Fork of the Freetronics LCD Shield library by KKempeneers.
Dependents: Thermo_Voltmeter Freetronics_16x2_LCD DR14_DHT11_LCD Freetronics_16x2_LCD3 ... more
Fork of freetronicsLCDShield by
freetronicsLCDShield Class Reference
Provides full LCD support for the HD44780 compatible LCD on the arduino shaped shield. More...
#include <freetronicsLCDShield.h>
Public Member Functions | |
freetronicsLCDShield (PinName rs, PinName e, PinName d0, PinName d1, PinName d2, PinName d3, PinName bl, PinName a0) | |
The constructor creates an freeTronics LCD Shield object, the pins are to be provided by the user. | |
void | writeCGRAM (char address, const char *ptr, char nbytes) |
Creates custom characters. | |
void | setCursorPosition (int line, int col) |
Sets the current cursor position. | |
void | setBackLight (bool blStatus) |
Sets the backlight. | |
void | setBackLight (float blIntensity) |
Sets the backlight. | |
void | setCursor (bool cStatus=true, bool blink=false) |
Sets cursor appearance. | |
void | shiftLeft (void) |
Shifts text. | |
void | shiftRight (void) |
Shifts text. | |
void | shift (bool direction) |
Shifts text. | |
void | cls (void) |
Clears the display, the cursor returns to its home position (0,0). | |
void | home (void) |
Returns the cursor to positition (0,0). | |
float | readButton (void) |
Reads the status of the buttons. |
Detailed Description
Provides full LCD support for the HD44780 compatible LCD on the arduino shaped shield.
http://www.freetronics.com/products/lcd-keypad-shield#.UnIr6_nkq0M
Definition at line 34 of file freetronicsLCDShield.h.
Constructor & Destructor Documentation
freetronicsLCDShield | ( | PinName | rs, |
PinName | e, | ||
PinName | d0, | ||
PinName | d1, | ||
PinName | d2, | ||
PinName | d3, | ||
PinName | bl, | ||
PinName | a0 | ||
) |
The constructor creates an freeTronics LCD Shield object, the pins are to be provided by the user.
In sequence, RegisterSelect, Enable, Data0 to Data3. Bl is the backlight and a0 is to be provided for button support. Bl should be a pin with PWM capabilities and a0 should be an analogue input.
The class inherits from stream, therfore writing to the display is as easy as calling printf() to display text or putc() to display a custom character
Example:
<instanceName>.printf("Hello World");
Definition at line 32 of file freetronicsLCDShield.cpp.
Member Function Documentation
void cls | ( | void | ) |
Clears the display, the cursor returns to its home position (0,0).
The user should preserve caution when clearing the display in the main program loop, this very quickly results in flickering. A better approach is to overwrite the display.
Definition at line 92 of file freetronicsLCDShield.cpp.
void home | ( | void | ) |
Returns the cursor to positition (0,0).
The display is NOT cleared.
This function differs from setCursorPosition(0,0) in the way that home() undoes all preceding shift operations. i.e. If the display is shifted one position right, the setCursorPosition(0,0) function call would place the cursor physically at the second character of the first row while home() places it at the first character of the first row.
Definition at line 98 of file freetronicsLCDShield.cpp.
float readButton | ( | void | ) |
Reads the status of the buttons.
Definition at line 135 of file freetronicsLCDShield.cpp.
void setBackLight | ( | float | blIntensity ) |
Sets the backlight.
The backlight intensity is specified by the normalized float argument 0 .. 1
Definition at line 66 of file freetronicsLCDShield.cpp.
void setBackLight | ( | bool | blStatus ) |
Sets the backlight.
The backlight is turned on (argument true) or off (false)
Definition at line 61 of file freetronicsLCDShield.cpp.
void setCursor | ( | bool | cStatus = true , |
bool | blink = false |
||
) |
Sets cursor appearance.
The cursor is set visible (1st argument true) or invisible (false). When the second argument is set when the cStatus is set the cursor blinks.
Definition at line 71 of file freetronicsLCDShield.cpp.
void setCursorPosition | ( | int | line, |
int | col | ||
) |
Sets the current cursor position.
To place the cursor at a specific location on the display call the setCursorPosition member function, the first argument is the line either 0 or 1, the second argument is the column 0 .. 15.
Definition at line 56 of file freetronicsLCDShield.cpp.
void shift | ( | bool | direction ) |
Shifts text.
Text on the display is shifted left if direction is set (true) or right is direction is reset (false)
Definition at line 79 of file freetronicsLCDShield.cpp.
void shiftLeft | ( | void | ) |
Shifts text.
Text on the display is shifted left.
Definition at line 84 of file freetronicsLCDShield.cpp.
void shiftRight | ( | void | ) |
Shifts text.
Text on the display is shifted right.
Definition at line 88 of file freetronicsLCDShield.cpp.
void writeCGRAM | ( | char | address, |
const char * | ptr, | ||
char | nbytes | ||
) |
Creates custom characters.
Characters that aren't included in the LCD controllers character map which includes typically all ASCII characters can be generated by writing bitmaps to the character generator ram memory space. For instance the degree sign '°' is an extended ASCII character not included in the character map. It can however be generated using the writeCGRAM member function. Each line of the 5x7 dot matrix is represented by a byte in which the lower 5 bits correspond to the pixel on the display. In total 8 bytes make up one custom character (the 8th byte represents the cursor space)
Example:
CGRAM_DATA[] = {0xC0, //0b00001100 0x12, //0b00010010 0x12, //0b00010010 0xC0, //0b00001100 0x00, //0b00000000 0x00, //0b00000000 0x00, //0b00000000 0x00}; //0b00000000 <instanceName>.writeCGRAM (0x00, &CGRAM_DATA[0], 8);
The '°' can hereafter be displayed by calling:
<instanceName>.putc (0);
Definition at line 104 of file freetronicsLCDShield.cpp.
Generated on Tue Jul 12 2022 18:11:05 by
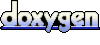