, p + 3, hostname_len ); 00121 if( ret != 0 ) 00122 { 00123 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_sni_wrapper", ret ); 00124 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00125 MBEDTLS_SSL_ALERT_MSG_UNRECOGNIZED_NAME ); 00126 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00127 } 00128 return( 0 ); 00129 } 00130 00131 servername_list_size -= hostname_len + 3; 00132 p += hostname_len + 3; 00133 } 00134 00135 if( servername_list_size != 0 ) 00136 { 00137 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00138 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00139 } 00140 00141 return( 0 ); 00142 } 00143 #endif /* MBEDTLS_SSL_SERVER_NAME_INDICATION */ 00144 00145 static int ssl_parse_renegotiation_info( mbedtls_ssl_context *ssl, 00146 const unsigned char *buf, 00147 size_t len ) 00148 { 00149 int ret; 00150 00151 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00152 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00153 { 00154 /* Check verify-data in constant-time. The length OTOH is no secret */ 00155 if( len != 1 + ssl->verify_data_len || 00156 buf[0] != ssl->verify_data_len || 00157 mbedtls_ssl_safer_memcmp( buf + 1, ssl->peer_verify_data, 00158 ssl->verify_data_len ) != 0 ) 00159 { 00160 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching renegotiation info" ) ); 00161 00162 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 00163 return( ret ); 00164 00165 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00166 } 00167 } 00168 else 00169 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00170 { 00171 if( len != 1 || buf[0] != 0x0 ) 00172 { 00173 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-zero length renegotiation info" ) ); 00174 00175 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 00176 return( ret ); 00177 00178 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00179 } 00180 00181 ssl->secure_renegotiation = MBEDTLS_SSL_SECURE_RENEGOTIATION; 00182 } 00183 00184 return( 0 ); 00185 } 00186 00187 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 00188 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 00189 static int ssl_parse_signature_algorithms_ext( mbedtls_ssl_context *ssl, 00190 const unsigned char *buf, 00191 size_t len ) 00192 { 00193 size_t sig_alg_list_size; 00194 const unsigned char *p; 00195 const unsigned char *end = buf + len; 00196 const int *md_cur; 00197 00198 00199 sig_alg_list_size = ( ( buf[0] << 8 ) | ( buf[1] ) ); 00200 if( sig_alg_list_size + 2 != len || 00201 sig_alg_list_size % 2 != 0 ) 00202 { 00203 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00204 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00205 } 00206 00207 /* 00208 * For now, ignore the SignatureAlgorithm part and rely on offered 00209 * ciphersuites only for that part. To be fixed later. 00210 * 00211 * So, just look at the HashAlgorithm part. 00212 */ 00213 for( md_cur = ssl->conf->sig_hashes; *md_cur != MBEDTLS_MD_NONE; md_cur++ ) { 00214 for( p = buf + 2; p < end; p += 2 ) { 00215 if( *md_cur == (int) mbedtls_ssl_md_alg_from_hash( p[0] ) ) { 00216 ssl->handshake->sig_alg = p[0]; 00217 goto have_sig_alg; 00218 } 00219 } 00220 } 00221 00222 /* Some key echanges do not need signatures at all */ 00223 MBEDTLS_SSL_DEBUG_MSG( 3, ( "no signature_algorithm in common" ) ); 00224 return( 0 ); 00225 00226 have_sig_alg: 00227 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, signature_algorithm ext: %d", 00228 ssl->handshake->sig_alg ) ); 00229 00230 return( 0 ); 00231 } 00232 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 00233 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 00234 00235 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 00236 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00237 static int ssl_parse_supported_elliptic_curves( mbedtls_ssl_context *ssl, 00238 const unsigned char *buf, 00239 size_t len ) 00240 { 00241 size_t list_size, our_size; 00242 const unsigned char *p; 00243 const mbedtls_ecp_curve_info *curve_info, **curves; 00244 00245 list_size = ( ( buf[0] << 8 ) | ( buf[1] ) ); 00246 if( list_size + 2 != len || 00247 list_size % 2 != 0 ) 00248 { 00249 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00250 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00251 } 00252 00253 /* Should never happen unless client duplicates the extension */ 00254 if( ssl->handshake->curves != NULL ) 00255 { 00256 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00257 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00258 } 00259 00260 /* Don't allow our peer to make us allocate too much memory, 00261 * and leave room for a final 0 */ 00262 our_size = list_size / 2 + 1; 00263 if( our_size > MBEDTLS_ECP_DP_MAX ) 00264 our_size = MBEDTLS_ECP_DP_MAX; 00265 00266 if( ( curves = mbedtls_calloc( our_size, sizeof( *curves ) ) ) == NULL ) 00267 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 00268 00269 ssl->handshake->curves = curves; 00270 00271 p = buf + 2; 00272 while( list_size > 0 && our_size > 1 ) 00273 { 00274 curve_info = mbedtls_ecp_curve_info_from_tls_id( ( p[0] << 8 ) | p[1] ); 00275 00276 if( curve_info != NULL ) 00277 { 00278 *curves++ = curve_info; 00279 our_size--; 00280 } 00281 00282 list_size -= 2; 00283 p += 2; 00284 } 00285 00286 return( 0 ); 00287 } 00288 00289 static int ssl_parse_supported_point_formats( mbedtls_ssl_context *ssl, 00290 const unsigned char *buf, 00291 size_t len ) 00292 { 00293 size_t list_size; 00294 const unsigned char *p; 00295 00296 list_size = buf[0]; 00297 if( list_size + 1 != len ) 00298 { 00299 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00300 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00301 } 00302 00303 p = buf + 1; 00304 while( list_size > 0 ) 00305 { 00306 if( p[0] == MBEDTLS_ECP_PF_UNCOMPRESSED || 00307 p[0] == MBEDTLS_ECP_PF_COMPRESSED ) 00308 { 00309 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) 00310 ssl->handshake->ecdh_ctx.point_format = p[0]; 00311 #endif 00312 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00313 ssl->handshake->ecjpake_ctx.point_format = p[0]; 00314 #endif 00315 MBEDTLS_SSL_DEBUG_MSG( 4, ( "point format selected: %d", p[0] ) ); 00316 return( 0 ); 00317 } 00318 00319 list_size--; 00320 p++; 00321 } 00322 00323 return( 0 ); 00324 } 00325 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || 00326 MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 00327 00328 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00329 static int ssl_parse_ecjpake_kkpp( mbedtls_ssl_context *ssl, 00330 const unsigned char *buf, 00331 size_t len ) 00332 { 00333 int ret; 00334 00335 if( mbedtls_ecjpake_check( &ssl->handshake->ecjpake_ctx ) != 0 ) 00336 { 00337 MBEDTLS_SSL_DEBUG_MSG( 3, ( "skip ecjpake kkpp extension" ) ); 00338 return( 0 ); 00339 } 00340 00341 if( ( ret = mbedtls_ecjpake_read_round_one( &ssl->handshake->ecjpake_ctx, 00342 buf, len ) ) != 0 ) 00343 { 00344 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_read_round_one", ret ); 00345 return( ret ); 00346 } 00347 00348 /* Only mark the extension as OK when we're sure it is */ 00349 ssl->handshake->cli_exts |= MBEDTLS_TLS_EXT_ECJPAKE_KKPP_OK; 00350 00351 return( 0 ); 00352 } 00353 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 00354 00355 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 00356 static int ssl_parse_max_fragment_length_ext( mbedtls_ssl_context *ssl, 00357 const unsigned char *buf, 00358 size_t len ) 00359 { 00360 if( len != 1 || buf[0] >= MBEDTLS_SSL_MAX_FRAG_LEN_INVALID ) 00361 { 00362 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00363 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00364 } 00365 00366 ssl->session_negotiate->mfl_code = buf[0]; 00367 00368 return( 0 ); 00369 } 00370 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 00371 00372 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 00373 static int ssl_parse_truncated_hmac_ext( mbedtls_ssl_context *ssl, 00374 const unsigned char *buf, 00375 size_t len ) 00376 { 00377 if( len != 0 ) 00378 { 00379 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00380 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00381 } 00382 00383 ((void) buf); 00384 00385 if( ssl->conf->trunc_hmac == MBEDTLS_SSL_TRUNC_HMAC_ENABLED ) 00386 ssl->session_negotiate->trunc_hmac = MBEDTLS_SSL_TRUNC_HMAC_ENABLED; 00387 00388 return( 0 ); 00389 } 00390 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 00391 00392 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 00393 static int ssl_parse_encrypt_then_mac_ext( mbedtls_ssl_context *ssl, 00394 const unsigned char *buf, 00395 size_t len ) 00396 { 00397 if( len != 0 ) 00398 { 00399 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00400 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00401 } 00402 00403 ((void) buf); 00404 00405 if( ssl->conf->encrypt_then_mac == MBEDTLS_SSL_ETM_ENABLED && 00406 ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_0 ) 00407 { 00408 ssl->session_negotiate->encrypt_then_mac = MBEDTLS_SSL_ETM_ENABLED; 00409 } 00410 00411 return( 0 ); 00412 } 00413 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 00414 00415 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 00416 static int ssl_parse_extended_ms_ext( mbedtls_ssl_context *ssl, 00417 const unsigned char *buf, 00418 size_t len ) 00419 { 00420 if( len != 0 ) 00421 { 00422 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00423 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00424 } 00425 00426 ((void) buf); 00427 00428 if( ssl->conf->extended_ms == MBEDTLS_SSL_EXTENDED_MS_ENABLED && 00429 ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_0 ) 00430 { 00431 ssl->handshake->extended_ms = MBEDTLS_SSL_EXTENDED_MS_ENABLED; 00432 } 00433 00434 return( 0 ); 00435 } 00436 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 00437 00438 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 00439 static int ssl_parse_session_ticket_ext( mbedtls_ssl_context *ssl, 00440 unsigned char *buf, 00441 size_t len ) 00442 { 00443 int ret; 00444 mbedtls_ssl_session session; 00445 00446 mbedtls_ssl_session_init( &session ); 00447 00448 if( ssl->conf->f_ticket_parse == NULL || 00449 ssl->conf->f_ticket_write == NULL ) 00450 { 00451 return( 0 ); 00452 } 00453 00454 /* Remember the client asked us to send a new ticket */ 00455 ssl->handshake->new_session_ticket = 1; 00456 00457 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket length: %d", len ) ); 00458 00459 if( len == 0 ) 00460 return( 0 ); 00461 00462 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00463 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00464 { 00465 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket rejected: renegotiating" ) ); 00466 return( 0 ); 00467 } 00468 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00469 00470 /* 00471 * Failures are ok: just ignore the ticket and proceed. 00472 */ 00473 if( ( ret = ssl->conf->f_ticket_parse( ssl->conf->p_ticket, &session, 00474 buf, len ) ) != 0 ) 00475 { 00476 mbedtls_ssl_session_free( &session ); 00477 00478 if( ret == MBEDTLS_ERR_SSL_INVALID_MAC ) 00479 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket is not authentic" ) ); 00480 else if( ret == MBEDTLS_ERR_SSL_SESSION_TICKET_EXPIRED ) 00481 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket is expired" ) ); 00482 else 00483 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_ticket_parse", ret ); 00484 00485 return( 0 ); 00486 } 00487 00488 /* 00489 * Keep the session ID sent by the client, since we MUST send it back to 00490 * inform them we're accepting the ticket (RFC 5077 section 3.4) 00491 */ 00492 session.id_len = ssl->session_negotiate->id_len; 00493 memcpy( &session.id, ssl->session_negotiate->id, session.id_len ); 00494 00495 mbedtls_ssl_session_free( ssl->session_negotiate ); 00496 memcpy( ssl->session_negotiate, &session, sizeof( mbedtls_ssl_session ) ); 00497 00498 /* Zeroize instead of free as we copied the content */ 00499 mbedtls_zeroize( &session, sizeof( mbedtls_ssl_session ) ); 00500 00501 MBEDTLS_SSL_DEBUG_MSG( 3, ( "session successfully restored from ticket" ) ); 00502 00503 ssl->handshake->resume = 1; 00504 00505 /* Don't send a new ticket after all, this one is OK */ 00506 ssl->handshake->new_session_ticket = 0; 00507 00508 return( 0 ); 00509 } 00510 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 00511 00512 #if defined(MBEDTLS_SSL_ALPN) 00513 static int ssl_parse_alpn_ext( mbedtls_ssl_context *ssl, 00514 const unsigned char *buf, size_t len ) 00515 { 00516 size_t list_len, cur_len, ours_len; 00517 const unsigned char *theirs, *start, *end; 00518 const char **ours; 00519 00520 /* If ALPN not configured, just ignore the extension */ 00521 if( ssl->conf->alpn_list == NULL ) 00522 return( 0 ); 00523 00524 /* 00525 * opaque ProtocolName<1..2^8-1>; 00526 * 00527 * struct { 00528 * ProtocolName protocol_name_list<2..2^16-1> 00529 * } ProtocolNameList; 00530 */ 00531 00532 /* Min length is 2 (list_len) + 1 (name_len) + 1 (name) */ 00533 if( len < 4 ) 00534 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00535 00536 list_len = ( buf[0] << 8 ) | buf[1]; 00537 if( list_len != len - 2 ) 00538 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00539 00540 /* 00541 * Use our order of preference 00542 */ 00543 start = buf + 2; 00544 end = buf + len; 00545 for( ours = ssl->conf->alpn_list; *ours != NULL; ours++ ) 00546 { 00547 ours_len = strlen( *ours ); 00548 for( theirs = start; theirs != end; theirs += cur_len ) 00549 { 00550 /* If the list is well formed, we should get equality first */ 00551 if( theirs > end ) 00552 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00553 00554 cur_len = *theirs++; 00555 00556 /* Empty strings MUST NOT be included */ 00557 if( cur_len == 0 ) 00558 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00559 00560 if( cur_len == ours_len && 00561 memcmp( theirs, *ours, cur_len ) == 0 ) 00562 { 00563 ssl->alpn_chosen = *ours; 00564 return( 0 ); 00565 } 00566 } 00567 } 00568 00569 /* If we get there, no match was found */ 00570 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00571 MBEDTLS_SSL_ALERT_MSG_NO_APPLICATION_PROTOCOL ); 00572 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00573 } 00574 #endif /* MBEDTLS_SSL_ALPN */ 00575 00576 /* 00577 * Auxiliary functions for ServerHello parsing and related actions 00578 */ 00579 00580 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00581 /* 00582 * Return 0 if the given key uses one of the acceptable curves, -1 otherwise 00583 */ 00584 #if defined(MBEDTLS_ECDSA_C) 00585 static int ssl_check_key_curve( mbedtls_pk_context *pk, 00586 const mbedtls_ecp_curve_info **curves ) 00587 { 00588 const mbedtls_ecp_curve_info **crv = curves; 00589 mbedtls_ecp_group_id grp_id = mbedtls_pk_ec( *pk )->grp .id ; 00590 00591 while( *crv != NULL ) 00592 { 00593 if( (*crv)->grp_id == grp_id ) 00594 return( 0 ); 00595 crv++; 00596 } 00597 00598 return( -1 ); 00599 } 00600 #endif /* MBEDTLS_ECDSA_C */ 00601 00602 /* 00603 * Try picking a certificate for this ciphersuite, 00604 * return 0 on success and -1 on failure. 00605 */ 00606 static int ssl_pick_cert( mbedtls_ssl_context *ssl, 00607 const mbedtls_ssl_ciphersuite_t * ciphersuite_info ) 00608 { 00609 mbedtls_ssl_key_cert *cur, *list, *fallback = NULL; 00610 mbedtls_pk_type_t pk_alg = mbedtls_ssl_get_ciphersuite_sig_pk_alg( ciphersuite_info ); 00611 uint32_t flags; 00612 00613 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 00614 if( ssl->handshake->sni_key_cert != NULL ) 00615 list = ssl->handshake->sni_key_cert; 00616 else 00617 #endif 00618 list = ssl->conf->key_cert; 00619 00620 if( pk_alg == MBEDTLS_PK_NONE ) 00621 return( 0 ); 00622 00623 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite requires certificate" ) ); 00624 00625 if( list == NULL ) 00626 { 00627 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server has no certificate" ) ); 00628 return( -1 ); 00629 } 00630 00631 for( cur = list; cur != NULL; cur = cur->next ) 00632 { 00633 MBEDTLS_SSL_DEBUG_CRT( 3, "candidate certificate chain, certificate", 00634 cur->cert ); 00635 00636 if( ! mbedtls_pk_can_do( cur->key, pk_alg ) ) 00637 { 00638 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate mismatch: key type" ) ); 00639 continue; 00640 } 00641 00642 /* 00643 * This avoids sending the client a cert it'll reject based on 00644 * keyUsage or other extensions. 00645 * 00646 * It also allows the user to provision different certificates for 00647 * different uses based on keyUsage, eg if they want to avoid signing 00648 * and decrypting with the same RSA key. 00649 */ 00650 if( mbedtls_ssl_check_cert_usage( cur->cert, ciphersuite_info, 00651 MBEDTLS_SSL_IS_SERVER, &flags ) != 0 ) 00652 { 00653 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate mismatch: " 00654 "(extended) key usage extension" ) ); 00655 continue; 00656 } 00657 00658 #if defined(MBEDTLS_ECDSA_C) 00659 if( pk_alg == MBEDTLS_PK_ECDSA && 00660 ssl_check_key_curve( cur->key, ssl->handshake->curves ) != 0 ) 00661 { 00662 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate mismatch: elliptic curve" ) ); 00663 continue; 00664 } 00665 #endif 00666 00667 /* 00668 * Try to select a SHA-1 certificate for pre-1.2 clients, but still 00669 * present them a SHA-higher cert rather than failing if it's the only 00670 * one we got that satisfies the other conditions. 00671 */ 00672 if( ssl->minor_ver < MBEDTLS_SSL_MINOR_VERSION_3 && 00673 cur->cert->sig_md != MBEDTLS_MD_SHA1 ) 00674 { 00675 if( fallback == NULL ) 00676 fallback = cur; 00677 { 00678 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate not preferred: " 00679 "sha-2 with pre-TLS 1.2 client" ) ); 00680 continue; 00681 } 00682 } 00683 00684 /* If we get there, we got a winner */ 00685 break; 00686 } 00687 00688 if( cur == NULL ) 00689 cur = fallback; 00690 00691 /* Do not update ssl->handshake->key_cert unless there is a match */ 00692 if( cur != NULL ) 00693 { 00694 ssl->handshake->key_cert = cur; 00695 MBEDTLS_SSL_DEBUG_CRT( 3, "selected certificate chain, certificate", 00696 ssl->handshake->key_cert->cert ); 00697 return( 0 ); 00698 } 00699 00700 return( -1 ); 00701 } 00702 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 00703 00704 /* 00705 * Check if a given ciphersuite is suitable for use with our config/keys/etc 00706 * Sets ciphersuite_info only if the suite matches. 00707 */ 00708 static int ssl_ciphersuite_match( mbedtls_ssl_context *ssl, int suite_id, 00709 const mbedtls_ssl_ciphersuite_t **ciphersuite_info ) 00710 { 00711 const mbedtls_ssl_ciphersuite_t *suite_info; 00712 00713 suite_info = mbedtls_ssl_ciphersuite_from_id( suite_id ); 00714 if( suite_info == NULL ) 00715 { 00716 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 00717 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00718 } 00719 00720 MBEDTLS_SSL_DEBUG_MSG( 3, ( "trying ciphersuite: %s", suite_info->name ) ); 00721 00722 if( suite_info->min_minor_ver > ssl->minor_ver || 00723 suite_info->max_minor_ver < ssl->minor_ver ) 00724 { 00725 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: version" ) ); 00726 return( 0 ); 00727 } 00728 00729 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00730 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 00731 ( suite_info->flags & MBEDTLS_CIPHERSUITE_NODTLS ) ) 00732 return( 0 ); 00733 #endif 00734 00735 #if defined(MBEDTLS_ARC4_C) 00736 if( ssl->conf->arc4_disabled == MBEDTLS_SSL_ARC4_DISABLED && 00737 suite_info->cipher == MBEDTLS_CIPHER_ARC4_128 ) 00738 { 00739 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: rc4" ) ); 00740 return( 0 ); 00741 } 00742 #endif 00743 00744 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00745 if( suite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE && 00746 ( ssl->handshake->cli_exts & MBEDTLS_TLS_EXT_ECJPAKE_KKPP_OK ) == 0 ) 00747 { 00748 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: ecjpake " 00749 "not configured or ext missing" ) ); 00750 return( 0 ); 00751 } 00752 #endif 00753 00754 00755 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) 00756 if( mbedtls_ssl_ciphersuite_uses_ec( suite_info ) && 00757 ( ssl->handshake->curves == NULL || 00758 ssl->handshake->curves[0] == NULL ) ) 00759 { 00760 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: " 00761 "no common elliptic curve" ) ); 00762 return( 0 ); 00763 } 00764 #endif 00765 00766 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 00767 /* If the ciphersuite requires a pre-shared key and we don't 00768 * have one, skip it now rather than failing later */ 00769 if( mbedtls_ssl_ciphersuite_uses_psk( suite_info ) && 00770 ssl->conf->f_psk == NULL && 00771 ( ssl->conf->psk == NULL || ssl->conf->psk_identity == NULL || 00772 ssl->conf->psk_identity_len == 0 || ssl->conf->psk_len == 0 ) ) 00773 { 00774 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: no pre-shared key" ) ); 00775 return( 0 ); 00776 } 00777 #endif 00778 00779 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00780 /* 00781 * Final check: if ciphersuite requires us to have a 00782 * certificate/key of a particular type: 00783 * - select the appropriate certificate if we have one, or 00784 * - try the next ciphersuite if we don't 00785 * This must be done last since we modify the key_cert list. 00786 */ 00787 if( ssl_pick_cert( ssl, suite_info ) != 0 ) 00788 { 00789 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: " 00790 "no suitable certificate" ) ); 00791 return( 0 ); 00792 } 00793 #endif 00794 00795 *ciphersuite_info = suite_info; 00796 return( 0 ); 00797 } 00798 00799 #if defined(MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO) 00800 static int ssl_parse_client_hello_v2( mbedtls_ssl_context *ssl ) 00801 { 00802 int ret, got_common_suite; 00803 unsigned int i, j; 00804 size_t n; 00805 unsigned int ciph_len, sess_len, chal_len; 00806 unsigned char *buf, *p; 00807 const int *ciphersuites; 00808 const mbedtls_ssl_ciphersuite_t *ciphersuite_info; 00809 00810 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse client hello v2" ) ); 00811 00812 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00813 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00814 { 00815 MBEDTLS_SSL_DEBUG_MSG( 1, ( "client hello v2 illegal for renegotiation" ) ); 00816 00817 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 00818 return( ret ); 00819 00820 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00821 } 00822 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00823 00824 buf = ssl->in_hdr; 00825 00826 MBEDTLS_SSL_DEBUG_BUF( 4, "record header", buf, 5 ); 00827 00828 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v2, message type: %d", 00829 buf[2] ) ); 00830 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v2, message len.: %d", 00831 ( ( buf[0] & 0x7F ) << 8 ) | buf[1] ) ); 00832 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v2, max. version: [%d:%d]", 00833 buf[3], buf[4] ) ); 00834 00835 /* 00836 * SSLv2 Client Hello 00837 * 00838 * Record layer: 00839 * 0 . 1 message length 00840 * 00841 * SSL layer: 00842 * 2 . 2 message type 00843 * 3 . 4 protocol version 00844 */ 00845 if( buf[2] != MBEDTLS_SSL_HS_CLIENT_HELLO || 00846 buf[3] != MBEDTLS_SSL_MAJOR_VERSION_3 ) 00847 { 00848 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00849 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00850 } 00851 00852 n = ( ( buf[0] << 8 ) | buf[1] ) & 0x7FFF; 00853 00854 if( n < 17 || n > 512 ) 00855 { 00856 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00857 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00858 } 00859 00860 ssl->major_ver = MBEDTLS_SSL_MAJOR_VERSION_3; 00861 ssl->minor_ver = ( buf[4] <= ssl->conf->max_minor_ver ) 00862 ? buf[4] : ssl->conf->max_minor_ver; 00863 00864 if( ssl->minor_ver < ssl->conf->min_minor_ver ) 00865 { 00866 MBEDTLS_SSL_DEBUG_MSG( 1, ( "client only supports ssl smaller than minimum" 00867 " [%d:%d] < [%d:%d]", 00868 ssl->major_ver, ssl->minor_ver, 00869 ssl->conf->min_major_ver, ssl->conf->min_minor_ver ) ); 00870 00871 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00872 MBEDTLS_SSL_ALERT_MSG_PROTOCOL_VERSION ); 00873 return( MBEDTLS_ERR_SSL_BAD_HS_PROTOCOL_VERSION ); 00874 } 00875 00876 ssl->handshake->max_major_ver = buf[3]; 00877 ssl->handshake->max_minor_ver = buf[4]; 00878 00879 if( ( ret = mbedtls_ssl_fetch_input( ssl, 2 + n ) ) != 0 ) 00880 { 00881 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 00882 return( ret ); 00883 } 00884 00885 ssl->handshake->update_checksum( ssl, buf + 2, n ); 00886 00887 buf = ssl->in_msg; 00888 n = ssl->in_left - 5; 00889 00890 /* 00891 * 0 . 1 ciphersuitelist length 00892 * 2 . 3 session id length 00893 * 4 . 5 challenge length 00894 * 6 . .. ciphersuitelist 00895 * .. . .. session id 00896 * .. . .. challenge 00897 */ 00898 MBEDTLS_SSL_DEBUG_BUF( 4, "record contents", buf, n ); 00899 00900 ciph_len = ( buf[0] << 8 ) | buf[1]; 00901 sess_len = ( buf[2] << 8 ) | buf[3]; 00902 chal_len = ( buf[4] << 8 ) | buf[5]; 00903 00904 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciph_len: %d, sess_len: %d, chal_len: %d", 00905 ciph_len, sess_len, chal_len ) ); 00906 00907 /* 00908 * Make sure each parameter length is valid 00909 */ 00910 if( ciph_len < 3 || ( ciph_len % 3 ) != 0 ) 00911 { 00912 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00913 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00914 } 00915 00916 if( sess_len > 32 ) 00917 { 00918 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00919 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00920 } 00921 00922 if( chal_len < 8 || chal_len > 32 ) 00923 { 00924 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00925 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00926 } 00927 00928 if( n != 6 + ciph_len + sess_len + chal_len ) 00929 { 00930 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00931 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00932 } 00933 00934 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, ciphersuitelist", 00935 buf + 6, ciph_len ); 00936 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, session id", 00937 buf + 6 + ciph_len, sess_len ); 00938 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, challenge", 00939 buf + 6 + ciph_len + sess_len, chal_len ); 00940 00941 p = buf + 6 + ciph_len; 00942 ssl->session_negotiate->id_len = sess_len; 00943 memset( ssl->session_negotiate->id, 0, 00944 sizeof( ssl->session_negotiate->id ) ); 00945 memcpy( ssl->session_negotiate->id, p, ssl->session_negotiate->id_len ); 00946 00947 p += sess_len; 00948 memset( ssl->handshake->randbytes, 0, 64 ); 00949 memcpy( ssl->handshake->randbytes + 32 - chal_len, p, chal_len ); 00950 00951 /* 00952 * Check for TLS_EMPTY_RENEGOTIATION_INFO_SCSV 00953 */ 00954 for( i = 0, p = buf + 6; i < ciph_len; i += 3, p += 3 ) 00955 { 00956 if( p[0] == 0 && p[1] == 0 && p[2] == MBEDTLS_SSL_EMPTY_RENEGOTIATION_INFO ) 00957 { 00958 MBEDTLS_SSL_DEBUG_MSG( 3, ( "received TLS_EMPTY_RENEGOTIATION_INFO " ) ); 00959 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00960 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 00961 { 00962 MBEDTLS_SSL_DEBUG_MSG( 1, ( "received RENEGOTIATION SCSV " 00963 "during renegotiation" ) ); 00964 00965 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 00966 return( ret ); 00967 00968 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00969 } 00970 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00971 ssl->secure_renegotiation = MBEDTLS_SSL_SECURE_RENEGOTIATION; 00972 break; 00973 } 00974 } 00975 00976 #if defined(MBEDTLS_SSL_FALLBACK_SCSV) 00977 for( i = 0, p = buf + 6; i < ciph_len; i += 3, p += 3 ) 00978 { 00979 if( p[0] == 0 && 00980 p[1] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE >> 8 ) & 0xff ) && 00981 p[2] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE ) & 0xff ) ) 00982 { 00983 MBEDTLS_SSL_DEBUG_MSG( 3, ( "received FALLBACK_SCSV" ) ); 00984 00985 if( ssl->minor_ver < ssl->conf->max_minor_ver ) 00986 { 00987 MBEDTLS_SSL_DEBUG_MSG( 1, ( "inapropriate fallback" ) ); 00988 00989 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00990 MBEDTLS_SSL_ALERT_MSG_INAPROPRIATE_FALLBACK ); 00991 00992 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00993 } 00994 00995 break; 00996 } 00997 } 00998 #endif /* MBEDTLS_SSL_FALLBACK_SCSV */ 00999 01000 got_common_suite = 0; 01001 ciphersuites = ssl->conf->ciphersuite_list[ssl->minor_ver]; 01002 ciphersuite_info = NULL; 01003 #if defined(MBEDTLS_SSL_SRV_RESPECT_CLIENT_PREFERENCE) 01004 for( j = 0, p = buf + 6; j < ciph_len; j += 3, p += 3 ) 01005 { 01006 for( i = 0; ciphersuites[i] != 0; i++ ) 01007 #else 01008 for( i = 0; ciphersuites[i] != 0; i++ ) 01009 { 01010 for( j = 0, p = buf + 6; j < ciph_len; j += 3, p += 3 ) 01011 #endif 01012 { 01013 if( p[0] != 0 || 01014 p[1] != ( ( ciphersuites[i] >> 8 ) & 0xFF ) || 01015 p[2] != ( ( ciphersuites[i] ) & 0xFF ) ) 01016 continue; 01017 01018 got_common_suite = 1; 01019 01020 if( ( ret = ssl_ciphersuite_match( ssl, ciphersuites[i], 01021 &ciphersuite_info ) ) != 0 ) 01022 return( ret ); 01023 01024 if( ciphersuite_info != NULL ) 01025 goto have_ciphersuite_v2; 01026 } 01027 } 01028 01029 if( got_common_suite ) 01030 { 01031 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got ciphersuites in common, " 01032 "but none of them usable" ) ); 01033 return( MBEDTLS_ERR_SSL_NO_USABLE_CIPHERSUITE ); 01034 } 01035 else 01036 { 01037 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no ciphersuites in common" ) ); 01038 return( MBEDTLS_ERR_SSL_NO_CIPHER_CHOSEN ); 01039 } 01040 01041 have_ciphersuite_v2: 01042 MBEDTLS_SSL_DEBUG_MSG( 2, ( "selected ciphersuite: %s", ciphersuite_info->name ) ); 01043 01044 ssl->session_negotiate->ciphersuite = ciphersuites[i]; 01045 ssl->transform_negotiate->ciphersuite_info = ciphersuite_info; 01046 mbedtls_ssl_optimize_checksum( ssl, ssl->transform_negotiate->ciphersuite_info ); 01047 01048 /* 01049 * SSLv2 Client Hello relevant renegotiation security checks 01050 */ 01051 if( ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01052 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_BREAK_HANDSHAKE ) 01053 { 01054 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation, breaking off handshake" ) ); 01055 01056 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 01057 return( ret ); 01058 01059 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01060 } 01061 01062 ssl->in_left = 0; 01063 ssl->state++; 01064 01065 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse client hello v2" ) ); 01066 01067 return( 0 ); 01068 } 01069 #endif /* MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO */ 01070 01071 static int ssl_parse_client_hello( mbedtls_ssl_context *ssl ) 01072 { 01073 int ret, got_common_suite; 01074 size_t i, j; 01075 size_t ciph_offset, comp_offset, ext_offset; 01076 size_t msg_len, ciph_len, sess_len, comp_len, ext_len; 01077 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01078 size_t cookie_offset, cookie_len; 01079 #endif 01080 unsigned char *buf, *p, *ext; 01081 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01082 int renegotiation_info_seen = 0; 01083 #endif 01084 int handshake_failure = 0; 01085 const int *ciphersuites; 01086 const mbedtls_ssl_ciphersuite_t *ciphersuite_info; 01087 int major, minor; 01088 01089 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse client hello" ) ); 01090 01091 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 01092 read_record_header: 01093 #endif 01094 /* 01095 * If renegotiating, then the input was read with mbedtls_ssl_read_record(), 01096 * otherwise read it ourselves manually in order to support SSLv2 01097 * ClientHello, which doesn't use the same record layer format. 01098 */ 01099 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01100 if( ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE ) 01101 #endif 01102 { 01103 if( ( ret = mbedtls_ssl_fetch_input( ssl, 5 ) ) != 0 ) 01104 { 01105 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 01106 return( ret ); 01107 } 01108 } 01109 01110 buf = ssl->in_hdr; 01111 01112 #if defined(MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO) 01113 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01114 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_STREAM ) 01115 #endif 01116 if( ( buf[0] & 0x80 ) != 0 ) 01117 return ssl_parse_client_hello_v2( ssl ); 01118 #endif 01119 01120 MBEDTLS_SSL_DEBUG_BUF( 4, "record header", buf, mbedtls_ssl_hdr_len( ssl ) ); 01121 01122 /* 01123 * SSLv3/TLS Client Hello 01124 * 01125 * Record layer: 01126 * 0 . 0 message type 01127 * 1 . 2 protocol version 01128 * 3 . 11 DTLS: epoch + record sequence number 01129 * 3 . 4 message length 01130 */ 01131 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, message type: %d", 01132 buf[0] ) ); 01133 01134 if( buf[0] != MBEDTLS_SSL_MSG_HANDSHAKE ) 01135 { 01136 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01137 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01138 } 01139 01140 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, message len.: %d", 01141 ( ssl->in_len[0] << 8 ) | ssl->in_len[1] ) ); 01142 01143 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, protocol version: [%d:%d]", 01144 buf[1], buf[2] ) ); 01145 01146 mbedtls_ssl_read_version( &major, &minor, ssl->conf->transport, buf + 1 ); 01147 01148 /* According to RFC 5246 Appendix E.1, the version here is typically 01149 * "{03,00}, the lowest version number supported by the client, [or] the 01150 * value of ClientHello.client_version", so the only meaningful check here 01151 * is the major version shouldn't be less than 3 */ 01152 if( major < MBEDTLS_SSL_MAJOR_VERSION_3 ) 01153 { 01154 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01155 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01156 } 01157 01158 /* For DTLS if this is the initial handshake, remember the client sequence 01159 * number to use it in our next message (RFC 6347 4.2.1) */ 01160 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01161 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM 01162 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01163 && ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE 01164 #endif 01165 ) 01166 { 01167 /* Epoch should be 0 for initial handshakes */ 01168 if( ssl->in_ctr[0] != 0 || ssl->in_ctr[1] != 0 ) 01169 { 01170 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01171 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01172 } 01173 01174 memcpy( ssl->out_ctr + 2, ssl->in_ctr + 2, 6 ); 01175 01176 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 01177 if( mbedtls_ssl_dtls_replay_check( ssl ) != 0 ) 01178 { 01179 MBEDTLS_SSL_DEBUG_MSG( 1, ( "replayed record, discarding" ) ); 01180 ssl->next_record_offset = 0; 01181 ssl->in_left = 0; 01182 goto read_record_header; 01183 } 01184 01185 /* No MAC to check yet, so we can update right now */ 01186 mbedtls_ssl_dtls_replay_update( ssl ); 01187 #endif 01188 } 01189 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01190 01191 msg_len = ( ssl->in_len[0] << 8 ) | ssl->in_len[1]; 01192 01193 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01194 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 01195 { 01196 /* Set by mbedtls_ssl_read_record() */ 01197 msg_len = ssl->in_hslen; 01198 } 01199 else 01200 #endif 01201 { 01202 if( msg_len > MBEDTLS_SSL_MAX_CONTENT_LEN ) 01203 { 01204 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01205 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01206 } 01207 01208 if( ( ret = mbedtls_ssl_fetch_input( ssl, mbedtls_ssl_hdr_len( ssl ) + msg_len ) ) != 0 ) 01209 { 01210 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 01211 return( ret ); 01212 } 01213 01214 /* Done reading this record, get ready for the next one */ 01215 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01216 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01217 ssl->next_record_offset = msg_len + mbedtls_ssl_hdr_len( ssl ); 01218 else 01219 #endif 01220 ssl->in_left = 0; 01221 } 01222 01223 buf = ssl->in_msg; 01224 01225 MBEDTLS_SSL_DEBUG_BUF( 4, "record contents", buf, msg_len ); 01226 01227 ssl->handshake->update_checksum( ssl, buf, msg_len ); 01228 01229 /* 01230 * Handshake layer: 01231 * 0 . 0 handshake type 01232 * 1 . 3 handshake length 01233 * 4 . 5 DTLS only: message seqence number 01234 * 6 . 8 DTLS only: fragment offset 01235 * 9 . 11 DTLS only: fragment length 01236 */ 01237 if( msg_len < mbedtls_ssl_hs_hdr_len( ssl ) ) 01238 { 01239 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01240 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01241 } 01242 01243 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, handshake type: %d", buf[0] ) ); 01244 01245 if( buf[0] != MBEDTLS_SSL_HS_CLIENT_HELLO ) 01246 { 01247 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01248 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01249 } 01250 01251 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, handshake len.: %d", 01252 ( buf[1] << 16 ) | ( buf[2] << 8 ) | buf[3] ) ); 01253 01254 /* We don't support fragmentation of ClientHello (yet?) */ 01255 if( buf[1] != 0 || 01256 msg_len != mbedtls_ssl_hs_hdr_len( ssl ) + ( ( buf[2] << 8 ) | buf[3] ) ) 01257 { 01258 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01259 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01260 } 01261 01262 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01263 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01264 { 01265 /* 01266 * Copy the client's handshake message_seq on initial handshakes, 01267 * check sequence number on renego. 01268 */ 01269 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01270 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01271 { 01272 /* This couldn't be done in ssl_prepare_handshake_record() */ 01273 unsigned int cli_msg_seq = ( ssl->in_msg[4] << 8 ) | 01274 ssl->in_msg[5]; 01275 01276 if( cli_msg_seq != ssl->handshake->in_msg_seq ) 01277 { 01278 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message_seq: " 01279 "%d (expected %d)", cli_msg_seq, 01280 ssl->handshake->in_msg_seq ) ); 01281 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01282 } 01283 01284 ssl->handshake->in_msg_seq++; 01285 } 01286 else 01287 #endif 01288 { 01289 unsigned int cli_msg_seq = ( ssl->in_msg[4] << 8 ) | 01290 ssl->in_msg[5]; 01291 ssl->handshake->out_msg_seq = cli_msg_seq; 01292 ssl->handshake->in_msg_seq = cli_msg_seq + 1; 01293 } 01294 01295 /* 01296 * For now we don't support fragmentation, so make sure 01297 * fragment_offset == 0 and fragment_length == length 01298 */ 01299 if( ssl->in_msg[6] != 0 || ssl->in_msg[7] != 0 || ssl->in_msg[8] != 0 || 01300 memcmp( ssl->in_msg + 1, ssl->in_msg + 9, 3 ) != 0 ) 01301 { 01302 MBEDTLS_SSL_DEBUG_MSG( 1, ( "ClientHello fragmentation not supported" ) ); 01303 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 01304 } 01305 } 01306 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01307 01308 buf += mbedtls_ssl_hs_hdr_len( ssl ); 01309 msg_len -= mbedtls_ssl_hs_hdr_len( ssl ); 01310 01311 /* 01312 * ClientHello layer: 01313 * 0 . 1 protocol version 01314 * 2 . 33 random bytes (starting with 4 bytes of Unix time) 01315 * 34 . 35 session id length (1 byte) 01316 * 35 . 34+x session id 01317 * 35+x . 35+x DTLS only: cookie length (1 byte) 01318 * 36+x . .. DTLS only: cookie 01319 * .. . .. ciphersuite list length (2 bytes) 01320 * .. . .. ciphersuite list 01321 * .. . .. compression alg. list length (1 byte) 01322 * .. . .. compression alg. list 01323 * .. . .. extensions length (2 bytes, optional) 01324 * .. . .. extensions (optional) 01325 */ 01326 01327 /* 01328 * Minimal length (with everything empty and extensions ommitted) is 01329 * 2 + 32 + 1 + 2 + 1 = 38 bytes. Check that first, so that we can 01330 * read at least up to session id length without worrying. 01331 */ 01332 if( msg_len < 38 ) 01333 { 01334 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01335 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01336 } 01337 01338 /* 01339 * Check and save the protocol version 01340 */ 01341 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, version", buf, 2 ); 01342 01343 mbedtls_ssl_read_version( &ssl->major_ver, &ssl->minor_ver, 01344 ssl->conf->transport, buf ); 01345 01346 ssl->handshake->max_major_ver = ssl->major_ver; 01347 ssl->handshake->max_minor_ver = ssl->minor_ver; 01348 01349 if( ssl->major_ver < ssl->conf->min_major_ver || 01350 ssl->minor_ver < ssl->conf->min_minor_ver ) 01351 { 01352 MBEDTLS_SSL_DEBUG_MSG( 1, ( "client only supports ssl smaller than minimum" 01353 " [%d:%d] < [%d:%d]", 01354 ssl->major_ver, ssl->minor_ver, 01355 ssl->conf->min_major_ver, ssl->conf->min_minor_ver ) ); 01356 01357 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01358 MBEDTLS_SSL_ALERT_MSG_PROTOCOL_VERSION ); 01359 01360 return( MBEDTLS_ERR_SSL_BAD_HS_PROTOCOL_VERSION ); 01361 } 01362 01363 if( ssl->major_ver > ssl->conf->max_major_ver ) 01364 { 01365 ssl->major_ver = ssl->conf->max_major_ver; 01366 ssl->minor_ver = ssl->conf->max_minor_ver; 01367 } 01368 else if( ssl->minor_ver > ssl->conf->max_minor_ver ) 01369 ssl->minor_ver = ssl->conf->max_minor_ver; 01370 01371 /* 01372 * Save client random (inc. Unix time) 01373 */ 01374 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, random bytes", buf + 2, 32 ); 01375 01376 memcpy( ssl->handshake->randbytes, buf + 2, 32 ); 01377 01378 /* 01379 * Check the session ID length and save session ID 01380 */ 01381 sess_len = buf[34]; 01382 01383 if( sess_len > sizeof( ssl->session_negotiate->id ) || 01384 sess_len + 34 + 2 > msg_len ) /* 2 for cipherlist length field */ 01385 { 01386 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01387 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01388 } 01389 01390 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, session id", buf + 35, sess_len ); 01391 01392 ssl->session_negotiate->id_len = sess_len; 01393 memset( ssl->session_negotiate->id, 0, 01394 sizeof( ssl->session_negotiate->id ) ); 01395 memcpy( ssl->session_negotiate->id, buf + 35, 01396 ssl->session_negotiate->id_len ); 01397 01398 /* 01399 * Check the cookie length and content 01400 */ 01401 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01402 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01403 { 01404 cookie_offset = 35 + sess_len; 01405 cookie_len = buf[cookie_offset]; 01406 01407 if( cookie_offset + 1 + cookie_len + 2 > msg_len ) 01408 { 01409 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01410 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01411 } 01412 01413 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, cookie", 01414 buf + cookie_offset + 1, cookie_len ); 01415 01416 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 01417 if( ssl->conf->f_cookie_check != NULL 01418 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01419 && ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE 01420 #endif 01421 ) 01422 { 01423 if( ssl->conf->f_cookie_check( ssl->conf->p_cookie, 01424 buf + cookie_offset + 1, cookie_len, 01425 ssl->cli_id, ssl->cli_id_len ) != 0 ) 01426 { 01427 MBEDTLS_SSL_DEBUG_MSG( 2, ( "cookie verification failed" ) ); 01428 ssl->handshake->verify_cookie_len = 1; 01429 } 01430 else 01431 { 01432 MBEDTLS_SSL_DEBUG_MSG( 2, ( "cookie verification passed" ) ); 01433 ssl->handshake->verify_cookie_len = 0; 01434 } 01435 } 01436 else 01437 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 01438 { 01439 /* We know we didn't send a cookie, so it should be empty */ 01440 if( cookie_len != 0 ) 01441 { 01442 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01443 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01444 } 01445 01446 MBEDTLS_SSL_DEBUG_MSG( 2, ( "cookie verification skipped" ) ); 01447 } 01448 01449 /* 01450 * Check the ciphersuitelist length (will be parsed later) 01451 */ 01452 ciph_offset = cookie_offset + 1 + cookie_len; 01453 } 01454 else 01455 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01456 ciph_offset = 35 + sess_len; 01457 01458 ciph_len = ( buf[ciph_offset + 0] << 8 ) 01459 | ( buf[ciph_offset + 1] ); 01460 01461 if( ciph_len < 2 || 01462 ciph_len + 2 + ciph_offset + 1 > msg_len || /* 1 for comp. alg. len */ 01463 ( ciph_len % 2 ) != 0 ) 01464 { 01465 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01466 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01467 } 01468 01469 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, ciphersuitelist", 01470 buf + ciph_offset + 2, ciph_len ); 01471 01472 /* 01473 * Check the compression algorithms length and pick one 01474 */ 01475 comp_offset = ciph_offset + 2 + ciph_len; 01476 01477 comp_len = buf[comp_offset]; 01478 01479 if( comp_len < 1 || 01480 comp_len > 16 || 01481 comp_len + comp_offset + 1 > msg_len ) 01482 { 01483 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01484 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01485 } 01486 01487 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, compression", 01488 buf + comp_offset + 1, comp_len ); 01489 01490 ssl->session_negotiate->compression = MBEDTLS_SSL_COMPRESS_NULL; 01491 #if defined(MBEDTLS_ZLIB_SUPPORT) 01492 for( i = 0; i < comp_len; ++i ) 01493 { 01494 if( buf[comp_offset + 1 + i] == MBEDTLS_SSL_COMPRESS_DEFLATE ) 01495 { 01496 ssl->session_negotiate->compression = MBEDTLS_SSL_COMPRESS_DEFLATE; 01497 break; 01498 } 01499 } 01500 #endif 01501 01502 /* See comments in ssl_write_client_hello() */ 01503 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01504 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01505 ssl->session_negotiate->compression = MBEDTLS_SSL_COMPRESS_NULL; 01506 #endif 01507 01508 /* 01509 * Check the extension length 01510 */ 01511 ext_offset = comp_offset + 1 + comp_len; 01512 if( msg_len > ext_offset ) 01513 { 01514 if( msg_len < ext_offset + 2 ) 01515 { 01516 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01517 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01518 } 01519 01520 ext_len = ( buf[ext_offset + 0] << 8 ) 01521 | ( buf[ext_offset + 1] ); 01522 01523 if( ( ext_len > 0 && ext_len < 4 ) || 01524 msg_len != ext_offset + 2 + ext_len ) 01525 { 01526 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01527 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01528 } 01529 } 01530 else 01531 ext_len = 0; 01532 01533 ext = buf + ext_offset + 2; 01534 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello extensions", ext, ext_len ); 01535 01536 while( ext_len != 0 ) 01537 { 01538 unsigned int ext_id = ( ( ext[0] << 8 ) 01539 | ( ext[1] ) ); 01540 unsigned int ext_size = ( ( ext[2] << 8 ) 01541 | ( ext[3] ) ); 01542 01543 if( ext_size + 4 > ext_len ) 01544 { 01545 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01546 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01547 } 01548 switch( ext_id ) 01549 { 01550 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 01551 case MBEDTLS_TLS_EXT_SERVERNAME: 01552 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found ServerName extension" ) ); 01553 if( ssl->conf->f_sni == NULL ) 01554 break; 01555 01556 ret = ssl_parse_servername_ext( ssl, ext + 4, ext_size ); 01557 if( ret != 0 ) 01558 return( ret ); 01559 break; 01560 #endif /* MBEDTLS_SSL_SERVER_NAME_INDICATION */ 01561 01562 case MBEDTLS_TLS_EXT_RENEGOTIATION_INFO: 01563 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found renegotiation extension" ) ); 01564 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01565 renegotiation_info_seen = 1; 01566 #endif 01567 01568 ret = ssl_parse_renegotiation_info( ssl, ext + 4, ext_size ); 01569 if( ret != 0 ) 01570 return( ret ); 01571 break; 01572 01573 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 01574 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 01575 case MBEDTLS_TLS_EXT_SIG_ALG: 01576 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found signature_algorithms extension" ) ); 01577 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01578 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01579 break; 01580 #endif 01581 01582 ret = ssl_parse_signature_algorithms_ext( ssl, ext + 4, ext_size ); 01583 if( ret != 0 ) 01584 return( ret ); 01585 break; 01586 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 01587 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 01588 01589 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 01590 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01591 case MBEDTLS_TLS_EXT_SUPPORTED_ELLIPTIC_CURVES: 01592 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found supported elliptic curves extension" ) ); 01593 01594 ret = ssl_parse_supported_elliptic_curves( ssl, ext + 4, ext_size ); 01595 if( ret != 0 ) 01596 return( ret ); 01597 break; 01598 01599 case MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS: 01600 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found supported point formats extension" ) ); 01601 ssl->handshake->cli_exts |= MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS_PRESENT; 01602 01603 ret = ssl_parse_supported_point_formats( ssl, ext + 4, ext_size ); 01604 if( ret != 0 ) 01605 return( ret ); 01606 break; 01607 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || 01608 MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01609 01610 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01611 case MBEDTLS_TLS_EXT_ECJPAKE_KKPP: 01612 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found ecjpake kkpp extension" ) ); 01613 01614 ret = ssl_parse_ecjpake_kkpp( ssl, ext + 4, ext_size ); 01615 if( ret != 0 ) 01616 return( ret ); 01617 break; 01618 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01619 01620 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 01621 case MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH: 01622 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found max fragment length extension" ) ); 01623 01624 ret = ssl_parse_max_fragment_length_ext( ssl, ext + 4, ext_size ); 01625 if( ret != 0 ) 01626 return( ret ); 01627 break; 01628 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 01629 01630 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 01631 case MBEDTLS_TLS_EXT_TRUNCATED_HMAC: 01632 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found truncated hmac extension" ) ); 01633 01634 ret = ssl_parse_truncated_hmac_ext( ssl, ext + 4, ext_size ); 01635 if( ret != 0 ) 01636 return( ret ); 01637 break; 01638 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 01639 01640 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01641 case MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC: 01642 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found encrypt then mac extension" ) ); 01643 01644 ret = ssl_parse_encrypt_then_mac_ext( ssl, ext + 4, ext_size ); 01645 if( ret != 0 ) 01646 return( ret ); 01647 break; 01648 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 01649 01650 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 01651 case MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET: 01652 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found extended master secret extension" ) ); 01653 01654 ret = ssl_parse_extended_ms_ext( ssl, ext + 4, ext_size ); 01655 if( ret != 0 ) 01656 return( ret ); 01657 break; 01658 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 01659 01660 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 01661 case MBEDTLS_TLS_EXT_SESSION_TICKET: 01662 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found session ticket extension" ) ); 01663 01664 ret = ssl_parse_session_ticket_ext( ssl, ext + 4, ext_size ); 01665 if( ret != 0 ) 01666 return( ret ); 01667 break; 01668 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 01669 01670 #if defined(MBEDTLS_SSL_ALPN) 01671 case MBEDTLS_TLS_EXT_ALPN: 01672 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found alpn extension" ) ); 01673 01674 ret = ssl_parse_alpn_ext( ssl, ext + 4, ext_size ); 01675 if( ret != 0 ) 01676 return( ret ); 01677 break; 01678 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 01679 01680 default: 01681 MBEDTLS_SSL_DEBUG_MSG( 3, ( "unknown extension found: %d (ignoring)", 01682 ext_id ) ); 01683 } 01684 01685 ext_len -= 4 + ext_size; 01686 ext += 4 + ext_size; 01687 01688 if( ext_len > 0 && ext_len < 4 ) 01689 { 01690 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01691 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01692 } 01693 } 01694 01695 #if defined(MBEDTLS_SSL_FALLBACK_SCSV) 01696 for( i = 0, p = buf + 41 + sess_len; i < ciph_len; i += 2, p += 2 ) 01697 { 01698 if( p[0] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE >> 8 ) & 0xff ) && 01699 p[1] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE ) & 0xff ) ) 01700 { 01701 MBEDTLS_SSL_DEBUG_MSG( 2, ( "received FALLBACK_SCSV" ) ); 01702 01703 if( ssl->minor_ver < ssl->conf->max_minor_ver ) 01704 { 01705 MBEDTLS_SSL_DEBUG_MSG( 1, ( "inapropriate fallback" ) ); 01706 01707 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01708 MBEDTLS_SSL_ALERT_MSG_INAPROPRIATE_FALLBACK ); 01709 01710 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01711 } 01712 01713 break; 01714 } 01715 } 01716 #endif /* MBEDTLS_SSL_FALLBACK_SCSV */ 01717 01718 /* 01719 * Check for TLS_EMPTY_RENEGOTIATION_INFO_SCSV 01720 */ 01721 for( i = 0, p = buf + ciph_offset + 2; i < ciph_len; i += 2, p += 2 ) 01722 { 01723 if( p[0] == 0 && p[1] == MBEDTLS_SSL_EMPTY_RENEGOTIATION_INFO ) 01724 { 01725 MBEDTLS_SSL_DEBUG_MSG( 3, ( "received TLS_EMPTY_RENEGOTIATION_INFO " ) ); 01726 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01727 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01728 { 01729 MBEDTLS_SSL_DEBUG_MSG( 1, ( "received RENEGOTIATION SCSV during renegotiation" ) ); 01730 01731 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 01732 return( ret ); 01733 01734 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01735 } 01736 #endif 01737 ssl->secure_renegotiation = MBEDTLS_SSL_SECURE_RENEGOTIATION; 01738 break; 01739 } 01740 } 01741 01742 /* 01743 * Renegotiation security checks 01744 */ 01745 if( ssl->secure_renegotiation != MBEDTLS_SSL_SECURE_RENEGOTIATION && 01746 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_BREAK_HANDSHAKE ) 01747 { 01748 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation, breaking off handshake" ) ); 01749 handshake_failure = 1; 01750 } 01751 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01752 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01753 ssl->secure_renegotiation == MBEDTLS_SSL_SECURE_RENEGOTIATION && 01754 renegotiation_info_seen == 0 ) 01755 { 01756 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation_info extension missing (secure)" ) ); 01757 handshake_failure = 1; 01758 } 01759 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01760 ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01761 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_NO_RENEGOTIATION ) 01762 { 01763 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation not allowed" ) ); 01764 handshake_failure = 1; 01765 } 01766 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01767 ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01768 renegotiation_info_seen == 1 ) 01769 { 01770 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation_info extension present (legacy)" ) ); 01771 handshake_failure = 1; 01772 } 01773 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 01774 01775 if( handshake_failure == 1 ) 01776 { 01777 if( ( ret = mbedtls_ssl_send_fatal_handshake_failure( ssl ) ) != 0 ) 01778 return( ret ); 01779 01780 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01781 } 01782 01783 /* 01784 * Search for a matching ciphersuite 01785 * (At the end because we need information from the EC-based extensions 01786 * and certificate from the SNI callback triggered by the SNI extension.) 01787 */ 01788 got_common_suite = 0; 01789 ciphersuites = ssl->conf->ciphersuite_list[ssl->minor_ver]; 01790 ciphersuite_info = NULL; 01791 #if defined(MBEDTLS_SSL_SRV_RESPECT_CLIENT_PREFERENCE) 01792 for( j = 0, p = buf + ciph_offset + 2; j < ciph_len; j += 2, p += 2 ) 01793 { 01794 for( i = 0; ciphersuites[i] != 0; i++ ) 01795 #else 01796 for( i = 0; ciphersuites[i] != 0; i++ ) 01797 { 01798 for( j = 0, p = buf + ciph_offset + 2; j < ciph_len; j += 2, p += 2 ) 01799 #endif 01800 { 01801 if( p[0] != ( ( ciphersuites[i] >> 8 ) & 0xFF ) || 01802 p[1] != ( ( ciphersuites[i] ) & 0xFF ) ) 01803 continue; 01804 01805 got_common_suite = 1; 01806 01807 if( ( ret = ssl_ciphersuite_match( ssl, ciphersuites[i], 01808 &ciphersuite_info ) ) != 0 ) 01809 return( ret ); 01810 01811 if( ciphersuite_info != NULL ) 01812 goto have_ciphersuite; 01813 } 01814 } 01815 01816 if( got_common_suite ) 01817 { 01818 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got ciphersuites in common, " 01819 "but none of them usable" ) ); 01820 mbedtls_ssl_send_fatal_handshake_failure( ssl ); 01821 return( MBEDTLS_ERR_SSL_NO_USABLE_CIPHERSUITE ); 01822 } 01823 else 01824 { 01825 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no ciphersuites in common" ) ); 01826 mbedtls_ssl_send_fatal_handshake_failure( ssl ); 01827 return( MBEDTLS_ERR_SSL_NO_CIPHER_CHOSEN ); 01828 } 01829 01830 have_ciphersuite: 01831 MBEDTLS_SSL_DEBUG_MSG( 2, ( "selected ciphersuite: %s", ciphersuite_info->name ) ); 01832 01833 ssl->session_negotiate->ciphersuite = ciphersuites[i]; 01834 ssl->transform_negotiate->ciphersuite_info = ciphersuite_info; 01835 mbedtls_ssl_optimize_checksum( ssl, ssl->transform_negotiate->ciphersuite_info ); 01836 01837 ssl->state++; 01838 01839 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01840 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01841 mbedtls_ssl_recv_flight_completed( ssl ); 01842 #endif 01843 01844 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse client hello" ) ); 01845 01846 return( 0 ); 01847 } 01848 01849 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 01850 static void ssl_write_truncated_hmac_ext( mbedtls_ssl_context *ssl, 01851 unsigned char *buf, 01852 size_t *olen ) 01853 { 01854 unsigned char *p = buf; 01855 01856 if( ssl->session_negotiate->trunc_hmac == MBEDTLS_SSL_TRUNC_HMAC_DISABLED ) 01857 { 01858 *olen = 0; 01859 return; 01860 } 01861 01862 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding truncated hmac extension" ) ); 01863 01864 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_TRUNCATED_HMAC >> 8 ) & 0xFF ); 01865 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_TRUNCATED_HMAC ) & 0xFF ); 01866 01867 *p++ = 0x00; 01868 *p++ = 0x00; 01869 01870 *olen = 4; 01871 } 01872 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 01873 01874 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01875 static void ssl_write_encrypt_then_mac_ext( mbedtls_ssl_context *ssl, 01876 unsigned char *buf, 01877 size_t *olen ) 01878 { 01879 unsigned char *p = buf; 01880 const mbedtls_ssl_ciphersuite_t *suite = NULL; 01881 const mbedtls_cipher_info_t *cipher = NULL; 01882 01883 if( ssl->session_negotiate->encrypt_then_mac == MBEDTLS_SSL_EXTENDED_MS_DISABLED || 01884 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 01885 { 01886 *olen = 0; 01887 return; 01888 } 01889 01890 /* 01891 * RFC 7366: "If a server receives an encrypt-then-MAC request extension 01892 * from a client and then selects a stream or Authenticated Encryption 01893 * with Associated Data (AEAD) ciphersuite, it MUST NOT send an 01894 * encrypt-then-MAC response extension back to the client." 01895 */ 01896 if( ( suite = mbedtls_ssl_ciphersuite_from_id( 01897 ssl->session_negotiate->ciphersuite ) ) == NULL || 01898 ( cipher = mbedtls_cipher_info_from_type( suite->cipher ) ) == NULL || 01899 cipher->mode != MBEDTLS_MODE_CBC ) 01900 { 01901 *olen = 0; 01902 return; 01903 } 01904 01905 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding encrypt then mac extension" ) ); 01906 01907 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC >> 8 ) & 0xFF ); 01908 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC ) & 0xFF ); 01909 01910 *p++ = 0x00; 01911 *p++ = 0x00; 01912 01913 *olen = 4; 01914 } 01915 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 01916 01917 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 01918 static void ssl_write_extended_ms_ext( mbedtls_ssl_context *ssl, 01919 unsigned char *buf, 01920 size_t *olen ) 01921 { 01922 unsigned char *p = buf; 01923 01924 if( ssl->handshake->extended_ms == MBEDTLS_SSL_EXTENDED_MS_DISABLED || 01925 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 01926 { 01927 *olen = 0; 01928 return; 01929 } 01930 01931 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding extended master secret " 01932 "extension" ) ); 01933 01934 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET >> 8 ) & 0xFF ); 01935 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET ) & 0xFF ); 01936 01937 *p++ = 0x00; 01938 *p++ = 0x00; 01939 01940 *olen = 4; 01941 } 01942 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 01943 01944 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 01945 static void ssl_write_session_ticket_ext( mbedtls_ssl_context *ssl, 01946 unsigned char *buf, 01947 size_t *olen ) 01948 { 01949 unsigned char *p = buf; 01950 01951 if( ssl->handshake->new_session_ticket == 0 ) 01952 { 01953 *olen = 0; 01954 return; 01955 } 01956 01957 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding session ticket extension" ) ); 01958 01959 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SESSION_TICKET >> 8 ) & 0xFF ); 01960 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SESSION_TICKET ) & 0xFF ); 01961 01962 *p++ = 0x00; 01963 *p++ = 0x00; 01964 01965 *olen = 4; 01966 } 01967 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 01968 01969 static void ssl_write_renegotiation_ext( mbedtls_ssl_context *ssl, 01970 unsigned char *buf, 01971 size_t *olen ) 01972 { 01973 unsigned char *p = buf; 01974 01975 if( ssl->secure_renegotiation != MBEDTLS_SSL_SECURE_RENEGOTIATION ) 01976 { 01977 *olen = 0; 01978 return; 01979 } 01980 01981 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, secure renegotiation extension" ) ); 01982 01983 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_RENEGOTIATION_INFO >> 8 ) & 0xFF ); 01984 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_RENEGOTIATION_INFO ) & 0xFF ); 01985 01986 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01987 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 01988 { 01989 *p++ = 0x00; 01990 *p++ = ( ssl->verify_data_len * 2 + 1 ) & 0xFF; 01991 *p++ = ssl->verify_data_len * 2 & 0xFF; 01992 01993 memcpy( p, ssl->peer_verify_data, ssl->verify_data_len ); 01994 p += ssl->verify_data_len; 01995 memcpy( p, ssl->own_verify_data, ssl->verify_data_len ); 01996 p += ssl->verify_data_len; 01997 } 01998 else 01999 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 02000 { 02001 *p++ = 0x00; 02002 *p++ = 0x01; 02003 *p++ = 0x00; 02004 } 02005 02006 *olen = p - buf; 02007 } 02008 02009 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 02010 static void ssl_write_max_fragment_length_ext( mbedtls_ssl_context *ssl, 02011 unsigned char *buf, 02012 size_t *olen ) 02013 { 02014 unsigned char *p = buf; 02015 02016 if( ssl->session_negotiate->mfl_code == MBEDTLS_SSL_MAX_FRAG_LEN_NONE ) 02017 { 02018 *olen = 0; 02019 return; 02020 } 02021 02022 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, max_fragment_length extension" ) ); 02023 02024 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH >> 8 ) & 0xFF ); 02025 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH ) & 0xFF ); 02026 02027 *p++ = 0x00; 02028 *p++ = 1; 02029 02030 *p++ = ssl->session_negotiate->mfl_code; 02031 02032 *olen = 5; 02033 } 02034 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 02035 02036 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 02037 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02038 static void ssl_write_supported_point_formats_ext( mbedtls_ssl_context *ssl, 02039 unsigned char *buf, 02040 size_t *olen ) 02041 { 02042 unsigned char *p = buf; 02043 ((void) ssl); 02044 02045 if( ( ssl->handshake->cli_exts & 02046 MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS_PRESENT ) == 0 ) 02047 { 02048 *olen = 0; 02049 return; 02050 } 02051 02052 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, supported_point_formats extension" ) ); 02053 02054 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS >> 8 ) & 0xFF ); 02055 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS ) & 0xFF ); 02056 02057 *p++ = 0x00; 02058 *p++ = 2; 02059 02060 *p++ = 1; 02061 *p++ = MBEDTLS_ECP_PF_UNCOMPRESSED; 02062 02063 *olen = 6; 02064 } 02065 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 02066 02067 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02068 static void ssl_write_ecjpake_kkpp_ext( mbedtls_ssl_context *ssl, 02069 unsigned char *buf, 02070 size_t *olen ) 02071 { 02072 int ret; 02073 unsigned char *p = buf; 02074 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 02075 size_t kkpp_len; 02076 02077 *olen = 0; 02078 02079 /* Skip costly computation if not needed */ 02080 if( ssl->transform_negotiate->ciphersuite_info->key_exchange != 02081 MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 02082 return; 02083 02084 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, ecjpake kkpp extension" ) ); 02085 02086 if( end - p < 4 ) 02087 { 02088 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 02089 return; 02090 } 02091 02092 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ECJPAKE_KKPP >> 8 ) & 0xFF ); 02093 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ECJPAKE_KKPP ) & 0xFF ); 02094 02095 ret = mbedtls_ecjpake_write_round_one( &ssl->handshake->ecjpake_ctx, 02096 p + 2, end - p - 2, &kkpp_len, 02097 ssl->conf->f_rng, ssl->conf->p_rng ); 02098 if( ret != 0 ) 02099 { 02100 MBEDTLS_SSL_DEBUG_RET( 1 , "mbedtls_ecjpake_write_round_one", ret ); 02101 return; 02102 } 02103 02104 *p++ = (unsigned char)( ( kkpp_len >> 8 ) & 0xFF ); 02105 *p++ = (unsigned char)( ( kkpp_len ) & 0xFF ); 02106 02107 *olen = kkpp_len + 4; 02108 } 02109 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 02110 02111 #if defined(MBEDTLS_SSL_ALPN ) 02112 static void ssl_write_alpn_ext( mbedtls_ssl_context *ssl, 02113 unsigned char *buf, size_t *olen ) 02114 { 02115 if( ssl->alpn_chosen == NULL ) 02116 { 02117 *olen = 0; 02118 return; 02119 } 02120 02121 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding alpn extension" ) ); 02122 02123 /* 02124 * 0 . 1 ext identifier 02125 * 2 . 3 ext length 02126 * 4 . 5 protocol list length 02127 * 6 . 6 protocol name length 02128 * 7 . 7+n protocol name 02129 */ 02130 buf[0] = (unsigned char)( ( MBEDTLS_TLS_EXT_ALPN >> 8 ) & 0xFF ); 02131 buf[1] = (unsigned char)( ( MBEDTLS_TLS_EXT_ALPN ) & 0xFF ); 02132 02133 *olen = 7 + strlen( ssl->alpn_chosen ); 02134 02135 buf[2] = (unsigned char)( ( ( *olen - 4 ) >> 8 ) & 0xFF ); 02136 buf[3] = (unsigned char)( ( ( *olen - 4 ) ) & 0xFF ); 02137 02138 buf[4] = (unsigned char)( ( ( *olen - 6 ) >> 8 ) & 0xFF ); 02139 buf[5] = (unsigned char)( ( ( *olen - 6 ) ) & 0xFF ); 02140 02141 buf[6] = (unsigned char)( ( ( *olen - 7 ) ) & 0xFF ); 02142 02143 memcpy( buf + 7, ssl->alpn_chosen, *olen - 7 ); 02144 } 02145 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C */ 02146 02147 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 02148 static int ssl_write_hello_verify_request( mbedtls_ssl_context *ssl ) 02149 { 02150 int ret; 02151 unsigned char *p = ssl->out_msg + 4; 02152 unsigned char *cookie_len_byte; 02153 02154 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write hello verify request" ) ); 02155 02156 /* 02157 * struct { 02158 * ProtocolVersion server_version; 02159 * opaque cookie<0..2^8-1>; 02160 * } HelloVerifyRequest; 02161 */ 02162 02163 /* The RFC is not clear on this point, but sending the actual negotiated 02164 * version looks like the most interoperable thing to do. */ 02165 mbedtls_ssl_write_version( ssl->major_ver, ssl->minor_ver, 02166 ssl->conf->transport, p ); 02167 MBEDTLS_SSL_DEBUG_BUF( 3, "server version", p, 2 ); 02168 p += 2; 02169 02170 /* If we get here, f_cookie_check is not null */ 02171 if( ssl->conf->f_cookie_write == NULL ) 02172 { 02173 MBEDTLS_SSL_DEBUG_MSG( 1, ( "inconsistent cookie callbacks" ) ); 02174 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02175 } 02176 02177 /* Skip length byte until we know the length */ 02178 cookie_len_byte = p++; 02179 02180 if( ( ret = ssl->conf->f_cookie_write( ssl->conf->p_cookie, 02181 &p, ssl->out_buf + MBEDTLS_SSL_BUFFER_LEN, 02182 ssl->cli_id, ssl->cli_id_len ) ) != 0 ) 02183 { 02184 MBEDTLS_SSL_DEBUG_RET( 1, "f_cookie_write", ret ); 02185 return( ret ); 02186 } 02187 02188 *cookie_len_byte = (unsigned char)( p - ( cookie_len_byte + 1 ) ); 02189 02190 MBEDTLS_SSL_DEBUG_BUF( 3, "cookie sent", cookie_len_byte + 1, *cookie_len_byte ); 02191 02192 ssl->out_msglen = p - ssl->out_msg; 02193 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 02194 ssl->out_msg[0] = MBEDTLS_SSL_HS_HELLO_VERIFY_REQUEST; 02195 02196 ssl->state = MBEDTLS_SSL_SERVER_HELLO_VERIFY_REQUEST_SENT; 02197 02198 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 02199 { 02200 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 02201 return( ret ); 02202 } 02203 02204 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write hello verify request" ) ); 02205 02206 return( 0 ); 02207 } 02208 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 02209 02210 static int ssl_write_server_hello( mbedtls_ssl_context *ssl ) 02211 { 02212 #if defined(MBEDTLS_HAVE_TIME) 02213 time_t t; 02214 #endif 02215 int ret; 02216 size_t olen, ext_len = 0, n; 02217 unsigned char *buf, *p; 02218 02219 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write server hello" ) ); 02220 02221 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 02222 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 02223 ssl->handshake->verify_cookie_len != 0 ) 02224 { 02225 MBEDTLS_SSL_DEBUG_MSG( 2, ( "client hello was not authenticated" ) ); 02226 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server hello" ) ); 02227 02228 return( ssl_write_hello_verify_request( ssl ) ); 02229 } 02230 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 02231 02232 if( ssl->conf->f_rng == NULL ) 02233 { 02234 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no RNG provided") ); 02235 return( MBEDTLS_ERR_SSL_NO_RNG ); 02236 } 02237 02238 /* 02239 * 0 . 0 handshake type 02240 * 1 . 3 handshake length 02241 * 4 . 5 protocol version 02242 * 6 . 9 UNIX time() 02243 * 10 . 37 random bytes 02244 */ 02245 buf = ssl->out_msg; 02246 p = buf + 4; 02247 02248 mbedtls_ssl_write_version( ssl->major_ver, ssl->minor_ver, 02249 ssl->conf->transport, p ); 02250 p += 2; 02251 02252 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, chosen version: [%d:%d]", 02253 buf[4], buf[5] ) ); 02254 02255 #if defined(MBEDTLS_HAVE_TIME) 02256 t = time( NULL ); 02257 *p++ = (unsigned char)( t >> 24 ); 02258 *p++ = (unsigned char)( t >> 16 ); 02259 *p++ = (unsigned char)( t >> 8 ); 02260 *p++ = (unsigned char)( t ); 02261 02262 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, current time: %lu", t ) ); 02263 #else 02264 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, p, 4 ) ) != 0 ) 02265 return( ret ); 02266 02267 p += 4; 02268 #endif /* MBEDTLS_HAVE_TIME */ 02269 02270 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, p, 28 ) ) != 0 ) 02271 return( ret ); 02272 02273 p += 28; 02274 02275 memcpy( ssl->handshake->randbytes + 32, buf + 6, 32 ); 02276 02277 MBEDTLS_SSL_DEBUG_BUF( 3, "server hello, random bytes", buf + 6, 32 ); 02278 02279 /* 02280 * Resume is 0 by default, see ssl_handshake_init(). 02281 * It may be already set to 1 by ssl_parse_session_ticket_ext(). 02282 * If not, try looking up session ID in our cache. 02283 */ 02284 if( ssl->handshake->resume == 0 && 02285 #if defined(MBEDTLS_SSL_RENEGOTIATION) 02286 ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE && 02287 #endif 02288 ssl->session_negotiate->id_len != 0 && 02289 ssl->conf->f_get_cache != NULL && 02290 ssl->conf->f_get_cache( ssl->conf->p_cache, ssl->session_negotiate ) == 0 ) 02291 { 02292 MBEDTLS_SSL_DEBUG_MSG( 3, ( "session successfully restored from cache" ) ); 02293 ssl->handshake->resume = 1; 02294 } 02295 02296 if( ssl->handshake->resume == 0 ) 02297 { 02298 /* 02299 * New session, create a new session id, 02300 * unless we're about to issue a session ticket 02301 */ 02302 ssl->state++; 02303 02304 #if defined(MBEDTLS_HAVE_TIME) 02305 ssl->session_negotiate->start = time( NULL ); 02306 #endif 02307 02308 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 02309 if( ssl->handshake->new_session_ticket != 0 ) 02310 { 02311 ssl->session_negotiate->id_len = n = 0; 02312 memset( ssl->session_negotiate->id, 0, 32 ); 02313 } 02314 else 02315 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 02316 { 02317 ssl->session_negotiate->id_len = n = 32; 02318 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, ssl->session_negotiate->id, 02319 n ) ) != 0 ) 02320 return( ret ); 02321 } 02322 } 02323 else 02324 { 02325 /* 02326 * Resuming a session 02327 */ 02328 n = ssl->session_negotiate->id_len; 02329 ssl->state = MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC; 02330 02331 if( ( ret = mbedtls_ssl_derive_keys( ssl ) ) != 0 ) 02332 { 02333 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_derive_keys", ret ); 02334 return( ret ); 02335 } 02336 } 02337 02338 /* 02339 * 38 . 38 session id length 02340 * 39 . 38+n session id 02341 * 39+n . 40+n chosen ciphersuite 02342 * 41+n . 41+n chosen compression alg. 02343 * 42+n . 43+n extensions length 02344 * 44+n . 43+n+m extensions 02345 */ 02346 *p++ = (unsigned char) ssl->session_negotiate->id_len; 02347 memcpy( p, ssl->session_negotiate->id, ssl->session_negotiate->id_len ); 02348 p += ssl->session_negotiate->id_len; 02349 02350 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, session id len.: %d", n ) ); 02351 MBEDTLS_SSL_DEBUG_BUF( 3, "server hello, session id", buf + 39, n ); 02352 MBEDTLS_SSL_DEBUG_MSG( 3, ( "%s session has been resumed", 02353 ssl->handshake->resume ? "a" : "no" ) ); 02354 02355 *p++ = (unsigned char)( ssl->session_negotiate->ciphersuite >> 8 ); 02356 *p++ = (unsigned char)( ssl->session_negotiate->ciphersuite ); 02357 *p++ = (unsigned char)( ssl->session_negotiate->compression ); 02358 02359 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, chosen ciphersuite: %s", 02360 mbedtls_ssl_get_ciphersuite_name( ssl->session_negotiate->ciphersuite ) ) ); 02361 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, compress alg.: 0x%02X", 02362 ssl->session_negotiate->compression ) ); 02363 02364 /* 02365 * First write extensions, then the total length 02366 */ 02367 ssl_write_renegotiation_ext( ssl, p + 2 + ext_len, &olen ); 02368 ext_len += olen; 02369 02370 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 02371 ssl_write_max_fragment_length_ext( ssl, p + 2 + ext_len, &olen ); 02372 ext_len += olen; 02373 #endif 02374 02375 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 02376 ssl_write_truncated_hmac_ext( ssl, p + 2 + ext_len, &olen ); 02377 ext_len += olen; 02378 #endif 02379 02380 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 02381 ssl_write_encrypt_then_mac_ext( ssl, p + 2 + ext_len, &olen ); 02382 ext_len += olen; 02383 #endif 02384 02385 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 02386 ssl_write_extended_ms_ext( ssl, p + 2 + ext_len, &olen ); 02387 ext_len += olen; 02388 #endif 02389 02390 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 02391 ssl_write_session_ticket_ext( ssl, p + 2 + ext_len, &olen ); 02392 ext_len += olen; 02393 #endif 02394 02395 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 02396 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02397 ssl_write_supported_point_formats_ext( ssl, p + 2 + ext_len, &olen ); 02398 ext_len += olen; 02399 #endif 02400 02401 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02402 ssl_write_ecjpake_kkpp_ext( ssl, p + 2 + ext_len, &olen ); 02403 ext_len += olen; 02404 #endif 02405 02406 #if defined(MBEDTLS_SSL_ALPN) 02407 ssl_write_alpn_ext( ssl, p + 2 + ext_len, &olen ); 02408 ext_len += olen; 02409 #endif 02410 02411 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, total extension length: %d", ext_len ) ); 02412 02413 if( ext_len > 0 ) 02414 { 02415 *p++ = (unsigned char)( ( ext_len >> 8 ) & 0xFF ); 02416 *p++ = (unsigned char)( ( ext_len ) & 0xFF ); 02417 p += ext_len; 02418 } 02419 02420 ssl->out_msglen = p - buf; 02421 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 02422 ssl->out_msg[0] = MBEDTLS_SSL_HS_SERVER_HELLO; 02423 02424 ret = mbedtls_ssl_write_record( ssl ); 02425 02426 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server hello" ) ); 02427 02428 return( ret ); 02429 } 02430 02431 #if !defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) && \ 02432 !defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) && \ 02433 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) && \ 02434 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 02435 static int ssl_write_certificate_request( mbedtls_ssl_context *ssl ) 02436 { 02437 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 02438 02439 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate request" ) ); 02440 02441 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02442 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 02443 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 02444 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 02445 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 02446 { 02447 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate request" ) ); 02448 ssl->state++; 02449 return( 0 ); 02450 } 02451 02452 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02453 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02454 } 02455 #else 02456 static int ssl_write_certificate_request( mbedtls_ssl_context *ssl ) 02457 { 02458 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 02459 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 02460 size_t dn_size, total_dn_size; /* excluding length bytes */ 02461 size_t ct_len, sa_len; /* including length bytes */ 02462 unsigned char *buf, *p; 02463 const unsigned char * const end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 02464 const mbedtls_x509_crt *crt; 02465 int authmode; 02466 02467 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate request" ) ); 02468 02469 ssl->state++; 02470 02471 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 02472 if( ssl->handshake->sni_authmode != MBEDTLS_SSL_VERIFY_UNSET ) 02473 authmode = ssl->handshake->sni_authmode; 02474 else 02475 #endif 02476 authmode = ssl->conf->authmode; 02477 02478 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02479 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 02480 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 02481 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 02482 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE || 02483 authmode == MBEDTLS_SSL_VERIFY_NONE ) 02484 { 02485 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate request" ) ); 02486 return( 0 ); 02487 } 02488 02489 /* 02490 * 0 . 0 handshake type 02491 * 1 . 3 handshake length 02492 * 4 . 4 cert type count 02493 * 5 .. m-1 cert types 02494 * m .. m+1 sig alg length (TLS 1.2 only) 02495 * m+1 .. n-1 SignatureAndHashAlgorithms (TLS 1.2 only) 02496 * n .. n+1 length of all DNs 02497 * n+2 .. n+3 length of DN 1 02498 * n+4 .. ... Distinguished Name #1 02499 * ... .. ... length of DN 2, etc. 02500 */ 02501 buf = ssl->out_msg; 02502 p = buf + 4; 02503 02504 /* 02505 * Supported certificate types 02506 * 02507 * ClientCertificateType certificate_types<1..2^8-1>; 02508 * enum { (255) } ClientCertificateType; 02509 */ 02510 ct_len = 0; 02511 02512 #if defined(MBEDTLS_RSA_C) 02513 p[1 + ct_len++] = MBEDTLS_SSL_CERT_TYPE_RSA_SIGN; 02514 #endif 02515 #if defined(MBEDTLS_ECDSA_C) 02516 p[1 + ct_len++] = MBEDTLS_SSL_CERT_TYPE_ECDSA_SIGN; 02517 #endif 02518 02519 p[0] = (unsigned char) ct_len++; 02520 p += ct_len; 02521 02522 sa_len = 0; 02523 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 02524 /* 02525 * Add signature_algorithms for verify (TLS 1.2) 02526 * 02527 * SignatureAndHashAlgorithm supported_signature_algorithms<2..2^16-2>; 02528 * 02529 * struct { 02530 * HashAlgorithm hash; 02531 * SignatureAlgorithm signature; 02532 * } SignatureAndHashAlgorithm; 02533 * 02534 * enum { (255) } HashAlgorithm; 02535 * enum { (255) } SignatureAlgorithm; 02536 */ 02537 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 02538 { 02539 /* 02540 * Only use current running hash algorithm that is already required 02541 * for requested ciphersuite. 02542 */ 02543 ssl->handshake->verify_sig_alg = MBEDTLS_SSL_HASH_SHA256; 02544 02545 if( ssl->transform_negotiate->ciphersuite_info->mac == 02546 MBEDTLS_MD_SHA384 ) 02547 { 02548 ssl->handshake->verify_sig_alg = MBEDTLS_SSL_HASH_SHA384; 02549 } 02550 02551 /* 02552 * Supported signature algorithms 02553 */ 02554 #if defined(MBEDTLS_RSA_C) 02555 p[2 + sa_len++] = ssl->handshake->verify_sig_alg; 02556 p[2 + sa_len++] = MBEDTLS_SSL_SIG_RSA; 02557 #endif 02558 #if defined(MBEDTLS_ECDSA_C) 02559 p[2 + sa_len++] = ssl->handshake->verify_sig_alg; 02560 p[2 + sa_len++] = MBEDTLS_SSL_SIG_ECDSA; 02561 #endif 02562 02563 p[0] = (unsigned char)( sa_len >> 8 ); 02564 p[1] = (unsigned char)( sa_len ); 02565 sa_len += 2; 02566 p += sa_len; 02567 } 02568 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 02569 02570 /* 02571 * DistinguishedName certificate_authorities<0..2^16-1>; 02572 * opaque DistinguishedName<1..2^16-1>; 02573 */ 02574 p += 2; 02575 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 02576 if( ssl->handshake->sni_ca_chain != NULL ) 02577 crt = ssl->handshake->sni_ca_chain; 02578 else 02579 #endif 02580 crt = ssl->conf->ca_chain; 02581 02582 total_dn_size = 0; 02583 while( crt != NULL && crt->version != 0 ) 02584 { 02585 dn_size = crt->subject_raw.len; 02586 02587 if( end < p || (size_t)( end - p ) < 2 + dn_size ) 02588 { 02589 MBEDTLS_SSL_DEBUG_MSG( 1, ( "skipping CAs: buffer too short" ) ); 02590 break; 02591 } 02592 02593 *p++ = (unsigned char)( dn_size >> 8 ); 02594 *p++ = (unsigned char)( dn_size ); 02595 memcpy( p, crt->subject_raw.p, dn_size ); 02596 p += dn_size; 02597 02598 MBEDTLS_SSL_DEBUG_BUF( 3, "requested DN", p - dn_size, dn_size ); 02599 02600 total_dn_size += 2 + dn_size; 02601 crt = crt->next; 02602 } 02603 02604 ssl->out_msglen = p - buf; 02605 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 02606 ssl->out_msg[0] = MBEDTLS_SSL_HS_CERTIFICATE_REQUEST; 02607 ssl->out_msg[4 + ct_len + sa_len] = (unsigned char)( total_dn_size >> 8 ); 02608 ssl->out_msg[5 + ct_len + sa_len] = (unsigned char)( total_dn_size ); 02609 02610 ret = mbedtls_ssl_write_record( ssl ); 02611 02612 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write certificate request" ) ); 02613 02614 return( ret ); 02615 } 02616 #endif /* !MBEDTLS_KEY_EXCHANGE_RSA_ENABLED && 02617 !MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED && 02618 !MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED && 02619 !MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED */ 02620 02621 #if defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 02622 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 02623 static int ssl_get_ecdh_params_from_cert( mbedtls_ssl_context *ssl ) 02624 { 02625 int ret; 02626 02627 if( ! mbedtls_pk_can_do( mbedtls_ssl_own_key( ssl ), MBEDTLS_PK_ECKEY ) ) 02628 { 02629 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server key not ECDH capable" ) ); 02630 return( MBEDTLS_ERR_SSL_PK_TYPE_MISMATCH ); 02631 } 02632 02633 if( ( ret = mbedtls_ecdh_get_params( &ssl->handshake->ecdh_ctx, 02634 mbedtls_pk_ec( *mbedtls_ssl_own_key( ssl ) ), 02635 MBEDTLS_ECDH_OURS ) ) != 0 ) 02636 { 02637 MBEDTLS_SSL_DEBUG_RET( 1, ( "mbedtls_ecdh_get_params" ), ret ); 02638 return( ret ); 02639 } 02640 02641 return( 0 ); 02642 } 02643 #endif /* MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || 02644 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 02645 02646 static int ssl_write_server_key_exchange( mbedtls_ssl_context *ssl ) 02647 { 02648 int ret; 02649 size_t n = 0; 02650 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02651 ssl->transform_negotiate->ciphersuite_info; 02652 02653 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 02654 defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) || \ 02655 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 02656 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) || \ 02657 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 02658 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02659 unsigned char *p = ssl->out_msg + 4; 02660 unsigned char *dig_signed = p; 02661 size_t dig_signed_len = 0, len; 02662 ((void) dig_signed); 02663 ((void) dig_signed_len); 02664 ((void) len); 02665 #endif 02666 02667 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write server key exchange" ) ); 02668 02669 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) || \ 02670 defined(MBEDTLS_KEY_EXCHANGE_PSK_ENABLED) || \ 02671 defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 02672 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA || 02673 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02674 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 02675 { 02676 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write server key exchange" ) ); 02677 ssl->state++; 02678 return( 0 ); 02679 } 02680 #endif 02681 02682 #if defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 02683 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 02684 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_RSA || 02685 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA ) 02686 { 02687 ssl_get_ecdh_params_from_cert( ssl ); 02688 02689 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write server key exchange" ) ); 02690 ssl->state++; 02691 return( 0 ); 02692 } 02693 #endif 02694 02695 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02696 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 02697 { 02698 size_t jlen; 02699 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 02700 02701 ret = mbedtls_ecjpake_write_round_two( &ssl->handshake->ecjpake_ctx, 02702 p, end - p, &jlen, ssl->conf->f_rng, ssl->conf->p_rng ); 02703 if( ret != 0 ) 02704 { 02705 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_write_round_two", ret ); 02706 return( ret ); 02707 } 02708 02709 p += jlen; 02710 n += jlen; 02711 } 02712 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 02713 02714 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) || \ 02715 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 02716 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 02717 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 02718 { 02719 /* TODO: Support identity hints */ 02720 *(p++) = 0x00; 02721 *(p++) = 0x00; 02722 02723 n += 2; 02724 } 02725 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED || 02726 MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED */ 02727 02728 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 02729 defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 02730 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_RSA || 02731 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK ) 02732 { 02733 if( ssl->conf->dhm_P.p == NULL || ssl->conf->dhm_G.p == NULL ) 02734 { 02735 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no DH parameters set" ) ); 02736 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 02737 } 02738 02739 /* 02740 * Ephemeral DH parameters: 02741 * 02742 * struct { 02743 * opaque dh_p<1..2^16-1>; 02744 * opaque dh_g<1..2^16-1>; 02745 * opaque dh_Ys<1..2^16-1>; 02746 * } ServerDHParams; 02747 */ 02748 if( ( ret = mbedtls_mpi_copy( &ssl->handshake->dhm_ctx.P, &ssl->conf->dhm_P ) ) != 0 || 02749 ( ret = mbedtls_mpi_copy( &ssl->handshake->dhm_ctx.G, &ssl->conf->dhm_G ) ) != 0 ) 02750 { 02751 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_mpi_copy", ret ); 02752 return( ret ); 02753 } 02754 02755 if( ( ret = mbedtls_dhm_make_params( &ssl->handshake->dhm_ctx, 02756 (int) mbedtls_mpi_size( &ssl->handshake->dhm_ctx.P ), 02757 p, &len, ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 02758 { 02759 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_make_params", ret ); 02760 return( ret ); 02761 } 02762 02763 dig_signed = p; 02764 dig_signed_len = len; 02765 02766 p += len; 02767 n += len; 02768 02769 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: X ", &ssl->handshake->dhm_ctx.X ); 02770 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: P ", &ssl->handshake->dhm_ctx.P ); 02771 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: G ", &ssl->handshake->dhm_ctx.G ); 02772 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: GX", &ssl->handshake->dhm_ctx.GX ); 02773 } 02774 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED || 02775 MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 02776 02777 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__ECDHE_ENABLED) 02778 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_RSA || 02779 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA || 02780 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 02781 { 02782 /* 02783 * Ephemeral ECDH parameters: 02784 * 02785 * struct { 02786 * ECParameters curve_params; 02787 * ECPoint public; 02788 * } ServerECDHParams; 02789 */ 02790 const mbedtls_ecp_curve_info **curve = NULL; 02791 const mbedtls_ecp_group_id *gid; 02792 02793 /* Match our preference list against the offered curves */ 02794 for( gid = ssl->conf->curve_list; *gid != MBEDTLS_ECP_DP_NONE; gid++ ) 02795 for( curve = ssl->handshake->curves; *curve != NULL; curve++ ) 02796 if( (*curve)->grp_id == *gid ) 02797 goto curve_matching_done; 02798 02799 curve_matching_done: 02800 if( curve == NULL || *curve == NULL ) 02801 { 02802 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no matching curve for ECDHE" ) ); 02803 return( MBEDTLS_ERR_SSL_NO_CIPHER_CHOSEN ); 02804 } 02805 02806 MBEDTLS_SSL_DEBUG_MSG( 2, ( "ECDHE curve: %s", (*curve)->name ) ); 02807 02808 if( ( ret = mbedtls_ecp_group_load( &ssl->handshake->ecdh_ctx.grp, 02809 (*curve)->grp_id ) ) != 0 ) 02810 { 02811 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecp_group_load", ret ); 02812 return( ret ); 02813 } 02814 02815 if( ( ret = mbedtls_ecdh_make_params( &ssl->handshake->ecdh_ctx, &len, 02816 p, MBEDTLS_SSL_MAX_CONTENT_LEN - n, 02817 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 02818 { 02819 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_make_params", ret ); 02820 return( ret ); 02821 } 02822 02823 dig_signed = p; 02824 dig_signed_len = len; 02825 02826 p += len; 02827 n += len; 02828 02829 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Q ", &ssl->handshake->ecdh_ctx.Q ); 02830 } 02831 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__ECDHE_ENABLED */ 02832 02833 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 02834 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 02835 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 02836 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_RSA || 02837 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_RSA || 02838 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA ) 02839 { 02840 size_t signature_len = 0; 02841 unsigned int hashlen = 0; 02842 unsigned char hash[64]; 02843 mbedtls_md_type_t md_alg = MBEDTLS_MD_NONE; 02844 02845 /* 02846 * Choose hash algorithm. NONE means MD5 + SHA1 here. 02847 */ 02848 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 02849 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 02850 { 02851 md_alg = mbedtls_ssl_md_alg_from_hash( ssl->handshake->sig_alg ); 02852 02853 if( md_alg == MBEDTLS_MD_NONE ) 02854 { 02855 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02856 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02857 } 02858 } 02859 else 02860 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 02861 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 02862 defined(MBEDTLS_SSL_PROTO_TLS1_1) 02863 if( ciphersuite_info->key_exchange == 02864 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA ) 02865 { 02866 md_alg = MBEDTLS_MD_SHA1; 02867 } 02868 else 02869 #endif 02870 { 02871 md_alg = MBEDTLS_MD_NONE; 02872 } 02873 02874 /* 02875 * Compute the hash to be signed 02876 */ 02877 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 02878 defined(MBEDTLS_SSL_PROTO_TLS1_1) 02879 if( md_alg == MBEDTLS_MD_NONE ) 02880 { 02881 mbedtls_md5_context mbedtls_md5; 02882 mbedtls_sha1_context mbedtls_sha1; 02883 02884 mbedtls_md5_init( &mbedtls_md5 ); 02885 mbedtls_sha1_init( &mbedtls_sha1 ); 02886 02887 /* 02888 * digitally-signed struct { 02889 * opaque md5_hash[16]; 02890 * opaque sha_hash[20]; 02891 * }; 02892 * 02893 * md5_hash 02894 * MD5(ClientHello.random + ServerHello.random 02895 * + ServerParams); 02896 * sha_hash 02897 * SHA(ClientHello.random + ServerHello.random 02898 * + ServerParams); 02899 */ 02900 mbedtls_md5_starts( &mbedtls_md5 ); 02901 mbedtls_md5_update( &mbedtls_md5, ssl->handshake->randbytes, 64 ); 02902 mbedtls_md5_update( &mbedtls_md5, dig_signed, dig_signed_len ); 02903 mbedtls_md5_finish( &mbedtls_md5, hash ); 02904 02905 mbedtls_sha1_starts( &mbedtls_sha1 ); 02906 mbedtls_sha1_update( &mbedtls_sha1, ssl->handshake->randbytes, 64 ); 02907 mbedtls_sha1_update( &mbedtls_sha1, dig_signed, dig_signed_len ); 02908 mbedtls_sha1_finish( &mbedtls_sha1, hash + 16 ); 02909 02910 hashlen = 36; 02911 02912 mbedtls_md5_free( &mbedtls_md5 ); 02913 mbedtls_sha1_free( &mbedtls_sha1 ); 02914 } 02915 else 02916 #endif /* MBEDTLS_SSL_PROTO_SSL3 || MBEDTLS_SSL_PROTO_TLS1 || \ 02917 MBEDTLS_SSL_PROTO_TLS1_1 */ 02918 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 02919 defined(MBEDTLS_SSL_PROTO_TLS1_2) 02920 if( md_alg != MBEDTLS_MD_NONE ) 02921 { 02922 mbedtls_md_context_t ctx; 02923 const mbedtls_md_info_t *md_info = mbedtls_md_info_from_type( md_alg ); 02924 02925 mbedtls_md_init( &ctx ); 02926 02927 /* Info from md_alg will be used instead */ 02928 hashlen = 0; 02929 02930 /* 02931 * digitally-signed struct { 02932 * opaque client_random[32]; 02933 * opaque server_random[32]; 02934 * ServerDHParams params; 02935 * }; 02936 */ 02937 if( ( ret = mbedtls_md_setup( &ctx, md_info, 0 ) ) != 0 ) 02938 { 02939 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_md_setup", ret ); 02940 return( ret ); 02941 } 02942 02943 mbedtls_md_starts( &ctx ); 02944 mbedtls_md_update( &ctx, ssl->handshake->randbytes, 64 ); 02945 mbedtls_md_update( &ctx, dig_signed, dig_signed_len ); 02946 mbedtls_md_finish( &ctx, hash ); 02947 mbedtls_md_free( &ctx ); 02948 } 02949 else 02950 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 || \ 02951 MBEDTLS_SSL_PROTO_TLS1_2 */ 02952 { 02953 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02954 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02955 } 02956 02957 MBEDTLS_SSL_DEBUG_BUF( 3, "parameters hash", hash, hashlen != 0 ? hashlen : 02958 (unsigned int) ( mbedtls_md_get_size( mbedtls_md_info_from_type( md_alg ) ) ) ); 02959 02960 /* 02961 * Make the signature 02962 */ 02963 if( mbedtls_ssl_own_key( ssl ) == NULL ) 02964 { 02965 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no private key" ) ); 02966 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 02967 } 02968 02969 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 02970 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 02971 { 02972 *(p++) = ssl->handshake->sig_alg; 02973 *(p++) = mbedtls_ssl_sig_from_pk( mbedtls_ssl_own_key( ssl ) ); 02974 02975 n += 2; 02976 } 02977 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 02978 02979 if( ( ret = mbedtls_pk_sign( mbedtls_ssl_own_key( ssl ), md_alg, hash, hashlen, 02980 p + 2 , &signature_len, 02981 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 02982 { 02983 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_pk_sign", ret ); 02984 return( ret ); 02985 } 02986 02987 *(p++) = (unsigned char)( signature_len >> 8 ); 02988 *(p++) = (unsigned char)( signature_len ); 02989 n += 2; 02990 02991 MBEDTLS_SSL_DEBUG_BUF( 3, "my signature", p, signature_len ); 02992 02993 n += signature_len; 02994 } 02995 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || 02996 MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 02997 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED */ 02998 02999 ssl->out_msglen = 4 + n; 03000 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03001 ssl->out_msg[0] = MBEDTLS_SSL_HS_SERVER_KEY_EXCHANGE; 03002 03003 ssl->state++; 03004 03005 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03006 { 03007 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03008 return( ret ); 03009 } 03010 03011 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server key exchange" ) ); 03012 03013 return( 0 ); 03014 } 03015 03016 static int ssl_write_server_hello_done( mbedtls_ssl_context *ssl ) 03017 { 03018 int ret; 03019 03020 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write server hello done" ) ); 03021 03022 ssl->out_msglen = 4; 03023 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03024 ssl->out_msg[0] = MBEDTLS_SSL_HS_SERVER_HELLO_DONE; 03025 03026 ssl->state++; 03027 03028 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03029 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03030 mbedtls_ssl_send_flight_completed( ssl ); 03031 #endif 03032 03033 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03034 { 03035 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03036 return( ret ); 03037 } 03038 03039 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server hello done" ) ); 03040 03041 return( 0 ); 03042 } 03043 03044 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 03045 defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 03046 static int ssl_parse_client_dh_public( mbedtls_ssl_context *ssl, unsigned char **p, 03047 const unsigned char *end ) 03048 { 03049 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 03050 size_t n; 03051 03052 /* 03053 * Receive G^Y mod P, premaster = (G^Y)^X mod P 03054 */ 03055 if( *p + 2 > end ) 03056 { 03057 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03058 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03059 } 03060 03061 n = ( (*p)[0] << 8 ) | (*p)[1]; 03062 *p += 2; 03063 03064 if( *p + n > end ) 03065 { 03066 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03067 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03068 } 03069 03070 if( ( ret = mbedtls_dhm_read_public( &ssl->handshake->dhm_ctx, *p, n ) ) != 0 ) 03071 { 03072 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_read_public", ret ); 03073 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_RP ); 03074 } 03075 03076 *p += n; 03077 03078 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: GY", &ssl->handshake->dhm_ctx.GY ); 03079 03080 return( ret ); 03081 } 03082 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED || 03083 MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 03084 03085 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) || \ 03086 defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 03087 static int ssl_parse_encrypted_pms( mbedtls_ssl_context *ssl, 03088 const unsigned char *p, 03089 const unsigned char *end, 03090 size_t pms_offset ) 03091 { 03092 int ret; 03093 size_t len = mbedtls_pk_get_len( mbedtls_ssl_own_key( ssl ) ); 03094 unsigned char *pms = ssl->handshake->premaster + pms_offset; 03095 unsigned char ver[2]; 03096 unsigned char fake_pms[48], peer_pms[48]; 03097 unsigned char mask; 03098 size_t i, peer_pmslen; 03099 unsigned int diff; 03100 03101 if( ! mbedtls_pk_can_do( mbedtls_ssl_own_key( ssl ), MBEDTLS_PK_RSA ) ) 03102 { 03103 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no RSA private key" ) ); 03104 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 03105 } 03106 03107 /* 03108 * Decrypt the premaster using own private RSA key 03109 */ 03110 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 03111 defined(MBEDTLS_SSL_PROTO_TLS1_2) 03112 if( ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_0 ) 03113 { 03114 if( *p++ != ( ( len >> 8 ) & 0xFF ) || 03115 *p++ != ( ( len ) & 0xFF ) ) 03116 { 03117 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03118 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03119 } 03120 } 03121 #endif 03122 03123 if( p + len != end ) 03124 { 03125 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03126 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03127 } 03128 03129 mbedtls_ssl_write_version( ssl->handshake->max_major_ver, 03130 ssl->handshake->max_minor_ver, 03131 ssl->conf->transport, ver ); 03132 03133 /* 03134 * Protection against Bleichenbacher's attack: invalid PKCS#1 v1.5 padding 03135 * must not cause the connection to end immediately; instead, send a 03136 * bad_record_mac later in the handshake. 03137 * Also, avoid data-dependant branches here to protect against 03138 * timing-based variants. 03139 */ 03140 ret = ssl->conf->f_rng( ssl->conf->p_rng, fake_pms, sizeof( fake_pms ) ); 03141 if( ret != 0 ) 03142 return( ret ); 03143 03144 ret = mbedtls_pk_decrypt( mbedtls_ssl_own_key( ssl ), p, len, 03145 peer_pms, &peer_pmslen, 03146 sizeof( peer_pms ), 03147 ssl->conf->f_rng, ssl->conf->p_rng ); 03148 03149 diff = (unsigned int) ret; 03150 diff |= peer_pmslen ^ 48; 03151 diff |= peer_pms[0] ^ ver[0]; 03152 diff |= peer_pms[1] ^ ver[1]; 03153 03154 #if defined(MBEDTLS_SSL_DEBUG_ALL) 03155 if( diff != 0 ) 03156 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03157 #endif 03158 03159 if( sizeof( ssl->handshake->premaster ) < pms_offset || 03160 sizeof( ssl->handshake->premaster ) - pms_offset < 48 ) 03161 { 03162 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03163 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03164 } 03165 ssl->handshake->pmslen = 48; 03166 03167 /* mask = diff ? 0xff : 0x00 using bit operations to avoid branches */ 03168 /* MSVC has a warning about unary minus on unsigned, but this is 03169 * well-defined and precisely what we want to do here */ 03170 #if defined(_MSC_VER) 03171 #pragma warning( push ) 03172 #pragma warning( disable : 4146 ) 03173 #endif 03174 mask = - ( ( diff | - diff ) >> ( sizeof( unsigned int ) * 8 - 1 ) ); 03175 #if defined(_MSC_VER) 03176 #pragma warning( pop ) 03177 #endif 03178 03179 for( i = 0; i < ssl->handshake->pmslen; i++ ) 03180 pms[i] = ( mask & fake_pms[i] ) | ( (~mask) & peer_pms[i] ); 03181 03182 return( 0 ); 03183 } 03184 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_ENABLED || 03185 MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED */ 03186 03187 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 03188 static int ssl_parse_client_psk_identity( mbedtls_ssl_context *ssl, unsigned char **p, 03189 const unsigned char *end ) 03190 { 03191 int ret = 0; 03192 size_t n; 03193 03194 if( ssl->conf->f_psk == NULL && 03195 ( ssl->conf->psk == NULL || ssl->conf->psk_identity == NULL || 03196 ssl->conf->psk_identity_len == 0 || ssl->conf->psk_len == 0 ) ) 03197 { 03198 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no pre-shared key" ) ); 03199 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 03200 } 03201 03202 /* 03203 * Receive client pre-shared key identity name 03204 */ 03205 if( *p + 2 > end ) 03206 { 03207 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03208 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03209 } 03210 03211 n = ( (*p)[0] << 8 ) | (*p)[1]; 03212 *p += 2; 03213 03214 if( n < 1 || n > 65535 || *p + n > end ) 03215 { 03216 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03217 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03218 } 03219 03220 if( ssl->conf->f_psk != NULL ) 03221 { 03222 if( ssl->conf->f_psk( ssl->conf->p_psk, ssl, *p, n ) != 0 ) 03223 ret = MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY; 03224 } 03225 else 03226 { 03227 /* Identity is not a big secret since clients send it in the clear, 03228 * but treat it carefully anyway, just in case */ 03229 if( n != ssl->conf->psk_identity_len || 03230 mbedtls_ssl_safer_memcmp( ssl->conf->psk_identity, *p, n ) != 0 ) 03231 { 03232 ret = MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY; 03233 } 03234 } 03235 03236 if( ret == MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY ) 03237 { 03238 MBEDTLS_SSL_DEBUG_BUF( 3, "Unknown PSK identity", *p, n ); 03239 if( ( ret = mbedtls_ssl_send_alert_message( ssl, 03240 MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03241 MBEDTLS_SSL_ALERT_MSG_UNKNOWN_PSK_IDENTITY ) ) != 0 ) 03242 { 03243 return( ret ); 03244 } 03245 03246 return( MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY ); 03247 } 03248 03249 *p += n; 03250 03251 return( 0 ); 03252 } 03253 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED */ 03254 03255 static int ssl_parse_client_key_exchange( mbedtls_ssl_context *ssl ) 03256 { 03257 int ret; 03258 const mbedtls_ssl_ciphersuite_t *ciphersuite_info; 03259 unsigned char *p, *end; 03260 03261 ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 03262 03263 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse client key exchange" ) ); 03264 03265 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 03266 { 03267 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 03268 return( ret ); 03269 } 03270 03271 p = ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ); 03272 end = ssl->in_msg + ssl->in_hslen; 03273 03274 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 03275 { 03276 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03277 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03278 } 03279 03280 if( ssl->in_msg[0] != MBEDTLS_SSL_HS_CLIENT_KEY_EXCHANGE ) 03281 { 03282 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03283 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03284 } 03285 03286 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) 03287 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_RSA ) 03288 { 03289 if( ( ret = ssl_parse_client_dh_public( ssl, &p, end ) ) != 0 ) 03290 { 03291 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_dh_public" ), ret ); 03292 return( ret ); 03293 } 03294 03295 if( p != end ) 03296 { 03297 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange" ) ); 03298 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03299 } 03300 03301 if( ( ret = mbedtls_dhm_calc_secret( &ssl->handshake->dhm_ctx, 03302 ssl->handshake->premaster, 03303 MBEDTLS_PREMASTER_SIZE, 03304 &ssl->handshake->pmslen, 03305 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 03306 { 03307 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_calc_secret", ret ); 03308 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_CS ); 03309 } 03310 03311 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: K ", &ssl->handshake->dhm_ctx.K ); 03312 } 03313 else 03314 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED */ 03315 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 03316 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 03317 defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 03318 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 03319 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_RSA || 03320 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA || 03321 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_RSA || 03322 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA ) 03323 { 03324 if( ( ret = mbedtls_ecdh_read_public( &ssl->handshake->ecdh_ctx, 03325 p, end - p) ) != 0 ) 03326 { 03327 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_read_public", ret ); 03328 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_RP ); 03329 } 03330 03331 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Qp ", &ssl->handshake->ecdh_ctx.Qp ); 03332 03333 if( ( ret = mbedtls_ecdh_calc_secret( &ssl->handshake->ecdh_ctx, 03334 &ssl->handshake->pmslen, 03335 ssl->handshake->premaster, 03336 MBEDTLS_MPI_MAX_SIZE, 03337 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 03338 { 03339 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_calc_secret", ret ); 03340 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_CS ); 03341 } 03342 03343 MBEDTLS_SSL_DEBUG_MPI( 3, "ECDH: z ", &ssl->handshake->ecdh_ctx.z ); 03344 } 03345 else 03346 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 03347 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED || 03348 MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED || 03349 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 03350 #if defined(MBEDTLS_KEY_EXCHANGE_PSK_ENABLED) 03351 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK ) 03352 { 03353 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03354 { 03355 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03356 return( ret ); 03357 } 03358 03359 if( p != end ) 03360 { 03361 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange" ) ); 03362 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03363 } 03364 03365 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03366 ciphersuite_info->key_exchange ) ) != 0 ) 03367 { 03368 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03369 return( ret ); 03370 } 03371 } 03372 else 03373 #endif /* MBEDTLS_KEY_EXCHANGE_PSK_ENABLED */ 03374 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 03375 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 03376 { 03377 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03378 { 03379 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03380 return( ret ); 03381 } 03382 03383 if( ( ret = ssl_parse_encrypted_pms( ssl, p, end, 2 ) ) != 0 ) 03384 { 03385 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_encrypted_pms" ), ret ); 03386 return( ret ); 03387 } 03388 03389 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03390 ciphersuite_info->key_exchange ) ) != 0 ) 03391 { 03392 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03393 return( ret ); 03394 } 03395 } 03396 else 03397 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED */ 03398 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 03399 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK ) 03400 { 03401 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03402 { 03403 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03404 return( ret ); 03405 } 03406 if( ( ret = ssl_parse_client_dh_public( ssl, &p, end ) ) != 0 ) 03407 { 03408 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_dh_public" ), ret ); 03409 return( ret ); 03410 } 03411 03412 if( p != end ) 03413 { 03414 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange" ) ); 03415 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03416 } 03417 03418 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03419 ciphersuite_info->key_exchange ) ) != 0 ) 03420 { 03421 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03422 return( ret ); 03423 } 03424 } 03425 else 03426 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 03427 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 03428 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 03429 { 03430 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03431 { 03432 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03433 return( ret ); 03434 } 03435 03436 if( ( ret = mbedtls_ecdh_read_public( &ssl->handshake->ecdh_ctx, 03437 p, end - p ) ) != 0 ) 03438 { 03439 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_read_public", ret ); 03440 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_RP ); 03441 } 03442 03443 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Qp ", &ssl->handshake->ecdh_ctx.Qp ); 03444 03445 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03446 ciphersuite_info->key_exchange ) ) != 0 ) 03447 { 03448 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03449 return( ret ); 03450 } 03451 } 03452 else 03453 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED */ 03454 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) 03455 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA ) 03456 { 03457 if( ( ret = ssl_parse_encrypted_pms( ssl, p, end, 0 ) ) != 0 ) 03458 { 03459 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_parse_encrypted_pms_secret" ), ret ); 03460 return( ret ); 03461 } 03462 } 03463 else 03464 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_ENABLED */ 03465 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 03466 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 03467 { 03468 ret = mbedtls_ecjpake_read_round_two( &ssl->handshake->ecjpake_ctx, 03469 p, end - p ); 03470 if( ret != 0 ) 03471 { 03472 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_read_round_two", ret ); 03473 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 03474 } 03475 03476 ret = mbedtls_ecjpake_derive_secret( &ssl->handshake->ecjpake_ctx, 03477 ssl->handshake->premaster, 32, &ssl->handshake->pmslen, 03478 ssl->conf->f_rng, ssl->conf->p_rng ); 03479 if( ret != 0 ) 03480 { 03481 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_derive_secret", ret ); 03482 return( ret ); 03483 } 03484 } 03485 else 03486 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 03487 { 03488 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03489 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03490 } 03491 03492 if( ( ret = mbedtls_ssl_derive_keys( ssl ) ) != 0 ) 03493 { 03494 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_derive_keys", ret ); 03495 return( ret ); 03496 } 03497 03498 ssl->state++; 03499 03500 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse client key exchange" ) ); 03501 03502 return( 0 ); 03503 } 03504 03505 #if !defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) && \ 03506 !defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) && \ 03507 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) && \ 03508 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 03509 static int ssl_parse_certificate_verify( mbedtls_ssl_context *ssl ) 03510 { 03511 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 03512 03513 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate verify" ) ); 03514 03515 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 03516 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 03517 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 03518 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 03519 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 03520 { 03521 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate verify" ) ); 03522 ssl->state++; 03523 return( 0 ); 03524 } 03525 03526 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03527 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03528 } 03529 #else 03530 static int ssl_parse_certificate_verify( mbedtls_ssl_context *ssl ) 03531 { 03532 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 03533 size_t i, sig_len; 03534 unsigned char hash[48]; 03535 unsigned char *hash_start = hash; 03536 size_t hashlen; 03537 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 03538 mbedtls_pk_type_t pk_alg; 03539 #endif 03540 mbedtls_md_type_t md_alg; 03541 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 03542 03543 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate verify" ) ); 03544 03545 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 03546 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 03547 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 03548 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 03549 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE || 03550 ssl->session_negotiate->peer_cert == NULL ) 03551 { 03552 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate verify" ) ); 03553 ssl->state++; 03554 return( 0 ); 03555 } 03556 03557 /* Needs to be done before read_record() to exclude current message */ 03558 ssl->handshake->calc_verify( ssl, hash ); 03559 03560 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 03561 { 03562 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 03563 return( ret ); 03564 } 03565 03566 ssl->state++; 03567 03568 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE || 03569 ssl->in_msg[0] != MBEDTLS_SSL_HS_CERTIFICATE_VERIFY ) 03570 { 03571 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03572 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03573 } 03574 03575 i = mbedtls_ssl_hs_hdr_len( ssl ); 03576 03577 /* 03578 * struct { 03579 * SignatureAndHashAlgorithm algorithm; -- TLS 1.2 only 03580 * opaque signature<0..2^16-1>; 03581 * } DigitallySigned; 03582 */ 03583 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 03584 defined(MBEDTLS_SSL_PROTO_TLS1_1) 03585 if( ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_3 ) 03586 { 03587 md_alg = MBEDTLS_MD_NONE; 03588 hashlen = 36; 03589 03590 /* For ECDSA, use SHA-1, not MD-5 + SHA-1 */ 03591 if( mbedtls_pk_can_do( &ssl->session_negotiate->peer_cert->pk, 03592 MBEDTLS_PK_ECDSA ) ) 03593 { 03594 hash_start += 16; 03595 hashlen -= 16; 03596 md_alg = MBEDTLS_MD_SHA1; 03597 } 03598 } 03599 else 03600 #endif /* MBEDTLS_SSL_PROTO_SSL3 || MBEDTLS_SSL_PROTO_TLS1 || 03601 MBEDTLS_SSL_PROTO_TLS1_1 */ 03602 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 03603 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 03604 { 03605 if( i + 2 > ssl->in_hslen ) 03606 { 03607 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03608 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03609 } 03610 03611 /* 03612 * Hash 03613 */ 03614 if( ssl->in_msg[i] != ssl->handshake->verify_sig_alg ) 03615 { 03616 MBEDTLS_SSL_DEBUG_MSG( 1, ( "peer not adhering to requested sig_alg" 03617 " for verify message" ) ); 03618 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03619 } 03620 03621 md_alg = mbedtls_ssl_md_alg_from_hash( ssl->handshake->verify_sig_alg ); 03622 03623 /* Info from md_alg will be used instead */ 03624 hashlen = 0; 03625 03626 i++; 03627 03628 /* 03629 * Signature 03630 */ 03631 if( ( pk_alg = mbedtls_ssl_pk_alg_from_sig( ssl->in_msg[i] ) ) 03632 == MBEDTLS_PK_NONE ) 03633 { 03634 MBEDTLS_SSL_DEBUG_MSG( 1, ( "peer not adhering to requested sig_alg" 03635 " for verify message" ) ); 03636 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03637 } 03638 03639 /* 03640 * Check the certificate's key type matches the signature alg 03641 */ 03642 if( ! mbedtls_pk_can_do( &ssl->session_negotiate->peer_cert->pk, pk_alg ) ) 03643 { 03644 MBEDTLS_SSL_DEBUG_MSG( 1, ( "sig_alg doesn't match cert key" ) ); 03645 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03646 } 03647 03648 i++; 03649 } 03650 else 03651 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 03652 { 03653 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03654 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03655 } 03656 03657 if( i + 2 > ssl->in_hslen ) 03658 { 03659 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03660 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03661 } 03662 03663 sig_len = ( ssl->in_msg[i] << 8 ) | ssl->in_msg[i+1]; 03664 i += 2; 03665 03666 if( i + sig_len != ssl->in_hslen ) 03667 { 03668 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03669 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03670 } 03671 03672 if( ( ret = mbedtls_pk_verify( &ssl->session_negotiate->peer_cert->pk, 03673 md_alg, hash_start, hashlen, 03674 ssl->in_msg + i, sig_len ) ) != 0 ) 03675 { 03676 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_pk_verify", ret ); 03677 return( ret ); 03678 } 03679 03680 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse certificate verify" ) ); 03681 03682 return( ret ); 03683 } 03684 #endif /* !MBEDTLS_KEY_EXCHANGE_RSA_ENABLED && 03685 !MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED && 03686 !MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED */ 03687 03688 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 03689 static int ssl_write_new_session_ticket( mbedtls_ssl_context *ssl ) 03690 { 03691 int ret; 03692 size_t tlen; 03693 uint32_t lifetime; 03694 03695 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write new session ticket" ) ); 03696 03697 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03698 ssl->out_msg[0] = MBEDTLS_SSL_HS_NEW_SESSION_TICKET; 03699 03700 /* 03701 * struct { 03702 * uint32 ticket_lifetime_hint; 03703 * opaque ticket<0..2^16-1>; 03704 * } NewSessionTicket; 03705 * 03706 * 4 . 7 ticket_lifetime_hint (0 = unspecified) 03707 * 8 . 9 ticket_len (n) 03708 * 10 . 9+n ticket content 03709 */ 03710 03711 if( ( ret = ssl->conf->f_ticket_write( ssl->conf->p_ticket, 03712 ssl->session_negotiate, 03713 ssl->out_msg + 10, 03714 ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN, 03715 &tlen, &lifetime ) ) != 0 ) 03716 { 03717 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_ticket_write", ret ); 03718 tlen = 0; 03719 } 03720 03721 ssl->out_msg[4] = ( lifetime >> 24 ) & 0xFF; 03722 ssl->out_msg[5] = ( lifetime >> 16 ) & 0xFF; 03723 ssl->out_msg[6] = ( lifetime >> 8 ) & 0xFF; 03724 ssl->out_msg[7] = ( lifetime ) & 0xFF; 03725 03726 ssl->out_msg[8] = (unsigned char)( ( tlen >> 8 ) & 0xFF ); 03727 ssl->out_msg[9] = (unsigned char)( ( tlen ) & 0xFF ); 03728 03729 ssl->out_msglen = 10 + tlen; 03730 03731 /* 03732 * Morally equivalent to updating ssl->state, but NewSessionTicket and 03733 * ChangeCipherSpec share the same state. 03734 */ 03735 ssl->handshake->new_session_ticket = 0; 03736 03737 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03738 { 03739 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03740 return( ret ); 03741 } 03742 03743 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write new session ticket" ) ); 03744 03745 return( 0 ); 03746 } 03747 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 03748 03749 /* 03750 * SSL handshake -- server side -- single step 03751 */ 03752 int mbedtls_ssl_handshake_server_step( mbedtls_ssl_context *ssl ) 03753 { 03754 int ret = 0; 03755 03756 if( ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER || ssl->handshake == NULL ) 03757 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 03758 03759 MBEDTLS_SSL_DEBUG_MSG( 2, ( "server state: %d", ssl->state ) ); 03760 03761 if( ( ret = mbedtls_ssl_flush_output( ssl ) ) != 0 ) 03762 return( ret ); 03763 03764 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03765 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 03766 ssl->handshake->retransmit_state == MBEDTLS_SSL_RETRANS_SENDING ) 03767 { 03768 if( ( ret = mbedtls_ssl_resend( ssl ) ) != 0 ) 03769 return( ret ); 03770 } 03771 #endif 03772 03773 switch( ssl->state ) 03774 { 03775 case MBEDTLS_SSL_HELLO_REQUEST: 03776 ssl->state = MBEDTLS_SSL_CLIENT_HELLO; 03777 break; 03778 03779 /* 03780 * <== ClientHello 03781 */ 03782 case MBEDTLS_SSL_CLIENT_HELLO: 03783 ret = ssl_parse_client_hello( ssl ); 03784 break; 03785 03786 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03787 case MBEDTLS_SSL_SERVER_HELLO_VERIFY_REQUEST_SENT: 03788 return( MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED ); 03789 #endif 03790 03791 /* 03792 * ==> ServerHello 03793 * Certificate 03794 * ( ServerKeyExchange ) 03795 * ( CertificateRequest ) 03796 * ServerHelloDone 03797 */ 03798 case MBEDTLS_SSL_SERVER_HELLO: 03799 ret = ssl_write_server_hello( ssl ); 03800 break; 03801 03802 case MBEDTLS_SSL_SERVER_CERTIFICATE: 03803 ret = mbedtls_ssl_write_certificate( ssl ); 03804 break; 03805 03806 case MBEDTLS_SSL_SERVER_KEY_EXCHANGE: 03807 ret = ssl_write_server_key_exchange( ssl ); 03808 break; 03809 03810 case MBEDTLS_SSL_CERTIFICATE_REQUEST: 03811 ret = ssl_write_certificate_request( ssl ); 03812 break; 03813 03814 case MBEDTLS_SSL_SERVER_HELLO_DONE: 03815 ret = ssl_write_server_hello_done( ssl ); 03816 break; 03817 03818 /* 03819 * <== ( Certificate/Alert ) 03820 * ClientKeyExchange 03821 * ( CertificateVerify ) 03822 * ChangeCipherSpec 03823 * Finished 03824 */ 03825 case MBEDTLS_SSL_CLIENT_CERTIFICATE: 03826 ret = mbedtls_ssl_parse_certificate( ssl ); 03827 break; 03828 03829 case MBEDTLS_SSL_CLIENT_KEY_EXCHANGE: 03830 ret = ssl_parse_client_key_exchange( ssl ); 03831 break; 03832 03833 case MBEDTLS_SSL_CERTIFICATE_VERIFY: 03834 ret = ssl_parse_certificate_verify( ssl ); 03835 break; 03836 03837 case MBEDTLS_SSL_CLIENT_CHANGE_CIPHER_SPEC: 03838 ret = mbedtls_ssl_parse_change_cipher_spec( ssl ); 03839 break; 03840 03841 case MBEDTLS_SSL_CLIENT_FINISHED: 03842 ret = mbedtls_ssl_parse_finished( ssl ); 03843 break; 03844 03845 /* 03846 * ==> ( NewSessionTicket ) 03847 * ChangeCipherSpec 03848 * Finished 03849 */ 03850 case MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC: 03851 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 03852 if( ssl->handshake->new_session_ticket != 0 ) 03853 ret = ssl_write_new_session_ticket( ssl ); 03854 else 03855 #endif 03856 ret = mbedtls_ssl_write_change_cipher_spec( ssl ); 03857 break; 03858 03859 case MBEDTLS_SSL_SERVER_FINISHED: 03860 ret = mbedtls_ssl_write_finished( ssl ); 03861 break; 03862 03863 case MBEDTLS_SSL_FLUSH_BUFFERS: 03864 MBEDTLS_SSL_DEBUG_MSG( 2, ( "handshake: done" ) ); 03865 ssl->state = MBEDTLS_SSL_HANDSHAKE_WRAPUP; 03866 break; 03867 03868 case MBEDTLS_SSL_HANDSHAKE_WRAPUP: 03869 mbedtls_ssl_handshake_wrapup( ssl ); 03870 break; 03871 03872 default: 03873 MBEDTLS_SSL_DEBUG_MSG( 1, ( "invalid state %d", ssl->state ) ); 03874 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 03875 } 03876 03877 return( ret ); 03878 } 03879 #endif /* MBEDTLS_SSL_SRV_C */
Generated on Tue Jul 12 2022 12:52:48 by
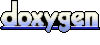