The Cayenne MQTT mbed Library provides functions to easily connect to the Cayenne IoT project builder.
Dependents: Cayenne-ESP8266Interface Cayenne-WIZnet_Library Cayenne-WIZnetInterface Cayenne-X-NUCLEO-IDW01M1 ... more
CayenneDataArray.h
00001 /* 00002 The MIT License(MIT) 00003 00004 Cayenne MQTT Client Library 00005 Copyright (c) 2016 myDevices 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated 00008 documentation files(the "Software"), to deal in the Software without restriction, including without limitation 00009 the rights to use, copy, modify, merge, publish, distribute, sublicense, and / or sell copies of the Software, 00010 and to permit persons to whom the Software is furnished to do so, subject to the following conditions : 00011 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE 00013 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.IN NO EVENT SHALL THE AUTHORS OR 00014 COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00015 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00016 */ 00017 00018 #ifndef _CAYENNEDATAARRAY_h 00019 #define _CAYENNEDATAARRAY_h 00020 00021 #include "CayenneUtils.h" 00022 00023 #if defined(__cplusplus) 00024 00025 // C++ version of the data array. This is defined here so it requires no source file. 00026 00027 namespace CayenneMQTT 00028 { 00029 /** 00030 * @class DataArray 00031 * Class for manipulating a data array. 00032 * @param BUFFER_SIZE Maximum buffer size to use for data array, in bytes. 00033 */ 00034 template<int BUFFER_SIZE = CAYENNE_MAX_PAYLOAD_VALUE_SIZE> 00035 class DataArray 00036 { 00037 public: 00038 /** 00039 * Construct an empty array. 00040 */ 00041 DataArray() { 00042 clear(); 00043 } 00044 00045 /** 00046 * Clear the array. 00047 */ 00048 void clear() { 00049 _buffer[0] = '['; // Opening bracket for array 00050 _buffer[1] = '\0'; 00051 _valueCount = 0; 00052 _index = 1; 00053 } 00054 00055 /** 00056 * Add the specified value to the array. 00057 * @param[in] value The value to add. 00058 * @param[in] valueInFlash If true the value string is in flash memory, otherwise false. 00059 */ 00060 void add(const char* value, bool valueInFlash = false) { 00061 size_t valueLength = 0; 00062 if (value) { 00063 valueLength = (valueInFlash ? CAYENNE_STRLEN(value) : strlen(value)) + 1; 00064 // Make sure the value string along with comma & array bracket will fit in buffer. 00065 if (_index + valueLength + 2 > BUFFER_SIZE) 00066 return; 00067 if (_valueCount > 0 && _index > 1) { 00068 if (_buffer[--_index - 1] == ']') 00069 --_index; 00070 _buffer[_index++] = ','; 00071 } 00072 valueInFlash ? CAYENNE_MEMCPY(_buffer + _index, value, valueLength) : memcpy(_buffer + _index, value, valueLength); 00073 _index += valueLength; 00074 if (_valueCount > 0 && _index > 1) { 00075 // Only append the closing bracket if multiple items are added. That way the getString() function can be used get 00076 // the string value without a closing bracket if this class is being used to convert single values to strings. 00077 _buffer[_index - 1] = ']'; 00078 _buffer[_index] = '\0'; 00079 } 00080 ++_valueCount; 00081 } 00082 } 00083 00084 /** 00085 * Add the specified value to the array. 00086 * @param[in] value The value to add. 00087 */ 00088 inline void add(const int value) { 00089 char str[2 + 8 * sizeof(value)]; 00090 #if defined(__AVR__) || defined (ARDUINO_ARCH_ARC32) 00091 itoa(value, str, 10); 00092 #else 00093 snprintf(str, sizeof(str), "%d", value); 00094 #endif 00095 add(str); 00096 } 00097 00098 /** 00099 * Add the specified value to the array. 00100 * @param[in] value The value to add. 00101 */ 00102 inline void add(const unsigned int value) { 00103 char str[1 + 8 * sizeof(value)]; 00104 #if defined(__AVR__) || defined (ARDUINO_ARCH_ARC32) 00105 utoa(value, str, 10); 00106 #else 00107 snprintf(str, sizeof(str), "%u", value); 00108 #endif 00109 add(str); 00110 } 00111 00112 /** 00113 * Add the specified value to the array. 00114 * @param[in] value The value to add. 00115 */ 00116 inline void add(const long value) { 00117 char str[2 + 8 * sizeof(value)]; 00118 #if defined(__AVR__) || defined (ARDUINO_ARCH_ARC32) 00119 ltoa(value, str, 10); 00120 #else 00121 snprintf(str, sizeof(str), "%ld", value); 00122 #endif 00123 add(str); 00124 } 00125 00126 /** 00127 * Add the specified value to the array. 00128 * @param[in] value The value to add. 00129 */ 00130 inline void add(const unsigned long value) { 00131 char str[1 + 8 * sizeof(value)]; 00132 #if defined(__AVR__) || defined (ARDUINO_ARCH_ARC32) 00133 ultoa(value, str, 10); 00134 #else 00135 snprintf(str, sizeof(str), "%lu", value); 00136 #endif 00137 add(str); 00138 } 00139 00140 #if defined (__AVR__) || defined (ARDUINO_ARCH_ARC32) || defined (ESP8266) 00141 /** 00142 * Add the specified value to the array. 00143 * @param[in] value The value to add. 00144 * @param[in] precision Number of digits after the decimal. 00145 */ 00146 inline void add(const float value, unsigned char precision = 3) { 00147 char str[33]; 00148 dtostrf(value, 1, precision, str); 00149 add(str); 00150 } 00151 00152 /** 00153 * Add the specified value to the array. 00154 * @param[in] value The value to add. 00155 * @param[in] precision Number of digits after the decimal. 00156 */ 00157 inline void add(const double value, unsigned char precision = 3) { 00158 char str[33]; 00159 dtostrf(value, 1, precision, str); 00160 add(str); 00161 } 00162 00163 #else 00164 /** 00165 * Add the specified value to the array. 00166 * @param[in] value The value to add. 00167 * @param[in] precision Number of digits after the decimal. 00168 */ 00169 inline void add(const float value, unsigned char precision = 3) { 00170 char str[33]; 00171 snprintf(str, 33, "%.*f", precision, value); 00172 add(str); 00173 } 00174 00175 /** 00176 * Add the specified value to the array. 00177 * @param[in] precision Number of digits after the decimal. 00178 */ 00179 inline void add(const double value, unsigned char precision = 3) { 00180 char str[33]; 00181 snprintf(str, 33, "%.*f", precision, value); 00182 add(str); 00183 } 00184 00185 #endif 00186 00187 #ifdef CAYENNE_USING_PROGMEM 00188 /** 00189 * Add the specified value to the array. 00190 * @param[in] value The value to add. 00191 */ 00192 void add(const __FlashStringHelper* value) { 00193 const char* valueString = reinterpret_cast<const char *>(value); 00194 add(valueString, true); 00195 } 00196 00197 #endif 00198 /** 00199 * Get the value string. 00200 * @return If there are multiple values this will return the full array string with brackets, otherwise just the single value string without brackets. 00201 */ 00202 const char* getString() const { 00203 if (_valueCount > 1) 00204 return _buffer; 00205 return &_buffer[1]; 00206 } 00207 00208 /** 00209 * Get the number of items in the value array. 00210 * @return Count of items. 00211 */ 00212 size_t getCount() const { 00213 return _valueCount; 00214 } 00215 00216 private: 00217 size_t _valueCount; 00218 char _buffer[BUFFER_SIZE]; 00219 size_t _index; 00220 }; 00221 } 00222 00223 typedef CayenneMQTT::DataArray<> CayenneDataArray; 00224 00225 #endif 00226 00227 #endif
Generated on Wed Jul 13 2022 15:49:28 by
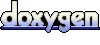