mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
pk.h
Go to the documentation of this file.
00001 /** 00002 * \file pk.h 00003 * 00004 * \brief Public Key abstraction layer 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 00024 #ifndef MBEDTLS_PK_H 00025 #define MBEDTLS_PK_H 00026 00027 #if !defined(MBEDTLS_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include MBEDTLS_CONFIG_FILE 00031 #endif 00032 00033 #include "md.h" 00034 00035 #if defined(MBEDTLS_RSA_C) 00036 #include "rsa.h" 00037 #endif 00038 00039 #if defined(MBEDTLS_ECP_C) 00040 #include "ecp.h" 00041 #endif 00042 00043 #if defined(MBEDTLS_ECDSA_C) 00044 #include "ecdsa.h" 00045 #endif 00046 00047 #if ( defined(__ARMCC_VERSION) || defined(_MSC_VER) ) && \ 00048 !defined(inline) && !defined(__cplusplus) 00049 #define inline __inline 00050 #endif 00051 00052 #define MBEDTLS_ERR_PK_ALLOC_FAILED -0x3F80 /**< Memory allocation failed. */ 00053 #define MBEDTLS_ERR_PK_TYPE_MISMATCH -0x3F00 /**< Type mismatch, eg attempt to encrypt with an ECDSA key */ 00054 #define MBEDTLS_ERR_PK_BAD_INPUT_DATA -0x3E80 /**< Bad input parameters to function. */ 00055 #define MBEDTLS_ERR_PK_FILE_IO_ERROR -0x3E00 /**< Read/write of file failed. */ 00056 #define MBEDTLS_ERR_PK_KEY_INVALID_VERSION -0x3D80 /**< Unsupported key version */ 00057 #define MBEDTLS_ERR_PK_KEY_INVALID_FORMAT -0x3D00 /**< Invalid key tag or value. */ 00058 #define MBEDTLS_ERR_PK_UNKNOWN_PK_ALG -0x3C80 /**< Key algorithm is unsupported (only RSA and EC are supported). */ 00059 #define MBEDTLS_ERR_PK_PASSWORD_REQUIRED -0x3C00 /**< Private key password can't be empty. */ 00060 #define MBEDTLS_ERR_PK_PASSWORD_MISMATCH -0x3B80 /**< Given private key password does not allow for correct decryption. */ 00061 #define MBEDTLS_ERR_PK_INVALID_PUBKEY -0x3B00 /**< The pubkey tag or value is invalid (only RSA and EC are supported). */ 00062 #define MBEDTLS_ERR_PK_INVALID_ALG -0x3A80 /**< The algorithm tag or value is invalid. */ 00063 #define MBEDTLS_ERR_PK_UNKNOWN_NAMED_CURVE -0x3A00 /**< Elliptic curve is unsupported (only NIST curves are supported). */ 00064 #define MBEDTLS_ERR_PK_FEATURE_UNAVAILABLE -0x3980 /**< Unavailable feature, e.g. RSA disabled for RSA key. */ 00065 #define MBEDTLS_ERR_PK_SIG_LEN_MISMATCH -0x3900 /**< The signature is valid but its length is less than expected. */ 00066 00067 #ifdef __cplusplus 00068 extern "C" { 00069 #endif 00070 00071 /** 00072 * \brief Public key types 00073 */ 00074 typedef enum { 00075 MBEDTLS_PK_NONE=0, 00076 MBEDTLS_PK_RSA, 00077 MBEDTLS_PK_ECKEY, 00078 MBEDTLS_PK_ECKEY_DH, 00079 MBEDTLS_PK_ECDSA, 00080 MBEDTLS_PK_RSA_ALT, 00081 MBEDTLS_PK_RSASSA_PSS, 00082 } mbedtls_pk_type_t; 00083 00084 /** 00085 * \brief Options for RSASSA-PSS signature verification. 00086 * See \c mbedtls_rsa_rsassa_pss_verify_ext() 00087 */ 00088 typedef struct 00089 { 00090 mbedtls_md_type_t mgf1_hash_id; 00091 int expected_salt_len; 00092 00093 } mbedtls_pk_rsassa_pss_options; 00094 00095 /** 00096 * \brief Types for interfacing with the debug module 00097 */ 00098 typedef enum 00099 { 00100 MBEDTLS_PK_DEBUG_NONE = 0, 00101 MBEDTLS_PK_DEBUG_MPI, 00102 MBEDTLS_PK_DEBUG_ECP, 00103 } mbedtls_pk_debug_type; 00104 00105 /** 00106 * \brief Item to send to the debug module 00107 */ 00108 typedef struct 00109 { 00110 mbedtls_pk_debug_type type; 00111 const char *name; 00112 void *value; 00113 } mbedtls_pk_debug_item; 00114 00115 /** Maximum number of item send for debugging, plus 1 */ 00116 #define MBEDTLS_PK_DEBUG_MAX_ITEMS 3 00117 00118 /** 00119 * \brief Public key information and operations 00120 */ 00121 typedef struct mbedtls_pk_info_t mbedtls_pk_info_t; 00122 00123 /** 00124 * \brief Public key container 00125 */ 00126 typedef struct 00127 { 00128 const mbedtls_pk_info_t * pk_info; /**< Public key informations */ 00129 void * pk_ctx; /**< Underlying public key context */ 00130 } mbedtls_pk_context; 00131 00132 #if defined(MBEDTLS_RSA_C) 00133 /** 00134 * Quick access to an RSA context inside a PK context. 00135 * 00136 * \warning You must make sure the PK context actually holds an RSA context 00137 * before using this function! 00138 */ 00139 static inline mbedtls_rsa_context *mbedtls_pk_rsa( const mbedtls_pk_context pk ) 00140 { 00141 return( (mbedtls_rsa_context *) (pk).pk_ctx ); 00142 } 00143 #endif /* MBEDTLS_RSA_C */ 00144 00145 #if defined(MBEDTLS_ECP_C) 00146 /** 00147 * Quick access to an EC context inside a PK context. 00148 * 00149 * \warning You must make sure the PK context actually holds an EC context 00150 * before using this function! 00151 */ 00152 static inline mbedtls_ecp_keypair *mbedtls_pk_ec( const mbedtls_pk_context pk ) 00153 { 00154 return( (mbedtls_ecp_keypair *) (pk).pk_ctx ); 00155 } 00156 #endif /* MBEDTLS_ECP_C */ 00157 00158 #if defined(MBEDTLS_PK_RSA_ALT_SUPPORT) 00159 /** 00160 * \brief Types for RSA-alt abstraction 00161 */ 00162 typedef int (*mbedtls_pk_rsa_alt_decrypt_func)( void *ctx, int mode, size_t *olen, 00163 const unsigned char *input, unsigned char *output, 00164 size_t output_max_len ); 00165 typedef int (*mbedtls_pk_rsa_alt_sign_func)( void *ctx, 00166 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng, 00167 int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, 00168 const unsigned char *hash, unsigned char *sig ); 00169 typedef size_t (*mbedtls_pk_rsa_alt_key_len_func)( void *ctx ); 00170 #endif /* MBEDTLS_PK_RSA_ALT_SUPPORT */ 00171 00172 /** 00173 * \brief Return information associated with the given PK type 00174 * 00175 * \param pk_type PK type to search for. 00176 * 00177 * \return The PK info associated with the type or NULL if not found. 00178 */ 00179 const mbedtls_pk_info_t *mbedtls_pk_info_from_type( mbedtls_pk_type_t pk_type ); 00180 00181 /** 00182 * \brief Initialize a mbedtls_pk_context (as NONE) 00183 */ 00184 void mbedtls_pk_init( mbedtls_pk_context *ctx ); 00185 00186 /** 00187 * \brief Free a mbedtls_pk_context 00188 */ 00189 void mbedtls_pk_free( mbedtls_pk_context *ctx ); 00190 00191 /** 00192 * \brief Initialize a PK context with the information given 00193 * and allocates the type-specific PK subcontext. 00194 * 00195 * \param ctx Context to initialize. Must be empty (type NONE). 00196 * \param info Information to use 00197 * 00198 * \return 0 on success, 00199 * MBEDTLS_ERR_PK_BAD_INPUT_DATA on invalid input, 00200 * MBEDTLS_ERR_PK_ALLOC_FAILED on allocation failure. 00201 * 00202 * \note For contexts holding an RSA-alt key, use 00203 * \c mbedtls_pk_setup_rsa_alt() instead. 00204 */ 00205 int mbedtls_pk_setup( mbedtls_pk_context *ctx, const mbedtls_pk_info_t *info ); 00206 00207 #if defined(MBEDTLS_PK_RSA_ALT_SUPPORT) 00208 /** 00209 * \brief Initialize an RSA-alt context 00210 * 00211 * \param ctx Context to initialize. Must be empty (type NONE). 00212 * \param key RSA key pointer 00213 * \param decrypt_func Decryption function 00214 * \param sign_func Signing function 00215 * \param key_len_func Function returning key length in bytes 00216 * 00217 * \return 0 on success, or MBEDTLS_ERR_PK_BAD_INPUT_DATA if the 00218 * context wasn't already initialized as RSA_ALT. 00219 * 00220 * \note This function replaces \c mbedtls_pk_setup() for RSA-alt. 00221 */ 00222 int mbedtls_pk_setup_rsa_alt( mbedtls_pk_context *ctx, void * key, 00223 mbedtls_pk_rsa_alt_decrypt_func decrypt_func, 00224 mbedtls_pk_rsa_alt_sign_func sign_func, 00225 mbedtls_pk_rsa_alt_key_len_func key_len_func ); 00226 #endif /* MBEDTLS_PK_RSA_ALT_SUPPORT */ 00227 00228 /** 00229 * \brief Get the size in bits of the underlying key 00230 * 00231 * \param ctx Context to use 00232 * 00233 * \return Key size in bits, or 0 on error 00234 */ 00235 size_t mbedtls_pk_get_bitlen( const mbedtls_pk_context *ctx ); 00236 00237 /** 00238 * \brief Get the length in bytes of the underlying key 00239 * \param ctx Context to use 00240 * 00241 * \return Key length in bytes, or 0 on error 00242 */ 00243 static inline size_t mbedtls_pk_get_len( const mbedtls_pk_context *ctx ) 00244 { 00245 return( ( mbedtls_pk_get_bitlen( ctx ) + 7 ) / 8 ); 00246 } 00247 00248 /** 00249 * \brief Tell if a context can do the operation given by type 00250 * 00251 * \param ctx Context to test 00252 * \param type Target type 00253 * 00254 * \return 0 if context can't do the operations, 00255 * 1 otherwise. 00256 */ 00257 int mbedtls_pk_can_do( const mbedtls_pk_context *ctx, mbedtls_pk_type_t type ); 00258 00259 /** 00260 * \brief Verify signature (including padding if relevant). 00261 * 00262 * \param ctx PK context to use 00263 * \param md_alg Hash algorithm used (see notes) 00264 * \param hash Hash of the message to sign 00265 * \param hash_len Hash length or 0 (see notes) 00266 * \param sig Signature to verify 00267 * \param sig_len Signature length 00268 * 00269 * \return 0 on success (signature is valid), 00270 * MBEDTLS_ERR_PK_SIG_LEN_MISMATCH if the signature is 00271 * valid but its actual length is less than sig_len, 00272 * or a specific error code. 00273 * 00274 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00275 * Use \c mbedtls_pk_verify_ext( MBEDTLS_PK_RSASSA_PSS, ... ) 00276 * to verify RSASSA_PSS signatures. 00277 * 00278 * \note If hash_len is 0, then the length associated with md_alg 00279 * is used instead, or an error returned if it is invalid. 00280 * 00281 * \note md_alg may be MBEDTLS_MD_NONE, only if hash_len != 0 00282 */ 00283 int mbedtls_pk_verify( mbedtls_pk_context *ctx, mbedtls_md_type_t md_alg, 00284 const unsigned char *hash, size_t hash_len, 00285 const unsigned char *sig, size_t sig_len ); 00286 00287 /** 00288 * \brief Verify signature, with options. 00289 * (Includes verification of the padding depending on type.) 00290 * 00291 * \param type Signature type (inc. possible padding type) to verify 00292 * \param options Pointer to type-specific options, or NULL 00293 * \param ctx PK context to use 00294 * \param md_alg Hash algorithm used (see notes) 00295 * \param hash Hash of the message to sign 00296 * \param hash_len Hash length or 0 (see notes) 00297 * \param sig Signature to verify 00298 * \param sig_len Signature length 00299 * 00300 * \return 0 on success (signature is valid), 00301 * MBEDTLS_ERR_PK_TYPE_MISMATCH if the PK context can't be 00302 * used for this type of signatures, 00303 * MBEDTLS_ERR_PK_SIG_LEN_MISMATCH if the signature is 00304 * valid but its actual length is less than sig_len, 00305 * or a specific error code. 00306 * 00307 * \note If hash_len is 0, then the length associated with md_alg 00308 * is used instead, or an error returned if it is invalid. 00309 * 00310 * \note md_alg may be MBEDTLS_MD_NONE, only if hash_len != 0 00311 * 00312 * \note If type is MBEDTLS_PK_RSASSA_PSS, then options must point 00313 * to a mbedtls_pk_rsassa_pss_options structure, 00314 * otherwise it must be NULL. 00315 */ 00316 int mbedtls_pk_verify_ext( mbedtls_pk_type_t type, const void *options, 00317 mbedtls_pk_context *ctx, mbedtls_md_type_t md_alg, 00318 const unsigned char *hash, size_t hash_len, 00319 const unsigned char *sig, size_t sig_len ); 00320 00321 /** 00322 * \brief Make signature, including padding if relevant. 00323 * 00324 * \param ctx PK context to use - must hold a private key 00325 * \param md_alg Hash algorithm used (see notes) 00326 * \param hash Hash of the message to sign 00327 * \param hash_len Hash length or 0 (see notes) 00328 * \param sig Place to write the signature 00329 * \param sig_len Number of bytes written 00330 * \param f_rng RNG function 00331 * \param p_rng RNG parameter 00332 * 00333 * \return 0 on success, or a specific error code. 00334 * 00335 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00336 * There is no interface in the PK module to make RSASSA-PSS 00337 * signatures yet. 00338 * 00339 * \note If hash_len is 0, then the length associated with md_alg 00340 * is used instead, or an error returned if it is invalid. 00341 * 00342 * \note For RSA, md_alg may be MBEDTLS_MD_NONE if hash_len != 0. 00343 * For ECDSA, md_alg may never be MBEDTLS_MD_NONE. 00344 */ 00345 int mbedtls_pk_sign( mbedtls_pk_context *ctx, mbedtls_md_type_t md_alg, 00346 const unsigned char *hash, size_t hash_len, 00347 unsigned char *sig, size_t *sig_len, 00348 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00349 00350 /** 00351 * \brief Decrypt message (including padding if relevant). 00352 * 00353 * \param ctx PK context to use - must hold a private key 00354 * \param input Input to decrypt 00355 * \param ilen Input size 00356 * \param output Decrypted output 00357 * \param olen Decrypted message length 00358 * \param osize Size of the output buffer 00359 * \param f_rng RNG function 00360 * \param p_rng RNG parameter 00361 * 00362 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00363 * 00364 * \return 0 on success, or a specific error code. 00365 */ 00366 int mbedtls_pk_decrypt( mbedtls_pk_context *ctx, 00367 const unsigned char *input, size_t ilen, 00368 unsigned char *output, size_t *olen, size_t osize, 00369 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00370 00371 /** 00372 * \brief Encrypt message (including padding if relevant). 00373 * 00374 * \param ctx PK context to use 00375 * \param input Message to encrypt 00376 * \param ilen Message size 00377 * \param output Encrypted output 00378 * \param olen Encrypted output length 00379 * \param osize Size of the output buffer 00380 * \param f_rng RNG function 00381 * \param p_rng RNG parameter 00382 * 00383 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00384 * 00385 * \return 0 on success, or a specific error code. 00386 */ 00387 int mbedtls_pk_encrypt( mbedtls_pk_context *ctx, 00388 const unsigned char *input, size_t ilen, 00389 unsigned char *output, size_t *olen, size_t osize, 00390 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00391 00392 /** 00393 * \brief Check if a public-private pair of keys matches. 00394 * 00395 * \param pub Context holding a public key. 00396 * \param prv Context holding a private (and public) key. 00397 * 00398 * \return 0 on success or MBEDTLS_ERR_PK_BAD_INPUT_DATA 00399 */ 00400 int mbedtls_pk_check_pair( const mbedtls_pk_context *pub, const mbedtls_pk_context *prv ); 00401 00402 /** 00403 * \brief Export debug information 00404 * 00405 * \param ctx Context to use 00406 * \param items Place to write debug items 00407 * 00408 * \return 0 on success or MBEDTLS_ERR_PK_BAD_INPUT_DATA 00409 */ 00410 int mbedtls_pk_debug( const mbedtls_pk_context *ctx, mbedtls_pk_debug_item *items ); 00411 00412 /** 00413 * \brief Access the type name 00414 * 00415 * \param ctx Context to use 00416 * 00417 * \return Type name on success, or "invalid PK" 00418 */ 00419 const char * mbedtls_pk_get_name( const mbedtls_pk_context *ctx ); 00420 00421 /** 00422 * \brief Get the key type 00423 * 00424 * \param ctx Context to use 00425 * 00426 * \return Type on success, or MBEDTLS_PK_NONE 00427 */ 00428 mbedtls_pk_type_t mbedtls_pk_get_type( const mbedtls_pk_context *ctx ); 00429 00430 #if defined(MBEDTLS_PK_PARSE_C) 00431 /** \ingroup pk_module */ 00432 /** 00433 * \brief Parse a private key in PEM or DER format 00434 * 00435 * \param ctx key to be initialized 00436 * \param key input buffer 00437 * \param keylen size of the buffer 00438 * (including the terminating null byte for PEM data) 00439 * \param pwd password for decryption (optional) 00440 * \param pwdlen size of the password 00441 * 00442 * \note On entry, ctx must be empty, either freshly initialised 00443 * with mbedtls_pk_init() or reset with mbedtls_pk_free(). If you need a 00444 * specific key type, check the result with mbedtls_pk_can_do(). 00445 * 00446 * \note The key is also checked for correctness. 00447 * 00448 * \return 0 if successful, or a specific PK or PEM error code 00449 */ 00450 int mbedtls_pk_parse_key( mbedtls_pk_context *ctx, 00451 const unsigned char *key, size_t keylen, 00452 const unsigned char *pwd, size_t pwdlen ); 00453 00454 /** \ingroup pk_module */ 00455 /** 00456 * \brief Parse a public key in PEM or DER format 00457 * 00458 * \param ctx key to be initialized 00459 * \param key input buffer 00460 * \param keylen size of the buffer 00461 * (including the terminating null byte for PEM data) 00462 * 00463 * \note On entry, ctx must be empty, either freshly initialised 00464 * with mbedtls_pk_init() or reset with mbedtls_pk_free(). If you need a 00465 * specific key type, check the result with mbedtls_pk_can_do(). 00466 * 00467 * \note The key is also checked for correctness. 00468 * 00469 * \return 0 if successful, or a specific PK or PEM error code 00470 */ 00471 int mbedtls_pk_parse_public_key( mbedtls_pk_context *ctx, 00472 const unsigned char *key, size_t keylen ); 00473 00474 #if defined(MBEDTLS_FS_IO) 00475 /** \ingroup pk_module */ 00476 /** 00477 * \brief Load and parse a private key 00478 * 00479 * \param ctx key to be initialized 00480 * \param path filename to read the private key from 00481 * \param password password to decrypt the file (can be NULL) 00482 * 00483 * \note On entry, ctx must be empty, either freshly initialised 00484 * with mbedtls_pk_init() or reset with mbedtls_pk_free(). If you need a 00485 * specific key type, check the result with mbedtls_pk_can_do(). 00486 * 00487 * \note The key is also checked for correctness. 00488 * 00489 * \return 0 if successful, or a specific PK or PEM error code 00490 */ 00491 int mbedtls_pk_parse_keyfile( mbedtls_pk_context *ctx, 00492 const char *path, const char *password ); 00493 00494 /** \ingroup pk_module */ 00495 /** 00496 * \brief Load and parse a public key 00497 * 00498 * \param ctx key to be initialized 00499 * \param path filename to read the private key from 00500 * 00501 * \note On entry, ctx must be empty, either freshly initialised 00502 * with mbedtls_pk_init() or reset with mbedtls_pk_free(). If you need a 00503 * specific key type, check the result with mbedtls_pk_can_do(). 00504 * 00505 * \note The key is also checked for correctness. 00506 * 00507 * \return 0 if successful, or a specific PK or PEM error code 00508 */ 00509 int mbedtls_pk_parse_public_keyfile( mbedtls_pk_context *ctx, const char *path ); 00510 #endif /* MBEDTLS_FS_IO */ 00511 #endif /* MBEDTLS_PK_PARSE_C */ 00512 00513 #if defined(MBEDTLS_PK_WRITE_C) 00514 /** 00515 * \brief Write a private key to a PKCS#1 or SEC1 DER structure 00516 * Note: data is written at the end of the buffer! Use the 00517 * return value to determine where you should start 00518 * using the buffer 00519 * 00520 * \param ctx private to write away 00521 * \param buf buffer to write to 00522 * \param size size of the buffer 00523 * 00524 * \return length of data written if successful, or a specific 00525 * error code 00526 */ 00527 int mbedtls_pk_write_key_der( mbedtls_pk_context *ctx, unsigned char *buf, size_t size ); 00528 00529 /** 00530 * \brief Write a public key to a SubjectPublicKeyInfo DER structure 00531 * Note: data is written at the end of the buffer! Use the 00532 * return value to determine where you should start 00533 * using the buffer 00534 * 00535 * \param ctx public key to write away 00536 * \param buf buffer to write to 00537 * \param size size of the buffer 00538 * 00539 * \return length of data written if successful, or a specific 00540 * error code 00541 */ 00542 int mbedtls_pk_write_pubkey_der( mbedtls_pk_context *ctx, unsigned char *buf, size_t size ); 00543 00544 #if defined(MBEDTLS_PEM_WRITE_C) 00545 /** 00546 * \brief Write a public key to a PEM string 00547 * 00548 * \param ctx public key to write away 00549 * \param buf buffer to write to 00550 * \param size size of the buffer 00551 * 00552 * \return 0 if successful, or a specific error code 00553 */ 00554 int mbedtls_pk_write_pubkey_pem( mbedtls_pk_context *ctx, unsigned char *buf, size_t size ); 00555 00556 /** 00557 * \brief Write a private key to a PKCS#1 or SEC1 PEM string 00558 * 00559 * \param ctx private to write away 00560 * \param buf buffer to write to 00561 * \param size size of the buffer 00562 * 00563 * \return 0 if successful, or a specific error code 00564 */ 00565 int mbedtls_pk_write_key_pem( mbedtls_pk_context *ctx, unsigned char *buf, size_t size ); 00566 #endif /* MBEDTLS_PEM_WRITE_C */ 00567 #endif /* MBEDTLS_PK_WRITE_C */ 00568 00569 /* 00570 * WARNING: Low-level functions. You probably do not want to use these unless 00571 * you are certain you do ;) 00572 */ 00573 00574 #if defined(MBEDTLS_PK_PARSE_C) 00575 /** 00576 * \brief Parse a SubjectPublicKeyInfo DER structure 00577 * 00578 * \param p the position in the ASN.1 data 00579 * \param end end of the buffer 00580 * \param pk the key to fill 00581 * 00582 * \return 0 if successful, or a specific PK error code 00583 */ 00584 int mbedtls_pk_parse_subpubkey( unsigned char **p, const unsigned char *end, 00585 mbedtls_pk_context *pk ); 00586 #endif /* MBEDTLS_PK_PARSE_C */ 00587 00588 #if defined(MBEDTLS_PK_WRITE_C) 00589 /** 00590 * \brief Write a subjectPublicKey to ASN.1 data 00591 * Note: function works backwards in data buffer 00592 * 00593 * \param p reference to current position pointer 00594 * \param start start of the buffer (for bounds-checking) 00595 * \param key public key to write away 00596 * 00597 * \return the length written or a negative error code 00598 */ 00599 int mbedtls_pk_write_pubkey( unsigned char **p, unsigned char *start, 00600 const mbedtls_pk_context *key ); 00601 #endif /* MBEDTLS_PK_WRITE_C */ 00602 00603 /* 00604 * Internal module functions. You probably do not want to use these unless you 00605 * know you do. 00606 */ 00607 #if defined(MBEDTLS_FS_IO) 00608 int mbedtls_pk_load_file( const char *path, unsigned char **buf, size_t *n ); 00609 #endif 00610 00611 #ifdef __cplusplus 00612 } 00613 #endif 00614 00615 #endif /* MBEDTLS_PK_H */
Generated on Tue Jul 12 2022 12:52:45 by
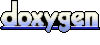