The Cayenne MQTT mbed Library provides functions to easily connect to the Cayenne IoT project builder.
Dependents: Cayenne-ESP8266Interface Cayenne-WIZnet_Library Cayenne-WIZnetInterface Cayenne-X-NUCLEO-IDW01M1 ... more
DataArray< BUFFER_SIZE > Class Template Reference
Class for manipulating a data array. More...
#include <CayenneDataArray.h>
Public Member Functions | |
DataArray () | |
Construct an empty array. | |
void | clear () |
Clear the array. | |
void | add (const char *value, bool valueInFlash=false) |
Add the specified value to the array. | |
void | add (const int value) |
Add the specified value to the array. | |
void | add (const unsigned int value) |
Add the specified value to the array. | |
void | add (const long value) |
Add the specified value to the array. | |
void | add (const unsigned long value) |
Add the specified value to the array. | |
void | add (const float value, unsigned char precision=3) |
Add the specified value to the array. | |
void | add (const double value, unsigned char precision=3) |
Add the specified value to the array. | |
void | add (const float value, unsigned char precision=3) |
Add the specified value to the array. | |
void | add (const double value, unsigned char precision=3) |
Add the specified value to the array. | |
void | add (const __FlashStringHelper *value) |
Add the specified value to the array. | |
const char * | getString () const |
Get the value string. | |
size_t | getCount () const |
Get the number of items in the value array. |
Detailed Description
template<int BUFFER_SIZE = CAYENNE_MAX_PAYLOAD_VALUE_SIZE>
class CayenneMQTT::DataArray< BUFFER_SIZE >
Class for manipulating a data array.
- Parameters:
-
BUFFER_SIZE Maximum buffer size to use for data array, in bytes.
Definition at line 35 of file CayenneDataArray.h.
Constructor & Destructor Documentation
DataArray | ( | ) |
Construct an empty array.
Definition at line 41 of file CayenneDataArray.h.
Member Function Documentation
void add | ( | const char * | value, |
bool | valueInFlash = false |
||
) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add. [in] valueInFlash If true the value string is in flash memory, otherwise false.
Definition at line 60 of file CayenneDataArray.h.
void add | ( | const unsigned int | value ) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add.
Definition at line 102 of file CayenneDataArray.h.
void add | ( | const double | value, |
unsigned char | precision = 3 |
||
) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add. [in] precision Number of digits after the decimal.
Definition at line 157 of file CayenneDataArray.h.
void add | ( | const __FlashStringHelper * | value ) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add.
Definition at line 192 of file CayenneDataArray.h.
void add | ( | const double | value, |
unsigned char | precision = 3 |
||
) |
Add the specified value to the array.
- Parameters:
-
[in] precision Number of digits after the decimal.
Definition at line 179 of file CayenneDataArray.h.
void add | ( | const float | value, |
unsigned char | precision = 3 |
||
) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add. [in] precision Number of digits after the decimal.
Definition at line 169 of file CayenneDataArray.h.
void add | ( | const long | value ) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add.
Definition at line 116 of file CayenneDataArray.h.
void add | ( | const float | value, |
unsigned char | precision = 3 |
||
) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add. [in] precision Number of digits after the decimal.
Definition at line 146 of file CayenneDataArray.h.
void add | ( | const unsigned long | value ) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add.
Definition at line 130 of file CayenneDataArray.h.
void add | ( | const int | value ) |
Add the specified value to the array.
- Parameters:
-
[in] value The value to add.
Definition at line 88 of file CayenneDataArray.h.
void clear | ( | ) |
Clear the array.
Definition at line 48 of file CayenneDataArray.h.
size_t getCount | ( | ) | const |
Get the number of items in the value array.
- Returns:
- Count of items.
Definition at line 212 of file CayenneDataArray.h.
const char* getString | ( | ) | const |
Get the value string.
- Returns:
- If there are multiple values this will return the full array string with brackets, otherwise just the single value string without brackets.
Definition at line 202 of file CayenneDataArray.h.
Generated on Wed Jul 13 2022 15:49:29 by
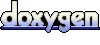