mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
bignum.h
Go to the documentation of this file.
00001 /** 00002 * \file bignum.h 00003 * 00004 * \brief Multi-precision integer library 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_BIGNUM_H 00024 #define MBEDTLS_BIGNUM_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 #include <stdint.h> 00034 00035 #if defined(MBEDTLS_FS_IO) 00036 #include <stdio.h> 00037 #endif 00038 00039 #define MBEDTLS_ERR_MPI_FILE_IO_ERROR -0x0002 /**< An error occurred while reading from or writing to a file. */ 00040 #define MBEDTLS_ERR_MPI_BAD_INPUT_DATA -0x0004 /**< Bad input parameters to function. */ 00041 #define MBEDTLS_ERR_MPI_INVALID_CHARACTER -0x0006 /**< There is an invalid character in the digit string. */ 00042 #define MBEDTLS_ERR_MPI_BUFFER_TOO_SMALL -0x0008 /**< The buffer is too small to write to. */ 00043 #define MBEDTLS_ERR_MPI_NEGATIVE_VALUE -0x000A /**< The input arguments are negative or result in illegal output. */ 00044 #define MBEDTLS_ERR_MPI_DIVISION_BY_ZERO -0x000C /**< The input argument for division is zero, which is not allowed. */ 00045 #define MBEDTLS_ERR_MPI_NOT_ACCEPTABLE -0x000E /**< The input arguments are not acceptable. */ 00046 #define MBEDTLS_ERR_MPI_ALLOC_FAILED -0x0010 /**< Memory allocation failed. */ 00047 00048 #define MBEDTLS_MPI_CHK(f) do { if( ( ret = f ) != 0 ) goto cleanup; } while( 0 ) 00049 00050 /* 00051 * Maximum size MPIs are allowed to grow to in number of limbs. 00052 */ 00053 #define MBEDTLS_MPI_MAX_LIMBS 10000 00054 00055 #if !defined(MBEDTLS_MPI_WINDOW_SIZE) 00056 /* 00057 * Maximum window size used for modular exponentiation. Default: 6 00058 * Minimum value: 1. Maximum value: 6. 00059 * 00060 * Result is an array of ( 2 << MBEDTLS_MPI_WINDOW_SIZE ) MPIs used 00061 * for the sliding window calculation. (So 64 by default) 00062 * 00063 * Reduction in size, reduces speed. 00064 */ 00065 #define MBEDTLS_MPI_WINDOW_SIZE 6 /**< Maximum windows size used. */ 00066 #endif /* !MBEDTLS_MPI_WINDOW_SIZE */ 00067 00068 #if !defined(MBEDTLS_MPI_MAX_SIZE) 00069 /* 00070 * Maximum size of MPIs allowed in bits and bytes for user-MPIs. 00071 * ( Default: 512 bytes => 4096 bits, Maximum tested: 2048 bytes => 16384 bits ) 00072 * 00073 * Note: Calculations can results temporarily in larger MPIs. So the number 00074 * of limbs required (MBEDTLS_MPI_MAX_LIMBS) is higher. 00075 */ 00076 #define MBEDTLS_MPI_MAX_SIZE 1024 /**< Maximum number of bytes for usable MPIs. */ 00077 #endif /* !MBEDTLS_MPI_MAX_SIZE */ 00078 00079 #define MBEDTLS_MPI_MAX_BITS ( 8 * MBEDTLS_MPI_MAX_SIZE ) /**< Maximum number of bits for usable MPIs. */ 00080 00081 /* 00082 * When reading from files with mbedtls_mpi_read_file() and writing to files with 00083 * mbedtls_mpi_write_file() the buffer should have space 00084 * for a (short) label, the MPI (in the provided radix), the newline 00085 * characters and the '\0'. 00086 * 00087 * By default we assume at least a 10 char label, a minimum radix of 10 00088 * (decimal) and a maximum of 4096 bit numbers (1234 decimal chars). 00089 * Autosized at compile time for at least a 10 char label, a minimum radix 00090 * of 10 (decimal) for a number of MBEDTLS_MPI_MAX_BITS size. 00091 * 00092 * This used to be statically sized to 1250 for a maximum of 4096 bit 00093 * numbers (1234 decimal chars). 00094 * 00095 * Calculate using the formula: 00096 * MBEDTLS_MPI_RW_BUFFER_SIZE = ceil(MBEDTLS_MPI_MAX_BITS / ln(10) * ln(2)) + 00097 * LabelSize + 6 00098 */ 00099 #define MBEDTLS_MPI_MAX_BITS_SCALE100 ( 100 * MBEDTLS_MPI_MAX_BITS ) 00100 #define MBEDTLS_LN_2_DIV_LN_10_SCALE100 332 00101 #define MBEDTLS_MPI_RW_BUFFER_SIZE ( ((MBEDTLS_MPI_MAX_BITS_SCALE100 + MBEDTLS_LN_2_DIV_LN_10_SCALE100 - 1) / MBEDTLS_LN_2_DIV_LN_10_SCALE100) + 10 + 6 ) 00102 00103 /* 00104 * Define the base integer type, architecture-wise. 00105 * 00106 * 32-bit integers can be forced on 64-bit arches (eg. for testing purposes) 00107 * by defining MBEDTLS_HAVE_INT32 and undefining MBEDTLS_HAVE_ASM 00108 */ 00109 #if ( ! defined(MBEDTLS_HAVE_INT32) && \ 00110 defined(_MSC_VER) && defined(_M_AMD64) ) 00111 #define MBEDTLS_HAVE_INT64 00112 typedef int64_t mbedtls_mpi_sint; 00113 typedef uint64_t mbedtls_mpi_uint; 00114 #else 00115 #if ( ! defined(MBEDTLS_HAVE_INT32) && \ 00116 defined(__GNUC__) && ( \ 00117 defined(__amd64__) || defined(__x86_64__) || \ 00118 defined(__ppc64__) || defined(__powerpc64__) || \ 00119 defined(__ia64__) || defined(__alpha__) || \ 00120 (defined(__sparc__) && defined(__arch64__)) || \ 00121 defined(__s390x__) || defined(__mips64) ) ) 00122 #define MBEDTLS_HAVE_INT64 00123 typedef int64_t mbedtls_mpi_sint; 00124 typedef uint64_t mbedtls_mpi_uint; 00125 typedef unsigned int mbedtls_t_udbl __attribute__((mode(TI))); 00126 #define MBEDTLS_HAVE_UDBL 00127 #else 00128 #define MBEDTLS_HAVE_INT32 00129 typedef int32_t mbedtls_mpi_sint; 00130 typedef uint32_t mbedtls_mpi_uint; 00131 typedef uint64_t mbedtls_t_udbl; 00132 #define MBEDTLS_HAVE_UDBL 00133 #endif /* !MBEDTLS_HAVE_INT32 && __GNUC__ && 64-bit platform */ 00134 #endif /* !MBEDTLS_HAVE_INT32 && _MSC_VER && _M_AMD64 */ 00135 00136 #ifdef __cplusplus 00137 extern "C" { 00138 #endif 00139 00140 /** 00141 * \brief MPI structure 00142 */ 00143 typedef struct 00144 { 00145 int s ; /*!< integer sign */ 00146 size_t n ; /*!< total # of limbs */ 00147 mbedtls_mpi_uint *p ; /*!< pointer to limbs */ 00148 } 00149 mbedtls_mpi; 00150 00151 /** 00152 * \brief Initialize one MPI (make internal references valid) 00153 * This just makes it ready to be set or freed, 00154 * but does not define a value for the MPI. 00155 * 00156 * \param X One MPI to initialize. 00157 */ 00158 void mbedtls_mpi_init( mbedtls_mpi *X ); 00159 00160 /** 00161 * \brief Unallocate one MPI 00162 * 00163 * \param X One MPI to unallocate. 00164 */ 00165 void mbedtls_mpi_free( mbedtls_mpi *X ); 00166 00167 /** 00168 * \brief Enlarge to the specified number of limbs 00169 * 00170 * \param X MPI to grow 00171 * \param nblimbs The target number of limbs 00172 * 00173 * \return 0 if successful, 00174 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00175 */ 00176 int mbedtls_mpi_grow( mbedtls_mpi *X, size_t nblimbs ); 00177 00178 /** 00179 * \brief Resize down, keeping at least the specified number of limbs 00180 * 00181 * \param X MPI to shrink 00182 * \param nblimbs The minimum number of limbs to keep 00183 * 00184 * \return 0 if successful, 00185 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00186 */ 00187 int mbedtls_mpi_shrink( mbedtls_mpi *X, size_t nblimbs ); 00188 00189 /** 00190 * \brief Copy the contents of Y into X 00191 * 00192 * \param X Destination MPI 00193 * \param Y Source MPI 00194 * 00195 * \return 0 if successful, 00196 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00197 */ 00198 int mbedtls_mpi_copy( mbedtls_mpi *X, const mbedtls_mpi *Y ); 00199 00200 /** 00201 * \brief Swap the contents of X and Y 00202 * 00203 * \param X First MPI value 00204 * \param Y Second MPI value 00205 */ 00206 void mbedtls_mpi_swap( mbedtls_mpi *X, mbedtls_mpi *Y ); 00207 00208 /** 00209 * \brief Safe conditional assignement X = Y if assign is 1 00210 * 00211 * \param X MPI to conditionally assign to 00212 * \param Y Value to be assigned 00213 * \param assign 1: perform the assignment, 0: keep X's original value 00214 * 00215 * \return 0 if successful, 00216 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00217 * 00218 * \note This function is equivalent to 00219 * if( assign ) mbedtls_mpi_copy( X, Y ); 00220 * except that it avoids leaking any information about whether 00221 * the assignment was done or not (the above code may leak 00222 * information through branch prediction and/or memory access 00223 * patterns analysis). 00224 */ 00225 int mbedtls_mpi_safe_cond_assign( mbedtls_mpi *X, const mbedtls_mpi *Y, unsigned char assign ); 00226 00227 /** 00228 * \brief Safe conditional swap X <-> Y if swap is 1 00229 * 00230 * \param X First mbedtls_mpi value 00231 * \param Y Second mbedtls_mpi value 00232 * \param assign 1: perform the swap, 0: keep X and Y's original values 00233 * 00234 * \return 0 if successful, 00235 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00236 * 00237 * \note This function is equivalent to 00238 * if( assign ) mbedtls_mpi_swap( X, Y ); 00239 * except that it avoids leaking any information about whether 00240 * the assignment was done or not (the above code may leak 00241 * information through branch prediction and/or memory access 00242 * patterns analysis). 00243 */ 00244 int mbedtls_mpi_safe_cond_swap( mbedtls_mpi *X, mbedtls_mpi *Y, unsigned char assign ); 00245 00246 /** 00247 * \brief Set value from integer 00248 * 00249 * \param X MPI to set 00250 * \param z Value to use 00251 * 00252 * \return 0 if successful, 00253 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00254 */ 00255 int mbedtls_mpi_lset( mbedtls_mpi *X, mbedtls_mpi_sint z ); 00256 00257 /** 00258 * \brief Get a specific bit from X 00259 * 00260 * \param X MPI to use 00261 * \param pos Zero-based index of the bit in X 00262 * 00263 * \return Either a 0 or a 1 00264 */ 00265 int mbedtls_mpi_get_bit( const mbedtls_mpi *X, size_t pos ); 00266 00267 /** 00268 * \brief Set a bit of X to a specific value of 0 or 1 00269 * 00270 * \note Will grow X if necessary to set a bit to 1 in a not yet 00271 * existing limb. Will not grow if bit should be set to 0 00272 * 00273 * \param X MPI to use 00274 * \param pos Zero-based index of the bit in X 00275 * \param val The value to set the bit to (0 or 1) 00276 * 00277 * \return 0 if successful, 00278 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00279 * MBEDTLS_ERR_MPI_BAD_INPUT_DATA if val is not 0 or 1 00280 */ 00281 int mbedtls_mpi_set_bit( mbedtls_mpi *X, size_t pos, unsigned char val ); 00282 00283 /** 00284 * \brief Return the number of zero-bits before the least significant 00285 * '1' bit 00286 * 00287 * Note: Thus also the zero-based index of the least significant '1' bit 00288 * 00289 * \param X MPI to use 00290 */ 00291 size_t mbedtls_mpi_lsb( const mbedtls_mpi *X ); 00292 00293 /** 00294 * \brief Return the number of bits up to and including the most 00295 * significant '1' bit' 00296 * 00297 * Note: Thus also the one-based index of the most significant '1' bit 00298 * 00299 * \param X MPI to use 00300 */ 00301 size_t mbedtls_mpi_bitlen( const mbedtls_mpi *X ); 00302 00303 /** 00304 * \brief Return the total size in bytes 00305 * 00306 * \param X MPI to use 00307 */ 00308 size_t mbedtls_mpi_size( const mbedtls_mpi *X ); 00309 00310 /** 00311 * \brief Import from an ASCII string 00312 * 00313 * \param X Destination MPI 00314 * \param radix Input numeric base 00315 * \param s Null-terminated string buffer 00316 * 00317 * \return 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code 00318 */ 00319 int mbedtls_mpi_read_string( mbedtls_mpi *X, int radix, const char *s ); 00320 00321 /** 00322 * \brief Export into an ASCII string 00323 * 00324 * \param X Source MPI 00325 * \param radix Output numeric base 00326 * \param buf Buffer to write the string to 00327 * \param buflen Length of buf 00328 * \param olen Length of the string written, including final NUL byte 00329 * 00330 * \return 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code. 00331 * *olen is always updated to reflect the amount 00332 * of data that has (or would have) been written. 00333 * 00334 * \note Call this function with buflen = 0 to obtain the 00335 * minimum required buffer size in *olen. 00336 */ 00337 int mbedtls_mpi_write_string( const mbedtls_mpi *X, int radix, 00338 char *buf, size_t buflen, size_t *olen ); 00339 00340 #if defined(MBEDTLS_FS_IO) 00341 /** 00342 * \brief Read X from an opened file 00343 * 00344 * \param X Destination MPI 00345 * \param radix Input numeric base 00346 * \param fin Input file handle 00347 * 00348 * \return 0 if successful, MBEDTLS_ERR_MPI_BUFFER_TOO_SMALL if 00349 * the file read buffer is too small or a 00350 * MBEDTLS_ERR_MPI_XXX error code 00351 */ 00352 int mbedtls_mpi_read_file( mbedtls_mpi *X, int radix, FILE *fin ); 00353 00354 /** 00355 * \brief Write X into an opened file, or stdout if fout is NULL 00356 * 00357 * \param p Prefix, can be NULL 00358 * \param X Source MPI 00359 * \param radix Output numeric base 00360 * \param fout Output file handle (can be NULL) 00361 * 00362 * \return 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code 00363 * 00364 * \note Set fout == NULL to print X on the console. 00365 */ 00366 int mbedtls_mpi_write_file( const char *p, const mbedtls_mpi *X, int radix, FILE *fout ); 00367 #endif /* MBEDTLS_FS_IO */ 00368 00369 /** 00370 * \brief Import X from unsigned binary data, big endian 00371 * 00372 * \param X Destination MPI 00373 * \param buf Input buffer 00374 * \param buflen Input buffer size 00375 * 00376 * \return 0 if successful, 00377 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00378 */ 00379 int mbedtls_mpi_read_binary( mbedtls_mpi *X, const unsigned char *buf, size_t buflen ); 00380 00381 /** 00382 * \brief Export X into unsigned binary data, big endian. 00383 * Always fills the whole buffer, which will start with zeros 00384 * if the number is smaller. 00385 * 00386 * \param X Source MPI 00387 * \param buf Output buffer 00388 * \param buflen Output buffer size 00389 * 00390 * \return 0 if successful, 00391 * MBEDTLS_ERR_MPI_BUFFER_TOO_SMALL if buf isn't large enough 00392 */ 00393 int mbedtls_mpi_write_binary( const mbedtls_mpi *X, unsigned char *buf, size_t buflen ); 00394 00395 /** 00396 * \brief Left-shift: X <<= count 00397 * 00398 * \param X MPI to shift 00399 * \param count Amount to shift 00400 * 00401 * \return 0 if successful, 00402 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00403 */ 00404 int mbedtls_mpi_shift_l( mbedtls_mpi *X, size_t count ); 00405 00406 /** 00407 * \brief Right-shift: X >>= count 00408 * 00409 * \param X MPI to shift 00410 * \param count Amount to shift 00411 * 00412 * \return 0 if successful, 00413 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00414 */ 00415 int mbedtls_mpi_shift_r( mbedtls_mpi *X, size_t count ); 00416 00417 /** 00418 * \brief Compare unsigned values 00419 * 00420 * \param X Left-hand MPI 00421 * \param Y Right-hand MPI 00422 * 00423 * \return 1 if |X| is greater than |Y|, 00424 * -1 if |X| is lesser than |Y| or 00425 * 0 if |X| is equal to |Y| 00426 */ 00427 int mbedtls_mpi_cmp_abs( const mbedtls_mpi *X, const mbedtls_mpi *Y ); 00428 00429 /** 00430 * \brief Compare signed values 00431 * 00432 * \param X Left-hand MPI 00433 * \param Y Right-hand MPI 00434 * 00435 * \return 1 if X is greater than Y, 00436 * -1 if X is lesser than Y or 00437 * 0 if X is equal to Y 00438 */ 00439 int mbedtls_mpi_cmp_mpi( const mbedtls_mpi *X, const mbedtls_mpi *Y ); 00440 00441 /** 00442 * \brief Compare signed values 00443 * 00444 * \param X Left-hand MPI 00445 * \param z The integer value to compare to 00446 * 00447 * \return 1 if X is greater than z, 00448 * -1 if X is lesser than z or 00449 * 0 if X is equal to z 00450 */ 00451 int mbedtls_mpi_cmp_int( const mbedtls_mpi *X, mbedtls_mpi_sint z ); 00452 00453 /** 00454 * \brief Unsigned addition: X = |A| + |B| 00455 * 00456 * \param X Destination MPI 00457 * \param A Left-hand MPI 00458 * \param B Right-hand MPI 00459 * 00460 * \return 0 if successful, 00461 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00462 */ 00463 int mbedtls_mpi_add_abs( mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00464 00465 /** 00466 * \brief Unsigned subtraction: X = |A| - |B| 00467 * 00468 * \param X Destination MPI 00469 * \param A Left-hand MPI 00470 * \param B Right-hand MPI 00471 * 00472 * \return 0 if successful, 00473 * MBEDTLS_ERR_MPI_NEGATIVE_VALUE if B is greater than A 00474 */ 00475 int mbedtls_mpi_sub_abs( mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00476 00477 /** 00478 * \brief Signed addition: X = A + B 00479 * 00480 * \param X Destination MPI 00481 * \param A Left-hand MPI 00482 * \param B Right-hand MPI 00483 * 00484 * \return 0 if successful, 00485 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00486 */ 00487 int mbedtls_mpi_add_mpi( mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00488 00489 /** 00490 * \brief Signed subtraction: X = A - B 00491 * 00492 * \param X Destination MPI 00493 * \param A Left-hand MPI 00494 * \param B Right-hand MPI 00495 * 00496 * \return 0 if successful, 00497 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00498 */ 00499 int mbedtls_mpi_sub_mpi( mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00500 00501 /** 00502 * \brief Signed addition: X = A + b 00503 * 00504 * \param X Destination MPI 00505 * \param A Left-hand MPI 00506 * \param b The integer value to add 00507 * 00508 * \return 0 if successful, 00509 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00510 */ 00511 int mbedtls_mpi_add_int( mbedtls_mpi *X, const mbedtls_mpi *A, mbedtls_mpi_sint b ); 00512 00513 /** 00514 * \brief Signed subtraction: X = A - b 00515 * 00516 * \param X Destination MPI 00517 * \param A Left-hand MPI 00518 * \param b The integer value to subtract 00519 * 00520 * \return 0 if successful, 00521 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00522 */ 00523 int mbedtls_mpi_sub_int( mbedtls_mpi *X, const mbedtls_mpi *A, mbedtls_mpi_sint b ); 00524 00525 /** 00526 * \brief Baseline multiplication: X = A * B 00527 * 00528 * \param X Destination MPI 00529 * \param A Left-hand MPI 00530 * \param B Right-hand MPI 00531 * 00532 * \return 0 if successful, 00533 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00534 */ 00535 int mbedtls_mpi_mul_mpi( mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00536 00537 /** 00538 * \brief Baseline multiplication: X = A * b 00539 * 00540 * \param X Destination MPI 00541 * \param A Left-hand MPI 00542 * \param b The unsigned integer value to multiply with 00543 * 00544 * \note b is unsigned 00545 * 00546 * \return 0 if successful, 00547 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00548 */ 00549 int mbedtls_mpi_mul_int( mbedtls_mpi *X, const mbedtls_mpi *A, mbedtls_mpi_uint b ); 00550 00551 /** 00552 * \brief Division by mbedtls_mpi: A = Q * B + R 00553 * 00554 * \param Q Destination MPI for the quotient 00555 * \param R Destination MPI for the rest value 00556 * \param A Left-hand MPI 00557 * \param B Right-hand MPI 00558 * 00559 * \return 0 if successful, 00560 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00561 * MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if B == 0 00562 * 00563 * \note Either Q or R can be NULL. 00564 */ 00565 int mbedtls_mpi_div_mpi( mbedtls_mpi *Q, mbedtls_mpi *R, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00566 00567 /** 00568 * \brief Division by int: A = Q * b + R 00569 * 00570 * \param Q Destination MPI for the quotient 00571 * \param R Destination MPI for the rest value 00572 * \param A Left-hand MPI 00573 * \param b Integer to divide by 00574 * 00575 * \return 0 if successful, 00576 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00577 * MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if b == 0 00578 * 00579 * \note Either Q or R can be NULL. 00580 */ 00581 int mbedtls_mpi_div_int( mbedtls_mpi *Q, mbedtls_mpi *R, const mbedtls_mpi *A, mbedtls_mpi_sint b ); 00582 00583 /** 00584 * \brief Modulo: R = A mod B 00585 * 00586 * \param R Destination MPI for the rest value 00587 * \param A Left-hand MPI 00588 * \param B Right-hand MPI 00589 * 00590 * \return 0 if successful, 00591 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00592 * MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if B == 0, 00593 * MBEDTLS_ERR_MPI_NEGATIVE_VALUE if B < 0 00594 */ 00595 int mbedtls_mpi_mod_mpi( mbedtls_mpi *R, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00596 00597 /** 00598 * \brief Modulo: r = A mod b 00599 * 00600 * \param r Destination mbedtls_mpi_uint 00601 * \param A Left-hand MPI 00602 * \param b Integer to divide by 00603 * 00604 * \return 0 if successful, 00605 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00606 * MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if b == 0, 00607 * MBEDTLS_ERR_MPI_NEGATIVE_VALUE if b < 0 00608 */ 00609 int mbedtls_mpi_mod_int( mbedtls_mpi_uint *r, const mbedtls_mpi *A, mbedtls_mpi_sint b ); 00610 00611 /** 00612 * \brief Sliding-window exponentiation: X = A^E mod N 00613 * 00614 * \param X Destination MPI 00615 * \param A Left-hand MPI 00616 * \param E Exponent MPI 00617 * \param N Modular MPI 00618 * \param _RR Speed-up MPI used for recalculations 00619 * 00620 * \return 0 if successful, 00621 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00622 * MBEDTLS_ERR_MPI_BAD_INPUT_DATA if N is negative or even or 00623 * if E is negative 00624 * 00625 * \note _RR is used to avoid re-computing R*R mod N across 00626 * multiple calls, which speeds up things a bit. It can 00627 * be set to NULL if the extra performance is unneeded. 00628 */ 00629 int mbedtls_mpi_exp_mod( mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *E, const mbedtls_mpi *N, mbedtls_mpi *_RR ); 00630 00631 /** 00632 * \brief Fill an MPI X with size bytes of random 00633 * 00634 * \param X Destination MPI 00635 * \param size Size in bytes 00636 * \param f_rng RNG function 00637 * \param p_rng RNG parameter 00638 * 00639 * \return 0 if successful, 00640 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00641 */ 00642 int mbedtls_mpi_fill_random( mbedtls_mpi *X, size_t size, 00643 int (*f_rng)(void *, unsigned char *, size_t), 00644 void *p_rng ); 00645 00646 /** 00647 * \brief Greatest common divisor: G = gcd(A, B) 00648 * 00649 * \param G Destination MPI 00650 * \param A Left-hand MPI 00651 * \param B Right-hand MPI 00652 * 00653 * \return 0 if successful, 00654 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00655 */ 00656 int mbedtls_mpi_gcd( mbedtls_mpi *G, const mbedtls_mpi *A, const mbedtls_mpi *B ); 00657 00658 /** 00659 * \brief Modular inverse: X = A^-1 mod N 00660 * 00661 * \param X Destination MPI 00662 * \param A Left-hand MPI 00663 * \param N Right-hand MPI 00664 * 00665 * \return 0 if successful, 00666 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00667 * MBEDTLS_ERR_MPI_BAD_INPUT_DATA if N is negative or nil 00668 MBEDTLS_ERR_MPI_NOT_ACCEPTABLE if A has no inverse mod N 00669 */ 00670 int mbedtls_mpi_inv_mod( mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *N ); 00671 00672 /** 00673 * \brief Miller-Rabin primality test 00674 * 00675 * \param X MPI to check 00676 * \param f_rng RNG function 00677 * \param p_rng RNG parameter 00678 * 00679 * \return 0 if successful (probably prime), 00680 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00681 * MBEDTLS_ERR_MPI_NOT_ACCEPTABLE if X is not prime 00682 */ 00683 int mbedtls_mpi_is_prime( const mbedtls_mpi *X, 00684 int (*f_rng)(void *, unsigned char *, size_t), 00685 void *p_rng ); 00686 00687 /** 00688 * \brief Prime number generation 00689 * 00690 * \param X Destination MPI 00691 * \param nbits Required size of X in bits 00692 * ( 3 <= nbits <= MBEDTLS_MPI_MAX_BITS ) 00693 * \param dh_flag If 1, then (X-1)/2 will be prime too 00694 * \param f_rng RNG function 00695 * \param p_rng RNG parameter 00696 * 00697 * \return 0 if successful (probably prime), 00698 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00699 * MBEDTLS_ERR_MPI_BAD_INPUT_DATA if nbits is < 3 00700 */ 00701 int mbedtls_mpi_gen_prime( mbedtls_mpi *X, size_t nbits, int dh_flag, 00702 int (*f_rng)(void *, unsigned char *, size_t), 00703 void *p_rng ); 00704 00705 /** 00706 * \brief Checkup routine 00707 * 00708 * \return 0 if successful, or 1 if the test failed 00709 */ 00710 int mbedtls_mpi_self_test( int verbose ); 00711 00712 #ifdef __cplusplus 00713 } 00714 #endif 00715 00716 #endif /* bignum.h */
Generated on Tue Jul 12 2022 12:52:41 by
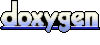