Basic implementation of Xbus message parsing and generation for embedded processors. The code has no dependencies and should also work for other MCU architectures than ARM provided a C99 compiler is available.
Dependents: MTi-1_example_LPC1768 MTi-1_rikbeun MTi-1_example MTi-1_example ... more
xbusmessage.h
00001 /*! 00002 * \file 00003 * \copyright Copyright (C) Xsens Technologies B.V., 2015. 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not 00006 * use this file except in compliance with the License. You may obtain a copy 00007 * of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the 00014 * License for the specific language governing permissions and limitations 00015 * under the License. 00016 */ 00017 00018 #ifndef __XBUSMESSAGE_H 00019 #define __XBUSMESSAGE_H 00020 00021 #include <stddef.h> 00022 #include <stdint.h> 00023 #include <stdbool.h> 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 /*! \brief Xbus message IDs. */ 00030 enum XsMessageId 00031 { 00032 XMID_Wakeup = 0x3E, 00033 XMID_WakeupAck = 0x3F, 00034 XMID_ReqDid = 0x00, 00035 XMID_DeviceId = 0x01, 00036 XMID_GotoConfig = 0x30, 00037 XMID_GotoConfigAck = 0x31, 00038 XMID_GotoMeasurement = 0x10, 00039 XMID_GotoMeasurementAck = 0x11, 00040 XMID_MtData2 = 0x36, 00041 XMID_ReqOutputConfig = 0xC0, 00042 XMID_SetOutputConfig = 0xC0, 00043 XMID_OutputConfig = 0xC1, 00044 XMID_Reset = 0x40, 00045 XMID_ResetAck = 0x41, 00046 XMID_Error = 0x42, 00047 }; 00048 00049 /*! \brief Xbus data message type IDs. */ 00050 enum XsDataIdentifier 00051 { 00052 XDI_PacketCounter = 0x1020, 00053 XDI_SampleTimeFine = 0x1060, 00054 XDI_Quaternion = 0x2010, 00055 XDI_DeltaV = 0x4010, 00056 XDI_Acceleration = 0x4020, 00057 XDI_RateOfTurn = 0x8020, 00058 XDI_DeltaQ = 0x8030, 00059 XDI_MagneticField = 0xC020, 00060 XDI_StatusWord = 0xE020, 00061 }; 00062 00063 /*! 00064 * \brief Low level format to use when formating Xbus messages for transmission. 00065 */ 00066 enum XbusLowLevelFormat 00067 { 00068 /*! \brief Format for use with I2C interface. */ 00069 XLLF_I2c, 00070 /*! \brief Format for use with SPI interface. */ 00071 XLLF_Spi, 00072 /*! \brief Format for use with UART interface. */ 00073 XLLF_Uart 00074 }; 00075 00076 /*! 00077 * \brief An Xbus message structure with optional payload. 00078 */ 00079 struct XbusMessage 00080 { 00081 /*! \brief The message ID of the message. */ 00082 enum XsMessageId mid; 00083 /*! 00084 * \brief The length of the payload. 00085 * 00086 * \note The meaning of the length is message dependent. For example, 00087 * for XMID_OutputConfig messages it is the number of OutputConfiguration 00088 * elements in the configuration array. 00089 */ 00090 uint16_t length; 00091 /*! \brief Pointer to the payload data. */ 00092 void* data; 00093 }; 00094 00095 /*! 00096 * \brief Output configuration structure. 00097 */ 00098 struct OutputConfiguration 00099 { 00100 /*! \brief Data type of the output. */ 00101 enum XsDataIdentifier dtype; 00102 /*! 00103 * \brief The output frequency in Hz, or 65535 if the value should be 00104 * included in every data message. 00105 */ 00106 uint16_t freq; 00107 }; 00108 00109 size_t XbusMessage_format(uint8_t* raw, struct XbusMessage const* message, enum XbusLowLevelFormat format); 00110 bool XbusMessage_getDataItem(void* item, enum XsDataIdentifier id, struct XbusMessage const* message); 00111 char const* XbusMessage_dataDescription(enum XsDataIdentifier id); 00112 00113 #ifdef __cplusplus 00114 } 00115 #endif // extern "C" 00116 00117 #endif // __XBUSMESSAGE_H
Generated on Thu Jul 14 2022 08:57:02 by
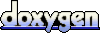